Loop through json array in string python
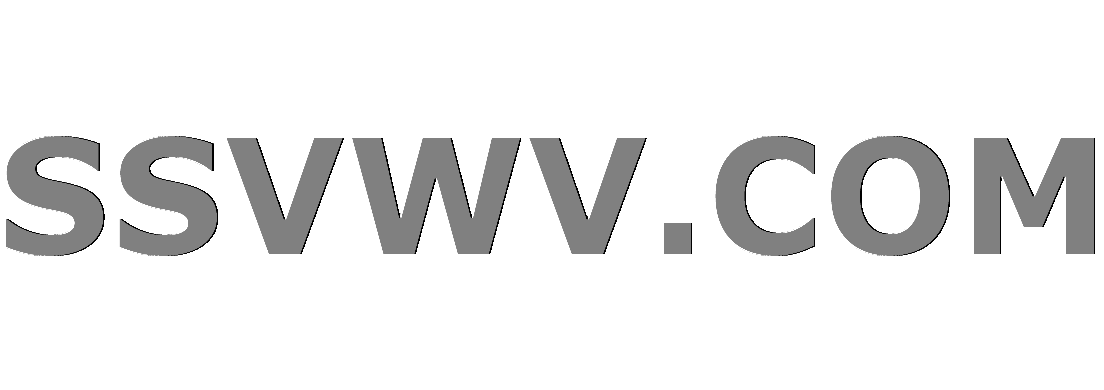
Multi tool use
up vote
0
down vote
favorite
I have a string and I created a JSON array which contains strings and values:
amount = 0
a = "asaf,almog,arnon,elbar"
values_li={'asaf':'1','almog':'6','elbar':'2'}
How can I create a loop that will search all items on values_li
in a
and for each item it will find it will do
amount = amount + value(the value that found from value_li in a)
I tried to do this but it doesn't work:
for k,v in values_li.items():
if k in a:
amount = amount + v
python json
add a comment |
up vote
0
down vote
favorite
I have a string and I created a JSON array which contains strings and values:
amount = 0
a = "asaf,almog,arnon,elbar"
values_li={'asaf':'1','almog':'6','elbar':'2'}
How can I create a loop that will search all items on values_li
in a
and for each item it will find it will do
amount = amount + value(the value that found from value_li in a)
I tried to do this but it doesn't work:
for k,v in values_li.items():
if k in a:
amount = amount + v
python json
ammount += int(v)
, you're trying to append a string. It would also be great if you in the future tell us why it isn't working by adding a traceback or description of the problem, not just what you want to achieve. I'm guessing tho that you're gettingTypeError: unsupported operand type(s) for +: 'int' and 'str'
. Hence my first part of my comment.
– Torxed
Nov 17 at 22:03
What do you mean by "it doesn't work"? What happens instead of what you want to happen?
– mkrieger1
Nov 17 at 22:03
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a string and I created a JSON array which contains strings and values:
amount = 0
a = "asaf,almog,arnon,elbar"
values_li={'asaf':'1','almog':'6','elbar':'2'}
How can I create a loop that will search all items on values_li
in a
and for each item it will find it will do
amount = amount + value(the value that found from value_li in a)
I tried to do this but it doesn't work:
for k,v in values_li.items():
if k in a:
amount = amount + v
python json
I have a string and I created a JSON array which contains strings and values:
amount = 0
a = "asaf,almog,arnon,elbar"
values_li={'asaf':'1','almog':'6','elbar':'2'}
How can I create a loop that will search all items on values_li
in a
and for each item it will find it will do
amount = amount + value(the value that found from value_li in a)
I tried to do this but it doesn't work:
for k,v in values_li.items():
if k in a:
amount = amount + v
python json
python json
edited Nov 17 at 22:05


mkrieger1
4,19421832
4,19421832
asked Nov 17 at 22:00
אסף מזון
23
23
ammount += int(v)
, you're trying to append a string. It would also be great if you in the future tell us why it isn't working by adding a traceback or description of the problem, not just what you want to achieve. I'm guessing tho that you're gettingTypeError: unsupported operand type(s) for +: 'int' and 'str'
. Hence my first part of my comment.
– Torxed
Nov 17 at 22:03
What do you mean by "it doesn't work"? What happens instead of what you want to happen?
