jackson nested object map implementation
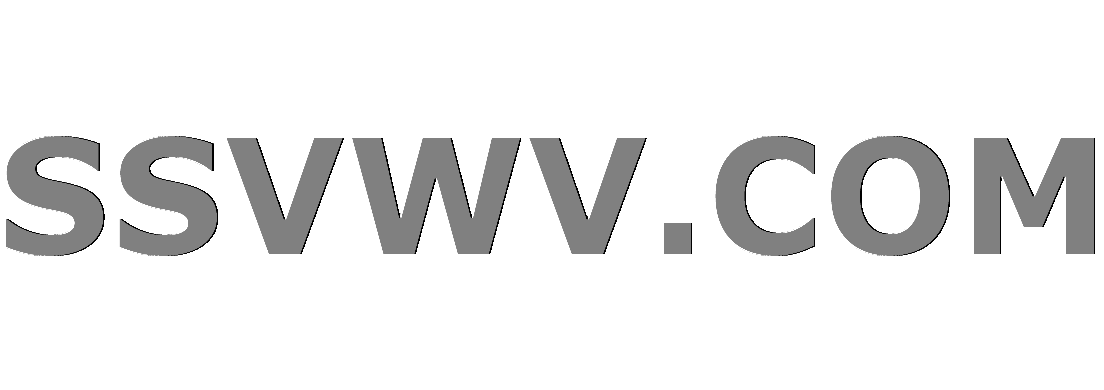
Multi tool use
Is there any way to tell jackson library whish Map implementation to use in nested object?
I have some Map extended from HashMap, I dont care of fields order
public class FieldsMap<K, V> extends HashMap<K, V> {
@Override
public V get(Object field) {
V value = super.get(field);
if (value == null && !containsKey(field)) {
log.warn("Query unknown field " + field);
}
return value;
}
}
And ObjectMapper.readValue return this class
final TypeReference<FieldsMap<String, Object>> mapType =
new TypeReference<FieldsMap<String, Object>>() {};
Map<String, Object> res = new ObjectMapper().readValue("{"a": {"b": 0}}", mapType);
res.get("c"); // warning
But nested object is always LinkedHashMap
System.out.println(res.get("a").getClass().getName()); // java.util.LinkedHashMap
((Map)res.get("a")).get("c"); // no warning
It will be helpful in test environments.
java jackson
add a comment |
Is there any way to tell jackson library whish Map implementation to use in nested object?
I have some Map extended from HashMap, I dont care of fields order
public class FieldsMap<K, V> extends HashMap<K, V> {
@Override
public V get(Object field) {
V value = super.get(field);
if (value == null && !containsKey(field)) {
log.warn("Query unknown field " + field);
}
return value;
}
}
And ObjectMapper.readValue return this class
final TypeReference<FieldsMap<String, Object>> mapType =
new TypeReference<FieldsMap<String, Object>>() {};
Map<String, Object> res = new ObjectMapper().readValue("{"a": {"b": 0}}", mapType);
res.get("c"); // warning
But nested object is always LinkedHashMap
System.out.println(res.get("a").getClass().getName()); // java.util.LinkedHashMap
((Map)res.get("a")).get("c"); // no warning
It will be helpful in test environments.
java jackson
your json is nested json, and always nested json is with key value pair and treated asMap
– Deadpool
Nov 23 '18 at 7:23
I mean subobjects in json tree, element "a" in my example. Need a comon solution where you dont know tree structure
– lunicon
Nov 23 '18 at 8:23
add a comment |
Is there any way to tell jackson library whish Map implementation to use in nested object?
I have some Map extended from HashMap, I dont care of fields order
public class FieldsMap<K, V> extends HashMap<K, V> {
@Override
public V get(Object field) {
V value = super.get(field);
if (value == null && !containsKey(field)) {
log.warn("Query unknown field " + field);
}
return value;
}
}
And ObjectMapper.readValue return this class
final TypeReference<FieldsMap<String, Object>> mapType =
new TypeReference<FieldsMap<String, Object>>() {};
Map<String, Object> res = new ObjectMapper().readValue("{"a": {"b": 0}}", mapType);
res.get("c"); // warning
But nested object is always LinkedHashMap
System.out.println(res.get("a").getClass().getName()); // java.util.LinkedHashMap
((Map)res.get("a")).get("c"); // no warning
It will be helpful in test environments.
java jackson
Is there any way to tell jackson library whish Map implementation to use in nested object?
