How to catch JSON response in the TEXTVIEW?
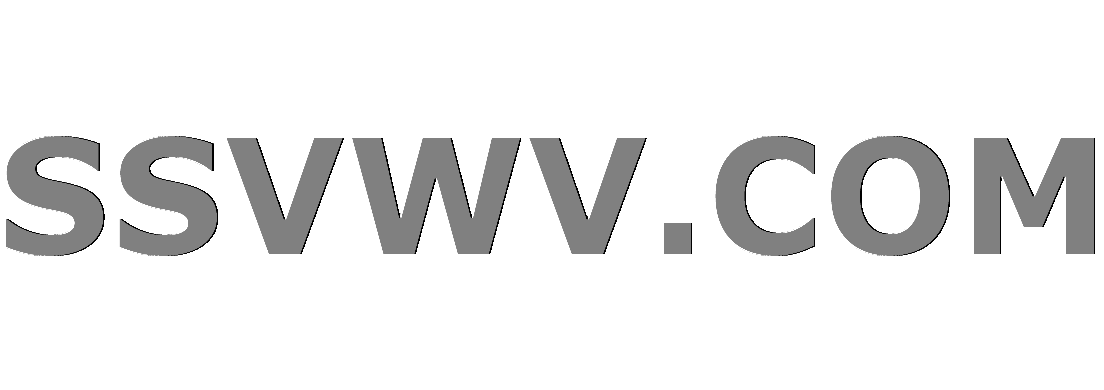
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm a beginner android developer I want to catch JSON array response like id,uid etc. Please help me to do this I took many help from YouTube but there is no solution. I'm stuck with it. I am giving all the files I have created for this and also the JSON.
JSON file
{
"status": 0,
"response_data": [
{
"id": "12",
"uid": "USER00000003",
"reason": "Test",
"type": "Plan Leave",
"SataDate": "2018-09-18",
"EndDate": "2018-09-25",
"ApprovedBy": "USER00000002",
"ApprovedDate": "2018-09-18",
"Status": "REJECTED",
"Remarks": "Test Reject"
},
{
"id": "13",
"uid": "USER00000003",
"reason": "Wedding",
"type": "Plan Leave",
"SataDate": "2018-01-28",
"EndDate": "2018-02-05",
"ApprovedBy": "USER00000002",
"ApprovedDate": "2018-09-18",
"Status": "APPROVED",
"Remarks": "Ok"
}
]
}
ApiInterface
public interface ApiInterface {
@GET("leave_dtls.php")
Call<ResponseData> getData();
}
ApiClient
public class ApiClient {
private final static String BASE_URL = "http://api.xxxx.com/app/";
public static ApiClient apiClient;
private Retrofit retrofit = null;
public static ApiClient getInstance() {
if (apiClient == null) {
apiClient = new ApiClient();
}
return apiClient;
}
//private static Retrofit storeRetrofit = null;
public Retrofit getClient() {
return getClient(null);
}
private Retrofit getClient(final Context context) {
HttpLoggingInterceptor interceptor = new HttpLoggingInterceptor();
interceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient.Builder client = new OkHttpClient.Builder();
client.readTimeout(60, TimeUnit.SECONDS);
client.writeTimeout(60, TimeUnit.SECONDS);
client.connectTimeout(60, TimeUnit.SECONDS);
client.addInterceptor(interceptor);
client.addInterceptor(new Interceptor() {
@Override
public okhttp3.Response intercept(Chain chain) throws IOException {
Request request = chain.request();
return chain.proceed(request);
}
});
retrofit = new Retrofit.Builder()
.baseUrl(BASE_URL)
.client(client.build())
.addConverterFactory(GsonConverterFactory.create())
.build();
return retrofit;
}
}
Response Data
public class ResponseData {
@SerializedName("response_data")
private List<ResponseDataItem> responseData;
@SerializedName("status")
private int status;
public void setResponseData(List<ResponseDataItem> responseData){
this.responseData = responseData;
}
public List<ResponseDataItem> getResponseData(){
return responseData;
}
public void setStatus(int status){
this.status = status;
}
public int getStatus(){
return status;
}
}
ResponseDataItem
public class ResponseDataItem {
@SerializedName("Status")
private String status;
@SerializedName("uid")
private String uid;
@SerializedName("reason")
private String reason;
@SerializedName("ApprovedDate")
private String approvedDate;
@SerializedName("Remarks")
private String remarks;
@SerializedName("ApprovedBy")
private String approvedBy;
@SerializedName("id")
private String id;
@SerializedName("type")
private String type;
@SerializedName("EndDate")
private String endDate;
@SerializedName("SataDate")
private String sataDate;
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public String getUid() {
return uid;
}
public void setUid(String uid) {
this.uid = uid;
}
public String getReason() {
return reason;
}
public void setReason(String reason) {
this.reason = reason;
}
public String getApprovedDate() {
return approvedDate;
}
public void setApprovedDate(String approvedDate) {
this.approvedDate = approvedDate;
}
public String getRemarks() {
return remarks;
}
public void setRemarks(String remarks) {
this.remarks = remarks;
}
public String getApprovedBy() {
return approvedBy;
}
public void setApprovedBy(String approvedBy) {
