Cloud Firestore Authentication iOS
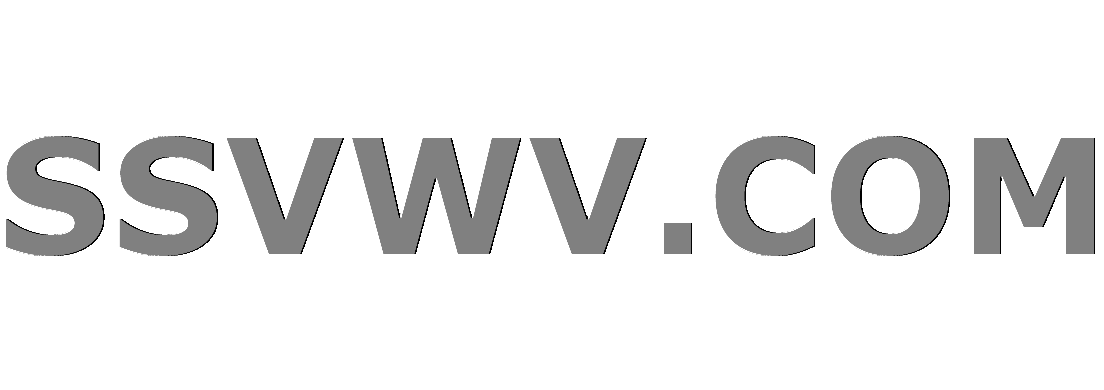
Multi tool use
up vote
0
down vote
favorite
I'm very new to iOS development (learning as I go) and I'm using Cloud Firestore to authenticate users via email/password. I'm trying to do a simple email & password authentication. I used one of the db rules from documentation and I don't think I have to create collections and documents in the rules. I've already tested my connection to the db so that's not an issue either. The code I have so far is below:
import UIKit
import Firebase
class SignUpViewController: UIViewController {
//Outlets
@IBOutlet weak var firstNameText: UITextField!
@IBOutlet weak var lastNameText: UITextField!
@IBOutlet weak var emailText: UITextField!
@IBOutlet weak var passwordText: UITextField!
@IBOutlet weak var signUpButton: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func signUpButtonTapped(_ sender: Any) {
guard let firstName = firstNameText.text,
let lastName = lastNameText.text,
let email = emailText.text,
let password = passwordText.text else { return }
Auth.auth().createUser(withEmail: email, password: password) { (authResult, error) in
if let error = error {
debugPrint("Error creating user: (error.localizedDescription)")
}
let changeRequest = Auth.auth().currentUser?.createProfileChangeRequest()
changeRequest?.displayName = firstName
changeRequest?.commitChanges(completion: { (error) in
if let error = error {
debugPrint(error.localizedDescription)
}
})
guard let userId = authResult?.user else { return }
Firestore.firestore().collection(USERS_REF).document(userId).setData([
USERNAME : firstName,
DATE_CREATED : FieldValue.serverTimestamp()
], completion: { (error) in
if let error = error {
debugPrint(error.localizedDescription)
} else {
self.dismiss(animated: true, completion: nil)
}
})
}
}
}
But I get the error:
- Cannot invoke 'setData' with an argument list of type '([String : Any], completion: ((Error?) -> Void)?)'
Is there something I'm not doing? Could someone help with this email/password authentication?
ios swift firebase-authentication google-cloud-firestore
add a comment |
up vote
0
down vote
favorite
I'm very new to iOS development (learning as I go) and I'm using Cloud Firestore to authenticate users via email/password. I'm trying to do a simple email & password authentication. I used one of the db rules from documentation and I don't think I have to create collections and documents in the rules. I've already tested my connection to the db so that's not an issue either. The code I have so far is below:
import UIKit
import Firebase
class SignUpViewController: UIViewController {
//Outlets
@IBOutlet weak var firstNameText: UITextField!
@IBOutlet weak var lastNameText: UITextField!
@IBOutlet weak var emailText: UITextField!
@IBOutlet weak var passwordText: UITextField!
