Solution of the binary to integer code through stream api explanation
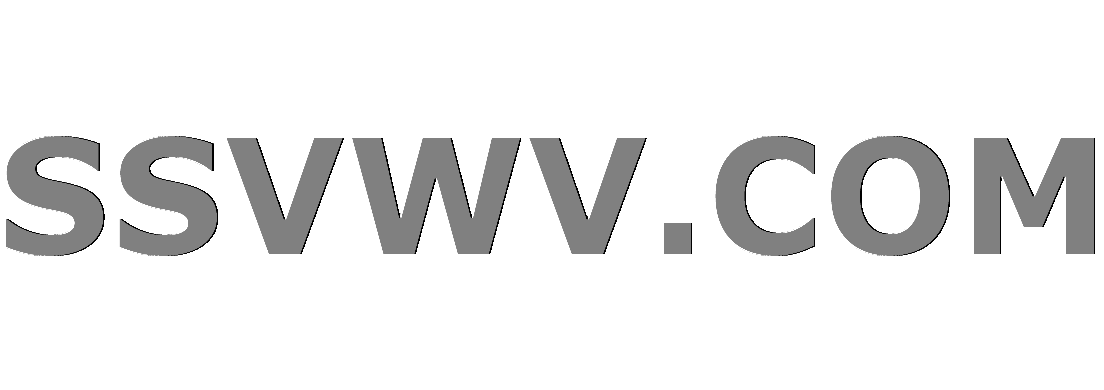
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
On solving a kata on codewars I came across a single line solution to the problem of converting a binary number (in the form of a list ) to integer.
I am unable to understand the solution where people have used java stream api reduce function.Please help me understand it.
Eg: [0, 0, 0, 1] is treated as 0001 which is the binary representation of 1.
import java.util.List;
public class BinaryArrayToNumber {
public static int ConvertBinaryArrayToInt(List<Integer> binary) {
return binary.stream().reduce((x, y) -> x * 2 + y).get();
}
}
java java-8
add a comment |
On solving a kata on codewars I came across a single line solution to the problem of converting a binary number (in the form of a list ) to integer.
I am unable to understand the solution where people have used java stream api reduce function.Please help me understand it.
Eg: [0, 0, 0, 1] is treated as 0001 which is the binary representation of 1.
import java.util.List;
public class BinaryArrayToNumber {
public static int ConvertBinaryArrayToInt(List<Integer> binary) {
return binary.stream().reduce((x, y) -> x * 2 + y).get();
}
}
java java-8
Only 1 binary number is stored in List ? Or every object of Integer contain a binary number?
– Khalid Shah
Nov 23 '18 at 12:52
only 1 binary number
– Pooja
Nov 23 '18 at 12:53
3
This solution is wrong, it only works for sequential streams and it's violatingStream.reduce
's contract, which mandates that the function must be associative ((x, y) -> x * 2 + y
clearly isn't).
– Federico Peralta Schaffner
Nov 23 '18 at 13:45
add a comment |
On solving a kata on codewars I came across a single line solution to the problem of converting a binary number (in the form of a list ) to integer.
I am unable to understand the solution where people have used java stream api reduce function.Please help me understand it.
Eg: [0, 0, 0, 1] is treated as 0001 which is the binary representation of 1.
import java.util.List;
public class BinaryArrayToNumber {
public static int ConvertBinaryArrayToInt(List<Integer> binary) {
return binary.stream().reduce((x, y) -> x * 2 + y).get();
}
}
java java-8
On solving a kata on codewars I came across a single line solution to the problem of converting a binary number (in the form of a list ) to integer.
I am unable to understand the solution where people have used java stream api reduce function.Please help me understand it.
Eg: [0, 0, 0, 1] is treated as 0001 which is the binary representation of 1.
import java.util.List;
public class BinaryArrayToNumber {
public static int ConvertBinaryArrayToInt(List<Integer> binary) {
return binary.stream().reduce((x, y) -> x * 2 + y).get();
}
}
java java-8
java java-8
edited Nov 23 '18 at 12:54
Pooja
asked Nov 23 '18 at 12:39
PoojaPooja
1861312
1861312
Only 1 binary number is stored in List ? Or every object of Integer contain a binary number?
