Java 8 Strem filter map in map — Map<String,Map>
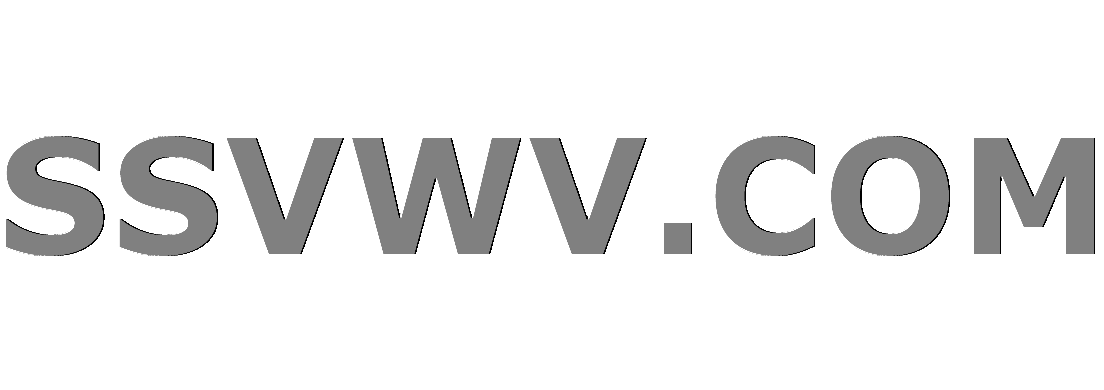
Multi tool use
How to filter a Map<String,Map<String,Employee>>
using Java 8 Filter?
I have to filter only when any of employee in the list having a field value Gender = "M".
Input:
Map<String,Map<String,Employee>>
Output:
Map<String,Map<String,Employee>>
Filter criteria:
Employee.genter = "M"
Also i have to return empty map if the filtered result is empty.
I tried the below, but it is not working as expected. It is returning the only if all the Employees are with gender "M".
tempCollection.entrySet().stream()
.filter(i -> i.getValue().entrySet().stream().allMatch(e-> "M".equals(e.getValue().getGender())))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
java java-8 hashmap java-stream
add a comment |
How to filter a Map<String,Map<String,Employee>>
using Java 8 Filter?
I have to filter only when any of employee in the list having a field value Gender = "M".
Input:
Map<String,Map<String,Employee>>
Output:
Map<String,Map<String,Employee>>
Filter criteria:
Employee.genter = "M"
Also i have to return empty map if the filtered result is empty.
I tried the below, but it is not working as expected. It is returning the only if all the Employees are with gender "M".
tempCollection.entrySet().stream()
.filter(i -> i.getValue().entrySet().stream().allMatch(e-> "M".equals(e.getValue().getGender())))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
java java-8 hashmap java-stream
Return all the employees whose gender is M.
– user1578872
2 days ago
add a comment |
How to filter a Map<String,Map<String,Employee>>
using Java 8 Filter?
I have to filter only when any of employee in the list having a field value Gender = "M".
Input:
Map<String,Map<String,Employee>>
Output:
Map<String,Map<String,Employee>>
Filter criteria:
Employee.genter = "M"
Also i have to return empty map if the filtered result is empty.
I tried the below, but it is not working as expected. It is returning the only if all the Employees are with gender "M".
tempCollection.entrySet().stream()
.filter(i -> i.getValue().entrySet().stream().allMatch(e-> "M".equals(e.getValue().getGender())))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
java java-8 hashmap java-stream
How to filter a Map<String,Map<String,Employee>>
using Java 8 Filter?
I have to filter only when any of employee in the list having a field value Gender = "M".
Input:
Map<String,Map<String,Employee>>
Output:
Map<String,Map<String,Employee>>
Filter criteria:
Employee.genter = "M"
Also i have to return empty map if the filtered result is empty.
I tried the below, but it is not working as expected. It is returning the only if all the Employees are with gender "M".
tempCollection.entrySet().stream()
.filter(i -> i.getValue().entrySet().stream().allMatch(e-> "M".equals(e.getValue().getGender())))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
java java-8 hashmap java-stream
java java-8 hashmap java-stream
edited 2 days ago


Aomine
39.3k73669
39.3k73669
asked 2 days ago
user1578872
1,54452564
1,54452564
Return all the employees whose gender is M.