this.approvedBy = approvedBy;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getEndDate() {
return endDate;
}
public void setEndDate(String endDate) {
this.endDate = endDate;
}
public String getSataDate() {
return sataDate;
}
public void setSataDate(String sataDate) {
this.sataDate = sataDate;
}
}
MainActivity
public class MainActivity extends AppCompatActivity {
TextView tvResponse;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tvResponse=findViewById(R.id.tvResponse);
ApiInterface apiInterface = ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
}
}
just I want to show the response in TEXTVIEW.
java

add a comment |
I'm a beginner android developer I want to catch JSON array response like id,uid etc. Please help me to do this I took many help from YouTube but there is no solution. I'm stuck with it. I am giving all the files I have created for this and also the JSON.
JSON file
{
"status": 0,
"response_data": [
{
"id": "12",
"uid": "USER00000003",
"reason": "Test",
"type": "Plan Leave",
"SataDate": "2018-09-18",
"EndDate": "2018-09-25",
"ApprovedBy": "USER00000002",
"ApprovedDate": "2018-09-18",
"Status": "REJECTED",
"Remarks": "Test Reject"
},
{
"id": "13",
"uid": "USER00000003",
"reason": "Wedding",
"type": "Plan Leave",
"SataDate": "2018-01-28",
"EndDate": "2018-02-05",
"ApprovedBy": "USER00000002",
"ApprovedDate": "2018-09-18",
"Status": "APPROVED",
"Remarks": "Ok"
}
]
}
ApiInterface
public interface ApiInterface {
@GET("leave_dtls.php")
Call<ResponseData> getData();
}
ApiClient
public class ApiClient {
private final static String BASE_URL = "http://api.xxxx.com/app/";
public static ApiClient apiClient;
private Retrofit retrofit = null;
public static ApiClient getInstance() {
if (apiClient == null) {
apiClient = new ApiClient();
}
return apiClient;
}
//private static Retrofit storeRetrofit = null;
public Retrofit getClient() {
return getClient(null);
}
private Retrofit getClient(final Context context) {
HttpLoggingInterceptor interceptor = new HttpLoggingInterceptor();
interceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient.Builder client = new OkHttpClient.Builder();
client.readTimeout(60, TimeUnit.SECONDS);
client.writeTimeout(60, TimeUnit.SECONDS);
client.connectTimeout(60, TimeUnit.SECONDS);
client.addInterceptor(interceptor);
client.addInterceptor(new Interceptor() {
@Override
public okhttp3.Response intercept(Chain chain) throws IOException {
Request request = chain.request();
return chain.proceed(request);
}
});
retrofit = new Retrofit.Builder()
.baseUrl(BASE_URL)
.client(client.build())
.addConverterFactory(GsonConverterFactory.create())
.build();
return retrofit;
}
}
Response Data
public class ResponseData {
@SerializedName("response_data")
private List<ResponseDataItem> responseData;
@SerializedName("status")
private int status;
public void setResponseData(List<ResponseDataItem> responseData){
this.responseData = responseData;
}
public List<ResponseDataItem> getResponseData(){
return responseData;
}
public void setStatus(int status){
this.status = status;
}
public int getStatus(){
return status;
}
}
ResponseDataItem
public class ResponseDataItem {
@SerializedName("Status")
private String status;
@SerializedName("uid")
private String uid;
@SerializedName("reason")
private String reason;
@SerializedName("ApprovedDate")
private String approvedDate;
@SerializedName("Remarks")
private String remarks;
@SerializedName("ApprovedBy")
private String approvedBy;
@SerializedName("id")
private String id;
@SerializedName("type")
private String type;
@SerializedName("EndDate")
private String endDate;
@SerializedName("SataDate")
private String sataDate;
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public String getUid() {
return uid;
}
public void setUid(String uid) {
this.uid = uid;
}
public String getReason() {
return reason;
