WPF C# How can I use a common ObservableCollection in two ViewModels?
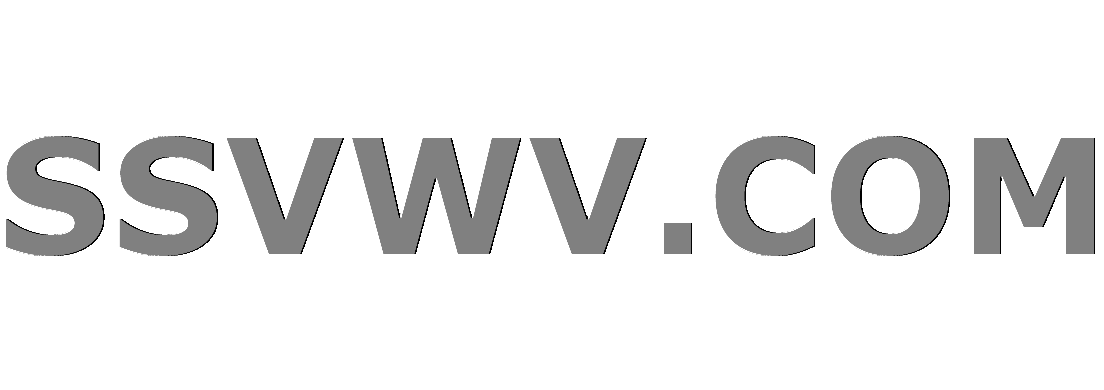
Multi tool use
up vote
0
down vote
favorite
I got a question about how can I use a common ObservableCollection in two ViewModels in WPF C#?
I got two views, first view consist of a a observablecollection of categories used in a combobox in some form. The second view is a window which enable adding, editing and removal of categories. Now I'm retrieving the categories for both views separately, but I want to combine and use a common observable collection, in order to get the new changes when I added, change or remove categories in the second view.
Both Views and viewmodels is controlled by a mainviewmodel:
public viewModel1 viewModel1 { get; set; }
public ViewModel2 ViewModel2 { get; set; }
public MainViewModel()
{
this.InitializeCommands();
this.viewModel1 = new viewModel1();
this.ViewModel2 = new ViewModel2();
this.ViewModel2.OnChangedCategory += (s, e) =>
{
this.viewModel1.Categories = GetCategories();
};
}
ViewModel for View1:
public ObservableCollection<Categories> GetCategories
{
get
{
if (this._getCategories == null)
{
this._getCategories = methods.GetCategories();
}
return this._getCategories ;
}
set
{
if (this._getCategories != value)
{
this._getCategories = value;
this.OnPropertyChanged("GetCategories");
}
}
}
ViewModel for View2:
public ObservableCollection<Categories> GetCategories
{
get
{
if (this._getCategories == null)
{
this._getCategories = methods.GetCategories();
}
return this._getCategories ;
}
set
{
if (this._getCategories != value)
{
this._getCategories = value;
this.OnPropertyChanged("GetCategories");
}
}
}
In theory, they are both identical, just two different observable collection in two viewmodels, I want to use the same observable collection in both views, if it's in the first or second ViewModel doesn't matter. But it makes maybe more sense if it's in viewmodel 2. Let's say if I decide have a common categories observable collection in ViewModel 2, how can Viewmodel 1 use this observable collection from ViewModel 2 and still be in sync upon changes?
How can I do this?
c# wpf xaml
add a comment |
up vote
0
down vote
favorite
I got a question about how can I use a common ObservableCollection in two ViewModels in WPF C#?
I got two views, first view consist of a a observablecollection of categories used in a combobox in some form. The second view is a window which enable adding, editing and removal of categories. Now I'm retrieving the categories for both views separately, but I want to combine and use a common observable collection, in order to get the new changes when I added, change or remove categories in the second view.
