How to use a specific query in Retrofit with Android?
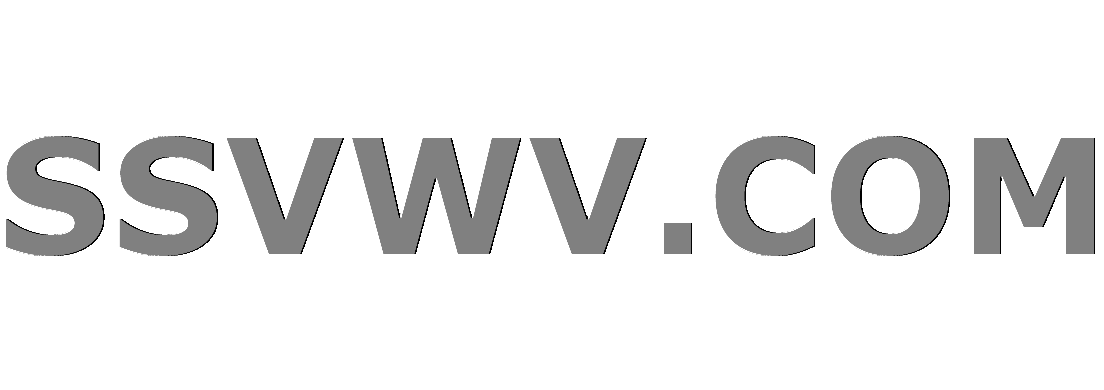
Multi tool use
I have an API
interface which has two overloaded methods:
public interface Api {
@GET("movie/now_playing")
Call<ApiResponse> getMovies(@Query("page") int page);
@GET("search/movie")
Call<ApiResponse> getMovies(@Query("query") String query, @Query("page") int page);
}
First one is used to get now playing movies and the second one to search for movies.
In my MainActivity
I use this code to display the data in a RecyclerView:
MovieViewModel movieViewModel = ViewModelProviders.of(this).get(MovieViewModel.class);
MovieAdapter adapter = new MovieAdapter(this);
movieViewModel.moviePagedList.observe(this, adapter::submitList);
recyclerView.setAdapter(adapter);
This is my MovieViewModel
class:
public class MovieViewModel extends ViewModel {
LiveData<PagedList<ApiResponse.Movie>> moviePagedList;
public MovieViewModel() {
MovieDataSourceFactory movieDataSourceFactory = new MovieDataSourceFactory();
PagedList.Config config = new PagedList.Config.Builder().setEnablePlaceholders(false).setPageSize(20).build();
moviePagedList = new LivePagedListBuilder<>(movieDataSourceFactory, config).build();
}
}
And this my MovieDataSourceFactory
class:
public class MovieDataSourceFactory extends DataSource.Factory<Integer, ApiResponse.Movie> {
private MutableLiveData<PageKeyedDataSource<Integer, ApiResponse.Movie>> movieLiveDataSource = new MutableLiveData<>();
@Override
public DataSource<Integer, ApiResponse.Movie> create() {
MovieDataSource movieDataSource = new MovieDataSource();
movieLiveDataSource.postValue(movieDataSource);
return movieDataSource;
}
}
And this is my MovieDataSource
class:
public class MovieDataSource extends PageKeyedDataSource<Integer, ApiResponse.Movie> {
@Override
public void loadInitial(@NonNull LoadInitialParams<Integer> params, @NonNull LoadInitialCallback<Integer, ApiResponse.Movie> callback) {
Api api = RetrofitClient.getInstance().getApi();
Callback<ApiResponse> call = new Callback<ApiResponse>() {
@Override
public void onResponse(@NonNull Call<ApiResponse> call, @NonNull Response<ApiResponse> response) {
if(response.body() != null){
callback.onResult(response.body().movieList, null, page + 1);
}
}
@Override
public void onFailure(@NonNull Call<ApiResponse> call, @NonNull Throwable t) {}
};
api.getMovies(page).enqueue(call);
}
//loadBefore and loadAfter methods
}
If I run this code, everything works fine, the first query is invoked and I get the result correctly. The question is, how can I dynamically use one or the other?
api.getMovies(query, page).enqueue(call);
android retrofit retrofit2 android-paging android-paging-library
|
show 10 more comments
I have an API
interface which has two overloaded methods:
public interface Api {
@GET("movie/now_playing")
Call<ApiResponse> getMovies(@Query("page") int page);
@GET("search/movie")
Call<ApiResponse> getMovies(@Query("query") String query, @Query("page") int page);
}
First one is used to get now playing movies and the second one to search for movies.