}
public void setReason(String reason) {
this.reason = reason;
}
public String getApprovedDate() {
return approvedDate;
}
public void setApprovedDate(String approvedDate) {
this.approvedDate = approvedDate;
}
public String getRemarks() {
return remarks;
}
public void setRemarks(String remarks) {
this.remarks = remarks;
}
public String getApprovedBy() {
return approvedBy;
}
public void setApprovedBy(String approvedBy) {
this.approvedBy = approvedBy;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getEndDate() {
return endDate;
}
public void setEndDate(String endDate) {
this.endDate = endDate;
}
public String getSataDate() {
return sataDate;
}
public void setSataDate(String sataDate) {
this.sataDate = sataDate;
}
}
MainActivity
public class MainActivity extends AppCompatActivity {
TextView tvResponse;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tvResponse=findViewById(R.id.tvResponse);
ApiInterface apiInterface = ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
}
}
just I want to show the response in TEXTVIEW.
java

add a comment |
I'm a beginner android developer I want to catch JSON array response like id,uid etc. Please help me to do this I took many help from YouTube but there is no solution. I'm stuck with it. I am giving all the files I have created for this and also the JSON.
JSON file
{
"status": 0,
"response_data": [
{
"id": "12",
"uid": "USER00000003",
"reason": "Test",
"type": "Plan Leave",
"SataDate": "2018-09-18",
"EndDate": "2018-09-25",
"ApprovedBy": "USER00000002",
"ApprovedDate": "2018-09-18",
"Status": "REJECTED",
"Remarks": "Test Reject"
},
{
"id": "13",
"uid": "USER00000003",
"reason": "Wedding",
"type": "Plan Leave",
"SataDate": "2018-01-28",
"EndDate": "2018-02-05",
"ApprovedBy": "USER00000002",
"ApprovedDate": "2018-09-18",
"Status": "APPROVED",
"Remarks": "Ok"
}
]
}
ApiInterface
public interface ApiInterface {
@GET("leave_dtls.php")
Call<ResponseData> getData();
}
ApiClient
public class ApiClient {
private final static String BASE_URL = "http://api.xxxx.com/app/";
public static ApiClient apiClient;
private Retrofit retrofit = null;
public static ApiClient getInstance() {
if (apiClient == null) {
apiClient = new ApiClient();
}
return apiClient;
}
//private static Retrofit storeRetrofit = null;
public Retrofit getClient() {
return getClient(null);
}
private Retrofit getClient(final Context context) {
HttpLoggingInterceptor interceptor = new HttpLoggingInterceptor();
interceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient.Builder client = new OkHttpClient.Builder();
client.readTimeout(60, TimeUnit.SECONDS);
client.writeTimeout(60, TimeUnit.SECONDS);
client.connectTimeout(60, TimeUnit.SECONDS);
client.addInterceptor(interceptor);
client.addInterceptor(new Interceptor() {
@Override
public okhttp3.Response intercept(Chain chain) throws IOException {
Request request = chain.request();
return chain.proceed(request);
}
});
retrofit = new Retrofit.Builder()
.baseUrl(BASE_URL)
.client(client.build())
.addConverterFactory(GsonConverterFactory.create())
.build();
return retrofit;
}
}
Response Data
public class ResponseData {
@SerializedName("response_data")
private List<ResponseDataItem> responseData;
@SerializedName("status")
private int status;
public void setResponseData(List<ResponseDataItem> responseData){
this.responseData = responseData;
}
public List<ResponseDataItem> getResponseData(){
return responseData;
}
public void setStatus(int status){
this.status = status;
}
public int getStatus(){
return status;
}
}
ResponseDataItem
public class ResponseDataItem {
@SerializedName("Status")
private String status;
@SerializedName("uid")
private String uid;
@SerializedName("reason")
private String reason;
@SerializedName("ApprovedDate")
private String approvedDate;
