error: incompatible types: unexpected return value : Java 8 [duplicate]
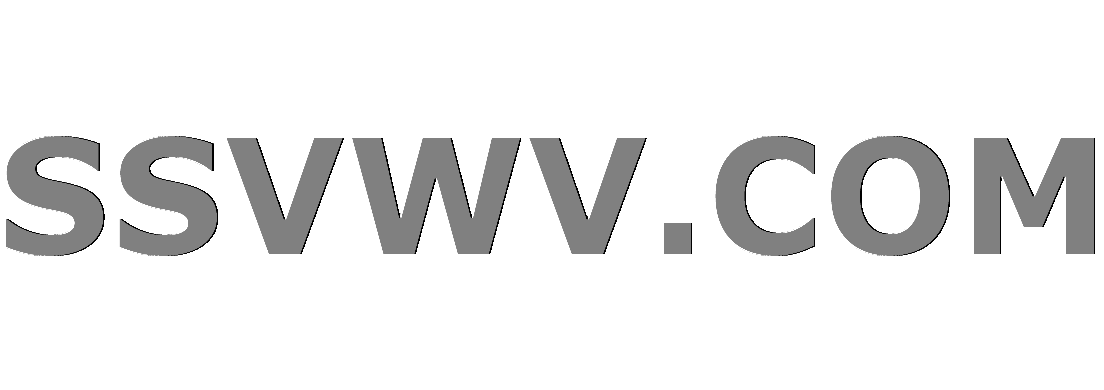
Multi tool use
up vote
9
down vote
favorite
This question already has an answer here:
error: incompatible types: unexpected return value Char compare to String
3 answers
I have written a simple method which returns the boolean value.
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs){
if(studentConfigs != null)
{
studentConfigs.forEach(studentConfig -> {
if(studentConfig.action() == null || !studentConfig.action().equals(Action.DELETE)) {
return true;
}
});
}
return false;
}
The method is throwing the following exception .
error: incompatible types: unexpected return value
studentConfigs.forEach(studentConfig ->
What's the problem with my code?
java foreach java-8 java-stream
marked as duplicate by Robert Harvey♦ Nov 26 at 16:06
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
add a comment |
up vote
9
down vote
favorite
This question already has an answer here:
error: incompatible types: unexpected return value Char compare to String
3 answers
I have written a simple method which returns the boolean value.
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs){
if(studentConfigs != null)
{
studentConfigs.forEach(studentConfig -> {
if(studentConfig.action() == null || !studentConfig.action().equals(Action.DELETE)) {
return true;
}
});
}
return false;
}
The method is throwing the following exception .
error: incompatible types: unexpected return value
studentConfigs.forEach(studentConfig ->
What's the problem with my code?
java foreach java-8 java-stream
marked as duplicate by Robert Harvey♦ Nov 26 at 16:06
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
add a comment |
up vote
9
down vote
favorite
up vote
9
down vote
favorite
This question already has an answer here:
error: incompatible types: unexpected return value Char compare to String
3 answers
I have written a simple method which returns the boolean value.
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs){
if(studentConfigs != null)
{
studentConfigs.forEach(studentConfig -> {
if(studentConfig.action() == null || !studentConfig.action().equals(Action.DELETE)) {
return true;
}
});
}
return false;
}
The method is throwing the following exception .
error: incompatible types: unexpected return value
studentConfigs.forEach(studentConfig ->
What's the problem with my code?
java foreach java-8 java-stream
This question already has an answer here:
error: incompatible types: unexpected return value Char compare to String
3 answers
I have written a simple method which returns the boolean value.
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs){
if(studentConfigs != null)
{
studentConfigs.forEach(studentConfig -> {
if(studentConfig.action() == null || !studentConfig.action().equals(Action.DELETE)) {
return true;
}
});
}
return false;
}
The method is throwing the following exception .
error: incompatible types: unexpected return value
studentConfigs.forEach(studentConfig ->
What's the problem with my code?
