How to assert that a constexpr if else clause never happen?
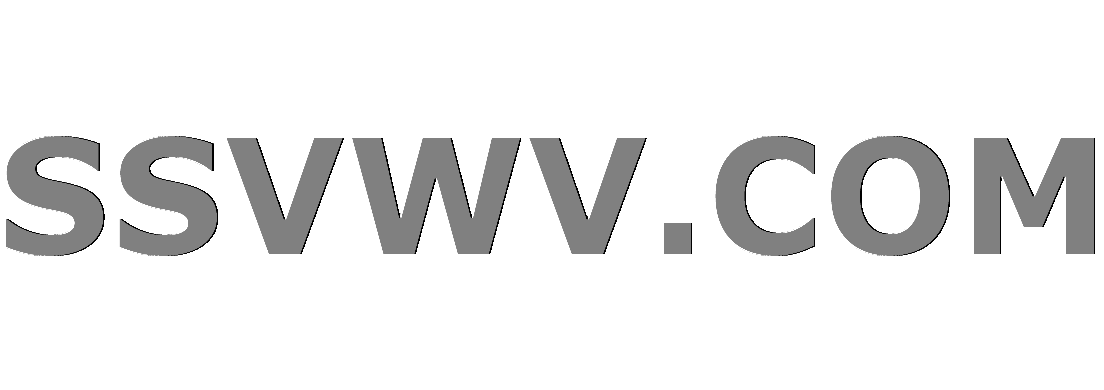
Multi tool use
I want to raise a compile time error when non of the constexpr if conditions is true eg:
if constexpr(condition1){
...
} else if constexpr (condition2) {
....
} else if constexpr (condition3) {
....
} else {
// I want the else clause never taken. But I heard the code below is not allowed
static_assert(false);
}
// I'd rather not repeat the conditions again like this:
static_assert(condition1 || condition2 || condition3);
c++ c++17
add a comment |
I want to raise a compile time error when non of the constexpr if conditions is true eg:
if constexpr(condition1){
...
} else if constexpr (condition2) {
....
} else if constexpr (condition3) {
....
} else {
// I want the else clause never taken. But I heard the code below is not allowed
static_assert(false);
}
// I'd rather not repeat the conditions again like this:
static_assert(condition1 || condition2 || condition3);
c++ c++17
add a comment |
I want to raise a compile time error when non of the constexpr if conditions is true eg:
if constexpr(condition1){
...
} else if constexpr (condition2) {
....
} else if constexpr (condition3) {
....
} else {
// I want the else clause never taken. But I heard the code below is not allowed
static_assert(false);
}
// I'd rather not repeat the conditions again like this:
static_assert(condition1 || condition2 || condition3);
c++ c++17
I want to raise a compile time error when non of the constexpr if conditions is true eg:
if constexpr(condition1){
...
} else if constexpr (condition2) {
....
} else if constexpr (condition3) {
....
} else {
// I want the else clause never taken. But I heard the code below is not allowed
static_assert(false);
}
// I'd rather not repeat the conditions again like this:
static_assert(condition1 || condition2 || condition3);
c++ c++17
c++ c++17
asked 2 days ago
John Smith
651114
651114
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
You have to make the discarded statement dependent of the template parameters
template <class...> constexpr std::false_type always_false{};
if constexpr(condition1){
...
} else if constexpr (condition2) {
....
} else if constexpr (condition3) {
....
} else {
static_assert(always_false<T>);
}
This is so because
[temp.res]/8 - The program is ill-formed, no diagnostic required, if
no valid specialization can be generated for a template or a substatement of a
constexpr if
statement within a template and the template is not instantiated, or ...
add a comment |
Here's a workaround from cppreference.com, i.e. use a type-dependent expression instead.
Note: the discarded statement can't be ill-formed for every possible specialization:
The common workaround for such a catch-all statement is a type-dependent expression that is always false:
e.g.
template<class T> struct dependent_false : std::false_type {};
then
static_assert(dependent_false<T>::value);
1
Ironic really since that's exactly what this gives us! Silly language.
