How to remove the white space in a string or make a regular expression that would accept all type of valid...
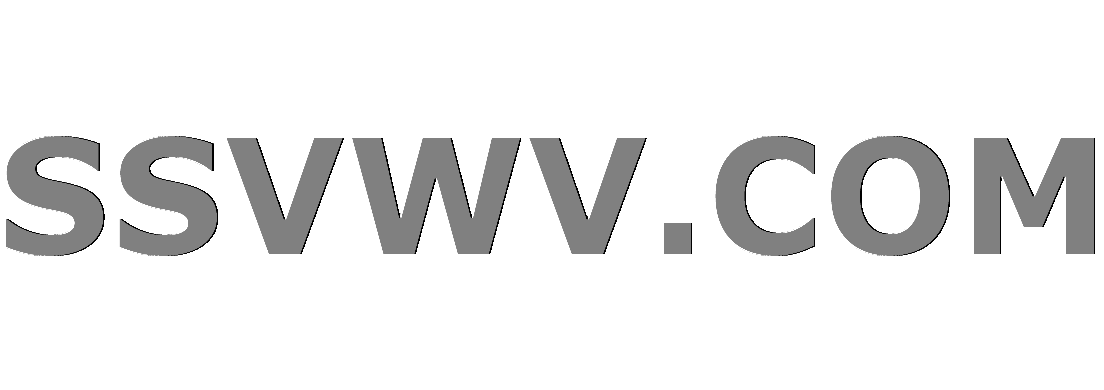
Multi tool use
I have a string which is separated by a ","
like string:= "abc@bk.com, cde@bk.com"
I want to make a regular expression which will cover all the whitespace after and before the emails or is there another function strings.Replace
to replace the whitespace? They both do the same work but I don't know which is better. If the regular expression is better then an you give a example and if strings.Replace
function is better then provide an example of that. I have tried a small code on it:-
package main
import (
"fmt"
"regexp"
"strings"
)
type User struct {
Name CustomerDetails `json:"name" bson:"name"`
}
type CustomerDetails struct {
Value string `json:"value" bson:"value"`
Note string `json:"note" bson:"note"`
SendNotifications bool `json:"send_notifications" bson:"send_notifications"`
}
func main() {
var emailRegexp = regexp.MustCompile("^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$")
var custName User
emails := "abc@bk.com, cde@bk.com"
splitEmails := strings.Split(emails, ",")
fmt.Println(splitEmails)
for _, email := range splitEmails {
email = strings.Replace(email, " ", "", -1)
if emailRegexp.MatchString(email) {
custName.Name = append(custName.Name, CustomerDetails{
Value: email,
})
}
}
fmt.Println(custName)
}
This example is based on the function strings.Replace
. Can anybody help me to solve this?
Thanks for your precious time.
regex string go whitespace
add a comment |
I have a string which is separated by a ","
like string:= "abc@bk.com, cde@bk.com"
I want to make a regular expression which will cover all the whitespace after and before the emails or is there another function strings.Replace
to replace the whitespace? They both do the same work but I don't know which is better. If the regular expression is better then an you give a example and if strings.Replace
function is better then provide an example of that. I have tried a small code on it:-
package main
import (
"fmt"
"regexp"
"strings"
)
type User struct {
Name CustomerDetails `json:"name" bson:"name"`
}
type CustomerDetails struct {
Value string `json:"value" bson:"value"`
Note string `json:"note" bson:"note"`
SendNotifications bool `json:"send_notifications" bson:"send_notifications"`
}
func main() {
var emailRegexp = regexp.MustCompile("^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$")
var custName User
emails := "abc@bk.com, cde@bk.com"
splitEmails := strings.Split(emails, ",")
fmt.Println(splitEmails)
for _, email := range splitEmails {
email = strings.Replace(email, " ", "", -1)
if emailRegexp.MatchString(email) {
custName.Name = append(custName.Name, CustomerDetails{
Value: email,
})
}
}
fmt.Println(custName)
}
This example is based on the function strings.Replace
. Can anybody help me to solve this?
Thanks for your precious time.
regex string go whitespace
1
You cannot and you must not try this: This will fail. Email addresses are far to complex to parse with a regexp. Do what Flimzy suggests.
– Volker
Nov 22 '18 at 8:18
add a comment |
I have a string which is separated by a ","
like string:= "abc@bk.com, cde@bk.com"
I want to make a regular expression which will cover all the whitespace after and before the emails or is there another function strings.Replace
to replace the whitespace? They both do the same work but I don't know which is better. If the regular expression is better then an you give a example and if strings.Replace
function is better then provide an example of that. I have tried a small code on it:-
package main
import (
"fmt"
"regexp"
"strings"
)
type User struct {
Name CustomerDetails `json:"name" bson:"name"`
}
type CustomerDetails struct {
Value string `json:"value" bson:"value"`
Note string `json:"note" bson:"note"`
SendNotifications bool `json:"send_notifications" bson:"send_notifications"`
}
func main() {
var emailRegexp = regexp.MustCompile("^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$")
var custName User
emails := "abc@bk.com, cde@bk.com"
splitEmails := strings.Split(emails, ",")
fmt.Println(splitEmails)
for _, email := range splitEmails {
email = strings.Replace(email, " ", "", -1)
if emailRegexp.MatchString(email) {
custName.Name = append(custName.Name, CustomerDetails{
Value: email,
})
}
}
fmt.Println(custName)
}
This example is based on the function strings.Replace
. Can anybody help me to solve this?
