How to pass data to the markup of a custom component?
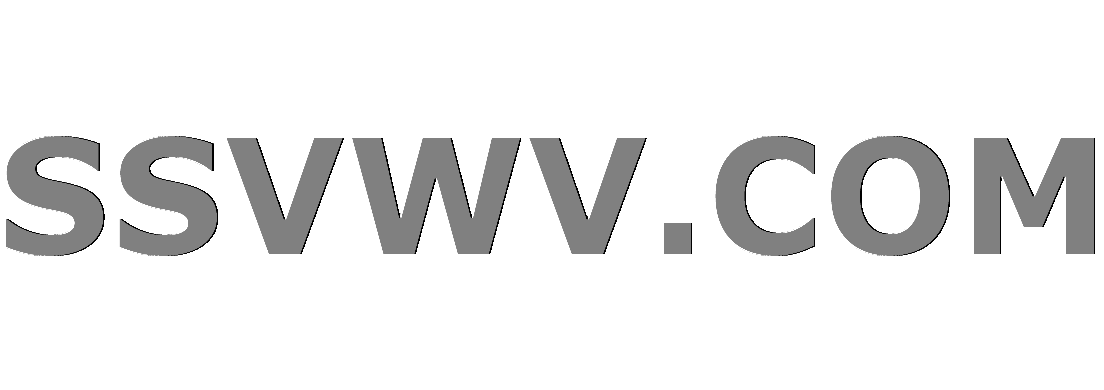
Multi tool use
I have a custom component that allows for editing a user. It displays a dialog which can be fed an existing user. Or not. It has the markup:
<button mat-button (click)="openUserDialog()">Edit</button>
and the controller:
@Component({
selector: 'app-user-edit',
templateUrl: './user-edit.component.html',
})
export class UserEditComponent implements OnChanges {
@Input() existingUser: User;
@Output() userEditedEvent: EventEmitter<User> = new EventEmitter<User>();
userDialogRef: MatDialogRef<UserDialogComponent>;
constructor(
private matDialog: MatDialog,
private userService: UserService
) { }
ngOnChanges() {
}
openUserDialog() {
this.userDialogRef = this.matDialog.open(UserDialogComponent, {
hasBackdrop: false,
data: {
user: this.existingUser
}
});
this.userDialogRef
.afterClosed()
.subscribe(user => {
// TODO validate the edited user
if (user) {
if (this.existingUser) {
user.id = this.existingUser.id;
this.userService.fullUpdate(user)
.subscribe(updatedUser => {
this.userEditedEvent.emit(updatedUser);
// TODO Add a hint that the user has been added
});
} else {
this.userService.add(user)
.subscribe(addedUser => {
this.userEditedEvent.emit(addedUser);
// TODO Add a hint that the user has been updated
});
}
}
});
}
}
The component is then being used in the users list page, once on top of the list to add a new user, with the markup:
<app-user-edit (userEditedEvent)="refreshList($event)"></app-user-edit>
and on each row of the list to edit the user, with the markup:
<app-user-edit [existingUser]="user" (userEditedEvent)="refreshList($event)"></app-user-edit>
The trouble is that the view displays the Edit label both to add and to edit a user.
How could I have a custom Add label on top of the list, and another Update label for each user ?
I feel like I may have overengineered the whole thing.
angular7
add a comment |
I have a custom component that allows for editing a user. It displays a dialog which can be fed an existing user. Or not. It has the markup:
<button mat-button (click)="openUserDialog()">Edit</button>
and the controller:
@Component({
selector: 'app-user-edit',
templateUrl: './user-edit.component.html',
})
export class UserEditComponent implements OnChanges {
@Input() existingUser: User;
@Output() userEditedEvent: EventEmitter<User> = new EventEmitter<User>();
userDialogRef: MatDialogRef<UserDialogComponent>;
constructor(
private matDialog: MatDialog,
private userService: UserService
) { }
ngOnChanges() {
}
openUserDialog() {
this.userDialogRef = this.matDialog.open(UserDialogComponent, {
hasBackdrop: false,
data: {
user: this.existingUser
}
});
this.userDialogRef
.afterClosed()
.subscribe(user => {
// TODO validate the edited user
if (user) {
if (this.existingUser) {
user.id = this.existingUser.id;
this.userService.fullUpdate(user)
.subscribe(updatedUser => {
this.userEditedEvent.emit(updatedUser);
// TODO Add a hint that the user has been added
});
} else {
this.userService.add(user)
.subscribe(addedUser => {
this.userEditedEvent.emit(addedUser);
// TODO Add a hint that the user has been updated
});
}
}
});
}
}
The component is then being used in the users list page, once on top of the list to add a new user, with the markup:
<app-user-edit (userEditedEvent)="refreshList($event)"></app-user-edit>
and on each row of the list to edit the user, with the markup:
<app-user-edit [existingUser]="user" (userEditedEvent)="refreshList($event)"></app-user-edit>
The trouble is that the view displays the Edit label both to add and to edit a user.
