How to output permuations of 0's and 1's of a given length recursively?
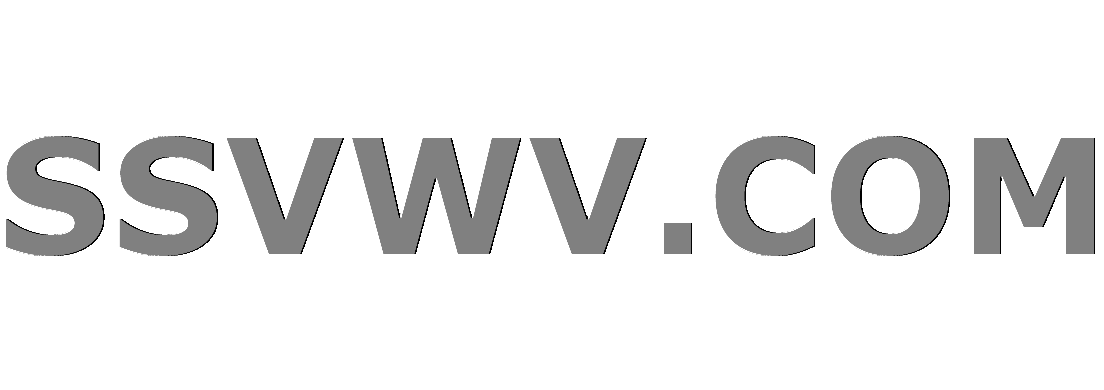
Multi tool use
up vote
-2
down vote
favorite
I am trying to print permutations of 0's and 1's recursively in Python.
I understand there is a permutations function in itertools, but was wondering how one could do it recursively, for e.g.
function name is print_01(k)
# ...
print(permutation)
# ...
...where k is the length of each permutation to be printed, so if you call it as print_01(2)
, the output would be something like this:
00
01
10
11
The output is always of length k.
How could I get this done recursively using print
?
python python-3.x recursion permutation
add a comment |
up vote
-2
down vote
favorite
I am trying to print permutations of 0's and 1's recursively in Python.
I understand there is a permutations function in itertools, but was wondering how one could do it recursively, for e.g.
function name is print_01(k)
# ...
print(permutation)
# ...
...where k is the length of each permutation to be printed, so if you call it as print_01(2)
, the output would be something like this:
00
01
10
11
The output is always of length k.
How could I get this done recursively using print
?
python python-3.x recursion permutation
I'm not sure if it's such a good idea to use a recursion due to the (maximal) recursion depth.. .
– bene
Nov 19 at 14:52
add a comment |
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
I am trying to print permutations of 0's and 1's recursively in Python.
I understand there is a permutations function in itertools, but was wondering how one could do it recursively, for e.g.
function name is print_01(k)
# ...
print(permutation)
# ...
...where k is the length of each permutation to be printed, so if you call it as print_01(2)
, the output would be something like this:
00
01
10
11
The output is always of length k.
How could I get this done recursively using print
?
python python-3.x recursion permutation
I am trying to print permutations of 0's and 1's recursively in Python.
I understand there is a permutations function in itertools, but was wondering how one could do it recursively, for e.g.
function name is print_01(k)
# ...
print(permutation)
# ...
...where k is the length of each permutation to be printed, so if you call it as print_01(2)
, the output would be something like this:
00
01
10
11
The output is always of length k.
How could I get this done recursively using print
?
python python-3.x recursion permutation
python python-3.x recursion permutation
edited Nov 23 at 20:18


trincot
115k1478109
115k1478109
asked Nov 19 at 14:50
computerprogram
31
31
I'm not sure if it's such a good idea to use a recursion due to the (maximal) recursion depth.. .
– bene
Nov 19 at 14:52
add a comment |
I'm not sure if it's such a good idea to use a recursion due to the (maximal) recursion depth.. .
– bene
Nov 19 at 14:52
I'm not sure if it's such a good idea to use a recursion due to the (maximal) recursion depth.. .
– bene
Nov 19 at 14:52
I'm not sure if it's such a good idea to use a recursion due to the (maximal) recursion depth.. .
– bene
Nov 19 at 14:52
add a comment |
5 Answers
5
active
oldest
votes
up vote
2
down vote
accepted
Without giving away the code, I'll attempt to give the hints needed for you to come up with the code.
The idea is to make the recursive call to add one more digit to an accumulated string, that initially is empty.