In my MainActivity
I use this code to display the data in a RecyclerView:
MovieViewModel movieViewModel = ViewModelProviders.of(this).get(MovieViewModel.class);
MovieAdapter adapter = new MovieAdapter(this);
movieViewModel.moviePagedList.observe(this, adapter::submitList);
recyclerView.setAdapter(adapter);
This is my MovieViewModel
class:
public class MovieViewModel extends ViewModel {
LiveData<PagedList<ApiResponse.Movie>> moviePagedList;
public MovieViewModel() {
MovieDataSourceFactory movieDataSourceFactory = new MovieDataSourceFactory();
PagedList.Config config = new PagedList.Config.Builder().setEnablePlaceholders(false).setPageSize(20).build();
moviePagedList = new LivePagedListBuilder<>(movieDataSourceFactory, config).build();
}
}
And this my MovieDataSourceFactory
class:
public class MovieDataSourceFactory extends DataSource.Factory<Integer, ApiResponse.Movie> {
private MutableLiveData<PageKeyedDataSource<Integer, ApiResponse.Movie>> movieLiveDataSource = new MutableLiveData<>();
@Override
public DataSource<Integer, ApiResponse.Movie> create() {
MovieDataSource movieDataSource = new MovieDataSource();
movieLiveDataSource.postValue(movieDataSource);
return movieDataSource;
}
}
And this is my MovieDataSource
class:
public class MovieDataSource extends PageKeyedDataSource<Integer, ApiResponse.Movie> {
@Override
public void loadInitial(@NonNull LoadInitialParams<Integer> params, @NonNull LoadInitialCallback<Integer, ApiResponse.Movie> callback) {
Api api = RetrofitClient.getInstance().getApi();
Callback<ApiResponse> call = new Callback<ApiResponse>() {
@Override
public void onResponse(@NonNull Call<ApiResponse> call, @NonNull Response<ApiResponse> response) {
if(response.body() != null){
callback.onResult(response.body().movieList, null, page + 1);
}
}
@Override
public void onFailure(@NonNull Call<ApiResponse> call, @NonNull Throwable t) {}
};
api.getMovies(page).enqueue(call);
}
//loadBefore and loadAfter methods
}
If I run this code, everything works fine, the first query is invoked and I get the result correctly. The question is, how can I dynamically use one or the other?
api.getMovies(query, page).enqueue(call);
android retrofit retrofit2 android-paging android-paging-library
codingbat.com/doc/java-if-boolean-logic.html or tutorialspoint.com/design_pattern/strategy_pattern.htm
– ZUNJAE
Nov 20 '18 at 9:25
Can you please be more specific regarding my use-case? Thanks!
– Johans Bormman
Nov 20 '18 at 9:26
What do you mean dynamically use one or the other? Like, having a method that receives for example a@QueryMap
and you just call it with an arbitrary number of query parameters?
– Fred
Nov 20 '18 at 9:27
You say you dynamically want to switch between eithergetMovies()
right? There are multiple ways of doings this, one of them being using a boolean flag. Example:if (true) { api.getMovies(a,b,c) } else { api.getMovies(a,b) }
. The issue with this is an if-statement mess. This can be solved by implementing a strategy pattern.
– ZUNJAE
Nov 20 '18 at 9:28
jetbrains.com/help/idea/… "When you have a method with lots of conditional logic (i.e., if statements), you're asking for trouble. Conditional logic is notoriously difficult to manage, and may cause you to create an entire state machine inside a single method."
– ZUNJAE
Nov 20 '18 at 9:29
|
show 10 more comments
I have an API
interface which has two overloaded methods:
public interface Api {
@GET("movie/now_playing")
Call<ApiResponse> getMovies(@Query("page") int page);
@GET("search/movie")
Call<ApiResponse> getMovies(@Query("query") String query, @Query("page") int page);
}
First one is used to get now playing movies and the second one to search for movies.
