Change the color of an option when selected - JavaScript
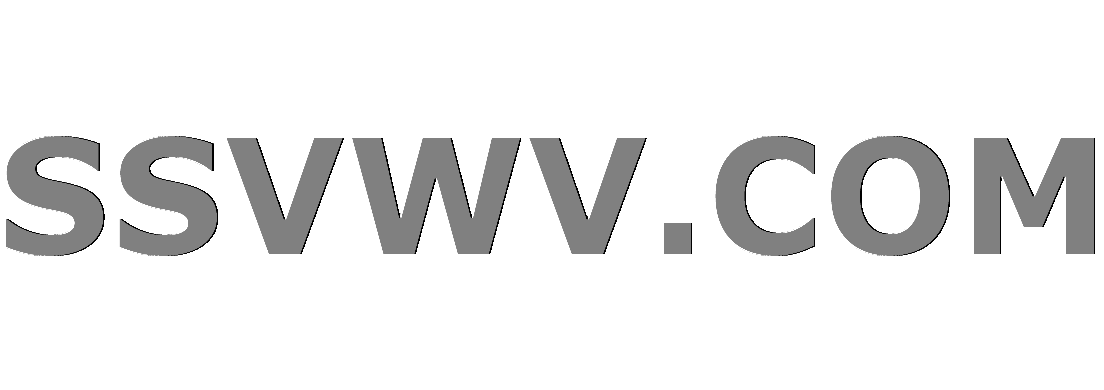
Multi tool use
up vote
0
down vote
favorite
I want an option to change color when selected by a user. For Example: a user selects the red option then a function would run that would change the color red. If the user then selected green then it would change green. etc.
<select onchange="changeColor();" class="color" id="rgb">
<option id="red" value="Red">Red</option>
<option id="green" value="Green">Green</option>
<option id="blue" value="Blue">Blue</option>
</select>
I'm started with the function below but im not sure where I went wrong.
function changeColor() {
var red = document.getElementById('red');
var green = document.getElementById('green');
var blue = document.getElementById('blue');
if(event.target.value == red) {
red.style.color = "red";
} else if(event.target.value == green) {
green.style.color = "green";
} else if(event.target.value == blue) {
blue.style.color = "blue";
} else {
alert("There was an error!");
}
};
javascript dom javascript-events html-select onchange
add a comment |
up vote
0
down vote
favorite
I want an option to change color when selected by a user. For Example: a user selects the red option then a function would run that would change the color red. If the user then selected green then it would change green. etc.
<select onchange="changeColor();" class="color" id="rgb">
<option id="red" value="Red">Red</option>
<option id="green" value="Green">Green</option>
<option id="blue" value="Blue">Blue</option>
</select>
I'm started with the function below but im not sure where I went wrong.
function changeColor() {
var red = document.getElementById('red');
var green = document.getElementById('green');
var blue = document.getElementById('blue');
if(event.target.value == red) {
red.style.color = "red";
} else if(event.target.value == green) {
green.style.color = "green";
} else if(event.target.value == blue) {
blue.style.color = "blue";
} else {
alert("There was an error!");
}
};
javascript dom javascript-events html-select onchange
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I want an option to change color when selected by a user. For Example: a user selects the red option then a function would run that would change the color red. If the user then selected green then it would change green. etc.
<select onchange="changeColor();" class="color" id="rgb">
<option id="red" value="Red">Red</option>
<option id="green" value="Green">Green</option>
<option id="blue" value="Blue">Blue</option>
</select>
I'm started with the function below but im not sure where I went wrong.
function changeColor() {
var red = document.getElementById('red');
var green = document.getElementById('green');
var blue = document.getElementById('blue');
if(event.target.value == red) {
red.style.color = "red";
} else if(event.target.value == green) {
green.style.color = "green";
} else if(event.target.value == blue) {
blue.style.color = "blue";
} else {
alert("There was an error!");
}
};
javascript dom javascript-events html-select onchange
I want an option to change color when selected by a user. For Example: a user selects the red option then a function would run that would change the color red. If the user then selected green then it would change green. etc.
<select onchange="changeColor();" class="color" id="rgb">
<option id="red" value="Red">Red</option>
<option id="green" value="Green">Green</option>
<option id="blue" value="Blue">Blue</option>
</select>
I'm started with the function below but im not sure where I went wrong.
