Strange behavior of LocationRequest priorities
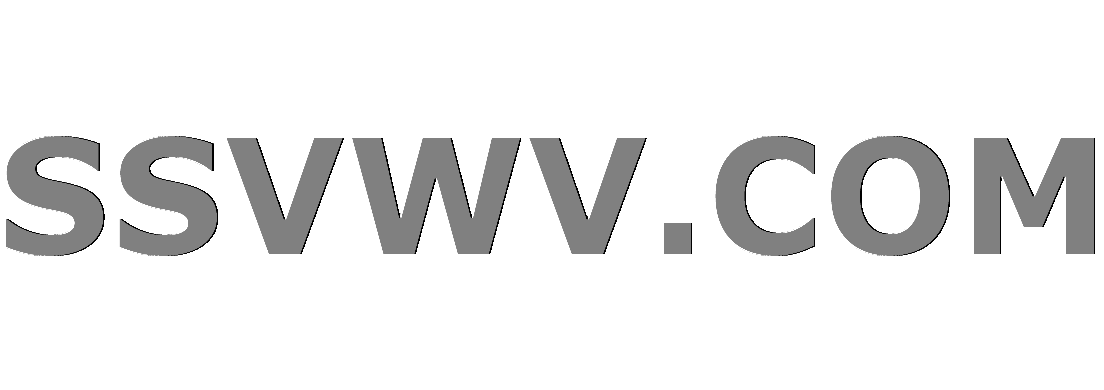
Multi tool use
So I have an app. It depends on user location. On lot of devices it worked fine, as I expect. There are problem with samsung s8, I checked 4 real s8 from different users and with different app that needs location, and the problem there.
But now my xiaomi mi a1 (with clean android) get android security update (8.1 November) and I get same problem as on s8.
The problem:
I turn off location service in settings of device. I start my app, or another app that need location (not google maps, it works awesome).
I get default system dialog to turn on location services. I press OK, and one time it start location service with all sources and another time it starts gps only. The "GPS only" works very bad, I wait one - two minutes and it not give my position and not set blue dot on map. I turn off location and close app, open and again this dialog, and after "ok" it start all sources, and I get location instantly.
As I understand, the mode that will be set on start location service depends on priority of LocationRequest. So why once it set mode "all sources" and once "gps only", its about LocationRequest.PRIORITY_HIGH_ACCURACY
. If I try to use LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY
(its my default for s8) on all devices that I checked, it will start mode "wifi and other networks", but all time the same mode.
I use FusedLocationClient.
My code to start system dialog:
LocationSettingsRequest.Builder builder = new LocationSettingsRequest.Builder()
.addLocationRequest(getLocationRequest());
builder.setAlwaysShow(true);
SettingsClient client = LocationServices.getSettingsClient(this);
Task<LocationSettingsResponse> task = client.checkLocationSettings(builder.build());
task.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(@NonNull Exception e) {
if (e instanceof ResolvableApiException) {
try {
// Show the dialog by calling startResolutionForResult(),
// and check the result in onActivityResult().
ResolvableApiException resolvable = (ResolvableApiException) e;
resolvable.startResolutionForResult(MapsActivity.this,
REQUEST_CHECK_SETTINGS);
} catch (IntentSender.SendIntentException sendEx) {
// Ignore the error.
if (sendEx.getMessage() != null) {
Crashlytics.logException(new RuntimeException("startGpsService: " + sendEx.getMessage()));
}
}
}
}
});
And my LocationRequest:
if (mLocationRequest == null) {
mLocationRequest = new LocationRequest();
if (Build.MANUFACTURER.toLowerCase().contains("samsung") &&
Build.MODEL.toLowerCase().contains("sm-g95")) {
mLocationRequest.setPriority(LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY);
} else {
mLocationRequest.setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY);
}
mLocationRequest.setInterval(2000);
mLocationRequest.setFastestInterval(1000);
}
return mLocationRequest;
I'll be glad to get any suggestions.
Update, I make some tests, this is the log:
2018-11-20 09:56:11.055 29850-29850 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:56:29.554 30111-30111 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:56:48.847 30309-30309 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:57:30.645 30647-30647 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:57:58.354 30908-30908 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:58:16.632 31159-31159 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:59:57.753 1665-1665 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:14.176 2200-2200 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:30.406 2963-2963 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:45.215 3398-3398 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
All the time when I get "location providers not enabled" it comes to onActivityResult
with (requestCode == REQUEST_CHECK_SETTINGS && resultCode == RESULT_CANCELED)
and turn on "Device only" mode.
Its looks like it depends on place where exactly was my finger on "Yes" button.
Additional question: If I can make custom view for system "turn on location" dialog ?

