Offer options if record already exists in Ruby on Rails
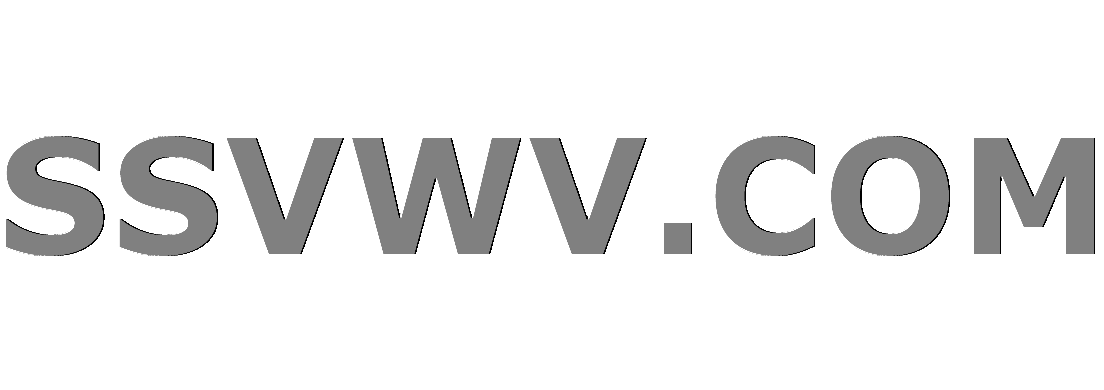
Multi tool use
up vote
0
down vote
favorite
I'm creating a database of products and want to check when a new product is created, if that product already exists.
99% of the time, the product should have a unique product code, but sometimes this will not be the case when a revised version comes out with the same product code.
I would like to have it so that the database checks if the product code exists already. If it does, then the user can either go ahead and create the product anyway, go to the product that already exists, or cancel.
I am trying to achieve this in the controller but cannot seem to get the syntax of the exists? method correct. Can someone point out where I am going wrong, please?
def create
@product = Product.new(product_params)
if Product.product_code.exists?(@product.product_code)
render 'new'
flash[:error] = "This product already exists."
elsif @product.save
redirect_to @product
else
render 'new'
end
end
ruby-on-rails ruby sqlite ruby-on-rails-5
add a comment |
up vote
0
down vote
favorite
I'm creating a database of products and want to check when a new product is created, if that product already exists.
99% of the time, the product should have a unique product code, but sometimes this will not be the case when a revised version comes out with the same product code.
I would like to have it so that the database checks if the product code exists already. If it does, then the user can either go ahead and create the product anyway, go to the product that already exists, or cancel.
I am trying to achieve this in the controller but cannot seem to get the syntax of the exists? method correct. Can someone point out where I am going wrong, please?
def create
@product = Product.new(product_params)
if Product.product_code.exists?(@product.product_code)
render 'new'
flash[:error] = "This product already exists."
elsif @product.save
redirect_to @product
else
render 'new'
end
end
ruby-on-rails ruby sqlite ruby-on-rails-5
You can use active record's validations (uniqueness validation) on the product_code field so@product
won't save if the product_code is already in use and then you can check if@product.errors[:product_code]
show the taken error.
– arieljuod
22 hours ago
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm creating a database of products and want to check when a new product is created, if that product already exists.
99% of the time, the product should have a unique product code, but sometimes this will not be the case when a revised version comes out with the same product code.
I would like to have it so that the database checks if the product code exists already. If it does, then the user can either go ahead and create the product anyway, go to the product that already exists, or cancel.
I am trying to achieve this in the controller but cannot seem to get the syntax of the exists? method correct. Can someone point out where I am going wrong, please?
def create
@product = Product.new(product_params)
if Product.product_code.exists?(@product.product_code)
render 'new'
flash[:error] = "This product already exists."
elsif @product.save
redirect_to @product
else
render 'new'
end
end
ruby-on-rails ruby sqlite ruby-on-rails-5
I'm creating a database of products and want to check when a new product is created, if that product already exists.
99% of the time, the product should have a unique product code, but sometimes this will not be the case when a revised version comes out with the same product code.
I would like to have it so that the database checks if the product code exists already. If it does, then the user can either go ahead and create the product anyway, go to the product that already exists, or cancel.