Both Views and viewmodels is controlled by a mainviewmodel:
public viewModel1 viewModel1 { get; set; }
public ViewModel2 ViewModel2 { get; set; }
public MainViewModel()
{
this.InitializeCommands();
this.viewModel1 = new viewModel1();
this.ViewModel2 = new ViewModel2();
this.ViewModel2.OnChangedCategory += (s, e) =>
{
this.viewModel1.Categories = GetCategories();
};
}
ViewModel for View1:
public ObservableCollection<Categories> GetCategories
{
get
{
if (this._getCategories == null)
{
this._getCategories = methods.GetCategories();
}
return this._getCategories ;
}
set
{
if (this._getCategories != value)
{
this._getCategories = value;
this.OnPropertyChanged("GetCategories");
}
}
}
ViewModel for View2:
public ObservableCollection<Categories> GetCategories
{
get
{
if (this._getCategories == null)
{
this._getCategories = methods.GetCategories();
}
return this._getCategories ;
}
set
{
if (this._getCategories != value)
{
this._getCategories = value;
this.OnPropertyChanged("GetCategories");
}
}
}
In theory, they are both identical, just two different observable collection in two viewmodels, I want to use the same observable collection in both views, if it's in the first or second ViewModel doesn't matter. But it makes maybe more sense if it's in viewmodel 2. Let's say if I decide have a common categories observable collection in ViewModel 2, how can Viewmodel 1 use this observable collection from ViewModel 2 and still be in sync upon changes?
How can I do this?
c# wpf xaml
2
Create a model class for the data and hand it over to both view models
– Nicolas
Nov 19 at 15:42
Create base class to handle that
– miechooy
Nov 19 at 15:43
@nicolas does updating the class update both viewmodels?
– user6870932
Nov 19 at 15:48
Are the View's DataContext set to MainViewModel.ViewModel1 & MainViewModel.ViewModel2 or directly to the individual ViewModels? Could you not have the ObservableCollection in the MainViewModel and bind to that?
– Coops
Nov 19 at 16:17
@Fredrik Linger If the model class exposes a property holding your data and both view models refer to this property, they all work with the same data (e. g.ObservableCollection
), which means if one of them makes changes, all of them "see" the changes (and get notified in theObservableCollection
case)
– Nicolas
Nov 19 at 20:45
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I got a question about how can I use a common ObservableCollection in two ViewModels in WPF C#?
I got two views, first view consist of a a observablecollection of categories used in a combobox in some form. The second view is a window which enable adding, editing and removal of categories. Now I'm retrieving the categories for both views separately, but I want to combine and use a common observable collection, in order to get the new changes when I added, change or remove categories in the second view.
Both Views and viewmodels is controlled by a mainviewmodel:
public viewModel1 viewModel1 { get; set; }
public ViewModel2 ViewModel2 { get; set; }
public MainViewModel()
{
this.InitializeCommands();
this.viewModel1 = new viewModel1();
this.ViewModel2 = new ViewModel2();
this.ViewModel2.OnChangedCategory += (s, e) =>
{
this.viewModel1.Categories = GetCategories();
};
}
ViewModel for View1:
public ObservableCollection<Categories> GetCategories
{
get
{
if (this._getCategories == null)
{
this._getCategories = methods.GetCategories();
}
return this._getCategories ;
}
set
{
if (this._getCategories != value)
{
this._getCategories = value;
this.OnPropertyChanged("GetCategories");
}
}
}
ViewModel for View2:
public ObservableCollection<Categories> GetCategories
{
get
{
if (this._getCategories == null)
{
this._getCategories = methods.GetCategories();
}
return this._getCategories ;
}
set
{
if (this._getCategories != value)
{
this._getCategories = value;
this.OnPropertyChanged("GetCategories");
}
}
}
In theory, they are both identical, just two different observable collection in two viewmodels, I want to use the same observable collection in both views, if it's in the first or second ViewModel doesn't matter. But it makes maybe more sense if it's in viewmodel 2. Let's say if I decide have a common categories observable collection in ViewModel 2, how can Viewmodel 1 use this observable collection from ViewModel 2 and still be in sync upon changes?
How can I do this?
c# wpf xaml
I got a question about how can I use a common ObservableCollection in two ViewModels in WPF C#?
