Unable to display FCM notification using 'node fcm-notification'
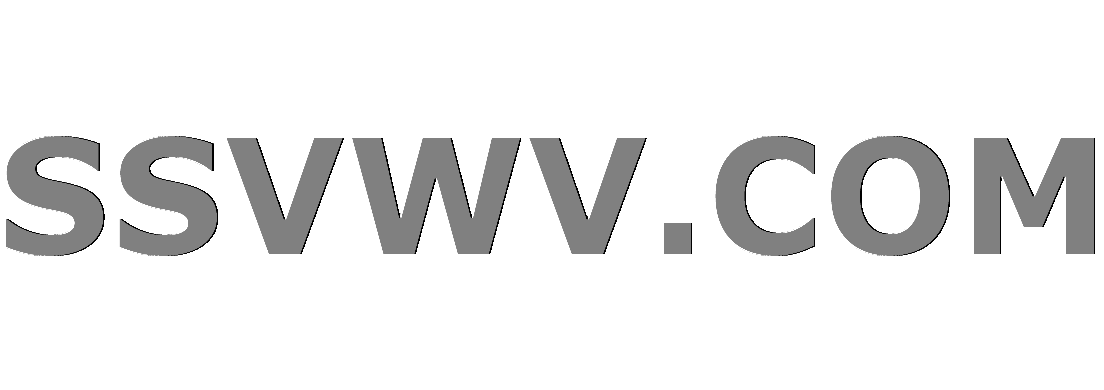
Multi tool use
up vote
0
down vote
favorite
I am working on a video calling app where a user gets notified via a notification when there is an incoming call and be able to click on the notification to start the call. Right now, the notification gets pushed when the app is closed or is running in the background, but doesn't when the app is opened in foreground.
Also, clicking on the notification when the app is in background or not running will only open the app and not start the video call. The only way I can start the call is by receiving a notification when the app is in background / not running, manually opening the application and then clicking on the notification.
Here is my code that handles the notification sending:
var fcm = require('fcm-notification');
var serverKey = require('./fcmKey.json');
var FCM = new fcm(serverKey);
const payloadBody = {
room,
caller,
language,
receiver
}
var message = {
token : deviceToken,
data : payloadBody,
notificaton: {
title : 'MyApp',
body: 'Somebody is calling'
}
FCM.send(message, function(err, response) {
if(err) {
console.error('Notification error: ${JSON.stringify(err)}');
} else {
console.error('Notification success: ${JSON.stringify(response)}');
}
}
My manifest also contains the fcm service like this:
<service android:name="com.evollu.react.fcm.MessagingService" android:enabled="true" android:exported="true">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>


add a comment |
up vote
0
down vote
favorite
I am working on a video calling app where a user gets notified via a notification when there is an incoming call and be able to click on the notification to start the call. Right now, the notification gets pushed when the app is closed or is running in the background, but doesn't when the app is opened in foreground.
Also, clicking on the notification when the app is in background or not running will only open the app and not start the video call. The only way I can start the call is by receiving a notification when the app is in background / not running, manually opening the application and then clicking on the notification.
Here is my code that handles the notification sending:
var fcm = require('fcm-notification');
var serverKey = require('./fcmKey.json');
var FCM = new fcm(serverKey);
const payloadBody = {
room,
caller,
language,
receiver
}
var message = {
token : deviceToken,
data : payloadBody,
notificaton: {
title : 'MyApp',
body: 'Somebody is calling'
}
FCM.send(message, function(err, response) {
if(err) {
console.error('Notification error: ${JSON.stringify(err)}');
} else {
console.error('Notification success: ${JSON.stringify(response)}');
}
}
My manifest also contains the fcm service like this:
<service android:name="com.evollu.react.fcm.MessagingService" android:enabled="true" android:exported="true">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>


