Assign return value from python method to a variable in bash script
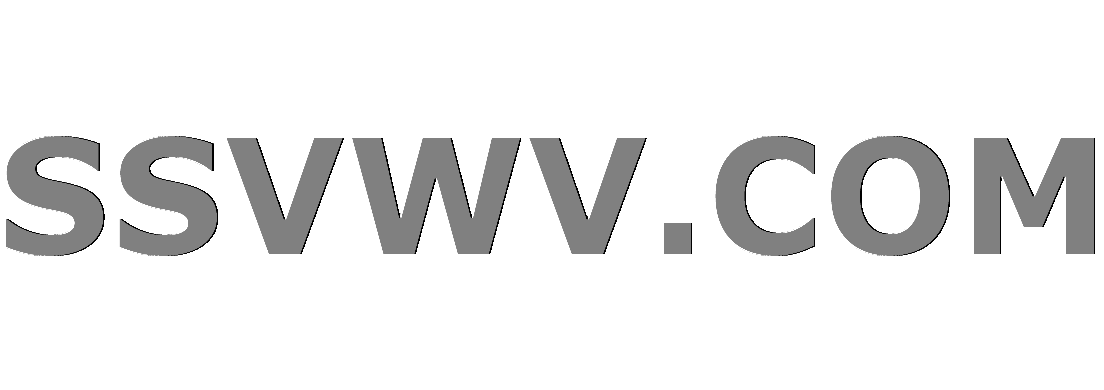
Multi tool use
up vote
0
down vote
favorite
From a shell script, I am executing python program. I am trying to assign the return value from python to a variable in shell script and comparing this variable in if else condition. I don't know where I made a mistake either in code or some syntax. Please help me getting the correct output.
test.sh
#!bin/sh
#!/usr/bin/python2.7
password="Testing@2134testing"
password_check=$(python password.py $password)
if [ "$password_check" == "True" ];then
echo "Correct"
else
echo "Incorrect"
fi
password.py
import re, sys
password = sys.argv[1]
def passwordCheck():
if re.match(r'(?=.*[A-Z])(?=.*[0-9])(?=.*[a-z])(?=.*[^A-Za-z0-9]).{14,}', password):
return True;
else:
return False;
Even the password meets the requirement, it is always showing incorrect.
python-2.7 shell
add a comment |
up vote
0
down vote
favorite
From a shell script, I am executing python program. I am trying to assign the return value from python to a variable in shell script and comparing this variable in if else condition. I don't know where I made a mistake either in code or some syntax. Please help me getting the correct output.
test.sh
#!bin/sh
#!/usr/bin/python2.7
password="Testing@2134testing"
password_check=$(python password.py $password)
if [ "$password_check" == "True" ];then
echo "Correct"
else
echo "Incorrect"
fi
password.py
import re, sys
password = sys.argv[1]
def passwordCheck():
if re.match(r'(?=.*[A-Z])(?=.*[0-9])(?=.*[a-z])(?=.*[^A-Za-z0-9]).{14,}', password):
return True;
else:
return False;
Even the password meets the requirement, it is always showing incorrect.
python-2.7 shell
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
From a shell script, I am executing python program. I am trying to assign the return value from python to a variable in shell script and comparing this variable in if else condition. I don't know where I made a mistake either in code or some syntax. Please help me getting the correct output.
test.sh
#!bin/sh
#!/usr/bin/python2.7
password="Testing@2134testing"
password_check=$(python password.py $password)
if [ "$password_check" == "True" ];then
echo "Correct"
else
echo "Incorrect"
fi
password.py
import re, sys
password = sys.argv[1]
def passwordCheck():
if re.match(r'(?=.*[A-Z])(?=.*[0-9])(?=.*[a-z])(?=.*[^A-Za-z0-9]).{14,}', password):
return True;
else:
return False;
Even the password meets the requirement, it is always showing incorrect.
python-2.7 shell
From a shell script, I am executing python program. I am trying to assign the return value from python to a variable in shell script and comparing this variable in if else condition. I don't know where I made a mistake either in code or some syntax. Please help me getting the correct output.
test.sh
#!bin/sh
#!/usr/bin/python2.7
password="Testing@2134testing"
password_check=$(python password.py $password)
if [ "$password_check" == "True" ];then
echo "Correct"
else
echo "Incorrect"
fi
password.py
import re, sys
password = sys.argv[1]
def passwordCheck():
if re.match(r'(?=.*[A-Z])(?=.*[0-9])(?=.*[a-z])(?=.*[^A-Za-z0-9]).{14,}', password):
return True;
else:
return False;
Even the password meets the requirement, it is always showing incorrect.
