Handling apollo-server mutation error with apollo-client
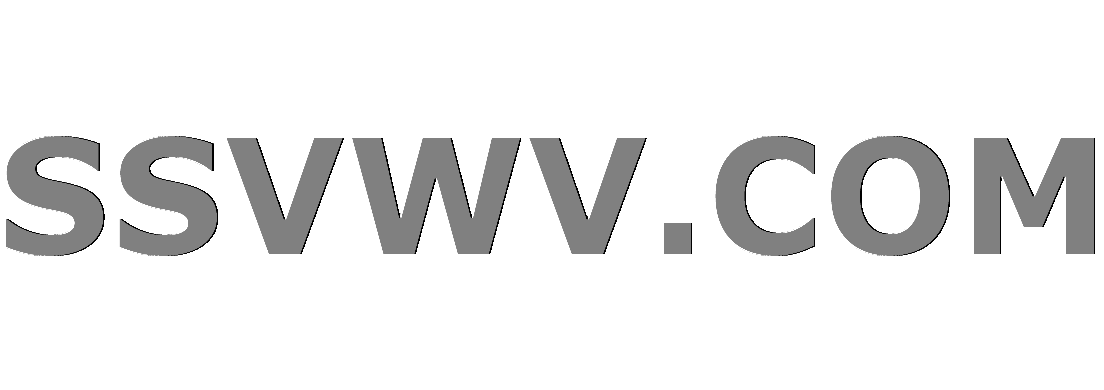
Multi tool use
up vote
0
down vote
favorite
I'm trying to solve how is the best way to create an error response on apollo-server side and handle it in appropriate way on client (apollo).
I was googling a lot but didn't get satisfactory answer.
So here is my actual implementation:
I created an interface and then new type MutationResponse (This is my own implementation of mutation response below).
interface IMutationResponse {
code: Int!
path: String!
fields: String!
message: String!
success: Boolean!
typeError: String!
}
type MutationResponse implements IMutationResponse {
code: Int!
path: String!
fields: String!
message: String!
success: Boolean!
typeError: String!
}
I created another type where Im using MutationResponse:
type CustomerResponse {
customer: Customer
photoFile: File
serverResponse: [MutationResponse]
}
This is my simplified implementation of mutation (createCustomer):
Mutation: {
createCustomer: async (_, { photoFile, customer }) => {
try {
const {
dataValues: { customerID, firstname, lastname, email, phone }
} = await models.Customer.create(customer);
const customerUniqueDir = path.join(customerPath,
`${customerID}`);
} catch (createCustomerError) {
console.log("createCustomerError", createCustomerError);
const serverResponse = createValidationErrorResponse(
400,
createCustomerError
);
// throw new ApolloError({ serverResponse });
return {
serverResponse
};
}
},
creating mutation response on apollo-server:
- First scenario
**If I'm in the catch block, server return serverResponse object **
...
catch(customerPhotoError) {
const serverResponse = createValidationErrorResponse(
400,
createCustomerError
);
return {
serverResponse
};
}
but on the apollo client I fall into the try block so it looks like that server didn't throw any exception and response was successful (200).
There is an option to parse the response and handle it in appropriate way (f.e. according to received status code in response).
Is this right solution ? It's good way how to thrown error on server and it's normal that you are handling this error in try block (or in then callback if you are not using async await) on client ?
- scenario
Apollo server 2 introduced error handling: https://www.apollographql.com/docs/apollo-server/features/errors.html
Okey nice ! As is written in article (posted above) apollo provide rich error feedback in response (errors:)
but
when I'm in catch block in my mutation and I throw on of provided apollo-error let say :
import { ApolloError } from "apollo-server";
.....
catch(createCustomerError) {
throw new ApolloError(serverResponse);
}
Mutation response is part of the array errors: provided by apollo and finally I fell into the catch block on client side but inside of the block I get Cannot read property 'data' of undefined. So I'm not able to access to data in catch block.
here is the response:
Is there any solution how I could solve it ?
