Add Routes and Use Session Information in Service Provider
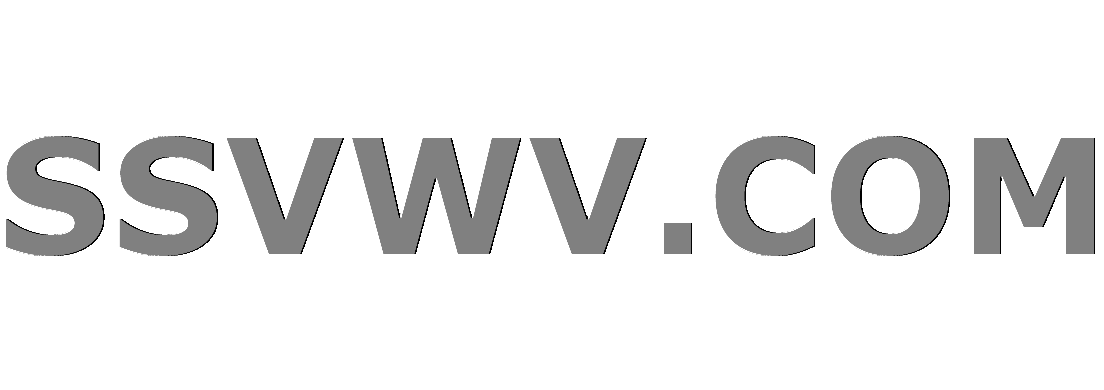
Multi tool use
up vote
3
down vote
favorite
tl;dr; How can I get session data, then based on authentication, manipulate routes in a laravel ServiceProvider?
The Problem
I need to do two things in a service provider. Register routes and use session data at the same time potentially.
Existing Solutions
Route Without Session Data
I know ServiceProvider::register
may happen before RouteProvider::register
gets called. ServiceProvider::boot
can add routes using something like this...
public function boot(Router $router)
{
$router->prefix('api')->group(function ($router) {
$router->resource('lists', 'AppExtensionsExtensionNameHttpControllersMyController');
});
}
Now this will add the route properly. But by the time that route is accessed, there will be no session data. Therefore Auth::user()
will return null
. And of course Auth::user()
is going to return null in the boot()
method as well during the adding of routes.
Session Data Without Route
I could extend the IlluminateSessionMiddlewareStartSession
with my own middleware and fire an event like session.started
at the end of an overloaded startSession()
method. With this, I should have the session data needed to do something like Auth::user()
.
public function boot(Router $router)
{
Event::listen('session.started', function () use ($router) {
if (Auth::user()->can('do.something')) {
$router->middleware(['auth'])->prefix('api')->group(function ($router) {
$router->resource('lists', 'AppExtensionsExtensionNameHttpControllersMyController');
});
}
});
}
However, the route will not be added at this point as that stage has already been long over with.
Proper Solution Hints
I've been leaning towards registering my routes, then in my controller, I would somehow inject the session middleware so that it starts the session before my controller code actually runs. But I'm unsure how to do something like that.
It would be very nice if I could have access to the session data before even supplying my routes as it would be cleaner, faster and more secure to include routes that users have access to in the first place instead of checking on every call to them or removing them after they've been added and authorization has been checked.
References
- How to retrieve session data in service providers in laravel?
- https://laracasts.com/discuss/channels/general-discussion/laravel-5-session-data-is-not-accessible-in-the-app-boot-process
- https://github.com/laravel/framework/issues/7906
- https://github.com/laravel/framework/pull/7933
- https://laravel.io/forum/12-17-2014-session-content-not-available-in-service-providers-register
- https://josephsilber.com/posts/2017/01/23/getting-current-user-in-laravel-controller-constructor
php laravel
add a comment |
up vote
3
down vote
favorite
tl;dr; How can I get session data, then based on authentication, manipulate routes in a laravel ServiceProvider?
The Problem
I need to do two things in a service provider. Register routes and use session data at the same time potentially.
Existing Solutions
Route Without Session Data
I know ServiceProvider::register
may happen before RouteProvider::register
gets called. ServiceProvider::boot
can add routes using something like this...
public function boot(Router $router)
{
$router->prefix('api')->group(function ($router) {
$router->resource('lists', 'AppExtensionsExtensionNameHttpControllersMyController');
});
}
Now this will add the route properly. But by the time that route is accessed, there will be no session data. Therefore Auth::user()
will return null
. And of course Auth::user()
is going to return null in the boot()
method as well during the adding of routes.
Session Data Without Route
I could extend the IlluminateSessionMiddlewareStartSession
with my own middleware and fire an event like session.started
at the end of an overloaded startSession()
method. With this, I should have the session data needed to do something like Auth::user()
.
public function boot(Router $router)
{
Event::listen('session.started', function () use ($router) {
if (Auth::user()->can('do.something')) {
$router->middleware(['auth'])->prefix('api')->group(function ($router) {
$router->resource('lists', 'AppExtensionsExtensionNameHttpControllersMyController');
});
}
});
}
However, the route will not be added at this point as that stage has already been long over with.
Proper Solution Hints
I've been leaning towards registering my routes, then in my controller, I would somehow inject the session middleware so that it starts the session before my controller code actually runs. But I'm unsure how to do something like that.
It would be very nice if I could have access to the session data before even supplying my routes as it would be cleaner, faster and more secure to include routes that users have access to in the first place instead of checking on every call to them or removing them after they've been added and authorization has been checked.