Thanks for your precious time.
regex string go whitespace
I have a string which is separated by a ","
like string:= "abc@bk.com, cde@bk.com"
I want to make a regular expression which will cover all the whitespace after and before the emails or is there another function strings.Replace
to replace the whitespace? They both do the same work but I don't know which is better. If the regular expression is better then an you give a example and if strings.Replace
function is better then provide an example of that. I have tried a small code on it:-
package main
import (
"fmt"
"regexp"
"strings"
)
type User struct {
Name CustomerDetails `json:"name" bson:"name"`
}
type CustomerDetails struct {
Value string `json:"value" bson:"value"`
Note string `json:"note" bson:"note"`
SendNotifications bool `json:"send_notifications" bson:"send_notifications"`
}
func main() {
var emailRegexp = regexp.MustCompile("^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$")
var custName User
emails := "abc@bk.com, cde@bk.com"
splitEmails := strings.Split(emails, ",")
fmt.Println(splitEmails)
for _, email := range splitEmails {
email = strings.Replace(email, " ", "", -1)
if emailRegexp.MatchString(email) {
custName.Name = append(custName.Name, CustomerDetails{
Value: email,
})
}
}
fmt.Println(custName)
}
This example is based on the function strings.Replace
. Can anybody help me to solve this?
Thanks for your precious time.
regex string go whitespace
regex string go whitespace
edited Nov 22 '18 at 7:29
Nick
31.6k121942
31.6k121942
asked Nov 22 '18 at 7:12
user10665991user10665991
64
64
1
You cannot and you must not try this: This will fail. Email addresses are far to complex to parse with a regexp. Do what Flimzy suggests.
– Volker
Nov 22 '18 at 8:18
add a comment |
1
You cannot and you must not try this: This will fail. Email addresses are far to complex to parse with a regexp. Do what Flimzy suggests.
– Volker
Nov 22 '18 at 8:18
1
1
You cannot and you must not try this: This will fail. Email addresses are far to complex to parse with a regexp. Do what Flimzy suggests.
– Volker
Nov 22 '18 at 8:18
You cannot and you must not try this: This will fail. Email addresses are far to complex to parse with a regexp. Do what Flimzy suggests.
– Volker
Nov 22 '18 at 8:18
add a comment |
2 Answers
2
active
oldest
votes
Do not use regular expressions for this. Your current approach, of splitting on white space, will break with valid email addresses that contain whitespace. While it is possible to parse many email addresses with a regular expression, the necessary regular expression is very, very ugly, and even that doesn't handle all corner cases.
Instead, to parse a list of email addresses, you should use the standard library's mail.ParseAddressList function.
The expression in the linked answer is often quoted, however "the regular expression does not cope with comments in email addresses. The RFC allows comments to be arbitrarily nested. A single regular expression cannot cope with this." ex-parrot.com/~pdw/Mail-RFC822-Address.html. There is also the matter that this is Perl syntax and does not work unmodified in RE2. So yeah, definitely use the parsing functions in the mail package.
– Peter
Nov 22 '18 at 9:10
@Peter: Thanks, I've added a comment to the effect that the mentioned RE isn't complete.
– Flimzy
Nov 22 '18 at 11:14
add a comment |
You may use .strip()
to clear whitespace on both sides.
Or re.split()
to split with pattern /,s*/
.
This question is about Go.
– Flimzy
Nov 22 '18 at 11:15
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53425599%2fhow-to-remove-the-white-space-in-a-string-or-make-a-regular-expression-that-woul%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Do not use regular expressions for this. Your current approach, of splitting on white space, will break with valid email addresses that contain whitespace. While it is possible to parse many email addresses with a regular expression, the necessary regular expression is very, very ugly, and even that doesn't handle all corner cases.
Instead, to parse a list of email addresses, you should use the standard library's mail.ParseAddressList function.
The expression in the linked answer is often quoted, however "the regular expression does not cope with comments in email addresses. The RFC allows comments to be arbitrarily nested. A single regular expression cannot cope with this." ex-parrot.com/~pdw/Mail-RFC822-Address.html. There is also the matter that this is Perl syntax and does not work unmodified in RE2. So yeah, definitely use the parsing functions in the mail package.
– Peter
Nov 22 '18 at 9:10
@Peter: Thanks, I've added a comment to the effect that the mentioned RE isn't complete.
– Flimzy
Nov 22 '18 at 11:14
add a comment |
Do not use regular expressions for this. Your current approach, of splitting on white space, will break with valid email addresses that contain whitespace. While it is possible to parse many email addresses with a regular expression, the necessary regular expression is very, very ugly, and even that doesn't handle all corner cases.
Instead, to parse a list of email addresses, you should use the standard library's mail.ParseAddressList function.
