'Unauthorized operation' when executing Powershell.Invoke()
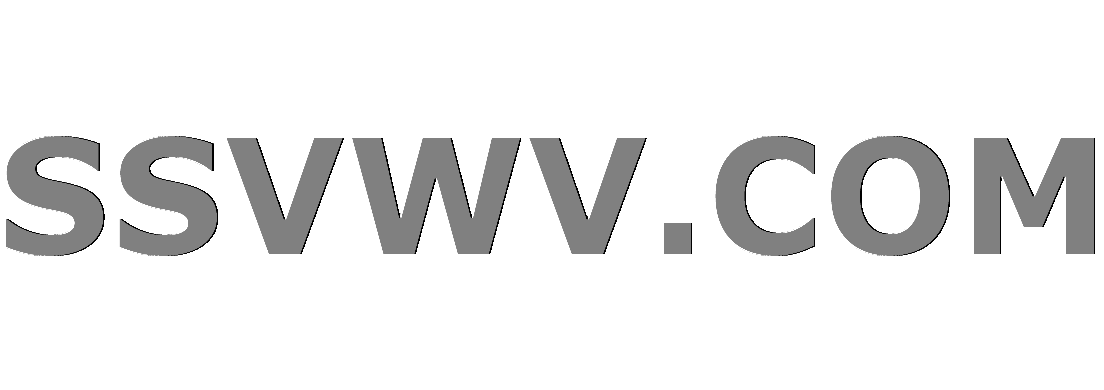
Multi tool use
up vote
0
down vote
favorite
I am running the below code, as referenced from:
https://stackoverflow.com/questions/17067260/invoke-powershell-command-from-c-sharp-with-different-credential
I believe all necessary code is shown below but basically when GetPSResults is called, a PSCredential object is passed in. The issue arises when I try to execute ps.Invoke().
I am running this on localhost, running a Powershell script as a 'generic' user that has been created for use in this program (those credentials are set in the PSCredential object). This user has also been given 'admin' rights on my localhost. That user is trying to retrieve logs from a remote server. Given the error, I am assuming this is some type of permissions issue but I am not extremely familiar with that side of things.
protected override void OnStart(string args)
{
GetLogs newGetLogs = new GetLogs();
PSCredential credential = new PSCredential(@"MYDOMAINMYUSERID", newGetLogs.GetSecurePassword("TESTPASSWORD"));
Collection<PSObject> returnValues = GetPSResults(credential);
}
public static Collection<PSObject> GetPSResults(PSCredential credential, bool throwErrors = true)
{
Collection<PSObject> toReturn = new Collection<PSObject>();
WSManConnectionInfo connectionInfo = new WSManConnectionInfo();
connectionInfo.Credential = credential;
using (Runspace runspace = RunspaceFactory.CreateRunspace(connectionInfo))
{
runspace.Open();
using (PowerShell ps = PowerShell.Create())
{
ps.Runspace = runspace;
ps.AddCommand("get-eventlog");
ps.AddParameter("ComputerName", "MYSERVERNAME");
ps.AddParameter("LogName", "MYLOGNAME");
ps.AddParameter("EntryType", "Error");
ps.AddParameter("Newest", 20);
toReturn = ps.Invoke();
if (throwErrors)
{
if (ps.HadErrors)
{
throw ps.Streams.Error.ElementAt(0).Exception;
}
}
}
runspace.Close();
}
return toReturn;
}
public SecureString GetSecurePassword(string password)
{
var securePassword = new SecureString();
foreach (var c in password)
{
securePassword.AppendChar(c);
}
return securePassword;
}
I am getting this error:
System.Management.Automation.RemoteException: 'Attempted to perform an unauthorized operation.'
Within Visual Studio, I can drill down into the error to see the below image. I see that there are multiple mentions of Registry in these messages... is that a sign of some sort of permissions issue?
I have seen some mention of WinRM but I am not completely clear on the type of updates I would need to make... either in the code or on my user account(s).
c# powershell runspace
add a comment |
up vote
0
down vote
favorite
I am running the below code, as referenced from:
https://stackoverflow.com/questions/17067260/invoke-powershell-command-from-c-sharp-with-different-credential
I believe all necessary code is shown below but basically when GetPSResults is called, a PSCredential object is passed in. The issue arises when I try to execute ps.Invoke().