In my MainActivity
I use this code to display the data in a RecyclerView:
MovieViewModel movieViewModel = ViewModelProviders.of(this).get(MovieViewModel.class);
MovieAdapter adapter = new MovieAdapter(this);
movieViewModel.moviePagedList.observe(this, adapter::submitList);
recyclerView.setAdapter(adapter);
This is my MovieViewModel
class:
public class MovieViewModel extends ViewModel {
LiveData<PagedList<ApiResponse.Movie>> moviePagedList;
public MovieViewModel() {
MovieDataSourceFactory movieDataSourceFactory = new MovieDataSourceFactory();
PagedList.Config config = new PagedList.Config.Builder().setEnablePlaceholders(false).setPageSize(20).build();
moviePagedList = new LivePagedListBuilder<>(movieDataSourceFactory, config).build();
}
}
And this my MovieDataSourceFactory
class:
public class MovieDataSourceFactory extends DataSource.Factory<Integer, ApiResponse.Movie> {
private MutableLiveData<PageKeyedDataSource<Integer, ApiResponse.Movie>> movieLiveDataSource = new MutableLiveData<>();
@Override
public DataSource<Integer, ApiResponse.Movie> create() {
MovieDataSource movieDataSource = new MovieDataSource();
movieLiveDataSource.postValue(movieDataSource);
return movieDataSource;
}
}
And this is my MovieDataSource
class:
public class MovieDataSource extends PageKeyedDataSource<Integer, ApiResponse.Movie> {
@Override
public void loadInitial(@NonNull LoadInitialParams<Integer> params, @NonNull LoadInitialCallback<Integer, ApiResponse.Movie> callback) {
Api api = RetrofitClient.getInstance().getApi();
Callback<ApiResponse> call = new Callback<ApiResponse>() {
@Override
public void onResponse(@NonNull Call<ApiResponse> call, @NonNull Response<ApiResponse> response) {
if(response.body() != null){
callback.onResult(response.body().movieList, null, page + 1);
}
}
@Override
public void onFailure(@NonNull Call<ApiResponse> call, @NonNull Throwable t) {}
};
api.getMovies(page).enqueue(call);
}
//loadBefore and loadAfter methods
}
If I run this code, everything works fine, the first query is invoked and I get the result correctly. The question is, how can I dynamically use one or the other?
api.getMovies(query, page).enqueue(call);
android retrofit retrofit2 android-paging android-paging-library
I have an API
interface which has two overloaded methods:
public interface Api {
@GET("movie/now_playing")
Call<ApiResponse> getMovies(@Query("page") int page);
@GET("search/movie")
Call<ApiResponse> getMovies(@Query("query") String query, @Query("page") int page);
}
First one is used to get now playing movies and the second one to search for movies.
In my MainActivity
I use this code to display the data in a RecyclerView:
MovieViewModel movieViewModel = ViewModelProviders.of(this).get(MovieViewModel.class);
MovieAdapter adapter = new MovieAdapter(this);
movieViewModel.moviePagedList.observe(this, adapter::submitList);
recyclerView.setAdapter(adapter);
This is my MovieViewModel
class:
public class MovieViewModel extends ViewModel {
LiveData<PagedList<ApiResponse.Movie>> moviePagedList;
public MovieViewModel() {
MovieDataSourceFactory movieDataSourceFactory = new MovieDataSourceFactory();
PagedList.Config config = new PagedList.Config.Builder().setEnablePlaceholders(false).setPageSize(20).build();
moviePagedList = new LivePagedListBuilder<>(movieDataSourceFactory, config).build();
}
}
And this my MovieDataSourceFactory
class:
public class MovieDataSourceFactory extends DataSource.Factory<Integer, ApiResponse.Movie> {
private MutableLiveData<PageKeyedDataSource<Integer, ApiResponse.Movie>> movieLiveDataSource = new MutableLiveData<>();
@Override
public DataSource<Integer, ApiResponse.Movie> create() {
MovieDataSource movieDataSource = new MovieDataSource();
movieLiveDataSource.postValue(movieDataSource);
return movieDataSource;
}
}
And this is my MovieDataSource
class:
public class MovieDataSource extends PageKeyedDataSource<Integer, ApiResponse.Movie> {
@Override
public void loadInitial(@NonNull LoadInitialParams<Integer> params, @NonNull LoadInitialCallback<Integer, ApiResponse.Movie> callback) {
Api api = RetrofitClient.getInstance().getApi();
Callback<ApiResponse> call = new Callback<ApiResponse>() {
@Override
public void onResponse(@NonNull Call<ApiResponse> call, @NonNull Response<ApiResponse> response) {
if(response.body() != null){
callback.onResult(response.body().movieList, null, page + 1);
}
}
@Override
public void onFailure(@NonNull Call<ApiResponse> call, @NonNull Throwable t) {}
};
api.getMovies(page).enqueue(call);
}
//loadBefore and loadAfter methods
}
If I run this code, everything works fine, the first query is invoked and I get the result correctly. The question is, how can I dynamically use one or the other?
