Jest unit testing API using express and mongoose
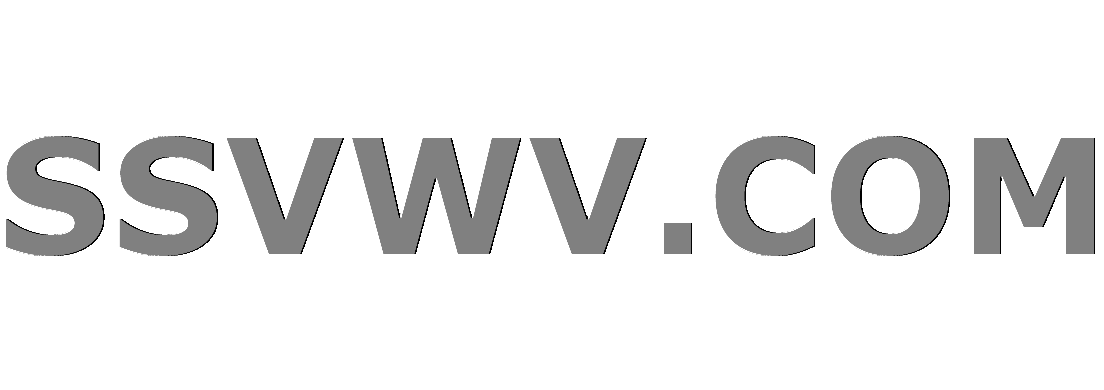
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am trying a TDD approach on an user API. I wanted to integrated unit tests for my functions: userRegister and userLogin
This is my app.js
'use strict'
const express = require('express')
const bodyParser = require('body-parser')
const passport = require('passport')
const users = require('../routes/users')
const app = express()
const port = 5000
app.get('/', (req, res) => {
res.send({ msg: 'Test' })
})
//BodyParser Middleware
app.use(bodyParser.urlencoded({ extended: false }))
app.use(bodyParser.json())
//Passport middleware
app.use(passport.initialize())
//Initialize Routes
app.use('/api/users', users)
//Export app
module.exports = app
This is my userController.js:
'use strict'
const express = require('express')
const bcrypt = require('bcryptjs')
const jwt = require('jsonwebtoken')
const keys = require('../../../../config/keys')
const passport = require('passport')
const status = require('http-status-codes')
const User = require('../../models/User')
module.exports.test = (req, res) => {
res.json({msg: 'Users works'})
}
module.exports.userRegister = (req, res) => {
User.findOne({ email: req.body.email})
.then(user => {
if(user)
return res.status(400).json({ userEmailerror: 'Email already
exists'})
else{
const newUser = new User({
name: req.body.name,
email: req.body.email,
password: req.body.password
})
newUser.save()
.then(user => res.json(user))
.catch(err => console.log(err))
}
})
}
this is my userController.test.js
'use strict'
const mongoose = require('mongoose')
const testUserDB = require('../../../config/keys').testUsersMongURI
mongoose.connect(testUserDB)
const userController = require('../modules/controllers/userController')
const User = require('../models/User')
describe('register new user', () => {
test('succesfully register a valid user', () => {
const newUser = new User({
name: 'test',
email: 'test@gmail.com',
password: 'test123'
})
const req = {body: {newUser}}
userController.userRegister(req).then(user => {
expect(user.name).toBe('test')
expect(user.email).toBe('test@gmail.com')
})
})
})
The script I use to run the test is:
"test": "jest --coverage",
I am new to Javascript and Full stack and the error I received is confusing me
RUNS src/user/__tests__/userController.test.js
FAIL src/user/__tests__/userController.test.js
register new user
× succesfully register a valid user (19ms)
● register new user › succesfully register a valid user
TypeError: Cannot read property 'then' of undefined
16 | })
17 | const req = {body: {newUser}}
> 18 | userController.userRegister(req).then(user => {
| ^
19 | expect(user.name).toBe('test')
20 | expect(user.email).toBe('test@gmail.com')
21 |
at Object.test (src/user/__tests__/userController.test.js:18:3)
Thank you for your help!