– Khalid Shah
Nov 23 '18 at 12:52
only 1 binary number
– Pooja
Nov 23 '18 at 12:53
3
This solution is wrong, it only works for sequential streams and it's violatingStream.reduce
's contract, which mandates that the function must be associative ((x, y) -> x * 2 + y
clearly isn't).
– Federico Peralta Schaffner
Nov 23 '18 at 13:45
add a comment |
Only 1 binary number is stored in List ? Or every object of Integer contain a binary number?
– Khalid Shah
Nov 23 '18 at 12:52
only 1 binary number
– Pooja
Nov 23 '18 at 12:53
3
This solution is wrong, it only works for sequential streams and it's violatingStream.reduce
's contract, which mandates that the function must be associative ((x, y) -> x * 2 + y
clearly isn't).
– Federico Peralta Schaffner
Nov 23 '18 at 13:45
Only 1 binary number is stored in List ? Or every object of Integer contain a binary number?
– Khalid Shah
Nov 23 '18 at 12:52
Only 1 binary number is stored in List ? Or every object of Integer contain a binary number?
– Khalid Shah
Nov 23 '18 at 12:52
only 1 binary number
– Pooja
Nov 23 '18 at 12:53
only 1 binary number
– Pooja
Nov 23 '18 at 12:53
3
3
This solution is wrong, it only works for sequential streams and it's violating
Stream.reduce
's contract, which mandates that the function must be associative ((x, y) -> x * 2 + y
clearly isn't).– Federico Peralta Schaffner
Nov 23 '18 at 13:45
This solution is wrong, it only works for sequential streams and it's violating
Stream.reduce
's contract, which mandates that the function must be associative ((x, y) -> x * 2 + y
clearly isn't).– Federico Peralta Schaffner
Nov 23 '18 at 13:45
add a comment |
3 Answers
3
active
oldest
votes
First of all the math behind it is based on the method described here
The working of the code is best understood with the help of an example. Consider a binary number 1011 (Decimal 11)
- The operation is streamed sequentially . From the way the reduce operation behaves we'll rename
x
asaccumulator
andy
asele
We can now state the above operation mathematically as
accumulator = (accumulator*2)+ ele
// refer here for why
Lets run the formula on 1011
- Streaming begins with the first element i.e the left side and goes sequentially
- start: accumulator = 0
- 1) accumulator = (0 * 2) + 1 //first element, accumulator = 1
- 2) accumulator = (1 * 2) + 0 //second element, accumulator = 2
- 3) accumulator = (2 * 2) + 1 //third element, accumulator = 5
- 4) accumulator = (5 * 2) + 1 //last element, accumulator = 11
You can extend this to n binary digits.
One important thing to note is that although this is okay for coding competitions, this should never be used in a real world appication as the reduce()
expects an associative operation and also gives no guarantees of running sequentially
What does .get() do?
– Pooja
Nov 23 '18 at 14:56
1
get() is a method in [java.util.Optional
] (docs.oracle.com/javase/9/docs/api/java/util/Optional.html) , its used to denote that there may or may not be a value present. Its like a safe alternative to usingnull
in your code. Over here, if you were to run the function on an emptyList
then you would end up with ajava.util.NoSuchElementException
denoting no values were there to process in the first place, otherwise it will return the result.
– Ryotsu
Nov 23 '18 at 15:04
add a comment |
The purpose of the reduce function is to accumulate the elements from the stream into a single result, using the given formula.
This accumulation process is done repeatedly for each element of the stream. Initially, 1st argument("x") takes 0, and the 2nd argument("y") takes first value from the stream. For each subsequent step, the result from the previous calculation will be used as the 1st argument, and the next value from the stream will be used as the 2nd argument.
