Grade calculator for a project with four aspects
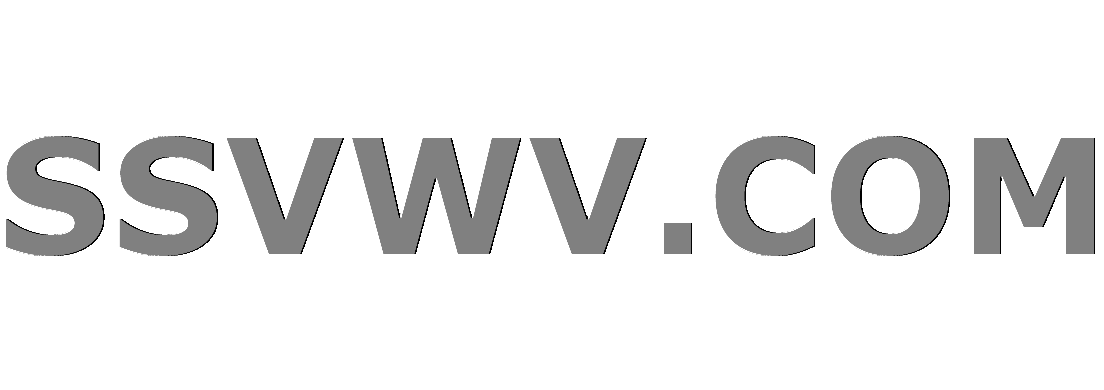
Multi tool use
I have a program that will ask the user to input their grades for 4 different sections of a project, then tell them what their total mark is, what grade they got and how many marks away they were from the next grade. I managed to make a single loop for all inputs rather than having a loop for each individual one, but there are still quite a lot of if statements to determine what grade they got and how far away they were from the next one, and I can't figure out how to optimise it since I'm still very new to Java.
import java.util.Arrays;
import java.util.InputMismatchException;
import java.util.Scanner;
public class PortfolioGrade {
public static void main(String args) {
// TODO Auto-generated method stub
String words = new String{"Analysis", "Design", "Implementation", "Evaluation"};
int marks = new int[words.length];
for(int counter = 1; counter <= words.length; counter++) {
System.out.println("Enter your mark for the '" + words[counter - 1] + "' part of the project: ");
while(true) {
try {
Scanner reader = new Scanner(System.in);
marks[counter - 1] = reader.nextInt();
if(marks[counter - 1] < 0 || marks[counter - 1] > 25) {
System.out.println("Please input a number between 0 and 25.");
continue;
}
break;
} catch(InputMismatchException e) {
System.out.println("Please input a valid integer.");
}
}
}
int totalmark = Arrays.stream(marks).sum();
String grade = null;
String nextgrade = null;
Integer marksaway = null;
if(totalmark < 2) {
grade = "U";
marksaway = 2 - totalmark;
nextgrade = "1";
} else if(totalmark >= 2 && totalmark < 4) {
grade = "1";
marksaway = 4 - totalmark;
nextgrade = "2";
} else if(totalmark >= 4 && totalmark < 13) {
grade = "2";
marksaway = 13 - totalmark;
nextgrade = "3";
} else if(totalmark >= 13 && totalmark < 22) {
grade = "3";
marksaway = 22 - totalmark;
nextgrade = "4";
} else if(totalmark >= 22 && totalmark < 31) {
grade = "4";
marksaway = 31 - totalmark;
nextgrade = "5";
} else if(totalmark >= 31 && totalmark < 41) {
grade = "5";
marksaway = 41 - totalmark;
nextgrade = "6";
} else if(totalmark >= 41 && totalmark < 54) {
grade = "6";
marksaway = 54 - totalmark;
nextgrade = "7";
} else if(totalmark >= 54 && totalmark < 67) {
grade = "7";
marksaway = 67 - totalmark;
nextgrade = "8";
} else if(totalmark >= 67 && totalmark < 80) {
grade = "8";
marksaway = 80 - totalmark;
nextgrade = "9";
} else if(totalmark >= 80) {
grade = "9";
}
System.out.println("Your total mark was " + totalmark + ".");
System.out.println("You got a Grade " + grade + ".");
if(grade == "9") {
System.out.println("You achieved the highest grade!");
} else if(marksaway == 1) {
System.out.println("You were " + marksaway + " mark away from a Grade " + nextgrade + ".");
} else {
System.out.println("You were " + marksaway + " marks away from a Grade " + nextgrade + ".");
}
}
}
java beginner
add a comment |
I have a program that will ask the user to input their grades for 4 different sections of a project, then tell them what their total mark is, what grade they got and how many marks away they were from the next grade. I managed to make a single loop for all inputs rather than having a loop for each individual one, but there are still quite a lot of if statements to determine what grade they got and how far away they were from the next one, and I can't figure out how to optimise it since I'm still very new to Java.
