Tor Hidden service on 2 ports?
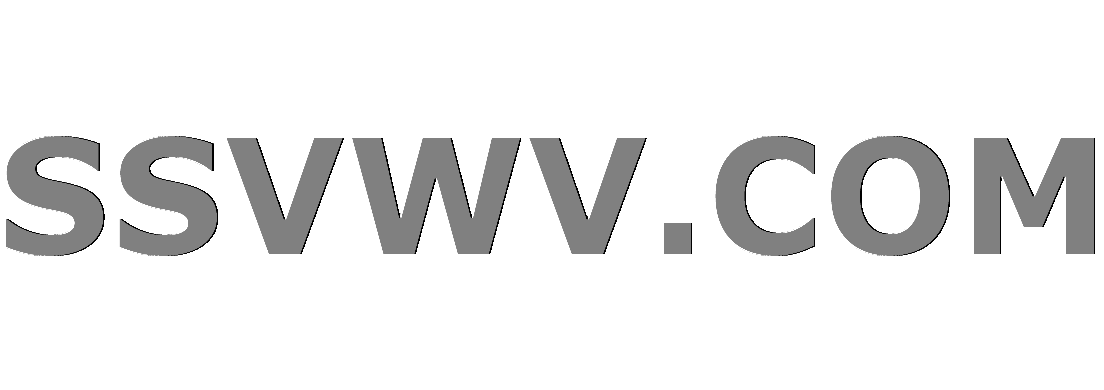
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am doing a p2p app based on Tor network completely. The Application uses 2 ports to communicate, say 8001 for control commands and 7001 for chat channel. The peer first connects to 8001 to request a chat, and when Me (the other peer) accepts his request, I try to connect to him on 7001 (he should be listening and waiting for me to do so). I did so as a form of authentication.
In summary, a single peer listens on 2 ports, one for control(8001) and depending on his side (chat request sender/receiver) he listens on 7001(chat). the negotiation goes as the following ( assuming control channel built on 8001 )
-Me sends CHATREQ to peer then listens on 7001
-Peer sends CHATOK to me and try to connect to my 7001
The problem I can't understand is that in most times, the connection with 7001 fails to connect although the one with 8001 went well !. What can make the connection on one port goes well and on the other fails ? Is there a thing I misunderstood ? Or it is a problem in the mechanism itself ( please I want to get to the problem not to modify the whole application to bypass it ! So, Working on 2 separate ports can't be changed :) )
Here are the relevant code parts
1- Socks5 connection function
public static Socket ConnectViaSocks(ushort Socksport, string RemoteAddress, ushort RemotePort, ref sbyte result)
{
Socket torsock = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
torsock.Connect("127.0.0.1", Socksport);
// Sending intial request : Authentication
byte AuthReq = { 0x05, 0x01, 0x00 };
torsock.Send(AuthReq);
// Getting reply
byte buffer = new byte[2];
int received = torsock.Receive(buffer);
if (buffer[1] != 0x00) { torsock.Close(); throw new MyException("SOCKS5 : Authentication error "); }
// Sending Connect request to a domain (.onion domain)
byte header = { 0x05, 0x01, 0x00, 0x03 };
byte DomainLength =(byte) RemoteAddress.Length;
byte DomainName = Encoding.ASCII.GetBytes(RemoteAddress);
byte ConnRequest = new byte[4+1+DomainLength+2]; //Request format = {0x05 0x01 0x00 0x03 Domainlength(1 byte) DomainNmame(Variable Bytes) portNo(2 bytes) }
System.Buffer.BlockCopy(header , 0, ConnRequest, 0, header.Length);
ConnRequest[header.Length] = DomainLength;
System.Buffer.BlockCopy(DomainName, 0, ConnRequest, header.Length+1, DomainName.Length);
ConnRequest[header.Length + 1 + DomainName.Length ] = (byte)(RemotePort>>8);
ConnRequest[header.Length + 1 + DomainName.Length + 1] = (byte)(RemotePort & 255);
torsock.Send(ConnRequest);
byte buffer2 = new byte[10];
received = torsock.Receive(buffer2);
if (buffer2[1] != 0x00) { torsock.Close(); }
result = (sbyte)buffer2[1];
return torsock;
}
2- Establishing hidden service (AKA: Ephemeral hidden service) command from a private key I have.
string command = "ADD_ONION RSA1024:" + PrivateKey + " Port=7001 Port=8001"; // Creating Onion hidden service on ports 7001,8001
torctrl.sendCommand(command);
NB: torctrl is a wrapper around a socket which sends the command string to tor control port.