Using procedural programming, return binary.stream().reduce((x, y) -> x * 2 + y).get(); in your case would be equivalent to the following snippet:
int x = 0;
for(int y : binary) {
x = x * 2 + y;
}
return x;
add a comment |
reduce
essentially enables one to "reduce" (also known as fold, accumulate) the streams elements into a single value.
You don't need to look any further than the reduce method documentation to find out the exact steps taken:
it provides an example saying it's equivalent to:
boolean foundAny = false;
T result = null;
for (T element : this stream) {
if (!foundAny) {
foundAny = true;
result = element;
}
else
result = accumulator.apply(result, element);
}
return foundAny ? Optional.of(result) : Optional.empty();
where accumulator
is the function you've supplied i.e. (x, y) -> x * 2 + y
6
But only for sequential streams. Since(x, y) -> x * 2 + y
is not an associative function, it’s abusingreduce
for a left-fold (which the Stream API does not offer). Executed in parallel, this solution could produce wrong numbers.
– Holger
Nov 23 '18 at 13:18
@Holger I think that comment would be more appropriate to the OP under their post rather than the code I've shown :).
– Aomine
Nov 23 '18 at 13:53
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446884%2fsolution-of-the-binary-to-integer-code-through-stream-api-explanation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
First of all the math behind it is based on the method described here
The working of the code is best understood with the help of an example. Consider a binary number 1011 (Decimal 11)
- The operation is streamed sequentially . From the way the reduce operation behaves we'll rename
x
asaccumulator
andy
asele
We can now state the above operation mathematically as
accumulator = (accumulator*2)+ ele
// refer here for why
Lets run the formula on 1011
- Streaming begins with the first element i.e the left side and goes sequentially
- start: accumulator = 0
- 1) accumulator = (0 * 2) + 1 //first element, accumulator = 1
- 2) accumulator = (1 * 2) + 0 //second element, accumulator = 2
- 3) accumulator = (2 * 2) + 1 //third element, accumulator = 5
- 4) accumulator = (5 * 2) + 1 //last element, accumulator = 11
You can extend this to n binary digits.
One important thing to note is that although this is okay for coding competitions, this should never be used in a real world appication as the reduce()
expects an associative operation and also gives no guarantees of running sequentially
What does .get() do?
– Pooja
Nov 23 '18 at 14:56
1
get() is a method in [java.util.Optional
] (docs.oracle.com/javase/9/docs/api/java/util/Optional.html) , its used to denote that there may or may not be a value present. Its like a safe alternative to usingnull
in your code. Over here, if you were to run the function on an emptyList
then you would end up with ajava.util.NoSuchElementException
denoting no values were there to process in the first place, otherwise it will return the result.
– Ryotsu
Nov 23 '18 at 15:04
add a comment |
First of all the math behind it is based on the method described here
The working of the code is best understood with the help of an example. Consider a binary number 1011 (Decimal 11)
- The operation is streamed sequentially . From the way the reduce operation behaves we'll rename
x
asaccumulator
andy
asele
We can now state the above operation mathematically as
accumulator = (accumulator*2)+ ele
// refer here for why
Lets run the formula on 1011
- Streaming begins with the first element i.e the left side and goes sequentially
- start: accumulator = 0
- 1) accumulator = (0 * 2) + 1 //first element, accumulator = 1
- 2) accumulator = (1 * 2) + 0 //second element, accumulator = 2
- 3) accumulator = (2 * 2) + 1 //third element, accumulator = 5
- 4) accumulator = (5 * 2) + 1 //last element, accumulator = 11
You can extend this to n binary digits.
One important thing to note is that although this is okay for coding competitions, this should never be used in a real world appication as the reduce()
expects an associative operation and also gives no guarantees of running sequentially
What does .get() do?
– Pooja
Nov 23 '18 at 14:56
1
get() is a method in [java.util.Optional
] (docs.oracle.com/javase/9/docs/api/java/util/Optional.html) , its used to denote that there may or may not be a value present. Its like a safe alternative to usingnull
in your code. Over here, if you were to run the function on an emptyList
then you would end up with ajava.util.NoSuchElementException
denoting no values were there to process in the first place, otherwise it will return the result.