Thanks for any help.
reactjs apollo-client apollo-server
add a comment |
up vote
0
down vote
favorite
I'm trying to solve how is the best way to create an error response on apollo-server side and handle it in appropriate way on client (apollo).
I was googling a lot but didn't get satisfactory answer.
So here is my actual implementation:
I created an interface and then new type MutationResponse (This is my own implementation of mutation response below).
interface IMutationResponse {
code: Int!
path: String!
fields: String!
message: String!
success: Boolean!
typeError: String!
}
type MutationResponse implements IMutationResponse {
code: Int!
path: String!
fields: String!
message: String!
success: Boolean!
typeError: String!
}
I created another type where Im using MutationResponse:
type CustomerResponse {
customer: Customer
photoFile: File
serverResponse: [MutationResponse]
}
This is my simplified implementation of mutation (createCustomer):
Mutation: {
createCustomer: async (_, { photoFile, customer }) => {
try {
const {
dataValues: { customerID, firstname, lastname, email, phone }
} = await models.Customer.create(customer);
const customerUniqueDir = path.join(customerPath,
`${customerID}`);
} catch (createCustomerError) {
console.log("createCustomerError", createCustomerError);
const serverResponse = createValidationErrorResponse(
400,
createCustomerError
);
// throw new ApolloError({ serverResponse });
return {
serverResponse
};
}
},
creating mutation response on apollo-server:
- First scenario
**If I'm in the catch block, server return serverResponse object **
...
catch(customerPhotoError) {
const serverResponse = createValidationErrorResponse(
400,
createCustomerError
);
return {
serverResponse
};
}
but on the apollo client I fall into the try block so it looks like that server didn't throw any exception and response was successful (200).
There is an option to parse the response and handle it in appropriate way (f.e. according to received status code in response).
Is this right solution ? It's good way how to thrown error on server and it's normal that you are handling this error in try block (or in then callback if you are not using async await) on client ?
- scenario
Apollo server 2 introduced error handling: https://www.apollographql.com/docs/apollo-server/features/errors.html
Okey nice ! As is written in article (posted above) apollo provide rich error feedback in response (errors:)
but
when I'm in catch block in my mutation and I throw on of provided apollo-error let say :
import { ApolloError } from "apollo-server";
.....
catch(createCustomerError) {
throw new ApolloError(serverResponse);
}
Mutation response is part of the array errors: provided by apollo and finally I fell into the catch block on client side but inside of the block I get Cannot read property 'data' of undefined. So I'm not able to access to data in catch block.
here is the response:
Is there any solution how I could solve it ?
Thanks for any help.
reactjs apollo-client apollo-server
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to solve how is the best way to create an error response on apollo-server side and handle it in appropriate way on client (apollo).
I was googling a lot but didn't get satisfactory answer.
So here is my actual implementation:
I created an interface and then new type MutationResponse (This is my own implementation of mutation response below).
interface IMutationResponse {
code: Int!
path: String!
fields: String!
message: String!
success: Boolean!
typeError: String!
}
type MutationResponse implements IMutationResponse {
code: Int!
path: String!
fields: String!
message: String!
success: Boolean!
typeError: String!
}
I created another type where Im using MutationResponse:
type CustomerResponse {
customer: Customer
photoFile: File
serverResponse: [MutationResponse]
}
This is my simplified implementation of mutation (createCustomer):
Mutation: {
createCustomer: async (_, { photoFile, customer }) => {
try {
const {
dataValues: { customerID, firstname, lastname, email, phone }
} = await models.Customer.create(customer);
const customerUniqueDir = path.join(customerPath,
`${customerID}`);
} catch (createCustomerError) {
console.log("createCustomerError", createCustomerError);
const serverResponse = createValidationErrorResponse(
400,
createCustomerError
);
// throw new ApolloError({ serverResponse });
return {
serverResponse
};
}
},
creating mutation response on apollo-server:
- First scenario
**If I'm in the catch block, server return serverResponse object **
...
catch(customerPhotoError) {
const serverResponse = createValidationErrorResponse(
400,
createCustomerError
);
return {
serverResponse
};
}
but on the apollo client I fall into the try block so it looks like that server didn't throw any exception and response was successful (200).