import java.util.Arrays;
import java.util.InputMismatchException;
import java.util.Scanner;
public class PortfolioGrade {
public static void main(String args) {
// TODO Auto-generated method stub
String words = new String{"Analysis", "Design", "Implementation", "Evaluation"};
int marks = new int[words.length];
for(int counter = 1; counter <= words.length; counter++) {
System.out.println("Enter your mark for the '" + words[counter - 1] + "' part of the project: ");
while(true) {
try {
Scanner reader = new Scanner(System.in);
marks[counter - 1] = reader.nextInt();
if(marks[counter - 1] < 0 || marks[counter - 1] > 25) {
System.out.println("Please input a number between 0 and 25.");
continue;
}
break;
} catch(InputMismatchException e) {
System.out.println("Please input a valid integer.");
}
}
}
int totalmark = Arrays.stream(marks).sum();
String grade = null;
String nextgrade = null;
Integer marksaway = null;
if(totalmark < 2) {
grade = "U";
marksaway = 2 - totalmark;
nextgrade = "1";
} else if(totalmark >= 2 && totalmark < 4) {
grade = "1";
marksaway = 4 - totalmark;
nextgrade = "2";
} else if(totalmark >= 4 && totalmark < 13) {
grade = "2";
marksaway = 13 - totalmark;
nextgrade = "3";
} else if(totalmark >= 13 && totalmark < 22) {
grade = "3";
marksaway = 22 - totalmark;
nextgrade = "4";
} else if(totalmark >= 22 && totalmark < 31) {
grade = "4";
marksaway = 31 - totalmark;
nextgrade = "5";
} else if(totalmark >= 31 && totalmark < 41) {
grade = "5";
marksaway = 41 - totalmark;
nextgrade = "6";
} else if(totalmark >= 41 && totalmark < 54) {
grade = "6";
marksaway = 54 - totalmark;
nextgrade = "7";
} else if(totalmark >= 54 && totalmark < 67) {
grade = "7";
marksaway = 67 - totalmark;
nextgrade = "8";
} else if(totalmark >= 67 && totalmark < 80) {
grade = "8";
marksaway = 80 - totalmark;
nextgrade = "9";
} else if(totalmark >= 80) {
grade = "9";
}
System.out.println("Your total mark was " + totalmark + ".");
System.out.println("You got a Grade " + grade + ".");
if(grade == "9") {
System.out.println("You achieved the highest grade!");
} else if(marksaway == 1) {
System.out.println("You were " + marksaway + " mark away from a Grade " + nextgrade + ".");
} else {
System.out.println("You were " + marksaway + " marks away from a Grade " + nextgrade + ".");
}
}
}
java beginner
add a comment |
I have a program that will ask the user to input their grades for 4 different sections of a project, then tell them what their total mark is, what grade they got and how many marks away they were from the next grade. I managed to make a single loop for all inputs rather than having a loop for each individual one, but there are still quite a lot of if statements to determine what grade they got and how far away they were from the next one, and I can't figure out how to optimise it since I'm still very new to Java.