I am running this on localhost, running a Powershell script as a 'generic' user that has been created for use in this program (those credentials are set in the PSCredential object). This user has also been given 'admin' rights on my localhost. That user is trying to retrieve logs from a remote server. Given the error, I am assuming this is some type of permissions issue but I am not extremely familiar with that side of things.
protected override void OnStart(string args)
{
GetLogs newGetLogs = new GetLogs();
PSCredential credential = new PSCredential(@"MYDOMAINMYUSERID", newGetLogs.GetSecurePassword("TESTPASSWORD"));
Collection<PSObject> returnValues = GetPSResults(credential);
}
public static Collection<PSObject> GetPSResults(PSCredential credential, bool throwErrors = true)
{
Collection<PSObject> toReturn = new Collection<PSObject>();
WSManConnectionInfo connectionInfo = new WSManConnectionInfo();
connectionInfo.Credential = credential;
using (Runspace runspace = RunspaceFactory.CreateRunspace(connectionInfo))
{
runspace.Open();
using (PowerShell ps = PowerShell.Create())
{
ps.Runspace = runspace;
ps.AddCommand("get-eventlog");
ps.AddParameter("ComputerName", "MYSERVERNAME");
ps.AddParameter("LogName", "MYLOGNAME");
ps.AddParameter("EntryType", "Error");
ps.AddParameter("Newest", 20);
toReturn = ps.Invoke();
if (throwErrors)
{
if (ps.HadErrors)
{
throw ps.Streams.Error.ElementAt(0).Exception;
}
}
}
runspace.Close();
}
return toReturn;
}
public SecureString GetSecurePassword(string password)
{
var securePassword = new SecureString();
foreach (var c in password)
{
securePassword.AppendChar(c);
}
return securePassword;
}
I am getting this error:
System.Management.Automation.RemoteException: 'Attempted to perform an unauthorized operation.'
Within Visual Studio, I can drill down into the error to see the below image. I see that there are multiple mentions of Registry in these messages... is that a sign of some sort of permissions issue?
I have seen some mention of WinRM but I am not completely clear on the type of updates I would need to make... either in the code or on my user account(s).
c# powershell runspace
Is there a reason you are calling PowerShell from C# instead of using the .NET methods for reading the Windows Event Log? (likeEventLog.GetEventLogs
– Gabriel Luci
13 hours ago
I assume this is in a Windows service. What credentials is the service running under?
– Gabriel Luci
13 hours ago
@GabrielLuci, great question. This particular app is planned to do some more involved operations, which will be easier with Powershell. Regarding your other question... Yes this is in a Windows service. If I understand you correctly, the service is running under localhost credentials.
– tbcodes33
13 hours ago
Do you mean that it is a local user account? If you run "services.msc" and look at the list of services, there is a "Log on as" column. What is there for this service?
– Gabriel Luci
13 hours ago
And I'd be curious to know what "more involved operations" are easier with PowerShell than through .NET. I have done PowerShell coding in C# before when I had no other choice (like with Microsoft Exchange and Office 365 administration) but it's awful. I have learned to avoid it at all costs.
– Gabriel Luci
13 hours ago
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am running the below code, as referenced from:
https://stackoverflow.com/questions/17067260/invoke-powershell-command-from-c-sharp-with-different-credential
I believe all necessary code is shown below but basically when GetPSResults is called, a PSCredential object is passed in. The issue arises when I try to execute ps.Invoke().
I am running this on localhost, running a Powershell script as a 'generic' user that has been created for use in this program (those credentials are set in the PSCredential object). This user has also been given 'admin' rights on my localhost. That user is trying to retrieve logs from a remote server. Given the error, I am assuming this is some type of permissions issue but I am not extremely familiar with that side of things.