I got two views, first view consist of a a observablecollection of categories used in a combobox in some form. The second view is a window which enable adding, editing and removal of categories. Now I'm retrieving the categories for both views separately, but I want to combine and use a common observable collection, in order to get the new changes when I added, change or remove categories in the second view.
Both Views and viewmodels is controlled by a mainviewmodel:
public viewModel1 viewModel1 { get; set; }
public ViewModel2 ViewModel2 { get; set; }
public MainViewModel()
{
this.InitializeCommands();
this.viewModel1 = new viewModel1();
this.ViewModel2 = new ViewModel2();
this.ViewModel2.OnChangedCategory += (s, e) =>
{
this.viewModel1.Categories = GetCategories();
};
}
ViewModel for View1:
public ObservableCollection<Categories> GetCategories
{
get
{
if (this._getCategories == null)
{
this._getCategories = methods.GetCategories();
}
return this._getCategories ;
}
set
{
if (this._getCategories != value)
{
this._getCategories = value;
this.OnPropertyChanged("GetCategories");
}
}
}
ViewModel for View2:
public ObservableCollection<Categories> GetCategories
{
get
{
if (this._getCategories == null)
{
this._getCategories = methods.GetCategories();
}
return this._getCategories ;
}
set
{
if (this._getCategories != value)
{
this._getCategories = value;
this.OnPropertyChanged("GetCategories");
}
}
}
In theory, they are both identical, just two different observable collection in two viewmodels, I want to use the same observable collection in both views, if it's in the first or second ViewModel doesn't matter. But it makes maybe more sense if it's in viewmodel 2. Let's say if I decide have a common categories observable collection in ViewModel 2, how can Viewmodel 1 use this observable collection from ViewModel 2 and still be in sync upon changes?
How can I do this?
c# wpf xaml
c# wpf xaml
asked Nov 19 at 15:40
user6870932
2
Create a model class for the data and hand it over to both view models
– Nicolas
Nov 19 at 15:42
Create base class to handle that
– miechooy
Nov 19 at 15:43
@nicolas does updating the class update both viewmodels?
– user6870932
Nov 19 at 15:48
Are the View's DataContext set to MainViewModel.ViewModel1 & MainViewModel.ViewModel2 or directly to the individual ViewModels? Could you not have the ObservableCollection in the MainViewModel and bind to that?
– Coops
Nov 19 at 16:17
@Fredrik Linger If the model class exposes a property holding your data and both view models refer to this property, they all work with the same data (e. g.ObservableCollection
), which means if one of them makes changes, all of them "see" the changes (and get notified in theObservableCollection
case)
– Nicolas
Nov 19 at 20:45
add a comment |
2
Create a model class for the data and hand it over to both view models
– Nicolas
Nov 19 at 15:42
Create base class to handle that
– miechooy
Nov 19 at 15:43
@nicolas does updating the class update both viewmodels?
– user6870932
Nov 19 at 15:48
Are the View's DataContext set to MainViewModel.ViewModel1 & MainViewModel.ViewModel2 or directly to the individual ViewModels? Could you not have the ObservableCollection in the MainViewModel and bind to that?
– Coops
Nov 19 at 16:17
@Fredrik Linger If the model class exposes a property holding your data and both view models refer to this property, they all work with the same data (e. g.ObservableCollection
), which means if one of them makes changes, all of them "see" the changes (and get notified in theObservableCollection
case)
– Nicolas
Nov 19 at 20:45
2
2
Create a model class for the data and hand it over to both view models
– Nicolas
Nov 19 at 15:42
Create a model class for the data and hand it over to both view models
– Nicolas
Nov 19 at 15:42
Create base class to handle that
– miechooy
Nov 19 at 15:43
Create base class to handle that
– miechooy
Nov 19 at 15:43
@nicolas does updating the class update both viewmodels?
– user6870932
Nov 19 at 15:48
@nicolas does updating the class update both viewmodels?
– user6870932
Nov 19 at 15:48
Are the View's DataContext set to MainViewModel.ViewModel1 & MainViewModel.ViewModel2 or directly to the individual ViewModels? Could you not have the ObservableCollection in the MainViewModel and bind to that?
