Nested Many to Many Relationship in Laravel
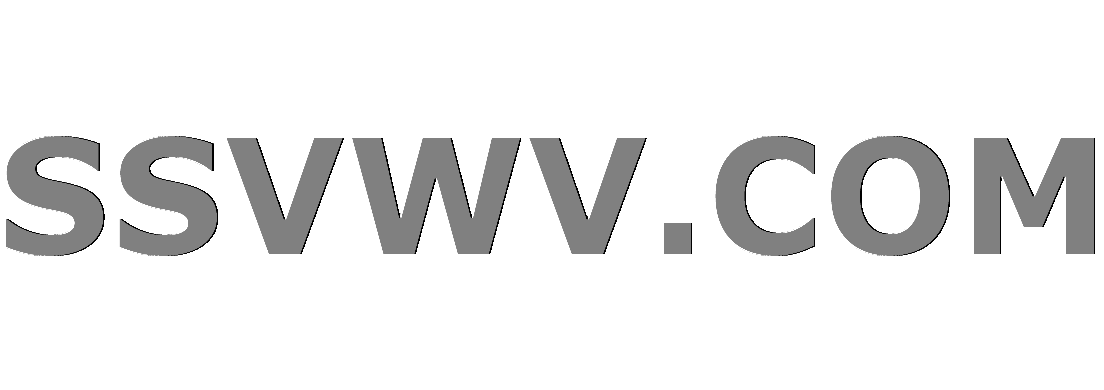
Multi tool use
up vote
0
down vote
favorite
I have the table structures as
products id
packs id
pack_products pack_id, product_id
pack_optional_products pack_id, product_id
So in the above table, pack
will have 2 type of products
, compulsory products
and optional products
, mean if customer buy the pack
the products which are attached in that pack as compulsory will automatically ordered, but customer also can see if there is any optional product in the pack so customer can also buy that product too.
Now to manage orders I have the following table.
orders id, customer_id
order_pack order_id, pack_id
Here everything works fine, using attach
method as $order->packs()->attach($pack["pack_id"]);
So here problem starts ( when I tried to add optional ordered products in the ordered pack), to manage optional products order, I have created the following table
order_pack_product order_id, pack_id, pack_optional_product_id
I have created a model as.
class Order extends Model
{
//
public function packs(){
return $this->belongsToMany(Pack::class)->withTimestamps()->withPivot('name');
}
public function parent() {
return $this->belongsTo(ParentCustomer::class);
}
}
class OrderPack extends Model
{
//
public function optionalProducts()
{
return $this->belongsToMany(PackOptionalProduct::class, 'order_pack_product')->withTimestamps();
}
}
And then I call the method as.
$order->packs()->optionalProducts()->attach($optionalProduct["pack_optional_product_id"]);
and I'm getting this error.
BadMethodCallException: Call to undefined method
IlluminateDatabaseEloquentRelationsBelongsToMany::optionalProducts()
in file
/.../....../laravel/framework/src/Illuminate/Support/Traits/ForwardsCalls.php
on line 50
SO complete code related to above tables will be this.
$order = new Order();
$order->parent_id = $parent->id;
$order->school_id = $schoolId;
if($order->save()){
foreach($json["packs"] as $pack){
$order->packs()->attach($pack["pack_id"], ["child_name" => $pack["child_name"]]);
foreach($pack["optional_products"] as $optionalProduct){
$order->packs()->optionalProducts()->attach($optionalProduct["pack_optional_product_id"]);
}
}
return response()->json(["status" => "ok", "order_id" => $order->id]);
}else{
return response()->json(["status" => "failed"]);
}
laravel many-to-many
add a comment |
up vote
0
down vote
favorite
I have the table structures as
products id
packs id
pack_products pack_id, product_id
pack_optional_products pack_id, product_id
So in the above table, pack
will have 2 type of products
, compulsory products
and optional products
, mean if customer buy the pack
the products which are attached in that pack as compulsory will automatically ordered, but customer also can see if there is any optional product in the pack so customer can also buy that product too.
