Undefined variable: user_id in Laravel view
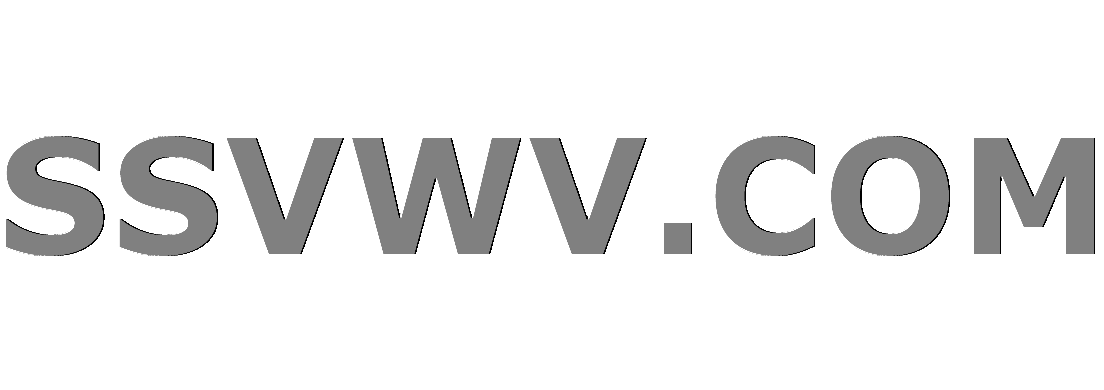
Multi tool use
The Routes
Route::get('/adminContacts/{user_id}', 'AdminContactsController@index')->name('adminContacts.index')->middleware('is_admin');
Route::get('/adminContacts/{user_id}/create', 'AdminContactsController@create')->name('adminContacts.create')->middleware('is_admin');
adminContactController
public function index($user_id)
{
// Confirm User exists
User::findOrFail($user_id);
$filter = request('filter', NULL);
$contacts = NULL;
if ($filter == NULL)
$contacts = Contacts::query()->where('owner_id', $user_id)->sortable()->paginate(5);
else
$contacts = Contacts::query()->where('owner_id', $user_id)->where('name', 'like', '%'.$filter.'%')
->orWhere('number', 'like', '%'.$filter.'%')
->sortable()->paginate(5);
return view('adminContacts.index')->withContacts($contacts)->withUserId($user_id);
}
/**
* Show the form for creating a new resource.
*
* @return IlluminateHttpResponse
*/
public function create($user_id)
{
return view('adminContacts.create')->withUserId($user_id);
}
index.blade.php
<div class="container">
<h1 class="jumbotron">Sample Phone Book</h1>
<a href="{{ route('adminContacts.create', ['user_id' => $user_id] ) }}" class="btn btn-primary btn-block" style="margin-bottom: 5px">Add Contact</a>
</div>
<!--Search Field -->
<div class="container">
<form method="GET" action="{{ route('adminContacts.index') }}">
<input type="text" name='filter' class='input' placeholder='Search' value="{{ request('filter') }}">
@if (request('filter'))
<a class="btn btn-primary btn-sm" href="{{ route('adminContacts.index') }}">X</a>
@endif
</form>
</div>
<!-- Table -->
<div class="container">
<div class="row" >
<table class="table table-hover" id="contactsTable">
<thead>
<tr>
<th>#</th>
<th>@sortablelink('name', 'Contact Name')</th>
<th>@sortablelink('number', 'Phone Number')</th>
<th>
<button class="btn btn-primary btn-sm">Prepend</button>
</th>
</tr>
</thead>
<!--Loop through all the cutomers and output them on the table-->
@foreach($contacts as $contact)
<tbody>
<tr>
<td>{{ $contact->id }}</td>
<td>{{ $contact->name }}</td>
<td>{{ $contact->number }}</td>
<td>
<a href="{{ route('adminContacts.edit', $contact->id) }}" class="btn btn-primary btn-sm">View</a>
</td>
</tr>
</tbody>
@endforeach
</table>
{!! $contacts->appends(Request::except('page'))->render() !!}
</div>
</div>
The error is:
Undefined variable: user_id (View: D:laragonwwwSampleContactsresourcesviewsadminContactsindex.blade.php)
Am I missing something? The idea is to pass the $user_id to the adminContact.create form where i can use it to set
$contact->owner_id = $user_id;
so the entered contact apears under that users table.
