Python pixel manipulation returning grey image instead of inverted
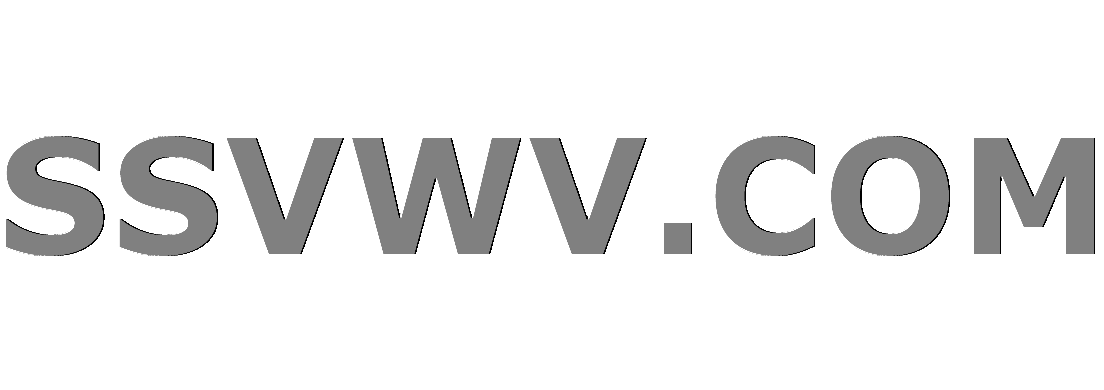
Multi tool use
up vote
0
down vote
favorite
I'm doing some prototype code for a project. I'm using Pillow to open the image and other minor things, but I want to invert the image manually using its pixel values. I used two's complement in hopes of inverting it. However, when I display the final image, it's a solid grey square instead of inverted colors. I just used a picture of a possum, 275 pixels by 183 pixels. Any idea why it displays grey block and not an inverted image?
#importing Image module
from PIL import Image
import numpy
#sets numpy to print out full array
numpy.set_printoptions(threshold=numpy.inf)
def twos_comp(val, bits):
"""compute the 2's complement of int value val"""
if (val & (1 << (bits - 1))) != 0: # if sign bit is set e.g., 8bit: 128-255
val = val - (1 << bits) # compute negative value
return val
im = Image.open('possum.jpg')
#im.show()
data = numpy.asarray(im)
#print(data)
#print("FINISHED PRINTING")
#print('NOW PRINTING BINARY')
data_binary = numpy.unpackbits(data)
data_binary.ravel()
#print(data_binary)
#print('FINISHED PRINTING')
#getting string of binary array
binaryString = numpy.array2string(data_binary)
binaryString = ''.join(binaryString.split())
binaryString = binaryString[:-1]
binaryString = binaryString[1:]
#print("Binary String: " + binaryString)
out = twos_comp(int(binaryString,2), len(binaryString))
#print('Now printing twos:')
#print(out)
#formatting non-binary two's comp as binary
outBinary = "{0:b}".format(out)
#print('Now printing binary twos: ' + outBinary)
outBinary = outBinary.encode('utf-8')
a_pil_image = Image.frombytes('RGB', (275, 183), outBinary)
a_pil_image.show()
python image numpy python-imaging-library pixel
add a comment |
up vote
0
down vote
favorite
I'm doing some prototype code for a project. I'm using Pillow to open the image and other minor things, but I want to invert the image manually using its pixel values. I used two's complement in hopes of inverting it. However, when I display the final image, it's a solid grey square instead of inverted colors. I just used a picture of a possum, 275 pixels by 183 pixels. Any idea why it displays grey block and not an inverted image?