add a comment |
So I have an app. It depends on user location. On lot of devices it worked fine, as I expect. There are problem with samsung s8, I checked 4 real s8 from different users and with different app that needs location, and the problem there.
But now my xiaomi mi a1 (with clean android) get android security update (8.1 November) and I get same problem as on s8.
The problem:
I turn off location service in settings of device. I start my app, or another app that need location (not google maps, it works awesome).
I get default system dialog to turn on location services. I press OK, and one time it start location service with all sources and another time it starts gps only. The "GPS only" works very bad, I wait one - two minutes and it not give my position and not set blue dot on map. I turn off location and close app, open and again this dialog, and after "ok" it start all sources, and I get location instantly.
As I understand, the mode that will be set on start location service depends on priority of LocationRequest. So why once it set mode "all sources" and once "gps only", its about LocationRequest.PRIORITY_HIGH_ACCURACY
. If I try to use LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY
(its my default for s8) on all devices that I checked, it will start mode "wifi and other networks", but all time the same mode.
I use FusedLocationClient.
My code to start system dialog:
LocationSettingsRequest.Builder builder = new LocationSettingsRequest.Builder()
.addLocationRequest(getLocationRequest());
builder.setAlwaysShow(true);
SettingsClient client = LocationServices.getSettingsClient(this);
Task<LocationSettingsResponse> task = client.checkLocationSettings(builder.build());
task.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(@NonNull Exception e) {
if (e instanceof ResolvableApiException) {
try {
// Show the dialog by calling startResolutionForResult(),
// and check the result in onActivityResult().
ResolvableApiException resolvable = (ResolvableApiException) e;
resolvable.startResolutionForResult(MapsActivity.this,
REQUEST_CHECK_SETTINGS);
} catch (IntentSender.SendIntentException sendEx) {
// Ignore the error.
if (sendEx.getMessage() != null) {
Crashlytics.logException(new RuntimeException("startGpsService: " + sendEx.getMessage()));
}
}
}
}
});
And my LocationRequest:
if (mLocationRequest == null) {
mLocationRequest = new LocationRequest();
if (Build.MANUFACTURER.toLowerCase().contains("samsung") &&
Build.MODEL.toLowerCase().contains("sm-g95")) {
mLocationRequest.setPriority(LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY);
} else {
mLocationRequest.setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY);
}
mLocationRequest.setInterval(2000);
mLocationRequest.setFastestInterval(1000);
}
return mLocationRequest;
I'll be glad to get any suggestions.
Update, I make some tests, this is the log:
2018-11-20 09:56:11.055 29850-29850 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:56:29.554 30111-30111 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:56:48.847 30309-30309 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:57:30.645 30647-30647 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:57:58.354 30908-30908 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:58:16.632 31159-31159 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:59:57.753 1665-1665 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:14.176 2200-2200 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:30.406 2963-2963 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:45.215 3398-3398 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
All the time when I get "location providers not enabled" it comes to onActivityResult
with (requestCode == REQUEST_CHECK_SETTINGS && resultCode == RESULT_CANCELED)
and turn on "Device only" mode.
Its looks like it depends on place where exactly was my finger on "Yes" button.
Additional question: If I can make custom view for system "turn on location" dialog ?

add a comment |
So I have an app. It depends on user location. On lot of devices it worked fine, as I expect. There are problem with samsung s8, I checked 4 real s8 from different users and with different app that needs location, and the problem there.
But now my xiaomi mi a1 (with clean android) get android security update (8.1 November) and I get same problem as on s8.
The problem:
I turn off location service in settings of device. I start my app, or another app that need location (not google maps, it works awesome).
I get default system dialog to turn on location services. I press OK, and one time it start location service with all sources and another time it starts gps only. The "GPS only" works very bad, I wait one - two minutes and it not give my position and not set blue dot on map. I turn off location and close app, open and again this dialog, and after "ok" it start all sources, and I get location instantly.
As I understand, the mode that will be set on start location service depends on priority of LocationRequest. So why once it set mode "all sources" and once "gps only", its about LocationRequest.PRIORITY_HIGH_ACCURACY
. If I try to use LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY
(its my default for s8) on all devices that I checked, it will start mode "wifi and other networks", but all time the same mode.
I use FusedLocationClient.
My code to start system dialog:
LocationSettingsRequest.Builder builder = new LocationSettingsRequest.Builder()
.addLocationRequest(getLocationRequest());
builder.setAlwaysShow(true);
SettingsClient client = LocationServices.getSettingsClient(this);
Task<LocationSettingsResponse> task = client.checkLocationSettings(builder.build());
task.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(@NonNull Exception e) {
if (e instanceof ResolvableApiException) {
try {
// Show the dialog by calling startResolutionForResult(),
// and check the result in onActivityResult().
ResolvableApiException resolvable = (ResolvableApiException) e;
resolvable.startResolutionForResult(MapsActivity.this,
REQUEST_CHECK_SETTINGS);
} catch (IntentSender.SendIntentException sendEx) {
// Ignore the error.
if (sendEx.getMessage() != null) {
Crashlytics.logException(new RuntimeException("startGpsService: " + sendEx.getMessage()));
}
}
}
}
});
And my LocationRequest:
if (mLocationRequest == null) {
mLocationRequest = new LocationRequest();
if (Build.MANUFACTURER.toLowerCase().contains("samsung") &&
Build.MODEL.toLowerCase().contains("sm-g95")) {
mLocationRequest.setPriority(LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY);
} else {
mLocationRequest.setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY);
}
mLocationRequest.setInterval(2000);
mLocationRequest.setFastestInterval(1000);
}
return mLocationRequest;
I'll be glad to get any suggestions.
Update, I make some tests, this is the log:
2018-11-20 09:56:11.055 29850-29850 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:56:29.554 30111-30111 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:56:48.847 30309-30309 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:57:30.645 30647-30647 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:57:58.354 30908-30908 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:58:16.632 31159-31159 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:59:57.753 1665-1665 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:14.176 2200-2200 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:30.406 2963-2963 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:45.215 3398-3398 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
All the time when I get "location providers not enabled" it comes to onActivityResult
with (requestCode == REQUEST_CHECK_SETTINGS && resultCode == RESULT_CANCELED)
and turn on "Device only" mode.
Its looks like it depends on place where exactly was my finger on "Yes" button.
Additional question: If I can make custom view for system "turn on location" dialog ?