This question already has an answer here:
error: incompatible types: unexpected return value Char compare to String
3 answers
java foreach java-8 java-stream
java foreach java-8 java-stream
edited Nov 26 at 12:55


Andrew Tobilko
24k84079
24k84079
asked Nov 26 at 12:14


Ram R
513
513
marked as duplicate by Robert Harvey♦ Nov 26 at 16:06
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
marked as duplicate by Robert Harvey♦ Nov 26 at 16:06
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
add a comment |
add a comment |
5 Answers
5
active
oldest
votes
up vote
10
down vote
accepted
The lambda expression passed to forEach
shouldn't have a return value.
It looks like you want to return true
if any of the elements of the input Collection
satisfies a condition:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs){
if(studentConfigs != null) {
if (studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE))) {
return true;
}
}
return false;
}
As Holger suggested, this can be reduced to a single statement:
return studentConfigs != null && studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE));
or
return studentConfigs != null ? studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE)) : false;
2
Why not a simple enhanced forloop? When do you choose one over the other?
– Luke Garrigan
Nov 26 at 12:22
1
@LukeGarrigan Enhanced for loop would also be fine. As to which one to choose, I'd go for the one more readable to you (and to other developers that may read your code). I think usinganyMatch
in this example better expresses what the method is doing, which is to find whether any of the elements of the input collection satisfies some condition.
– Eran
Nov 26 at 12:26
1
@LukeGarrigan Java is an evolving language. Higher order functions are working their way in. I would suggest you embrace them, rather than get left behind
– Alexander
Nov 26 at 14:16
1
@LukeGarrigan "I suppose it's just because I don't use it as often as I potentially should." Yep, pretty much. This is the future of Java, so you'll only see more and more of these higher order functions.
– Alexander
Nov 26 at 15:12
1
@LukeGarrigan well, you would usecollection.contains(obj)
instead of looping over the collection to test each element for equality, wouldn’t you? Socollection.stream().anyMatch(condition)
is just a generalization of the idea, when the condition is more complex than plain equality.
– Holger
Nov 26 at 15:23
|
show 2 more comments
up vote
5
down vote
Alternatively with Java9 and above you can use Stream.ofNullable
and update as:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs) {
return Stream.ofNullable(studentConfigs)
.flatMap(Collection::stream)
.anyMatch(studentConfig -> studentConfig.action() == null || !studentConfig.action().equals(Action.DELETE));
}
4
It’s debatable whetherStream.ofNullable(studentConfigs) .flatMap(Collection::stream) …
is simpler thanstudentConfigs != null && studentConfigs.stream() …
– Holger
Nov 26 at 12:54
@Holger By simpler here you meant readability aspect or performance as well? Readability I believe would be opinion based, I find it easy to read now, maybe sometimes back I wouldn't have.
– nullpointer
Nov 26 at 13:06
2
I only meant readability when I wrote the comment, but now that you’re asking… There is the lack-of-laziness issue withflatMap
which still exists in Java 9, which would be a reason not to use your variant before Java 10. But in recent versions, I wouldn’t think about any remaining minor performance differences.
– Holger
Nov 26 at 13:13
add a comment |
up vote
4
down vote
I don't recommend you use Stream API here. Look at how clear and simple the foreach
version is:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigurations) {
if(studentConfigurations == null) {
return false;
}
for (StudentConfiguration configuration : studentConfigurations) {
if (!Action.DELETE.equals(configuration.action())) {
return true;
}
}
return false;
}
Otherwise, if you are a fanatic guy,
private boolean isActionAvailable(Collection<StudentConfiguration> configs) {
return configs != null &&
configs.stream()
.map(StudentConfiguration::action)
.anyMatch(Predicate.isEqual(Action.DELETE).negate()));
}
add a comment |
up vote
1
down vote
The return
statement in your lambda will terminate that lambda, not the isActionAvailable()
method. Therefore, the inferred type of the lambda is now wrong, because forEach
expects a Consumer
.
See the other answers for how to solve that problem.
add a comment |
up vote
1
down vote
This is signature of forEach() method forEach(Consumer<? super T> action)
.