import java.util.Arrays;
import java.util.InputMismatchException;
import java.util.Scanner;
public class PortfolioGrade {
public static void main(String args) {
// TODO Auto-generated method stub
String words = new String{"Analysis", "Design", "Implementation", "Evaluation"};
int marks = new int[words.length];
for(int counter = 1; counter <= words.length; counter++) {
System.out.println("Enter your mark for the '" + words[counter - 1] + "' part of the project: ");
while(true) {
try {
Scanner reader = new Scanner(System.in);
marks[counter - 1] = reader.nextInt();
if(marks[counter - 1] < 0 || marks[counter - 1] > 25) {
System.out.println("Please input a number between 0 and 25.");
continue;
}
break;
} catch(InputMismatchException e) {
System.out.println("Please input a valid integer.");
}
}
}
int totalmark = Arrays.stream(marks).sum();
String grade = null;
String nextgrade = null;
Integer marksaway = null;
if(totalmark < 2) {
grade = "U";
marksaway = 2 - totalmark;
nextgrade = "1";
} else if(totalmark >= 2 && totalmark < 4) {
grade = "1";
marksaway = 4 - totalmark;
nextgrade = "2";
} else if(totalmark >= 4 && totalmark < 13) {
grade = "2";
marksaway = 13 - totalmark;
nextgrade = "3";
} else if(totalmark >= 13 && totalmark < 22) {
grade = "3";
marksaway = 22 - totalmark;
nextgrade = "4";
} else if(totalmark >= 22 && totalmark < 31) {
grade = "4";
marksaway = 31 - totalmark;
nextgrade = "5";
} else if(totalmark >= 31 && totalmark < 41) {
grade = "5";
marksaway = 41 - totalmark;
nextgrade = "6";
} else if(totalmark >= 41 && totalmark < 54) {
grade = "6";
marksaway = 54 - totalmark;
nextgrade = "7";
} else if(totalmark >= 54 && totalmark < 67) {
grade = "7";
marksaway = 67 - totalmark;
nextgrade = "8";
} else if(totalmark >= 67 && totalmark < 80) {
grade = "8";
marksaway = 80 - totalmark;
nextgrade = "9";
} else if(totalmark >= 80) {
grade = "9";
}
System.out.println("Your total mark was " + totalmark + ".");
System.out.println("You got a Grade " + grade + ".");
if(grade == "9") {
System.out.println("You achieved the highest grade!");
} else if(marksaway == 1) {
System.out.println("You were " + marksaway + " mark away from a Grade " + nextgrade + ".");
} else {
System.out.println("You were " + marksaway + " marks away from a Grade " + nextgrade + ".");
}
}
}
java beginner
I have a program that will ask the user to input their grades for 4 different sections of a project, then tell them what their total mark is, what grade they got and how many marks away they were from the next grade. I managed to make a single loop for all inputs rather than having a loop for each individual one, but there are still quite a lot of if statements to determine what grade they got and how far away they were from the next one, and I can't figure out how to optimise it since I'm still very new to Java.
import java.util.Arrays;
import java.util.InputMismatchException;
import java.util.Scanner;
public class PortfolioGrade {
public static void main(String args) {
// TODO Auto-generated method stub
String words = new String{"Analysis", "Design", "Implementation", "Evaluation"};
int marks = new int[words.length];
for(int counter = 1; counter <= words.length; counter++) {
System.out.println("Enter your mark for the '" + words[counter - 1] + "' part of the project: ");
while(true) {
try {
Scanner reader = new Scanner(System.in);
marks[counter - 1] = reader.nextInt();
if(marks[counter - 1] < 0 || marks[counter - 1] > 25) {
System.out.println("Please input a number between 0 and 25.");
continue;
}
break;
} catch(InputMismatchException e) {
System.out.println("Please input a valid integer.");
}
}
}
int totalmark = Arrays.stream(marks).sum();
String grade = null;
String nextgrade = null;
Integer marksaway = null;
if(totalmark < 2) {
grade = "U";
marksaway = 2 - totalmark;
nextgrade = "1";
} else if(totalmark >= 2 && totalmark < 4) {
grade = "1";
marksaway = 4 - totalmark;
nextgrade = "2";
} else if(totalmark >= 4 && totalmark < 13) {
grade = "2";
marksaway = 13 - totalmark;
nextgrade = "3";
} else if(totalmark >= 13 && totalmark < 22) {
grade = "3";
marksaway = 22 - totalmark;
nextgrade = "4";
} else if(totalmark >= 22 && totalmark < 31) {
grade = "4";
marksaway = 31 - totalmark;
nextgrade = "5";
} else if(totalmark >= 31 && totalmark < 41) {
grade = "5";
marksaway = 41 - totalmark;
nextgrade = "6";
} else if(totalmark >= 41 && totalmark < 54) {
grade = "6";
marksaway = 54 - totalmark;
nextgrade = "7";
} else if(totalmark >= 54 && totalmark < 67) {
grade = "7";
marksaway = 67 - totalmark;
nextgrade = "8";
} else if(totalmark >= 67 && totalmark < 80) {
grade = "8";
marksaway = 80 - totalmark;
nextgrade = "9";
} else if(totalmark >= 80) {
grade = "9";
}
System.out.println("Your total mark was " + totalmark + ".");
System.out.println("You got a Grade " + grade + ".");
if(grade == "9") {
System.out.println("You achieved the highest grade!");
} else if(marksaway == 1) {
System.out.println("You were " + marksaway + " mark away from a Grade " + nextgrade + ".");
} else {
System.out.println("You were " + marksaway + " marks away from a Grade " + nextgrade + ".");
}
}
}
java beginner
java beginner
edited Dec 12 '18 at 13:59


200_success
128k15151413
128k15151413
asked Dec 12 '18 at 12:44


Gameskiller01
1549
1549
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Because there are no holes you can simply have an array of "breakpoints".
