Iterating over AFHTTPSessionManager while in background mode
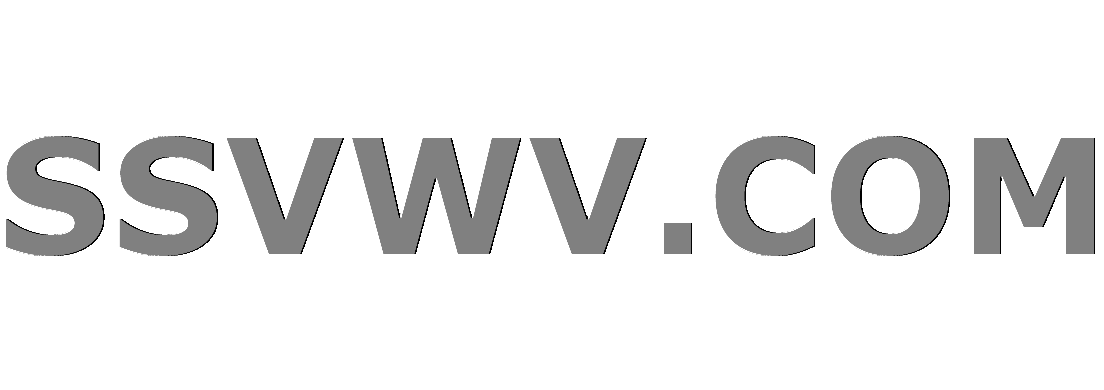
Multi tool use
I am using AFHTTPSessionManager to retrieve information from an API multiple times. It works correctly when its not in background mode, though once the app is closed AFHTTPSessionManager call doesn't seem to want to work, it blocks.
-(void)requestGET:(NSString *)strURL xml:(BOOL)xml Token:(NSString *)token success:(void (^)(NSDictionary *responseObject))success failure:(void (^)(NSError *error))failure {
BOOL isNetworkAvailable = [self checkNetConnection];
if (!isNetworkAvailable) {
NSLog(@"No connection");
[self showAlert:@"Please check your internet connection"];
return;
}else{
AFHTTPSessionManager *manager = [AFHTTPSessionManager manager];
[manager.requestSerializer setValue:[NSString stringWithFormat:@"Bearer %@",token] forHTTPHeaderField:@"Authorization"];
if(xml){
// Content xml
manager.responseSerializer = [AFHTTPResponseSerializer new];
manager.responseSerializer.acceptableContentTypes = [NSSet setWithObjects:@"application/vnd.garmin.tcx+xml", nil];
}else{
// Content json
manager.responseSerializer.acceptableContentTypes = [NSSet setWithObjects:@"application/json", nil];
}
[manager GET:strURL parameters:nil progress:nil success:^(NSURLSessionDataTask * _Nonnull task, id _Nullable responseObject) {
if([responseObject isKindOfClass:[NSDictionary class]]) {
// Return json
if(success) {
success(responseObject);
}
}else{
// Return xml to nsdictionary
if(success){
NSString* responseStr = [[NSString alloc] initWithData:responseObject encoding:NSUTF8StringEncoding];
NSDictionary * XMLDictionary = [XMLReader dictionaryForXMLString:responseStr error:nil];
success(XMLDictionary);
}
}
} failure:^(NSURLSessionDataTask * _Nullable task, NSError * _Nonnull error) {
NSLog(@"%@", error);
if(failure) {
failure(error);
}
}];
}
}
The above method is called below multiple times to grab the data from the API and pump it out.
-(void)getFitbitURL{
// Create group
dispatch_group_t group = dispatch_group_create();
// Retrieve array
NSMutableArray *URLS = [self generateURLS];
for (NSMutableArray *entity in URLS){
NSString *url = entity[0];
// Enter group
dispatch_group_enter(group);
NSString *token = [FitbitAuthHandler getToken];
FitbitAPIManager *manager = [FitbitAPIManager sharedManager];
// Get URL
[manager requestGET:url xml:0 Token:token success:^(NSDictionary *responseObject) {
// Do stuff with output
printf("%s",[[responseObject description]UTF8String]);
// Leave group
dispatch_group_leave(group);
} failure:^(NSError *error) {
NSLog("%@",error);
// Leave group
dispatch_group_leave(group);
}];
}
// Wait for all of group to complete
dispatch_group_notify(group, dispatch_get_main_queue(), ^{
NSLog(@"Complete");
});
}
I have tried to research on the internet how to do this but all my ideas have failed, my applicationDidEnterBackground method works correctly when the app closes but everything else doesn't work correctly, such as the url requests. Any suggestions would be appreciated
.
ios objective-c background
add a comment |
I am using AFHTTPSessionManager to retrieve information from an API multiple times. It works correctly when its not in background mode, though once the app is closed AFHTTPSessionManager call doesn't seem to want to work, it blocks.