It takes reference of Consumer interface which has method void accept(T t)
.
In your code you are overriding accept()
and returning a value which is not valid as accept()
has void return type.
Therefore it is showing error
error: incompatible types: unexpected return value
studentConfigs.forEach(studentConfig ->
add a comment |
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
10
down vote
accepted
The lambda expression passed to forEach
shouldn't have a return value.
It looks like you want to return true
if any of the elements of the input Collection
satisfies a condition:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs){
if(studentConfigs != null) {
if (studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE))) {
return true;
}
}
return false;
}
As Holger suggested, this can be reduced to a single statement:
return studentConfigs != null && studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE));
or
return studentConfigs != null ? studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE)) : false;
2
Why not a simple enhanced forloop? When do you choose one over the other?
– Luke Garrigan
Nov 26 at 12:22
1
@LukeGarrigan Enhanced for loop would also be fine. As to which one to choose, I'd go for the one more readable to you (and to other developers that may read your code). I think usinganyMatch
in this example better expresses what the method is doing, which is to find whether any of the elements of the input collection satisfies some condition.
– Eran
Nov 26 at 12:26
1
@LukeGarrigan Java is an evolving language. Higher order functions are working their way in. I would suggest you embrace them, rather than get left behind
– Alexander
Nov 26 at 14:16
1
@LukeGarrigan "I suppose it's just because I don't use it as often as I potentially should." Yep, pretty much. This is the future of Java, so you'll only see more and more of these higher order functions.
– Alexander
Nov 26 at 15:12
1
@LukeGarrigan well, you would usecollection.contains(obj)
instead of looping over the collection to test each element for equality, wouldn’t you? Socollection.stream().anyMatch(condition)
is just a generalization of the idea, when the condition is more complex than plain equality.
– Holger
Nov 26 at 15:23
|
show 2 more comments
up vote
10
down vote
accepted
The lambda expression passed to forEach
shouldn't have a return value.
It looks like you want to return true
if any of the elements of the input Collection
satisfies a condition:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs){
if(studentConfigs != null) {
if (studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE))) {
return true;
}
}
return false;
}
As Holger suggested, this can be reduced to a single statement:
return studentConfigs != null && studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE));
or
return studentConfigs != null ? studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE)) : false;
2
Why not a simple enhanced forloop? When do you choose one over the other?
– Luke Garrigan
Nov 26 at 12:22
1
@LukeGarrigan Enhanced for loop would also be fine. As to which one to choose, I'd go for the one more readable to you (and to other developers that may read your code). I think usinganyMatch
in this example better expresses what the method is doing, which is to find whether any of the elements of the input collection satisfies some condition.
– Eran
Nov 26 at 12:26
1
@LukeGarrigan Java is an evolving language. Higher order functions are working their way in. I would suggest you embrace them, rather than get left behind
– Alexander
Nov 26 at 14:16
1
@LukeGarrigan "I suppose it's just because I don't use it as often as I potentially should." Yep, pretty much. This is the future of Java, so you'll only see more and more of these higher order functions.
– Alexander
Nov 26 at 15:12
1
@LukeGarrigan well, you would usecollection.contains(obj)
instead of looping over the collection to test each element for equality, wouldn’t you? Socollection.stream().anyMatch(condition)
is just a generalization of the idea, when the condition is more complex than plain equality.
– Holger
Nov 26 at 15:23
|
show 2 more comments
up vote
10
down vote
accepted
up vote
10
down vote
accepted
The lambda expression passed to forEach
shouldn't have a return value.
It looks like you want to return true
if any of the elements of the input Collection
satisfies a condition:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs){
if(studentConfigs != null) {
if (studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE))) {
return true;
}
}
return false;
}
As Holger suggested, this can be reduced to a single statement:
return studentConfigs != null && studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE));
or
return studentConfigs != null ? studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE)) : false;
The lambda expression passed to forEach
shouldn't have a return value.