– user1578872
2 days ago
add a comment |
Return all the employees whose gender is M.
– user1578872
2 days ago
Return all the employees whose gender is M.
– user1578872
2 days ago
Return all the employees whose gender is M.
– user1578872
2 days ago
add a comment |
5 Answers
5
active
oldest
votes
You could simply iterate on the key-value pairs and filter as:
Map<String, Map<String, Employee>> output = new HashMap<>();
tempCollection.forEach((k, v) -> {
if (v.values().stream().anyMatch(i -> "M".equals(i.getGender()))) {
output.put(k, v.entrySet()
.stream()
.filter(i -> "M".equals(i.getValue().getGender()))
.collect(toMap(Map.Entry::getKey, Map.Entry::getValue)));
}
});
anyMatch returns all the result even there is a single match, I want the key value to be removed if there is no match,
– user1578872
2 days ago
If none of the employee is with the Gender "M", it still returns Map<String,emptyMap()>
– user1578872
2 days ago
@user1578872 i have to return empty map if the filtered result is empty... that's what you meant, right?
– nullpointer
2 days ago
It should be completeEmpty map in this case. Like, return emptyMap(), not Map<String,emptyMap()>
– user1578872
2 days ago
2
I thinkput
would be more ideal rather thanputIfAbsent
as thekey
will always be unique.
– Aomine
2 days ago
|
show 3 more comments
The function allMatch
only matches if every element in the stream matches the predicate; you can use anyMatch
to match if any element matches the predicate:
tempCollection.entrySet().stream()
.filter(i -> i.getValue().entrySet().stream().anyMatch(e-> "M".equals(e.getValue().getGender())))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
add a comment |
Seems like what you're after is given a Entry<String,Map<String,Employee>>
if there's any employee who has a gender of "M" then filter the inner Map<String,Employee>
to contain only entries with a gender "M".
in which case you can filter
along with anyMatch
for the first criterion. Further, at the collecting phase, you can then apply the filtering on the inner map:
tempCollection.entrySet().stream()
.filter(i -> i.getValue().values().stream().anyMatch(e -> "M".equals(e.getGender())))
.collect(toMap(Map.Entry::getKey,
v -> v.getValue().entrySet().stream()
.filter(i -> "M".equals(i.getValue().getGender()))
.collect(toMap(Map.Entry::getKey, Map.Entry::getValue))));
anyMatch returns all the values in the map even there is only one employee with Gender M.
– user1578872
2 days ago
@user1578872 right, thanks to the accepted answer, I can now understand your requirement. the description wasn't very clear IMO. anyway edited to accommodate.
– Aomine
2 days ago
add a comment |
You may do it like so,
Map<String, Map<String, Employee>> maleEmpMap = tempCollection.entrySet().stream()
.map(e -> new AbstractMap.SimpleEntry<>(e.getKey(),
e.getValue().entrySet().stream().filter(emp -> "M".equals(emp.getValue().getGender()))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue))))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
Take each map entry from the outer map and create a new map entry using the same key and the newly constructed nested map with the same key and which has only male employees as it's value. Finally collect all those matching entries into a new outer map. This will gracefully handle the empty scenario which you mentioned in the problem statement above.
add a comment |
Other way would be like this:
map.values()
.removeIf(entry->entry.values().stream().anyMatch(e -> !"M".equals(e.getGender())));
map.entrySet()
.removeIf(entry->entry.getValue().size() == 0);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53927656%2fjava-8-strem-filter-map-in-map-mapstring-mapstring-employee%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could simply iterate on the key-value pairs and filter as:
Map<String, Map<String, Employee>> output = new HashMap<>();
tempCollection.forEach((k, v) -> {
if (v.values().stream().anyMatch(i -> "M".equals(i.getGender()))) {
output.put(k, v.entrySet()
.stream()
.filter(i -> "M".equals(i.getValue().getGender()))
.collect(toMap(Map.Entry::getKey, Map.Entry::getValue)));
}
});
anyMatch returns all the result even there is a single match, I want the key value to be removed if there is no match,
– user1578872
2 days ago
If none of the employee is with the Gender "M", it still returns Map<String,emptyMap()>
– user1578872
2 days ago
@user1578872 i have to return empty map if the filtered result is empty... that's what you meant, right?