php laravel
add a comment |
The Routes
Route::get('/adminContacts/{user_id}', 'AdminContactsController@index')->name('adminContacts.index')->middleware('is_admin');
Route::get('/adminContacts/{user_id}/create', 'AdminContactsController@create')->name('adminContacts.create')->middleware('is_admin');
adminContactController
public function index($user_id)
{
// Confirm User exists
User::findOrFail($user_id);
$filter = request('filter', NULL);
$contacts = NULL;
if ($filter == NULL)
$contacts = Contacts::query()->where('owner_id', $user_id)->sortable()->paginate(5);
else
$contacts = Contacts::query()->where('owner_id', $user_id)->where('name', 'like', '%'.$filter.'%')
->orWhere('number', 'like', '%'.$filter.'%')
->sortable()->paginate(5);
return view('adminContacts.index')->withContacts($contacts)->withUserId($user_id);
}
/**
* Show the form for creating a new resource.
*
* @return IlluminateHttpResponse
*/
public function create($user_id)
{
return view('adminContacts.create')->withUserId($user_id);
}
index.blade.php
<div class="container">
<h1 class="jumbotron">Sample Phone Book</h1>
<a href="{{ route('adminContacts.create', ['user_id' => $user_id] ) }}" class="btn btn-primary btn-block" style="margin-bottom: 5px">Add Contact</a>
</div>
<!--Search Field -->
<div class="container">
<form method="GET" action="{{ route('adminContacts.index') }}">
<input type="text" name='filter' class='input' placeholder='Search' value="{{ request('filter') }}">
@if (request('filter'))
<a class="btn btn-primary btn-sm" href="{{ route('adminContacts.index') }}">X</a>
@endif
</form>
</div>
<!-- Table -->
<div class="container">
<div class="row" >
<table class="table table-hover" id="contactsTable">
<thead>
<tr>
<th>#</th>
<th>@sortablelink('name', 'Contact Name')</th>
<th>@sortablelink('number', 'Phone Number')</th>
<th>
<button class="btn btn-primary btn-sm">Prepend</button>
</th>
</tr>
</thead>
<!--Loop through all the cutomers and output them on the table-->
@foreach($contacts as $contact)
<tbody>
<tr>
<td>{{ $contact->id }}</td>
<td>{{ $contact->name }}</td>
<td>{{ $contact->number }}</td>
<td>
<a href="{{ route('adminContacts.edit', $contact->id) }}" class="btn btn-primary btn-sm">View</a>
</td>
</tr>
</tbody>
@endforeach
</table>
{!! $contacts->appends(Request::except('page'))->render() !!}
</div>
</div>
The error is:
Undefined variable: user_id (View: D:laragonwwwSampleContactsresourcesviewsadminContactsindex.blade.php)
Am I missing something? The idea is to pass the $user_id to the adminContact.create form where i can use it to set
$contact->owner_id = $user_id;
so the entered contact apears under that users table.
php laravel
Possible duplicate of "Notice: Undefined variable", "Notice: Undefined index", and "Notice: Undefined offset" using PHP
– GrumpyCrouton
Nov 21 '18 at 15:01
add a comment |
The Routes
Route::get('/adminContacts/{user_id}', 'AdminContactsController@index')->name('adminContacts.index')->middleware('is_admin');
Route::get('/adminContacts/{user_id}/create', 'AdminContactsController@create')->name('adminContacts.create')->middleware('is_admin');
adminContactController
public function index($user_id)
{
// Confirm User exists
User::findOrFail($user_id);
$filter = request('filter', NULL);
$contacts = NULL;
if ($filter == NULL)
$contacts = Contacts::query()->where('owner_id', $user_id)->sortable()->paginate(5);
else
$contacts = Contacts::query()->where('owner_id', $user_id)->where('name', 'like', '%'.$filter.'%')
->orWhere('number', 'like', '%'.$filter.'%')
->sortable()->paginate(5);
return view('adminContacts.index')->withContacts($contacts)->withUserId($user_id);
}
/**
* Show the form for creating a new resource.