It looks like you want to return true
if any of the elements of the input Collection
satisfies a condition:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs){
if(studentConfigs != null) {
if (studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE))) {
return true;
}
}
return false;
}
As Holger suggested, this can be reduced to a single statement:
return studentConfigs != null && studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE));
or
return studentConfigs != null ? studentConfigs.stream().anyMatch(sc -> sc.action() == null || !sc.action().equals(Action.DELETE)) : false;
edited Nov 26 at 12:55
answered Nov 26 at 12:19


Eran
275k36442523
275k36442523
2
Why not a simple enhanced forloop? When do you choose one over the other?
– Luke Garrigan
Nov 26 at 12:22
1
@LukeGarrigan Enhanced for loop would also be fine. As to which one to choose, I'd go for the one more readable to you (and to other developers that may read your code). I think usinganyMatch
in this example better expresses what the method is doing, which is to find whether any of the elements of the input collection satisfies some condition.
– Eran
Nov 26 at 12:26
1
@LukeGarrigan Java is an evolving language. Higher order functions are working their way in. I would suggest you embrace them, rather than get left behind
– Alexander
Nov 26 at 14:16
1
@LukeGarrigan "I suppose it's just because I don't use it as often as I potentially should." Yep, pretty much. This is the future of Java, so you'll only see more and more of these higher order functions.
– Alexander
Nov 26 at 15:12
1
@LukeGarrigan well, you would usecollection.contains(obj)
instead of looping over the collection to test each element for equality, wouldn’t you? Socollection.stream().anyMatch(condition)
is just a generalization of the idea, when the condition is more complex than plain equality.
– Holger
Nov 26 at 15:23
|
show 2 more comments
2
Why not a simple enhanced forloop? When do you choose one over the other?
– Luke Garrigan
Nov 26 at 12:22
1
@LukeGarrigan Enhanced for loop would also be fine. As to which one to choose, I'd go for the one more readable to you (and to other developers that may read your code). I think usinganyMatch
in this example better expresses what the method is doing, which is to find whether any of the elements of the input collection satisfies some condition.
– Eran
Nov 26 at 12:26
1
@LukeGarrigan Java is an evolving language. Higher order functions are working their way in. I would suggest you embrace them, rather than get left behind
– Alexander
Nov 26 at 14:16
1
@LukeGarrigan "I suppose it's just because I don't use it as often as I potentially should." Yep, pretty much. This is the future of Java, so you'll only see more and more of these higher order functions.
– Alexander
Nov 26 at 15:12
1
@LukeGarrigan well, you would usecollection.contains(obj)
instead of looping over the collection to test each element for equality, wouldn’t you? Socollection.stream().anyMatch(condition)
is just a generalization of the idea, when the condition is more complex than plain equality.
– Holger
Nov 26 at 15:23
2
2
Why not a simple enhanced forloop? When do you choose one over the other?
– Luke Garrigan
Nov 26 at 12:22
Why not a simple enhanced forloop? When do you choose one over the other?
– Luke Garrigan
Nov 26 at 12:22
1
1
@LukeGarrigan Enhanced for loop would also be fine. As to which one to choose, I'd go for the one more readable to you (and to other developers that may read your code). I think using
anyMatch
in this example better expresses what the method is doing, which is to find whether any of the elements of the input collection satisfies some condition.– Eran
Nov 26 at 12:26
@LukeGarrigan Enhanced for loop would also be fine. As to which one to choose, I'd go for the one more readable to you (and to other developers that may read your code). I think using
anyMatch
in this example better expresses what the method is doing, which is to find whether any of the elements of the input collection satisfies some condition.– Eran
Nov 26 at 12:26
1
1
@LukeGarrigan Java is an evolving language. Higher order functions are working their way in. I would suggest you embrace them, rather than get left behind
– Alexander
Nov 26 at 14:16
@LukeGarrigan Java is an evolving language. Higher order functions are working their way in. I would suggest you embrace them, rather than get left behind
– Alexander
Nov 26 at 14:16
1
1
@LukeGarrigan "I suppose it's just because I don't use it as often as I potentially should." Yep, pretty much. This is the future of Java, so you'll only see more and more of these higher order functions.