#importing Image module
from PIL import Image
import numpy
#sets numpy to print out full array
numpy.set_printoptions(threshold=numpy.inf)
def twos_comp(val, bits):
"""compute the 2's complement of int value val"""
if (val & (1 << (bits - 1))) != 0: # if sign bit is set e.g., 8bit: 128-255
val = val - (1 << bits) # compute negative value
return val
im = Image.open('possum.jpg')
#im.show()
data = numpy.asarray(im)
#print(data)
#print("FINISHED PRINTING")
#print('NOW PRINTING BINARY')
data_binary = numpy.unpackbits(data)
data_binary.ravel()
#print(data_binary)
#print('FINISHED PRINTING')
#getting string of binary array
binaryString = numpy.array2string(data_binary)
binaryString = ''.join(binaryString.split())
binaryString = binaryString[:-1]
binaryString = binaryString[1:]
#print("Binary String: " + binaryString)
out = twos_comp(int(binaryString,2), len(binaryString))
#print('Now printing twos:')
#print(out)
#formatting non-binary two's comp as binary
outBinary = "{0:b}".format(out)
#print('Now printing binary twos: ' + outBinary)
outBinary = outBinary.encode('utf-8')
a_pil_image = Image.frombytes('RGB', (275, 183), outBinary)
a_pil_image.show()
python image numpy python-imaging-library pixel
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm doing some prototype code for a project. I'm using Pillow to open the image and other minor things, but I want to invert the image manually using its pixel values. I used two's complement in hopes of inverting it. However, when I display the final image, it's a solid grey square instead of inverted colors. I just used a picture of a possum, 275 pixels by 183 pixels. Any idea why it displays grey block and not an inverted image?
#importing Image module
from PIL import Image
import numpy
#sets numpy to print out full array
numpy.set_printoptions(threshold=numpy.inf)
def twos_comp(val, bits):
"""compute the 2's complement of int value val"""
if (val & (1 << (bits - 1))) != 0: # if sign bit is set e.g., 8bit: 128-255
val = val - (1 << bits) # compute negative value
return val
im = Image.open('possum.jpg')
#im.show()
data = numpy.asarray(im)
#print(data)
#print("FINISHED PRINTING")
#print('NOW PRINTING BINARY')
data_binary = numpy.unpackbits(data)
data_binary.ravel()
#print(data_binary)
#print('FINISHED PRINTING')
#getting string of binary array
binaryString = numpy.array2string(data_binary)
binaryString = ''.join(binaryString.split())
binaryString = binaryString[:-1]
binaryString = binaryString[1:]
#print("Binary String: " + binaryString)
out = twos_comp(int(binaryString,2), len(binaryString))
#print('Now printing twos:')
#print(out)
#formatting non-binary two's comp as binary
outBinary = "{0:b}".format(out)
#print('Now printing binary twos: ' + outBinary)
outBinary = outBinary.encode('utf-8')
a_pil_image = Image.frombytes('RGB', (275, 183), outBinary)
a_pil_image.show()
python image numpy python-imaging-library pixel
I'm doing some prototype code for a project. I'm using Pillow to open the image and other minor things, but I want to invert the image manually using its pixel values. I used two's complement in hopes of inverting it. However, when I display the final image, it's a solid grey square instead of inverted colors. I just used a picture of a possum, 275 pixels by 183 pixels. Any idea why it displays grey block and not an inverted image?
#importing Image module
from PIL import Image
import numpy
#sets numpy to print out full array
numpy.set_printoptions(threshold=numpy.inf)
def twos_comp(val, bits):
"""compute the 2's complement of int value val"""
if (val & (1 << (bits - 1))) != 0: # if sign bit is set e.g., 8bit: 128-255
val = val - (1 << bits) # compute negative value
return val
im = Image.open('possum.jpg')
#im.show()
data = numpy.asarray(im)
#print(data)
#print("FINISHED PRINTING")
#print('NOW PRINTING BINARY')
data_binary = numpy.unpackbits(data)
data_binary.ravel()
#print(data_binary)
#print('FINISHED PRINTING')
#getting string of binary array
binaryString = numpy.array2string(data_binary)
binaryString = ''.join(binaryString.split())
binaryString = binaryString[:-1]
binaryString = binaryString[1:]
#print("Binary String: " + binaryString)
out = twos_comp(int(binaryString,2), len(binaryString))
#print('Now printing twos:')
#print(out)
#formatting non-binary two's comp as binary
outBinary = "{0:b}".format(out)
#print('Now printing binary twos: ' + outBinary)
outBinary = outBinary.encode('utf-8')
a_pil_image = Image.frombytes('RGB', (275, 183), outBinary)
a_pil_image.show()
python image numpy python-imaging-library pixel
python image numpy python-imaging-library pixel
asked Nov 18 at 22:39
Braydon Rekart
34
34
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
You can invert quite simply with PIL/Pillow:
from PIL import Image, ImageChops
# Load image from disk and ensure RGB
im = Image.open('lena.png').convert('RGB')
# Invert image and save to disk
res = ImageChops.invert(im)
res.save('result.png')
Turns Lena into negated Lena:
Or, if you want to be more mathematical about it:
from PIL import Image
import numpy as np
im = Image.open('lena.png').convert('RGB')
# Make Numpy array
imnp = np.array(im)
# Invert
imnp = 255 - imnp
# Save
Image.fromarray(imnp).save('result.png')
If you imagine a black image is represented by (0,0,0) and a white image by (255,255,255), it is hopefully not hard to see that inversion of colours is achieved by subtracting from 255 rather than using two's complement.
