Not receiving Products from function in Angular
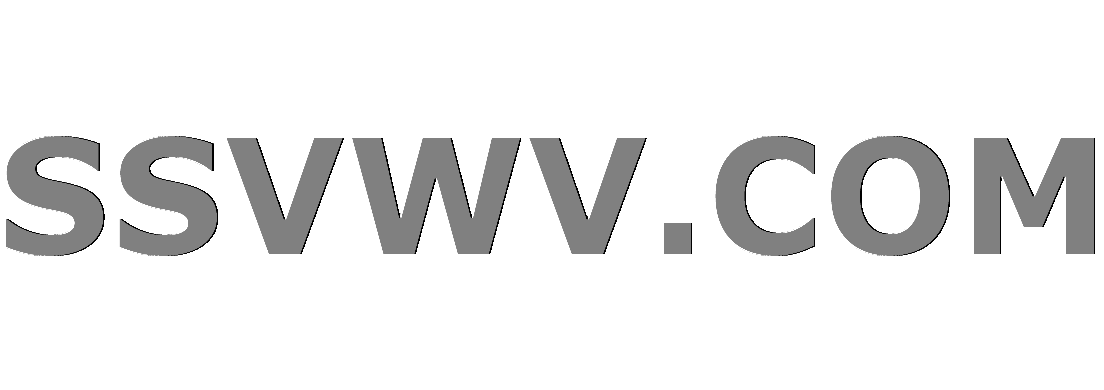
Multi tool use
up vote
1
down vote
favorite
I am working through the tutorial files in the book Pro Angular for a Store Front application. I am currently working on an administration section and am getting an error saying "Failed to load resources, cannot connect to the server". This is my rest.datasource.ts file:
import { Injectable } from "@angular/core";
import { Http, Request, RequestMethod } from "@angular/http";
import { Observable } from "rxjs/Observable";
import { Product } from "./product.model";
import { Cart } from "./cart.model";
import { Order } from "./order.model";
import "rxjs/add/operator/map";
const PROTOCOL = "http";
const PORT = 3000;
@Injectable()
export class RestDataSource {
baseUrl: string;
auth_token: string;
constructor(private http: Http) {
this.baseUrl = `${PROTOCOL}://${location.hostname}:${PORT}/`;
}
authenticate(user: string, pass: string): Observable<boolean> {
return this.http.request(new Request({
method: RequestMethod.Post,
url: this.baseUrl + "login",
body: { name: user, password: pass }
})).map(response => {
let r = response.json();
this.auth_token = r.success ? r.token : null;
return r.success;
});
}
getProducts(): Observable<Product> {
return this.sendRequest(RequestMethod.Get, "products");
}
saveProduct(product: Product): Observable<Product> {
return this.sendRequest(RequestMethod.Post, "products",
product, true);
}
updateProduct(product): Observable<Product> {
return this.sendRequest(RequestMethod.Put,
`products/${product.id}`, product, true);
}
deleteProduct(id: number): Observable<Product> {
return this.sendRequest(RequestMethod.Delete,
`products/${id}`, null, true);
}
getOrders(): Observable<Order> {
return this.sendRequest(RequestMethod.Get,
"orders", null, true);
}
deleteOrder(id: number): Observable<Order> {
return this.sendRequest(RequestMethod.Delete,
`orders/${id}`, null, true);
}
updateOrder(order: Order): Observable<Order> {
return this.sendRequest(RequestMethod.Put,
`orders/${order.id}`, order, true);
}
saveOrder(order: Order): Observable<Order> {
return this.sendRequest(RequestMethod.Post,
"orders", order);
}
private sendRequest(verb: RequestMethod,
url: string, body?: Product | Order, auth: boolean = false)
: Observable<Product | Product | Order | Order> {
let request = new Request({
method: verb,
url: this.baseUrl + url,
body: body
});
if (auth && this.auth_token != null) {
request.headers.set("Authorization", `Bearer<${this.auth_token}>`);
}
return this.http.request(request).map(response => response.json());
}
}
and here is my static.datasource.ts file:
import { Injectable } from "@angular/core";
import { Product } from "./product.model";
import { Observable } from "rxjs/Observable";
import "rxjs/add/observable/from";
import { Order } from "./order.model";
@Injectable()
export class StaticDataSource {
//Populate product fields with Inventory information
private products: Product = [
//products omitted for brevity
];
getProducts(): Observable<Product> {
return Observable.from([this.products]);
}
saveOrder(order: Order): Observable<Order> {
console.log(JSON.stringify(order));
return Observable.from([order]);
}
}
I am following the tutorial exactly but for some reason the site falls apart now that I am on Chapter 9 creating the admin section. Any help or pointers would be greatly appreciated.