– Alexander
Nov 26 at 15:12
@LukeGarrigan "I suppose it's just because I don't use it as often as I potentially should." Yep, pretty much. This is the future of Java, so you'll only see more and more of these higher order functions.
– Alexander
Nov 26 at 15:12
1
1
@LukeGarrigan well, you would use
collection.contains(obj)
instead of looping over the collection to test each element for equality, wouldn’t you? So collection.stream().anyMatch(condition)
is just a generalization of the idea, when the condition is more complex than plain equality.– Holger
Nov 26 at 15:23
@LukeGarrigan well, you would use
collection.contains(obj)
instead of looping over the collection to test each element for equality, wouldn’t you? So collection.stream().anyMatch(condition)
is just a generalization of the idea, when the condition is more complex than plain equality.– Holger
Nov 26 at 15:23
|
show 2 more comments
up vote
5
down vote
Alternatively with Java9 and above you can use Stream.ofNullable
and update as:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs) {
return Stream.ofNullable(studentConfigs)
.flatMap(Collection::stream)
.anyMatch(studentConfig -> studentConfig.action() == null || !studentConfig.action().equals(Action.DELETE));
}
4
It’s debatable whetherStream.ofNullable(studentConfigs) .flatMap(Collection::stream) …
is simpler thanstudentConfigs != null && studentConfigs.stream() …
– Holger
Nov 26 at 12:54
@Holger By simpler here you meant readability aspect or performance as well? Readability I believe would be opinion based, I find it easy to read now, maybe sometimes back I wouldn't have.
– nullpointer
Nov 26 at 13:06
2
I only meant readability when I wrote the comment, but now that you’re asking… There is the lack-of-laziness issue withflatMap
which still exists in Java 9, which would be a reason not to use your variant before Java 10. But in recent versions, I wouldn’t think about any remaining minor performance differences.
– Holger
Nov 26 at 13:13
add a comment |
up vote
5
down vote
Alternatively with Java9 and above you can use Stream.ofNullable
and update as:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs) {
return Stream.ofNullable(studentConfigs)
.flatMap(Collection::stream)
.anyMatch(studentConfig -> studentConfig.action() == null || !studentConfig.action().equals(Action.DELETE));
}
4
It’s debatable whetherStream.ofNullable(studentConfigs) .flatMap(Collection::stream) …
is simpler thanstudentConfigs != null && studentConfigs.stream() …
– Holger
Nov 26 at 12:54
@Holger By simpler here you meant readability aspect or performance as well? Readability I believe would be opinion based, I find it easy to read now, maybe sometimes back I wouldn't have.
– nullpointer
Nov 26 at 13:06
2
I only meant readability when I wrote the comment, but now that you’re asking… There is the lack-of-laziness issue withflatMap
which still exists in Java 9, which would be a reason not to use your variant before Java 10. But in recent versions, I wouldn’t think about any remaining minor performance differences.
– Holger
Nov 26 at 13:13
add a comment |
up vote
5
down vote
up vote
5
down vote
Alternatively with Java9 and above you can use Stream.ofNullable
and update as:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs) {
return Stream.ofNullable(studentConfigs)
.flatMap(Collection::stream)
.anyMatch(studentConfig -> studentConfig.action() == null || !studentConfig.action().equals(Action.DELETE));
}
Alternatively with Java9 and above you can use Stream.ofNullable
and update as:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigs) {
return Stream.ofNullable(studentConfigs)
.flatMap(Collection::stream)
.anyMatch(studentConfig -> studentConfig.action() == null || !studentConfig.action().equals(Action.DELETE));
}
answered Nov 26 at 12:33


nullpointer
36.9k1072144
36.9k1072144
4
It’s debatable whetherStream.ofNullable(studentConfigs) .flatMap(Collection::stream) …
is simpler thanstudentConfigs != null && studentConfigs.stream() …
– Holger
Nov 26 at 12:54
@Holger By simpler here you meant readability aspect or performance as well? Readability I believe would be opinion based, I find it easy to read now, maybe sometimes back I wouldn't have.