– Lightness Races in Orbit
2 days ago
add a comment |
taking a slightly different tack...
#include <ciso646>
template<auto x> void something();
template<class...Conditions>
constexpr int which(Conditions... cond)
{
int sel = 0;
bool found = false;
auto elect = [&found, &sel](auto cond)
{
if (not found)
{
if (cond)
{
found = true;
}
else
{
++sel;
}
}
};
(elect(cond), ...);
if (not found) throw "you have a logic error";
return sel;
}
template<bool condition1, bool condition2, bool condition3>
void foo()
{
auto constexpr sel = which(condition1, condition2, condition3);
switch(sel)
{
case 0:
something<1>();
break;
case 1:
something<2>();
break;
case 2:
something<3>();
break;
}
}
int main()
{
foo<false, true, false>();
// foo<false, false, false>(); // fails to compile
}
As I understand it, which
is evaluated in constexpr context, which means that it is legal unless the program has to follow a code path that is illegal in a constexpr context.
For all expected cases, the throw
path is not taken, so the function is legal. When illegal inputs are provided, we go down the ill-formed path, which causes a compiler error.
I'd be interested to know whether this solution is strictly correct from a language-lawyer perspective.
It works on gcc, clang and MSVC.
...or for fans of obfuscated code...
template<class...Conditions>
constexpr int which(Conditions... cond)
{
auto sel = 0;
((cond or (++sel, false)) or ...) or (throw "program is ill-formed", false);
return sel;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53945490%2fhow-to-assert-that-a-constexpr-if-else-clause-never-happen%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You have to make the discarded statement dependent of the template parameters
template <class...> constexpr std::false_type always_false{};
if constexpr(condition1){
...
} else if constexpr (condition2) {
....
} else if constexpr (condition3) {
....
} else {
static_assert(always_false<T>);
}
This is so because
[temp.res]/8 - The program is ill-formed, no diagnostic required, if
no valid specialization can be generated for a template or a substatement of a
constexpr if
statement within a template and the template is not instantiated, or ...
add a comment |
You have to make the discarded statement dependent of the template parameters
template <class...> constexpr std::false_type always_false{};
if constexpr(condition1){
...
} else if constexpr (condition2) {
....
} else if constexpr (condition3) {
....
} else {
static_assert(always_false<T>);
}
This is so because
[temp.res]/8 - The program is ill-formed, no diagnostic required, if
no valid specialization can be generated for a template or a substatement of a
constexpr if
statement within a template and the template is not instantiated, or ...
add a comment |
You have to make the discarded statement dependent of the template parameters
template <class...> constexpr std::false_type always_false{};
if constexpr(condition1){
...
} else if constexpr (condition2) {
....
} else if constexpr (condition3) {
....
} else {
static_assert(always_false<T>);
}
This is so because
[temp.res]/8 - The program is ill-formed, no diagnostic required, if
no valid specialization can be generated for a template or a substatement of a
constexpr if
statement within a template and the template is not instantiated, or ...
You have to make the discarded statement dependent of the template parameters
template <class...> constexpr std::false_type always_false{};
if constexpr(condition1){
...
} else if constexpr (condition2) {
....
} else if constexpr (condition3) {
....
} else {
static_assert(always_false<T>);
}
This is so because
[temp.res]/8 - The program is ill-formed, no diagnostic required, if
no valid specialization can be generated for a template or a substatement of a
constexpr if
statement within a template and the template is not instantiated, or ...
edited 2 days ago
answered 2 days ago
Jans
8,06522535
8,06522535
add a comment |
add a comment |
Here's a workaround from cppreference.com, i.e. use a type-dependent expression instead.
Note: the discarded statement can't be ill-formed for every possible specialization:
The common workaround for such a catch-all statement is a type-dependent expression that is always false:
e.g.
template<class T> struct dependent_false : std::false_type {};
then
static_assert(dependent_false<T>::value);
1
Ironic really since that's exactly what this gives us! Silly language.