The so-called "base case" of the recursion is where the accumulated string has the desired length. This is where you would output it (or store it somewhere)
You'll need a loop to visit the two possible digits.
Let me know if this is enough for you to get going.
Spoiler alert (only look below after having tried):
thank you for posting that code, is there a link where i can read about how that for loop works?
– computerprogram
Nov 19 at 15:40
The loop just iterates through the string "01", taking each character in turn. Sodigit
will first be "0" and in the second (last) iteration it will be "1"
– trincot
Nov 19 at 15:41
add a comment |
up vote
2
down vote
Here is an idea:
Instead of doing that recursively, use binary development of numbers:
def print_01(k):
end = 1<<k #this is the integer with binary developpement 1 followed by k zeros
for j in range(end): # iterate until end means getting all k - 0-1 combinations
comb = bin(j)[2:].zfill(k)
print [int(x) for x in comb]
add a comment |
up vote
0
down vote
Do you really need to permute ... ?
If not try below
def print_01(length):
for i in range(1 << length):
print(format(i, '0{}b'.format(length)))
def main():
'''The Main'''
print_01(2)
print_01(3)
if __name__ == '__main__':
main()
add a comment |
up vote
0
down vote
Here's a simple recursive version:
#!/usr/bin/python -E
#string of binary 1s and 0s, all possible combinations for a given length
def swap(string, loc, val):
newstring = string[:loc]+val+string[loc+1:]
return newstring
def printperms(string,n):
length = len(string)
if n > 0:
string = swap(string, (length - n), "0")
printperms(string, (n -1))
string = swap(string, (length - n), "1")
printperms(string, (n -1))
else:
print(string)
mystring = "000"
length = len(mystring)
printperms(mystring, length)
mystring = mystring+" "
print("############################################")
printperms(mystring,length+1)
add a comment |
up vote
0
down vote
Let's keep it simple:
def print_01(bits, n=0):
if n.bit_length() <= bits:
print('{:0{}b}'.format(n, bits))
print_01(bits, n + 1)
The int
type has a method bit_length()
that tells you the minimum number of binary characters needed to represent this number -- we use that for control. The nested formatting allows us to pass the output width as a variable.
USAGE
>>> print_01(3)
000
001
010
011
100
101
110
111
>>>
add a comment |
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Without giving away the code, I'll attempt to give the hints needed for you to come up with the code.
The idea is to make the recursive call to add one more digit to an accumulated string, that initially is empty.
The so-called "base case" of the recursion is where the accumulated string has the desired length. This is where you would output it (or store it somewhere)
You'll need a loop to visit the two possible digits.
Let me know if this is enough for you to get going.
Spoiler alert (only look below after having tried):
thank you for posting that code, is there a link where i can read about how that for loop works?
– computerprogram
Nov 19 at 15:40
The loop just iterates through the string "01", taking each character in turn. Sodigit
will first be "0" and in the second (last) iteration it will be "1"
– trincot
Nov 19 at 15:41
add a comment |
up vote
2
down vote
accepted
Without giving away the code, I'll attempt to give the hints needed for you to come up with the code.
The idea is to make the recursive call to add one more digit to an accumulated string, that initially is empty.
The so-called "base case" of the recursion is where the accumulated string has the desired length. This is where you would output it (or store it somewhere)
You'll need a loop to visit the two possible digits.
Let me know if this is enough for you to get going.
Spoiler alert (only look below after having tried):
thank you for posting that code, is there a link where i can read about how that for loop works?
– computerprogram
Nov 19 at 15:40
The loop just iterates through the string "01", taking each character in turn. Sodigit
will first be "0" and in the second (last) iteration it will be "1"
– trincot
Nov 19 at 15:41
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Without giving away the code, I'll attempt to give the hints needed for you to come up with the code.
The idea is to make the recursive call to add one more digit to an accumulated string, that initially is empty.
The so-called "base case" of the recursion is where the accumulated string has the desired length. This is where you would output it (or store it somewhere)
You'll need a loop to visit the two possible digits.
Let me know if this is enough for you to get going.
Spoiler alert (only look below after having tried):
Without giving away the code, I'll attempt to give the hints needed for you to come up with the code.
The idea is to make the recursive call to add one more digit to an accumulated string, that initially is empty.
The so-called "base case" of the recursion is where the accumulated string has the desired length. This is where you would output it (or store it somewhere)
You'll need a loop to visit the two possible digits.
Let me know if this is enough for you to get going.