api.getMovies(query, page).enqueue(call);
android retrofit retrofit2 android-paging android-paging-library
android retrofit retrofit2 android-paging android-paging-library
edited Nov 20 '18 at 10:53
asked Nov 20 '18 at 9:16
Johans Bormman
459
459
codingbat.com/doc/java-if-boolean-logic.html or tutorialspoint.com/design_pattern/strategy_pattern.htm
– ZUNJAE
Nov 20 '18 at 9:25
Can you please be more specific regarding my use-case? Thanks!
– Johans Bormman
Nov 20 '18 at 9:26
What do you mean dynamically use one or the other? Like, having a method that receives for example a@QueryMap
and you just call it with an arbitrary number of query parameters?
– Fred
Nov 20 '18 at 9:27
You say you dynamically want to switch between eithergetMovies()
right? There are multiple ways of doings this, one of them being using a boolean flag. Example:if (true) { api.getMovies(a,b,c) } else { api.getMovies(a,b) }
. The issue with this is an if-statement mess. This can be solved by implementing a strategy pattern.
– ZUNJAE
Nov 20 '18 at 9:28
jetbrains.com/help/idea/… "When you have a method with lots of conditional logic (i.e., if statements), you're asking for trouble. Conditional logic is notoriously difficult to manage, and may cause you to create an entire state machine inside a single method."
– ZUNJAE
Nov 20 '18 at 9:29
|
show 10 more comments
codingbat.com/doc/java-if-boolean-logic.html or tutorialspoint.com/design_pattern/strategy_pattern.htm
– ZUNJAE
Nov 20 '18 at 9:25
Can you please be more specific regarding my use-case? Thanks!
– Johans Bormman
Nov 20 '18 at 9:26
What do you mean dynamically use one or the other? Like, having a method that receives for example a@QueryMap
and you just call it with an arbitrary number of query parameters?
– Fred
Nov 20 '18 at 9:27
You say you dynamically want to switch between eithergetMovies()
right? There are multiple ways of doings this, one of them being using a boolean flag. Example:if (true) { api.getMovies(a,b,c) } else { api.getMovies(a,b) }
. The issue with this is an if-statement mess. This can be solved by implementing a strategy pattern.
– ZUNJAE
Nov 20 '18 at 9:28
jetbrains.com/help/idea/… "When you have a method with lots of conditional logic (i.e., if statements), you're asking for trouble. Conditional logic is notoriously difficult to manage, and may cause you to create an entire state machine inside a single method."
– ZUNJAE
Nov 20 '18 at 9:29
codingbat.com/doc/java-if-boolean-logic.html or tutorialspoint.com/design_pattern/strategy_pattern.htm
– ZUNJAE
Nov 20 '18 at 9:25
codingbat.com/doc/java-if-boolean-logic.html or tutorialspoint.com/design_pattern/strategy_pattern.htm
– ZUNJAE
Nov 20 '18 at 9:25
Can you please be more specific regarding my use-case? Thanks!
– Johans Bormman
Nov 20 '18 at 9:26
Can you please be more specific regarding my use-case? Thanks!