javascript angular
add a comment |
up vote
1
down vote
favorite
I am working through the tutorial files in the book Pro Angular for a Store Front application. I am currently working on an administration section and am getting an error saying "Failed to load resources, cannot connect to the server". This is my rest.datasource.ts file:
import { Injectable } from "@angular/core";
import { Http, Request, RequestMethod } from "@angular/http";
import { Observable } from "rxjs/Observable";
import { Product } from "./product.model";
import { Cart } from "./cart.model";
import { Order } from "./order.model";
import "rxjs/add/operator/map";
const PROTOCOL = "http";
const PORT = 3000;
@Injectable()
export class RestDataSource {
baseUrl: string;
auth_token: string;
constructor(private http: Http) {
this.baseUrl = `${PROTOCOL}://${location.hostname}:${PORT}/`;
}
authenticate(user: string, pass: string): Observable<boolean> {
return this.http.request(new Request({
method: RequestMethod.Post,
url: this.baseUrl + "login",
body: { name: user, password: pass }
})).map(response => {
let r = response.json();
this.auth_token = r.success ? r.token : null;
return r.success;
});
}
getProducts(): Observable<Product> {
return this.sendRequest(RequestMethod.Get, "products");
}
saveProduct(product: Product): Observable<Product> {
return this.sendRequest(RequestMethod.Post, "products",
product, true);
}
updateProduct(product): Observable<Product> {
return this.sendRequest(RequestMethod.Put,
`products/${product.id}`, product, true);
}
deleteProduct(id: number): Observable<Product> {
return this.sendRequest(RequestMethod.Delete,
`products/${id}`, null, true);
}
getOrders(): Observable<Order> {
return this.sendRequest(RequestMethod.Get,
"orders", null, true);
}
deleteOrder(id: number): Observable<Order> {
return this.sendRequest(RequestMethod.Delete,
`orders/${id}`, null, true);
}
updateOrder(order: Order): Observable<Order> {
return this.sendRequest(RequestMethod.Put,
`orders/${order.id}`, order, true);
}
saveOrder(order: Order): Observable<Order> {
return this.sendRequest(RequestMethod.Post,
"orders", order);
}
private sendRequest(verb: RequestMethod,
url: string, body?: Product | Order, auth: boolean = false)
: Observable<Product | Product | Order | Order> {
let request = new Request({
method: verb,
url: this.baseUrl + url,
body: body
});
if (auth && this.auth_token != null) {
request.headers.set("Authorization", `Bearer<${this.auth_token}>`);
}
return this.http.request(request).map(response => response.json());
}
}
and here is my static.datasource.ts file:
import { Injectable } from "@angular/core";
import { Product } from "./product.model";
import { Observable } from "rxjs/Observable";
import "rxjs/add/observable/from";
import { Order } from "./order.model";
@Injectable()
export class StaticDataSource {
//Populate product fields with Inventory information
private products: Product = [
//products omitted for brevity
];
getProducts(): Observable<Product> {
return Observable.from([this.products]);
}
saveOrder(order: Order): Observable<Order> {
console.log(JSON.stringify(order));
return Observable.from([order]);
}
}
I am following the tutorial exactly but for some reason the site falls apart now that I am on Chapter 9 creating the admin section. Any help or pointers would be greatly appreciated.
javascript angular
1
Was my mistake. Fixed. Thanks!
– still2blue
Nov 18 at 19:35
1
Note that@angular/http
is deprecated in favour of@angular/common/http
; you should probably find a more up-to-date resource.
– jonrsharpe
Nov 18 at 19:43
Did you figure this out?
– nkuma_12
Nov 18 at 20:32
Hmmthis.baseUrl = `${PROTOCOL}://${location.hostname}:${PORT}/`;
did you definelocation
anywhere?