int steps = new int { 2, 4, 13, 22, 31, 41, 54, 67, 80 };
int i;
for(i=0; i<steps.length && totalmark>=steps[i]; i++);
grade = i==0 ? "U" : ""+i;
if(i<steps.length) marksaway=steps[i]-totalmark;
nextgrade=""+(i+1);
1
Would you mind giving me a fairly simple explanation as to how this works?
– Gameskiller01
Dec 12 '18 at 15:18
1
The loop loops over the array and breaks out if toolmark greater the current array-entry. I.e. totalmark=3. First loop, i is 0 and totalmark is greater than steps[0] -> continue -- Second loop, i is 1 and totalmark is not greater than steps[1] -> break out of the loop with i=1. Set grade to "1", marksaway to steps[1]-totalmark and nextgrade to "2"
– Holger
Dec 12 '18 at 16:01
add a comment |
I tidied up the first section for you a bit and then implemented the technique suggested by @Holger, all seems to work perfectly after running a few tests.
import java.util.Arrays;
import java.util.InputMismatchException;
import java.util.Scanner;
public class MarkCalculator {
public static void main(String args) {
// TODO Auto-generated method stub
String words = new String{"Analysis", "Design", "Implementation", "Evaluation"};
int steps = { 2, 4, 13, 22, 31, 41, 54, 67, 80 };
int marks = new int[4];
Scanner reader = new Scanner(System.in); // Keep instantiation of scanner outside of loop - once is enough
int totalmark = 0;
int inputMark = 0;
int marksaway = Integer.MIN_VALUE;
String grade = "";
String nextgrade = "";
for(int counter = 0; counter<words.length; counter++) {
System.out.println("Enter your mark for the '" + words[counter] + "' part of the project: ");
try {
do {
inputMark = reader.nextInt();
if(inputMark < 0 || inputMark > 25)
System.out.println("Please input a number between 0 and 25.");
else
marks[counter] = inputMark;
} while(inputMark < 0 || inputMark > 25);
} catch(InputMismatchException e) {
System.out.println("Please input a valid integer.");
reader.nextLine();
counter--;
}
}
totalmark = Arrays.stream(marks).sum();
for(int i=0; i<steps.length && totalmark>=steps[i]; i++) {
grade = (i==0 ? "U" : ""+i);
if(i < steps.length) {
marksaway = steps[(i+1)]-totalmark;
nextgrade = "" + (i+1);
}
}
System.out.println("Your total mark was " + totalmark + ".");
System.out.println("You got a Grade " + grade + ".");
if(grade == "9") {
System.out.println("You achieved the highest grade!");
} else if(marksaway == 1) {
System.out.println("You were " + marksaway + " mark away from a Grade " + nextgrade + ".");
} else {
System.out.println("You were " + marksaway + " marks away from a Grade " + nextgrade + ".");
}
}
}
Would a while true loop not be necessary in this case in order to skip the iteration if the user enters an invalid input?
– Gameskiller01
Dec 12 '18 at 15:38
1
You are totally right my mistake, my edit should fix this and avoid the ugly while(true). There is still an issue with the case when someone enters a character other than an integer, will have a look at it.
