angular 6 interceptor is called after request is sent
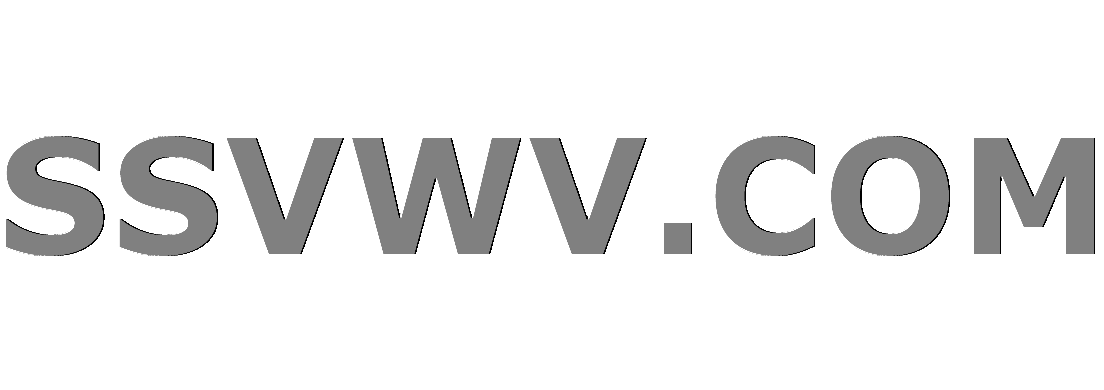
Multi tool use
I'm working on an angular 6 app where I want to add an jwt-token to the authorize-header of every request. For this scenario I want to use an interceptor.
The code looks like this:
import { HttpEvent, HttpHandler, HttpInterceptor, HttpRequest } from "@angular/common/http";
import { Injectable } from "@angular/core";
import { Observable } from "rxjs";
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
let sessionId = localStorage.getItem('sessionId');
if (sessionId) {
request = request.clone({
setHeaders: {
Authorization: `Bearer ${sessionId}`
}
});
console.log("With AuthHeader");
}
return next.handle(request);
}
}
Here is my code for sending the request:
httpQuery(ressource): Observable<T> {
var absoluteUrl = this.getAbsoluteUrl(ressource);
console.log("Sending");
return this.httpClient
.get<T>(absoluteUrl);
}
However I face the following problem:
The interceptor gets fired, but AFTER the request is sent. I can see this in developer console where the log-message from the http-method comes right before the log-message from the interceptor:
As you can see, this leeds to a 401 response from the server which expects the authorization-header.
I read a ton of tutorials and stackoverflow-questions about interceptor, but I can't see my mistake.
Maybe some of guys have a hint for me?
Thank you and best regards,
Alex
angular interceptor angular-http-interceptors
add a comment |
I'm working on an angular 6 app where I want to add an jwt-token to the authorize-header of every request. For this scenario I want to use an interceptor.
The code looks like this:
import { HttpEvent, HttpHandler, HttpInterceptor, HttpRequest } from "@angular/common/http";
import { Injectable } from "@angular/core";
import { Observable } from "rxjs";
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
let sessionId = localStorage.getItem('sessionId');
if (sessionId) {
request = request.clone({
setHeaders: {
Authorization: `Bearer ${sessionId}`
}
});
console.log("With AuthHeader");
}
return next.handle(request);
}
}
Here is my code for sending the request:
httpQuery(ressource): Observable<T> {
var absoluteUrl = this.getAbsoluteUrl(ressource);
console.log("Sending");
return this.httpClient
.get<T>(absoluteUrl);
}
However I face the following problem:
The interceptor gets fired, but AFTER the request is sent. I can see this in developer console where the log-message from the http-method comes right before the log-message from the interceptor:
As you can see, this leeds to a 401 response from the server which expects the authorization-header.
I read a ton of tutorials and stackoverflow-questions about interceptor, but I can't see my mistake.
Maybe some of guys have a hint for me?
Thank you and best regards,
Alex
angular interceptor angular-http-interceptors
Please check your network tab for the request where the Authorization header is added or not.