function changeColor() {
var red = document.getElementById('red');
var green = document.getElementById('green');
var blue = document.getElementById('blue');
if(event.target.value == red) {
red.style.color = "red";
} else if(event.target.value == green) {
green.style.color = "green";
} else if(event.target.value == blue) {
blue.style.color = "blue";
} else {
alert("There was an error!");
}
};
javascript dom javascript-events html-select onchange
javascript dom javascript-events html-select onchange
edited Nov 18 at 0:15
asked Nov 18 at 0:10
Gorbles
12
12
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
1
down vote
It looks like you haven't added an 'event' parameter to your function. Try this:
function changeColor(event) {
var red = document.getElementById(red);
var green = document.getElementById(green);
var blue = document.getElementById(blue);
if (event.target.value == red) {
red.style.color = "red";
} else if (event.target.value == green) {
green.style.color = "green";
} else if (event.target.value == blue) {
blue.style.color = "blue";
} else {
alert("There was an error!");
}};
that's only 1/2 of how to add it
– charlietfl
Nov 18 at 0:41
add a comment |
up vote
1
down vote
Try this. When you select an option you will recieve the <select>
element in colorParam
. If you select the first option, you will get Red
in colorParam.value
, but you are using IDs in lowerCase, so you can use toLowerCase()
function to convert it. Then select the option
element and apply styles.
function changeColor(colorParam) {
let color = colorParam.value.toLowerCase();
var optionElement = document.getElementById(color);
optionElement.style.color = color;
};
<select onchange="changeColor(this);" class="color" id="rgb">
<option id="red" value="Red">Red</option>
<option id="green" value="Green">Green</option>
<option id="blue" value="Blue">Blue</option>
<option id="white" value="White">White</option>
<option id="pink" value="Pink">Pink</option>
</select>
add a comment |
up vote
0
down vote
I'm not sure you can style an <option>
element. You can style the <select>
element though. However, color
doesn't seem to be a property you can change, background-color
is.
If that's all you need you could as well inline the javascript code in the onchange
handler attribute. You would need to set the values of the <option>
elements to valid css colours.
<select onchange="this.style.backgroundColor=this.value">
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
It looks like you haven't added an 'event' parameter to your function. Try this:
function changeColor(event) {
var red = document.getElementById(red);
var green = document.getElementById(green);
var blue = document.getElementById(blue);
if (event.target.value == red) {
red.style.color = "red";
} else if (event.target.value == green) {
green.style.color = "green";
} else if (event.target.value == blue) {
blue.style.color = "blue";
} else {
alert("There was an error!");
}};
that's only 1/2 of how to add it
– charlietfl
Nov 18 at 0:41
add a comment |
up vote
1
down vote
It looks like you haven't added an 'event' parameter to your function. Try this:
function changeColor(event) {
var red = document.getElementById(red);
var green = document.getElementById(green);
var blue = document.getElementById(blue);
if (event.target.value == red) {
red.style.color = "red";
} else if (event.target.value == green) {
green.style.color = "green";
} else if (event.target.value == blue) {
blue.style.color = "blue";
} else {
alert("There was an error!");
}};
that's only 1/2 of how to add it
– charlietfl
Nov 18 at 0:41
add a comment |
up vote
1
down vote
up vote
1
down vote
It looks like you haven't added an 'event' parameter to your function. Try this:
function changeColor(event) {
var red = document.getElementById(red);
var green = document.getElementById(green);
var blue = document.getElementById(blue);
if (event.target.value == red) {
red.style.color = "red";
} else if (event.target.value == green) {
green.style.color = "green";
} else if (event.target.value == blue) {
blue.style.color = "blue";
} else {
alert("There was an error!");
}};
It looks like you haven't added an 'event' parameter to your function. Try this:
function changeColor(event) {
var red = document.getElementById(red);
var green = document.getElementById(green);
var blue = document.getElementById(blue);
if (event.target.value == red) {
red.style.color = "red";
} else if (event.target.value == green) {
green.style.color = "green";
} else if (event.target.value == blue) {
blue.style.color = "blue";
} else {
alert("There was an error!");
}};
answered Nov 18 at 0:16
nevace
213
213
that's only 1/2 of how to add it
– charlietfl
Nov 18 at 0:41
add a comment |
that's only 1/2 of how to add it
– charlietfl
Nov 18 at 0:41
that's only 1/2 of how to add it
– charlietfl
Nov 18 at 0:41
that's only 1/2 of how to add it
– charlietfl
Nov 18 at 0:41
add a comment |
up vote
1
down vote
Try this. When you select an option you will recieve the <select>
element in colorParam
. If you select the first option, you will get Red
in colorParam.value
, but you are using IDs in lowerCase, so you can use toLowerCase()
function to convert it. Then select the option
element and apply styles.
function changeColor(colorParam) {
let color = colorParam.value.toLowerCase();
var optionElement = document.getElementById(color);
optionElement.style.color = color;
};
<select onchange="changeColor(this);" class="color" id="rgb">
<option id="red" value="Red">Red</option>
<option id="green" value="Green">Green</option>
<option id="blue" value="Blue">Blue</option>
<option id="white" value="White">White</option>
<option id="pink" value="Pink">Pink</option>
</select>
add a comment |
up vote
1
down vote
Try this. When you select an option you will recieve the <select>
element in colorParam
. If you select the first option, you will get Red
in colorParam.value
, but you are using IDs in lowerCase, so you can use toLowerCase()
function to convert it. Then select the option
element and apply styles.
