Swift return object of type from an array containing class elements
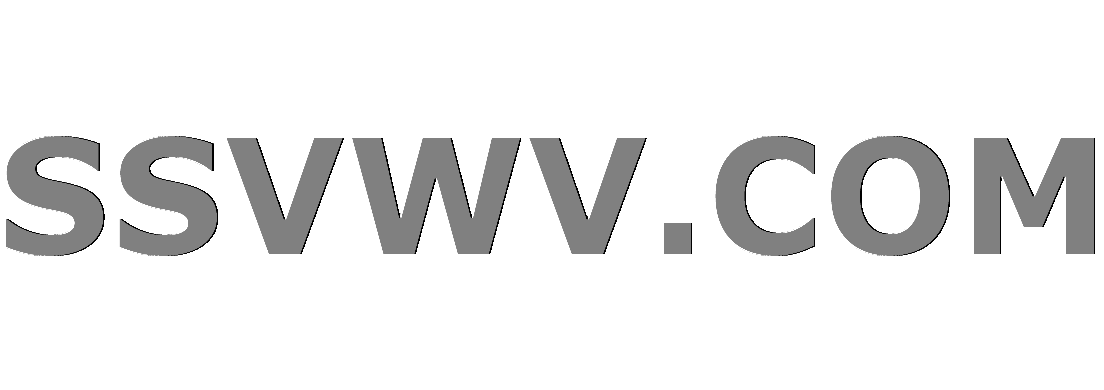
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
Here is a small code I wrote to explain the problem:
class Vehicle{
var name:String = ""
var tyres: Int = 0
}
class Bus:Vehicle{
var make:String = "Leyland"
}
class Car: Vehicle{
var model:String = "Polo"
}
let myVehicles:[Vehicle] = [
Vehicle(),
Car(),
Bus()
]
for aVehicle in myVehicles{
if(aVehicle is Bus){
print("Bus found")
}
}
From the code, I can loop through and get the object of type Bus
. However, I need a function to do the same thing and return the element of that type if available. I tried using generics but it does not work. I will need something like this:
func getVehicle(type:T.type)->T?{
// loop through the array, find if the object is of the given type.
// Return that type object.
}
arrays swift object generics subclass
add a comment |
Here is a small code I wrote to explain the problem:
class Vehicle{
var name:String = ""
var tyres: Int = 0
}
class Bus:Vehicle{
var make:String = "Leyland"
}
class Car: Vehicle{
var model:String = "Polo"
}
let myVehicles:[Vehicle] = [
Vehicle(),
Car(),
Bus()
]
for aVehicle in myVehicles{
if(aVehicle is Bus){
print("Bus found")
}
}
From the code, I can loop through and get the object of type Bus
. However, I need a function to do the same thing and return the element of that type if available. I tried using generics but it does not work. I will need something like this:
func getVehicle(type:T.type)->T?{
// loop through the array, find if the object is of the given type.
// Return that type object.
}
arrays swift object generics subclass
add a comment |
Here is a small code I wrote to explain the problem:
class Vehicle{
var name:String = ""
var tyres: Int = 0
}
class Bus:Vehicle{
var make:String = "Leyland"
}
class Car: Vehicle{
var model:String = "Polo"
}
let myVehicles:[Vehicle] = [
Vehicle(),
Car(),
Bus()
]
for aVehicle in myVehicles{
if(aVehicle is Bus){
print("Bus found")
}
}
From the code, I can loop through and get the object of type Bus
. However, I need a function to do the same thing and return the element of that type if available. I tried using generics but it does not work. I will need something like this:
func getVehicle(type:T.type)->T?{
// loop through the array, find if the object is of the given type.
// Return that type object.
}
arrays swift object generics subclass
Here is a small code I wrote to explain the problem:
class Vehicle{
var name:String = ""
var tyres: Int = 0
}
class Bus:Vehicle{
var make:String = "Leyland"
}
class Car: Vehicle{
var model:String = "Polo"
}
let myVehicles:[Vehicle] = [
Vehicle(),
Car(),
Bus()
]
for aVehicle in myVehicles{
if(aVehicle is Bus){
print("Bus found")
}
}
From the code, I can loop through and get the object of type Bus
. However, I need a function to do the same thing and return the element of that type if available. I tried using generics but it does not work. I will need something like this:
func getVehicle(type:T.type)->T?{
// loop through the array, find if the object is of the given type.
// Return that type object.
}
arrays swift object generics subclass
arrays swift object generics subclass
asked Mar 17 '17 at 9:50
NareshkumarNareshkumar
1,69821629
1,69821629
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
Use foo as? T
to try to cast foo
as type T
.
for aVehicle in myVehicles{
if let bus = aVehicle as? Bus {
print("Bus found", bus.make)
}
}
Your getVehicle
can thus be written as:
func getVehicle<T>() -> T? {
for aVehicle in myVehicles {
if let v = aVehicle as? T {
return v
}
}
return nil
}
let bus: Bus? = getVehicle()
or functionally:
func getVehicle<T>() -> T? {
return myVehicles.lazy.flatMap { $0 as? T }.first
}
let bus: Bus? = getVehicle()
(Note that we need to specify the returned variable as Bus?
so getVehicle
can infer the T
.)