3- Chat channel establishment:
3.1 : Initiator peer sends Chat request :
NB. Globals.SKEY = onion address without .onion ,
SockThreads.SockTxThread = thread that sends (message) on (contactSocket) to the peer
cmd.StrCmd = formats the Chat request to unified message format accross the application
Console.WriteLine("[Notice] Sending chat request to " + onion);
message = cmd.StrCmd(Globals.SKEY, Globals.nickname, "CHATREQ", 0, "");
Socket contactSocket = Globals.OnionSocks[onion];
Thread myNewThreadTx = new Thread(() => SockThreads.SockTxThread(contactSocket, message));
myNewThreadTx.Start();
3.2 Responding to CHAT REQ
NB: MsgOnion: Address of peer sent me the chat request
Console.ForegroundColor = System.ConsoleColor.DarkRed;
Console.WriteLine("[Notice] Starting Chat channel creation");
ToPeerMsg = cmd.StrCmd(Globals.SKEY, Globals.nickname, "CHATOK", 0, "");
Thread myNewThreadTx = new Thread(() => SockThreads.SockTxThread(sock, ToPeerMsg));
myNewThreadTx.Start();
Thread.Sleep(1000);
// Connecting to chat port of incoming user
Socket Chatsock = SocketSocks.ConnectViaSocks(9150, MsgOnion + ".onion", 7001, ref result);
if (result == 0)
{ /* Some code to Rx/Tx messages,if come here no problem at all :)*/}
3.3 : Responding to CHAT OK
Thread ChatListenThread = new Thread(() =>SockThreads.SockChatListener());
ChatListenThread.Start();
ChatListenThread: Thread listens on 7001 and if got a connection does the important work to chat(opening threads, controls,..etc). The relevant part to question:
#region Create listener and accept sockets
TcpListener CtrlListener = new TcpListener(System.Net.IPAddress.Any, 7001);
CtrlListener.Start();
#endregion
#region Wait for a connection
Socket sock;
sock = CtrlListener.AcceptSocket();
Console.WriteLine("[Notice] Chat channel established with peer .... Congrats =) ");
c# winforms p2p tor
add a comment |
I am doing a p2p app based on Tor network completely. The Application uses 2 ports to communicate, say 8001 for control commands and 7001 for chat channel. The peer first connects to 8001 to request a chat, and when Me (the other peer) accepts his request, I try to connect to him on 7001 (he should be listening and waiting for me to do so). I did so as a form of authentication.
In summary, a single peer listens on 2 ports, one for control(8001) and depending on his side (chat request sender/receiver) he listens on 7001(chat). the negotiation goes as the following ( assuming control channel built on 8001 )
-Me sends CHATREQ to peer then listens on 7001
-Peer sends CHATOK to me and try to connect to my 7001
The problem I can't understand is that in most times, the connection with 7001 fails to connect although the one with 8001 went well !. What can make the connection on one port goes well and on the other fails ? Is there a thing I misunderstood ? Or it is a problem in the mechanism itself ( please I want to get to the problem not to modify the whole application to bypass it ! So, Working on 2 separate ports can't be changed :) )
Here are the relevant code parts
1- Socks5 connection function
public static Socket ConnectViaSocks(ushort Socksport, string RemoteAddress, ushort RemotePort, ref sbyte result)
{
Socket torsock = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
torsock.Connect("127.0.0.1", Socksport);
// Sending intial request : Authentication
byte AuthReq = { 0x05, 0x01, 0x00 };
torsock.Send(AuthReq);
// Getting reply
byte buffer = new byte[2];
int received = torsock.Receive(buffer);
if (buffer[1] != 0x00) { torsock.Close(); throw new MyException("SOCKS5 : Authentication error "); }
// Sending Connect request to a domain (.onion domain)
byte header = { 0x05, 0x01, 0x00, 0x03 };
byte DomainLength =(byte) RemoteAddress.Length;
byte DomainName = Encoding.ASCII.GetBytes(RemoteAddress);
byte ConnRequest = new byte[4+1+DomainLength+2]; //Request format = {0x05 0x01 0x00 0x03 Domainlength(1 byte) DomainNmame(Variable Bytes) portNo(2 bytes) }
System.Buffer.BlockCopy(header , 0, ConnRequest, 0, header.Length);
ConnRequest[header.Length] = DomainLength;
System.Buffer.BlockCopy(DomainName, 0, ConnRequest, header.Length+1, DomainName.Length);
ConnRequest[header.Length + 1 + DomainName.Length ] = (byte)(RemotePort>>8);
ConnRequest[header.Length + 1 + DomainName.Length + 1] = (byte)(RemotePort & 255);
torsock.Send(ConnRequest);
byte buffer2 = new byte[10];
received = torsock.Receive(buffer2);
if (buffer2[1] != 0x00) { torsock.Close(); }
result = (sbyte)buffer2[1];
return torsock;
}
2- Establishing hidden service (AKA: Ephemeral hidden service) command from a private key I have.
string command = "ADD_ONION RSA1024:" + PrivateKey + " Port=7001 Port=8001"; // Creating Onion hidden service on ports 7001,8001
torctrl.sendCommand(command);
NB: torctrl is a wrapper around a socket which sends the command string to tor control port.