*
* @return IlluminateHttpResponse
*/
public function create($user_id)
{
return view('adminContacts.create')->withUserId($user_id);
}
index.blade.php
<div class="container">
<h1 class="jumbotron">Sample Phone Book</h1>
<a href="{{ route('adminContacts.create', ['user_id' => $user_id] ) }}" class="btn btn-primary btn-block" style="margin-bottom: 5px">Add Contact</a>
</div>
<!--Search Field -->
<div class="container">
<form method="GET" action="{{ route('adminContacts.index') }}">
<input type="text" name='filter' class='input' placeholder='Search' value="{{ request('filter') }}">
@if (request('filter'))
<a class="btn btn-primary btn-sm" href="{{ route('adminContacts.index') }}">X</a>
@endif
</form>
</div>
<!-- Table -->
<div class="container">
<div class="row" >
<table class="table table-hover" id="contactsTable">
<thead>
<tr>
<th>#</th>
<th>@sortablelink('name', 'Contact Name')</th>
<th>@sortablelink('number', 'Phone Number')</th>
<th>
<button class="btn btn-primary btn-sm">Prepend</button>
</th>
</tr>
</thead>
<!--Loop through all the cutomers and output them on the table-->
@foreach($contacts as $contact)
<tbody>
<tr>
<td>{{ $contact->id }}</td>
<td>{{ $contact->name }}</td>
<td>{{ $contact->number }}</td>
<td>
<a href="{{ route('adminContacts.edit', $contact->id) }}" class="btn btn-primary btn-sm">View</a>
</td>
</tr>
</tbody>
@endforeach
</table>
{!! $contacts->appends(Request::except('page'))->render() !!}
</div>
</div>
The error is:
Undefined variable: user_id (View: D:laragonwwwSampleContactsresourcesviewsadminContactsindex.blade.php)
Am I missing something? The idea is to pass the $user_id to the adminContact.create form where i can use it to set
$contact->owner_id = $user_id;
so the entered contact apears under that users table.
php laravel
The Routes
Route::get('/adminContacts/{user_id}', 'AdminContactsController@index')->name('adminContacts.index')->middleware('is_admin');
Route::get('/adminContacts/{user_id}/create', 'AdminContactsController@create')->name('adminContacts.create')->middleware('is_admin');
adminContactController
public function index($user_id)
{
// Confirm User exists
User::findOrFail($user_id);
$filter = request('filter', NULL);
$contacts = NULL;
if ($filter == NULL)
$contacts = Contacts::query()->where('owner_id', $user_id)->sortable()->paginate(5);
else
$contacts = Contacts::query()->where('owner_id', $user_id)->where('name', 'like', '%'.$filter.'%')
->orWhere('number', 'like', '%'.$filter.'%')
->sortable()->paginate(5);
return view('adminContacts.index')->withContacts($contacts)->withUserId($user_id);
}
/**
* Show the form for creating a new resource.
*
* @return IlluminateHttpResponse
*/
public function create($user_id)
{
return view('adminContacts.create')->withUserId($user_id);
}
index.blade.php
<div class="container">
<h1 class="jumbotron">Sample Phone Book</h1>
<a href="{{ route('adminContacts.create', ['user_id' => $user_id] ) }}" class="btn btn-primary btn-block" style="margin-bottom: 5px">Add Contact</a>
</div>
<!--Search Field -->
<div class="container">
<form method="GET" action="{{ route('adminContacts.index') }}">
<input type="text" name='filter' class='input' placeholder='Search' value="{{ request('filter') }}">
@if (request('filter'))
<a class="btn btn-primary btn-sm" href="{{ route('adminContacts.index') }}">X</a>
@endif
</form>
</div>
<!-- Table -->
<div class="container">
<div class="row" >
<table class="table table-hover" id="contactsTable">
<thead>
<tr>
<th>#</th>
<th>@sortablelink('name', 'Contact Name')</th>
<th>@sortablelink('number', 'Phone Number')</th>
<th>
<button class="btn btn-primary btn-sm">Prepend</button>
</th>
</tr>
</thead>
<!--Loop through all the cutomers and output them on the table-->
@foreach($contacts as $contact)
<tbody>
<tr>
<td>{{ $contact->id }}</td>
<td>{{ $contact->name }}</td>
<td>{{ $contact->number }}</td>
<td>
<a href="{{ route('adminContacts.edit', $contact->id) }}" class="btn btn-primary btn-sm">View</a>
</td>
</tr>
</tbody>
@endforeach
</table>
{!! $contacts->appends(Request::except('page'))->render() !!}
</div>
</div>
The error is:
Undefined variable: user_id (View: D:laragonwwwSampleContactsresourcesviewsadminContactsindex.blade.php)
Am I missing something? The idea is to pass the $user_id to the adminContact.create form where i can use it to set
$contact->owner_id = $user_id;
so the entered contact apears under that users table.