function changeColor(colorParam) {
let color = colorParam.value.toLowerCase();
var optionElement = document.getElementById(color);
optionElement.style.color = color;
};
<select onchange="changeColor(this);" class="color" id="rgb">
<option id="red" value="Red">Red</option>
<option id="green" value="Green">Green</option>
<option id="blue" value="Blue">Blue</option>
<option id="white" value="White">White</option>
<option id="pink" value="Pink">Pink</option>
</select>
add a comment |
up vote
1
down vote
up vote
1
down vote
Try this. When you select an option you will recieve the <select>
element in colorParam
. If you select the first option, you will get Red
in colorParam.value
, but you are using IDs in lowerCase, so you can use toLowerCase()
function to convert it. Then select the option
element and apply styles.
function changeColor(colorParam) {
let color = colorParam.value.toLowerCase();
var optionElement = document.getElementById(color);
optionElement.style.color = color;
};
<select onchange="changeColor(this);" class="color" id="rgb">
<option id="red" value="Red">Red</option>
<option id="green" value="Green">Green</option>
<option id="blue" value="Blue">Blue</option>
<option id="white" value="White">White</option>
<option id="pink" value="Pink">Pink</option>
</select>
Try this. When you select an option you will recieve the <select>
element in colorParam
. If you select the first option, you will get Red
in colorParam.value
, but you are using IDs in lowerCase, so you can use toLowerCase()
function to convert it. Then select the option
element and apply styles.
function changeColor(colorParam) {
let color = colorParam.value.toLowerCase();
var optionElement = document.getElementById(color);
optionElement.style.color = color;
};
<select onchange="changeColor(this);" class="color" id="rgb">
<option id="red" value="Red">Red</option>
<option id="green" value="Green">Green</option>
<option id="blue" value="Blue">Blue</option>
<option id="white" value="White">White</option>
<option id="pink" value="Pink">Pink</option>
</select>
function changeColor(colorParam) {
let color = colorParam.value.toLowerCase();
var optionElement = document.getElementById(color);
optionElement.style.color = color;
};
<select onchange="changeColor(this);" class="color" id="rgb">
<option id="red" value="Red">Red</option>
<option id="green" value="Green">Green</option>
<option id="blue" value="Blue">Blue</option>
<option id="white" value="White">White</option>
<option id="pink" value="Pink">Pink</option>
</select>
function changeColor(colorParam) {
let color = colorParam.value.toLowerCase();
var optionElement = document.getElementById(color);
optionElement.style.color = color;
};
<select onchange="changeColor(this);" class="color" id="rgb">
<option id="red" value="Red">Red</option>
<option id="green" value="Green">Green</option>
<option id="blue" value="Blue">Blue</option>
<option id="white" value="White">White</option>
<option id="pink" value="Pink">Pink</option>
</select>
edited Nov 18 at 0:45
answered Nov 18 at 0:19
eag845
424410
424410
add a comment |
add a comment |
up vote
0
down vote
I'm not sure you can style an <option>
element. You can style the <select>
element though. However, color
doesn't seem to be a property you can change, background-color
is.
If that's all you need you could as well inline the javascript code in the onchange
handler attribute. You would need to set the values of the <option>
elements to valid css colours.
<select onchange="this.style.backgroundColor=this.value">
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
add a comment |
up vote
0
down vote
I'm not sure you can style an <option>
element. You can style the <select>
element though. However, color
doesn't seem to be a property you can change, background-color
is.
If that's all you need you could as well inline the javascript code in the onchange
handler attribute. You would need to set the values of the <option>
elements to valid css colours.
<select onchange="this.style.backgroundColor=this.value">
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
add a comment |
up vote
0
down vote
up vote
0
down vote
I'm not sure you can style an <option>
element. You can style the <select>
element though. However, color
doesn't seem to be a property you can change, background-color
is.
If that's all you need you could as well inline the javascript code in the onchange
handler attribute. You would need to set the values of the <option>
elements to valid css colours.
<select onchange="this.style.backgroundColor=this.value">
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
I'm not sure you can style an <option>
element. You can style the <select>
element though. However, color
doesn't seem to be a property you can change, background-color
is.
If that's all you need you could as well inline the javascript code in the onchange
handler attribute. You would need to set the values of the <option>
elements to valid css colours.
<select onchange="this.style.backgroundColor=this.value">
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
<select onchange="this.style.backgroundColor=this.value">
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
<select onchange="this.style.backgroundColor=this.value">
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
edited Nov 19 at 8:33
answered Nov 18 at 0:37
customcommander
715313
715313
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53356775%2fchange-the-color-of-an-option-when-selected-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
F,r yIzkETCTkdGxs