– Johans Bormman
Nov 20 '18 at 9:26
What do you mean dynamically use one or the other? Like, having a method that receives for example a
@QueryMap
and you just call it with an arbitrary number of query parameters?– Fred
Nov 20 '18 at 9:27
What do you mean dynamically use one or the other? Like, having a method that receives for example a
@QueryMap
and you just call it with an arbitrary number of query parameters?– Fred
Nov 20 '18 at 9:27
You say you dynamically want to switch between either
getMovies()
right? There are multiple ways of doings this, one of them being using a boolean flag. Example: if (true) { api.getMovies(a,b,c) } else { api.getMovies(a,b) }
. The issue with this is an if-statement mess. This can be solved by implementing a strategy pattern.– ZUNJAE
Nov 20 '18 at 9:28
You say you dynamically want to switch between either
getMovies()
right? There are multiple ways of doings this, one of them being using a boolean flag. Example: if (true) { api.getMovies(a,b,c) } else { api.getMovies(a,b) }
. The issue with this is an if-statement mess. This can be solved by implementing a strategy pattern.– ZUNJAE
Nov 20 '18 at 9:28
jetbrains.com/help/idea/… "When you have a method with lots of conditional logic (i.e., if statements), you're asking for trouble. Conditional logic is notoriously difficult to manage, and may cause you to create an entire state machine inside a single method."
– ZUNJAE
Nov 20 '18 at 9:29
jetbrains.com/help/idea/… "When you have a method with lots of conditional logic (i.e., if statements), you're asking for trouble. Conditional logic is notoriously difficult to manage, and may cause you to create an entire state machine inside a single method."
– ZUNJAE
Nov 20 '18 at 9:29
|
show 10 more comments
1 Answer
1
active
oldest
votes
boolean globalVariableShouldUseGetMoviesCallWithOnlyOneArgument = true
private void doNetworkCallAndCheckBooleanStatus(){
if (globalVariableShouldUseGetMoviesCallWithOnlyOneArgument) {
getMovies(thisIsOneArgumentAsYouCanSee);
} else {
getMovies(thisIsUsingTwoArguments, asYouCanSee);
}
}
private void someOtherMethodInTheApp() {
globalVariableShouldUseGetMoviesCallWithOnlyOneArgument = false
doNetworkCallAndCheckBooleanStatus()
}
Thanks for your answer but I it doesn't work. I need this in realtime. Using those 4 lines it is displaying by default the result from the first query. Once I press a button, I want to display the result of the second query, ok? Cand you help me achieve this?
– Johans Bormman
Nov 20 '18 at 10:45
I need the first argument of thegetMovies()
method from the else part to be dynamically.
– Johans Bormman
Nov 20 '18 at 10:47
Do you know what if-statements are?
– ZUNJAE
Nov 20 '18 at 10:50
No, this not what I want. Let me explain again. I need that data in real-time. For example, now, in myMainActivity
I use those 4 lines of code to display data in aRecyclerView
, which works fine because is calling the method with one argument. Now I want at button click, to send a String to theapi.getMovies("MyStringFromMaiNQActivity", page).enqueue(call);
in order to use the second method. Am I clear now?
– Johans Bormman
Nov 20 '18 at 10:55
Let's see if I understand you correctly. Sometimes you want to call getMovies with 1 argument and on button click 2 arguments, right?
– ZUNJAE
Nov 20 '18 at 10:57
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53389701%2fhow-to-use-a-specific-query-in-retrofit-with-android%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
boolean globalVariableShouldUseGetMoviesCallWithOnlyOneArgument = true
private void doNetworkCallAndCheckBooleanStatus(){
if (globalVariableShouldUseGetMoviesCallWithOnlyOneArgument) {
getMovies(thisIsOneArgumentAsYouCanSee);
} else {
getMovies(thisIsUsingTwoArguments, asYouCanSee);
}
}
private void someOtherMethodInTheApp() {
globalVariableShouldUseGetMoviesCallWithOnlyOneArgument = false
doNetworkCallAndCheckBooleanStatus()
}
Thanks for your answer but I it doesn't work. I need this in realtime. Using those 4 lines it is displaying by default the result from the first query. Once I press a button, I want to display the result of the second query, ok? Cand you help me achieve this?
– Johans Bormman
Nov 20 '18 at 10:45
I need the first argument of thegetMovies()
method from the else part to be dynamically.
– Johans Bormman
Nov 20 '18 at 10:47
Do you know what if-statements are?
– ZUNJAE
Nov 20 '18 at 10:50
No, this not what I want. Let me explain again. I need that data in real-time. For example, now, in myMainActivity
I use those 4 lines of code to display data in aRecyclerView
, which works fine because is calling the method with one argument. Now I want at button click, to send a String to theapi.getMovies("MyStringFromMaiNQActivity", page).enqueue(call);
in order to use the second method. Am I clear now?