So, I dont have to specify any class type for this method?
– Nareshkumar
Mar 17 '17 at 10:01
2
@Nareshkumar. The method is generic. Which type of vehicle is used is inferred from the type of the variable to which it is assigned. See kennytm writeslet bus: Bus? = getVehicle()
not justlet bus = getVehicle()
. It's the explicit type ofbus
that pickes the rightT
– JeremyP
Mar 17 '17 at 10:04
Functionally solution is quite cool I love it !!
– Oleg Gordiichuk
Mar 17 '17 at 10:04
add a comment |
You can write it like this :
func getVehicle<T>(type:T)-> [T]{
return myVehicles.filter{ $0 is T }.map{$0 as! T }
}
add a comment |
You can also use this:
func getVehicle<T>(type: T.Type) -> T? {
return myVehicles.filter { type(of: $0) == type }.first as? T
}
Usage:
getVehicle(type: Bus.self)
add a comment |
You can also use this:
let a = array.flatMap({ $0 as? MyTypeClass })
// a == [MyTypeClass] no optional
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f42854206%2fswift-return-object-of-type-from-an-array-containing-class-elements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Use foo as? T
to try to cast foo
as type T
.
for aVehicle in myVehicles{
if let bus = aVehicle as? Bus {
print("Bus found", bus.make)
}
}
Your getVehicle
can thus be written as:
func getVehicle<T>() -> T? {
for aVehicle in myVehicles {
if let v = aVehicle as? T {
return v
}
}
return nil
}
let bus: Bus? = getVehicle()
or functionally:
func getVehicle<T>() -> T? {
return myVehicles.lazy.flatMap { $0 as? T }.first
}
let bus: Bus? = getVehicle()
(Note that we need to specify the returned variable as Bus?
so getVehicle
can infer the T
.)
So, I dont have to specify any class type for this method?
– Nareshkumar
Mar 17 '17 at 10:01
2
@Nareshkumar. The method is generic. Which type of vehicle is used is inferred from the type of the variable to which it is assigned. See kennytm writeslet bus: Bus? = getVehicle()
not justlet bus = getVehicle()
. It's the explicit type ofbus
that pickes the rightT
– JeremyP
Mar 17 '17 at 10:04
Functionally solution is quite cool I love it !!
– Oleg Gordiichuk
Mar 17 '17 at 10:04
add a comment |
Use foo as? T
to try to cast foo
as type T
.
for aVehicle in myVehicles{
if let bus = aVehicle as? Bus {
print("Bus found", bus.make)
}
}
Your getVehicle
can thus be written as:
func getVehicle<T>() -> T? {
for aVehicle in myVehicles {
if let v = aVehicle as? T {
return v
}
}
return nil
}
let bus: Bus? = getVehicle()
or functionally:
func getVehicle<T>() -> T? {
return myVehicles.lazy.flatMap { $0 as? T }.first
}
let bus: Bus? = getVehicle()
(Note that we need to specify the returned variable as Bus?
so getVehicle
can infer the T
.)
So, I dont have to specify any class type for this method?
– Nareshkumar
Mar 17 '17 at 10:01
2
@Nareshkumar. The method is generic. Which type of vehicle is used is inferred from the type of the variable to which it is assigned. See kennytm writeslet bus: Bus? = getVehicle()
not justlet bus = getVehicle()
. It's the explicit type ofbus
that pickes the rightT
– JeremyP
Mar 17 '17 at 10:04
Functionally solution is quite cool I love it !!
– Oleg Gordiichuk
Mar 17 '17 at 10:04
add a comment |
Use foo as? T
to try to cast foo
as type T
.
for aVehicle in myVehicles{
if let bus = aVehicle as? Bus {
print("Bus found", bus.make)
}
}
Your getVehicle
can thus be written as:
func getVehicle<T>() -> T? {
for aVehicle in myVehicles {
if let v = aVehicle as? T {
return v
}
}
return nil
}
let bus: Bus? = getVehicle()
or functionally:
func getVehicle<T>() -> T? {
return myVehicles.lazy.flatMap { $0 as? T }.first
}
let bus: Bus? = getVehicle()
(Note that we need to specify the returned variable as Bus?
so getVehicle
can infer the T
.)
Use foo as? T
to try to cast foo
as type T
.
for aVehicle in myVehicles{
if let bus = aVehicle as? Bus {
print("Bus found", bus.make)
}
}
Your getVehicle
can thus be written as:
func getVehicle<T>() -> T? {
for aVehicle in myVehicles {
if let v = aVehicle as? T {
return v
}
}
return nil
}
let bus: Bus? = getVehicle()
or functionally:
func getVehicle<T>() -> T? {
return myVehicles.lazy.flatMap { $0 as? T }.first
}
let bus: Bus? = getVehicle()
(Note that we need to specify the returned variable as Bus?
so getVehicle
can infer the T
.)
edited Mar 17 '17 at 10:10
answered Mar 17 '17 at 9:57
kennytmkennytm
410k80920926
410k80920926
So, I dont have to specify any class type for this method?