-(void)requestGET:(NSString *)strURL xml:(BOOL)xml Token:(NSString *)token success:(void (^)(NSDictionary *responseObject))success failure:(void (^)(NSError *error))failure {
BOOL isNetworkAvailable = [self checkNetConnection];
if (!isNetworkAvailable) {
NSLog(@"No connection");
[self showAlert:@"Please check your internet connection"];
return;
}else{
AFHTTPSessionManager *manager = [AFHTTPSessionManager manager];
[manager.requestSerializer setValue:[NSString stringWithFormat:@"Bearer %@",token] forHTTPHeaderField:@"Authorization"];
if(xml){
// Content xml
manager.responseSerializer = [AFHTTPResponseSerializer new];
manager.responseSerializer.acceptableContentTypes = [NSSet setWithObjects:@"application/vnd.garmin.tcx+xml", nil];
}else{
// Content json
manager.responseSerializer.acceptableContentTypes = [NSSet setWithObjects:@"application/json", nil];
}
[manager GET:strURL parameters:nil progress:nil success:^(NSURLSessionDataTask * _Nonnull task, id _Nullable responseObject) {
if([responseObject isKindOfClass:[NSDictionary class]]) {
// Return json
if(success) {
success(responseObject);
}
}else{
// Return xml to nsdictionary
if(success){
NSString* responseStr = [[NSString alloc] initWithData:responseObject encoding:NSUTF8StringEncoding];
NSDictionary * XMLDictionary = [XMLReader dictionaryForXMLString:responseStr error:nil];
success(XMLDictionary);
}
}
} failure:^(NSURLSessionDataTask * _Nullable task, NSError * _Nonnull error) {
NSLog(@"%@", error);
if(failure) {
failure(error);
}
}];
}
}
The above method is called below multiple times to grab the data from the API and pump it out.
-(void)getFitbitURL{
// Create group
dispatch_group_t group = dispatch_group_create();
// Retrieve array
NSMutableArray *URLS = [self generateURLS];
for (NSMutableArray *entity in URLS){
NSString *url = entity[0];
// Enter group
dispatch_group_enter(group);
NSString *token = [FitbitAuthHandler getToken];
FitbitAPIManager *manager = [FitbitAPIManager sharedManager];
// Get URL
[manager requestGET:url xml:0 Token:token success:^(NSDictionary *responseObject) {
// Do stuff with output
printf("%s",[[responseObject description]UTF8String]);
// Leave group
dispatch_group_leave(group);
} failure:^(NSError *error) {
NSLog("%@",error);
// Leave group
dispatch_group_leave(group);
}];
}
// Wait for all of group to complete
dispatch_group_notify(group, dispatch_get_main_queue(), ^{
NSLog(@"Complete");
});
}
I have tried to research on the internet how to do this but all my ideas have failed, my applicationDidEnterBackground method works correctly when the app closes but everything else doesn't work correctly, such as the url requests. Any suggestions would be appreciated
.
ios objective-c background
add a comment |
I am using AFHTTPSessionManager to retrieve information from an API multiple times. It works correctly when its not in background mode, though once the app is closed AFHTTPSessionManager call doesn't seem to want to work, it blocks.
-(void)requestGET:(NSString *)strURL xml:(BOOL)xml Token:(NSString *)token success:(void (^)(NSDictionary *responseObject))success failure:(void (^)(NSError *error))failure {
BOOL isNetworkAvailable = [self checkNetConnection];
if (!isNetworkAvailable) {
NSLog(@"No connection");
[self showAlert:@"Please check your internet connection"];
return;
}else{
AFHTTPSessionManager *manager = [AFHTTPSessionManager manager];
[manager.requestSerializer setValue:[NSString stringWithFormat:@"Bearer %@",token] forHTTPHeaderField:@"Authorization"];
if(xml){
// Content xml
manager.responseSerializer = [AFHTTPResponseSerializer new];
manager.responseSerializer.acceptableContentTypes = [NSSet setWithObjects:@"application/vnd.garmin.tcx+xml", nil];
}else{
// Content json
manager.responseSerializer.acceptableContentTypes = [NSSet setWithObjects:@"application/json", nil];
}
[manager GET:strURL parameters:nil progress:nil success:^(NSURLSessionDataTask * _Nonnull task, id _Nullable responseObject) {
if([responseObject isKindOfClass:[NSDictionary class]]) {
// Return json
if(success) {
success(responseObject);
}
}else{
// Return xml to nsdictionary
if(success){
NSString* responseStr = [[NSString alloc] initWithData:responseObject encoding:NSUTF8StringEncoding];
NSDictionary * XMLDictionary = [XMLReader dictionaryForXMLString:responseStr error:nil];
success(XMLDictionary);
}
}
} failure:^(NSURLSessionDataTask * _Nullable task, NSError * _Nonnull error) {
NSLog(@"%@", error);
if(failure) {
failure(error);
}
}];
}
}
The above method is called below multiple times to grab the data from the API and pump it out.