I can't do this. As I said in the post, I want to convert them manually using the image's pixels themselves and modifying them on my own, not use the library to do all the work.
– Braydon Rekart
Nov 21 at 21:30
Sorry, I didn't see in your question where you said you couldn't use a library. Just use the second piece of code I supplied - that doesn't use a library and it mathematically subtracts each pixel itself from 255.
– Mark Setchell
Nov 21 at 22:08
I could probably have been clearer in my question. Thank you.
– Braydon Rekart
Nov 22 at 5:27
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53366141%2fpython-pixel-manipulation-returning-grey-image-instead-of-inverted%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You can invert quite simply with PIL/Pillow:
from PIL import Image, ImageChops
# Load image from disk and ensure RGB
im = Image.open('lena.png').convert('RGB')
# Invert image and save to disk
res = ImageChops.invert(im)
res.save('result.png')
Turns Lena into negated Lena:
Or, if you want to be more mathematical about it:
from PIL import Image
import numpy as np
im = Image.open('lena.png').convert('RGB')
# Make Numpy array
imnp = np.array(im)
# Invert
imnp = 255 - imnp
# Save
Image.fromarray(imnp).save('result.png')
If you imagine a black image is represented by (0,0,0) and a white image by (255,255,255), it is hopefully not hard to see that inversion of colours is achieved by subtracting from 255 rather than using two's complement.
I can't do this. As I said in the post, I want to convert them manually using the image's pixels themselves and modifying them on my own, not use the library to do all the work.
– Braydon Rekart
Nov 21 at 21:30
Sorry, I didn't see in your question where you said you couldn't use a library. Just use the second piece of code I supplied - that doesn't use a library and it mathematically subtracts each pixel itself from 255.
– Mark Setchell
Nov 21 at 22:08
I could probably have been clearer in my question. Thank you.
– Braydon Rekart
Nov 22 at 5:27
add a comment |
up vote
0
down vote
You can invert quite simply with PIL/Pillow:
from PIL import Image, ImageChops
# Load image from disk and ensure RGB
im = Image.open('lena.png').convert('RGB')
# Invert image and save to disk
res = ImageChops.invert(im)
res.save('result.png')
Turns Lena into negated Lena:
Or, if you want to be more mathematical about it:
from PIL import Image
import numpy as np
im = Image.open('lena.png').convert('RGB')
# Make Numpy array
imnp = np.array(im)
# Invert
imnp = 255 - imnp
# Save
Image.fromarray(imnp).save('result.png')
If you imagine a black image is represented by (0,0,0) and a white image by (255,255,255), it is hopefully not hard to see that inversion of colours is achieved by subtracting from 255 rather than using two's complement.
I can't do this. As I said in the post, I want to convert them manually using the image's pixels themselves and modifying them on my own, not use the library to do all the work.
– Braydon Rekart
Nov 21 at 21:30
Sorry, I didn't see in your question where you said you couldn't use a library. Just use the second piece of code I supplied - that doesn't use a library and it mathematically subtracts each pixel itself from 255.