– Coops
Nov 19 at 16:17
Are the View's DataContext set to MainViewModel.ViewModel1 & MainViewModel.ViewModel2 or directly to the individual ViewModels? Could you not have the ObservableCollection in the MainViewModel and bind to that?
– Coops
Nov 19 at 16:17
@Fredrik Linger If the model class exposes a property holding your data and both view models refer to this property, they all work with the same data (e. g.
ObservableCollection
), which means if one of them makes changes, all of them "see" the changes (and get notified in the ObservableCollection
case)– Nicolas
Nov 19 at 20:45
@Fredrik Linger If the model class exposes a property holding your data and both view models refer to this property, they all work with the same data (e. g.
ObservableCollection
), which means if one of them makes changes, all of them "see" the changes (and get notified in the ObservableCollection
case)– Nicolas
Nov 19 at 20:45
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
You could make it a resource and then any code could get at it.
The sample associated with this article has two pairs of usercontrols share two such collections and sounds fairly similar to your requirement.
https://social.technet.microsoft.com/wiki/contents/articles/29859.wpf-tips-bind-to-current-item-of-collection.aspx
Hi, I tested this method, but it doesn't seem like I manage to get the item in the collection to update properly. Can the items in the collection be manipulated directly?
– user6870932
Nov 20 at 9:59
It's an observablecollection, you can do anything to it that you can do to an observablecollection. The sample allows you to edit in one usercontrol and see the results in the other.
– Andy
Nov 20 at 10:12
It's a ListCollectionView? My combobox is using the ListCollectionView or should it use Observable? The tutorial was a bit confusing, could you be kind and provide me some code example?
– user6870932
Nov 20 at 11:00
Working code is here gallery.technet.microsoft.com/…
– Andy
Nov 20 at 11:22
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378081%2fwpf-c-sharp-how-can-i-use-a-common-observablecollection-in-two-viewmodels%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You could make it a resource and then any code could get at it.
The sample associated with this article has two pairs of usercontrols share two such collections and sounds fairly similar to your requirement.
https://social.technet.microsoft.com/wiki/contents/articles/29859.wpf-tips-bind-to-current-item-of-collection.aspx
Hi, I tested this method, but it doesn't seem like I manage to get the item in the collection to update properly. Can the items in the collection be manipulated directly?
– user6870932
Nov 20 at 9:59
It's an observablecollection, you can do anything to it that you can do to an observablecollection. The sample allows you to edit in one usercontrol and see the results in the other.
– Andy
Nov 20 at 10:12
It's a ListCollectionView? My combobox is using the ListCollectionView or should it use Observable? The tutorial was a bit confusing, could you be kind and provide me some code example?
– user6870932
Nov 20 at 11:00
Working code is here gallery.technet.microsoft.com/…
– Andy
Nov 20 at 11:22
add a comment |
up vote
0
down vote
You could make it a resource and then any code could get at it.
The sample associated with this article has two pairs of usercontrols share two such collections and sounds fairly similar to your requirement.
https://social.technet.microsoft.com/wiki/contents/articles/29859.wpf-tips-bind-to-current-item-of-collection.aspx
Hi, I tested this method, but it doesn't seem like I manage to get the item in the collection to update properly. Can the items in the collection be manipulated directly?
– user6870932
Nov 20 at 9:59
It's an observablecollection, you can do anything to it that you can do to an observablecollection. The sample allows you to edit in one usercontrol and see the results in the other.
– Andy
Nov 20 at 10:12
It's a ListCollectionView? My combobox is using the ListCollectionView or should it use Observable? The tutorial was a bit confusing, could you be kind and provide me some code example?
– user6870932
Nov 20 at 11:00
Working code is here gallery.technet.microsoft.com/…
– Andy
Nov 20 at 11:22
add a comment |
up vote
0
down vote
up vote
0
down vote
You could make it a resource and then any code could get at it.