php laravel
php laravel
asked Nov 21 '18 at 14:57


Commander SpudsCommander Spuds
12
12
Possible duplicate of "Notice: Undefined variable", "Notice: Undefined index", and "Notice: Undefined offset" using PHP
– GrumpyCrouton
Nov 21 '18 at 15:01
add a comment |
Possible duplicate of "Notice: Undefined variable", "Notice: Undefined index", and "Notice: Undefined offset" using PHP
– GrumpyCrouton
Nov 21 '18 at 15:01
Possible duplicate of "Notice: Undefined variable", "Notice: Undefined index", and "Notice: Undefined offset" using PHP
– GrumpyCrouton
Nov 21 '18 at 15:01
Possible duplicate of "Notice: Undefined variable", "Notice: Undefined index", and "Notice: Undefined offset" using PHP
– GrumpyCrouton
Nov 21 '18 at 15:01
add a comment |
3 Answers
3
active
oldest
votes
I don't think you can pass variables into your view like you're doing now.
In your controller, try changing this line:
return view('adminContacts.index')->withContacts($contacts)->withUserId($user_id);
To this:
return view('adminContacts.index')->with('contacts', $contacts)->with('user_id', $user_id);
If I do change it to the above I get a different error:Missing required parameters for [Route: adminContacts.index] [URI: adminContacts/{user_id}]. (View: D:laragonwwwSampleContactsresourcesviewsadminContactsindex.blade.php)
– Commander Spuds
Nov 22 '18 at 9:02
add a comment |
You could explicitly name the variable when passing it to the view, either this way
return view('adminContacts.index')->with('user_id', $user_id);
Or
return view('adminContract.index', compact('user_id'));
I'm not sure what the name of the variable becomes when passed to the view via withUserId
, but I'm guessing this is the issue.
add a comment |
Found the problem.
@extends('layouts.app')
@section('content')
<div class="container">
<h1 class="jumbotron">Sample Phone Book</h1>
<a href="{{ route('adminContacts.create', ['user_id' => $user_id] ) }}" class="btn btn-primary btn-block" style="margin-bottom: 5px">Add Contact</a>
</div>
<!--Search Field -->
<div class="container">
<form method="GET" action="{{ route('adminContacts.index', $user_id) }}">
<input type="text" name='filter' class='input' placeholder='Search' value="{{ request('filter') }}">
@if (request('filter'))
<a class="btn btn-primary btn-sm" href="{{ route('adminContacts.index', $user_id) }}">X</a>
@endif
</form>
</div>
<!-- Table -->
<div class="container">
<div class="row" >
<table class="table table-hover" id="contactsTable">
<thead>
<tr>
<th>#</th>
<th>@sortablelink('name', 'Contact Name')</th>
<th>@sortablelink('number', 'Phone Number')</th>
<th>
<button class="btn btn-primary btn-sm">Prepend</button>
</th>
</tr>
</thead>
<!--Loop through all the cutomers and output them on the table-->
@foreach($contacts as $contact)
<tbody>
<tr>
<td>{{ $contact->id }}</td>
<td>{{ $contact->name }}</td>
<td>{{ $contact->number }}</td>
<td>
<a href="{{ route('adminContacts.edit', $contact->id) }}" class="btn btn-primary btn-sm">View</a>
</td>
</tr>
</tbody>
@endforeach
</table>
{!! $contacts->appends(Request::except('page'))->render() !!}
</div>
</div>
@endsection
Anywhere where there is a {{ route('adminContacts.index') }}
it also needed the $user_id
to be passed along with it because I'm also using it in the routes as '/adminContacts/{user_id}'
Thank you for all the help.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414775%2fundefined-variable-user-id-in-laravel-view%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
I don't think you can pass variables into your view like you're doing now.