So I have an app. It depends on user location. On lot of devices it worked fine, as I expect. There are problem with samsung s8, I checked 4 real s8 from different users and with different app that needs location, and the problem there.
But now my xiaomi mi a1 (with clean android) get android security update (8.1 November) and I get same problem as on s8.
The problem:
I turn off location service in settings of device. I start my app, or another app that need location (not google maps, it works awesome).
I get default system dialog to turn on location services. I press OK, and one time it start location service with all sources and another time it starts gps only. The "GPS only" works very bad, I wait one - two minutes and it not give my position and not set blue dot on map. I turn off location and close app, open and again this dialog, and after "ok" it start all sources, and I get location instantly.
As I understand, the mode that will be set on start location service depends on priority of LocationRequest. So why once it set mode "all sources" and once "gps only", its about LocationRequest.PRIORITY_HIGH_ACCURACY
. If I try to use LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY
(its my default for s8) on all devices that I checked, it will start mode "wifi and other networks", but all time the same mode.
I use FusedLocationClient.
My code to start system dialog:
LocationSettingsRequest.Builder builder = new LocationSettingsRequest.Builder()
.addLocationRequest(getLocationRequest());
builder.setAlwaysShow(true);
SettingsClient client = LocationServices.getSettingsClient(this);
Task<LocationSettingsResponse> task = client.checkLocationSettings(builder.build());
task.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(@NonNull Exception e) {
if (e instanceof ResolvableApiException) {
try {
// Show the dialog by calling startResolutionForResult(),
// and check the result in onActivityResult().
ResolvableApiException resolvable = (ResolvableApiException) e;
resolvable.startResolutionForResult(MapsActivity.this,
REQUEST_CHECK_SETTINGS);
} catch (IntentSender.SendIntentException sendEx) {
// Ignore the error.
if (sendEx.getMessage() != null) {
Crashlytics.logException(new RuntimeException("startGpsService: " + sendEx.getMessage()));
}
}
}
}
});
And my LocationRequest:
if (mLocationRequest == null) {
mLocationRequest = new LocationRequest();
if (Build.MANUFACTURER.toLowerCase().contains("samsung") &&
Build.MODEL.toLowerCase().contains("sm-g95")) {
mLocationRequest.setPriority(LocationRequest.PRIORITY_BALANCED_POWER_ACCURACY);
} else {
mLocationRequest.setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY);
}
mLocationRequest.setInterval(2000);
mLocationRequest.setFastestInterval(1000);
}
return mLocationRequest;
I'll be glad to get any suggestions.
Update, I make some tests, this is the log:
2018-11-20 09:56:11.055 29850-29850 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:56:29.554 30111-30111 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:56:48.847 30309-30309 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:57:30.645 30647-30647 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:57:58.354 30908-30908 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 09:58:16.632 31159-31159 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
2018-11-20 09:59:57.753 1665-1665 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:14.176 2200-2200 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:30.406 2963-2963 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: NETWORK_PROVIDER && GPS_PROVIDER both enabled
2018-11-20 10:00:45.215 3398-3398 D/TAG_MAP_PROVIDER_CHECK: onActivityResult: location providers not enabled
All the time when I get "location providers not enabled" it comes to onActivityResult
with (requestCode == REQUEST_CHECK_SETTINGS && resultCode == RESULT_CANCELED)
and turn on "Device only" mode.
Its looks like it depends on place where exactly was my finger on "Yes" button.
Additional question: If I can make custom view for system "turn on location" dialog ?


edited Nov 20 at 11:02
asked Nov 20 at 6:57


Vadim Eksler
355214
355214
add a comment |
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53387741%2fstrange-behavior-of-locationrequest-priorities%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53387741%2fstrange-behavior-of-locationrequest-priorities%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XXoy ZnCHdKcwZXxRsrdyOp EGlj0N1VH 0vTgHA2knB