– Ryotsu
Nov 23 '18 at 15:04
add a comment |
First of all the math behind it is based on the method described here
The working of the code is best understood with the help of an example. Consider a binary number 1011 (Decimal 11)
- The operation is streamed sequentially . From the way the reduce operation behaves we'll rename
x
asaccumulator
andy
asele
We can now state the above operation mathematically as
accumulator = (accumulator*2)+ ele
// refer here for why
Lets run the formula on 1011
- Streaming begins with the first element i.e the left side and goes sequentially
- start: accumulator = 0
- 1) accumulator = (0 * 2) + 1 //first element, accumulator = 1
- 2) accumulator = (1 * 2) + 0 //second element, accumulator = 2
- 3) accumulator = (2 * 2) + 1 //third element, accumulator = 5
- 4) accumulator = (5 * 2) + 1 //last element, accumulator = 11
You can extend this to n binary digits.
One important thing to note is that although this is okay for coding competitions, this should never be used in a real world appication as the reduce()
expects an associative operation and also gives no guarantees of running sequentially
First of all the math behind it is based on the method described here
The working of the code is best understood with the help of an example. Consider a binary number 1011 (Decimal 11)
- The operation is streamed sequentially . From the way the reduce operation behaves we'll rename
x
asaccumulator
andy
asele
We can now state the above operation mathematically as
accumulator = (accumulator*2)+ ele
// refer here for why
Lets run the formula on 1011
- Streaming begins with the first element i.e the left side and goes sequentially
- start: accumulator = 0
- 1) accumulator = (0 * 2) + 1 //first element, accumulator = 1
- 2) accumulator = (1 * 2) + 0 //second element, accumulator = 2
- 3) accumulator = (2 * 2) + 1 //third element, accumulator = 5
- 4) accumulator = (5 * 2) + 1 //last element, accumulator = 11
You can extend this to n binary digits.
One important thing to note is that although this is okay for coding competitions, this should never be used in a real world appication as the reduce()
expects an associative operation and also gives no guarantees of running sequentially
edited Nov 23 '18 at 13:26
answered Nov 23 '18 at 13:12


RyotsuRyotsu
620514
620514
What does .get() do?
– Pooja
Nov 23 '18 at 14:56
1
get() is a method in [java.util.Optional
] (docs.oracle.com/javase/9/docs/api/java/util/Optional.html) , its used to denote that there may or may not be a value present. Its like a safe alternative to usingnull
in your code. Over here, if you were to run the function on an emptyList
then you would end up with ajava.util.NoSuchElementException
denoting no values were there to process in the first place, otherwise it will return the result.
– Ryotsu
Nov 23 '18 at 15:04
add a comment |
What does .get() do?
– Pooja
Nov 23 '18 at 14:56
1
get() is a method in [java.util.Optional
] (docs.oracle.com/javase/9/docs/api/java/util/Optional.html) , its used to denote that there may or may not be a value present. Its like a safe alternative to usingnull
in your code. Over here, if you were to run the function on an emptyList
then you would end up with ajava.util.NoSuchElementException
denoting no values were there to process in the first place, otherwise it will return the result.
– Ryotsu
Nov 23 '18 at 15:04
What does .get() do?
– Pooja
Nov 23 '18 at 14:56
What does .get() do?
– Pooja
Nov 23 '18 at 14:56
1
1
get() is a method in [
java.util.Optional
] (docs.oracle.com/javase/9/docs/api/java/util/Optional.html) , its used to denote that there may or may not be a value present. Its like a safe alternative to using null
in your code. Over here, if you were to run the function on an empty List
then you would end up with a java.util.NoSuchElementException
denoting no values were there to process in the first place, otherwise it will return the result.– Ryotsu
Nov 23 '18 at 15:04
get() is a method in [
java.util.Optional
] (docs.oracle.com/javase/9/docs/api/java/util/Optional.html) , its used to denote that there may or may not be a value present. Its like a safe alternative to using null
in your code. Over here, if you were to run the function on an empty List
then you would end up with a java.util.NoSuchElementException
denoting no values were there to process in the first place, otherwise it will return the result.– Ryotsu
Nov 23 '18 at 15:04
add a comment |
The purpose of the reduce function is to accumulate the elements from the stream into a single result, using the given formula.