python-2.7 shell
python-2.7 shell
asked Nov 19 at 11:49
Technocrat
35
35
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
When you run this:
password_check=$(python password.py $password)
You are assigning the output of the password.py
program to the password_check
variable. The problem is that your password.py
program doesn't produce any output: in fact, it never even runs the passwordCheck
function, because your Python script never calls passwordCheck
. Do make things work the way you're trying to use the script, you would need to modify it to (a) actually call the passwordCheck
method and (b) print output instead of return
ing a value:
import re, sys
password = sys.argv[1]
def passwordCheck():
if re.match(r'(?=.*[A-Z])(?=.*[0-9])(?=.*[a-z])(?=.*[^A-Za-z0-9]).{14,}', password):
print 'okay'
return True
else:
print 'fail'
return False
passwordCheck()
And then check for that value in your shell script:
#!bin/sh
password="Testing@2134testing"
password_check=$(python password.py $password)
if [ "$password_check" == "okay" ];then
echo "Correct"
else
echo "Incorrect"
fi
Note that Python doesn't require a terminal ;
on lines, so instead
of:
return False;
You should write:
return False
Additionally, in this example we're using the output of the script
to determine success/failure. A slightly more robust solution is to
use the exit code of the script instead. You can probably find a
variety of examples of using this technique if you explore online a
bit.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
When you run this:
password_check=$(python password.py $password)
You are assigning the output of the password.py
program to the password_check
variable. The problem is that your password.py
program doesn't produce any output: in fact, it never even runs the passwordCheck
function, because your Python script never calls passwordCheck
. Do make things work the way you're trying to use the script, you would need to modify it to (a) actually call the passwordCheck
method and (b) print output instead of return
ing a value:
import re, sys
password = sys.argv[1]
def passwordCheck():
if re.match(r'(?=.*[A-Z])(?=.*[0-9])(?=.*[a-z])(?=.*[^A-Za-z0-9]).{14,}', password):
print 'okay'
return True
else:
print 'fail'
return False
passwordCheck()
And then check for that value in your shell script:
#!bin/sh
password="Testing@2134testing"
password_check=$(python password.py $password)
if [ "$password_check" == "okay" ];then
echo "Correct"
else
echo "Incorrect"
fi
Note that Python doesn't require a terminal ;
on lines, so instead
of:
return False;
You should write:
return False
Additionally, in this example we're using the output of the script
to determine success/failure. A slightly more robust solution is to
use the exit code of the script instead. You can probably find a
variety of examples of using this technique if you explore online a
bit.
add a comment |
up vote
0
down vote
accepted
When you run this:
password_check=$(python password.py $password)
You are assigning the output of the password.py
program to the password_check
variable. The problem is that your password.py
program doesn't produce any output: in fact, it never even runs the passwordCheck
function, because your Python script never calls passwordCheck
. Do make things work the way you're trying to use the script, you would need to modify it to (a) actually call the passwordCheck
method and (b) print output instead of return
ing a value:
import re, sys
password = sys.argv[1]
def passwordCheck():
if re.match(r'(?=.*[A-Z])(?=.*[0-9])(?=.*[a-z])(?=.*[^A-Za-z0-9]).{14,}', password):
print 'okay'
return True
else:
print 'fail'
return False
passwordCheck()
And then check for that value in your shell script:
#!bin/sh
password="Testing@2134testing"
password_check=$(python password.py $password)
if [ "$password_check" == "okay" ];then
echo "Correct"
else
echo "Incorrect"
fi
Note that Python doesn't require a terminal ;
on lines, so instead
of:
return False;
You should write:
return False
Additionally, in this example we're using the output of the script
to determine success/failure. A slightly more robust solution is to
use the exit code of the script instead. You can probably find a
variety of examples of using this technique if you explore online a
bit.
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
When you run this:
password_check=$(python password.py $password)
You are assigning the output of the password.py
program to the password_check
variable. The problem is that your password.py
program doesn't produce any output: in fact, it never even runs the passwordCheck
function, because your Python script never calls passwordCheck
. Do make things work the way you're trying to use the script, you would need to modify it to (a) actually call the passwordCheck
method and (b) print output instead of return
ing a value:
import re, sys
password = sys.argv[1]
def passwordCheck():
if re.match(r'(?=.*[A-Z])(?=.*[0-9])(?=.*[a-z])(?=.*[^A-Za-z0-9]).{14,}', password):
print 'okay'
return True
else:
print 'fail'
return False
passwordCheck()
And then check for that value in your shell script:
#!bin/sh
password="Testing@2134testing"
password_check=$(python password.py $password)
if [ "$password_check" == "okay" ];then
echo "Correct"
else
echo "Incorrect"
fi
Note that Python doesn't require a terminal ;
on lines, so instead
of:
return False;
You should write:
return False
Additionally, in this example we're using the output of the script
to determine success/failure. A slightly more robust solution is to
use the exit code of the script instead. You can probably find a
variety of examples of using this technique if you explore online a
bit.
When you run this:
password_check=$(python password.py $password)
You are assigning the output of the password.py
program to the password_check
variable. The problem is that your password.py
program doesn't produce any output: in fact, it never even runs the passwordCheck
function, because your Python script never calls passwordCheck
. Do make things work the way you're trying to use the script, you would need to modify it to (a) actually call the passwordCheck
method and (b) print output instead of return
ing a value:
import re, sys
password = sys.argv[1]
def passwordCheck():
if re.match(r'(?=.*[A-Z])(?=.*[0-9])(?=.*[a-z])(?=.*[^A-Za-z0-9]).{14,}', password):
print 'okay'
return True
else:
print 'fail'
return False
passwordCheck()
And then check for that value in your shell script:
#!bin/sh
password="Testing@2134testing"
password_check=$(python password.py $password)
if [ "$password_check" == "okay" ];then
echo "Correct"
else
echo "Incorrect"
fi
Note that Python doesn't require a terminal ;
on lines, so instead
of:
return False;
You should write:
return False
Additionally, in this example we're using the output of the script
to determine success/failure. A slightly more robust solution is to
use the exit code of the script instead. You can probably find a
variety of examples of using this technique if you explore online a
bit.
answered Nov 19 at 12:06
larsks
112k18184194
112k18184194
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53374040%2fassign-return-value-from-python-method-to-a-variable-in-bash-script%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
1x1ZNr0vCf,QSxLnDXGin3g10k01sL U7S9,fS5 6RbVcBs,EqHNrl,T60 jkwHO24TxHb8,Y2GSwxP5aDXyPgV16Ut