Spoiler alert (only look below after having tried):
edited Nov 19 at 15:21
answered Nov 19 at 15:03


trincot
115k1478109
115k1478109
thank you for posting that code, is there a link where i can read about how that for loop works?
– computerprogram
Nov 19 at 15:40
The loop just iterates through the string "01", taking each character in turn. Sodigit
will first be "0" and in the second (last) iteration it will be "1"
– trincot
Nov 19 at 15:41
add a comment |
thank you for posting that code, is there a link where i can read about how that for loop works?
– computerprogram
Nov 19 at 15:40
The loop just iterates through the string "01", taking each character in turn. Sodigit
will first be "0" and in the second (last) iteration it will be "1"
– trincot
Nov 19 at 15:41
thank you for posting that code, is there a link where i can read about how that for loop works?
– computerprogram
Nov 19 at 15:40
thank you for posting that code, is there a link where i can read about how that for loop works?
– computerprogram
Nov 19 at 15:40
The loop just iterates through the string "01", taking each character in turn. So
digit
will first be "0" and in the second (last) iteration it will be "1"– trincot
Nov 19 at 15:41
The loop just iterates through the string "01", taking each character in turn. So
digit
will first be "0" and in the second (last) iteration it will be "1"– trincot
Nov 19 at 15:41
add a comment |
up vote
2
down vote
Here is an idea:
Instead of doing that recursively, use binary development of numbers:
def print_01(k):
end = 1<<k #this is the integer with binary developpement 1 followed by k zeros
for j in range(end): # iterate until end means getting all k - 0-1 combinations
comb = bin(j)[2:].zfill(k)
print [int(x) for x in comb]
add a comment |
up vote
2
down vote
Here is an idea:
Instead of doing that recursively, use binary development of numbers:
def print_01(k):
end = 1<<k #this is the integer with binary developpement 1 followed by k zeros
for j in range(end): # iterate until end means getting all k - 0-1 combinations
comb = bin(j)[2:].zfill(k)
print [int(x) for x in comb]
add a comment |
up vote
2
down vote
up vote
2
down vote
Here is an idea:
Instead of doing that recursively, use binary development of numbers:
def print_01(k):
end = 1<<k #this is the integer with binary developpement 1 followed by k zeros
for j in range(end): # iterate until end means getting all k - 0-1 combinations
comb = bin(j)[2:].zfill(k)
print [int(x) for x in comb]
Here is an idea:
Instead of doing that recursively, use binary development of numbers:
def print_01(k):
end = 1<<k #this is the integer with binary developpement 1 followed by k zeros
for j in range(end): # iterate until end means getting all k - 0-1 combinations
comb = bin(j)[2:].zfill(k)
print [int(x) for x in comb]
edited Nov 19 at 15:11
answered Nov 19 at 15:05
Matina G
50119
50119
add a comment |
add a comment |
up vote
0
down vote
Do you really need to permute ... ?
If not try below
def print_01(length):
for i in range(1 << length):
print(format(i, '0{}b'.format(length)))
def main():
'''The Main'''
print_01(2)
print_01(3)
if __name__ == '__main__':
main()
add a comment |
up vote
0
down vote
Do you really need to permute ... ?
If not try below
def print_01(length):
for i in range(1 << length):
print(format(i, '0{}b'.format(length)))
def main():
'''The Main'''
print_01(2)
print_01(3)
if __name__ == '__main__':
main()
add a comment |
up vote
0
down vote
up vote
0
down vote
Do you really need to permute ... ?
If not try below
def print_01(length):
for i in range(1 << length):
print(format(i, '0{}b'.format(length)))
def main():
'''The Main'''
print_01(2)
print_01(3)
if __name__ == '__main__':
main()
Do you really need to permute ... ?
