Detect if the player is landed on top of a box with rigidbody
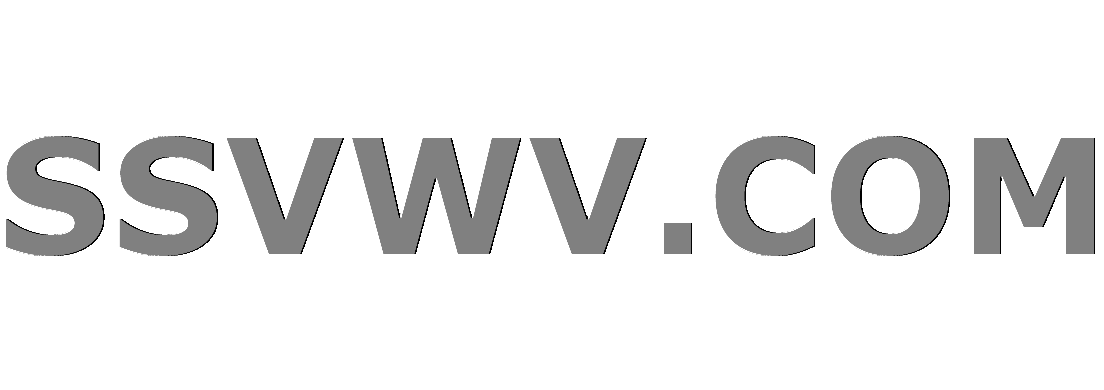
Multi tool use
up vote
2
down vote
favorite
I'm working on a Unity game where the player shoots on cubes to change their weight (it can be positive or negative, last one meaning 'falling' to the roof) and i'm experiencing a problem with rigidbodies, spherecasting and detecting if the player is grounded or not.
When my player is on the ground or on top of any object with a collider, I detect it as 'grounded' using the following function:
if (Physics.SphereCast(transform.position, m_Capsule.radius, Vector3.down, out hitInfo,
((m_Capsule.height / 2f) - m_Capsule.radius) + advancedSettings.groundRoofCheckDistance, Physics.AllLayers, QueryTriggerInteraction.Ignore))
{
m_IsGrounded = true;
}
where advancedSettings.groundRoofCheckDistance
is set to 0.01f.
Until there, everything works fine. But now, when I try to get on top of a cube with a non-kinematic rigidbody, I can't get that boolean to be true.
Here are two captures to illustrate my problem :
In this one, the player is falling on a non-kinematic rigidbody box and the boolean circled in red is m_isGrounded (false):
And here, same but the cube is kinematic and the ground is detected just fine:
I really can't figure out why the rigidbodies do that, or if I have a problem with my ground detection function, so any help is welcome.
Thanks!
PS: I'm using Unity 2018.2.15f1
c# unity3d rigid-bodies
add a comment |
up vote
2
down vote
favorite
I'm working on a Unity game where the player shoots on cubes to change their weight (it can be positive or negative, last one meaning 'falling' to the roof) and i'm experiencing a problem with rigidbodies, spherecasting and detecting if the player is grounded or not.
When my player is on the ground or on top of any object with a collider, I detect it as 'grounded' using the following function:
if (Physics.SphereCast(transform.position, m_Capsule.radius, Vector3.down, out hitInfo,
((m_Capsule.height / 2f) - m_Capsule.radius) + advancedSettings.groundRoofCheckDistance, Physics.AllLayers, QueryTriggerInteraction.Ignore))
{
m_IsGrounded = true;
}
where advancedSettings.groundRoofCheckDistance
is set to 0.01f.
Until there, everything works fine. But now, when I try to get on top of a cube with a non-kinematic rigidbody, I can't get that boolean to be true.
Here are two captures to illustrate my problem :
In this one, the player is falling on a non-kinematic rigidbody box and the boolean circled in red is m_isGrounded (false):
And here, same but the cube is kinematic and the ground is detected just fine:
I really can't figure out why the rigidbodies do that, or if I have a problem with my ground detection function, so any help is welcome.
Thanks!