– pandamakes
Nov 18 at 21:12
Also, I would check the version of rxjs you are using. Iirc, rxjs 6 changed how operators work: github.com/ReactiveX/rxjs/blob/master/docs_app/content/guide/v6/…
– pandamakes
Nov 18 at 21:16
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am working through the tutorial files in the book Pro Angular for a Store Front application. I am currently working on an administration section and am getting an error saying "Failed to load resources, cannot connect to the server". This is my rest.datasource.ts file:
import { Injectable } from "@angular/core";
import { Http, Request, RequestMethod } from "@angular/http";
import { Observable } from "rxjs/Observable";
import { Product } from "./product.model";
import { Cart } from "./cart.model";
import { Order } from "./order.model";
import "rxjs/add/operator/map";
const PROTOCOL = "http";
const PORT = 3000;
@Injectable()
export class RestDataSource {
baseUrl: string;
auth_token: string;
constructor(private http: Http) {
this.baseUrl = `${PROTOCOL}://${location.hostname}:${PORT}/`;
}
authenticate(user: string, pass: string): Observable<boolean> {
return this.http.request(new Request({
method: RequestMethod.Post,
url: this.baseUrl + "login",
body: { name: user, password: pass }
})).map(response => {
let r = response.json();
this.auth_token = r.success ? r.token : null;
return r.success;
});
}
getProducts(): Observable<Product> {
return this.sendRequest(RequestMethod.Get, "products");
}
saveProduct(product: Product): Observable<Product> {
return this.sendRequest(RequestMethod.Post, "products",
product, true);
}
updateProduct(product): Observable<Product> {
return this.sendRequest(RequestMethod.Put,
`products/${product.id}`, product, true);
}
deleteProduct(id: number): Observable<Product> {
return this.sendRequest(RequestMethod.Delete,
`products/${id}`, null, true);
}
getOrders(): Observable<Order> {
return this.sendRequest(RequestMethod.Get,
"orders", null, true);
}
deleteOrder(id: number): Observable<Order> {
return this.sendRequest(RequestMethod.Delete,
`orders/${id}`, null, true);
}
updateOrder(order: Order): Observable<Order> {
return this.sendRequest(RequestMethod.Put,
`orders/${order.id}`, order, true);
}
saveOrder(order: Order): Observable<Order> {
return this.sendRequest(RequestMethod.Post,
"orders", order);
}
private sendRequest(verb: RequestMethod,
url: string, body?: Product | Order, auth: boolean = false)
: Observable<Product | Product | Order | Order> {
let request = new Request({
method: verb,
url: this.baseUrl + url,
body: body
});
if (auth && this.auth_token != null) {
request.headers.set("Authorization", `Bearer<${this.auth_token}>`);
}
return this.http.request(request).map(response => response.json());
}
}
and here is my static.datasource.ts file:
import { Injectable } from "@angular/core";
import { Product } from "./product.model";
import { Observable } from "rxjs/Observable";
import "rxjs/add/observable/from";
import { Order } from "./order.model";
@Injectable()
export class StaticDataSource {
//Populate product fields with Inventory information
private products: Product = [
//products omitted for brevity
];
getProducts(): Observable<Product> {
return Observable.from([this.products]);
}
saveOrder(order: Order): Observable<Order> {
console.log(JSON.stringify(order));
return Observable.from([order]);
}
}
I am following the tutorial exactly but for some reason the site falls apart now that I am on Chapter 9 creating the admin section. Any help or pointers would be greatly appreciated.
javascript angular
I am working through the tutorial files in the book Pro Angular for a Store Front application. I am currently working on an administration section and am getting an error saying "Failed to load resources, cannot connect to the server". This is my rest.datasource.ts file:
import { Injectable } from "@angular/core";
import { Http, Request, RequestMethod } from "@angular/http";
import { Observable } from "rxjs/Observable";
import { Product } from "./product.model";
import { Cart } from "./cart.model";
import { Order } from "./order.model";
import "rxjs/add/operator/map";
const PROTOCOL = "http";
const PORT = 3000;
@Injectable()
export class RestDataSource {
baseUrl: string;
auth_token: string;
constructor(private http: Http) {
this.baseUrl = `${PROTOCOL}://${location.hostname}:${PORT}/`;
}
authenticate(user: string, pass: string): Observable<boolean> {
return this.http.request(new Request({
method: RequestMethod.Post,
url: this.baseUrl + "login",
body: { name: user, password: pass }
})).map(response => {
let r = response.json();
this.auth_token = r.success ? r.token : null;
return r.success;
});
}
getProducts(): Observable<Product> {
return this.sendRequest(RequestMethod.Get, "products");
}
saveProduct(product: Product): Observable<Product> {
return this.sendRequest(RequestMethod.Post, "products",
product, true);
}
updateProduct(product): Observable<Product> {
return this.sendRequest(RequestMethod.Put,
`products/${product.id}`, product, true);
}
deleteProduct(id: number): Observable<Product> {
return this.sendRequest(RequestMethod.Delete,
`products/${id}`, null, true);
}
getOrders(): Observable<Order> {
return this.sendRequest(RequestMethod.Get,
"orders", null, true);
}
deleteOrder(id: number): Observable<Order> {
return this.sendRequest(RequestMethod.Delete,
`orders/${id}`, null, true);
}
updateOrder(order: Order): Observable<Order> {
return this.sendRequest(RequestMethod.Put,
`orders/${order.id}`, order, true);
}
saveOrder(order: Order): Observable<Order> {
return this.sendRequest(RequestMethod.Post,
"orders", order);
}
private sendRequest(verb: RequestMethod,
url: string, body?: Product | Order, auth: boolean = false)
: Observable<Product | Product | Order | Order> {
let request = new Request({
method: verb,
url: this.baseUrl + url,
body: body
});
if (auth && this.auth_token != null) {
request.headers.set("Authorization", `Bearer<${this.auth_token}>`);
}
return this.http.request(request).map(response => response.json());
}
}
and here is my static.datasource.ts file:
import { Injectable } from "@angular/core";
import { Product } from "./product.model";
import { Observable } from "rxjs/Observable";
import "rxjs/add/observable/from";
import { Order } from "./order.model";
@Injectable()
export class StaticDataSource {
//Populate product fields with Inventory information
private products: Product = [
//products omitted for brevity
];
getProducts(): Observable<Product> {
return Observable.from([this.products]);
}
saveOrder(order: Order): Observable<Order> {
console.log(JSON.stringify(order));
return Observable.from([order]);
}
}
I am following the tutorial exactly but for some reason the site falls apart now that I am on Chapter 9 creating the admin section. Any help or pointers would be greatly appreciated.
