Focus on TextField not working when button pressed in Dialog - Material UI
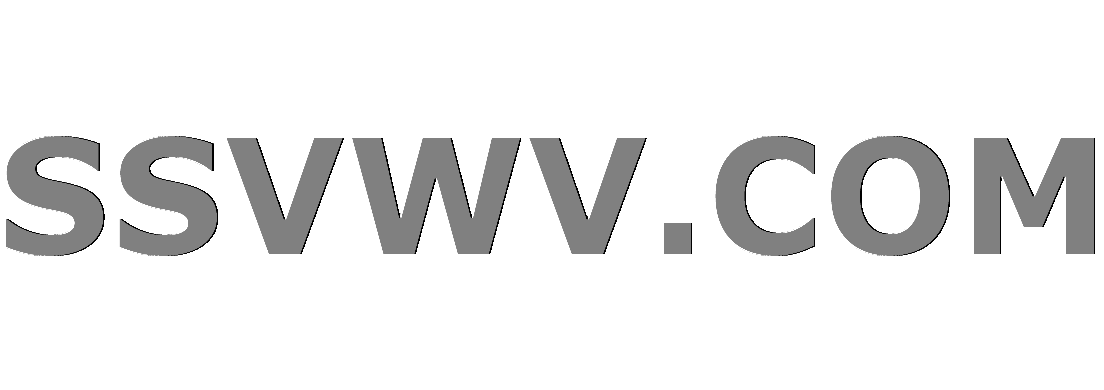
Multi tool use
My goal is to set focus on a Material UI TextField after closing a Dialog by clicking a button in this Dialog.
The following piece of code works when I call it from a button that is not in a dialog component:
focusTitle = () => {
this.setState({ isOpen: false });
this.myRef.current.focus();
};
Code of button:
<Button onClick={this.focusTitle} />
Code of textfield I want to focus on:
<TextField
inputRef={this.myRef}
label="Title"
id="title"
multiline
rowsMax="4"
value={this.state.title}
autoFocus={true}
className={classes.title}
helperText="Enter a catchy title"
onChange={e => this.onTitleChange(e.target.value)}
/>
But as soon as I try to call the focusTitle() from a button within a dialog it does not trigger the focus.
Code of dialog:
<Dialog
open={this.state.isOpen}
onClose={this.focusTitle}
aria-labelledby="alert-dialog-title"
aria-describedby="alert-dialog-description"
>
<DialogTitle id="alert-dialog-title">{"Warning"}</DialogTitle>
<DialogContent>
<DialogContentText id="alert-dialog-description">
Warning Message!
</DialogContentText>
</DialogContent>
<DialogActions>
<Button onClick={this.focusTitle} color="primary">
OK
</Button>
</DialogActions>
</Dialog>
Anyone has an idea why my .focus() is not working in the case of the dialog? If I log this.refs.myRef in both cases it shows the exact same result.
Thank you!
javascript reactjs material-ui
add a comment |
My goal is to set focus on a Material UI TextField after closing a Dialog by clicking a button in this Dialog.
The following piece of code works when I call it from a button that is not in a dialog component:
focusTitle = () => {
this.setState({ isOpen: false });
this.myRef.current.focus();
};
Code of button:
<Button onClick={this.focusTitle} />
Code of textfield I want to focus on:
<TextField
inputRef={this.myRef}
label="Title"
id="title"
multiline
rowsMax="4"
value={this.state.title}
autoFocus={true}
className={classes.title}
helperText="Enter a catchy title"
onChange={e => this.onTitleChange(e.target.value)}
/>
But as soon as I try to call the focusTitle() from a button within a dialog it does not trigger the focus.
Code of dialog:
<Dialog
open={this.state.isOpen}
onClose={this.focusTitle}
aria-labelledby="alert-dialog-title"
aria-describedby="alert-dialog-description"
>
<DialogTitle id="alert-dialog-title">{"Warning"}</DialogTitle>
<DialogContent>
<DialogContentText id="alert-dialog-description">
Warning Message!
</DialogContentText>
</DialogContent>
<DialogActions>
<Button onClick={this.focusTitle} color="primary">
OK
</Button>
</DialogActions>
</Dialog>
Anyone has an idea why my .focus() is not working in the case of the dialog? If I log this.refs.myRef in both cases it shows the exact same result.
Thank you!
javascript reactjs material-ui
add a comment |
My goal is to set focus on a Material UI TextField after closing a Dialog by clicking a button in this Dialog.
The following piece of code works when I call it from a button that is not in a dialog component:
focusTitle = () => {
this.setState({ isOpen: false });
this.myRef.current.focus();
};
Code of button:
<Button onClick={this.focusTitle} />
Code of textfield I want to focus on:
<TextField
inputRef={this.myRef}
label="Title"
id="title"
multiline
rowsMax="4"
value={this.state.title}
autoFocus={true}
className={classes.title}
helperText="Enter a catchy title"
onChange={e => this.onTitleChange(e.target.value)}
/>
But as soon as I try to call the focusTitle() from a button within a dialog it does not trigger the focus.
