Print specific keys and values from a deep nested dictionary in python 3.X
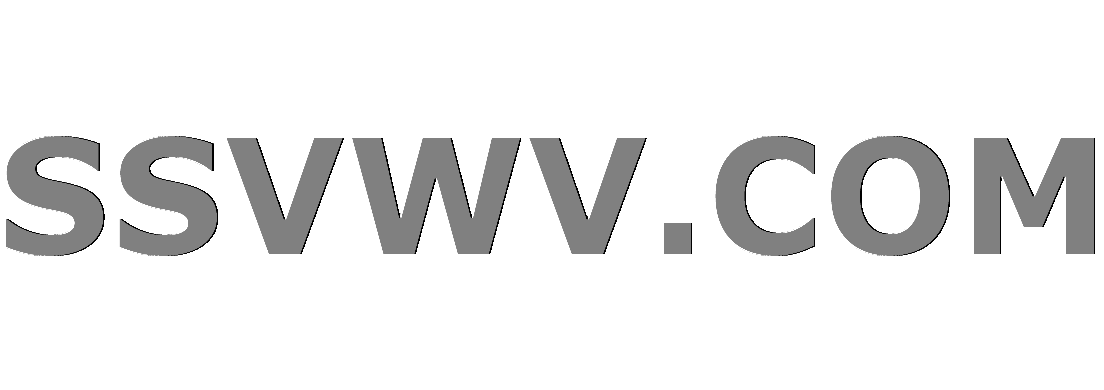
Multi tool use
I am new to python and I've tried to search but can seem to find a sample of what I am trying to accomplish. Any ideas are much appreciated. I am working with a nested dictionary with lots of key and values but I only want to print specific ones using a filtered list variable.
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": true, "is_up": true, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
I would like to a filter through it and choose which keys and values to print out
filtered_list = ['peers', 'remote_id', 'remote_as', 'uptime']
and achieve a out out of
peers: 15.1.1.1
remote_id: 15.1.1.1
remote_as: 65002
uptime: 13002
python python-3.x
add a comment |
I am new to python and I've tried to search but can seem to find a sample of what I am trying to accomplish. Any ideas are much appreciated. I am working with a nested dictionary with lots of key and values but I only want to print specific ones using a filtered list variable.
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": true, "is_up": true, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
I would like to a filter through it and choose which keys and values to print out
filtered_list = ['peers', 'remote_id', 'remote_as', 'uptime']
and achieve a out out of
peers: 15.1.1.1
remote_id: 15.1.1.1
remote_as: 65002
uptime: 13002
python python-3.x
add a comment |
I am new to python and I've tried to search but can seem to find a sample of what I am trying to accomplish. Any ideas are much appreciated. I am working with a nested dictionary with lots of key and values but I only want to print specific ones using a filtered list variable.
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": true, "is_up": true, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
I would like to a filter through it and choose which keys and values to print out
filtered_list = ['peers', 'remote_id', 'remote_as', 'uptime']
and achieve a out out of
peers: 15.1.1.1
remote_id: 15.1.1.1
remote_as: 65002
uptime: 13002
python python-3.x
I am new to python and I've tried to search but can seem to find a sample of what I am trying to accomplish. Any ideas are much appreciated. I am working with a nested dictionary with lots of key and values but I only want to print specific ones using a filtered list variable.
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": true, "is_up": true, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
I would like to a filter through it and choose which keys and values to print out
filtered_list = ['peers', 'remote_id', 'remote_as', 'uptime']
and achieve a out out of
peers: 15.1.1.1
remote_id: 15.1.1.1
remote_as: 65002
uptime: 13002
python python-3.x
python python-3.x
asked Dec 30 '18 at 5:02
JHCTac
685
685
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Use recursion and isinstance
:
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": True, "is_up": True, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
filtered_list = ['peers', 'remote_id', 'remote_as', 'uptime']
def seek_keys(d, key_list):
for k, v in d.items():
if k in key_list:
if isinstance(v, dict):
print(k + ": " + list(v.keys())[0])
else:
print(k + ": " + str(v))
if isinstance(v, dict):
seek_keys(v, key_list)
seek_keys(my_nested_dict, filtered_list)
Note: There is a built in assumption here that if you ever want the "value" from a key whose value is another dictionary, you get the first key.