– Mark Setchell
Nov 21 at 22:08
I could probably have been clearer in my question. Thank you.
– Braydon Rekart
Nov 22 at 5:27
add a comment |
up vote
0
down vote
up vote
0
down vote
You can invert quite simply with PIL/Pillow:
from PIL import Image, ImageChops
# Load image from disk and ensure RGB
im = Image.open('lena.png').convert('RGB')
# Invert image and save to disk
res = ImageChops.invert(im)
res.save('result.png')
Turns Lena into negated Lena:
Or, if you want to be more mathematical about it:
from PIL import Image
import numpy as np
im = Image.open('lena.png').convert('RGB')
# Make Numpy array
imnp = np.array(im)
# Invert
imnp = 255 - imnp
# Save
Image.fromarray(imnp).save('result.png')
If you imagine a black image is represented by (0,0,0) and a white image by (255,255,255), it is hopefully not hard to see that inversion of colours is achieved by subtracting from 255 rather than using two's complement.
You can invert quite simply with PIL/Pillow:
from PIL import Image, ImageChops
# Load image from disk and ensure RGB
im = Image.open('lena.png').convert('RGB')
# Invert image and save to disk
res = ImageChops.invert(im)
res.save('result.png')
Turns Lena into negated Lena:
Or, if you want to be more mathematical about it:
from PIL import Image
import numpy as np
im = Image.open('lena.png').convert('RGB')
# Make Numpy array
imnp = np.array(im)
# Invert
imnp = 255 - imnp
# Save
Image.fromarray(imnp).save('result.png')
If you imagine a black image is represented by (0,0,0) and a white image by (255,255,255), it is hopefully not hard to see that inversion of colours is achieved by subtracting from 255 rather than using two's complement.
edited Nov 19 at 18:55
answered Nov 19 at 18:19


Mark Setchell
85.4k673172
85.4k673172
I can't do this. As I said in the post, I want to convert them manually using the image's pixels themselves and modifying them on my own, not use the library to do all the work.
– Braydon Rekart
Nov 21 at 21:30
Sorry, I didn't see in your question where you said you couldn't use a library. Just use the second piece of code I supplied - that doesn't use a library and it mathematically subtracts each pixel itself from 255.
– Mark Setchell
Nov 21 at 22:08
I could probably have been clearer in my question. Thank you.
– Braydon Rekart
Nov 22 at 5:27
add a comment |
I can't do this. As I said in the post, I want to convert them manually using the image's pixels themselves and modifying them on my own, not use the library to do all the work.
– Braydon Rekart
Nov 21 at 21:30
Sorry, I didn't see in your question where you said you couldn't use a library. Just use the second piece of code I supplied - that doesn't use a library and it mathematically subtracts each pixel itself from 255.
– Mark Setchell
Nov 21 at 22:08
I could probably have been clearer in my question. Thank you.
– Braydon Rekart
Nov 22 at 5:27
I can't do this. As I said in the post, I want to convert them manually using the image's pixels themselves and modifying them on my own, not use the library to do all the work.
– Braydon Rekart
Nov 21 at 21:30
I can't do this. As I said in the post, I want to convert them manually using the image's pixels themselves and modifying them on my own, not use the library to do all the work.
– Braydon Rekart
Nov 21 at 21:30
Sorry, I didn't see in your question where you said you couldn't use a library. Just use the second piece of code I supplied - that doesn't use a library and it mathematically subtracts each pixel itself from 255.
– Mark Setchell
Nov 21 at 22:08
Sorry, I didn't see in your question where you said you couldn't use a library. Just use the second piece of code I supplied - that doesn't use a library and it mathematically subtracts each pixel itself from 255.
– Mark Setchell
Nov 21 at 22:08
I could probably have been clearer in my question. Thank you.
– Braydon Rekart
Nov 22 at 5:27
I could probably have been clearer in my question. Thank you.
– Braydon Rekart
Nov 22 at 5:27
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53366141%2fpython-pixel-manipulation-returning-grey-image-instead-of-inverted%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lAeSk799Awy,EU g9D3X27rikkCo