This accumulation process is done repeatedly for each element of the stream. Initially, 1st argument("x") takes 0, and the 2nd argument("y") takes first value from the stream. For each subsequent step, the result from the previous calculation will be used as the 1st argument, and the next value from the stream will be used as the 2nd argument.
Using procedural programming, return binary.stream().reduce((x, y) -> x * 2 + y).get(); in your case would be equivalent to the following snippet:
int x = 0;
for(int y : binary) {
x = x * 2 + y;
}
return x;
add a comment |
The purpose of the reduce function is to accumulate the elements from the stream into a single result, using the given formula.
This accumulation process is done repeatedly for each element of the stream. Initially, 1st argument("x") takes 0, and the 2nd argument("y") takes first value from the stream. For each subsequent step, the result from the previous calculation will be used as the 1st argument, and the next value from the stream will be used as the 2nd argument.
Using procedural programming, return binary.stream().reduce((x, y) -> x * 2 + y).get(); in your case would be equivalent to the following snippet:
int x = 0;
for(int y : binary) {
x = x * 2 + y;
}
return x;
add a comment |
The purpose of the reduce function is to accumulate the elements from the stream into a single result, using the given formula.
This accumulation process is done repeatedly for each element of the stream. Initially, 1st argument("x") takes 0, and the 2nd argument("y") takes first value from the stream. For each subsequent step, the result from the previous calculation will be used as the 1st argument, and the next value from the stream will be used as the 2nd argument.
Using procedural programming, return binary.stream().reduce((x, y) -> x * 2 + y).get(); in your case would be equivalent to the following snippet:
int x = 0;
for(int y : binary) {
x = x * 2 + y;
}
return x;
The purpose of the reduce function is to accumulate the elements from the stream into a single result, using the given formula.
This accumulation process is done repeatedly for each element of the stream. Initially, 1st argument("x") takes 0, and the 2nd argument("y") takes first value from the stream. For each subsequent step, the result from the previous calculation will be used as the 1st argument, and the next value from the stream will be used as the 2nd argument.
Using procedural programming, return binary.stream().reduce((x, y) -> x * 2 + y).get(); in your case would be equivalent to the following snippet:
int x = 0;
for(int y : binary) {
x = x * 2 + y;
}
return x;
answered Nov 23 '18 at 13:13
elyorelyor
515214
515214
add a comment |
add a comment |
reduce
essentially enables one to "reduce" (also known as fold, accumulate) the streams elements into a single value.
You don't need to look any further than the reduce method documentation to find out the exact steps taken:
it provides an example saying it's equivalent to:
boolean foundAny = false;
T result = null;
for (T element : this stream) {
if (!foundAny) {
foundAny = true;
result = element;
}
else
result = accumulator.apply(result, element);
}
return foundAny ? Optional.of(result) : Optional.empty();
where accumulator
is the function you've supplied i.e. (x, y) -> x * 2 + y
6
But only for sequential streams. Since(x, y) -> x * 2 + y
is not an associative function, it’s abusingreduce
for a left-fold (which the Stream API does not offer). Executed in parallel, this solution could produce wrong numbers.
– Holger
Nov 23 '18 at 13:18
@Holger I think that comment would be more appropriate to the OP under their post rather than the code I've shown :).
– Aomine
Nov 23 '18 at 13:53
add a comment |
reduce
essentially enables one to "reduce" (also known as fold, accumulate) the streams elements into a single value.
You don't need to look any further than the reduce method documentation to find out the exact steps taken:
it provides an example saying it's equivalent to:
boolean foundAny = false;
T result = null;
for (T element : this stream) {
if (!foundAny) {
foundAny = true;
result = element;
}
else
result = accumulator.apply(result, element);
}
return foundAny ? Optional.of(result) : Optional.empty();
where accumulator
is the function you've supplied i.e. (x, y) -> x * 2 + y
6
But only for sequential streams. Since(x, y) -> x * 2 + y
is not an associative function, it’s abusingreduce
for a left-fold (which the Stream API does not offer). Executed in parallel, this solution could produce wrong numbers.