– Sheik Althaf
Nov 20 '18 at 13:47
I did and it is not sent (with different browsers). Interesting side note: fiddler doesn't notice this particular request at all. Every request I make is captured by fiddler, but this one request which results in a 401-response not. Only Wireshark captures this request and the response. And in Wireshark there is no authorization-header as well.
– Alex
Nov 20 '18 at 13:55
add a comment |
I'm working on an angular 6 app where I want to add an jwt-token to the authorize-header of every request. For this scenario I want to use an interceptor.
The code looks like this:
import { HttpEvent, HttpHandler, HttpInterceptor, HttpRequest } from "@angular/common/http";
import { Injectable } from "@angular/core";
import { Observable } from "rxjs";
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
let sessionId = localStorage.getItem('sessionId');
if (sessionId) {
request = request.clone({
setHeaders: {
Authorization: `Bearer ${sessionId}`
}
});
console.log("With AuthHeader");
}
return next.handle(request);
}
}
Here is my code for sending the request:
httpQuery(ressource): Observable<T> {
var absoluteUrl = this.getAbsoluteUrl(ressource);
console.log("Sending");
return this.httpClient
.get<T>(absoluteUrl);
}
However I face the following problem:
The interceptor gets fired, but AFTER the request is sent. I can see this in developer console where the log-message from the http-method comes right before the log-message from the interceptor:
As you can see, this leeds to a 401 response from the server which expects the authorization-header.
I read a ton of tutorials and stackoverflow-questions about interceptor, but I can't see my mistake.
Maybe some of guys have a hint for me?
Thank you and best regards,
Alex
angular interceptor angular-http-interceptors
I'm working on an angular 6 app where I want to add an jwt-token to the authorize-header of every request. For this scenario I want to use an interceptor.
The code looks like this:
import { HttpEvent, HttpHandler, HttpInterceptor, HttpRequest } from "@angular/common/http";
import { Injectable } from "@angular/core";
import { Observable } from "rxjs";
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
let sessionId = localStorage.getItem('sessionId');
if (sessionId) {
request = request.clone({
setHeaders: {
Authorization: `Bearer ${sessionId}`
}
});
console.log("With AuthHeader");
}
return next.handle(request);
}
}
Here is my code for sending the request:
httpQuery(ressource): Observable<T> {
var absoluteUrl = this.getAbsoluteUrl(ressource);
console.log("Sending");
return this.httpClient
.get<T>(absoluteUrl);
}
However I face the following problem:
The interceptor gets fired, but AFTER the request is sent. I can see this in developer console where the log-message from the http-method comes right before the log-message from the interceptor:
As you can see, this leeds to a 401 response from the server which expects the authorization-header.
I read a ton of tutorials and stackoverflow-questions about interceptor, but I can't see my mistake.
Maybe some of guys have a hint for me?
Thank you and best regards,
Alex
import { HttpEvent, HttpHandler, HttpInterceptor, HttpRequest } from "@angular/common/http";
import { Injectable } from "@angular/core";
import { Observable } from "rxjs";
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
let sessionId = localStorage.getItem('sessionId');
if (sessionId) {
request = request.clone({
setHeaders: {
Authorization: `Bearer ${sessionId}`
}
});
console.log("With AuthHeader");
}
return next.handle(request);
}
}
import { HttpEvent, HttpHandler, HttpInterceptor, HttpRequest } from "@angular/common/http";
import { Injectable } from "@angular/core";
import { Observable } from "rxjs";
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
let sessionId = localStorage.getItem('sessionId');
if (sessionId) {
request = request.clone({
setHeaders: {
Authorization: `Bearer ${sessionId}`
}
});
console.log("With AuthHeader");
}
return next.handle(request);
}
}
httpQuery(ressource): Observable<T> {
var absoluteUrl = this.getAbsoluteUrl(ressource);
console.log("Sending");
return this.httpClient
.get<T>(absoluteUrl);
}
httpQuery(ressource): Observable<T> {
var absoluteUrl = this.getAbsoluteUrl(ressource);
console.log("Sending");
return this.httpClient
.get<T>(absoluteUrl);
}
angular interceptor angular-http-interceptors
angular interceptor angular-http-interceptors
asked Nov 20 '18 at 13:31
AlexAlex
81346
81346
Please check your network tab for the request where the Authorization header is added or not.