– Nareshkumar
Mar 17 '17 at 10:01
2
@Nareshkumar. The method is generic. Which type of vehicle is used is inferred from the type of the variable to which it is assigned. See kennytm writeslet bus: Bus? = getVehicle()
not justlet bus = getVehicle()
. It's the explicit type ofbus
that pickes the rightT
– JeremyP
Mar 17 '17 at 10:04
Functionally solution is quite cool I love it !!
– Oleg Gordiichuk
Mar 17 '17 at 10:04
add a comment |
So, I dont have to specify any class type for this method?
– Nareshkumar
Mar 17 '17 at 10:01
2
@Nareshkumar. The method is generic. Which type of vehicle is used is inferred from the type of the variable to which it is assigned. See kennytm writeslet bus: Bus? = getVehicle()
not justlet bus = getVehicle()
. It's the explicit type ofbus
that pickes the rightT
– JeremyP
Mar 17 '17 at 10:04
Functionally solution is quite cool I love it !!
– Oleg Gordiichuk
Mar 17 '17 at 10:04
So, I dont have to specify any class type for this method?
– Nareshkumar
Mar 17 '17 at 10:01
So, I dont have to specify any class type for this method?
– Nareshkumar
Mar 17 '17 at 10:01
2
2
@Nareshkumar. The method is generic. Which type of vehicle is used is inferred from the type of the variable to which it is assigned. See kennytm writes
let bus: Bus? = getVehicle()
not just let bus = getVehicle()
. It's the explicit type of bus
that pickes the right T
– JeremyP
Mar 17 '17 at 10:04
@Nareshkumar. The method is generic. Which type of vehicle is used is inferred from the type of the variable to which it is assigned. See kennytm writes
let bus: Bus? = getVehicle()
not just let bus = getVehicle()
. It's the explicit type of bus
that pickes the right T
– JeremyP
Mar 17 '17 at 10:04
Functionally solution is quite cool I love it !!
– Oleg Gordiichuk
Mar 17 '17 at 10:04
Functionally solution is quite cool I love it !!
– Oleg Gordiichuk
Mar 17 '17 at 10:04
add a comment |
You can write it like this :
func getVehicle<T>(type:T)-> [T]{
return myVehicles.filter{ $0 is T }.map{$0 as! T }
}
add a comment |
You can write it like this :
func getVehicle<T>(type:T)-> [T]{
return myVehicles.filter{ $0 is T }.map{$0 as! T }
}
add a comment |
You can write it like this :
func getVehicle<T>(type:T)-> [T]{
return myVehicles.filter{ $0 is T }.map{$0 as! T }
}
You can write it like this :
func getVehicle<T>(type:T)-> [T]{
return myVehicles.filter{ $0 is T }.map{$0 as! T }
}
answered Mar 17 '17 at 10:00


Oleg GordiichukOleg Gordiichuk
9,98854377
9,98854377
add a comment |
add a comment |
You can also use this:
func getVehicle<T>(type: T.Type) -> T? {
return myVehicles.filter { type(of: $0) == type }.first as? T
}
Usage:
getVehicle(type: Bus.self)
add a comment |
You can also use this:
func getVehicle<T>(type: T.Type) -> T? {
return myVehicles.filter { type(of: $0) == type }.first as? T
}
Usage:
getVehicle(type: Bus.self)
add a comment |
You can also use this:
func getVehicle<T>(type: T.Type) -> T? {
return myVehicles.filter { type(of: $0) == type }.first as? T
}
Usage:
getVehicle(type: Bus.self)
You can also use this:
func getVehicle<T>(type: T.Type) -> T? {
return myVehicles.filter { type(of: $0) == type }.first as? T
}
Usage:
getVehicle(type: Bus.self)
answered Mar 17 '17 at 10:23


Kane CheshireKane Cheshire
1,1541117
1,1541117
add a comment |
add a comment |
You can also use this:
let a = array.flatMap({ $0 as? MyTypeClass })
// a == [MyTypeClass] no optional
add a comment |
You can also use this:
let a = array.flatMap({ $0 as? MyTypeClass })
// a == [MyTypeClass] no optional
add a comment |
You can also use this:
let a = array.flatMap({ $0 as? MyTypeClass })
// a == [MyTypeClass] no optional
You can also use this:
let a = array.flatMap({ $0 as? MyTypeClass })
// a == [MyTypeClass] no optional
answered Nov 23 '18 at 19:01


YannickStephYannickSteph
7,69123839
7,69123839
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f42854206%2fswift-return-object-of-type-from-an-array-containing-class-elements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3R3kCopoH 9 SEkptJ4J9iTYeIfMQa C,ccuHbRGd95pPGRx0D