How could I have a custom Add label on top of the list, and another Update label for each user ?
I feel like I may have overengineered the whole thing.
angular7
add a comment |
I have a custom component that allows for editing a user. It displays a dialog which can be fed an existing user. Or not. It has the markup:
<button mat-button (click)="openUserDialog()">Edit</button>
and the controller:
@Component({
selector: 'app-user-edit',
templateUrl: './user-edit.component.html',
})
export class UserEditComponent implements OnChanges {
@Input() existingUser: User;
@Output() userEditedEvent: EventEmitter<User> = new EventEmitter<User>();
userDialogRef: MatDialogRef<UserDialogComponent>;
constructor(
private matDialog: MatDialog,
private userService: UserService
) { }
ngOnChanges() {
}
openUserDialog() {
this.userDialogRef = this.matDialog.open(UserDialogComponent, {
hasBackdrop: false,
data: {
user: this.existingUser
}
});
this.userDialogRef
.afterClosed()
.subscribe(user => {
// TODO validate the edited user
if (user) {
if (this.existingUser) {
user.id = this.existingUser.id;
this.userService.fullUpdate(user)
.subscribe(updatedUser => {
this.userEditedEvent.emit(updatedUser);
// TODO Add a hint that the user has been added
});
} else {
this.userService.add(user)
.subscribe(addedUser => {
this.userEditedEvent.emit(addedUser);
// TODO Add a hint that the user has been updated
});
}
}
});
}
}
The component is then being used in the users list page, once on top of the list to add a new user, with the markup:
<app-user-edit (userEditedEvent)="refreshList($event)"></app-user-edit>
and on each row of the list to edit the user, with the markup:
<app-user-edit [existingUser]="user" (userEditedEvent)="refreshList($event)"></app-user-edit>
The trouble is that the view displays the Edit label both to add and to edit a user.
How could I have a custom Add label on top of the list, and another Update label for each user ?
I feel like I may have overengineered the whole thing.
angular7
I have a custom component that allows for editing a user. It displays a dialog which can be fed an existing user. Or not. It has the markup:
<button mat-button (click)="openUserDialog()">Edit</button>
and the controller:
@Component({
selector: 'app-user-edit',
templateUrl: './user-edit.component.html',
})
export class UserEditComponent implements OnChanges {
@Input() existingUser: User;
@Output() userEditedEvent: EventEmitter<User> = new EventEmitter<User>();
userDialogRef: MatDialogRef<UserDialogComponent>;
constructor(
private matDialog: MatDialog,
private userService: UserService
) { }
ngOnChanges() {
}
openUserDialog() {
this.userDialogRef = this.matDialog.open(UserDialogComponent, {
hasBackdrop: false,
data: {
user: this.existingUser
}
});
this.userDialogRef
.afterClosed()
.subscribe(user => {
// TODO validate the edited user
if (user) {
if (this.existingUser) {
user.id = this.existingUser.id;
this.userService.fullUpdate(user)
.subscribe(updatedUser => {
this.userEditedEvent.emit(updatedUser);
// TODO Add a hint that the user has been added
});
} else {
this.userService.add(user)
.subscribe(addedUser => {
this.userEditedEvent.emit(addedUser);
// TODO Add a hint that the user has been updated
});
}
}
});
}
}
The component is then being used in the users list page, once on top of the list to add a new user, with the markup:
<app-user-edit (userEditedEvent)="refreshList($event)"></app-user-edit>
and on each row of the list to edit the user, with the markup:
<app-user-edit [existingUser]="user" (userEditedEvent)="refreshList($event)"></app-user-edit>
The trouble is that the view displays the Edit label both to add and to edit a user.