I have some Map extended from HashMap, I dont care of fields order
public class FieldsMap<K, V> extends HashMap<K, V> {
@Override
public V get(Object field) {
V value = super.get(field);
if (value == null && !containsKey(field)) {
log.warn("Query unknown field " + field);
}
return value;
}
}
And ObjectMapper.readValue return this class
final TypeReference<FieldsMap<String, Object>> mapType =
new TypeReference<FieldsMap<String, Object>>() {};
Map<String, Object> res = new ObjectMapper().readValue("{"a": {"b": 0}}", mapType);
res.get("c"); // warning
But nested object is always LinkedHashMap
System.out.println(res.get("a").getClass().getName()); // java.util.LinkedHashMap
((Map)res.get("a")).get("c"); // no warning
It will be helpful in test environments.
java jackson
java jackson
edited Nov 28 '18 at 20:07
lunicon
asked Nov 23 '18 at 7:08


luniconlunicon
93011022
93011022
your json is nested json, and always nested json is with key value pair and treated asMap
– Deadpool
Nov 23 '18 at 7:23
I mean subobjects in json tree, element "a" in my example. Need a comon solution where you dont know tree structure
– lunicon
Nov 23 '18 at 8:23
add a comment |
your json is nested json, and always nested json is with key value pair and treated asMap
– Deadpool
Nov 23 '18 at 7:23
I mean subobjects in json tree, element "a" in my example. Need a comon solution where you dont know tree structure
– lunicon
Nov 23 '18 at 8:23
your json is nested json, and always nested json is with key value pair and treated as
Map
– Deadpool
Nov 23 '18 at 7:23
your json is nested json, and always nested json is with key value pair and treated as
Map
– Deadpool
Nov 23 '18 at 7:23
I mean subobjects in json tree, element "a" in my example. Need a comon solution where you dont know tree structure
– lunicon
Nov 23 '18 at 8:23
I mean subobjects in json tree, element "a" in my example. Need a comon solution where you dont know tree structure
– lunicon
Nov 23 '18 at 8:23
add a comment |
1 Answer
1
active
oldest
votes
You must add a an abstract type mapping to your ObjectMapper. This is done with a module:
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.module.SimpleModule;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public class FieldsMap<K, V> extends HashMap<K, V> {
@Override
public V get(Object field) {
V value = super.get(field);
if (value == null && !containsKey(field)) {
System.out.println("Query unknown field " + field);
}
return value;
}
public static void main(String args) throws IOException {
final TypeReference<FieldsMap<String, Object>> mapType = new TypeReference<FieldsMap<String, Object>>() {};
ObjectMapper objectMapper = new ObjectMapper();
SimpleModule module = new SimpleModule().addAbstractTypeMapping(Map.class, FieldsMap.class);
objectMapper.registerModule(module);
Map<String, Object> res = objectMapper.readValue("{"a": {"b": 0}}", mapType);
res.get("c");
System.out.println(res.get("a").getClass().getName());
((Map)res.get("a")).get("c");
}
}
This will instruct Jackson to use your custom type FieldsMap
each time a Map
is needed.
The output is:
Query unknown field c
FieldsMap
Query unknown field c
I tried. Unfortunately not working.
– lunicon
Nov 28 '18 at 13:53
@lunicon It's weird, because it does work for me. I reproduced the exact same sample you provided. What version of Jackson do you use ?
– Benoit
Nov 28 '18 at 13:55
dependencies 'com.fasterxml.jackson.core:jackson-core:2.4.2'. Maybe I'm using it in wrong way. Can you provide full example
– lunicon
Nov 28 '18 at 13:58
@lunicon I updated my answer with a full working example.