– nullpointer
Nov 26 at 13:06
2
I only meant readability when I wrote the comment, but now that you’re asking… There is the lack-of-laziness issue withflatMap
which still exists in Java 9, which would be a reason not to use your variant before Java 10. But in recent versions, I wouldn’t think about any remaining minor performance differences.
– Holger
Nov 26 at 13:13
add a comment |
4
It’s debatable whetherStream.ofNullable(studentConfigs) .flatMap(Collection::stream) …
is simpler thanstudentConfigs != null && studentConfigs.stream() …
– Holger
Nov 26 at 12:54
@Holger By simpler here you meant readability aspect or performance as well? Readability I believe would be opinion based, I find it easy to read now, maybe sometimes back I wouldn't have.
– nullpointer
Nov 26 at 13:06
2
I only meant readability when I wrote the comment, but now that you’re asking… There is the lack-of-laziness issue withflatMap
which still exists in Java 9, which would be a reason not to use your variant before Java 10. But in recent versions, I wouldn’t think about any remaining minor performance differences.
– Holger
Nov 26 at 13:13
4
4
It’s debatable whether
Stream.ofNullable(studentConfigs) .flatMap(Collection::stream) …
is simpler than studentConfigs != null && studentConfigs.stream() …
– Holger
Nov 26 at 12:54
It’s debatable whether
Stream.ofNullable(studentConfigs) .flatMap(Collection::stream) …
is simpler than studentConfigs != null && studentConfigs.stream() …
– Holger
Nov 26 at 12:54
@Holger By simpler here you meant readability aspect or performance as well? Readability I believe would be opinion based, I find it easy to read now, maybe sometimes back I wouldn't have.
– nullpointer
Nov 26 at 13:06
@Holger By simpler here you meant readability aspect or performance as well? Readability I believe would be opinion based, I find it easy to read now, maybe sometimes back I wouldn't have.
– nullpointer
Nov 26 at 13:06
2
2
I only meant readability when I wrote the comment, but now that you’re asking… There is the lack-of-laziness issue with
flatMap
which still exists in Java 9, which would be a reason not to use your variant before Java 10. But in recent versions, I wouldn’t think about any remaining minor performance differences.– Holger
Nov 26 at 13:13
I only meant readability when I wrote the comment, but now that you’re asking… There is the lack-of-laziness issue with
flatMap
which still exists in Java 9, which would be a reason not to use your variant before Java 10. But in recent versions, I wouldn’t think about any remaining minor performance differences.– Holger
Nov 26 at 13:13
add a comment |
up vote
4
down vote
I don't recommend you use Stream API here. Look at how clear and simple the foreach
version is:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigurations) {
if(studentConfigurations == null) {
return false;
}
for (StudentConfiguration configuration : studentConfigurations) {
if (!Action.DELETE.equals(configuration.action())) {
return true;
}
}
return false;
}
Otherwise, if you are a fanatic guy,
private boolean isActionAvailable(Collection<StudentConfiguration> configs) {
return configs != null &&
configs.stream()
.map(StudentConfiguration::action)
.anyMatch(Predicate.isEqual(Action.DELETE).negate()));
}
add a comment |
up vote
4
down vote
I don't recommend you use Stream API here. Look at how clear and simple the foreach
version is:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigurations) {
if(studentConfigurations == null) {
return false;
}
for (StudentConfiguration configuration : studentConfigurations) {
if (!Action.DELETE.equals(configuration.action())) {
return true;
}
}
return false;
}
Otherwise, if you are a fanatic guy,
private boolean isActionAvailable(Collection<StudentConfiguration> configs) {
return configs != null &&
configs.stream()
.map(StudentConfiguration::action)
.anyMatch(Predicate.isEqual(Action.DELETE).negate()));
}
add a comment |
up vote
4
down vote
up vote
4
down vote
I don't recommend you use Stream API here. Look at how clear and simple the foreach
version is:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigurations) {
if(studentConfigurations == null) {
return false;
}