– Sheik Althaf
Nov 20 '18 at 13:47
I did and it is not sent (with different browsers). Interesting side note: fiddler doesn't notice this particular request at all. Every request I make is captured by fiddler, but this one request which results in a 401-response not. Only Wireshark captures this request and the response. And in Wireshark there is no authorization-header as well.
– Alex
Nov 20 '18 at 13:55
add a comment |
Please check your network tab for the request where the Authorization header is added or not.
– Sheik Althaf
Nov 20 '18 at 13:47
I did and it is not sent (with different browsers). Interesting side note: fiddler doesn't notice this particular request at all. Every request I make is captured by fiddler, but this one request which results in a 401-response not. Only Wireshark captures this request and the response. And in Wireshark there is no authorization-header as well.
– Alex
Nov 20 '18 at 13:55
Please check your network tab for the request where the Authorization header is added or not.
– Sheik Althaf
Nov 20 '18 at 13:47
Please check your network tab for the request where the Authorization header is added or not.
– Sheik Althaf
Nov 20 '18 at 13:47
I did and it is not sent (with different browsers). Interesting side note: fiddler doesn't notice this particular request at all. Every request I make is captured by fiddler, but this one request which results in a 401-response not. Only Wireshark captures this request and the response. And in Wireshark there is no authorization-header as well.
– Alex
Nov 20 '18 at 13:55
I did and it is not sent (with different browsers). Interesting side note: fiddler doesn't notice this particular request at all. Every request I make is captured by fiddler, but this one request which results in a 401-response not. Only Wireshark captures this request and the response. And in Wireshark there is no authorization-header as well.
– Alex
Nov 20 '18 at 13:55
add a comment |
2 Answers
2
active
oldest
votes
What you are showing is just fine
console.log("Sending"); //here you print to the cosole
return this.httpClient // here you actually MAKE http call.
.get<T>(absoluteUrl);
So your interceptor is called DURING request execution and BEFORE actual XHR request is made.
Thank you for the replay. As you can see in the screenshot, the error (which occurs because of the 401 response from the server) is catched BEFORE the interceptor is executed, too. And also, apart from the log, the authorization-header not gets sent whith the request...
– Alex
Nov 20 '18 at 13:38
No, this is just how browser console works. Order of printout is not guaranteed and might be delayed in case of objects (exception here). I faces similar wtf issue in the past.
– Antoniossss
Nov 20 '18 at 13:42
This may be true but as I said, apart from the log it doesn't work
– Alex
Nov 20 '18 at 13:45
And that is the different part of story. You can see in the broser what kind of request is actually made and you will see that probably bearer is there.
– Antoniossss
Nov 20 '18 at 14:00
I checked the actual request in the network-tab of different browsers as well as in wireshark and I can say, there is no authorization-header
– Alex
Nov 20 '18 at 14:03
|
show 1 more comment
Please try like this one
const headers = request.headers.append('Authorization', `Bearer ${sessionId}`);
const d = request.clone({ headers: headers });
return next.handle(request);
Thanks for the reply. I tried this but it doesn't work
– Alex
Nov 20 '18 at 14:41
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53394140%2fangular-6-interceptor-is-called-after-request-is-sent%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
What you are showing is just fine
console.log("Sending"); //here you print to the cosole
return this.httpClient // here you actually MAKE http call.
.get<T>(absoluteUrl);
So your interceptor is called DURING request execution and BEFORE actual XHR request is made.
Thank you for the replay. As you can see in the screenshot, the error (which occurs because of the 401 response from the server) is catched BEFORE the interceptor is executed, too. And also, apart from the log, the authorization-header not gets sent whith the request...