– Benoit
Nov 28 '18 at 15:33
I thought that the error was in inner class usage. No, this feature added in later version.. Thanks by the way
– lunicon
Nov 28 '18 at 20:06
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53442080%2fjackson-nested-object-map-implementation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You must add a an abstract type mapping to your ObjectMapper. This is done with a module:
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.module.SimpleModule;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public class FieldsMap<K, V> extends HashMap<K, V> {
@Override
public V get(Object field) {
V value = super.get(field);
if (value == null && !containsKey(field)) {
System.out.println("Query unknown field " + field);
}
return value;
}
public static void main(String args) throws IOException {
final TypeReference<FieldsMap<String, Object>> mapType = new TypeReference<FieldsMap<String, Object>>() {};
ObjectMapper objectMapper = new ObjectMapper();
SimpleModule module = new SimpleModule().addAbstractTypeMapping(Map.class, FieldsMap.class);
objectMapper.registerModule(module);
Map<String, Object> res = objectMapper.readValue("{"a": {"b": 0}}", mapType);
res.get("c");
System.out.println(res.get("a").getClass().getName());
((Map)res.get("a")).get("c");
}
}
This will instruct Jackson to use your custom type FieldsMap
each time a Map
is needed.
The output is:
Query unknown field c
FieldsMap
Query unknown field c
I tried. Unfortunately not working.
– lunicon
Nov 28 '18 at 13:53
@lunicon It's weird, because it does work for me. I reproduced the exact same sample you provided. What version of Jackson do you use ?
– Benoit
Nov 28 '18 at 13:55
dependencies 'com.fasterxml.jackson.core:jackson-core:2.4.2'. Maybe I'm using it in wrong way. Can you provide full example
– lunicon
Nov 28 '18 at 13:58
@lunicon I updated my answer with a full working example.
– Benoit
Nov 28 '18 at 15:33
I thought that the error was in inner class usage. No, this feature added in later version.. Thanks by the way
– lunicon
Nov 28 '18 at 20:06
add a comment |
You must add a an abstract type mapping to your ObjectMapper. This is done with a module:
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.module.SimpleModule;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public class FieldsMap<K, V> extends HashMap<K, V> {
@Override
public V get(Object field) {
V value = super.get(field);
if (value == null && !containsKey(field)) {
System.out.println("Query unknown field " + field);
}
return value;
}
public static void main(String args) throws IOException {
final TypeReference<FieldsMap<String, Object>> mapType = new TypeReference<FieldsMap<String, Object>>() {};
ObjectMapper objectMapper = new ObjectMapper();
SimpleModule module = new SimpleModule().addAbstractTypeMapping(Map.class, FieldsMap.class);
objectMapper.registerModule(module);
Map<String, Object> res = objectMapper.readValue("{"a": {"b": 0}}", mapType);
res.get("c");
System.out.println(res.get("a").getClass().getName());
((Map)res.get("a")).get("c");
}
}
This will instruct Jackson to use your custom type FieldsMap
each time a Map
is needed.
The output is:
Query unknown field c
FieldsMap
Query unknown field c
I tried. Unfortunately not working.
– lunicon
Nov 28 '18 at 13:53
@lunicon It's weird, because it does work for me. I reproduced the exact same sample you provided. What version of Jackson do you use ?
– Benoit
Nov 28 '18 at 13:55
dependencies 'com.fasterxml.jackson.core:jackson-core:2.4.2'. Maybe I'm using it in wrong way. Can you provide full example
– lunicon
Nov 28 '18 at 13:58
@lunicon I updated my answer with a full working example.
– Benoit
Nov 28 '18 at 15:33
I thought that the error was in inner class usage. No, this feature added in later version.. Thanks by the way
– lunicon
Nov 28 '18 at 20:06
add a comment |
You must add a an abstract type mapping to your ObjectMapper. This is done with a module:
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.module.SimpleModule;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public class FieldsMap<K, V> extends HashMap<K, V> {
@Override
public V get(Object field) {
V value = super.get(field);
if (value == null && !containsKey(field)) {
System.out.println("Query unknown field " + field);
}
return value;
}
public static void main(String args) throws IOException {
final TypeReference<FieldsMap<String, Object>> mapType = new TypeReference<FieldsMap<String, Object>>() {};
ObjectMapper objectMapper = new ObjectMapper();
SimpleModule module = new SimpleModule().addAbstractTypeMapping(Map.class, FieldsMap.class);
objectMapper.registerModule(module);
Map<String, Object> res = objectMapper.readValue("{"a": {"b": 0}}", mapType);
res.get("c");
System.out.println(res.get("a").getClass().getName());
((Map)res.get("a")).get("c");
}
}
This will instruct Jackson to use your custom type FieldsMap
each time a Map
is needed.