PS: I'm using Unity 2018.2.15f1
c# unity3d rigid-bodies
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I'm working on a Unity game where the player shoots on cubes to change their weight (it can be positive or negative, last one meaning 'falling' to the roof) and i'm experiencing a problem with rigidbodies, spherecasting and detecting if the player is grounded or not.
When my player is on the ground or on top of any object with a collider, I detect it as 'grounded' using the following function:
if (Physics.SphereCast(transform.position, m_Capsule.radius, Vector3.down, out hitInfo,
((m_Capsule.height / 2f) - m_Capsule.radius) + advancedSettings.groundRoofCheckDistance, Physics.AllLayers, QueryTriggerInteraction.Ignore))
{
m_IsGrounded = true;
}
where advancedSettings.groundRoofCheckDistance
is set to 0.01f.
Until there, everything works fine. But now, when I try to get on top of a cube with a non-kinematic rigidbody, I can't get that boolean to be true.
Here are two captures to illustrate my problem :
In this one, the player is falling on a non-kinematic rigidbody box and the boolean circled in red is m_isGrounded (false):
And here, same but the cube is kinematic and the ground is detected just fine:
I really can't figure out why the rigidbodies do that, or if I have a problem with my ground detection function, so any help is welcome.
Thanks!
PS: I'm using Unity 2018.2.15f1
c# unity3d rigid-bodies
I'm working on a Unity game where the player shoots on cubes to change their weight (it can be positive or negative, last one meaning 'falling' to the roof) and i'm experiencing a problem with rigidbodies, spherecasting and detecting if the player is grounded or not.
When my player is on the ground or on top of any object with a collider, I detect it as 'grounded' using the following function:
if (Physics.SphereCast(transform.position, m_Capsule.radius, Vector3.down, out hitInfo,
((m_Capsule.height / 2f) - m_Capsule.radius) + advancedSettings.groundRoofCheckDistance, Physics.AllLayers, QueryTriggerInteraction.Ignore))
{
m_IsGrounded = true;
}
where advancedSettings.groundRoofCheckDistance
is set to 0.01f.
Until there, everything works fine. But now, when I try to get on top of a cube with a non-kinematic rigidbody, I can't get that boolean to be true.
Here are two captures to illustrate my problem :
In this one, the player is falling on a non-kinematic rigidbody box and the boolean circled in red is m_isGrounded (false):
And here, same but the cube is kinematic and the ground is detected just fine:
I really can't figure out why the rigidbodies do that, or if I have a problem with my ground detection function, so any help is welcome.
Thanks!
PS: I'm using Unity 2018.2.15f1
c# unity3d rigid-bodies
c# unity3d rigid-bodies
edited Nov 19 at 17:25


Programmer
75k1080142
75k1080142
asked Nov 19 at 15:25


Studio Pie
114
114
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
There are many ways to detect if player is grounded or not. If raycast, SphereCast and other ray based detection API are not working properly, try something different. Use the callback functions such as OnCollisionEnter
and OnCollisionExit
with flag. Check that flag in the Update
function.
bool m_IsGrounded;
void OnCollisionEnter(Collision collision)
{
if (collision.collider.CompareTag("Ground"))
m_IsGrounded = true;
}
void OnCollisionExit(Collision collision)
{
if (collision.collider.CompareTag("Ground"))
m_IsGrounded = false;
}
void Update()
{
if (m_IsGrounded)
{
Debug.Log("Grounded");
}
}
Note that this is checking for the "Ground" tag so your ground object must be on the "Ground" tag. You have to manually create this tag from the Editor then change your ground object tag to this.
I tried that, spent the day on adapting rest of my code but it now works. Thanks.