for (StudentConfiguration configuration : studentConfigurations) {
if (!Action.DELETE.equals(configuration.action())) {
return true;
}
}
return false;
}
Otherwise, if you are a fanatic guy,
private boolean isActionAvailable(Collection<StudentConfiguration> configs) {
return configs != null &&
configs.stream()
.map(StudentConfiguration::action)
.anyMatch(Predicate.isEqual(Action.DELETE).negate()));
}
I don't recommend you use Stream API here. Look at how clear and simple the foreach
version is:
private boolean isActionAvailable(Collection<StudentConfiguration> studentConfigurations) {
if(studentConfigurations == null) {
return false;
}
for (StudentConfiguration configuration : studentConfigurations) {
if (!Action.DELETE.equals(configuration.action())) {
return true;
}
}
return false;
}
Otherwise, if you are a fanatic guy,
private boolean isActionAvailable(Collection<StudentConfiguration> configs) {
return configs != null &&
configs.stream()
.map(StudentConfiguration::action)
.anyMatch(Predicate.isEqual(Action.DELETE).negate()));
}
edited Nov 26 at 12:57
answered Nov 26 at 12:45


Andrew Tobilko
24k84079
24k84079
add a comment |
add a comment |
up vote
1
down vote
The return
statement in your lambda will terminate that lambda, not the isActionAvailable()
method. Therefore, the inferred type of the lambda is now wrong, because forEach
expects a Consumer
.
See the other answers for how to solve that problem.
add a comment |
up vote
1
down vote
The return
statement in your lambda will terminate that lambda, not the isActionAvailable()
method. Therefore, the inferred type of the lambda is now wrong, because forEach
expects a Consumer
.
See the other answers for how to solve that problem.
add a comment |
up vote
1
down vote
up vote
1
down vote
The return
statement in your lambda will terminate that lambda, not the isActionAvailable()
method. Therefore, the inferred type of the lambda is now wrong, because forEach
expects a Consumer
.
See the other answers for how to solve that problem.
The return
statement in your lambda will terminate that lambda, not the isActionAvailable()
method. Therefore, the inferred type of the lambda is now wrong, because forEach
expects a Consumer
.
See the other answers for how to solve that problem.
answered Nov 26 at 12:50
ohlec
1,695717
1,695717
add a comment |
add a comment |
up vote
1
down vote
This is signature of forEach() method forEach(Consumer<? super T> action)
.
It takes reference of Consumer interface which has method void accept(T t)
.
In your code you are overriding accept()
and returning a value which is not valid as accept()
has void return type.
Therefore it is showing error
error: incompatible types: unexpected return value
studentConfigs.forEach(studentConfig ->
add a comment |
up vote
1
down vote
This is signature of forEach() method forEach(Consumer<? super T> action)
.
It takes reference of Consumer interface which has method void accept(T t)
.
In your code you are overriding accept()
and returning a value which is not valid as accept()
has void return type.
Therefore it is showing error
error: incompatible types: unexpected return value
studentConfigs.forEach(studentConfig ->
add a comment |
up vote
1
down vote
up vote
1
down vote
This is signature of forEach() method forEach(Consumer<? super T> action)
.
It takes reference of Consumer interface which has method void accept(T t)
.
In your code you are overriding accept()
and returning a value which is not valid as accept()
has void return type.
Therefore it is showing error
error: incompatible types: unexpected return value
studentConfigs.forEach(studentConfig ->
This is signature of forEach() method forEach(Consumer<? super T> action)
.
It takes reference of Consumer interface which has method void accept(T t)
.
In your code you are overriding accept()
and returning a value which is not valid as accept()
has void return type.
Therefore it is showing error
error: incompatible types: unexpected return value
studentConfigs.forEach(studentConfig ->
answered Nov 26 at 12:56
Tarun
631414
631414
add a comment |
add a comment |
LEAlo5U,MHp1,Ynr Z0D0RY46bcw4afi5,jp05MRMsV 1MXhQRiYOktMCaQlEs680jA,2o4WemycdbGmwXUKLTFO dtCLeZy2Q1U3QMIK8