Hi Jacob I tried that code however I am getting the following error: TypeError: 'dict_keys' object does not support indexing
– JHCTac
Dec 30 '18 at 5:24
1
Nice answer man.
– U9-Forward
Dec 30 '18 at 5:24
1
@JHCTac Now i edited for Jacob
– U9-Forward
Dec 30 '18 at 5:25
Wow that worked perfectly @U9-Forward and @JacobIRR! That is exactly what I was looking for.
– JHCTac
Dec 30 '18 at 5:29
1
@JHCTac Nice to help,
– U9-Forward
Dec 30 '18 at 5:30
add a comment |
On top of @JacobIRR;s answer, I would say you can try caching the recursed data in a flat dict. That way, it will be much faster than recurse every time. You need not worry about memory since the values in the flat dict will simply refer to the original objects in the deep dictionary. I will leave the modification of JacobIRR's code to you :).
New contributor
Karthikeyan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
@JacobIRR has posted a great answer, but since you are attempting to join the matching keys with the its corresponding value, a much shorter solution arises:
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": True, "is_up": True, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
_list = ['peers', 'remote_id', 'remote_as', 'uptime']
def _join(a, b):
return '{}:{}n'.format(a, _keys(b, True) if isinstance(b, dict) else b)
def _keys(_d, flag = False):
return ''.join(_join(a, b) if a in _list else (a+'n' if flag else '')+_keys(b)
for a, b in _d.items())
print(get_keys(my_nested_dict))
Output:
peers:15.1.1.1
remote_id:15.1.1.1
remote_as:65002
uptime:13002
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53975391%2fprint-specific-keys-and-values-from-a-deep-nested-dictionary-in-python-3-x%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Use recursion and isinstance
:
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": True, "is_up": True, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
filtered_list = ['peers', 'remote_id', 'remote_as', 'uptime']
def seek_keys(d, key_list):
for k, v in d.items():
if k in key_list:
if isinstance(v, dict):
print(k + ": " + list(v.keys())[0])
else:
print(k + ": " + str(v))
if isinstance(v, dict):
seek_keys(v, key_list)
seek_keys(my_nested_dict, filtered_list)
Note: There is a built in assumption here that if you ever want the "value" from a key whose value is another dictionary, you get the first key.
Hi Jacob I tried that code however I am getting the following error: TypeError: 'dict_keys' object does not support indexing
– JHCTac
Dec 30 '18 at 5:24
1
Nice answer man.
– U9-Forward
Dec 30 '18 at 5:24
1
@JHCTac Now i edited for Jacob
– U9-Forward
Dec 30 '18 at 5:25
Wow that worked perfectly @U9-Forward and @JacobIRR! That is exactly what I was looking for.
– JHCTac
Dec 30 '18 at 5:29
1
@JHCTac Nice to help,
– U9-Forward
Dec 30 '18 at 5:30
add a comment |
Use recursion and isinstance
:
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": True, "is_up": True, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
filtered_list = ['peers', 'remote_id', 'remote_as', 'uptime']
def seek_keys(d, key_list):
for k, v in d.items():
if k in key_list:
if isinstance(v, dict):
print(k + ": " + list(v.keys())[0])
else:
print(k + ": " + str(v))
if isinstance(v, dict):
seek_keys(v, key_list)
seek_keys(my_nested_dict, filtered_list)
Note: There is a built in assumption here that if you ever want the "value" from a key whose value is another dictionary, you get the first key.
Hi Jacob I tried that code however I am getting the following error: TypeError: 'dict_keys' object does not support indexing
– JHCTac
Dec 30 '18 at 5:24
1
Nice answer man.
– U9-Forward
Dec 30 '18 at 5:24
1
@JHCTac Now i edited for Jacob
– U9-Forward
Dec 30 '18 at 5:25
Wow that worked perfectly @U9-Forward and @JacobIRR! That is exactly what I was looking for.