Now to manage orders I have the following table.
orders id, customer_id
order_pack order_id, pack_id
Here everything works fine, using attach
method as $order->packs()->attach($pack["pack_id"]);
So here problem starts ( when I tried to add optional ordered products in the ordered pack), to manage optional products order, I have created the following table
order_pack_product order_id, pack_id, pack_optional_product_id
I have created a model as.
class Order extends Model
{
//
public function packs(){
return $this->belongsToMany(Pack::class)->withTimestamps()->withPivot('name');
}
public function parent() {
return $this->belongsTo(ParentCustomer::class);
}
}
class OrderPack extends Model
{
//
public function optionalProducts()
{
return $this->belongsToMany(PackOptionalProduct::class, 'order_pack_product')->withTimestamps();
}
}
And then I call the method as.
$order->packs()->optionalProducts()->attach($optionalProduct["pack_optional_product_id"]);
and I'm getting this error.
BadMethodCallException: Call to undefined method
IlluminateDatabaseEloquentRelationsBelongsToMany::optionalProducts()
in file
/.../....../laravel/framework/src/Illuminate/Support/Traits/ForwardsCalls.php
on line 50
SO complete code related to above tables will be this.
$order = new Order();
$order->parent_id = $parent->id;
$order->school_id = $schoolId;
if($order->save()){
foreach($json["packs"] as $pack){
$order->packs()->attach($pack["pack_id"], ["child_name" => $pack["child_name"]]);
foreach($pack["optional_products"] as $optionalProduct){
$order->packs()->optionalProducts()->attach($optionalProduct["pack_optional_product_id"]);
}
}
return response()->json(["status" => "ok", "order_id" => $order->id]);
}else{
return response()->json(["status" => "failed"]);
}
laravel many-to-many
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have the table structures as
products id
packs id
pack_products pack_id, product_id
pack_optional_products pack_id, product_id
So in the above table, pack
will have 2 type of products
, compulsory products
and optional products
, mean if customer buy the pack
the products which are attached in that pack as compulsory will automatically ordered, but customer also can see if there is any optional product in the pack so customer can also buy that product too.
Now to manage orders I have the following table.
orders id, customer_id
order_pack order_id, pack_id
Here everything works fine, using attach
method as $order->packs()->attach($pack["pack_id"]);
So here problem starts ( when I tried to add optional ordered products in the ordered pack), to manage optional products order, I have created the following table
order_pack_product order_id, pack_id, pack_optional_product_id
I have created a model as.
class Order extends Model
{
//
public function packs(){
return $this->belongsToMany(Pack::class)->withTimestamps()->withPivot('name');
}
public function parent() {
return $this->belongsTo(ParentCustomer::class);
}
}
class OrderPack extends Model
{
//
public function optionalProducts()
{
return $this->belongsToMany(PackOptionalProduct::class, 'order_pack_product')->withTimestamps();
}
}
And then I call the method as.
$order->packs()->optionalProducts()->attach($optionalProduct["pack_optional_product_id"]);
and I'm getting this error.
BadMethodCallException: Call to undefined method
IlluminateDatabaseEloquentRelationsBelongsToMany::optionalProducts()
in file
/.../....../laravel/framework/src/Illuminate/Support/Traits/ForwardsCalls.php
on line 50
SO complete code related to above tables will be this.
$order = new Order();
$order->parent_id = $parent->id;
$order->school_id = $schoolId;
if($order->save()){
foreach($json["packs"] as $pack){
$order->packs()->attach($pack["pack_id"], ["child_name" => $pack["child_name"]]);
foreach($pack["optional_products"] as $optionalProduct){
$order->packs()->optionalProducts()->attach($optionalProduct["pack_optional_product_id"]);
}
}
return response()->json(["status" => "ok", "order_id" => $order->id]);
}else{
return response()->json(["status" => "failed"]);
}
laravel many-to-many
I have the table structures as
products id
packs id
pack_products pack_id, product_id
pack_optional_products pack_id, product_id
So in the above table, pack
will have 2 type of products
, compulsory products
and optional products
, mean if customer buy the pack
the products which are attached in that pack as compulsory will automatically ordered, but customer also can see if there is any optional product in the pack so customer can also buy that product too.
Now to manage orders I have the following table.
orders id, customer_id
order_pack order_id, pack_id
Here everything works fine, using attach
method as $order->packs()->attach($pack["pack_id"]);
So here problem starts ( when I tried to add optional ordered products in the ordered pack), to manage optional products order, I have created the following table
order_pack_product order_id, pack_id, pack_optional_product_id
I have created a model as.
class Order extends Model
{
//
public function packs(){
return $this->belongsToMany(Pack::class)->withTimestamps()->withPivot('name');
}
public function parent() {
return $this->belongsTo(ParentCustomer::class);
}
}
class OrderPack extends Model
{
//
public function optionalProducts()
{
return $this->belongsToMany(PackOptionalProduct::class, 'order_pack_product')->withTimestamps();
}
}
And then I call the method as.