In your controller, try changing this line:
return view('adminContacts.index')->withContacts($contacts)->withUserId($user_id);
To this:
return view('adminContacts.index')->with('contacts', $contacts)->with('user_id', $user_id);
If I do change it to the above I get a different error:Missing required parameters for [Route: adminContacts.index] [URI: adminContacts/{user_id}]. (View: D:laragonwwwSampleContactsresourcesviewsadminContactsindex.blade.php)
– Commander Spuds
Nov 22 '18 at 9:02
add a comment |
I don't think you can pass variables into your view like you're doing now.
In your controller, try changing this line:
return view('adminContacts.index')->withContacts($contacts)->withUserId($user_id);
To this:
return view('adminContacts.index')->with('contacts', $contacts)->with('user_id', $user_id);
If I do change it to the above I get a different error:Missing required parameters for [Route: adminContacts.index] [URI: adminContacts/{user_id}]. (View: D:laragonwwwSampleContactsresourcesviewsadminContactsindex.blade.php)
– Commander Spuds
Nov 22 '18 at 9:02
add a comment |
I don't think you can pass variables into your view like you're doing now.
In your controller, try changing this line:
return view('adminContacts.index')->withContacts($contacts)->withUserId($user_id);
To this:
return view('adminContacts.index')->with('contacts', $contacts)->with('user_id', $user_id);
I don't think you can pass variables into your view like you're doing now.
In your controller, try changing this line:
return view('adminContacts.index')->withContacts($contacts)->withUserId($user_id);
To this:
return view('adminContacts.index')->with('contacts', $contacts)->with('user_id', $user_id);
answered Nov 21 '18 at 15:10


rpm192rpm192
1,3771621
1,3771621
If I do change it to the above I get a different error:Missing required parameters for [Route: adminContacts.index] [URI: adminContacts/{user_id}]. (View: D:laragonwwwSampleContactsresourcesviewsadminContactsindex.blade.php)
– Commander Spuds
Nov 22 '18 at 9:02
add a comment |
If I do change it to the above I get a different error:Missing required parameters for [Route: adminContacts.index] [URI: adminContacts/{user_id}]. (View: D:laragonwwwSampleContactsresourcesviewsadminContactsindex.blade.php)
– Commander Spuds
Nov 22 '18 at 9:02
If I do change it to the above I get a different error:
Missing required parameters for [Route: adminContacts.index] [URI: adminContacts/{user_id}]. (View: D:laragonwwwSampleContactsresourcesviewsadminContactsindex.blade.php)
– Commander Spuds
Nov 22 '18 at 9:02
If I do change it to the above I get a different error:
Missing required parameters for [Route: adminContacts.index] [URI: adminContacts/{user_id}]. (View: D:laragonwwwSampleContactsresourcesviewsadminContactsindex.blade.php)
– Commander Spuds
Nov 22 '18 at 9:02
add a comment |
You could explicitly name the variable when passing it to the view, either this way
return view('adminContacts.index')->with('user_id', $user_id);
Or
return view('adminContract.index', compact('user_id'));
I'm not sure what the name of the variable becomes when passed to the view via withUserId
, but I'm guessing this is the issue.
add a comment |
You could explicitly name the variable when passing it to the view, either this way
return view('adminContacts.index')->with('user_id', $user_id);
Or
return view('adminContract.index', compact('user_id'));
I'm not sure what the name of the variable becomes when passed to the view via withUserId
, but I'm guessing this is the issue.
add a comment |
You could explicitly name the variable when passing it to the view, either this way
return view('adminContacts.index')->with('user_id', $user_id);
Or
return view('adminContract.index', compact('user_id'));
I'm not sure what the name of the variable becomes when passed to the view via withUserId
, but I'm guessing this is the issue.