Code of dialog:
<Dialog
open={this.state.isOpen}
onClose={this.focusTitle}
aria-labelledby="alert-dialog-title"
aria-describedby="alert-dialog-description"
>
<DialogTitle id="alert-dialog-title">{"Warning"}</DialogTitle>
<DialogContent>
<DialogContentText id="alert-dialog-description">
Warning Message!
</DialogContentText>
</DialogContent>
<DialogActions>
<Button onClick={this.focusTitle} color="primary">
OK
</Button>
</DialogActions>
</Dialog>
Anyone has an idea why my .focus() is not working in the case of the dialog? If I log this.refs.myRef in both cases it shows the exact same result.
Thank you!
javascript reactjs material-ui
My goal is to set focus on a Material UI TextField after closing a Dialog by clicking a button in this Dialog.
The following piece of code works when I call it from a button that is not in a dialog component:
focusTitle = () => {
this.setState({ isOpen: false });
this.myRef.current.focus();
};
Code of button:
<Button onClick={this.focusTitle} />
Code of textfield I want to focus on:
<TextField
inputRef={this.myRef}
label="Title"
id="title"
multiline
rowsMax="4"
value={this.state.title}
autoFocus={true}
className={classes.title}
helperText="Enter a catchy title"
onChange={e => this.onTitleChange(e.target.value)}
/>
But as soon as I try to call the focusTitle() from a button within a dialog it does not trigger the focus.
Code of dialog:
<Dialog
open={this.state.isOpen}
onClose={this.focusTitle}
aria-labelledby="alert-dialog-title"
aria-describedby="alert-dialog-description"
>
<DialogTitle id="alert-dialog-title">{"Warning"}</DialogTitle>
<DialogContent>
<DialogContentText id="alert-dialog-description">
Warning Message!
</DialogContentText>
</DialogContent>
<DialogActions>
<Button onClick={this.focusTitle} color="primary">
OK
</Button>
</DialogActions>
</Dialog>
Anyone has an idea why my .focus() is not working in the case of the dialog? If I log this.refs.myRef in both cases it shows the exact same result.
Thank you!
javascript reactjs material-ui
javascript reactjs material-ui
edited Nov 21 '18 at 9:04
Nicnel
asked Nov 20 '18 at 19:56
NicnelNicnel
185
185
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Your dialog probably has a closing animation. As long as the animation is running, the focus()
function will not be called correctly.
To prevent this, create a callback for the animation or a timeout for the duration of the animation, to trigger the focus()
afterwards. Like this:
focusTitle = () => {
this.setState({ isOpen: false });
setTimeout(
function() {
this.myRef.current.focus();
}
.bind(this),
500 // Change to duration of the animation
};
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53400609%2ffocus-on-textfield-not-working-when-button-pressed-in-dialog-material-ui%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your dialog probably has a closing animation. As long as the animation is running, the focus()
function will not be called correctly.
To prevent this, create a callback for the animation or a timeout for the duration of the animation, to trigger the focus()
afterwards. Like this:
focusTitle = () => {
this.setState({ isOpen: false });
setTimeout(
function() {
this.myRef.current.focus();
}
.bind(this),
500 // Change to duration of the animation
};
add a comment |
Your dialog probably has a closing animation. As long as the animation is running, the focus()
function will not be called correctly.
To prevent this, create a callback for the animation or a timeout for the duration of the animation, to trigger the focus()
afterwards. Like this:
focusTitle = () => {
this.setState({ isOpen: false });
setTimeout(
function() {
this.myRef.current.focus();
}
.bind(this),
500 // Change to duration of the animation
};
add a comment |
Your dialog probably has a closing animation. As long as the animation is running, the focus()
function will not be called correctly.
To prevent this, create a callback for the animation or a timeout for the duration of the animation, to trigger the focus()
afterwards. Like this:
focusTitle = () => {
this.setState({ isOpen: false });
setTimeout(
function() {
this.myRef.current.focus();
}
.bind(this),
500 // Change to duration of the animation
};
Your dialog probably has a closing animation. As long as the animation is running, the focus()
function will not be called correctly.
To prevent this, create a callback for the animation or a timeout for the duration of the animation, to trigger the focus()
afterwards. Like this:
focusTitle = () => {
this.setState({ isOpen: false });
setTimeout(
function() {
this.myRef.current.focus();
}
.bind(this),
500 // Change to duration of the animation
};
answered Nov 21 '18 at 9:34


Thomas van BroekhovenThomas van Broekhoven
474312
474312
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53400609%2ffocus-on-textfield-not-working-when-button-pressed-in-dialog-material-ui%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ADJ6EdE8izb6h8