@SerializedName("Remarks")
private String remarks;
@SerializedName("ApprovedBy")
private String approvedBy;
@SerializedName("id")
private String id;
@SerializedName("type")
private String type;
@SerializedName("EndDate")
private String endDate;
@SerializedName("SataDate")
private String sataDate;
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public String getUid() {
return uid;
}
public void setUid(String uid) {
this.uid = uid;
}
public String getReason() {
return reason;
}
public void setReason(String reason) {
this.reason = reason;
}
public String getApprovedDate() {
return approvedDate;
}
public void setApprovedDate(String approvedDate) {
this.approvedDate = approvedDate;
}
public String getRemarks() {
return remarks;
}
public void setRemarks(String remarks) {
this.remarks = remarks;
}
public String getApprovedBy() {
return approvedBy;
}
public void setApprovedBy(String approvedBy) {
this.approvedBy = approvedBy;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getEndDate() {
return endDate;
}
public void setEndDate(String endDate) {
this.endDate = endDate;
}
public String getSataDate() {
return sataDate;
}
public void setSataDate(String sataDate) {
this.sataDate = sataDate;
}
}
MainActivity
public class MainActivity extends AppCompatActivity {
TextView tvResponse;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tvResponse=findViewById(R.id.tvResponse);
ApiInterface apiInterface = ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
}
}
just I want to show the response in TEXTVIEW.
java

I'm a beginner android developer I want to catch JSON array response like id,uid etc. Please help me to do this I took many help from YouTube but there is no solution. I'm stuck with it. I am giving all the files I have created for this and also the JSON.
JSON file
{
"status": 0,
"response_data": [
{
"id": "12",
"uid": "USER00000003",
"reason": "Test",
"type": "Plan Leave",
"SataDate": "2018-09-18",
"EndDate": "2018-09-25",
"ApprovedBy": "USER00000002",
"ApprovedDate": "2018-09-18",
"Status": "REJECTED",
"Remarks": "Test Reject"
},
{
"id": "13",
"uid": "USER00000003",
"reason": "Wedding",
"type": "Plan Leave",
"SataDate": "2018-01-28",
"EndDate": "2018-02-05",
"ApprovedBy": "USER00000002",
"ApprovedDate": "2018-09-18",
"Status": "APPROVED",
"Remarks": "Ok"
}
]
}
ApiInterface
public interface ApiInterface {
@GET("leave_dtls.php")
Call<ResponseData> getData();
}
ApiClient
public class ApiClient {
private final static String BASE_URL = "http://api.xxxx.com/app/";
public static ApiClient apiClient;
private Retrofit retrofit = null;
public static ApiClient getInstance() {
if (apiClient == null) {
apiClient = new ApiClient();
}
return apiClient;
}
//private static Retrofit storeRetrofit = null;
public Retrofit getClient() {
return getClient(null);
}
private Retrofit getClient(final Context context) {
HttpLoggingInterceptor interceptor = new HttpLoggingInterceptor();
interceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient.Builder client = new OkHttpClient.Builder();
client.readTimeout(60, TimeUnit.SECONDS);
client.writeTimeout(60, TimeUnit.SECONDS);
client.connectTimeout(60, TimeUnit.SECONDS);
client.addInterceptor(interceptor);
client.addInterceptor(new Interceptor() {
@Override
public okhttp3.Response intercept(Chain chain) throws IOException {
Request request = chain.request();
return chain.proceed(request);
}
});
retrofit = new Retrofit.Builder()
.baseUrl(BASE_URL)
.client(client.build())
.addConverterFactory(GsonConverterFactory.create())
.build();
return retrofit;
}
}
Response Data
public class ResponseData {
@SerializedName("response_data")
private List<ResponseDataItem> responseData;
@SerializedName("status")
private int status;
public void setResponseData(List<ResponseDataItem> responseData){
this.responseData = responseData;