3- Chat channel establishment:
3.1 : Initiator peer sends Chat request :
NB. Globals.SKEY = onion address without .onion ,
SockThreads.SockTxThread = thread that sends (message) on (contactSocket) to the peer
cmd.StrCmd = formats the Chat request to unified message format accross the application
Console.WriteLine("[Notice] Sending chat request to " + onion);
message = cmd.StrCmd(Globals.SKEY, Globals.nickname, "CHATREQ", 0, "");
Socket contactSocket = Globals.OnionSocks[onion];
Thread myNewThreadTx = new Thread(() => SockThreads.SockTxThread(contactSocket, message));
myNewThreadTx.Start();
3.2 Responding to CHAT REQ
NB: MsgOnion: Address of peer sent me the chat request
Console.ForegroundColor = System.ConsoleColor.DarkRed;
Console.WriteLine("[Notice] Starting Chat channel creation");
ToPeerMsg = cmd.StrCmd(Globals.SKEY, Globals.nickname, "CHATOK", 0, "");
Thread myNewThreadTx = new Thread(() => SockThreads.SockTxThread(sock, ToPeerMsg));
myNewThreadTx.Start();
Thread.Sleep(1000);
// Connecting to chat port of incoming user
Socket Chatsock = SocketSocks.ConnectViaSocks(9150, MsgOnion + ".onion", 7001, ref result);
if (result == 0)
{ /* Some code to Rx/Tx messages,if come here no problem at all :)*/}
3.3 : Responding to CHAT OK
Thread ChatListenThread = new Thread(() =>SockThreads.SockChatListener());
ChatListenThread.Start();
ChatListenThread: Thread listens on 7001 and if got a connection does the important work to chat(opening threads, controls,..etc). The relevant part to question:
#region Create listener and accept sockets
TcpListener CtrlListener = new TcpListener(System.Net.IPAddress.Any, 7001);
CtrlListener.Start();
#endregion
#region Wait for a connection
Socket sock;
sock = CtrlListener.AcceptSocket();
Console.WriteLine("[Notice] Chat channel established with peer .... Congrats =) ");
c# winforms p2p tor
add a comment |
I am doing a p2p app based on Tor network completely. The Application uses 2 ports to communicate, say 8001 for control commands and 7001 for chat channel. The peer first connects to 8001 to request a chat, and when Me (the other peer) accepts his request, I try to connect to him on 7001 (he should be listening and waiting for me to do so). I did so as a form of authentication.
In summary, a single peer listens on 2 ports, one for control(8001) and depending on his side (chat request sender/receiver) he listens on 7001(chat). the negotiation goes as the following ( assuming control channel built on 8001 )
-Me sends CHATREQ to peer then listens on 7001
-Peer sends CHATOK to me and try to connect to my 7001
The problem I can't understand is that in most times, the connection with 7001 fails to connect although the one with 8001 went well !. What can make the connection on one port goes well and on the other fails ? Is there a thing I misunderstood ? Or it is a problem in the mechanism itself ( please I want to get to the problem not to modify the whole application to bypass it ! So, Working on 2 separate ports can't be changed :) )
Here are the relevant code parts
1- Socks5 connection function
public static Socket ConnectViaSocks(ushort Socksport, string RemoteAddress, ushort RemotePort, ref sbyte result)
{
Socket torsock = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
torsock.Connect("127.0.0.1", Socksport);
// Sending intial request : Authentication
byte AuthReq = { 0x05, 0x01, 0x00 };
torsock.Send(AuthReq);
// Getting reply
byte buffer = new byte[2];
int received = torsock.Receive(buffer);
if (buffer[1] != 0x00) { torsock.Close(); throw new MyException("SOCKS5 : Authentication error "); }
// Sending Connect request to a domain (.onion domain)
byte header = { 0x05, 0x01, 0x00, 0x03 };
byte DomainLength =(byte) RemoteAddress.Length;
byte DomainName = Encoding.ASCII.GetBytes(RemoteAddress);
byte ConnRequest = new byte[4+1+DomainLength+2]; //Request format = {0x05 0x01 0x00 0x03 Domainlength(1 byte) DomainNmame(Variable Bytes) portNo(2 bytes) }
System.Buffer.BlockCopy(header , 0, ConnRequest, 0, header.Length);
ConnRequest[header.Length] = DomainLength;
System.Buffer.BlockCopy(DomainName, 0, ConnRequest, header.Length+1, DomainName.Length);
ConnRequest[header.Length + 1 + DomainName.Length ] = (byte)(RemotePort>>8);
ConnRequest[header.Length + 1 + DomainName.Length + 1] = (byte)(RemotePort & 255);
torsock.Send(ConnRequest);
byte buffer2 = new byte[10];
received = torsock.Receive(buffer2);
if (buffer2[1] != 0x00) { torsock.Close(); }
result = (sbyte)buffer2[1];
return torsock;
}
2- Establishing hidden service (AKA: Ephemeral hidden service) command from a private key I have.