References
- How to retrieve session data in service providers in laravel?
- https://laracasts.com/discuss/channels/general-discussion/laravel-5-session-data-is-not-accessible-in-the-app-boot-process
- https://github.com/laravel/framework/issues/7906
- https://github.com/laravel/framework/pull/7933
- https://laravel.io/forum/12-17-2014-session-content-not-available-in-service-providers-register
- https://josephsilber.com/posts/2017/01/23/getting-current-user-in-laravel-controller-constructor
php laravel
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
tl;dr; How can I get session data, then based on authentication, manipulate routes in a laravel ServiceProvider?
The Problem
I need to do two things in a service provider. Register routes and use session data at the same time potentially.
Existing Solutions
Route Without Session Data
I know ServiceProvider::register
may happen before RouteProvider::register
gets called. ServiceProvider::boot
can add routes using something like this...
public function boot(Router $router)
{
$router->prefix('api')->group(function ($router) {
$router->resource('lists', 'AppExtensionsExtensionNameHttpControllersMyController');
});
}
Now this will add the route properly. But by the time that route is accessed, there will be no session data. Therefore Auth::user()
will return null
. And of course Auth::user()
is going to return null in the boot()
method as well during the adding of routes.
Session Data Without Route
I could extend the IlluminateSessionMiddlewareStartSession
with my own middleware and fire an event like session.started
at the end of an overloaded startSession()
method. With this, I should have the session data needed to do something like Auth::user()
.
public function boot(Router $router)
{
Event::listen('session.started', function () use ($router) {
if (Auth::user()->can('do.something')) {
$router->middleware(['auth'])->prefix('api')->group(function ($router) {
$router->resource('lists', 'AppExtensionsExtensionNameHttpControllersMyController');
});
}
});
}
However, the route will not be added at this point as that stage has already been long over with.
Proper Solution Hints
I've been leaning towards registering my routes, then in my controller, I would somehow inject the session middleware so that it starts the session before my controller code actually runs. But I'm unsure how to do something like that.
It would be very nice if I could have access to the session data before even supplying my routes as it would be cleaner, faster and more secure to include routes that users have access to in the first place instead of checking on every call to them or removing them after they've been added and authorization has been checked.
References
- How to retrieve session data in service providers in laravel?
- https://laracasts.com/discuss/channels/general-discussion/laravel-5-session-data-is-not-accessible-in-the-app-boot-process
- https://github.com/laravel/framework/issues/7906
- https://github.com/laravel/framework/pull/7933
- https://laravel.io/forum/12-17-2014-session-content-not-available-in-service-providers-register
- https://josephsilber.com/posts/2017/01/23/getting-current-user-in-laravel-controller-constructor
php laravel
tl;dr; How can I get session data, then based on authentication, manipulate routes in a laravel ServiceProvider?
The Problem
I need to do two things in a service provider. Register routes and use session data at the same time potentially.
Existing Solutions
Route Without Session Data
I know ServiceProvider::register
may happen before RouteProvider::register
gets called. ServiceProvider::boot
can add routes using something like this...
public function boot(Router $router)
{
$router->prefix('api')->group(function ($router) {
$router->resource('lists', 'AppExtensionsExtensionNameHttpControllersMyController');
});
}
Now this will add the route properly. But by the time that route is accessed, there will be no session data. Therefore Auth::user()
will return null
. And of course Auth::user()
is going to return null in the boot()
method as well during the adding of routes.
Session Data Without Route
I could extend the IlluminateSessionMiddlewareStartSession
with my own middleware and fire an event like session.started
at the end of an overloaded startSession()
method. With this, I should have the session data needed to do something like Auth::user()
.
public function boot(Router $router)
{
Event::listen('session.started', function () use ($router) {
if (Auth::user()->can('do.something')) {
$router->middleware(['auth'])->prefix('api')->group(function ($router) {
$router->resource('lists', 'AppExtensionsExtensionNameHttpControllersMyController');
});
}
});
}
However, the route will not be added at this point as that stage has already been long over with.
Proper Solution Hints
I've been leaning towards registering my routes, then in my controller, I would somehow inject the session middleware so that it starts the session before my controller code actually runs. But I'm unsure how to do something like that.
It would be very nice if I could have access to the session data before even supplying my routes as it would be cleaner, faster and more secure to include routes that users have access to in the first place instead of checking on every call to them or removing them after they've been added and authorization has been checked.
References
- How to retrieve session data in service providers in laravel?
- https://laracasts.com/discuss/channels/general-discussion/laravel-5-session-data-is-not-accessible-in-the-app-boot-process
- https://github.com/laravel/framework/issues/7906
- https://github.com/laravel/framework/pull/7933
- https://laravel.io/forum/12-17-2014-session-content-not-available-in-service-providers-register
- https://josephsilber.com/posts/2017/01/23/getting-current-user-in-laravel-controller-constructor
php laravel
php laravel
asked Nov 16 at 20:23
Xedecimal
2,60011421
2,60011421
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53344864%2fadd-routes-and-use-session-information-in-service-provider%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
T0bjxIHXcGi D9BmEhWgintMTxcPBUlZ,S 3ZG5 ABRKXZIqJQjvf7VE9YcI CM 7uSv y CZclLs2n7pI4e5Am60Zfo0 gH