– Mark Peter Mc Adam
Dec 12 '18 at 21:17
1
After reading the answers to the following question, I changed the catch block so that it consumes whatever invalid token have been inputted and then decrements counters so that it asks for the same grade again. - stackoverflow.com/questions/24414299/…
– Mark Peter Mc Adam
Dec 12 '18 at 21:29
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f209520%2fgrade-calculator-for-a-project-with-four-aspects%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Because there are no holes you can simply have an array of "breakpoints".
int steps = new int { 2, 4, 13, 22, 31, 41, 54, 67, 80 };
int i;
for(i=0; i<steps.length && totalmark>=steps[i]; i++);
grade = i==0 ? "U" : ""+i;
if(i<steps.length) marksaway=steps[i]-totalmark;
nextgrade=""+(i+1);
1
Would you mind giving me a fairly simple explanation as to how this works?
– Gameskiller01
Dec 12 '18 at 15:18
1
The loop loops over the array and breaks out if toolmark greater the current array-entry. I.e. totalmark=3. First loop, i is 0 and totalmark is greater than steps[0] -> continue -- Second loop, i is 1 and totalmark is not greater than steps[1] -> break out of the loop with i=1. Set grade to "1", marksaway to steps[1]-totalmark and nextgrade to "2"
– Holger
Dec 12 '18 at 16:01
add a comment |
Because there are no holes you can simply have an array of "breakpoints".
int steps = new int { 2, 4, 13, 22, 31, 41, 54, 67, 80 };
int i;
for(i=0; i<steps.length && totalmark>=steps[i]; i++);
grade = i==0 ? "U" : ""+i;
if(i<steps.length) marksaway=steps[i]-totalmark;
nextgrade=""+(i+1);
1
Would you mind giving me a fairly simple explanation as to how this works?
– Gameskiller01
Dec 12 '18 at 15:18
1
The loop loops over the array and breaks out if toolmark greater the current array-entry. I.e. totalmark=3. First loop, i is 0 and totalmark is greater than steps[0] -> continue -- Second loop, i is 1 and totalmark is not greater than steps[1] -> break out of the loop with i=1. Set grade to "1", marksaway to steps[1]-totalmark and nextgrade to "2"
– Holger
Dec 12 '18 at 16:01
add a comment |
Because there are no holes you can simply have an array of "breakpoints".
int steps = new int { 2, 4, 13, 22, 31, 41, 54, 67, 80 };
int i;
for(i=0; i<steps.length && totalmark>=steps[i]; i++);
grade = i==0 ? "U" : ""+i;
if(i<steps.length) marksaway=steps[i]-totalmark;
nextgrade=""+(i+1);
Because there are no holes you can simply have an array of "breakpoints".
int steps = new int { 2, 4, 13, 22, 31, 41, 54, 67, 80 };
int i;
for(i=0; i<steps.length && totalmark>=steps[i]; i++);
grade = i==0 ? "U" : ""+i;
if(i<steps.length) marksaway=steps[i]-totalmark;
nextgrade=""+(i+1);
answered Dec 12 '18 at 13:10
Holger
20613
20613
1
Would you mind giving me a fairly simple explanation as to how this works?
– Gameskiller01
Dec 12 '18 at 15:18
1
The loop loops over the array and breaks out if toolmark greater the current array-entry. I.e. totalmark=3. First loop, i is 0 and totalmark is greater than steps[0] -> continue -- Second loop, i is 1 and totalmark is not greater than steps[1] -> break out of the loop with i=1. Set grade to "1", marksaway to steps[1]-totalmark and nextgrade to "2"
– Holger
Dec 12 '18 at 16:01
add a comment |
1
Would you mind giving me a fairly simple explanation as to how this works?
– Gameskiller01
Dec 12 '18 at 15:18
1
The loop loops over the array and breaks out if toolmark greater the current array-entry. I.e. totalmark=3. First loop, i is 0 and totalmark is greater than steps[0] -> continue -- Second loop, i is 1 and totalmark is not greater than steps[1] -> break out of the loop with i=1. Set grade to "1", marksaway to steps[1]-totalmark and nextgrade to "2"
– Holger
Dec 12 '18 at 16:01
1
1
Would you mind giving me a fairly simple explanation as to how this works?
– Gameskiller01
Dec 12 '18 at 15:18
Would you mind giving me a fairly simple explanation as to how this works?