string command = "ADD_ONION RSA1024:" + PrivateKey + " Port=7001 Port=8001"; // Creating Onion hidden service on ports 7001,8001
torctrl.sendCommand(command);
NB: torctrl is a wrapper around a socket which sends the command string to tor control port.
3- Chat channel establishment:
3.1 : Initiator peer sends Chat request :
NB. Globals.SKEY = onion address without .onion ,
SockThreads.SockTxThread = thread that sends (message) on (contactSocket) to the peer
cmd.StrCmd = formats the Chat request to unified message format accross the application
Console.WriteLine("[Notice] Sending chat request to " + onion);
message = cmd.StrCmd(Globals.SKEY, Globals.nickname, "CHATREQ", 0, "");
Socket contactSocket = Globals.OnionSocks[onion];
Thread myNewThreadTx = new Thread(() => SockThreads.SockTxThread(contactSocket, message));
myNewThreadTx.Start();
3.2 Responding to CHAT REQ
NB: MsgOnion: Address of peer sent me the chat request
Console.ForegroundColor = System.ConsoleColor.DarkRed;
Console.WriteLine("[Notice] Starting Chat channel creation");
ToPeerMsg = cmd.StrCmd(Globals.SKEY, Globals.nickname, "CHATOK", 0, "");
Thread myNewThreadTx = new Thread(() => SockThreads.SockTxThread(sock, ToPeerMsg));
myNewThreadTx.Start();
Thread.Sleep(1000);
// Connecting to chat port of incoming user
Socket Chatsock = SocketSocks.ConnectViaSocks(9150, MsgOnion + ".onion", 7001, ref result);
if (result == 0)
{ /* Some code to Rx/Tx messages,if come here no problem at all :)*/}
3.3 : Responding to CHAT OK
Thread ChatListenThread = new Thread(() =>SockThreads.SockChatListener());
ChatListenThread.Start();
ChatListenThread: Thread listens on 7001 and if got a connection does the important work to chat(opening threads, controls,..etc). The relevant part to question:
#region Create listener and accept sockets
TcpListener CtrlListener = new TcpListener(System.Net.IPAddress.Any, 7001);
CtrlListener.Start();
#endregion
#region Wait for a connection
Socket sock;
sock = CtrlListener.AcceptSocket();
Console.WriteLine("[Notice] Chat channel established with peer .... Congrats =) ");
c# winforms p2p tor
I am doing a p2p app based on Tor network completely. The Application uses 2 ports to communicate, say 8001 for control commands and 7001 for chat channel. The peer first connects to 8001 to request a chat, and when Me (the other peer) accepts his request, I try to connect to him on 7001 (he should be listening and waiting for me to do so). I did so as a form of authentication.