@IBOutlet weak var signUpButton: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func signUpButtonTapped(_ sender: Any) {
guard let firstName = firstNameText.text,
let lastName = lastNameText.text,
let email = emailText.text,
let password = passwordText.text else { return }
Auth.auth().createUser(withEmail: email, password: password) { (authResult, error) in
if let error = error {
debugPrint("Error creating user: (error.localizedDescription)")
}
let changeRequest = Auth.auth().currentUser?.createProfileChangeRequest()
changeRequest?.displayName = firstName
changeRequest?.commitChanges(completion: { (error) in
if let error = error {
debugPrint(error.localizedDescription)
}
})
guard let userId = authResult?.user else { return }
Firestore.firestore().collection(USERS_REF).document(userId).setData([
USERNAME : firstName,
DATE_CREATED : FieldValue.serverTimestamp()
], completion: { (error) in
if let error = error {
debugPrint(error.localizedDescription)
} else {
self.dismiss(animated: true, completion: nil)
}
})
}
}
}
But I get the error:
- Cannot invoke 'setData' with an argument list of type '([String : Any], completion: ((Error?) -> Void)?)'
Is there something I'm not doing? Could someone help with this email/password authentication?
ios swift firebase-authentication google-cloud-firestore
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm very new to iOS development (learning as I go) and I'm using Cloud Firestore to authenticate users via email/password. I'm trying to do a simple email & password authentication. I used one of the db rules from documentation and I don't think I have to create collections and documents in the rules. I've already tested my connection to the db so that's not an issue either. The code I have so far is below:
import UIKit
import Firebase
class SignUpViewController: UIViewController {
//Outlets
@IBOutlet weak var firstNameText: UITextField!
@IBOutlet weak var lastNameText: UITextField!
@IBOutlet weak var emailText: UITextField!
@IBOutlet weak var passwordText: UITextField!
@IBOutlet weak var signUpButton: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func signUpButtonTapped(_ sender: Any) {
guard let firstName = firstNameText.text,
let lastName = lastNameText.text,
let email = emailText.text,
let password = passwordText.text else { return }
Auth.auth().createUser(withEmail: email, password: password) { (authResult, error) in
if let error = error {
debugPrint("Error creating user: (error.localizedDescription)")
}
let changeRequest = Auth.auth().currentUser?.createProfileChangeRequest()
changeRequest?.displayName = firstName
changeRequest?.commitChanges(completion: { (error) in
if let error = error {
debugPrint(error.localizedDescription)
}
})
guard let userId = authResult?.user else { return }
Firestore.firestore().collection(USERS_REF).document(userId).setData([
USERNAME : firstName,
DATE_CREATED : FieldValue.serverTimestamp()
], completion: { (error) in
if let error = error {
debugPrint(error.localizedDescription)
} else {
self.dismiss(animated: true, completion: nil)
}
})
}
}
}
But I get the error:
- Cannot invoke 'setData' with an argument list of type '([String : Any], completion: ((Error?) -> Void)?)'
Is there something I'm not doing? Could someone help with this email/password authentication?
ios swift firebase-authentication google-cloud-firestore
I'm very new to iOS development (learning as I go) and I'm using Cloud Firestore to authenticate users via email/password. I'm trying to do a simple email & password authentication. I used one of the db rules from documentation and I don't think I have to create collections and documents in the rules. I've already tested my connection to the db so that's not an issue either. The code I have so far is below:
import UIKit
import Firebase
class SignUpViewController: UIViewController {
//Outlets
@IBOutlet weak var firstNameText: UITextField!
@IBOutlet weak var lastNameText: UITextField!
@IBOutlet weak var emailText: UITextField!
@IBOutlet weak var passwordText: UITextField!
@IBOutlet weak var signUpButton: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func signUpButtonTapped(_ sender: Any) {
guard let firstName = firstNameText.text,
let lastName = lastNameText.text,
let email = emailText.text,
let password = passwordText.text else { return }
Auth.auth().createUser(withEmail: email, password: password) { (authResult, error) in
if let error = error {
debugPrint("Error creating user: (error.localizedDescription)")
}
let changeRequest = Auth.auth().currentUser?.createProfileChangeRequest()
changeRequest?.displayName = firstName
changeRequest?.commitChanges(completion: { (error) in
if let error = error {
debugPrint(error.localizedDescription)
}
})
guard let userId = authResult?.user else { return }
Firestore.firestore().collection(USERS_REF).document(userId).setData([
USERNAME : firstName,
DATE_CREATED : FieldValue.serverTimestamp()
], completion: { (error) in
if let error = error {
debugPrint(error.localizedDescription)
} else {
self.dismiss(animated: true, completion: nil)
}
})
}
}
}
But I get the error:
- Cannot invoke 'setData' with an argument list of type '([String : Any], completion: ((Error?) -> Void)?)'