– Studio Pie
Nov 20 at 18:05
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
There are many ways to detect if player is grounded or not. If raycast, SphereCast and other ray based detection API are not working properly, try something different. Use the callback functions such as OnCollisionEnter
and OnCollisionExit
with flag. Check that flag in the Update
function.
bool m_IsGrounded;
void OnCollisionEnter(Collision collision)
{
if (collision.collider.CompareTag("Ground"))
m_IsGrounded = true;
}
void OnCollisionExit(Collision collision)
{
if (collision.collider.CompareTag("Ground"))
m_IsGrounded = false;
}
void Update()
{
if (m_IsGrounded)
{
Debug.Log("Grounded");
}
}
Note that this is checking for the "Ground" tag so your ground object must be on the "Ground" tag. You have to manually create this tag from the Editor then change your ground object tag to this.
I tried that, spent the day on adapting rest of my code but it now works. Thanks.
– Studio Pie
Nov 20 at 18:05
add a comment |
up vote
1
down vote
accepted
There are many ways to detect if player is grounded or not. If raycast, SphereCast and other ray based detection API are not working properly, try something different. Use the callback functions such as OnCollisionEnter
and OnCollisionExit
with flag. Check that flag in the Update
function.
bool m_IsGrounded;
void OnCollisionEnter(Collision collision)
{
if (collision.collider.CompareTag("Ground"))
m_IsGrounded = true;
}
void OnCollisionExit(Collision collision)
{
if (collision.collider.CompareTag("Ground"))
m_IsGrounded = false;
}
void Update()
{
if (m_IsGrounded)
{
Debug.Log("Grounded");
}
}
Note that this is checking for the "Ground" tag so your ground object must be on the "Ground" tag. You have to manually create this tag from the Editor then change your ground object tag to this.
I tried that, spent the day on adapting rest of my code but it now works. Thanks.
– Studio Pie
Nov 20 at 18:05
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
There are many ways to detect if player is grounded or not. If raycast, SphereCast and other ray based detection API are not working properly, try something different. Use the callback functions such as OnCollisionEnter
and OnCollisionExit
with flag. Check that flag in the Update
function.
bool m_IsGrounded;
void OnCollisionEnter(Collision collision)
{
if (collision.collider.CompareTag("Ground"))
m_IsGrounded = true;
}
void OnCollisionExit(Collision collision)
{
if (collision.collider.CompareTag("Ground"))
m_IsGrounded = false;
}
void Update()
{
if (m_IsGrounded)
{
Debug.Log("Grounded");
}
}
Note that this is checking for the "Ground" tag so your ground object must be on the "Ground" tag. You have to manually create this tag from the Editor then change your ground object tag to this.
There are many ways to detect if player is grounded or not. If raycast, SphereCast and other ray based detection API are not working properly, try something different. Use the callback functions such as OnCollisionEnter
and OnCollisionExit
with flag. Check that flag in the Update
function.
bool m_IsGrounded;
void OnCollisionEnter(Collision collision)
{
if (collision.collider.CompareTag("Ground"))
m_IsGrounded = true;
}
void OnCollisionExit(Collision collision)
{
if (collision.collider.CompareTag("Ground"))
m_IsGrounded = false;
}
void Update()
{
if (m_IsGrounded)
{
Debug.Log("Grounded");
}
}
Note that this is checking for the "Ground" tag so your ground object must be on the "Ground" tag. You have to manually create this tag from the Editor then change your ground object tag to this.
answered Nov 19 at 17:34


Programmer
75k1080142
75k1080142
I tried that, spent the day on adapting rest of my code but it now works. Thanks.
– Studio Pie
Nov 20 at 18:05
add a comment |
I tried that, spent the day on adapting rest of my code but it now works. Thanks.
– Studio Pie
Nov 20 at 18:05
I tried that, spent the day on adapting rest of my code but it now works. Thanks.
– Studio Pie
Nov 20 at 18:05
I tried that, spent the day on adapting rest of my code but it now works. Thanks.
– Studio Pie
Nov 20 at 18:05
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53377778%2fdetect-if-the-player-is-landed-on-top-of-a-box-with-rigidbody%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Iy0SSPxP,7VU6Bp,q8Op3ETD 9UE8WA7,hBSpQjr6HWFaZkANV WFFf6bwqEtXGR56,WoEQyDnRI5Tsjk2obqvcQ7nIMJa2WlR4