How could I have a custom Add label on top of the list, and another Update label for each user ?
I feel like I may have overengineered the whole thing.
angular7
angular7
asked Nov 22 '18 at 12:52


StephaneStephane
2,476114981
2,476114981
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can add another @Input parameter say label and pass the value of the label from the mark up.
export class UserEditComponent implements OnChanges {
@Input() existingUser: User;
@Input() label: string = "Edit" // set default to EDIT. If preferred can initialised to empty.
Mark up for ADD:
<app-user-edit (userEditedEvent)="refreshList($event)" label="ADD"></app-user-edit>
Mark up for EDIT:
<app-user-edit [existingUser]="user" (userEditedEvent)="refreshList($event)" label="EDIT"></app-user-edit>
Also, bind parameter label in view where it needs to be shown.
When I specify the attributelabel]="Add"
no label is displayed. When I specify the attributelabel]="Add me"
the browser console shows the runtime error:Unexpected token 'me' at column 5 in [Add me]
.
– Stephane
Nov 22 '18 at 13:53
sorry, remove the square brackets.Have edited the answer accordingly
– user3392782
Nov 22 '18 at 14:07
Interesting.. why some@Input
member variables need the bracket in the markup, while others don't ?
– Stephane
Nov 22 '18 at 14:21
1
brackets make Angular evaluate that as an expression. You can still use but will have to pass the value like [label]=" 'Add me' ". Hope that helps.
– user3392782
Nov 22 '18 at 14:28
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431487%2fhow-to-pass-data-to-the-markup-of-a-custom-component%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can add another @Input parameter say label and pass the value of the label from the mark up.
export class UserEditComponent implements OnChanges {
@Input() existingUser: User;
@Input() label: string = "Edit" // set default to EDIT. If preferred can initialised to empty.
Mark up for ADD:
<app-user-edit (userEditedEvent)="refreshList($event)" label="ADD"></app-user-edit>
Mark up for EDIT:
<app-user-edit [existingUser]="user" (userEditedEvent)="refreshList($event)" label="EDIT"></app-user-edit>
Also, bind parameter label in view where it needs to be shown.
When I specify the attributelabel]="Add"
no label is displayed. When I specify the attributelabel]="Add me"
the browser console shows the runtime error:Unexpected token 'me' at column 5 in [Add me]
.
– Stephane
Nov 22 '18 at 13:53
sorry, remove the square brackets.Have edited the answer accordingly
– user3392782
Nov 22 '18 at 14:07
Interesting.. why some@Input
member variables need the bracket in the markup, while others don't ?
– Stephane
Nov 22 '18 at 14:21
1
brackets make Angular evaluate that as an expression. You can still use but will have to pass the value like [label]=" 'Add me' ". Hope that helps.
– user3392782
Nov 22 '18 at 14:28
add a comment |
You can add another @Input parameter say label and pass the value of the label from the mark up.
export class UserEditComponent implements OnChanges {
@Input() existingUser: User;
@Input() label: string = "Edit" // set default to EDIT. If preferred can initialised to empty.
Mark up for ADD:
<app-user-edit (userEditedEvent)="refreshList($event)" label="ADD"></app-user-edit>
Mark up for EDIT:
<app-user-edit [existingUser]="user" (userEditedEvent)="refreshList($event)" label="EDIT"></app-user-edit>
Also, bind parameter label in view where it needs to be shown.
When I specify the attributelabel]="Add"
no label is displayed. When I specify the attributelabel]="Add me"
the browser console shows the runtime error:Unexpected token 'me' at column 5 in [Add me]
.
– Stephane
Nov 22 '18 at 13:53
sorry, remove the square brackets.Have edited the answer accordingly
– user3392782
Nov 22 '18 at 14:07
Interesting.. why some@Input
member variables need the bracket in the markup, while others don't ?
– Stephane
Nov 22 '18 at 14:21
1
brackets make Angular evaluate that as an expression. You can still use but will have to pass the value like [label]=" 'Add me' ". Hope that helps.
