Complex transactions managment with Spring, Oracle DB and Hibernate insert XAResource failure
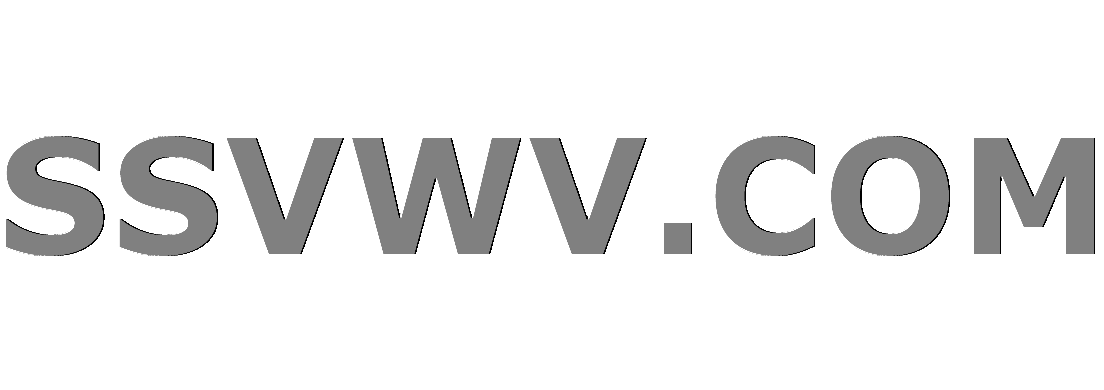
Multi tool use
Case:
A file with over 1 mln records. Read the file and store records in Oracle DB.
Solution:
Use Hibernate statelessSession interface since there is no need to use persistence context in this particular case. Load records from file in a while loop 100k every time and commit them immediately.
Implementation:
At the top I have a method loadAllDictionaries() annotated with @Transactional with a timeout of 7200.
It starts a chain of methods and get to the while loop:
while(!(recordList = loadIDictionariesPackage(reader)).isEmpty()){
processRecords(recordList, dictionaryVersion, dictionaryType, filename);
}
Now, processRecords(...) does some stuff and at some point invokes the following(notice the propagation)
@Transactional(propagation = Propagation.REQUIRES_NEW, timeout = 900) //timeout is unnecessary since it takes around 2,5 minutes.
public void saveInStatelessSession(List<T> entities) throws HibernateException {
StatelessSession session = this.getSessionFactory().openStatelessSession();
session.beginTransaction();
entities.forEach(entity -> session.insert(getEntityName(), entity));
session.getTransaction().commit();
session.close();
}
So, after the while loop is finished and over 1 mln records are stored in DB the flow returns to loadAllDictionaries() method and enters another @Transactional method addNewLog(...)
@Transactional
public void addNewLog(AbstractLogEntry message) {
final EventLogDefinition definition = logEntryDAO.getDefinitionForEntry(message);
doAddNewLog(message, definition, null);
}
Here getDefinitionForEntry() throws an exception
com.atomikos.datasource.ResourceException: XA resource 'dataSource': resume for XID '3139322E3136382E35362E312E746D313534323739343839373335343033363332:3139322E3136382E35362E312E746D33373939' raised -4: the supplied XID is invalid for this XA resource
Now, whats strange. It only occurs if ~200k or more records are saved. It doesn't matter If I change the batch from 100k to 10k which increases the number of transactions. I thought it might be the atomicos timeout but in my app context file it is set to 3000
<bean id="atomikosUserTransaction" class="com.atomikos.icatch.jta.J2eeUserTransaction" depends-on="userTransactionService">
<property name="transactionTimeout" value="3000" />
</bean>
If I delete the line
@Transactional(propagation = Propagation.REQUIRES_NEW, timeout = 900)
from saveInStatelessSession method than when loadAllDictionaries() transaction tries to commit the data I get
ERROR org.springframework.transaction.UnexpectedRollbackException: JTA transaction unexpectedly rolled back (maybe due to a timeout); nested exception is javax.transaction.RollbackException: Prepare: NO vote
Do you have any thoughts on what might be the issue here?
java spring oracle hibernate xa
add a comment |
Case:
A file with over 1 mln records. Read the file and store records in Oracle DB.