The output is:
Query unknown field c
FieldsMap
Query unknown field c
You must add a an abstract type mapping to your ObjectMapper. This is done with a module:
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.module.SimpleModule;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
public class FieldsMap<K, V> extends HashMap<K, V> {
@Override
public V get(Object field) {
V value = super.get(field);
if (value == null && !containsKey(field)) {
System.out.println("Query unknown field " + field);
}
return value;
}
public static void main(String args) throws IOException {
final TypeReference<FieldsMap<String, Object>> mapType = new TypeReference<FieldsMap<String, Object>>() {};
ObjectMapper objectMapper = new ObjectMapper();
SimpleModule module = new SimpleModule().addAbstractTypeMapping(Map.class, FieldsMap.class);
objectMapper.registerModule(module);
Map<String, Object> res = objectMapper.readValue("{"a": {"b": 0}}", mapType);
res.get("c");
System.out.println(res.get("a").getClass().getName());
((Map)res.get("a")).get("c");
}
}
This will instruct Jackson to use your custom type FieldsMap
each time a Map
is needed.
The output is:
Query unknown field c
FieldsMap
Query unknown field c
edited Nov 28 '18 at 15:30
answered Nov 28 '18 at 10:18


BenoitBenoit
2,5462929
2,5462929
I tried. Unfortunately not working.
– lunicon
Nov 28 '18 at 13:53
@lunicon It's weird, because it does work for me. I reproduced the exact same sample you provided. What version of Jackson do you use ?
– Benoit
Nov 28 '18 at 13:55
dependencies 'com.fasterxml.jackson.core:jackson-core:2.4.2'. Maybe I'm using it in wrong way. Can you provide full example
– lunicon
Nov 28 '18 at 13:58
@lunicon I updated my answer with a full working example.
– Benoit
Nov 28 '18 at 15:33
I thought that the error was in inner class usage. No, this feature added in later version.. Thanks by the way
– lunicon
Nov 28 '18 at 20:06
add a comment |
I tried. Unfortunately not working.
– lunicon
Nov 28 '18 at 13:53
@lunicon It's weird, because it does work for me. I reproduced the exact same sample you provided. What version of Jackson do you use ?
– Benoit
Nov 28 '18 at 13:55
dependencies 'com.fasterxml.jackson.core:jackson-core:2.4.2'. Maybe I'm using it in wrong way. Can you provide full example
– lunicon
Nov 28 '18 at 13:58
@lunicon I updated my answer with a full working example.
– Benoit
Nov 28 '18 at 15:33
I thought that the error was in inner class usage. No, this feature added in later version.. Thanks by the way
– lunicon
Nov 28 '18 at 20:06
I tried. Unfortunately not working.
– lunicon
Nov 28 '18 at 13:53
I tried. Unfortunately not working.
– lunicon
Nov 28 '18 at 13:53
@lunicon It's weird, because it does work for me. I reproduced the exact same sample you provided. What version of Jackson do you use ?
– Benoit
Nov 28 '18 at 13:55
@lunicon It's weird, because it does work for me. I reproduced the exact same sample you provided. What version of Jackson do you use ?
– Benoit
Nov 28 '18 at 13:55
dependencies 'com.fasterxml.jackson.core:jackson-core:2.4.2'. Maybe I'm using it in wrong way. Can you provide full example
– lunicon
Nov 28 '18 at 13:58
dependencies 'com.fasterxml.jackson.core:jackson-core:2.4.2'. Maybe I'm using it in wrong way. Can you provide full example
– lunicon
Nov 28 '18 at 13:58
@lunicon I updated my answer with a full working example.
– Benoit
Nov 28 '18 at 15:33
@lunicon I updated my answer with a full working example.
– Benoit
Nov 28 '18 at 15:33
I thought that the error was in inner class usage. No, this feature added in later version.. Thanks by the way
– lunicon
Nov 28 '18 at 20:06
I thought that the error was in inner class usage. No, this feature added in later version.. Thanks by the way
– lunicon
Nov 28 '18 at 20:06
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53442080%2fjackson-nested-object-map-implementation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
X,xouM7e4A,zgnaSR2j0uet1,UHxaL
your json is nested json, and always nested json is with key value pair and treated as
Map
– Deadpool
Nov 23 '18 at 7:23
I mean subobjects in json tree, element "a" in my example. Need a comon solution where you dont know tree structure
– lunicon
Nov 23 '18 at 8:23