– Lightness Races in Orbit
2 days ago
add a comment |
Here's a workaround from cppreference.com, i.e. use a type-dependent expression instead.
Note: the discarded statement can't be ill-formed for every possible specialization:
The common workaround for such a catch-all statement is a type-dependent expression that is always false:
e.g.
template<class T> struct dependent_false : std::false_type {};
then
static_assert(dependent_false<T>::value);
1
Ironic really since that's exactly what this gives us! Silly language.
– Lightness Races in Orbit
2 days ago
add a comment |
Here's a workaround from cppreference.com, i.e. use a type-dependent expression instead.
Note: the discarded statement can't be ill-formed for every possible specialization:
The common workaround for such a catch-all statement is a type-dependent expression that is always false:
e.g.
template<class T> struct dependent_false : std::false_type {};
then
static_assert(dependent_false<T>::value);
Here's a workaround from cppreference.com, i.e. use a type-dependent expression instead.
Note: the discarded statement can't be ill-formed for every possible specialization:
The common workaround for such a catch-all statement is a type-dependent expression that is always false:
e.g.
template<class T> struct dependent_false : std::false_type {};
then
static_assert(dependent_false<T>::value);
answered 2 days ago
songyuanyao
89.8k11170234
89.8k11170234
1
Ironic really since that's exactly what this gives us! Silly language.
– Lightness Races in Orbit
2 days ago
add a comment |
1
Ironic really since that's exactly what this gives us! Silly language.
– Lightness Races in Orbit
2 days ago
1
1
Ironic really since that's exactly what this gives us! Silly language.
– Lightness Races in Orbit
2 days ago
Ironic really since that's exactly what this gives us! Silly language.
– Lightness Races in Orbit
2 days ago
add a comment |
taking a slightly different tack...
#include <ciso646>
template<auto x> void something();
template<class...Conditions>
constexpr int which(Conditions... cond)
{
int sel = 0;
bool found = false;
auto elect = [&found, &sel](auto cond)
{
if (not found)
{
if (cond)
{
found = true;
}
else
{
++sel;
}
}
};
(elect(cond), ...);
if (not found) throw "you have a logic error";
return sel;
}
template<bool condition1, bool condition2, bool condition3>
void foo()
{
auto constexpr sel = which(condition1, condition2, condition3);
switch(sel)
{
case 0:
something<1>();
break;
case 1:
something<2>();
break;
case 2:
something<3>();
break;
}
}
int main()
{
foo<false, true, false>();
// foo<false, false, false>(); // fails to compile
}
As I understand it, which
is evaluated in constexpr context, which means that it is legal unless the program has to follow a code path that is illegal in a constexpr context.
For all expected cases, the throw
path is not taken, so the function is legal. When illegal inputs are provided, we go down the ill-formed path, which causes a compiler error.
I'd be interested to know whether this solution is strictly correct from a language-lawyer perspective.
It works on gcc, clang and MSVC.
...or for fans of obfuscated code...
template<class...Conditions>
constexpr int which(Conditions... cond)
{
auto sel = 0;
((cond or (++sel, false)) or ...) or (throw "program is ill-formed", false);
return sel;
}
add a comment |
taking a slightly different tack...
#include <ciso646>
template<auto x> void something();
template<class...Conditions>
constexpr int which(Conditions... cond)
{
int sel = 0;
bool found = false;
auto elect = [&found, &sel](auto cond)
{
if (not found)
{
if (cond)
{
found = true;
}
else
{
++sel;
}
}
};
(elect(cond), ...);
if (not found) throw "you have a logic error";
return sel;
}
template<bool condition1, bool condition2, bool condition3>
void foo()
{
auto constexpr sel = which(condition1, condition2, condition3);
switch(sel)
{
case 0:
something<1>();
break;
case 1:
something<2>();
break;
case 2:
something<3>();
break;
}
}
int main()
{
foo<false, true, false>();
// foo<false, false, false>(); // fails to compile
}
As I understand it, which
is evaluated in constexpr context, which means that it is legal unless the program has to follow a code path that is illegal in a constexpr context.