– mkrieger1
Nov 17 at 22:03
add a comment |
ammount += int(v)
, you're trying to append a string. It would also be great if you in the future tell us why it isn't working by adding a traceback or description of the problem, not just what you want to achieve. I'm guessing tho that you're gettingTypeError: unsupported operand type(s) for +: 'int' and 'str'
. Hence my first part of my comment.
– Torxed
Nov 17 at 22:03
What do you mean by "it doesn't work"? What happens instead of what you want to happen?
– mkrieger1
Nov 17 at 22:03
ammount += int(v)
, you're trying to append a string. It would also be great if you in the future tell us why it isn't working by adding a traceback or description of the problem, not just what you want to achieve. I'm guessing tho that you're getting TypeError: unsupported operand type(s) for +: 'int' and 'str'
. Hence my first part of my comment.– Torxed
Nov 17 at 22:03
ammount += int(v)
, you're trying to append a string. It would also be great if you in the future tell us why it isn't working by adding a traceback or description of the problem, not just what you want to achieve. I'm guessing tho that you're getting TypeError: unsupported operand type(s) for +: 'int' and 'str'
. Hence my first part of my comment.– Torxed
Nov 17 at 22:03
What do you mean by "it doesn't work"? What happens instead of what you want to happen?
– mkrieger1
Nov 17 at 22:03
What do you mean by "it doesn't work"? What happens instead of what you want to happen?
– mkrieger1
Nov 17 at 22:03
add a comment |
3 Answers
3
active
oldest
votes
up vote
0
down vote
It's working.
I figure out my problem.
v is a string and I tried to do math with a string so I had to convert v to an int
amount = amount + int(v)
Now It's working :)
add a comment |
up vote
0
down vote
You should be careful using:
if k in a:
a
is the string: "asaf,almog,arnon,elbar"
not a list. This means that:
"bar" in a # == True
"as" in a # == True
..etc Which is probably not what you want.
You should consider splitting it into an array, then you'll only get complete matches. With that you can simply use:
a = "asaf,almog,arnon,elbar".split(',')
values_li={'asaf':'1','almog':'6','elbar':'2'}
amount = sum([int(values_li[k]) for k in a if k in values_li])
# 9
add a comment |
up vote
0
down vote
collections.Counter()
is your friend:
from collections import Counter
a = "asaf,almog,arnon,elbar"
values_li = Counter({'asaf':1,'almog':6,'elbar':2})
values_li.update(a.split(','))
values_li
That will result in:
Counter({'almog': 7, 'elbar': 3, 'asaf': 2, 'arnon': 1})
And if you want the sum of all values in values_li
, you can simply do:
sum(values_li.values())
Which will result in 13
, for the key/value pairs in your example.
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
It's working.
I figure out my problem.
v is a string and I tried to do math with a string so I had to convert v to an int
amount = amount + int(v)
Now It's working :)
add a comment |
up vote
0
down vote
It's working.
I figure out my problem.
v is a string and I tried to do math with a string so I had to convert v to an int
amount = amount + int(v)
Now It's working :)
add a comment |
up vote
0
down vote
up vote
0
down vote
It's working.
I figure out my problem.
v is a string and I tried to do math with a string so I had to convert v to an int
amount = amount + int(v)
Now It's working :)
It's working.
I figure out my problem.
v is a string and I tried to do math with a string so I had to convert v to an int
amount = amount + int(v)
Now It's working :)
answered Nov 17 at 22:17
אסף מזון
23
23
add a comment |
add a comment |
up vote
0
down vote
You should be careful using:
if k in a:
a
is the string: "asaf,almog,arnon,elbar"
not a list. This means that:
"bar" in a # == True
"as" in a # == True
..etc Which is probably not what you want.
You should consider splitting it into an array, then you'll only get complete matches. With that you can simply use:
a = "asaf,almog,arnon,elbar".split(',')
values_li={'asaf':'1','almog':'6','elbar':'2'}
amount = sum([int(values_li[k]) for k in a if k in values_li])
# 9
add a comment |
up vote
0
down vote
You should be careful using:
if k in a:
a
is the string: "asaf,almog,arnon,elbar"
not a list. This means that:
"bar" in a # == True
"as" in a # == True
..etc Which is probably not what you want.