– user3392782
Nov 22 '18 at 14:28
add a comment |
You can add another @Input parameter say label and pass the value of the label from the mark up.
export class UserEditComponent implements OnChanges {
@Input() existingUser: User;
@Input() label: string = "Edit" // set default to EDIT. If preferred can initialised to empty.
Mark up for ADD:
<app-user-edit (userEditedEvent)="refreshList($event)" label="ADD"></app-user-edit>
Mark up for EDIT:
<app-user-edit [existingUser]="user" (userEditedEvent)="refreshList($event)" label="EDIT"></app-user-edit>
Also, bind parameter label in view where it needs to be shown.
You can add another @Input parameter say label and pass the value of the label from the mark up.
export class UserEditComponent implements OnChanges {
@Input() existingUser: User;
@Input() label: string = "Edit" // set default to EDIT. If preferred can initialised to empty.
Mark up for ADD:
<app-user-edit (userEditedEvent)="refreshList($event)" label="ADD"></app-user-edit>
Mark up for EDIT:
<app-user-edit [existingUser]="user" (userEditedEvent)="refreshList($event)" label="EDIT"></app-user-edit>
Also, bind parameter label in view where it needs to be shown.
edited Nov 22 '18 at 14:08
answered Nov 22 '18 at 13:17
user3392782user3392782
1614
1614
When I specify the attributelabel]="Add"
no label is displayed. When I specify the attributelabel]="Add me"
the browser console shows the runtime error:Unexpected token 'me' at column 5 in [Add me]
.
– Stephane
Nov 22 '18 at 13:53
sorry, remove the square brackets.Have edited the answer accordingly
– user3392782
Nov 22 '18 at 14:07
Interesting.. why some@Input
member variables need the bracket in the markup, while others don't ?
– Stephane
Nov 22 '18 at 14:21
1
brackets make Angular evaluate that as an expression. You can still use but will have to pass the value like [label]=" 'Add me' ". Hope that helps.
– user3392782
Nov 22 '18 at 14:28
add a comment |
When I specify the attributelabel]="Add"
no label is displayed. When I specify the attributelabel]="Add me"
the browser console shows the runtime error:Unexpected token 'me' at column 5 in [Add me]
.
– Stephane
Nov 22 '18 at 13:53
sorry, remove the square brackets.Have edited the answer accordingly
– user3392782
Nov 22 '18 at 14:07
Interesting.. why some@Input
member variables need the bracket in the markup, while others don't ?
– Stephane
Nov 22 '18 at 14:21
1
brackets make Angular evaluate that as an expression. You can still use but will have to pass the value like [label]=" 'Add me' ". Hope that helps.
– user3392782
Nov 22 '18 at 14:28
When I specify the attribute
label]="Add"
no label is displayed. When I specify the attribute label]="Add me"
the browser console shows the runtime error: Unexpected token 'me' at column 5 in [Add me]
.– Stephane
Nov 22 '18 at 13:53
When I specify the attribute
label]="Add"
no label is displayed. When I specify the attribute label]="Add me"
the browser console shows the runtime error: Unexpected token 'me' at column 5 in [Add me]
.– Stephane
Nov 22 '18 at 13:53
sorry, remove the square brackets.Have edited the answer accordingly
– user3392782
Nov 22 '18 at 14:07
sorry, remove the square brackets.Have edited the answer accordingly
– user3392782
Nov 22 '18 at 14:07
Interesting.. why some
@Input
member variables need the bracket in the markup, while others don't ?– Stephane
Nov 22 '18 at 14:21
Interesting.. why some
@Input
member variables need the bracket in the markup, while others don't ?– Stephane
Nov 22 '18 at 14:21
1
1
brackets make Angular evaluate that as an expression. You can still use but will have to pass the value like [label]=" 'Add me' ". Hope that helps.
– user3392782
Nov 22 '18 at 14:28
brackets make Angular evaluate that as an expression. You can still use but will have to pass the value like [label]=" 'Add me' ". Hope that helps.
– user3392782
Nov 22 '18 at 14:28
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53431487%2fhow-to-pass-data-to-the-markup-of-a-custom-component%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
R,xHBq2Kye5urtHJUIwoUJ