}
public List<ResponseDataItem> getResponseData(){
return responseData;
}
public void setStatus(int status){
this.status = status;
}
public int getStatus(){
return status;
}
}
ResponseDataItem
public class ResponseDataItem {
@SerializedName("Status")
private String status;
@SerializedName("uid")
private String uid;
@SerializedName("reason")
private String reason;
@SerializedName("ApprovedDate")
private String approvedDate;
@SerializedName("Remarks")
private String remarks;
@SerializedName("ApprovedBy")
private String approvedBy;
@SerializedName("id")
private String id;
@SerializedName("type")
private String type;
@SerializedName("EndDate")
private String endDate;
@SerializedName("SataDate")
private String sataDate;
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public String getUid() {
return uid;
}
public void setUid(String uid) {
this.uid = uid;
}
public String getReason() {
return reason;
}
public void setReason(String reason) {
this.reason = reason;
}
public String getApprovedDate() {
return approvedDate;
}
public void setApprovedDate(String approvedDate) {
this.approvedDate = approvedDate;
}
public String getRemarks() {
return remarks;
}
public void setRemarks(String remarks) {
this.remarks = remarks;
}
public String getApprovedBy() {
return approvedBy;
}
public void setApprovedBy(String approvedBy) {
this.approvedBy = approvedBy;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getEndDate() {
return endDate;
}
public void setEndDate(String endDate) {
this.endDate = endDate;
}
public String getSataDate() {
return sataDate;
}
public void setSataDate(String sataDate) {
this.sataDate = sataDate;
}
}
MainActivity
public class MainActivity extends AppCompatActivity {
TextView tvResponse;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
tvResponse=findViewById(R.id.tvResponse);
ApiInterface apiInterface = ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
}
}
just I want to show the response in TEXTVIEW.
java

java

asked Nov 23 '18 at 12:16
Mr. PaulMr. Paul
11
11
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
ApiInterface apiInterface =
ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
tvResponse.setText(response.body().getResponseData().toString());
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
just add this line for showing data in textview,
as per your current question, if your question is different then kindly elaborate more
add a comment |
Problem is that you pass null value in getClient() method.You not getting your client.
Use below code :
public static Retrofit getClient() {
HttpLoggingInterceptor httpLoggingInterceptor = new HttpLoggingInterceptor();
httpLoggingInterceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient.Builder builder = new OkHttpClient.Builder();
builder.networkInterceptors().add(httpLoggingInterceptor);
builder.interceptors().add(httpLoggingInterceptor);
okHttpClient = builder.build();
retrofit = new Retrofit.Builder().baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.client(okHttpClient)
.build();
return retrofit;
}
// then call **getClient()** like below:
ApiInterface apiInterface = ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
add a comment |
tvResponse.setText("show some text");
in your example you could also use append
if there's more than one item to show. put this in your method onResponse
:
List<ResponseDataItem> data=response.body().getResponseData();
for (ResponseDataItem responseItem : data) {
tvResponse.append(responseItem.getId() + "n");
tvResponse.append(responseItem.getUId() + "n");
// append any other fields you want to show
}
add a comment |
First of all, you are not passing Context
while creating ApiInterface
object. That's why your object will not be created properly. You should pass Context
as i'm doing.