I am trying to achieve this in the controller but cannot seem to get the syntax of the exists? method correct. Can someone point out where I am going wrong, please?
def create
@product = Product.new(product_params)
if Product.product_code.exists?(@product.product_code)
render 'new'
flash[:error] = "This product already exists."
elsif @product.save
redirect_to @product
else
render 'new'
end
end
ruby-on-rails ruby sqlite ruby-on-rails-5
ruby-on-rails ruby sqlite ruby-on-rails-5
asked 23 hours ago
Emily
255
255
You can use active record's validations (uniqueness validation) on the product_code field so@product
won't save if the product_code is already in use and then you can check if@product.errors[:product_code]
show the taken error.
– arieljuod
22 hours ago
add a comment |
You can use active record's validations (uniqueness validation) on the product_code field so@product
won't save if the product_code is already in use and then you can check if@product.errors[:product_code]
show the taken error.
– arieljuod
22 hours ago
You can use active record's validations (uniqueness validation) on the product_code field so
@product
won't save if the product_code is already in use and then you can check if @product.errors[:product_code]
show the taken error.– arieljuod
22 hours ago
You can use active record's validations (uniqueness validation) on the product_code field so
@product
won't save if the product_code is already in use and then you can check if @product.errors[:product_code]
show the taken error.– arieljuod
22 hours ago
add a comment |
2 Answers
2
active
oldest
votes
up vote
-1
down vote
accepted
Instead if Product.product_code.exists?(@product.product_code)
possibly you should use if @product.product_code.present?
UPD: thanks to all, commented below.
Correct use of exists?
is Product.where(product_code: @product.product_code).exists?
I tried that - unfortunately it gives an error: undefined method `exists?' for "1234":String
– Emily
23 hours ago
what error message it gives? Post it here.
– igor_rb
23 hours ago
undefined method `exists?' for "1234":String - 1234 is the product code I was trying out.
– Emily
23 hours ago
I updated my answer
– igor_rb
23 hours ago
1
Correct use ofexists?
isProduct.where(product_code: @product.product_code).exists?
– Dorian
12 hours ago
|
show 3 more comments
up vote
0
down vote
You should note that what you are doing in the controller or adding a standard uniqueness validation to the model will not allow duplicate Products to be created at all.
This will just keep sending the user back to the form:
def create
@product = Product.new(product_params)
if Product.exists?(product_code: @product.product_code)
render 'new'
flash[:error] = "This product already exists."
elsif @product.save
redirect_to @product
else
render 'new'
end
end
If you want to simply warn the user once you can attach a virtual attribute on the model and use it as condition for the validation:
class Product < ApplicationRecord
attribute :dup_warning, :boolean, default: true
validate :lax_product_code_uniquenes
def lax_product_code_uniqueness
if new_record? && !dup_warning && Product.exists(product_code: self.product_code)
errors.add(:product_code, 'is not unique - are you sure?')
self.dup_warning = true
end
end
end
Then add the virtual attribute to the form:
<%= form_with(model: @product) do |f| %>
...
<%= f.hidden_input(:dup_warning) %>
...
<% end %>
And you don't need to really do anything in the controller besides add dup_warning
to the params whitelist.
def create
@product = Product.new(product_params)
if @product.save
redirect_to @product
else
render 'new'
end
end
def product_params
params.require(:product)
.permit(:foo, :bar, :product_code, :dup_warning)
end
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
-1
down vote
accepted
Instead if Product.product_code.exists?(@product.product_code)
possibly you should use if @product.product_code.present?
UPD: thanks to all, commented below.
Correct use of exists?
is Product.where(product_code: @product.product_code).exists?
I tried that - unfortunately it gives an error: undefined method `exists?' for "1234":String
– Emily
23 hours ago
what error message it gives? Post it here.
– igor_rb
23 hours ago
undefined method `exists?' for "1234":String - 1234 is the product code I was trying out.
– Emily
23 hours ago
I updated my answer
– igor_rb
23 hours ago
1
Correct use ofexists?
isProduct.where(product_code: @product.product_code).exists?
– Dorian
12 hours ago
|
show 3 more comments
up vote
-1
down vote
accepted
Instead if Product.product_code.exists?(@product.product_code)
possibly you should use if @product.product_code.present?
UPD: thanks to all, commented below.
Correct use of exists?
is Product.where(product_code: @product.product_code).exists?