protected override void OnStart(string args)
{
GetLogs newGetLogs = new GetLogs();
PSCredential credential = new PSCredential(@"MYDOMAINMYUSERID", newGetLogs.GetSecurePassword("TESTPASSWORD"));
Collection<PSObject> returnValues = GetPSResults(credential);
}
public static Collection<PSObject> GetPSResults(PSCredential credential, bool throwErrors = true)
{
Collection<PSObject> toReturn = new Collection<PSObject>();
WSManConnectionInfo connectionInfo = new WSManConnectionInfo();
connectionInfo.Credential = credential;
using (Runspace runspace = RunspaceFactory.CreateRunspace(connectionInfo))
{
runspace.Open();
using (PowerShell ps = PowerShell.Create())
{
ps.Runspace = runspace;
ps.AddCommand("get-eventlog");
ps.AddParameter("ComputerName", "MYSERVERNAME");
ps.AddParameter("LogName", "MYLOGNAME");
ps.AddParameter("EntryType", "Error");
ps.AddParameter("Newest", 20);
toReturn = ps.Invoke();
if (throwErrors)
{
if (ps.HadErrors)
{
throw ps.Streams.Error.ElementAt(0).Exception;
}
}
}
runspace.Close();
}
return toReturn;
}
public SecureString GetSecurePassword(string password)
{
var securePassword = new SecureString();
foreach (var c in password)
{
securePassword.AppendChar(c);
}
return securePassword;
}
I am getting this error:
System.Management.Automation.RemoteException: 'Attempted to perform an unauthorized operation.'
Within Visual Studio, I can drill down into the error to see the below image. I see that there are multiple mentions of Registry in these messages... is that a sign of some sort of permissions issue?
I have seen some mention of WinRM but I am not completely clear on the type of updates I would need to make... either in the code or on my user account(s).
c# powershell runspace
I am running the below code, as referenced from:
https://stackoverflow.com/questions/17067260/invoke-powershell-command-from-c-sharp-with-different-credential
I believe all necessary code is shown below but basically when GetPSResults is called, a PSCredential object is passed in. The issue arises when I try to execute ps.Invoke().
I am running this on localhost, running a Powershell script as a 'generic' user that has been created for use in this program (those credentials are set in the PSCredential object). This user has also been given 'admin' rights on my localhost. That user is trying to retrieve logs from a remote server. Given the error, I am assuming this is some type of permissions issue but I am not extremely familiar with that side of things.
protected override void OnStart(string args)
{
GetLogs newGetLogs = new GetLogs();
PSCredential credential = new PSCredential(@"MYDOMAINMYUSERID", newGetLogs.GetSecurePassword("TESTPASSWORD"));
Collection<PSObject> returnValues = GetPSResults(credential);
}
public static Collection<PSObject> GetPSResults(PSCredential credential, bool throwErrors = true)
{
Collection<PSObject> toReturn = new Collection<PSObject>();
WSManConnectionInfo connectionInfo = new WSManConnectionInfo();
connectionInfo.Credential = credential;
using (Runspace runspace = RunspaceFactory.CreateRunspace(connectionInfo))
{
runspace.Open();
using (PowerShell ps = PowerShell.Create())
{
ps.Runspace = runspace;
ps.AddCommand("get-eventlog");
ps.AddParameter("ComputerName", "MYSERVERNAME");
ps.AddParameter("LogName", "MYLOGNAME");
ps.AddParameter("EntryType", "Error");
ps.AddParameter("Newest", 20);
toReturn = ps.Invoke();
if (throwErrors)
{
if (ps.HadErrors)
{
throw ps.Streams.Error.ElementAt(0).Exception;
}
}
}
runspace.Close();
}
return toReturn;
}
public SecureString GetSecurePassword(string password)
{
var securePassword = new SecureString();
foreach (var c in password)
{
securePassword.AppendChar(c);
}
return securePassword;
}
I am getting this error:
System.Management.Automation.RemoteException: 'Attempted to perform an unauthorized operation.'
Within Visual Studio, I can drill down into the error to see the below image. I see that there are multiple mentions of Registry in these messages... is that a sign of some sort of permissions issue?
I have seen some mention of WinRM but I am not completely clear on the type of updates I would need to make... either in the code or on my user account(s).
c# powershell runspace
c# powershell runspace
asked 14 hours ago
tbcodes33
33
33
Is there a reason you are calling PowerShell from C# instead of using the .NET methods for reading the Windows Event Log? (likeEventLog.GetEventLogs
– Gabriel Luci
13 hours ago
I assume this is in a Windows service. What credentials is the service running under?
– Gabriel Luci
13 hours ago
@GabrielLuci, great question. This particular app is planned to do some more involved operations, which will be easier with Powershell. Regarding your other question... Yes this is in a Windows service. If I understand you correctly, the service is running under localhost credentials.
– tbcodes33
13 hours ago
Do you mean that it is a local user account? If you run "services.msc" and look at the list of services, there is a "Log on as" column. What is there for this service?