– Alex
Nov 20 '18 at 13:38
No, this is just how browser console works. Order of printout is not guaranteed and might be delayed in case of objects (exception here). I faces similar wtf issue in the past.
– Antoniossss
Nov 20 '18 at 13:42
This may be true but as I said, apart from the log it doesn't work
– Alex
Nov 20 '18 at 13:45
And that is the different part of story. You can see in the broser what kind of request is actually made and you will see that probably bearer is there.
– Antoniossss
Nov 20 '18 at 14:00
I checked the actual request in the network-tab of different browsers as well as in wireshark and I can say, there is no authorization-header
– Alex
Nov 20 '18 at 14:03
|
show 1 more comment
What you are showing is just fine
console.log("Sending"); //here you print to the cosole
return this.httpClient // here you actually MAKE http call.
.get<T>(absoluteUrl);
So your interceptor is called DURING request execution and BEFORE actual XHR request is made.
Thank you for the replay. As you can see in the screenshot, the error (which occurs because of the 401 response from the server) is catched BEFORE the interceptor is executed, too. And also, apart from the log, the authorization-header not gets sent whith the request...
– Alex
Nov 20 '18 at 13:38
No, this is just how browser console works. Order of printout is not guaranteed and might be delayed in case of objects (exception here). I faces similar wtf issue in the past.
– Antoniossss
Nov 20 '18 at 13:42
This may be true but as I said, apart from the log it doesn't work
– Alex
Nov 20 '18 at 13:45
And that is the different part of story. You can see in the broser what kind of request is actually made and you will see that probably bearer is there.
– Antoniossss
Nov 20 '18 at 14:00
I checked the actual request in the network-tab of different browsers as well as in wireshark and I can say, there is no authorization-header
– Alex
Nov 20 '18 at 14:03
|
show 1 more comment
What you are showing is just fine
console.log("Sending"); //here you print to the cosole
return this.httpClient // here you actually MAKE http call.
.get<T>(absoluteUrl);
So your interceptor is called DURING request execution and BEFORE actual XHR request is made.
What you are showing is just fine
console.log("Sending"); //here you print to the cosole
return this.httpClient // here you actually MAKE http call.
.get<T>(absoluteUrl);
So your interceptor is called DURING request execution and BEFORE actual XHR request is made.
answered Nov 20 '18 at 13:33
AntoniossssAntoniossss
15.2k12253
15.2k12253
Thank you for the replay. As you can see in the screenshot, the error (which occurs because of the 401 response from the server) is catched BEFORE the interceptor is executed, too. And also, apart from the log, the authorization-header not gets sent whith the request...
– Alex
Nov 20 '18 at 13:38
No, this is just how browser console works. Order of printout is not guaranteed and might be delayed in case of objects (exception here). I faces similar wtf issue in the past.
– Antoniossss
Nov 20 '18 at 13:42
This may be true but as I said, apart from the log it doesn't work
– Alex
Nov 20 '18 at 13:45
And that is the different part of story. You can see in the broser what kind of request is actually made and you will see that probably bearer is there.
– Antoniossss
Nov 20 '18 at 14:00
I checked the actual request in the network-tab of different browsers as well as in wireshark and I can say, there is no authorization-header
– Alex
Nov 20 '18 at 14:03
|
show 1 more comment
Thank you for the replay. As you can see in the screenshot, the error (which occurs because of the 401 response from the server) is catched BEFORE the interceptor is executed, too. And also, apart from the log, the authorization-header not gets sent whith the request...
– Alex
Nov 20 '18 at 13:38
No, this is just how browser console works. Order of printout is not guaranteed and might be delayed in case of objects (exception here). I faces similar wtf issue in the past.
– Antoniossss
Nov 20 '18 at 13:42
This may be true but as I said, apart from the log it doesn't work
– Alex
Nov 20 '18 at 13:45
And that is the different part of story. You can see in the broser what kind of request is actually made and you will see that probably bearer is there.