Secondly, You have Call<ResponseData>
in your ApiInterface
and here you're trying to fetch response in a List<>
. so you should get response body in Model Class object.
Update your code with this.
String data = "";
ApiInterface apiInterface = ApiClient.getInstance().getClient(MainActivity.this).create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
ResponseData data = response.body();
for(int i=0; i< data.getResponseData().size(); i++){
String id = data.getResponseData().getId();
String uid = data.getResponseData().getUid();
//Similarly you can get others
data = data + "Id= "+ id +", uId= "+ uid + "n";
}
//setdetails to TextView
tvResponse.setText(data);
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
Hope this helps you !
Thanks for your comment.But sir I need to show Response Data Item's data on textview
– Mr. Paul
Nov 23 '18 at 13:07
updated code. See now.. it will fetch all items ids and uids
– Ali Ahmed
Nov 23 '18 at 13:57
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446581%2fhow-to-catch-json-response-in-the-textview%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
ApiInterface apiInterface =
ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
tvResponse.setText(response.body().getResponseData().toString());
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
just add this line for showing data in textview,
as per your current question, if your question is different then kindly elaborate more
add a comment |
ApiInterface apiInterface =
ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
tvResponse.setText(response.body().getResponseData().toString());
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
just add this line for showing data in textview,
as per your current question, if your question is different then kindly elaborate more
add a comment |
ApiInterface apiInterface =
ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
tvResponse.setText(response.body().getResponseData().toString());
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
just add this line for showing data in textview,
as per your current question, if your question is different then kindly elaborate more
ApiInterface apiInterface =
ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
tvResponse.setText(response.body().getResponseData().toString());
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
just add this line for showing data in textview,
as per your current question, if your question is different then kindly elaborate more
answered Nov 23 '18 at 12:34


Akash DubeyAkash Dubey
610827
610827
add a comment |
add a comment |
Problem is that you pass null value in getClient() method.You not getting your client.
Use below code :
public static Retrofit getClient() {
HttpLoggingInterceptor httpLoggingInterceptor = new HttpLoggingInterceptor();
httpLoggingInterceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient.Builder builder = new OkHttpClient.Builder();
builder.networkInterceptors().add(httpLoggingInterceptor);
builder.interceptors().add(httpLoggingInterceptor);
okHttpClient = builder.build();
retrofit = new Retrofit.Builder().baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.client(okHttpClient)
.build();
return retrofit;
}
// then call **getClient()** like below:
ApiInterface apiInterface = ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
add a comment |
Problem is that you pass null value in getClient() method.You not getting your client.
Use below code :
public static Retrofit getClient() {
HttpLoggingInterceptor httpLoggingInterceptor = new HttpLoggingInterceptor();
httpLoggingInterceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient.Builder builder = new OkHttpClient.Builder();
builder.networkInterceptors().add(httpLoggingInterceptor);
builder.interceptors().add(httpLoggingInterceptor);
okHttpClient = builder.build();
retrofit = new Retrofit.Builder().baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.client(okHttpClient)
.build();
return retrofit;
}
// then call **getClient()** like below:
ApiInterface apiInterface = ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
add a comment |
Problem is that you pass null value in getClient() method.You not getting your client.
Use below code :
public static Retrofit getClient() {
HttpLoggingInterceptor httpLoggingInterceptor = new HttpLoggingInterceptor();
httpLoggingInterceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient.Builder builder = new OkHttpClient.Builder();
builder.networkInterceptors().add(httpLoggingInterceptor);
builder.interceptors().add(httpLoggingInterceptor);
okHttpClient = builder.build();
retrofit = new Retrofit.Builder().baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.client(okHttpClient)
.build();
return retrofit;
}
// then call **getClient()** like below:
ApiInterface apiInterface = ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
Problem is that you pass null value in getClient() method.You not getting your client.