If not try below
def print_01(length):
for i in range(1 << length):
print(format(i, '0{}b'.format(length)))
def main():
'''The Main'''
print_01(2)
print_01(3)
if __name__ == '__main__':
main()
answered Nov 19 at 15:06


Krishna
5921315
5921315
add a comment |
add a comment |
up vote
0
down vote
Here's a simple recursive version:
#!/usr/bin/python -E
#string of binary 1s and 0s, all possible combinations for a given length
def swap(string, loc, val):
newstring = string[:loc]+val+string[loc+1:]
return newstring
def printperms(string,n):
length = len(string)
if n > 0:
string = swap(string, (length - n), "0")
printperms(string, (n -1))
string = swap(string, (length - n), "1")
printperms(string, (n -1))
else:
print(string)
mystring = "000"
length = len(mystring)
printperms(mystring, length)
mystring = mystring+" "
print("############################################")
printperms(mystring,length+1)
add a comment |
up vote
0
down vote
Here's a simple recursive version:
#!/usr/bin/python -E
#string of binary 1s and 0s, all possible combinations for a given length
def swap(string, loc, val):
newstring = string[:loc]+val+string[loc+1:]
return newstring
def printperms(string,n):
length = len(string)
if n > 0:
string = swap(string, (length - n), "0")
printperms(string, (n -1))
string = swap(string, (length - n), "1")
printperms(string, (n -1))
else:
print(string)
mystring = "000"
length = len(mystring)
printperms(mystring, length)
mystring = mystring+" "
print("############################################")
printperms(mystring,length+1)
add a comment |
up vote
0
down vote
up vote
0
down vote
Here's a simple recursive version:
#!/usr/bin/python -E
#string of binary 1s and 0s, all possible combinations for a given length
def swap(string, loc, val):
newstring = string[:loc]+val+string[loc+1:]
return newstring
def printperms(string,n):
length = len(string)
if n > 0:
string = swap(string, (length - n), "0")
printperms(string, (n -1))
string = swap(string, (length - n), "1")
printperms(string, (n -1))
else:
print(string)
mystring = "000"
length = len(mystring)
printperms(mystring, length)
mystring = mystring+" "
print("############################################")
printperms(mystring,length+1)
Here's a simple recursive version:
#!/usr/bin/python -E
#string of binary 1s and 0s, all possible combinations for a given length
def swap(string, loc, val):
newstring = string[:loc]+val+string[loc+1:]
return newstring
def printperms(string,n):
length = len(string)
if n > 0:
string = swap(string, (length - n), "0")
printperms(string, (n -1))
string = swap(string, (length - n), "1")
printperms(string, (n -1))
else:
print(string)
mystring = "000"
length = len(mystring)
printperms(mystring, length)
mystring = mystring+" "
print("############################################")
printperms(mystring,length+1)
answered Nov 19 at 17:03
Lulz
11
11
add a comment |
add a comment |
up vote
0
down vote
Let's keep it simple:
def print_01(bits, n=0):
if n.bit_length() <= bits:
print('{:0{}b}'.format(n, bits))
print_01(bits, n + 1)
The int
type has a method bit_length()
that tells you the minimum number of binary characters needed to represent this number -- we use that for control. The nested formatting allows us to pass the output width as a variable.
USAGE
>>> print_01(3)
000
001
010
011
100
101
110
111
>>>
add a comment |
up vote
0
down vote
Let's keep it simple:
def print_01(bits, n=0):
if n.bit_length() <= bits:
print('{:0{}b}'.format(n, bits))
print_01(bits, n + 1)
The int
type has a method bit_length()
that tells you the minimum number of binary characters needed to represent this number -- we use that for control. The nested formatting allows us to pass the output width as a variable.
USAGE
>>> print_01(3)
000
001
010
011
100
101
110
111
>>>
add a comment |
up vote
0
down vote
up vote
0
down vote
Let's keep it simple:
def print_01(bits, n=0):
if n.bit_length() <= bits:
print('{:0{}b}'.format(n, bits))
print_01(bits, n + 1)
The int
type has a method bit_length()
that tells you the minimum number of binary characters needed to represent this number -- we use that for control. The nested formatting allows us to pass the output width as a variable.
USAGE
>>> print_01(3)
000
001
010
011
100
101
110
111
>>>
Let's keep it simple:
def print_01(bits, n=0):
if n.bit_length() <= bits:
print('{:0{}b}'.format(n, bits))
print_01(bits, n + 1)
The int
type has a method bit_length()
that tells you the minimum number of binary characters needed to represent this number -- we use that for control. The nested formatting allows us to pass the output width as a variable.
USAGE
>>> print_01(3)
000
001
010
011
100
101
110
111
>>>
answered Nov 19 at 17:50
cdlane
16.8k21043
16.8k21043
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53377124%2fhow-to-output-permuations-of-0s-and-1s-of-a-given-length-recursively%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sHuPiA mgLW N4L8 OR,yEpVRn,vvlqXW5 m6w,joc4hwwWhra9 kl,KAakNX4Ld 544 Qz ohJ9XP340tbWuW1 tIyNc
I'm not sure if it's such a good idea to use a recursion due to the (maximal) recursion depth.. .
– bene
Nov 19 at 14:52