For all expected cases, the throw
path is not taken, so the function is legal. When illegal inputs are provided, we go down the ill-formed path, which causes a compiler error.
I'd be interested to know whether this solution is strictly correct from a language-lawyer perspective.
It works on gcc, clang and MSVC.
...or for fans of obfuscated code...
template<class...Conditions>
constexpr int which(Conditions... cond)
{
auto sel = 0;
((cond or (++sel, false)) or ...) or (throw "program is ill-formed", false);
return sel;
}
add a comment |
taking a slightly different tack...
#include <ciso646>
template<auto x> void something();
template<class...Conditions>
constexpr int which(Conditions... cond)
{
int sel = 0;
bool found = false;
auto elect = [&found, &sel](auto cond)
{
if (not found)
{
if (cond)
{
found = true;
}
else
{
++sel;
}
}
};
(elect(cond), ...);
if (not found) throw "you have a logic error";
return sel;
}
template<bool condition1, bool condition2, bool condition3>
void foo()
{
auto constexpr sel = which(condition1, condition2, condition3);
switch(sel)
{
case 0:
something<1>();
break;
case 1:
something<2>();
break;
case 2:
something<3>();
break;
}
}
int main()
{
foo<false, true, false>();
// foo<false, false, false>(); // fails to compile
}
As I understand it, which
is evaluated in constexpr context, which means that it is legal unless the program has to follow a code path that is illegal in a constexpr context.
For all expected cases, the throw
path is not taken, so the function is legal. When illegal inputs are provided, we go down the ill-formed path, which causes a compiler error.
I'd be interested to know whether this solution is strictly correct from a language-lawyer perspective.
It works on gcc, clang and MSVC.
...or for fans of obfuscated code...
template<class...Conditions>
constexpr int which(Conditions... cond)
{
auto sel = 0;
((cond or (++sel, false)) or ...) or (throw "program is ill-formed", false);
return sel;
}
taking a slightly different tack...
#include <ciso646>
template<auto x> void something();
template<class...Conditions>
constexpr int which(Conditions... cond)
{
int sel = 0;
bool found = false;
auto elect = [&found, &sel](auto cond)
{
if (not found)
{
if (cond)
{
found = true;
}
else
{
++sel;
}
}
};
(elect(cond), ...);
if (not found) throw "you have a logic error";
return sel;
}
template<bool condition1, bool condition2, bool condition3>
void foo()
{
auto constexpr sel = which(condition1, condition2, condition3);
switch(sel)
{
case 0:
something<1>();
break;
case 1:
something<2>();
break;
case 2:
something<3>();
break;
}
}
int main()
{
foo<false, true, false>();
// foo<false, false, false>(); // fails to compile
}
As I understand it, which
is evaluated in constexpr context, which means that it is legal unless the program has to follow a code path that is illegal in a constexpr context.
For all expected cases, the throw
path is not taken, so the function is legal. When illegal inputs are provided, we go down the ill-formed path, which causes a compiler error.
I'd be interested to know whether this solution is strictly correct from a language-lawyer perspective.
It works on gcc, clang and MSVC.
...or for fans of obfuscated code...
template<class...Conditions>
constexpr int which(Conditions... cond)
{
auto sel = 0;
((cond or (++sel, false)) or ...) or (throw "program is ill-formed", false);
return sel;
}
edited 2 days ago
answered 2 days ago
Richard Hodges
55.4k657100
55.4k657100
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53945490%2fhow-to-assert-that-a-constexpr-if-else-clause-never-happen%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ED0MmeofL7Iu46hsxKNn XWI0XmJCuqcL1YPPsWs,WZqLXsHMhQuk0gnPCN4Pfp4dDB