The sample associated with this article has two pairs of usercontrols share two such collections and sounds fairly similar to your requirement.
https://social.technet.microsoft.com/wiki/contents/articles/29859.wpf-tips-bind-to-current-item-of-collection.aspx
You could make it a resource and then any code could get at it.
The sample associated with this article has two pairs of usercontrols share two such collections and sounds fairly similar to your requirement.
https://social.technet.microsoft.com/wiki/contents/articles/29859.wpf-tips-bind-to-current-item-of-collection.aspx
answered Nov 19 at 16:14
Andy
2,6311106
2,6311106
Hi, I tested this method, but it doesn't seem like I manage to get the item in the collection to update properly. Can the items in the collection be manipulated directly?
– user6870932
Nov 20 at 9:59
It's an observablecollection, you can do anything to it that you can do to an observablecollection. The sample allows you to edit in one usercontrol and see the results in the other.
– Andy
Nov 20 at 10:12
It's a ListCollectionView? My combobox is using the ListCollectionView or should it use Observable? The tutorial was a bit confusing, could you be kind and provide me some code example?
– user6870932
Nov 20 at 11:00
Working code is here gallery.technet.microsoft.com/…
– Andy
Nov 20 at 11:22
add a comment |
Hi, I tested this method, but it doesn't seem like I manage to get the item in the collection to update properly. Can the items in the collection be manipulated directly?
– user6870932
Nov 20 at 9:59
It's an observablecollection, you can do anything to it that you can do to an observablecollection. The sample allows you to edit in one usercontrol and see the results in the other.
– Andy
Nov 20 at 10:12
It's a ListCollectionView? My combobox is using the ListCollectionView or should it use Observable? The tutorial was a bit confusing, could you be kind and provide me some code example?
– user6870932
Nov 20 at 11:00
Working code is here gallery.technet.microsoft.com/…
– Andy
Nov 20 at 11:22
Hi, I tested this method, but it doesn't seem like I manage to get the item in the collection to update properly. Can the items in the collection be manipulated directly?
– user6870932
Nov 20 at 9:59
Hi, I tested this method, but it doesn't seem like I manage to get the item in the collection to update properly. Can the items in the collection be manipulated directly?
– user6870932
Nov 20 at 9:59
It's an observablecollection, you can do anything to it that you can do to an observablecollection. The sample allows you to edit in one usercontrol and see the results in the other.
– Andy
Nov 20 at 10:12
It's an observablecollection, you can do anything to it that you can do to an observablecollection. The sample allows you to edit in one usercontrol and see the results in the other.
– Andy
Nov 20 at 10:12
It's a ListCollectionView? My combobox is using the ListCollectionView or should it use Observable? The tutorial was a bit confusing, could you be kind and provide me some code example?
– user6870932
Nov 20 at 11:00
It's a ListCollectionView? My combobox is using the ListCollectionView or should it use Observable? The tutorial was a bit confusing, could you be kind and provide me some code example?
– user6870932
Nov 20 at 11:00
Working code is here gallery.technet.microsoft.com/…
– Andy
Nov 20 at 11:22
Working code is here gallery.technet.microsoft.com/…
– Andy
Nov 20 at 11:22
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378081%2fwpf-c-sharp-how-can-i-use-a-common-observablecollection-in-two-viewmodels%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8wW,IvtBR5,vAShV iOpAgx13gLl5BTzg6HdG
2
Create a model class for the data and hand it over to both view models
– Nicolas
Nov 19 at 15:42
Create base class to handle that
– miechooy
Nov 19 at 15:43
@nicolas does updating the class update both viewmodels?
– user6870932
Nov 19 at 15:48
Are the View's DataContext set to MainViewModel.ViewModel1 & MainViewModel.ViewModel2 or directly to the individual ViewModels? Could you not have the ObservableCollection in the MainViewModel and bind to that?
– Coops
Nov 19 at 16:17
@Fredrik Linger If the model class exposes a property holding your data and both view models refer to this property, they all work with the same data (e. g.
ObservableCollection
), which means if one of them makes changes, all of them "see" the changes (and get notified in theObservableCollection
case)– Nicolas
Nov 19 at 20:45