Solution:
Use Hibernate statelessSession interface since there is no need to use persistence context in this particular case. Load records from file in a while loop 100k every time and commit them immediately.
Implementation:
At the top I have a method loadAllDictionaries() annotated with @Transactional with a timeout of 7200.
It starts a chain of methods and get to the while loop:
while(!(recordList = loadIDictionariesPackage(reader)).isEmpty()){
processRecords(recordList, dictionaryVersion, dictionaryType, filename);
}
Now, processRecords(...) does some stuff and at some point invokes the following(notice the propagation)
@Transactional(propagation = Propagation.REQUIRES_NEW, timeout = 900) //timeout is unnecessary since it takes around 2,5 minutes.
public void saveInStatelessSession(List<T> entities) throws HibernateException {
StatelessSession session = this.getSessionFactory().openStatelessSession();
session.beginTransaction();
entities.forEach(entity -> session.insert(getEntityName(), entity));
session.getTransaction().commit();
session.close();
}
So, after the while loop is finished and over 1 mln records are stored in DB the flow returns to loadAllDictionaries() method and enters another @Transactional method addNewLog(...)
@Transactional
public void addNewLog(AbstractLogEntry message) {
final EventLogDefinition definition = logEntryDAO.getDefinitionForEntry(message);
doAddNewLog(message, definition, null);
}
Here getDefinitionForEntry() throws an exception
com.atomikos.datasource.ResourceException: XA resource 'dataSource': resume for XID '3139322E3136382E35362E312E746D313534323739343839373335343033363332:3139322E3136382E35362E312E746D33373939' raised -4: the supplied XID is invalid for this XA resource
Now, whats strange. It only occurs if ~200k or more records are saved. It doesn't matter If I change the batch from 100k to 10k which increases the number of transactions. I thought it might be the atomicos timeout but in my app context file it is set to 3000
<bean id="atomikosUserTransaction" class="com.atomikos.icatch.jta.J2eeUserTransaction" depends-on="userTransactionService">
<property name="transactionTimeout" value="3000" />
</bean>
If I delete the line
@Transactional(propagation = Propagation.REQUIRES_NEW, timeout = 900)
from saveInStatelessSession method than when loadAllDictionaries() transaction tries to commit the data I get
ERROR org.springframework.transaction.UnexpectedRollbackException: JTA transaction unexpectedly rolled back (maybe due to a timeout); nested exception is javax.transaction.RollbackException: Prepare: NO vote
Do you have any thoughts on what might be the issue here?
java spring oracle hibernate xa
add a comment |
Case:
A file with over 1 mln records. Read the file and store records in Oracle DB.
Solution:
Use Hibernate statelessSession interface since there is no need to use persistence context in this particular case. Load records from file in a while loop 100k every time and commit them immediately.
Implementation:
At the top I have a method loadAllDictionaries() annotated with @Transactional with a timeout of 7200.
It starts a chain of methods and get to the while loop:
while(!(recordList = loadIDictionariesPackage(reader)).isEmpty()){
processRecords(recordList, dictionaryVersion, dictionaryType, filename);
}
Now, processRecords(...) does some stuff and at some point invokes the following(notice the propagation)
@Transactional(propagation = Propagation.REQUIRES_NEW, timeout = 900) //timeout is unnecessary since it takes around 2,5 minutes.
public void saveInStatelessSession(List<T> entities) throws HibernateException {
StatelessSession session = this.getSessionFactory().openStatelessSession();
session.beginTransaction();
entities.forEach(entity -> session.insert(getEntityName(), entity));
session.getTransaction().commit();
session.close();
}
So, after the while loop is finished and over 1 mln records are stored in DB the flow returns to loadAllDictionaries() method and enters another @Transactional method addNewLog(...)