You could explicitly name the variable when passing it to the view, either this way
return view('adminContacts.index')->with('user_id', $user_id);
Or
return view('adminContract.index', compact('user_id'));
I'm not sure what the name of the variable becomes when passed to the view via withUserId
, but I'm guessing this is the issue.
answered Nov 21 '18 at 15:11
Nick DawesNick Dawes
919
919
add a comment |
add a comment |
Found the problem.
@extends('layouts.app')
@section('content')
<div class="container">
<h1 class="jumbotron">Sample Phone Book</h1>
<a href="{{ route('adminContacts.create', ['user_id' => $user_id] ) }}" class="btn btn-primary btn-block" style="margin-bottom: 5px">Add Contact</a>
</div>
<!--Search Field -->
<div class="container">
<form method="GET" action="{{ route('adminContacts.index', $user_id) }}">
<input type="text" name='filter' class='input' placeholder='Search' value="{{ request('filter') }}">
@if (request('filter'))
<a class="btn btn-primary btn-sm" href="{{ route('adminContacts.index', $user_id) }}">X</a>
@endif
</form>
</div>
<!-- Table -->
<div class="container">
<div class="row" >
<table class="table table-hover" id="contactsTable">
<thead>
<tr>
<th>#</th>
<th>@sortablelink('name', 'Contact Name')</th>
<th>@sortablelink('number', 'Phone Number')</th>
<th>
<button class="btn btn-primary btn-sm">Prepend</button>
</th>
</tr>
</thead>
<!--Loop through all the cutomers and output them on the table-->
@foreach($contacts as $contact)
<tbody>
<tr>
<td>{{ $contact->id }}</td>
<td>{{ $contact->name }}</td>
<td>{{ $contact->number }}</td>
<td>
<a href="{{ route('adminContacts.edit', $contact->id) }}" class="btn btn-primary btn-sm">View</a>
</td>
</tr>
</tbody>
@endforeach
</table>
{!! $contacts->appends(Request::except('page'))->render() !!}
</div>
</div>
@endsection
Anywhere where there is a {{ route('adminContacts.index') }}
it also needed the $user_id
to be passed along with it because I'm also using it in the routes as '/adminContacts/{user_id}'
Thank you for all the help.
add a comment |
Found the problem.
@extends('layouts.app')
@section('content')
<div class="container">
<h1 class="jumbotron">Sample Phone Book</h1>
<a href="{{ route('adminContacts.create', ['user_id' => $user_id] ) }}" class="btn btn-primary btn-block" style="margin-bottom: 5px">Add Contact</a>
</div>
<!--Search Field -->
<div class="container">
<form method="GET" action="{{ route('adminContacts.index', $user_id) }}">
<input type="text" name='filter' class='input' placeholder='Search' value="{{ request('filter') }}">
@if (request('filter'))
<a class="btn btn-primary btn-sm" href="{{ route('adminContacts.index', $user_id) }}">X</a>
@endif
</form>
</div>
<!-- Table -->
<div class="container">
<div class="row" >
<table class="table table-hover" id="contactsTable">
<thead>
<tr>
<th>#</th>
<th>@sortablelink('name', 'Contact Name')</th>
<th>@sortablelink('number', 'Phone Number')</th>
<th>
<button class="btn btn-primary btn-sm">Prepend</button>
</th>
</tr>
</thead>
<!--Loop through all the cutomers and output them on the table-->
@foreach($contacts as $contact)
<tbody>
<tr>
<td>{{ $contact->id }}</td>
<td>{{ $contact->name }}</td>
<td>{{ $contact->number }}</td>
<td>
<a href="{{ route('adminContacts.edit', $contact->id) }}" class="btn btn-primary btn-sm">View</a>
</td>
</tr>
</tbody>
@endforeach
</table>
{!! $contacts->appends(Request::except('page'))->render() !!}
</div>
</div>
@endsection
Anywhere where there is a {{ route('adminContacts.index') }}
it also needed the $user_id
to be passed along with it because I'm also using it in the routes as '/adminContacts/{user_id}'
Thank you for all the help.
add a comment |
Found the problem.