Is there something I'm not doing? Could someone help with this email/password authentication?
ios swift firebase-authentication google-cloud-firestore
ios swift firebase-authentication google-cloud-firestore
edited Nov 20 at 18:09
asked Nov 19 at 12:18
CadenJoe
11
11
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
First check how variables are named properly. In swift we use camelCase.
Second you have tried to assign your textField object to your password inside dictionary.
Third you have tried to print and do a segue outside login action.
Consider editing your code:
@IBAction func SMLoginTapped(_ sender: Any) {
print("Login button tapped!")
guard let emailText = EmailTextField.text, !emailText.isEmpty else { return }
guard let passwordText = PasswordTextField.text, !passwordText.isEmpty else { return }
let saveData: [String: Any] = ["email": emailText, "password": passwordText]
docRef.setData(saveData) { (error) in
if let error = error {
print("Oh no! Got an error: (error.localizedDescription)")
} else {
print("Data has been saved to Firestore!")
self.performSegue(withIdentifier: "SecondViewSegue", sender: self)
}
}
}
Thanks man, the projects builds now but it won't login the user. It recognizes the tap (gives "login button tapped" message) but doesn't actually login the user and go to the next segue. Any reason why?
– CadenJoe
Nov 20 at 12:27
Try usingdb
insteaddocRef
– DionizB
Nov 20 at 15:28
I've taken a different approach, but not sure about the above error. I changed the user reference to authResult and then this error came up. Any idea how to troubleshoot it? @DionizB
– CadenJoe
Nov 20 at 18:12
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
First check how variables are named properly. In swift we use camelCase.
Second you have tried to assign your textField object to your password inside dictionary.
Third you have tried to print and do a segue outside login action.
Consider editing your code:
@IBAction func SMLoginTapped(_ sender: Any) {
print("Login button tapped!")
guard let emailText = EmailTextField.text, !emailText.isEmpty else { return }
guard let passwordText = PasswordTextField.text, !passwordText.isEmpty else { return }
let saveData: [String: Any] = ["email": emailText, "password": passwordText]
docRef.setData(saveData) { (error) in
if let error = error {
print("Oh no! Got an error: (error.localizedDescription)")
} else {
print("Data has been saved to Firestore!")
self.performSegue(withIdentifier: "SecondViewSegue", sender: self)
}
}
}
Thanks man, the projects builds now but it won't login the user. It recognizes the tap (gives "login button tapped" message) but doesn't actually login the user and go to the next segue. Any reason why?
– CadenJoe
Nov 20 at 12:27
Try usingdb
insteaddocRef
– DionizB
Nov 20 at 15:28
I've taken a different approach, but not sure about the above error. I changed the user reference to authResult and then this error came up. Any idea how to troubleshoot it? @DionizB
– CadenJoe
Nov 20 at 18:12
add a comment |
up vote
1
down vote
First check how variables are named properly. In swift we use camelCase.
Second you have tried to assign your textField object to your password inside dictionary.
Third you have tried to print and do a segue outside login action.
Consider editing your code:
@IBAction func SMLoginTapped(_ sender: Any) {
print("Login button tapped!")
guard let emailText = EmailTextField.text, !emailText.isEmpty else { return }
guard let passwordText = PasswordTextField.text, !passwordText.isEmpty else { return }
let saveData: [String: Any] = ["email": emailText, "password": passwordText]
docRef.setData(saveData) { (error) in
if let error = error {
print("Oh no! Got an error: (error.localizedDescription)")
} else {
print("Data has been saved to Firestore!")
self.performSegue(withIdentifier: "SecondViewSegue", sender: self)
}
}
}
Thanks man, the projects builds now but it won't login the user. It recognizes the tap (gives "login button tapped" message) but doesn't actually login the user and go to the next segue. Any reason why?