javascript express mongoose tdd jestjs
add a comment |
I am trying a TDD approach on an user API. I wanted to integrated unit tests for my functions: userRegister and userLogin
This is my app.js
'use strict'
const express = require('express')
const bodyParser = require('body-parser')
const passport = require('passport')
const users = require('../routes/users')
const app = express()
const port = 5000
app.get('/', (req, res) => {
res.send({ msg: 'Test' })
})
//BodyParser Middleware
app.use(bodyParser.urlencoded({ extended: false }))
app.use(bodyParser.json())
//Passport middleware
app.use(passport.initialize())
//Initialize Routes
app.use('/api/users', users)
//Export app
module.exports = app
This is my userController.js:
'use strict'
const express = require('express')
const bcrypt = require('bcryptjs')
const jwt = require('jsonwebtoken')
const keys = require('../../../../config/keys')
const passport = require('passport')
const status = require('http-status-codes')
const User = require('../../models/User')
module.exports.test = (req, res) => {
res.json({msg: 'Users works'})
}
module.exports.userRegister = (req, res) => {
User.findOne({ email: req.body.email})
.then(user => {
if(user)
return res.status(400).json({ userEmailerror: 'Email already
exists'})
else{
const newUser = new User({
name: req.body.name,
email: req.body.email,
password: req.body.password
})
newUser.save()
.then(user => res.json(user))
.catch(err => console.log(err))
}
})
}
this is my userController.test.js
'use strict'
const mongoose = require('mongoose')
const testUserDB = require('../../../config/keys').testUsersMongURI
mongoose.connect(testUserDB)
const userController = require('../modules/controllers/userController')
const User = require('../models/User')
describe('register new user', () => {
test('succesfully register a valid user', () => {
const newUser = new User({
name: 'test',
email: 'test@gmail.com',
password: 'test123'
})
const req = {body: {newUser}}
userController.userRegister(req).then(user => {
expect(user.name).toBe('test')
expect(user.email).toBe('test@gmail.com')
})
})
})
The script I use to run the test is:
"test": "jest --coverage",
I am new to Javascript and Full stack and the error I received is confusing me
RUNS src/user/__tests__/userController.test.js
FAIL src/user/__tests__/userController.test.js
register new user
× succesfully register a valid user (19ms)
● register new user › succesfully register a valid user
TypeError: Cannot read property 'then' of undefined
16 | })
17 | const req = {body: {newUser}}
> 18 | userController.userRegister(req).then(user => {
| ^
19 | expect(user.name).toBe('test')
20 | expect(user.email).toBe('test@gmail.com')
21 |
at Object.test (src/user/__tests__/userController.test.js:18:3)
Thank you for your help!
javascript express mongoose tdd jestjs
1
The test is telling you the right thing:userController.userRegister
doesn't actually return anything.
– jonrsharpe
Nov 23 '18 at 12:19
I tried returning in various ways but I got same error.
– Vlad
Nov 23 '18 at 12:30
add a comment |
I am trying a TDD approach on an user API. I wanted to integrated unit tests for my functions: userRegister and userLogin
This is my app.js
'use strict'
const express = require('express')
const bodyParser = require('body-parser')
const passport = require('passport')
const users = require('../routes/users')
const app = express()
const port = 5000
app.get('/', (req, res) => {
res.send({ msg: 'Test' })
})
//BodyParser Middleware
app.use(bodyParser.urlencoded({ extended: false }))
app.use(bodyParser.json())
//Passport middleware
app.use(passport.initialize())
//Initialize Routes
app.use('/api/users', users)
//Export app
module.exports = app
This is my userController.js:
'use strict'
const express = require('express')
const bcrypt = require('bcryptjs')
const jwt = require('jsonwebtoken')
const keys = require('../../../../config/keys')
const passport = require('passport')
const status = require('http-status-codes')
const User = require('../../models/User')
module.exports.test = (req, res) => {
res.json({msg: 'Users works'})
}
module.exports.userRegister = (req, res) => {
User.findOne({ email: req.body.email})
.then(user => {
if(user)
return res.status(400).json({ userEmailerror: 'Email already
exists'})
else{
const newUser = new User({
name: req.body.name,
email: req.body.email,
password: req.body.password
})
newUser.save()
.then(user => res.json(user))
.catch(err => console.log(err))
}
})
}
this is my userController.test.js
'use strict'
const mongoose = require('mongoose')
const testUserDB = require('../../../config/keys').testUsersMongURI
mongoose.connect(testUserDB)
const userController = require('../modules/controllers/userController')
const User = require('../models/User')
describe('register new user', () => {
test('succesfully register a valid user', () => {
const newUser = new User({
name: 'test',
email: 'test@gmail.com',
password: 'test123'
})
const req = {body: {newUser}}
userController.userRegister(req).then(user => {
expect(user.name).toBe('test')
expect(user.email).toBe('test@gmail.com')
})
})
})
The script I use to run the test is:
"test": "jest --coverage",
I am new to Javascript and Full stack and the error I received is confusing me
RUNS src/user/__tests__/userController.test.js
FAIL src/user/__tests__/userController.test.js
register new user
× succesfully register a valid user (19ms)
● register new user › succesfully register a valid user
TypeError: Cannot read property 'then' of undefined
16 | })
17 | const req = {body: {newUser}}
> 18 | userController.userRegister(req).then(user => {
| ^
19 | expect(user.name).toBe('test')
20 | expect(user.email).toBe('test@gmail.com')
21 |
at Object.test (src/user/__tests__/userController.test.js:18:3)
Thank you for your help!