$order->packs()->optionalProducts()->attach($optionalProduct["pack_optional_product_id"]);
and I'm getting this error.
BadMethodCallException: Call to undefined method
IlluminateDatabaseEloquentRelationsBelongsToMany::optionalProducts()
in file
/.../....../laravel/framework/src/Illuminate/Support/Traits/ForwardsCalls.php
on line 50
SO complete code related to above tables will be this.
$order = new Order();
$order->parent_id = $parent->id;
$order->school_id = $schoolId;
if($order->save()){
foreach($json["packs"] as $pack){
$order->packs()->attach($pack["pack_id"], ["child_name" => $pack["child_name"]]);
foreach($pack["optional_products"] as $optionalProduct){
$order->packs()->optionalProducts()->attach($optionalProduct["pack_optional_product_id"]);
}
}
return response()->json(["status" => "ok", "order_id" => $order->id]);
}else{
return response()->json(["status" => "failed"]);
}
laravel many-to-many
laravel many-to-many
edited Nov 17 at 16:19
Jonas Staudenmeir
11.4k2932
11.4k2932
asked Nov 17 at 11:51
Asif Mushtaq
1,3251534
1,3251534
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
optionalProducts()
is method of model, not of relation packs()
.
Method $order->packs()
- returns relation object, attribute $order->packs
- returns collection of pack models.
You need to iterate trough collection, to attach optionalProducts to every one pack model.
foreach($pack["optional_products"] as $optionalProduct){
foreach($order->packs as $item){
$item->optionalProducts()->attach($optionalProduct["pack_optional_product_id"])
}
}
Perhaps, this code will not correct the error, then you need to correct your code logic.
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
optionalProducts()
is method of model, not of relation packs()
.
Method $order->packs()
- returns relation object, attribute $order->packs
- returns collection of pack models.
You need to iterate trough collection, to attach optionalProducts to every one pack model.
foreach($pack["optional_products"] as $optionalProduct){
foreach($order->packs as $item){
$item->optionalProducts()->attach($optionalProduct["pack_optional_product_id"])
}
}
Perhaps, this code will not correct the error, then you need to correct your code logic.
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
optionalProducts()
is method of model, not of relation packs()
.
Method $order->packs()
- returns relation object, attribute $order->packs
- returns collection of pack models.
You need to iterate trough collection, to attach optionalProducts to every one pack model.
foreach($pack["optional_products"] as $optionalProduct){
foreach($order->packs as $item){
$item->optionalProducts()->attach($optionalProduct["pack_optional_product_id"])
}
}
Perhaps, this code will not correct the error, then you need to correct your code logic.
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
up vote
0
down vote
optionalProducts()
is method of model, not of relation packs()
.
Method $order->packs()
- returns relation object, attribute $order->packs
- returns collection of pack models.
You need to iterate trough collection, to attach optionalProducts to every one pack model.
foreach($pack["optional_products"] as $optionalProduct){
foreach($order->packs as $item){
$item->optionalProducts()->attach($optionalProduct["pack_optional_product_id"])
}
}
Perhaps, this code will not correct the error, then you need to correct your code logic.
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
optionalProducts()
is method of model, not of relation packs()
.
Method $order->packs()
- returns relation object, attribute $order->packs
- returns collection of pack models.
You need to iterate trough collection, to attach optionalProducts to every one pack model.
foreach($pack["optional_products"] as $optionalProduct){
foreach($order->packs as $item){
$item->optionalProducts()->attach($optionalProduct["pack_optional_product_id"])
}
}
Perhaps, this code will not correct the error, then you need to correct your code logic.
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited Nov 17 at 15:18
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered Nov 17 at 14:23
IndianCoding
4597
4597
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
IndianCoding is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53350955%2fnested-many-to-many-relationship-in-laravel%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MHFHij uV Et0FLxEgmiyjYjp24 p9,6LYLAH5TxO8cA6,dG9Z2wmocPrmE22TDkdx,B7y,RQKNL9x0eu2eXfcrypSCdKYy6kJtaz4Cj