The expression in the linked answer is often quoted, however "the regular expression does not cope with comments in email addresses. The RFC allows comments to be arbitrarily nested. A single regular expression cannot cope with this." ex-parrot.com/~pdw/Mail-RFC822-Address.html. There is also the matter that this is Perl syntax and does not work unmodified in RE2. So yeah, definitely use the parsing functions in the mail package.
– Peter
Nov 22 '18 at 9:10
@Peter: Thanks, I've added a comment to the effect that the mentioned RE isn't complete.
– Flimzy
Nov 22 '18 at 11:14
add a comment |
Do not use regular expressions for this. Your current approach, of splitting on white space, will break with valid email addresses that contain whitespace. While it is possible to parse many email addresses with a regular expression, the necessary regular expression is very, very ugly, and even that doesn't handle all corner cases.
Instead, to parse a list of email addresses, you should use the standard library's mail.ParseAddressList function.
Do not use regular expressions for this. Your current approach, of splitting on white space, will break with valid email addresses that contain whitespace. While it is possible to parse many email addresses with a regular expression, the necessary regular expression is very, very ugly, and even that doesn't handle all corner cases.
Instead, to parse a list of email addresses, you should use the standard library's mail.ParseAddressList function.
edited Nov 22 '18 at 11:22
answered Nov 22 '18 at 7:46
FlimzyFlimzy
38.8k106597
38.8k106597
The expression in the linked answer is often quoted, however "the regular expression does not cope with comments in email addresses. The RFC allows comments to be arbitrarily nested. A single regular expression cannot cope with this." ex-parrot.com/~pdw/Mail-RFC822-Address.html. There is also the matter that this is Perl syntax and does not work unmodified in RE2. So yeah, definitely use the parsing functions in the mail package.
– Peter
Nov 22 '18 at 9:10
@Peter: Thanks, I've added a comment to the effect that the mentioned RE isn't complete.
– Flimzy
Nov 22 '18 at 11:14
add a comment |
The expression in the linked answer is often quoted, however "the regular expression does not cope with comments in email addresses. The RFC allows comments to be arbitrarily nested. A single regular expression cannot cope with this." ex-parrot.com/~pdw/Mail-RFC822-Address.html. There is also the matter that this is Perl syntax and does not work unmodified in RE2. So yeah, definitely use the parsing functions in the mail package.
– Peter
Nov 22 '18 at 9:10
@Peter: Thanks, I've added a comment to the effect that the mentioned RE isn't complete.
– Flimzy
Nov 22 '18 at 11:14
The expression in the linked answer is often quoted, however "the regular expression does not cope with comments in email addresses. The RFC allows comments to be arbitrarily nested. A single regular expression cannot cope with this." ex-parrot.com/~pdw/Mail-RFC822-Address.html. There is also the matter that this is Perl syntax and does not work unmodified in RE2. So yeah, definitely use the parsing functions in the mail package.
– Peter
Nov 22 '18 at 9:10
The expression in the linked answer is often quoted, however "the regular expression does not cope with comments in email addresses. The RFC allows comments to be arbitrarily nested. A single regular expression cannot cope with this." ex-parrot.com/~pdw/Mail-RFC822-Address.html. There is also the matter that this is Perl syntax and does not work unmodified in RE2. So yeah, definitely use the parsing functions in the mail package.
– Peter
Nov 22 '18 at 9:10
@Peter: Thanks, I've added a comment to the effect that the mentioned RE isn't complete.
– Flimzy
Nov 22 '18 at 11:14
@Peter: Thanks, I've added a comment to the effect that the mentioned RE isn't complete.
– Flimzy
Nov 22 '18 at 11:14
add a comment |
You may use .strip()
to clear whitespace on both sides.
Or re.split()
to split with pattern /,s*/
.
This question is about Go.
– Flimzy
Nov 22 '18 at 11:15
add a comment |
You may use .strip()
to clear whitespace on both sides.
Or re.split()
to split with pattern /,s*/
.
This question is about Go.
– Flimzy
Nov 22 '18 at 11:15
add a comment |
You may use .strip()
to clear whitespace on both sides.
Or re.split()
to split with pattern /,s*/
.
You may use .strip()
to clear whitespace on both sides.
Or re.split()
to split with pattern /,s*/
.
answered Nov 22 '18 at 7:51
TechnobulkaTechnobulka
14
14
This question is about Go.
– Flimzy
Nov 22 '18 at 11:15
add a comment |
This question is about Go.
– Flimzy
Nov 22 '18 at 11:15
This question is about Go.
– Flimzy
Nov 22 '18 at 11:15
This question is about Go.
– Flimzy
Nov 22 '18 at 11:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53425599%2fhow-to-remove-the-white-space-in-a-string-or-make-a-regular-expression-that-woul%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7E8YWCNZsy u6Xadnp fvlw2JQpSAK3x72wgyy0d,onqV hggfirwaP9mHP PR1AP7Gf2,unJRh7X nHM,1g,AXayCS rGAzf iEk4I8b,2F ob
1
You cannot and you must not try this: This will fail. Email addresses are far to complex to parse with a regexp. Do what Flimzy suggests.
– Volker
Nov 22 '18 at 8:18