There is an option to parse the response and handle it in appropriate way (f.e. according to received status code in response).
Is this right solution ? It's good way how to thrown error on server and it's normal that you are handling this error in try block (or in then callback if you are not using async await) on client ?
- scenario
Apollo server 2 introduced error handling: https://www.apollographql.com/docs/apollo-server/features/errors.html
Okey nice ! As is written in article (posted above) apollo provide rich error feedback in response (errors:)
but
when I'm in catch block in my mutation and I throw on of provided apollo-error let say :
import { ApolloError } from "apollo-server";
.....
catch(createCustomerError) {
throw new ApolloError(serverResponse);
}
Mutation response is part of the array errors: provided by apollo and finally I fell into the catch block on client side but inside of the block I get Cannot read property 'data' of undefined. So I'm not able to access to data in catch block.
here is the response:
Is there any solution how I could solve it ?
Thanks for any help.
reactjs apollo-client apollo-server
I'm trying to solve how is the best way to create an error response on apollo-server side and handle it in appropriate way on client (apollo).
I was googling a lot but didn't get satisfactory answer.
So here is my actual implementation:
I created an interface and then new type MutationResponse (This is my own implementation of mutation response below).
interface IMutationResponse {
code: Int!
path: String!
fields: String!
message: String!
success: Boolean!
typeError: String!
}
type MutationResponse implements IMutationResponse {
code: Int!
path: String!
fields: String!
message: String!
success: Boolean!
typeError: String!
}
I created another type where Im using MutationResponse:
type CustomerResponse {
customer: Customer
photoFile: File
serverResponse: [MutationResponse]
}
This is my simplified implementation of mutation (createCustomer):
Mutation: {
createCustomer: async (_, { photoFile, customer }) => {
try {
const {
dataValues: { customerID, firstname, lastname, email, phone }
} = await models.Customer.create(customer);
const customerUniqueDir = path.join(customerPath,
`${customerID}`);
} catch (createCustomerError) {
console.log("createCustomerError", createCustomerError);
const serverResponse = createValidationErrorResponse(
400,
createCustomerError
);
// throw new ApolloError({ serverResponse });
return {
serverResponse
};
}
},
creating mutation response on apollo-server:
- First scenario
**If I'm in the catch block, server return serverResponse object **
...
catch(customerPhotoError) {
const serverResponse = createValidationErrorResponse(
400,
createCustomerError
);
return {
serverResponse
};
}
but on the apollo client I fall into the try block so it looks like that server didn't throw any exception and response was successful (200).
There is an option to parse the response and handle it in appropriate way (f.e. according to received status code in response).
Is this right solution ? It's good way how to thrown error on server and it's normal that you are handling this error in try block (or in then callback if you are not using async await) on client ?
- scenario
Apollo server 2 introduced error handling: https://www.apollographql.com/docs/apollo-server/features/errors.html
Okey nice ! As is written in article (posted above) apollo provide rich error feedback in response (errors:)
but
when I'm in catch block in my mutation and I throw on of provided apollo-error let say :
import { ApolloError } from "apollo-server";
.....
catch(createCustomerError) {
throw new ApolloError(serverResponse);
}
Mutation response is part of the array errors: provided by apollo and finally I fell into the catch block on client side but inside of the block I get Cannot read property 'data' of undefined. So I'm not able to access to data in catch block.
here is the response:
Is there any solution how I could solve it ?
Thanks for any help.
reactjs apollo-client apollo-server
reactjs apollo-client apollo-server
asked yesterday
Morty
53211
53211
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53343707%2fhandling-apollo-server-mutation-error-with-apollo-client%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sn,RnA vHKV6j3Gx,5UyTYx2dW,7bRSPLMw4bUhrd8B1,Le boOxDcr5RR7rc