– Gameskiller01
Dec 12 '18 at 15:18
1
1
The loop loops over the array and breaks out if toolmark greater the current array-entry. I.e. totalmark=3. First loop, i is 0 and totalmark is greater than steps[0] -> continue -- Second loop, i is 1 and totalmark is not greater than steps[1] -> break out of the loop with i=1. Set grade to "1", marksaway to steps[1]-totalmark and nextgrade to "2"
– Holger
Dec 12 '18 at 16:01
The loop loops over the array and breaks out if toolmark greater the current array-entry. I.e. totalmark=3. First loop, i is 0 and totalmark is greater than steps[0] -> continue -- Second loop, i is 1 and totalmark is not greater than steps[1] -> break out of the loop with i=1. Set grade to "1", marksaway to steps[1]-totalmark and nextgrade to "2"
– Holger
Dec 12 '18 at 16:01
add a comment |
I tidied up the first section for you a bit and then implemented the technique suggested by @Holger, all seems to work perfectly after running a few tests.
import java.util.Arrays;
import java.util.InputMismatchException;
import java.util.Scanner;
public class MarkCalculator {
public static void main(String args) {
// TODO Auto-generated method stub
String words = new String{"Analysis", "Design", "Implementation", "Evaluation"};
int steps = { 2, 4, 13, 22, 31, 41, 54, 67, 80 };
int marks = new int[4];
Scanner reader = new Scanner(System.in); // Keep instantiation of scanner outside of loop - once is enough
int totalmark = 0;
int inputMark = 0;
int marksaway = Integer.MIN_VALUE;
String grade = "";
String nextgrade = "";
for(int counter = 0; counter<words.length; counter++) {
System.out.println("Enter your mark for the '" + words[counter] + "' part of the project: ");
try {
do {
inputMark = reader.nextInt();
if(inputMark < 0 || inputMark > 25)
System.out.println("Please input a number between 0 and 25.");
else
marks[counter] = inputMark;
} while(inputMark < 0 || inputMark > 25);
} catch(InputMismatchException e) {
System.out.println("Please input a valid integer.");
reader.nextLine();
counter--;
}
}
totalmark = Arrays.stream(marks).sum();
for(int i=0; i<steps.length && totalmark>=steps[i]; i++) {
grade = (i==0 ? "U" : ""+i);
if(i < steps.length) {
marksaway = steps[(i+1)]-totalmark;
nextgrade = "" + (i+1);
}
}
System.out.println("Your total mark was " + totalmark + ".");
System.out.println("You got a Grade " + grade + ".");
if(grade == "9") {
System.out.println("You achieved the highest grade!");
} else if(marksaway == 1) {
System.out.println("You were " + marksaway + " mark away from a Grade " + nextgrade + ".");
} else {
System.out.println("You were " + marksaway + " marks away from a Grade " + nextgrade + ".");
}
}
}
Would a while true loop not be necessary in this case in order to skip the iteration if the user enters an invalid input?
– Gameskiller01
Dec 12 '18 at 15:38
1
You are totally right my mistake, my edit should fix this and avoid the ugly while(true). There is still an issue with the case when someone enters a character other than an integer, will have a look at it.
– Mark Peter Mc Adam
Dec 12 '18 at 21:17
1
After reading the answers to the following question, I changed the catch block so that it consumes whatever invalid token have been inputted and then decrements counters so that it asks for the same grade again. - stackoverflow.com/questions/24414299/…
– Mark Peter Mc Adam
Dec 12 '18 at 21:29
add a comment |
I tidied up the first section for you a bit and then implemented the technique suggested by @Holger, all seems to work perfectly after running a few tests.