Use below code :
public static Retrofit getClient() {
HttpLoggingInterceptor httpLoggingInterceptor = new HttpLoggingInterceptor();
httpLoggingInterceptor.setLevel(HttpLoggingInterceptor.Level.BODY);
OkHttpClient.Builder builder = new OkHttpClient.Builder();
builder.networkInterceptors().add(httpLoggingInterceptor);
builder.interceptors().add(httpLoggingInterceptor);
okHttpClient = builder.build();
retrofit = new Retrofit.Builder().baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.client(okHttpClient)
.build();
return retrofit;
}
// then call **getClient()** like below:
ApiInterface apiInterface = ApiClient.getInstance().getClient().create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
List<ResponseDataItem> data=response.body().getResponseData();
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
answered Nov 23 '18 at 12:37


Rishav SinglaRishav Singla
520210
520210
add a comment |
add a comment |
tvResponse.setText("show some text");
in your example you could also use append
if there's more than one item to show. put this in your method onResponse
:
List<ResponseDataItem> data=response.body().getResponseData();
for (ResponseDataItem responseItem : data) {
tvResponse.append(responseItem.getId() + "n");
tvResponse.append(responseItem.getUId() + "n");
// append any other fields you want to show
}
add a comment |
tvResponse.setText("show some text");
in your example you could also use append
if there's more than one item to show. put this in your method onResponse
:
List<ResponseDataItem> data=response.body().getResponseData();
for (ResponseDataItem responseItem : data) {
tvResponse.append(responseItem.getId() + "n");
tvResponse.append(responseItem.getUId() + "n");
// append any other fields you want to show
}
add a comment |
tvResponse.setText("show some text");
in your example you could also use append
if there's more than one item to show. put this in your method onResponse
:
List<ResponseDataItem> data=response.body().getResponseData();
for (ResponseDataItem responseItem : data) {
tvResponse.append(responseItem.getId() + "n");
tvResponse.append(responseItem.getUId() + "n");
// append any other fields you want to show
}
tvResponse.setText("show some text");
in your example you could also use append
if there's more than one item to show. put this in your method onResponse
:
List<ResponseDataItem> data=response.body().getResponseData();
for (ResponseDataItem responseItem : data) {
tvResponse.append(responseItem.getId() + "n");
tvResponse.append(responseItem.getUId() + "n");
// append any other fields you want to show
}
answered Nov 23 '18 at 13:06
Milo BemMilo Bem
822418
822418
add a comment |
add a comment |
First of all, you are not passing Context
while creating ApiInterface
object. That's why your object will not be created properly. You should pass Context
as i'm doing.
Secondly, You have Call<ResponseData>
in your ApiInterface
and here you're trying to fetch response in a List<>
. so you should get response body in Model Class object.
Update your code with this.
String data = "";
ApiInterface apiInterface = ApiClient.getInstance().getClient(MainActivity.this).create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
ResponseData data = response.body();
for(int i=0; i< data.getResponseData().size(); i++){
String id = data.getResponseData().getId();
String uid = data.getResponseData().getUid();
//Similarly you can get others
data = data + "Id= "+ id +", uId= "+ uid + "n";
}
//setdetails to TextView
tvResponse.setText(data);
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
Hope this helps you !
Thanks for your comment.But sir I need to show Response Data Item's data on textview
– Mr. Paul
Nov 23 '18 at 13:07
updated code. See now.. it will fetch all items ids and uids
– Ali Ahmed
Nov 23 '18 at 13:57
add a comment |
First of all, you are not passing Context
while creating ApiInterface
object. That's why your object will not be created properly. You should pass Context
as i'm doing.
Secondly, You have Call<ResponseData>
in your ApiInterface
and here you're trying to fetch response in a List<>
. so you should get response body in Model Class object.
Update your code with this.