I tried that - unfortunately it gives an error: undefined method `exists?' for "1234":String
– Emily
23 hours ago
what error message it gives? Post it here.
– igor_rb
23 hours ago
undefined method `exists?' for "1234":String - 1234 is the product code I was trying out.
– Emily
23 hours ago
I updated my answer
– igor_rb
23 hours ago
1
Correct use ofexists?
isProduct.where(product_code: @product.product_code).exists?
– Dorian
12 hours ago
|
show 3 more comments
up vote
-1
down vote
accepted
up vote
-1
down vote
accepted
Instead if Product.product_code.exists?(@product.product_code)
possibly you should use if @product.product_code.present?
UPD: thanks to all, commented below.
Correct use of exists?
is Product.where(product_code: @product.product_code).exists?
Instead if Product.product_code.exists?(@product.product_code)
possibly you should use if @product.product_code.present?
UPD: thanks to all, commented below.
Correct use of exists?
is Product.where(product_code: @product.product_code).exists?
edited 11 hours ago
answered 23 hours ago


igor_rb
853624
853624
I tried that - unfortunately it gives an error: undefined method `exists?' for "1234":String
– Emily
23 hours ago
what error message it gives? Post it here.
– igor_rb
23 hours ago
undefined method `exists?' for "1234":String - 1234 is the product code I was trying out.
– Emily
23 hours ago
I updated my answer
– igor_rb
23 hours ago
1
Correct use ofexists?
isProduct.where(product_code: @product.product_code).exists?
– Dorian
12 hours ago
|
show 3 more comments
I tried that - unfortunately it gives an error: undefined method `exists?' for "1234":String
– Emily
23 hours ago
what error message it gives? Post it here.
– igor_rb
23 hours ago
undefined method `exists?' for "1234":String - 1234 is the product code I was trying out.
– Emily
23 hours ago
I updated my answer
– igor_rb
23 hours ago
1
Correct use ofexists?
isProduct.where(product_code: @product.product_code).exists?
– Dorian
12 hours ago
I tried that - unfortunately it gives an error: undefined method `exists?' for "1234":String
– Emily
23 hours ago
I tried that - unfortunately it gives an error: undefined method `exists?' for "1234":String
– Emily
23 hours ago
what error message it gives? Post it here.
– igor_rb
23 hours ago
what error message it gives? Post it here.
– igor_rb
23 hours ago
undefined method `exists?' for "1234":String - 1234 is the product code I was trying out.
– Emily
23 hours ago
undefined method `exists?' for "1234":String - 1234 is the product code I was trying out.
– Emily
23 hours ago
I updated my answer
– igor_rb
23 hours ago
I updated my answer
– igor_rb
23 hours ago
1
1
Correct use of
exists?
is Product.where(product_code: @product.product_code).exists?
– Dorian
12 hours ago
Correct use of
exists?
is Product.where(product_code: @product.product_code).exists?
– Dorian
12 hours ago
|
show 3 more comments
up vote
0
down vote
You should note that what you are doing in the controller or adding a standard uniqueness validation to the model will not allow duplicate Products to be created at all.
This will just keep sending the user back to the form:
def create
@product = Product.new(product_params)
if Product.exists?(product_code: @product.product_code)
render 'new'
flash[:error] = "This product already exists."
elsif @product.save
redirect_to @product
else
render 'new'
end
end
If you want to simply warn the user once you can attach a virtual attribute on the model and use it as condition for the validation:
class Product < ApplicationRecord
attribute :dup_warning, :boolean, default: true
validate :lax_product_code_uniquenes
def lax_product_code_uniqueness
if new_record? && !dup_warning && Product.exists(product_code: self.product_code)
errors.add(:product_code, 'is not unique - are you sure?')
self.dup_warning = true
end
end
end
Then add the virtual attribute to the form:
<%= form_with(model: @product) do |f| %>
...
<%= f.hidden_input(:dup_warning) %>
...
<% end %>
And you don't need to really do anything in the controller besides add dup_warning
to the params whitelist.
def create
@product = Product.new(product_params)
if @product.save
redirect_to @product
else
render 'new'
end
end
def product_params
params.require(:product)
.permit(:foo, :bar, :product_code, :dup_warning)
end
add a comment |
up vote
0
down vote
You should note that what you are doing in the controller or adding a standard uniqueness validation to the model will not allow duplicate Products to be created at all.