– Johans Bormman
Nov 20 '18 at 10:55
Let's see if I understand you correctly. Sometimes you want to call getMovies with 1 argument and on button click 2 arguments, right?
– ZUNJAE
Nov 20 '18 at 10:57
|
show 1 more comment
boolean globalVariableShouldUseGetMoviesCallWithOnlyOneArgument = true
private void doNetworkCallAndCheckBooleanStatus(){
if (globalVariableShouldUseGetMoviesCallWithOnlyOneArgument) {
getMovies(thisIsOneArgumentAsYouCanSee);
} else {
getMovies(thisIsUsingTwoArguments, asYouCanSee);
}
}
private void someOtherMethodInTheApp() {
globalVariableShouldUseGetMoviesCallWithOnlyOneArgument = false
doNetworkCallAndCheckBooleanStatus()
}
Thanks for your answer but I it doesn't work. I need this in realtime. Using those 4 lines it is displaying by default the result from the first query. Once I press a button, I want to display the result of the second query, ok? Cand you help me achieve this?
– Johans Bormman
Nov 20 '18 at 10:45
I need the first argument of thegetMovies()
method from the else part to be dynamically.
– Johans Bormman
Nov 20 '18 at 10:47
Do you know what if-statements are?
– ZUNJAE
Nov 20 '18 at 10:50
No, this not what I want. Let me explain again. I need that data in real-time. For example, now, in myMainActivity
I use those 4 lines of code to display data in aRecyclerView
, which works fine because is calling the method with one argument. Now I want at button click, to send a String to theapi.getMovies("MyStringFromMaiNQActivity", page).enqueue(call);
in order to use the second method. Am I clear now?
– Johans Bormman
Nov 20 '18 at 10:55
Let's see if I understand you correctly. Sometimes you want to call getMovies with 1 argument and on button click 2 arguments, right?
– ZUNJAE
Nov 20 '18 at 10:57
|
show 1 more comment
boolean globalVariableShouldUseGetMoviesCallWithOnlyOneArgument = true
private void doNetworkCallAndCheckBooleanStatus(){
if (globalVariableShouldUseGetMoviesCallWithOnlyOneArgument) {
getMovies(thisIsOneArgumentAsYouCanSee);
} else {
getMovies(thisIsUsingTwoArguments, asYouCanSee);
}
}
private void someOtherMethodInTheApp() {
globalVariableShouldUseGetMoviesCallWithOnlyOneArgument = false
doNetworkCallAndCheckBooleanStatus()
}
boolean globalVariableShouldUseGetMoviesCallWithOnlyOneArgument = true
private void doNetworkCallAndCheckBooleanStatus(){
if (globalVariableShouldUseGetMoviesCallWithOnlyOneArgument) {
getMovies(thisIsOneArgumentAsYouCanSee);
} else {
getMovies(thisIsUsingTwoArguments, asYouCanSee);
}
}
private void someOtherMethodInTheApp() {
globalVariableShouldUseGetMoviesCallWithOnlyOneArgument = false
doNetworkCallAndCheckBooleanStatus()
}
answered Nov 20 '18 at 9:47


ZUNJAE
6501618
6501618
Thanks for your answer but I it doesn't work. I need this in realtime. Using those 4 lines it is displaying by default the result from the first query. Once I press a button, I want to display the result of the second query, ok? Cand you help me achieve this?
– Johans Bormman
Nov 20 '18 at 10:45
I need the first argument of thegetMovies()
method from the else part to be dynamically.
– Johans Bormman
Nov 20 '18 at 10:47
Do you know what if-statements are?
– ZUNJAE
Nov 20 '18 at 10:50
No, this not what I want. Let me explain again. I need that data in real-time. For example, now, in myMainActivity
I use those 4 lines of code to display data in aRecyclerView
, which works fine because is calling the method with one argument. Now I want at button click, to send a String to theapi.getMovies("MyStringFromMaiNQActivity", page).enqueue(call);
in order to use the second method. Am I clear now?
– Johans Bormman
Nov 20 '18 at 10:55
Let's see if I understand you correctly. Sometimes you want to call getMovies with 1 argument and on button click 2 arguments, right?
– ZUNJAE
Nov 20 '18 at 10:57
|
show 1 more comment
Thanks for your answer but I it doesn't work. I need this in realtime. Using those 4 lines it is displaying by default the result from the first query. Once I press a button, I want to display the result of the second query, ok? Cand you help me achieve this?
