Testing function using jest and enzyme
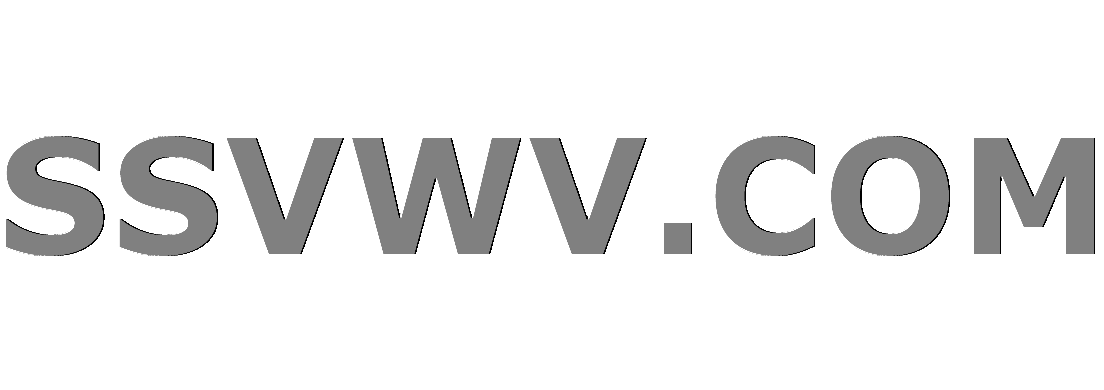
Multi tool use
I have a function which looks like this:
getPhoneComp() {
if (this.props.contactDetails.countPhone === 1)
return (<PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} />);
else if (this.props.contactDetails.countPhone === 2) {
return (
<div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[1]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
</div>
);
} else if (this.props.contactDetails.countPhone === 3) {
return (
<div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[1]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[2]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
</div>
);
}
else if (this.props.contactDetails.countPhone === 0) {
return (
<div />
);
}
}
Then in my render function, I am calling the function as shown below:
render() {
return (
<div>
{this.getPhoneComp()}
</div>
);
}
So, currently I am writing a test case for this component as shown below:
it('renders the Phone component', () => {
let contactDetailsState={contactDetails:{
webId:'',
contactId:'',
isContactPresent:true,
firstName:'',
lastName:'',
middleName:'',
customerSet:[{customerNumber:'1379',
customerName:'K F I INCORPORATED',
serviceAccountCount:2,
customerContactRelationshipType:'Z00001',
notificationEmailIndex:'E1',
customerStatus:'',
notificationVoiceIndex:'P2',
customerType:'S',
notificationPhoneIndex:'P1',
contactId:'0104489742',
notificationEmail:'xx1212@a.com',
notificationVoice:'4564564564',
notificationSms:'5656565566'}],
emailSet:[{notificationEmail:'xx1212@a.com',
notificationEmailIndex:'E1'},
{notificationEmail:'xx1@a.com',notificationEmailIndex:'E2'}],
emailSetWithNoAlert:[{notificationEmail:'xx1212@a.com',notificationEmailIndex:'E1'},
{notificationEmail:'xx1@a.com',notificationEmailIndex:'E2'},
{notificationEmail:'No email alerts',notificationEmailIndex:'NaN'}],
phoneSet:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',
notificationPhoneType:'M'},{notificationPhone:'4564564564',
notificationPhoneIndex:'P2',notificationPhoneType:'L'}],phoneSetWithNoAlert
:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',
notificationPhoneType:'M'},{notificationPhone:'4564564564',
notificationPhoneIndex:'P2',notificationPhoneType:'L'},
{notificationPhone:'No Phone Alerts',notificationPhoneIndex:'NaN',
notificationPhoneType:'L'},{notificationPhone:'No Text Alerts',
notificationPhoneIndex:'NaN',notificationPhoneType:'M'}],
mobileSet:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',notificationPhoneType:'M',contactId:'0104489742'}],
isMobilePresent:true,countEmail:2,countPhone:2,countMobile:1,totalphoneCount:2,
enrollCustomer:['1379'],suffix:'Jr.',prefix:'Mr.'}};
let errorHandlerFn=jest.fn();
let updateMobileNoFn=jest.fn();
let getPhoneCompFn=jest.fn();
let phoneComponent = mount(<PhoneContainer contactDetails={contactDetailsState} errorHandler={errorHandlerFn} updateMobileNo={updateMobileNoFn} getPhoneComp={getPhoneCompFn} />);
expect(getPhoneCompFn).toHaveBeenCalled();
});
So, I am trying to run the function, but while running this test, I get the below error:
Expected mock function to have been called.