– Gabriel Luci
13 hours ago
And I'd be curious to know what "more involved operations" are easier with PowerShell than through .NET. I have done PowerShell coding in C# before when I had no other choice (like with Microsoft Exchange and Office 365 administration) but it's awful. I have learned to avoid it at all costs.
– Gabriel Luci
13 hours ago
add a comment |
Is there a reason you are calling PowerShell from C# instead of using the .NET methods for reading the Windows Event Log? (likeEventLog.GetEventLogs
– Gabriel Luci
13 hours ago
I assume this is in a Windows service. What credentials is the service running under?
– Gabriel Luci
13 hours ago
@GabrielLuci, great question. This particular app is planned to do some more involved operations, which will be easier with Powershell. Regarding your other question... Yes this is in a Windows service. If I understand you correctly, the service is running under localhost credentials.
– tbcodes33
13 hours ago
Do you mean that it is a local user account? If you run "services.msc" and look at the list of services, there is a "Log on as" column. What is there for this service?
– Gabriel Luci
13 hours ago
And I'd be curious to know what "more involved operations" are easier with PowerShell than through .NET. I have done PowerShell coding in C# before when I had no other choice (like with Microsoft Exchange and Office 365 administration) but it's awful. I have learned to avoid it at all costs.
– Gabriel Luci
13 hours ago
Is there a reason you are calling PowerShell from C# instead of using the .NET methods for reading the Windows Event Log? (like
EventLog.GetEventLogs
– Gabriel Luci
13 hours ago
Is there a reason you are calling PowerShell from C# instead of using the .NET methods for reading the Windows Event Log? (like
EventLog.GetEventLogs
– Gabriel Luci
13 hours ago
I assume this is in a Windows service. What credentials is the service running under?
– Gabriel Luci
13 hours ago
I assume this is in a Windows service. What credentials is the service running under?
– Gabriel Luci
13 hours ago
@GabrielLuci, great question. This particular app is planned to do some more involved operations, which will be easier with Powershell. Regarding your other question... Yes this is in a Windows service. If I understand you correctly, the service is running under localhost credentials.
– tbcodes33
13 hours ago
@GabrielLuci, great question. This particular app is planned to do some more involved operations, which will be easier with Powershell. Regarding your other question... Yes this is in a Windows service. If I understand you correctly, the service is running under localhost credentials.
– tbcodes33
13 hours ago
Do you mean that it is a local user account? If you run "services.msc" and look at the list of services, there is a "Log on as" column. What is there for this service?
– Gabriel Luci
13 hours ago
Do you mean that it is a local user account? If you run "services.msc" and look at the list of services, there is a "Log on as" column. What is there for this service?
– Gabriel Luci
13 hours ago
And I'd be curious to know what "more involved operations" are easier with PowerShell than through .NET. I have done PowerShell coding in C# before when I had no other choice (like with Microsoft Exchange and Office 365 administration) but it's awful. I have learned to avoid it at all costs.
– Gabriel Luci
13 hours ago
And I'd be curious to know what "more involved operations" are easier with PowerShell than through .NET. I have done PowerShell coding in C# before when I had no other choice (like with Microsoft Exchange and Office 365 administration) but it's awful. I have learned to avoid it at all costs.
– Gabriel Luci
13 hours ago
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53343739%2funauthorized-operation-when-executing-powershell-invoke%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xQF2x945CWYRglTbmQDHlOeqGUnjxAl86daVtcfB21z53MR5I IUkC9,pcTuBi4bR,J9kBC,0o0RY1tfs
Is there a reason you are calling PowerShell from C# instead of using the .NET methods for reading the Windows Event Log? (like
EventLog.GetEventLogs
– Gabriel Luci
13 hours ago
I assume this is in a Windows service. What credentials is the service running under?
– Gabriel Luci
13 hours ago
@GabrielLuci, great question. This particular app is planned to do some more involved operations, which will be easier with Powershell. Regarding your other question... Yes this is in a Windows service. If I understand you correctly, the service is running under localhost credentials.
– tbcodes33
13 hours ago
Do you mean that it is a local user account? If you run "services.msc" and look at the list of services, there is a "Log on as" column. What is there for this service?
– Gabriel Luci
13 hours ago
And I'd be curious to know what "more involved operations" are easier with PowerShell than through .NET. I have done PowerShell coding in C# before when I had no other choice (like with Microsoft Exchange and Office 365 administration) but it's awful. I have learned to avoid it at all costs.
– Gabriel Luci
13 hours ago