You should consider splitting it into an array, then you'll only get complete matches. With that you can simply use:
a = "asaf,almog,arnon,elbar".split(',')
values_li={'asaf':'1','almog':'6','elbar':'2'}
amount = sum([int(values_li[k]) for k in a if k in values_li])
# 9
add a comment |
up vote
0
down vote
up vote
0
down vote
You should be careful using:
if k in a:
a
is the string: "asaf,almog,arnon,elbar"
not a list. This means that:
"bar" in a # == True
"as" in a # == True
..etc Which is probably not what you want.
You should consider splitting it into an array, then you'll only get complete matches. With that you can simply use:
a = "asaf,almog,arnon,elbar".split(',')
values_li={'asaf':'1','almog':'6','elbar':'2'}
amount = sum([int(values_li[k]) for k in a if k in values_li])
# 9
You should be careful using:
if k in a:
a
is the string: "asaf,almog,arnon,elbar"
not a list. This means that:
"bar" in a # == True
"as" in a # == True
..etc Which is probably not what you want.
You should consider splitting it into an array, then you'll only get complete matches. With that you can simply use:
a = "asaf,almog,arnon,elbar".split(',')
values_li={'asaf':'1','almog':'6','elbar':'2'}
amount = sum([int(values_li[k]) for k in a if k in values_li])
# 9
answered Nov 17 at 22:28


Mark Meyer
29.8k32549
29.8k32549
add a comment |
add a comment |
up vote
0
down vote
collections.Counter()
is your friend:
from collections import Counter
a = "asaf,almog,arnon,elbar"
values_li = Counter({'asaf':1,'almog':6,'elbar':2})
values_li.update(a.split(','))
values_li
That will result in:
Counter({'almog': 7, 'elbar': 3, 'asaf': 2, 'arnon': 1})
And if you want the sum of all values in values_li
, you can simply do:
sum(values_li.values())
Which will result in 13
, for the key/value pairs in your example.
add a comment |
up vote
0
down vote
collections.Counter()
is your friend:
from collections import Counter
a = "asaf,almog,arnon,elbar"
values_li = Counter({'asaf':1,'almog':6,'elbar':2})
values_li.update(a.split(','))
values_li
That will result in:
Counter({'almog': 7, 'elbar': 3, 'asaf': 2, 'arnon': 1})
And if you want the sum of all values in values_li
, you can simply do:
sum(values_li.values())
Which will result in 13
, for the key/value pairs in your example.
add a comment |
up vote
0
down vote
up vote
0
down vote
collections.Counter()
is your friend:
from collections import Counter
a = "asaf,almog,arnon,elbar"
values_li = Counter({'asaf':1,'almog':6,'elbar':2})
values_li.update(a.split(','))
values_li
That will result in:
Counter({'almog': 7, 'elbar': 3, 'asaf': 2, 'arnon': 1})
And if you want the sum of all values in values_li
, you can simply do:
sum(values_li.values())
Which will result in 13
, for the key/value pairs in your example.
collections.Counter()
is your friend:
from collections import Counter
a = "asaf,almog,arnon,elbar"
values_li = Counter({'asaf':1,'almog':6,'elbar':2})
values_li.update(a.split(','))
values_li
That will result in:
Counter({'almog': 7, 'elbar': 3, 'asaf': 2, 'arnon': 1})
And if you want the sum of all values in values_li
, you can simply do:
sum(values_li.values())
Which will result in 13
, for the key/value pairs in your example.
edited Nov 17 at 22:41
answered Nov 17 at 22:36


accdias
38229
38229
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53355949%2floop-through-json-array-in-string-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3pvcVX0GG5jrk5,c frkw4lUmGXEaLPXFxn6V,IQT,d,07Pmja xyibFuZQHkRPIWFWIr hchqsv1V34lwn4vzVwkqWEZNE0b,qC
ammount += int(v)
, you're trying to append a string. It would also be great if you in the future tell us why it isn't working by adding a traceback or description of the problem, not just what you want to achieve. I'm guessing tho that you're gettingTypeError: unsupported operand type(s) for +: 'int' and 'str'
. Hence my first part of my comment.– Torxed
Nov 17 at 22:03
What do you mean by "it doesn't work"? What happens instead of what you want to happen?
– mkrieger1
Nov 17 at 22:03