in
expect(getPhoneCompFn).toHaveBeenCalled();
line in the test. So, how do I test if the function is called?
reactjs jestjs enzyme
add a comment |
I have a function which looks like this:
getPhoneComp() {
if (this.props.contactDetails.countPhone === 1)
return (<PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} />);
else if (this.props.contactDetails.countPhone === 2) {
return (
<div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[1]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
</div>
);
} else if (this.props.contactDetails.countPhone === 3) {
return (
<div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[1]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[2]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
</div>
);
}
else if (this.props.contactDetails.countPhone === 0) {
return (
<div />
);
}
}
Then in my render function, I am calling the function as shown below:
render() {
return (
<div>
{this.getPhoneComp()}
</div>
);
}
So, currently I am writing a test case for this component as shown below:
it('renders the Phone component', () => {
let contactDetailsState={contactDetails:{
webId:'',
contactId:'',
isContactPresent:true,
firstName:'',
lastName:'',
middleName:'',
customerSet:[{customerNumber:'1379',
customerName:'K F I INCORPORATED',
serviceAccountCount:2,
customerContactRelationshipType:'Z00001',
notificationEmailIndex:'E1',
customerStatus:'',
notificationVoiceIndex:'P2',
customerType:'S',
notificationPhoneIndex:'P1',
contactId:'0104489742',
notificationEmail:'xx1212@a.com',
notificationVoice:'4564564564',
notificationSms:'5656565566'}],
emailSet:[{notificationEmail:'xx1212@a.com',
notificationEmailIndex:'E1'},
{notificationEmail:'xx1@a.com',notificationEmailIndex:'E2'}],
emailSetWithNoAlert:[{notificationEmail:'xx1212@a.com',notificationEmailIndex:'E1'},
{notificationEmail:'xx1@a.com',notificationEmailIndex:'E2'},
{notificationEmail:'No email alerts',notificationEmailIndex:'NaN'}],
phoneSet:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',
notificationPhoneType:'M'},{notificationPhone:'4564564564',
notificationPhoneIndex:'P2',notificationPhoneType:'L'}],phoneSetWithNoAlert
:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',
notificationPhoneType:'M'},{notificationPhone:'4564564564',
notificationPhoneIndex:'P2',notificationPhoneType:'L'},
{notificationPhone:'No Phone Alerts',notificationPhoneIndex:'NaN',
notificationPhoneType:'L'},{notificationPhone:'No Text Alerts',
notificationPhoneIndex:'NaN',notificationPhoneType:'M'}],
mobileSet:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',notificationPhoneType:'M',contactId:'0104489742'}],
isMobilePresent:true,countEmail:2,countPhone:2,countMobile:1,totalphoneCount:2,
enrollCustomer:['1379'],suffix:'Jr.',prefix:'Mr.'}};
let errorHandlerFn=jest.fn();
let updateMobileNoFn=jest.fn();
let getPhoneCompFn=jest.fn();
let phoneComponent = mount(<PhoneContainer contactDetails={contactDetailsState} errorHandler={errorHandlerFn} updateMobileNo={updateMobileNoFn} getPhoneComp={getPhoneCompFn} />);
expect(getPhoneCompFn).toHaveBeenCalled();
});
So, I am trying to run the function, but while running this test, I get the below error:
Expected mock function to have been called.
in
expect(getPhoneCompFn).toHaveBeenCalled();
line in the test. So, how do I test if the function is called?