– JHCTac
Dec 30 '18 at 5:29
1
@JHCTac Nice to help,
– U9-Forward
Dec 30 '18 at 5:30
add a comment |
Use recursion and isinstance
:
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": True, "is_up": True, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
filtered_list = ['peers', 'remote_id', 'remote_as', 'uptime']
def seek_keys(d, key_list):
for k, v in d.items():
if k in key_list:
if isinstance(v, dict):
print(k + ": " + list(v.keys())[0])
else:
print(k + ": " + str(v))
if isinstance(v, dict):
seek_keys(v, key_list)
seek_keys(my_nested_dict, filtered_list)
Note: There is a built in assumption here that if you ever want the "value" from a key whose value is another dictionary, you get the first key.
Use recursion and isinstance
:
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": True, "is_up": True, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
filtered_list = ['peers', 'remote_id', 'remote_as', 'uptime']
def seek_keys(d, key_list):
for k, v in d.items():
if k in key_list:
if isinstance(v, dict):
print(k + ": " + list(v.keys())[0])
else:
print(k + ": " + str(v))
if isinstance(v, dict):
seek_keys(v, key_list)
seek_keys(my_nested_dict, filtered_list)
Note: There is a built in assumption here that if you ever want the "value" from a key whose value is another dictionary, you get the first key.
edited Dec 30 '18 at 5:24


U9-Forward
13k21137
13k21137
answered Dec 30 '18 at 5:10


JacobIRR
3,26021128
3,26021128
Hi Jacob I tried that code however I am getting the following error: TypeError: 'dict_keys' object does not support indexing
– JHCTac
Dec 30 '18 at 5:24
1
Nice answer man.
– U9-Forward
Dec 30 '18 at 5:24
1
@JHCTac Now i edited for Jacob
– U9-Forward
Dec 30 '18 at 5:25
Wow that worked perfectly @U9-Forward and @JacobIRR! That is exactly what I was looking for.
– JHCTac
Dec 30 '18 at 5:29
1
@JHCTac Nice to help,
– U9-Forward
Dec 30 '18 at 5:30
add a comment |
Hi Jacob I tried that code however I am getting the following error: TypeError: 'dict_keys' object does not support indexing
– JHCTac
Dec 30 '18 at 5:24
1
Nice answer man.
– U9-Forward
Dec 30 '18 at 5:24
1
@JHCTac Now i edited for Jacob
– U9-Forward
Dec 30 '18 at 5:25
Wow that worked perfectly @U9-Forward and @JacobIRR! That is exactly what I was looking for.
– JHCTac
Dec 30 '18 at 5:29
1
@JHCTac Nice to help,
– U9-Forward
Dec 30 '18 at 5:30
Hi Jacob I tried that code however I am getting the following error: TypeError: 'dict_keys' object does not support indexing
– JHCTac
Dec 30 '18 at 5:24
Hi Jacob I tried that code however I am getting the following error: TypeError: 'dict_keys' object does not support indexing
– JHCTac
Dec 30 '18 at 5:24
1
1
Nice answer man.
– U9-Forward
Dec 30 '18 at 5:24
Nice answer man.
– U9-Forward
Dec 30 '18 at 5:24
1
1
@JHCTac Now i edited for Jacob
– U9-Forward
Dec 30 '18 at 5:25
@JHCTac Now i edited for Jacob
– U9-Forward
Dec 30 '18 at 5:25
Wow that worked perfectly @U9-Forward and @JacobIRR! That is exactly what I was looking for.
– JHCTac
Dec 30 '18 at 5:29
Wow that worked perfectly @U9-Forward and @JacobIRR! That is exactly what I was looking for.
– JHCTac
Dec 30 '18 at 5:29
1
1
@JHCTac Nice to help,
– U9-Forward
Dec 30 '18 at 5:30
@JHCTac Nice to help,
– U9-Forward
Dec 30 '18 at 5:30
add a comment |
On top of @JacobIRR;s answer, I would say you can try caching the recursed data in a flat dict. That way, it will be much faster than recurse every time. You need not worry about memory since the values in the flat dict will simply refer to the original objects in the deep dictionary. I will leave the modification of JacobIRR's code to you :).
New contributor
Karthikeyan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
On top of @JacobIRR;s answer, I would say you can try caching the recursed data in a flat dict. That way, it will be much faster than recurse every time. You need not worry about memory since the values in the flat dict will simply refer to the original objects in the deep dictionary. I will leave the modification of JacobIRR's code to you :).