import java.util.Arrays;
import java.util.InputMismatchException;
import java.util.Scanner;
public class MarkCalculator {
public static void main(String args) {
// TODO Auto-generated method stub
String words = new String{"Analysis", "Design", "Implementation", "Evaluation"};
int steps = { 2, 4, 13, 22, 31, 41, 54, 67, 80 };
int marks = new int[4];
Scanner reader = new Scanner(System.in); // Keep instantiation of scanner outside of loop - once is enough
int totalmark = 0;
int inputMark = 0;
int marksaway = Integer.MIN_VALUE;
String grade = "";
String nextgrade = "";
for(int counter = 0; counter<words.length; counter++) {
System.out.println("Enter your mark for the '" + words[counter] + "' part of the project: ");
try {
do {
inputMark = reader.nextInt();
if(inputMark < 0 || inputMark > 25)
System.out.println("Please input a number between 0 and 25.");
else
marks[counter] = inputMark;
} while(inputMark < 0 || inputMark > 25);
} catch(InputMismatchException e) {
System.out.println("Please input a valid integer.");
reader.nextLine();
counter--;
}
}
totalmark = Arrays.stream(marks).sum();
for(int i=0; i<steps.length && totalmark>=steps[i]; i++) {
grade = (i==0 ? "U" : ""+i);
if(i < steps.length) {
marksaway = steps[(i+1)]-totalmark;
nextgrade = "" + (i+1);
}
}
System.out.println("Your total mark was " + totalmark + ".");
System.out.println("You got a Grade " + grade + ".");
if(grade == "9") {
System.out.println("You achieved the highest grade!");
} else if(marksaway == 1) {
System.out.println("You were " + marksaway + " mark away from a Grade " + nextgrade + ".");
} else {
System.out.println("You were " + marksaway + " marks away from a Grade " + nextgrade + ".");
}
}
}
Would a while true loop not be necessary in this case in order to skip the iteration if the user enters an invalid input?
– Gameskiller01
Dec 12 '18 at 15:38
1
You are totally right my mistake, my edit should fix this and avoid the ugly while(true). There is still an issue with the case when someone enters a character other than an integer, will have a look at it.
– Mark Peter Mc Adam
Dec 12 '18 at 21:17
1
After reading the answers to the following question, I changed the catch block so that it consumes whatever invalid token have been inputted and then decrements counters so that it asks for the same grade again. - stackoverflow.com/questions/24414299/…
– Mark Peter Mc Adam
Dec 12 '18 at 21:29
add a comment |
I tidied up the first section for you a bit and then implemented the technique suggested by @Holger, all seems to work perfectly after running a few tests.
import java.util.Arrays;
import java.util.InputMismatchException;
import java.util.Scanner;
public class MarkCalculator {
public static void main(String args) {
// TODO Auto-generated method stub
String words = new String{"Analysis", "Design", "Implementation", "Evaluation"};
int steps = { 2, 4, 13, 22, 31, 41, 54, 67, 80 };
int marks = new int[4];
Scanner reader = new Scanner(System.in); // Keep instantiation of scanner outside of loop - once is enough
int totalmark = 0;
int inputMark = 0;
int marksaway = Integer.MIN_VALUE;
String grade = "";
String nextgrade = "";
for(int counter = 0; counter<words.length; counter++) {
System.out.println("Enter your mark for the '" + words[counter] + "' part of the project: ");
try {
do {
inputMark = reader.nextInt();
if(inputMark < 0 || inputMark > 25)
System.out.println("Please input a number between 0 and 25.");
else
marks[counter] = inputMark;
} while(inputMark < 0 || inputMark > 25);
} catch(InputMismatchException e) {
System.out.println("Please input a valid integer.");
reader.nextLine();
counter--;
}
}
totalmark = Arrays.stream(marks).sum();
for(int i=0; i<steps.length && totalmark>=steps[i]; i++) {
grade = (i==0 ? "U" : ""+i);
if(i < steps.length) {
marksaway = steps[(i+1)]-totalmark;
nextgrade = "" + (i+1);
}
}
System.out.println("Your total mark was " + totalmark + ".");
System.out.println("You got a Grade " + grade + ".");
if(grade == "9") {
System.out.println("You achieved the highest grade!");
} else if(marksaway == 1) {
System.out.println("You were " + marksaway + " mark away from a Grade " + nextgrade + ".");
} else {
System.out.println("You were " + marksaway + " marks away from a Grade " + nextgrade + ".");
}
}
}
I tidied up the first section for you a bit and then implemented the technique suggested by @Holger, all seems to work perfectly after running a few tests.