reactjs jestjs enzyme
add a comment |
I have a function which looks like this:
getPhoneComp() {
if (this.props.contactDetails.countPhone === 1)
return (<PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} />);
else if (this.props.contactDetails.countPhone === 2) {
return (
<div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[1]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
</div>
);
} else if (this.props.contactDetails.countPhone === 3) {
return (
<div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[1]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[2]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
</div>
);
}
else if (this.props.contactDetails.countPhone === 0) {
return (
<div />
);
}
}
Then in my render function, I am calling the function as shown below:
render() {
return (
<div>
{this.getPhoneComp()}
</div>
);
}
So, currently I am writing a test case for this component as shown below:
it('renders the Phone component', () => {
let contactDetailsState={contactDetails:{
webId:'',
contactId:'',
isContactPresent:true,
firstName:'',
lastName:'',
middleName:'',
customerSet:[{customerNumber:'1379',
customerName:'K F I INCORPORATED',
serviceAccountCount:2,
customerContactRelationshipType:'Z00001',
notificationEmailIndex:'E1',
customerStatus:'',
notificationVoiceIndex:'P2',
customerType:'S',
notificationPhoneIndex:'P1',
contactId:'0104489742',
notificationEmail:'xx1212@a.com',
notificationVoice:'4564564564',
notificationSms:'5656565566'}],
emailSet:[{notificationEmail:'xx1212@a.com',
notificationEmailIndex:'E1'},
{notificationEmail:'xx1@a.com',notificationEmailIndex:'E2'}],
emailSetWithNoAlert:[{notificationEmail:'xx1212@a.com',notificationEmailIndex:'E1'},
{notificationEmail:'xx1@a.com',notificationEmailIndex:'E2'},
{notificationEmail:'No email alerts',notificationEmailIndex:'NaN'}],
phoneSet:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',
notificationPhoneType:'M'},{notificationPhone:'4564564564',
notificationPhoneIndex:'P2',notificationPhoneType:'L'}],phoneSetWithNoAlert
:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',
notificationPhoneType:'M'},{notificationPhone:'4564564564',
notificationPhoneIndex:'P2',notificationPhoneType:'L'},
{notificationPhone:'No Phone Alerts',notificationPhoneIndex:'NaN',
notificationPhoneType:'L'},{notificationPhone:'No Text Alerts',
notificationPhoneIndex:'NaN',notificationPhoneType:'M'}],
mobileSet:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',notificationPhoneType:'M',contactId:'0104489742'}],
isMobilePresent:true,countEmail:2,countPhone:2,countMobile:1,totalphoneCount:2,
enrollCustomer:['1379'],suffix:'Jr.',prefix:'Mr.'}};
let errorHandlerFn=jest.fn();
let updateMobileNoFn=jest.fn();
let getPhoneCompFn=jest.fn();
let phoneComponent = mount(<PhoneContainer contactDetails={contactDetailsState} errorHandler={errorHandlerFn} updateMobileNo={updateMobileNoFn} getPhoneComp={getPhoneCompFn} />);
expect(getPhoneCompFn).toHaveBeenCalled();
});
So, I am trying to run the function, but while running this test, I get the below error:
Expected mock function to have been called.
in
expect(getPhoneCompFn).toHaveBeenCalled();
line in the test. So, how do I test if the function is called?