add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am working on a video calling app where a user gets notified via a notification when there is an incoming call and be able to click on the notification to start the call. Right now, the notification gets pushed when the app is closed or is running in the background, but doesn't when the app is opened in foreground.
Also, clicking on the notification when the app is in background or not running will only open the app and not start the video call. The only way I can start the call is by receiving a notification when the app is in background / not running, manually opening the application and then clicking on the notification.
Here is my code that handles the notification sending:
var fcm = require('fcm-notification');
var serverKey = require('./fcmKey.json');
var FCM = new fcm(serverKey);
const payloadBody = {
room,
caller,
language,
receiver
}
var message = {
token : deviceToken,
data : payloadBody,
notificaton: {
title : 'MyApp',
body: 'Somebody is calling'
}
FCM.send(message, function(err, response) {
if(err) {
console.error('Notification error: ${JSON.stringify(err)}');
} else {
console.error('Notification success: ${JSON.stringify(response)}');
}
}
My manifest also contains the fcm service like this:
<service android:name="com.evollu.react.fcm.MessagingService" android:enabled="true" android:exported="true">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>


I am working on a video calling app where a user gets notified via a notification when there is an incoming call and be able to click on the notification to start the call. Right now, the notification gets pushed when the app is closed or is running in the background, but doesn't when the app is opened in foreground.
Also, clicking on the notification when the app is in background or not running will only open the app and not start the video call. The only way I can start the call is by receiving a notification when the app is in background / not running, manually opening the application and then clicking on the notification.
Here is my code that handles the notification sending:
var fcm = require('fcm-notification');
var serverKey = require('./fcmKey.json');
var FCM = new fcm(serverKey);
const payloadBody = {
room,
caller,
language,
receiver
}
var message = {
token : deviceToken,
data : payloadBody,
notificaton: {
title : 'MyApp',
body: 'Somebody is calling'
}
FCM.send(message, function(err, response) {
if(err) {
console.error('Notification error: ${JSON.stringify(err)}');
} else {
console.error('Notification success: ${JSON.stringify(response)}');
}
}
My manifest also contains the fcm service like this:
<service android:name="com.evollu.react.fcm.MessagingService" android:enabled="true" android:exported="true">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>




asked Nov 19 at 17:08


Eduard Constantinescu
1
1
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
What you're describing with respect to foreground and background behavior is exactly how notification type messages are supposed to work. If you want to take control over the behavior of the notification that gets displayed to the user, you should remove the notification payload from the message, and build the whole thing yourself in response to the data payload. You'll receive that message in a FirebaseMessagingService subclass that you create.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53379554%2funable-to-display-fcm-notification-using-node-fcm-notification%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
What you're describing with respect to foreground and background behavior is exactly how notification type messages are supposed to work. If you want to take control over the behavior of the notification that gets displayed to the user, you should remove the notification payload from the message, and build the whole thing yourself in response to the data payload. You'll receive that message in a FirebaseMessagingService subclass that you create.
add a comment |
up vote
0
down vote
What you're describing with respect to foreground and background behavior is exactly how notification type messages are supposed to work. If you want to take control over the behavior of the notification that gets displayed to the user, you should remove the notification payload from the message, and build the whole thing yourself in response to the data payload. You'll receive that message in a FirebaseMessagingService subclass that you create.
add a comment |
up vote
0
down vote
up vote
0
down vote
What you're describing with respect to foreground and background behavior is exactly how notification type messages are supposed to work. If you want to take control over the behavior of the notification that gets displayed to the user, you should remove the notification payload from the message, and build the whole thing yourself in response to the data payload. You'll receive that message in a FirebaseMessagingService subclass that you create.
What you're describing with respect to foreground and background behavior is exactly how notification type messages are supposed to work. If you want to take control over the behavior of the notification that gets displayed to the user, you should remove the notification payload from the message, and build the whole thing yourself in response to the data payload. You'll receive that message in a FirebaseMessagingService subclass that you create.
answered Nov 19 at 17:44


Doug Stevenson
68.2k880100
68.2k880100
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53379554%2funable-to-display-fcm-notification-using-node-fcm-notification%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Aq1qdgUAb