In summary, a single peer listens on 2 ports, one for control(8001) and depending on his side (chat request sender/receiver) he listens on 7001(chat). the negotiation goes as the following ( assuming control channel built on 8001 )
-Me sends CHATREQ to peer then listens on 7001
-Peer sends CHATOK to me and try to connect to my 7001
The problem I can't understand is that in most times, the connection with 7001 fails to connect although the one with 8001 went well !. What can make the connection on one port goes well and on the other fails ? Is there a thing I misunderstood ? Or it is a problem in the mechanism itself ( please I want to get to the problem not to modify the whole application to bypass it ! So, Working on 2 separate ports can't be changed :) )
Here are the relevant code parts
1- Socks5 connection function
public static Socket ConnectViaSocks(ushort Socksport, string RemoteAddress, ushort RemotePort, ref sbyte result)
{
Socket torsock = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
torsock.Connect("127.0.0.1", Socksport);
// Sending intial request : Authentication
byte AuthReq = { 0x05, 0x01, 0x00 };
torsock.Send(AuthReq);
// Getting reply
byte buffer = new byte[2];
int received = torsock.Receive(buffer);
if (buffer[1] != 0x00) { torsock.Close(); throw new MyException("SOCKS5 : Authentication error "); }
// Sending Connect request to a domain (.onion domain)
byte header = { 0x05, 0x01, 0x00, 0x03 };
byte DomainLength =(byte) RemoteAddress.Length;
byte DomainName = Encoding.ASCII.GetBytes(RemoteAddress);
byte ConnRequest = new byte[4+1+DomainLength+2]; //Request format = {0x05 0x01 0x00 0x03 Domainlength(1 byte) DomainNmame(Variable Bytes) portNo(2 bytes) }
System.Buffer.BlockCopy(header , 0, ConnRequest, 0, header.Length);
ConnRequest[header.Length] = DomainLength;
System.Buffer.BlockCopy(DomainName, 0, ConnRequest, header.Length+1, DomainName.Length);
ConnRequest[header.Length + 1 + DomainName.Length ] = (byte)(RemotePort>>8);
ConnRequest[header.Length + 1 + DomainName.Length + 1] = (byte)(RemotePort & 255);
torsock.Send(ConnRequest);
byte buffer2 = new byte[10];
received = torsock.Receive(buffer2);
if (buffer2[1] != 0x00) { torsock.Close(); }
result = (sbyte)buffer2[1];
return torsock;
}
2- Establishing hidden service (AKA: Ephemeral hidden service) command from a private key I have.
string command = "ADD_ONION RSA1024:" + PrivateKey + " Port=7001 Port=8001"; // Creating Onion hidden service on ports 7001,8001
torctrl.sendCommand(command);
NB: torctrl is a wrapper around a socket which sends the command string to tor control port.
3- Chat channel establishment:
3.1 : Initiator peer sends Chat request :
NB. Globals.SKEY = onion address without .onion ,
SockThreads.SockTxThread = thread that sends (message) on (contactSocket) to the peer
cmd.StrCmd = formats the Chat request to unified message format accross the application
Console.WriteLine("[Notice] Sending chat request to " + onion);
message = cmd.StrCmd(Globals.SKEY, Globals.nickname, "CHATREQ", 0, "");
Socket contactSocket = Globals.OnionSocks[onion];
Thread myNewThreadTx = new Thread(() => SockThreads.SockTxThread(contactSocket, message));
myNewThreadTx.Start();
3.2 Responding to CHAT REQ
NB: MsgOnion: Address of peer sent me the chat request
Console.ForegroundColor = System.ConsoleColor.DarkRed;
Console.WriteLine("[Notice] Starting Chat channel creation");
ToPeerMsg = cmd.StrCmd(Globals.SKEY, Globals.nickname, "CHATOK", 0, "");
Thread myNewThreadTx = new Thread(() => SockThreads.SockTxThread(sock, ToPeerMsg));
myNewThreadTx.Start();
Thread.Sleep(1000);
// Connecting to chat port of incoming user
Socket Chatsock = SocketSocks.ConnectViaSocks(9150, MsgOnion + ".onion", 7001, ref result);
if (result == 0)
{ /* Some code to Rx/Tx messages,if come here no problem at all :)*/}
3.3 : Responding to CHAT OK
Thread ChatListenThread = new Thread(() =>SockThreads.SockChatListener());
ChatListenThread.Start();
ChatListenThread: Thread listens on 7001 and if got a connection does the important work to chat(opening threads, controls,..etc). The relevant part to question:
#region Create listener and accept sockets
TcpListener CtrlListener = new TcpListener(System.Net.IPAddress.Any, 7001);
CtrlListener.Start();
#endregion
#region Wait for a connection
Socket sock;
sock = CtrlListener.AcceptSocket();
Console.WriteLine("[Notice] Chat channel established with peer .... Congrats =) ");
c# winforms p2p tor
c# winforms p2p tor
asked Nov 23 '18 at 20:31
CodeLOLCodeLOL
164
164
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53452635%2ftor-hidden-service-on-2-ports%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53452635%2ftor-hidden-service-on-2-ports%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YxJ6 qdX,7X8C0u,FmqCOSPTV,wLxn y 6H0FXBkaMMl8x8eyKVdTK0ti3LRbb9,L,dxJrYHj5nL