reactjs jestjs enzyme
I have a function which looks like this:
getPhoneComp() {
if (this.props.contactDetails.countPhone === 1)
return (<PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} />);
else if (this.props.contactDetails.countPhone === 2) {
return (
<div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[1]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
</div>
);
} else if (this.props.contactDetails.countPhone === 3) {
return (
<div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[0]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[1]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
<div className={classes.sceESpace}><PhoneComp contactDetails={this.props.contactDetails.phoneSet[2]} contactId={this.props.contactDetails.contactId} errorHandler={this.props.errorHandler} updateMobileNo={this.props.updateMobileNo} /></div>
</div>
);
}
else if (this.props.contactDetails.countPhone === 0) {
return (
<div />
);
}
}
Then in my render function, I am calling the function as shown below:
render() {
return (
<div>
{this.getPhoneComp()}
</div>
);
}
So, currently I am writing a test case for this component as shown below:
it('renders the Phone component', () => {
let contactDetailsState={contactDetails:{
webId:'',
contactId:'',
isContactPresent:true,
firstName:'',
lastName:'',
middleName:'',
customerSet:[{customerNumber:'1379',
customerName:'K F I INCORPORATED',
serviceAccountCount:2,
customerContactRelationshipType:'Z00001',
notificationEmailIndex:'E1',
customerStatus:'',
notificationVoiceIndex:'P2',
customerType:'S',
notificationPhoneIndex:'P1',
contactId:'0104489742',
notificationEmail:'xx1212@a.com',
notificationVoice:'4564564564',
notificationSms:'5656565566'}],
emailSet:[{notificationEmail:'xx1212@a.com',
notificationEmailIndex:'E1'},
{notificationEmail:'xx1@a.com',notificationEmailIndex:'E2'}],
emailSetWithNoAlert:[{notificationEmail:'xx1212@a.com',notificationEmailIndex:'E1'},
{notificationEmail:'xx1@a.com',notificationEmailIndex:'E2'},
{notificationEmail:'No email alerts',notificationEmailIndex:'NaN'}],
phoneSet:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',
notificationPhoneType:'M'},{notificationPhone:'4564564564',
notificationPhoneIndex:'P2',notificationPhoneType:'L'}],phoneSetWithNoAlert
:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',
notificationPhoneType:'M'},{notificationPhone:'4564564564',
notificationPhoneIndex:'P2',notificationPhoneType:'L'},
{notificationPhone:'No Phone Alerts',notificationPhoneIndex:'NaN',
notificationPhoneType:'L'},{notificationPhone:'No Text Alerts',
notificationPhoneIndex:'NaN',notificationPhoneType:'M'}],
mobileSet:[{notificationPhone:'5656565566',notificationPhoneIndex:'P1',notificationPhoneType:'M',contactId:'0104489742'}],
isMobilePresent:true,countEmail:2,countPhone:2,countMobile:1,totalphoneCount:2,
enrollCustomer:['1379'],suffix:'Jr.',prefix:'Mr.'}};
let errorHandlerFn=jest.fn();
let updateMobileNoFn=jest.fn();
let getPhoneCompFn=jest.fn();
let phoneComponent = mount(<PhoneContainer contactDetails={contactDetailsState} errorHandler={errorHandlerFn} updateMobileNo={updateMobileNoFn} getPhoneComp={getPhoneCompFn} />);
expect(getPhoneCompFn).toHaveBeenCalled();
});
So, I am trying to run the function, but while running this test, I get the below error:
Expected mock function to have been called.
in
expect(getPhoneCompFn).toHaveBeenCalled();
line in the test. So, how do I test if the function is called?
reactjs jestjs enzyme
reactjs jestjs enzyme
edited Nov 22 '18 at 10:24
skyboyer
3,75311229
3,75311229
asked Nov 22 '18 at 7:21
pranamipranami
239313
239313
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
getPhoneComp
is not a prop but a method. Since it's prototype method, it can be mocked on class prototype:
getPhoneCompFn=jest.spyOn(PhoneContainer.prototype, 'getPhoneComp')
.mockImplementation(() => {});
let phoneComponent = mount(<PhoneContainer ... />);
Thanks, it worked.Now the error is gone and I can see my function's coverage %age has increased, but the coverage for statements,branch and lines has decreased and is below 50% now, Previously when I was usingexpect(getPhoneCompFn).toHaveBeenCalled();
, I could see the coverage for the other 3 was increased and that of functions was 0% and also I was getting the mentioned error.Why is this happening? I mean I don't even have any other lines to be tested in my render function, it just calls thegetPhoneComp()
method.
– pranami
Nov 22 '18 at 7:46
1
Likely because realgetPhoneComp
is never called because it's stubbed. I assume this is unit test. In unit tests, you stub/mock everything but tested unit. You need to test realgetPhoneComp
in another test. It's preferable to useshallow
for that.
– estus
Nov 22 '18 at 7:53
ohh, okay. Thanks for the help, will write a new test for the function then.
– pranami
Nov 22 '18 at 7:55
I have tried writing a test case for my function separately, but its still not increasing the coverage.I have posted a separate question for this: stackoverflow.com/questions/53428619/… . Can you please have a look at it once.