@extends('layouts.app')
@section('content')
<div class="container">
<h1 class="jumbotron">Sample Phone Book</h1>
<a href="{{ route('adminContacts.create', ['user_id' => $user_id] ) }}" class="btn btn-primary btn-block" style="margin-bottom: 5px">Add Contact</a>
</div>
<!--Search Field -->
<div class="container">
<form method="GET" action="{{ route('adminContacts.index', $user_id) }}">
<input type="text" name='filter' class='input' placeholder='Search' value="{{ request('filter') }}">
@if (request('filter'))
<a class="btn btn-primary btn-sm" href="{{ route('adminContacts.index', $user_id) }}">X</a>
@endif
</form>
</div>
<!-- Table -->
<div class="container">
<div class="row" >
<table class="table table-hover" id="contactsTable">
<thead>
<tr>
<th>#</th>
<th>@sortablelink('name', 'Contact Name')</th>
<th>@sortablelink('number', 'Phone Number')</th>
<th>
<button class="btn btn-primary btn-sm">Prepend</button>
</th>
</tr>
</thead>
<!--Loop through all the cutomers and output them on the table-->
@foreach($contacts as $contact)
<tbody>
<tr>
<td>{{ $contact->id }}</td>
<td>{{ $contact->name }}</td>
<td>{{ $contact->number }}</td>
<td>
<a href="{{ route('adminContacts.edit', $contact->id) }}" class="btn btn-primary btn-sm">View</a>
</td>
</tr>
</tbody>
@endforeach
</table>
{!! $contacts->appends(Request::except('page'))->render() !!}
</div>
</div>
@endsection
Anywhere where there is a {{ route('adminContacts.index') }}
it also needed the $user_id
to be passed along with it because I'm also using it in the routes as '/adminContacts/{user_id}'
Thank you for all the help.
Found the problem.
@extends('layouts.app')
@section('content')
<div class="container">
<h1 class="jumbotron">Sample Phone Book</h1>
<a href="{{ route('adminContacts.create', ['user_id' => $user_id] ) }}" class="btn btn-primary btn-block" style="margin-bottom: 5px">Add Contact</a>
</div>
<!--Search Field -->
<div class="container">
<form method="GET" action="{{ route('adminContacts.index', $user_id) }}">
<input type="text" name='filter' class='input' placeholder='Search' value="{{ request('filter') }}">
@if (request('filter'))
<a class="btn btn-primary btn-sm" href="{{ route('adminContacts.index', $user_id) }}">X</a>
@endif
</form>
</div>
<!-- Table -->
<div class="container">
<div class="row" >
<table class="table table-hover" id="contactsTable">
<thead>
<tr>
<th>#</th>
<th>@sortablelink('name', 'Contact Name')</th>
<th>@sortablelink('number', 'Phone Number')</th>
<th>
<button class="btn btn-primary btn-sm">Prepend</button>
</th>
</tr>
</thead>
<!--Loop through all the cutomers and output them on the table-->
@foreach($contacts as $contact)
<tbody>
<tr>
<td>{{ $contact->id }}</td>
<td>{{ $contact->name }}</td>
<td>{{ $contact->number }}</td>
<td>
<a href="{{ route('adminContacts.edit', $contact->id) }}" class="btn btn-primary btn-sm">View</a>
</td>
</tr>
</tbody>
@endforeach
</table>
{!! $contacts->appends(Request::except('page'))->render() !!}
</div>
</div>
@endsection
Anywhere where there is a {{ route('adminContacts.index') }}
it also needed the $user_id
to be passed along with it because I'm also using it in the routes as '/adminContacts/{user_id}'
Thank you for all the help.
answered Nov 22 '18 at 14:16


Commander SpudsCommander Spuds
12
12
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414775%2fundefined-variable-user-id-in-laravel-view%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zP6ySniUkqW3RV,5pniX0 eIKDZ fKfhNy8lG VrgLJ
Possible duplicate of "Notice: Undefined variable", "Notice: Undefined index", and "Notice: Undefined offset" using PHP
– GrumpyCrouton
Nov 21 '18 at 15:01