@Transactional
public void addNewLog(AbstractLogEntry message) {
final EventLogDefinition definition = logEntryDAO.getDefinitionForEntry(message);
doAddNewLog(message, definition, null);
}
Here getDefinitionForEntry() throws an exception
com.atomikos.datasource.ResourceException: XA resource 'dataSource': resume for XID '3139322E3136382E35362E312E746D313534323739343839373335343033363332:3139322E3136382E35362E312E746D33373939' raised -4: the supplied XID is invalid for this XA resource
Now, whats strange. It only occurs if ~200k or more records are saved. It doesn't matter If I change the batch from 100k to 10k which increases the number of transactions. I thought it might be the atomicos timeout but in my app context file it is set to 3000
<bean id="atomikosUserTransaction" class="com.atomikos.icatch.jta.J2eeUserTransaction" depends-on="userTransactionService">
<property name="transactionTimeout" value="3000" />
</bean>
If I delete the line
@Transactional(propagation = Propagation.REQUIRES_NEW, timeout = 900)
from saveInStatelessSession method than when loadAllDictionaries() transaction tries to commit the data I get
ERROR org.springframework.transaction.UnexpectedRollbackException: JTA transaction unexpectedly rolled back (maybe due to a timeout); nested exception is javax.transaction.RollbackException: Prepare: NO vote
Do you have any thoughts on what might be the issue here?
java spring oracle hibernate xa
Case:
A file with over 1 mln records. Read the file and store records in Oracle DB.
Solution:
Use Hibernate statelessSession interface since there is no need to use persistence context in this particular case. Load records from file in a while loop 100k every time and commit them immediately.
Implementation:
At the top I have a method loadAllDictionaries() annotated with @Transactional with a timeout of 7200.
It starts a chain of methods and get to the while loop:
while(!(recordList = loadIDictionariesPackage(reader)).isEmpty()){
processRecords(recordList, dictionaryVersion, dictionaryType, filename);
}
Now, processRecords(...) does some stuff and at some point invokes the following(notice the propagation)
@Transactional(propagation = Propagation.REQUIRES_NEW, timeout = 900) //timeout is unnecessary since it takes around 2,5 minutes.
public void saveInStatelessSession(List<T> entities) throws HibernateException {
StatelessSession session = this.getSessionFactory().openStatelessSession();
session.beginTransaction();
entities.forEach(entity -> session.insert(getEntityName(), entity));
session.getTransaction().commit();
session.close();
}
So, after the while loop is finished and over 1 mln records are stored in DB the flow returns to loadAllDictionaries() method and enters another @Transactional method addNewLog(...)
@Transactional
public void addNewLog(AbstractLogEntry message) {
final EventLogDefinition definition = logEntryDAO.getDefinitionForEntry(message);
doAddNewLog(message, definition, null);
}
Here getDefinitionForEntry() throws an exception
com.atomikos.datasource.ResourceException: XA resource 'dataSource': resume for XID '3139322E3136382E35362E312E746D313534323739343839373335343033363332:3139322E3136382E35362E312E746D33373939' raised -4: the supplied XID is invalid for this XA resource
Now, whats strange. It only occurs if ~200k or more records are saved. It doesn't matter If I change the batch from 100k to 10k which increases the number of transactions. I thought it might be the atomicos timeout but in my app context file it is set to 3000
<bean id="atomikosUserTransaction" class="com.atomikos.icatch.jta.J2eeUserTransaction" depends-on="userTransactionService">
<property name="transactionTimeout" value="3000" />
</bean>
If I delete the line
@Transactional(propagation = Propagation.REQUIRES_NEW, timeout = 900)
from saveInStatelessSession method than when loadAllDictionaries() transaction tries to commit the data I get
ERROR org.springframework.transaction.UnexpectedRollbackException: JTA transaction unexpectedly rolled back (maybe due to a timeout); nested exception is javax.transaction.RollbackException: Prepare: NO vote
Do you have any thoughts on what might be the issue here?
java spring oracle hibernate xa
java spring oracle hibernate xa
edited Nov 21 '18 at 10:55
lvi
asked Nov 21 '18 at 10:45
lvilvi
49127
49127
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53410382%2fcomplex-transactions-managment-with-spring-oracle-db-and-hibernate-insert-xares%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53410382%2fcomplex-transactions-managment-with-spring-oracle-db-and-hibernate-insert-xares%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
V,8pGbP,lzoSLk rOcml,KZXzdRcZqZ4erzR2fDH9YDHksvytdKbvz cILS