– pranami
Nov 22 '18 at 10:16
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53425736%2ftesting-function-using-jest-and-enzyme%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
getPhoneComp
is not a prop but a method. Since it's prototype method, it can be mocked on class prototype:
getPhoneCompFn=jest.spyOn(PhoneContainer.prototype, 'getPhoneComp')
.mockImplementation(() => {});
let phoneComponent = mount(<PhoneContainer ... />);
Thanks, it worked.Now the error is gone and I can see my function's coverage %age has increased, but the coverage for statements,branch and lines has decreased and is below 50% now, Previously when I was usingexpect(getPhoneCompFn).toHaveBeenCalled();
, I could see the coverage for the other 3 was increased and that of functions was 0% and also I was getting the mentioned error.Why is this happening? I mean I don't even have any other lines to be tested in my render function, it just calls thegetPhoneComp()
method.
– pranami
Nov 22 '18 at 7:46
1
Likely because realgetPhoneComp
is never called because it's stubbed. I assume this is unit test. In unit tests, you stub/mock everything but tested unit. You need to test realgetPhoneComp
in another test. It's preferable to useshallow
for that.
– estus
Nov 22 '18 at 7:53
ohh, okay. Thanks for the help, will write a new test for the function then.
– pranami
Nov 22 '18 at 7:55
I have tried writing a test case for my function separately, but its still not increasing the coverage.I have posted a separate question for this: stackoverflow.com/questions/53428619/… . Can you please have a look at it once.
– pranami
Nov 22 '18 at 10:16
add a comment |
getPhoneComp
is not a prop but a method. Since it's prototype method, it can be mocked on class prototype:
getPhoneCompFn=jest.spyOn(PhoneContainer.prototype, 'getPhoneComp')
.mockImplementation(() => {});
let phoneComponent = mount(<PhoneContainer ... />);
Thanks, it worked.Now the error is gone and I can see my function's coverage %age has increased, but the coverage for statements,branch and lines has decreased and is below 50% now, Previously when I was usingexpect(getPhoneCompFn).toHaveBeenCalled();
, I could see the coverage for the other 3 was increased and that of functions was 0% and also I was getting the mentioned error.Why is this happening? I mean I don't even have any other lines to be tested in my render function, it just calls thegetPhoneComp()
method.
– pranami
Nov 22 '18 at 7:46
1
Likely because realgetPhoneComp
is never called because it's stubbed. I assume this is unit test. In unit tests, you stub/mock everything but tested unit. You need to test realgetPhoneComp
in another test. It's preferable to useshallow
for that.
– estus
Nov 22 '18 at 7:53
ohh, okay. Thanks for the help, will write a new test for the function then.
– pranami
Nov 22 '18 at 7:55
I have tried writing a test case for my function separately, but its still not increasing the coverage.I have posted a separate question for this: stackoverflow.com/questions/53428619/… . Can you please have a look at it once.
– pranami
Nov 22 '18 at 10:16
add a comment |
getPhoneComp
is not a prop but a method. Since it's prototype method, it can be mocked on class prototype:
getPhoneCompFn=jest.spyOn(PhoneContainer.prototype, 'getPhoneComp')
.mockImplementation(() => {});
let phoneComponent = mount(<PhoneContainer ... />);
getPhoneComp
is not a prop but a method. Since it's prototype method, it can be mocked on class prototype:
getPhoneCompFn=jest.spyOn(PhoneContainer.prototype, 'getPhoneComp')
.mockImplementation(() => {});
let phoneComponent = mount(<PhoneContainer ... />);
answered Nov 22 '18 at 7:31
estusestus
73.6k22108224
73.6k22108224
Thanks, it worked.Now the error is gone and I can see my function's coverage %age has increased, but the coverage for statements,branch and lines has decreased and is below 50% now, Previously when I was usingexpect(getPhoneCompFn).toHaveBeenCalled();
, I could see the coverage for the other 3 was increased and that of functions was 0% and also I was getting the mentioned error.Why is this happening? I mean I don't even have any other lines to be tested in my render function, it just calls thegetPhoneComp()
method.