New contributor
Karthikeyan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
On top of @JacobIRR;s answer, I would say you can try caching the recursed data in a flat dict. That way, it will be much faster than recurse every time. You need not worry about memory since the values in the flat dict will simply refer to the original objects in the deep dictionary. I will leave the modification of JacobIRR's code to you :).
New contributor
Karthikeyan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
On top of @JacobIRR;s answer, I would say you can try caching the recursed data in a flat dict. That way, it will be much faster than recurse every time. You need not worry about memory since the values in the flat dict will simply refer to the original objects in the deep dictionary. I will leave the modification of JacobIRR's code to you :).
New contributor
Karthikeyan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Karthikeyan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered 2 days ago
Karthikeyan
1
1
New contributor
Karthikeyan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Karthikeyan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Karthikeyan is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
@JacobIRR has posted a great answer, but since you are attempting to join the matching keys with the its corresponding value, a much shorter solution arises:
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": True, "is_up": True, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
_list = ['peers', 'remote_id', 'remote_as', 'uptime']
def _join(a, b):
return '{}:{}n'.format(a, _keys(b, True) if isinstance(b, dict) else b)
def _keys(_d, flag = False):
return ''.join(_join(a, b) if a in _list else (a+'n' if flag else '')+_keys(b)
for a, b in _d.items())
print(get_keys(my_nested_dict))
Output:
peers:15.1.1.1
remote_id:15.1.1.1
remote_as:65002
uptime:13002
add a comment |
@JacobIRR has posted a great answer, but since you are attempting to join the matching keys with the its corresponding value, a much shorter solution arises:
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": True, "is_up": True, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
_list = ['peers', 'remote_id', 'remote_as', 'uptime']
def _join(a, b):
return '{}:{}n'.format(a, _keys(b, True) if isinstance(b, dict) else b)
def _keys(_d, flag = False):
return ''.join(_join(a, b) if a in _list else (a+'n' if flag else '')+_keys(b)
for a, b in _d.items())
print(get_keys(my_nested_dict))
Output:
peers:15.1.1.1
remote_id:15.1.1.1
remote_as:65002
uptime:13002
add a comment |
@JacobIRR has posted a great answer, but since you are attempting to join the matching keys with the its corresponding value, a much shorter solution arises:
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": True, "is_up": True, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
_list = ['peers', 'remote_id', 'remote_as', 'uptime']
def _join(a, b):
return '{}:{}n'.format(a, _keys(b, True) if isinstance(b, dict) else b)
def _keys(_d, flag = False):
return ''.join(_join(a, b) if a in _list else (a+'n' if flag else '')+_keys(b)
for a, b in _d.items())
print(get_keys(my_nested_dict))
Output:
peers:15.1.1.1
remote_id:15.1.1.1
remote_as:65002
uptime:13002
@JacobIRR has posted a great answer, but since you are attempting to join the matching keys with the its corresponding value, a much shorter solution arises:
my_nested_dict = {"global": {"peers": {"15.1.1.1": {"remote_id": "15.1.1.1", "address_family": {"ipv4": {"sent_prefixes": 1, "received_prefixes": 4, "accepted_prefixes": 4}}, "remote_as": 65002, "uptime": 13002, "is_enabled": True, "is_up": True, "description": "== R3 BGP Neighbor ==", "local_as": 65002}}, "router_id": "15.1.1.2"}}
_list = ['peers', 'remote_id', 'remote_as', 'uptime']
def _join(a, b):
return '{}:{}n'.format(a, _keys(b, True) if isinstance(b, dict) else b)
def _keys(_d, flag = False):
return ''.join(_join(a, b) if a in _list else (a+'n' if flag else '')+_keys(b)
for a, b in _d.items())
print(get_keys(my_nested_dict))
Output:
peers:15.1.1.1
remote_id:15.1.1.1
remote_as:65002
uptime:13002
answered 2 days ago


Ajax1234
40.3k42653
40.3k42653
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53975391%2fprint-specific-keys-and-values-from-a-deep-nested-dictionary-in-python-3-x%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VFYw5E2eY7orLNbwaiUH14qrOJV31Mcn5CxRWCt047YZzgQBSg,wyMVuQRLsa