– Johans Bormman
Nov 20 '18 at 10:45
I need the first argument of thegetMovies()
method from the else part to be dynamically.
– Johans Bormman
Nov 20 '18 at 10:47
Do you know what if-statements are?
– ZUNJAE
Nov 20 '18 at 10:50
No, this not what I want. Let me explain again. I need that data in real-time. For example, now, in myMainActivity
I use those 4 lines of code to display data in aRecyclerView
, which works fine because is calling the method with one argument. Now I want at button click, to send a String to theapi.getMovies("MyStringFromMaiNQActivity", page).enqueue(call);
in order to use the second method. Am I clear now?
– Johans Bormman
Nov 20 '18 at 10:55
Let's see if I understand you correctly. Sometimes you want to call getMovies with 1 argument and on button click 2 arguments, right?
– ZUNJAE
Nov 20 '18 at 10:57
Thanks for your answer but I it doesn't work. I need this in realtime. Using those 4 lines it is displaying by default the result from the first query. Once I press a button, I want to display the result of the second query, ok? Cand you help me achieve this?
– Johans Bormman
Nov 20 '18 at 10:45
Thanks for your answer but I it doesn't work. I need this in realtime. Using those 4 lines it is displaying by default the result from the first query. Once I press a button, I want to display the result of the second query, ok? Cand you help me achieve this?
– Johans Bormman
Nov 20 '18 at 10:45
I need the first argument of the
getMovies()
method from the else part to be dynamically.– Johans Bormman
Nov 20 '18 at 10:47
I need the first argument of the
getMovies()
method from the else part to be dynamically.– Johans Bormman
Nov 20 '18 at 10:47
Do you know what if-statements are?
– ZUNJAE
Nov 20 '18 at 10:50
Do you know what if-statements are?
– ZUNJAE
Nov 20 '18 at 10:50
No, this not what I want. Let me explain again. I need that data in real-time. For example, now, in my
MainActivity
I use those 4 lines of code to display data in a RecyclerView
, which works fine because is calling the method with one argument. Now I want at button click, to send a String to the api.getMovies("MyStringFromMaiNQActivity", page).enqueue(call);
in order to use the second method. Am I clear now?– Johans Bormman
Nov 20 '18 at 10:55
No, this not what I want. Let me explain again. I need that data in real-time. For example, now, in my
MainActivity
I use those 4 lines of code to display data in a RecyclerView
, which works fine because is calling the method with one argument. Now I want at button click, to send a String to the api.getMovies("MyStringFromMaiNQActivity", page).enqueue(call);
in order to use the second method. Am I clear now?– Johans Bormman
Nov 20 '18 at 10:55
Let's see if I understand you correctly. Sometimes you want to call getMovies with 1 argument and on button click 2 arguments, right?
– ZUNJAE
Nov 20 '18 at 10:57
Let's see if I understand you correctly. Sometimes you want to call getMovies with 1 argument and on button click 2 arguments, right?
– ZUNJAE
Nov 20 '18 at 10:57
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53389701%2fhow-to-use-a-specific-query-in-retrofit-with-android%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gntCUAIRSGc 1PjYV
codingbat.com/doc/java-if-boolean-logic.html or tutorialspoint.com/design_pattern/strategy_pattern.htm
– ZUNJAE
Nov 20 '18 at 9:25
Can you please be more specific regarding my use-case? Thanks!
– Johans Bormman
Nov 20 '18 at 9:26
What do you mean dynamically use one or the other? Like, having a method that receives for example a
@QueryMap
and you just call it with an arbitrary number of query parameters?– Fred
Nov 20 '18 at 9:27
You say you dynamically want to switch between either
getMovies()
right? There are multiple ways of doings this, one of them being using a boolean flag. Example:if (true) { api.getMovies(a,b,c) } else { api.getMovies(a,b) }
. The issue with this is an if-statement mess. This can be solved by implementing a strategy pattern.– ZUNJAE
Nov 20 '18 at 9:28
jetbrains.com/help/idea/… "When you have a method with lots of conditional logic (i.e., if statements), you're asking for trouble. Conditional logic is notoriously difficult to manage, and may cause you to create an entire state machine inside a single method."
– ZUNJAE
Nov 20 '18 at 9:29