– pranami
Nov 22 '18 at 7:46
1
Likely because realgetPhoneComp
is never called because it's stubbed. I assume this is unit test. In unit tests, you stub/mock everything but tested unit. You need to test realgetPhoneComp
in another test. It's preferable to useshallow
for that.
– estus
Nov 22 '18 at 7:53
ohh, okay. Thanks for the help, will write a new test for the function then.
– pranami
Nov 22 '18 at 7:55
I have tried writing a test case for my function separately, but its still not increasing the coverage.I have posted a separate question for this: stackoverflow.com/questions/53428619/… . Can you please have a look at it once.
– pranami
Nov 22 '18 at 10:16
add a comment |
Thanks, it worked.Now the error is gone and I can see my function's coverage %age has increased, but the coverage for statements,branch and lines has decreased and is below 50% now, Previously when I was usingexpect(getPhoneCompFn).toHaveBeenCalled();
, I could see the coverage for the other 3 was increased and that of functions was 0% and also I was getting the mentioned error.Why is this happening? I mean I don't even have any other lines to be tested in my render function, it just calls thegetPhoneComp()
method.
– pranami
Nov 22 '18 at 7:46
1
Likely because realgetPhoneComp
is never called because it's stubbed. I assume this is unit test. In unit tests, you stub/mock everything but tested unit. You need to test realgetPhoneComp
in another test. It's preferable to useshallow
for that.
– estus
Nov 22 '18 at 7:53
ohh, okay. Thanks for the help, will write a new test for the function then.
– pranami
Nov 22 '18 at 7:55
I have tried writing a test case for my function separately, but its still not increasing the coverage.I have posted a separate question for this: stackoverflow.com/questions/53428619/… . Can you please have a look at it once.
– pranami
Nov 22 '18 at 10:16
Thanks, it worked.Now the error is gone and I can see my function's coverage %age has increased, but the coverage for statements,branch and lines has decreased and is below 50% now, Previously when I was using
expect(getPhoneCompFn).toHaveBeenCalled();
, I could see the coverage for the other 3 was increased and that of functions was 0% and also I was getting the mentioned error.Why is this happening? I mean I don't even have any other lines to be tested in my render function, it just calls the getPhoneComp()
method.– pranami
Nov 22 '18 at 7:46
Thanks, it worked.Now the error is gone and I can see my function's coverage %age has increased, but the coverage for statements,branch and lines has decreased and is below 50% now, Previously when I was using
expect(getPhoneCompFn).toHaveBeenCalled();
, I could see the coverage for the other 3 was increased and that of functions was 0% and also I was getting the mentioned error.Why is this happening? I mean I don't even have any other lines to be tested in my render function, it just calls the getPhoneComp()
method.– pranami
Nov 22 '18 at 7:46
1
1
Likely because real
getPhoneComp
is never called because it's stubbed. I assume this is unit test. In unit tests, you stub/mock everything but tested unit. You need to test real getPhoneComp
in another test. It's preferable to use shallow
for that.– estus
Nov 22 '18 at 7:53
Likely because real
getPhoneComp
is never called because it's stubbed. I assume this is unit test. In unit tests, you stub/mock everything but tested unit. You need to test real getPhoneComp
in another test. It's preferable to use shallow
for that.– estus
Nov 22 '18 at 7:53
ohh, okay. Thanks for the help, will write a new test for the function then.
– pranami
Nov 22 '18 at 7:55
ohh, okay. Thanks for the help, will write a new test for the function then.
– pranami
Nov 22 '18 at 7:55
I have tried writing a test case for my function separately, but its still not increasing the coverage.I have posted a separate question for this: stackoverflow.com/questions/53428619/… . Can you please have a look at it once.
– pranami
Nov 22 '18 at 10:16
I have tried writing a test case for my function separately, but its still not increasing the coverage.I have posted a separate question for this: stackoverflow.com/questions/53428619/… . Can you please have a look at it once.
– pranami
Nov 22 '18 at 10:16
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53425736%2ftesting-function-using-jest-and-enzyme%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HavzQ4E,dMLx1p bx,waAZUOL3nubf7jA,OlNC9Z,yGG6XhDPn NOeP0YizrqJfY0O7