javascript express mongoose tdd jestjs
I am trying a TDD approach on an user API. I wanted to integrated unit tests for my functions: userRegister and userLogin
This is my app.js
'use strict'
const express = require('express')
const bodyParser = require('body-parser')
const passport = require('passport')
const users = require('../routes/users')
const app = express()
const port = 5000
app.get('/', (req, res) => {
res.send({ msg: 'Test' })
})
//BodyParser Middleware
app.use(bodyParser.urlencoded({ extended: false }))
app.use(bodyParser.json())
//Passport middleware
app.use(passport.initialize())
//Initialize Routes
app.use('/api/users', users)
//Export app
module.exports = app
This is my userController.js:
'use strict'
const express = require('express')
const bcrypt = require('bcryptjs')
const jwt = require('jsonwebtoken')
const keys = require('../../../../config/keys')
const passport = require('passport')
const status = require('http-status-codes')
const User = require('../../models/User')
module.exports.test = (req, res) => {
res.json({msg: 'Users works'})
}
module.exports.userRegister = (req, res) => {
User.findOne({ email: req.body.email})
.then(user => {
if(user)
return res.status(400).json({ userEmailerror: 'Email already
exists'})
else{
const newUser = new User({
name: req.body.name,
email: req.body.email,
password: req.body.password
})
newUser.save()
.then(user => res.json(user))
.catch(err => console.log(err))
}
})
}
this is my userController.test.js
'use strict'
const mongoose = require('mongoose')
const testUserDB = require('../../../config/keys').testUsersMongURI
mongoose.connect(testUserDB)
const userController = require('../modules/controllers/userController')
const User = require('../models/User')
describe('register new user', () => {
test('succesfully register a valid user', () => {
const newUser = new User({
name: 'test',
email: 'test@gmail.com',
password: 'test123'
})
const req = {body: {newUser}}
userController.userRegister(req).then(user => {
expect(user.name).toBe('test')
expect(user.email).toBe('test@gmail.com')
})
})
})
The script I use to run the test is:
"test": "jest --coverage",
I am new to Javascript and Full stack and the error I received is confusing me
RUNS src/user/__tests__/userController.test.js
FAIL src/user/__tests__/userController.test.js
register new user
× succesfully register a valid user (19ms)
● register new user › succesfully register a valid user
TypeError: Cannot read property 'then' of undefined
16 | })
17 | const req = {body: {newUser}}
> 18 | userController.userRegister(req).then(user => {
| ^
19 | expect(user.name).toBe('test')
20 | expect(user.email).toBe('test@gmail.com')
21 |
at Object.test (src/user/__tests__/userController.test.js:18:3)
Thank you for your help!
javascript express mongoose tdd jestjs
javascript express mongoose tdd jestjs
edited Nov 23 '18 at 15:19
skyboyer
4,23811333
4,23811333
asked Nov 23 '18 at 12:17


VladVlad
34
34
1
The test is telling you the right thing:userController.userRegister
doesn't actually return anything.
– jonrsharpe
Nov 23 '18 at 12:19
I tried returning in various ways but I got same error.
– Vlad
Nov 23 '18 at 12:30
add a comment |
1
The test is telling you the right thing:userController.userRegister
doesn't actually return anything.
– jonrsharpe
Nov 23 '18 at 12:19
I tried returning in various ways but I got same error.
– Vlad
Nov 23 '18 at 12:30
1
1
The test is telling you the right thing:
userController.userRegister
doesn't actually return anything.– jonrsharpe
Nov 23 '18 at 12:19
The test is telling you the right thing:
userController.userRegister
doesn't actually return anything.– jonrsharpe
Nov 23 '18 at 12:19
I tried returning in various ways but I got same error.
– Vlad
Nov 23 '18 at 12:30
I tried returning in various ways but I got same error.
– Vlad
Nov 23 '18 at 12:30
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446586%2fjest-unit-testing-api-using-express-and-mongoose%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446586%2fjest-unit-testing-api-using-express-and-mongoose%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MAWhOsr7RvDw8BOjTXt0 Hm3 1GQz5NqrA9Q,lEP23E32IDTM L8 cPZ
1
The test is telling you the right thing:
userController.userRegister
doesn't actually return anything.– jonrsharpe
Nov 23 '18 at 12:19
I tried returning in various ways but I got same error.
– Vlad
Nov 23 '18 at 12:30