javascript angular
javascript angular
edited Nov 18 at 19:35
asked Nov 18 at 19:30
still2blue
9110
9110
1
Was my mistake. Fixed. Thanks!
– still2blue
Nov 18 at 19:35
1
Note that@angular/http
is deprecated in favour of@angular/common/http
; you should probably find a more up-to-date resource.
– jonrsharpe
Nov 18 at 19:43
Did you figure this out?
– nkuma_12
Nov 18 at 20:32
Hmmthis.baseUrl = `${PROTOCOL}://${location.hostname}:${PORT}/`;
did you definelocation
anywhere?
– pandamakes
Nov 18 at 21:12
Also, I would check the version of rxjs you are using. Iirc, rxjs 6 changed how operators work: github.com/ReactiveX/rxjs/blob/master/docs_app/content/guide/v6/…
– pandamakes
Nov 18 at 21:16
add a comment |
1
Was my mistake. Fixed. Thanks!
– still2blue
Nov 18 at 19:35
1
Note that@angular/http
is deprecated in favour of@angular/common/http
; you should probably find a more up-to-date resource.
– jonrsharpe
Nov 18 at 19:43
Did you figure this out?
– nkuma_12
Nov 18 at 20:32
Hmmthis.baseUrl = `${PROTOCOL}://${location.hostname}:${PORT}/`;
did you definelocation
anywhere?
– pandamakes
Nov 18 at 21:12
Also, I would check the version of rxjs you are using. Iirc, rxjs 6 changed how operators work: github.com/ReactiveX/rxjs/blob/master/docs_app/content/guide/v6/…
– pandamakes
Nov 18 at 21:16
1
1
Was my mistake. Fixed. Thanks!
– still2blue
Nov 18 at 19:35
Was my mistake. Fixed. Thanks!
– still2blue
Nov 18 at 19:35
1
1
Note that
@angular/http
is deprecated in favour of @angular/common/http
; you should probably find a more up-to-date resource.– jonrsharpe
Nov 18 at 19:43
Note that
@angular/http
is deprecated in favour of @angular/common/http
; you should probably find a more up-to-date resource.– jonrsharpe
Nov 18 at 19:43
Did you figure this out?
– nkuma_12
Nov 18 at 20:32
Did you figure this out?
– nkuma_12
Nov 18 at 20:32
Hmm
this.baseUrl = `${PROTOCOL}://${location.hostname}:${PORT}/`;
did you define location
anywhere?– pandamakes
Nov 18 at 21:12
Hmm
this.baseUrl = `${PROTOCOL}://${location.hostname}:${PORT}/`;
did you define location
anywhere?– pandamakes
Nov 18 at 21:12
Also, I would check the version of rxjs you are using. Iirc, rxjs 6 changed how operators work: github.com/ReactiveX/rxjs/blob/master/docs_app/content/guide/v6/…
– pandamakes
Nov 18 at 21:16
Also, I would check the version of rxjs you are using. Iirc, rxjs 6 changed how operators work: github.com/ReactiveX/rxjs/blob/master/docs_app/content/guide/v6/…
– pandamakes
Nov 18 at 21:16
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53364673%2fnot-receiving-products-from-function-in-angular%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
v6b f f6ncYJY3,h3kYprFv1 fk9HwVIhByrBMUhb6pw0 lz5W3h,zHLX,Ax,nF
1
Was my mistake. Fixed. Thanks!
– still2blue
Nov 18 at 19:35
1
Note that
@angular/http
is deprecated in favour of@angular/common/http
; you should probably find a more up-to-date resource.– jonrsharpe
Nov 18 at 19:43
Did you figure this out?
– nkuma_12
Nov 18 at 20:32
Hmm
this.baseUrl = `${PROTOCOL}://${location.hostname}:${PORT}/`;
did you definelocation
anywhere?– pandamakes
Nov 18 at 21:12
Also, I would check the version of rxjs you are using. Iirc, rxjs 6 changed how operators work: github.com/ReactiveX/rxjs/blob/master/docs_app/content/guide/v6/…
– pandamakes
Nov 18 at 21:16