import java.util.Arrays;
import java.util.InputMismatchException;
import java.util.Scanner;
public class MarkCalculator {
public static void main(String args) {
// TODO Auto-generated method stub
String words = new String{"Analysis", "Design", "Implementation", "Evaluation"};
int steps = { 2, 4, 13, 22, 31, 41, 54, 67, 80 };
int marks = new int[4];
Scanner reader = new Scanner(System.in); // Keep instantiation of scanner outside of loop - once is enough
int totalmark = 0;
int inputMark = 0;
int marksaway = Integer.MIN_VALUE;
String grade = "";
String nextgrade = "";
for(int counter = 0; counter<words.length; counter++) {
System.out.println("Enter your mark for the '" + words[counter] + "' part of the project: ");
try {
do {
inputMark = reader.nextInt();
if(inputMark < 0 || inputMark > 25)
System.out.println("Please input a number between 0 and 25.");
else
marks[counter] = inputMark;
} while(inputMark < 0 || inputMark > 25);
} catch(InputMismatchException e) {
System.out.println("Please input a valid integer.");
reader.nextLine();
counter--;
}
}
totalmark = Arrays.stream(marks).sum();
for(int i=0; i<steps.length && totalmark>=steps[i]; i++) {
grade = (i==0 ? "U" : ""+i);
if(i < steps.length) {
marksaway = steps[(i+1)]-totalmark;
nextgrade = "" + (i+1);
}
}
System.out.println("Your total mark was " + totalmark + ".");
System.out.println("You got a Grade " + grade + ".");
if(grade == "9") {
System.out.println("You achieved the highest grade!");
} else if(marksaway == 1) {
System.out.println("You were " + marksaway + " mark away from a Grade " + nextgrade + ".");
} else {
System.out.println("You were " + marksaway + " marks away from a Grade " + nextgrade + ".");
}
}
}
edited Dec 12 '18 at 21:28
answered Dec 12 '18 at 13:48


Mark Peter Mc Adam
564
564
Would a while true loop not be necessary in this case in order to skip the iteration if the user enters an invalid input?
– Gameskiller01
Dec 12 '18 at 15:38
1
You are totally right my mistake, my edit should fix this and avoid the ugly while(true). There is still an issue with the case when someone enters a character other than an integer, will have a look at it.
– Mark Peter Mc Adam
Dec 12 '18 at 21:17
1
After reading the answers to the following question, I changed the catch block so that it consumes whatever invalid token have been inputted and then decrements counters so that it asks for the same grade again. - stackoverflow.com/questions/24414299/…
– Mark Peter Mc Adam
Dec 12 '18 at 21:29
add a comment |
Would a while true loop not be necessary in this case in order to skip the iteration if the user enters an invalid input?
– Gameskiller01
Dec 12 '18 at 15:38
1
You are totally right my mistake, my edit should fix this and avoid the ugly while(true). There is still an issue with the case when someone enters a character other than an integer, will have a look at it.
– Mark Peter Mc Adam
Dec 12 '18 at 21:17
1
After reading the answers to the following question, I changed the catch block so that it consumes whatever invalid token have been inputted and then decrements counters so that it asks for the same grade again. - stackoverflow.com/questions/24414299/…
– Mark Peter Mc Adam
Dec 12 '18 at 21:29
Would a while true loop not be necessary in this case in order to skip the iteration if the user enters an invalid input?
– Gameskiller01
Dec 12 '18 at 15:38
Would a while true loop not be necessary in this case in order to skip the iteration if the user enters an invalid input?
– Gameskiller01
Dec 12 '18 at 15:38
1
1
You are totally right my mistake, my edit should fix this and avoid the ugly while(true). There is still an issue with the case when someone enters a character other than an integer, will have a look at it.
– Mark Peter Mc Adam
Dec 12 '18 at 21:17
You are totally right my mistake, my edit should fix this and avoid the ugly while(true). There is still an issue with the case when someone enters a character other than an integer, will have a look at it.
– Mark Peter Mc Adam
Dec 12 '18 at 21:17
1
1
After reading the answers to the following question, I changed the catch block so that it consumes whatever invalid token have been inputted and then decrements counters so that it asks for the same grade again. - stackoverflow.com/questions/24414299/…
– Mark Peter Mc Adam
Dec 12 '18 at 21:29
After reading the answers to the following question, I changed the catch block so that it consumes whatever invalid token have been inputted and then decrements counters so that it asks for the same grade again. - stackoverflow.com/questions/24414299/…
– Mark Peter Mc Adam
Dec 12 '18 at 21:29
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f209520%2fgrade-calculator-for-a-project-with-four-aspects%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yKpZXYuzC6pc