This will just keep sending the user back to the form:
def create
@product = Product.new(product_params)
if Product.exists?(product_code: @product.product_code)
render 'new'
flash[:error] = "This product already exists."
elsif @product.save
redirect_to @product
else
render 'new'
end
end
If you want to simply warn the user once you can attach a virtual attribute on the model and use it as condition for the validation:
class Product < ApplicationRecord
attribute :dup_warning, :boolean, default: true
validate :lax_product_code_uniquenes
def lax_product_code_uniqueness
if new_record? && !dup_warning && Product.exists(product_code: self.product_code)
errors.add(:product_code, 'is not unique - are you sure?')
self.dup_warning = true
end
end
end
Then add the virtual attribute to the form:
<%= form_with(model: @product) do |f| %>
...
<%= f.hidden_input(:dup_warning) %>
...
<% end %>
And you don't need to really do anything in the controller besides add dup_warning
to the params whitelist.
def create
@product = Product.new(product_params)
if @product.save
redirect_to @product
else
render 'new'
end
end
def product_params
params.require(:product)
.permit(:foo, :bar, :product_code, :dup_warning)
end
add a comment |
up vote
0
down vote
up vote
0
down vote
You should note that what you are doing in the controller or adding a standard uniqueness validation to the model will not allow duplicate Products to be created at all.
This will just keep sending the user back to the form:
def create
@product = Product.new(product_params)
if Product.exists?(product_code: @product.product_code)
render 'new'
flash[:error] = "This product already exists."
elsif @product.save
redirect_to @product
else
render 'new'
end
end
If you want to simply warn the user once you can attach a virtual attribute on the model and use it as condition for the validation:
class Product < ApplicationRecord
attribute :dup_warning, :boolean, default: true
validate :lax_product_code_uniquenes
def lax_product_code_uniqueness
if new_record? && !dup_warning && Product.exists(product_code: self.product_code)
errors.add(:product_code, 'is not unique - are you sure?')
self.dup_warning = true
end
end
end
Then add the virtual attribute to the form:
<%= form_with(model: @product) do |f| %>
...
<%= f.hidden_input(:dup_warning) %>
...
<% end %>
And you don't need to really do anything in the controller besides add dup_warning
to the params whitelist.
def create
@product = Product.new(product_params)
if @product.save
redirect_to @product
else
render 'new'
end
end
def product_params
params.require(:product)
.permit(:foo, :bar, :product_code, :dup_warning)
end
You should note that what you are doing in the controller or adding a standard uniqueness validation to the model will not allow duplicate Products to be created at all.
This will just keep sending the user back to the form:
def create
@product = Product.new(product_params)
if Product.exists?(product_code: @product.product_code)
render 'new'
flash[:error] = "This product already exists."
elsif @product.save
redirect_to @product
else
render 'new'
end
end
If you want to simply warn the user once you can attach a virtual attribute on the model and use it as condition for the validation:
class Product < ApplicationRecord
attribute :dup_warning, :boolean, default: true
validate :lax_product_code_uniquenes
def lax_product_code_uniqueness
if new_record? && !dup_warning && Product.exists(product_code: self.product_code)
errors.add(:product_code, 'is not unique - are you sure?')
self.dup_warning = true
end
end
end
Then add the virtual attribute to the form:
<%= form_with(model: @product) do |f| %>
...
<%= f.hidden_input(:dup_warning) %>
...
<% end %>
And you don't need to really do anything in the controller besides add dup_warning
to the params whitelist.
def create
@product = Product.new(product_params)
if @product.save
redirect_to @product
else
render 'new'
end
end
def product_params
params.require(:product)
.permit(:foo, :bar, :product_code, :dup_warning)
end
answered 17 hours ago


max
43.7k856103
43.7k856103
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53343720%2foffer-options-if-record-already-exists-in-ruby-on-rails%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YvrxUC,1OWKlIuQ jQxQfaEBl3Skk02QkdsoncjwfMNMZGNhn8rE,0LdPiFiAFzkkuCX OT
You can use active record's validations (uniqueness validation) on the product_code field so
@product
won't save if the product_code is already in use and then you can check if@product.errors[:product_code]
show the taken error.– arieljuod
22 hours ago