String data = "";
ApiInterface apiInterface = ApiClient.getInstance().getClient(MainActivity.this).create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
ResponseData data = response.body();
for(int i=0; i< data.getResponseData().size(); i++){
String id = data.getResponseData().getId();
String uid = data.getResponseData().getUid();
//Similarly you can get others
data = data + "Id= "+ id +", uId= "+ uid + "n";
}
//setdetails to TextView
tvResponse.setText(data);
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
Hope this helps you !
Thanks for your comment.But sir I need to show Response Data Item's data on textview
– Mr. Paul
Nov 23 '18 at 13:07
updated code. See now.. it will fetch all items ids and uids
– Ali Ahmed
Nov 23 '18 at 13:57
add a comment |
First of all, you are not passing Context
while creating ApiInterface
object. That's why your object will not be created properly. You should pass Context
as i'm doing.
Secondly, You have Call<ResponseData>
in your ApiInterface
and here you're trying to fetch response in a List<>
. so you should get response body in Model Class object.
Update your code with this.
String data = "";
ApiInterface apiInterface = ApiClient.getInstance().getClient(MainActivity.this).create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
ResponseData data = response.body();
for(int i=0; i< data.getResponseData().size(); i++){
String id = data.getResponseData().getId();
String uid = data.getResponseData().getUid();
//Similarly you can get others
data = data + "Id= "+ id +", uId= "+ uid + "n";
}
//setdetails to TextView
tvResponse.setText(data);
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
Hope this helps you !
First of all, you are not passing Context
while creating ApiInterface
object. That's why your object will not be created properly. You should pass Context
as i'm doing.
Secondly, You have Call<ResponseData>
in your ApiInterface
and here you're trying to fetch response in a List<>
. so you should get response body in Model Class object.
Update your code with this.
String data = "";
ApiInterface apiInterface = ApiClient.getInstance().getClient(MainActivity.this).create(ApiInterface.class);
Call<ResponseData> responseDataCall=apiInterface.getData();
responseDataCall.enqueue(new Callback<ResponseData>() {
@Override
public void onResponse(Call<ResponseData> call, Response<ResponseData> response) {
if (response.isSuccessful() && response.body()!=null && response!=null){
ResponseData data = response.body();
for(int i=0; i< data.getResponseData().size(); i++){
String id = data.getResponseData().getId();
String uid = data.getResponseData().getUid();
//Similarly you can get others
data = data + "Id= "+ id +", uId= "+ uid + "n";
}
//setdetails to TextView
tvResponse.setText(data);
}
}
@Override
public void onFailure(Call<ResponseData> call, Throwable t) {
t.printStackTrace();
}
});
Hope this helps you !
edited Nov 23 '18 at 14:03
answered Nov 23 '18 at 12:26


Ali AhmedAli Ahmed
1,3891314
1,3891314
Thanks for your comment.But sir I need to show Response Data Item's data on textview
– Mr. Paul
Nov 23 '18 at 13:07
updated code. See now.. it will fetch all items ids and uids
– Ali Ahmed
Nov 23 '18 at 13:57
add a comment |
Thanks for your comment.But sir I need to show Response Data Item's data on textview
– Mr. Paul
Nov 23 '18 at 13:07
updated code. See now.. it will fetch all items ids and uids
– Ali Ahmed
Nov 23 '18 at 13:57
Thanks for your comment.But sir I need to show Response Data Item's data on textview
– Mr. Paul
Nov 23 '18 at 13:07
Thanks for your comment.But sir I need to show Response Data Item's data on textview
– Mr. Paul
Nov 23 '18 at 13:07
updated code. See now.. it will fetch all items ids and uids
– Ali Ahmed
Nov 23 '18 at 13:57
updated code. See now.. it will fetch all items ids and uids
– Ali Ahmed
Nov 23 '18 at 13:57
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446581%2fhow-to-catch-json-response-in-the-textview%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
o8ltHNH2aoDnby61XTfVfy2,ERF1S,hD jkKWZEhaez2Fve,xB,o3