-(void)getFitbitURL{
// Create group
dispatch_group_t group = dispatch_group_create();
// Retrieve array
NSMutableArray *URLS = [self generateURLS];
for (NSMutableArray *entity in URLS){
NSString *url = entity[0];
// Enter group
dispatch_group_enter(group);
NSString *token = [FitbitAuthHandler getToken];
FitbitAPIManager *manager = [FitbitAPIManager sharedManager];
// Get URL
[manager requestGET:url xml:0 Token:token success:^(NSDictionary *responseObject) {
// Do stuff with output
printf("%s",[[responseObject description]UTF8String]);
// Leave group
dispatch_group_leave(group);
} failure:^(NSError *error) {
NSLog("%@",error);
// Leave group
dispatch_group_leave(group);
}];
}
// Wait for all of group to complete
dispatch_group_notify(group, dispatch_get_main_queue(), ^{
NSLog(@"Complete");
});
}
I have tried to research on the internet how to do this but all my ideas have failed, my applicationDidEnterBackground method works correctly when the app closes but everything else doesn't work correctly, such as the url requests. Any suggestions would be appreciated
.
ios objective-c background
I am using AFHTTPSessionManager to retrieve information from an API multiple times. It works correctly when its not in background mode, though once the app is closed AFHTTPSessionManager call doesn't seem to want to work, it blocks.
-(void)requestGET:(NSString *)strURL xml:(BOOL)xml Token:(NSString *)token success:(void (^)(NSDictionary *responseObject))success failure:(void (^)(NSError *error))failure {
BOOL isNetworkAvailable = [self checkNetConnection];
if (!isNetworkAvailable) {
NSLog(@"No connection");
[self showAlert:@"Please check your internet connection"];
return;
}else{
AFHTTPSessionManager *manager = [AFHTTPSessionManager manager];
[manager.requestSerializer setValue:[NSString stringWithFormat:@"Bearer %@",token] forHTTPHeaderField:@"Authorization"];
if(xml){
// Content xml
manager.responseSerializer = [AFHTTPResponseSerializer new];
manager.responseSerializer.acceptableContentTypes = [NSSet setWithObjects:@"application/vnd.garmin.tcx+xml", nil];
}else{
// Content json
manager.responseSerializer.acceptableContentTypes = [NSSet setWithObjects:@"application/json", nil];
}
[manager GET:strURL parameters:nil progress:nil success:^(NSURLSessionDataTask * _Nonnull task, id _Nullable responseObject) {
if([responseObject isKindOfClass:[NSDictionary class]]) {
// Return json
if(success) {
success(responseObject);
}
}else{
// Return xml to nsdictionary
if(success){
NSString* responseStr = [[NSString alloc] initWithData:responseObject encoding:NSUTF8StringEncoding];
NSDictionary * XMLDictionary = [XMLReader dictionaryForXMLString:responseStr error:nil];
success(XMLDictionary);
}
}
} failure:^(NSURLSessionDataTask * _Nullable task, NSError * _Nonnull error) {
NSLog(@"%@", error);
if(failure) {
failure(error);
}
}];
}
}
The above method is called below multiple times to grab the data from the API and pump it out.
-(void)getFitbitURL{
// Create group
dispatch_group_t group = dispatch_group_create();
// Retrieve array
NSMutableArray *URLS = [self generateURLS];
for (NSMutableArray *entity in URLS){
NSString *url = entity[0];
// Enter group
dispatch_group_enter(group);
NSString *token = [FitbitAuthHandler getToken];
FitbitAPIManager *manager = [FitbitAPIManager sharedManager];
// Get URL
[manager requestGET:url xml:0 Token:token success:^(NSDictionary *responseObject) {
// Do stuff with output
printf("%s",[[responseObject description]UTF8String]);
// Leave group
dispatch_group_leave(group);
} failure:^(NSError *error) {
NSLog("%@",error);
// Leave group
dispatch_group_leave(group);
}];
}
// Wait for all of group to complete
dispatch_group_notify(group, dispatch_get_main_queue(), ^{
NSLog(@"Complete");
});
}
I have tried to research on the internet how to do this but all my ideas have failed, my applicationDidEnterBackground method works correctly when the app closes but everything else doesn't work correctly, such as the url requests. Any suggestions would be appreciated
.
ios objective-c background
ios objective-c background
edited Nov 21 '18 at 2:11
Jim Howard
asked Nov 21 '18 at 2:00


Jim HowardJim Howard
63
63
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404308%2fiterating-over-afhttpsessionmanager-while-in-background-mode%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404308%2fiterating-over-afhttpsessionmanager-while-in-background-mode%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tHe N45TC jxU4U,YSNb prOa661TVuLELkMv7zP tJOQAInMhwgz3M8Q19PCvhxwcq2D,N8,YbklPQtHE