– Antoniossss
Nov 20 '18 at 14:00
I checked the actual request in the network-tab of different browsers as well as in wireshark and I can say, there is no authorization-header
– Alex
Nov 20 '18 at 14:03
Thank you for the replay. As you can see in the screenshot, the error (which occurs because of the 401 response from the server) is catched BEFORE the interceptor is executed, too. And also, apart from the log, the authorization-header not gets sent whith the request...
– Alex
Nov 20 '18 at 13:38
Thank you for the replay. As you can see in the screenshot, the error (which occurs because of the 401 response from the server) is catched BEFORE the interceptor is executed, too. And also, apart from the log, the authorization-header not gets sent whith the request...
– Alex
Nov 20 '18 at 13:38
No, this is just how browser console works. Order of printout is not guaranteed and might be delayed in case of objects (exception here). I faces similar wtf issue in the past.
– Antoniossss
Nov 20 '18 at 13:42
No, this is just how browser console works. Order of printout is not guaranteed and might be delayed in case of objects (exception here). I faces similar wtf issue in the past.
– Antoniossss
Nov 20 '18 at 13:42
This may be true but as I said, apart from the log it doesn't work
– Alex
Nov 20 '18 at 13:45
This may be true but as I said, apart from the log it doesn't work
– Alex
Nov 20 '18 at 13:45
And that is the different part of story. You can see in the broser what kind of request is actually made and you will see that probably bearer is there.
– Antoniossss
Nov 20 '18 at 14:00
And that is the different part of story. You can see in the broser what kind of request is actually made and you will see that probably bearer is there.
– Antoniossss
Nov 20 '18 at 14:00
I checked the actual request in the network-tab of different browsers as well as in wireshark and I can say, there is no authorization-header
– Alex
Nov 20 '18 at 14:03
I checked the actual request in the network-tab of different browsers as well as in wireshark and I can say, there is no authorization-header
– Alex
Nov 20 '18 at 14:03
|
show 1 more comment
Please try like this one
const headers = request.headers.append('Authorization', `Bearer ${sessionId}`);
const d = request.clone({ headers: headers });
return next.handle(request);
Thanks for the reply. I tried this but it doesn't work
– Alex
Nov 20 '18 at 14:41
add a comment |
Please try like this one
const headers = request.headers.append('Authorization', `Bearer ${sessionId}`);
const d = request.clone({ headers: headers });
return next.handle(request);
Thanks for the reply. I tried this but it doesn't work
– Alex
Nov 20 '18 at 14:41
add a comment |
Please try like this one
const headers = request.headers.append('Authorization', `Bearer ${sessionId}`);
const d = request.clone({ headers: headers });
return next.handle(request);
Please try like this one
const headers = request.headers.append('Authorization', `Bearer ${sessionId}`);
const d = request.clone({ headers: headers });
return next.handle(request);
answered Nov 20 '18 at 14:02


Sheik AlthafSheik Althaf
26717
26717
Thanks for the reply. I tried this but it doesn't work
– Alex
Nov 20 '18 at 14:41
add a comment |
Thanks for the reply. I tried this but it doesn't work
– Alex
Nov 20 '18 at 14:41
Thanks for the reply. I tried this but it doesn't work
– Alex
Nov 20 '18 at 14:41
Thanks for the reply. I tried this but it doesn't work
– Alex
Nov 20 '18 at 14:41
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53394140%2fangular-6-interceptor-is-called-after-request-is-sent%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KE,bBOuTt5g QaGc,QiSwNV,DMQB,Mf ZGYTJpAhX,3jO5sdxgLoPUdRm,z4qF8,NdPhJ ND2J,mV
Please check your network tab for the request where the Authorization header is added or not.
– Sheik Althaf
Nov 20 '18 at 13:47
I did and it is not sent (with different browsers). Interesting side note: fiddler doesn't notice this particular request at all. Every request I make is captured by fiddler, but this one request which results in a 401-response not. Only Wireshark captures this request and the response. And in Wireshark there is no authorization-header as well.
– Alex
Nov 20 '18 at 13:55