– Holger
Nov 23 '18 at 13:18
@Holger I think that comment would be more appropriate to the OP under their post rather than the code I've shown :).
– Aomine
Nov 23 '18 at 13:53
add a comment |
reduce
essentially enables one to "reduce" (also known as fold, accumulate) the streams elements into a single value.
You don't need to look any further than the reduce method documentation to find out the exact steps taken:
it provides an example saying it's equivalent to:
boolean foundAny = false;
T result = null;
for (T element : this stream) {
if (!foundAny) {
foundAny = true;
result = element;
}
else
result = accumulator.apply(result, element);
}
return foundAny ? Optional.of(result) : Optional.empty();
where accumulator
is the function you've supplied i.e. (x, y) -> x * 2 + y
reduce
essentially enables one to "reduce" (also known as fold, accumulate) the streams elements into a single value.
You don't need to look any further than the reduce method documentation to find out the exact steps taken:
it provides an example saying it's equivalent to:
boolean foundAny = false;
T result = null;
for (T element : this stream) {
if (!foundAny) {
foundAny = true;
result = element;
}
else
result = accumulator.apply(result, element);
}
return foundAny ? Optional.of(result) : Optional.empty();
where accumulator
is the function you've supplied i.e. (x, y) -> x * 2 + y
answered Nov 23 '18 at 13:14


AomineAomine
42.6k74677
42.6k74677
6
But only for sequential streams. Since(x, y) -> x * 2 + y
is not an associative function, it’s abusingreduce
for a left-fold (which the Stream API does not offer). Executed in parallel, this solution could produce wrong numbers.
– Holger
Nov 23 '18 at 13:18
@Holger I think that comment would be more appropriate to the OP under their post rather than the code I've shown :).
– Aomine
Nov 23 '18 at 13:53
add a comment |
6
But only for sequential streams. Since(x, y) -> x * 2 + y
is not an associative function, it’s abusingreduce
for a left-fold (which the Stream API does not offer). Executed in parallel, this solution could produce wrong numbers.
– Holger
Nov 23 '18 at 13:18
@Holger I think that comment would be more appropriate to the OP under their post rather than the code I've shown :).
– Aomine
Nov 23 '18 at 13:53
6
6
But only for sequential streams. Since
(x, y) -> x * 2 + y
is not an associative function, it’s abusing reduce
for a left-fold (which the Stream API does not offer). Executed in parallel, this solution could produce wrong numbers.– Holger
Nov 23 '18 at 13:18
But only for sequential streams. Since
(x, y) -> x * 2 + y
is not an associative function, it’s abusing reduce
for a left-fold (which the Stream API does not offer). Executed in parallel, this solution could produce wrong numbers.– Holger
Nov 23 '18 at 13:18
@Holger I think that comment would be more appropriate to the OP under their post rather than the code I've shown :).
– Aomine
Nov 23 '18 at 13:53
@Holger I think that comment would be more appropriate to the OP under their post rather than the code I've shown :).
– Aomine
Nov 23 '18 at 13:53
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446884%2fsolution-of-the-binary-to-integer-code-through-stream-api-explanation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eGvWt AL,k6g,buYnsZtX,k,d2lVy2FTXeS7,ccncudC50WKCZban75RVdKgH0VM
Only 1 binary number is stored in List ? Or every object of Integer contain a binary number?
– Khalid Shah
Nov 23 '18 at 12:52
only 1 binary number
– Pooja
Nov 23 '18 at 12:53
3
This solution is wrong, it only works for sequential streams and it's violating
Stream.reduce
's contract, which mandates that the function must be associative ((x, y) -> x * 2 + y
clearly isn't).– Federico Peralta Schaffner
Nov 23 '18 at 13:45