– CadenJoe
Nov 20 at 12:27
Try usingdb
insteaddocRef
– DionizB
Nov 20 at 15:28
I've taken a different approach, but not sure about the above error. I changed the user reference to authResult and then this error came up. Any idea how to troubleshoot it? @DionizB
– CadenJoe
Nov 20 at 18:12
add a comment |
up vote
1
down vote
up vote
1
down vote
First check how variables are named properly. In swift we use camelCase.
Second you have tried to assign your textField object to your password inside dictionary.
Third you have tried to print and do a segue outside login action.
Consider editing your code:
@IBAction func SMLoginTapped(_ sender: Any) {
print("Login button tapped!")
guard let emailText = EmailTextField.text, !emailText.isEmpty else { return }
guard let passwordText = PasswordTextField.text, !passwordText.isEmpty else { return }
let saveData: [String: Any] = ["email": emailText, "password": passwordText]
docRef.setData(saveData) { (error) in
if let error = error {
print("Oh no! Got an error: (error.localizedDescription)")
} else {
print("Data has been saved to Firestore!")
self.performSegue(withIdentifier: "SecondViewSegue", sender: self)
}
}
}
First check how variables are named properly. In swift we use camelCase.
Second you have tried to assign your textField object to your password inside dictionary.
Third you have tried to print and do a segue outside login action.
Consider editing your code:
@IBAction func SMLoginTapped(_ sender: Any) {
print("Login button tapped!")
guard let emailText = EmailTextField.text, !emailText.isEmpty else { return }
guard let passwordText = PasswordTextField.text, !passwordText.isEmpty else { return }
let saveData: [String: Any] = ["email": emailText, "password": passwordText]
docRef.setData(saveData) { (error) in
if let error = error {
print("Oh no! Got an error: (error.localizedDescription)")
} else {
print("Data has been saved to Firestore!")
self.performSegue(withIdentifier: "SecondViewSegue", sender: self)
}
}
}
answered Nov 19 at 13:20
DionizB
8661313
8661313
Thanks man, the projects builds now but it won't login the user. It recognizes the tap (gives "login button tapped" message) but doesn't actually login the user and go to the next segue. Any reason why?
– CadenJoe
Nov 20 at 12:27
Try usingdb
insteaddocRef
– DionizB
Nov 20 at 15:28
I've taken a different approach, but not sure about the above error. I changed the user reference to authResult and then this error came up. Any idea how to troubleshoot it? @DionizB
– CadenJoe
Nov 20 at 18:12
add a comment |
Thanks man, the projects builds now but it won't login the user. It recognizes the tap (gives "login button tapped" message) but doesn't actually login the user and go to the next segue. Any reason why?
– CadenJoe
Nov 20 at 12:27
Try usingdb
insteaddocRef
– DionizB
Nov 20 at 15:28
I've taken a different approach, but not sure about the above error. I changed the user reference to authResult and then this error came up. Any idea how to troubleshoot it? @DionizB
– CadenJoe
Nov 20 at 18:12
Thanks man, the projects builds now but it won't login the user. It recognizes the tap (gives "login button tapped" message) but doesn't actually login the user and go to the next segue. Any reason why?
– CadenJoe
Nov 20 at 12:27
Thanks man, the projects builds now but it won't login the user. It recognizes the tap (gives "login button tapped" message) but doesn't actually login the user and go to the next segue. Any reason why?
– CadenJoe
Nov 20 at 12:27
Try using
db
instead docRef
– DionizB
Nov 20 at 15:28
Try using
db
instead docRef
– DionizB
Nov 20 at 15:28
I've taken a different approach, but not sure about the above error. I changed the user reference to authResult and then this error came up. Any idea how to troubleshoot it? @DionizB
– CadenJoe
Nov 20 at 18:12
I've taken a different approach, but not sure about the above error. I changed the user reference to authResult and then this error came up. Any idea how to troubleshoot it? @DionizB
– CadenJoe
Nov 20 at 18:12
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53374479%2fcloud-firestore-authentication-ios%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
IjftwIDqSjlM,WQgFW62BTvI2gijh