– nullpointer
2 days ago
It should be completeEmpty map in this case. Like, return emptyMap(), not Map<String,emptyMap()>
– user1578872
2 days ago
2
I thinkput
would be more ideal rather thanputIfAbsent
as thekey
will always be unique.
– Aomine
2 days ago
|
show 3 more comments
You could simply iterate on the key-value pairs and filter as:
Map<String, Map<String, Employee>> output = new HashMap<>();
tempCollection.forEach((k, v) -> {
if (v.values().stream().anyMatch(i -> "M".equals(i.getGender()))) {
output.put(k, v.entrySet()
.stream()
.filter(i -> "M".equals(i.getValue().getGender()))
.collect(toMap(Map.Entry::getKey, Map.Entry::getValue)));
}
});
anyMatch returns all the result even there is a single match, I want the key value to be removed if there is no match,
– user1578872
2 days ago
If none of the employee is with the Gender "M", it still returns Map<String,emptyMap()>
– user1578872
2 days ago
@user1578872 i have to return empty map if the filtered result is empty... that's what you meant, right?
– nullpointer
2 days ago
It should be completeEmpty map in this case. Like, return emptyMap(), not Map<String,emptyMap()>
– user1578872
2 days ago
2
I thinkput
would be more ideal rather thanputIfAbsent
as thekey
will always be unique.
– Aomine
2 days ago
|
show 3 more comments
You could simply iterate on the key-value pairs and filter as:
Map<String, Map<String, Employee>> output = new HashMap<>();
tempCollection.forEach((k, v) -> {
if (v.values().stream().anyMatch(i -> "M".equals(i.getGender()))) {
output.put(k, v.entrySet()
.stream()
.filter(i -> "M".equals(i.getValue().getGender()))
.collect(toMap(Map.Entry::getKey, Map.Entry::getValue)));
}
});
You could simply iterate on the key-value pairs and filter as:
Map<String, Map<String, Employee>> output = new HashMap<>();
tempCollection.forEach((k, v) -> {
if (v.values().stream().anyMatch(i -> "M".equals(i.getGender()))) {
output.put(k, v.entrySet()
.stream()
.filter(i -> "M".equals(i.getValue().getGender()))
.collect(toMap(Map.Entry::getKey, Map.Entry::getValue)));
}
});
edited 2 days ago
answered 2 days ago


nullpointer
41.4k1087175
41.4k1087175
anyMatch returns all the result even there is a single match, I want the key value to be removed if there is no match,
– user1578872
2 days ago
If none of the employee is with the Gender "M", it still returns Map<String,emptyMap()>
– user1578872
2 days ago
@user1578872 i have to return empty map if the filtered result is empty... that's what you meant, right?
– nullpointer
2 days ago
It should be completeEmpty map in this case. Like, return emptyMap(), not Map<String,emptyMap()>
– user1578872
2 days ago
2
I thinkput
would be more ideal rather thanputIfAbsent
as thekey
will always be unique.
– Aomine
2 days ago
|
show 3 more comments
anyMatch returns all the result even there is a single match, I want the key value to be removed if there is no match,
– user1578872
2 days ago
If none of the employee is with the Gender "M", it still returns Map<String,emptyMap()>
– user1578872
2 days ago
@user1578872 i have to return empty map if the filtered result is empty... that's what you meant, right?
– nullpointer
2 days ago
It should be completeEmpty map in this case. Like, return emptyMap(), not Map<String,emptyMap()>
– user1578872
2 days ago
2
I thinkput
would be more ideal rather thanputIfAbsent
as thekey
will always be unique.
– Aomine
2 days ago
anyMatch returns all the result even there is a single match, I want the key value to be removed if there is no match,
– user1578872
2 days ago
anyMatch returns all the result even there is a single match, I want the key value to be removed if there is no match,
– user1578872
2 days ago
If none of the employee is with the Gender "M", it still returns Map<String,emptyMap()>
– user1578872
2 days ago
If none of the employee is with the Gender "M", it still returns Map<String,emptyMap()>
– user1578872
2 days ago
@user1578872 i have to return empty map if the filtered result is empty... that's what you meant, right?
– nullpointer
2 days ago
@user1578872 i have to return empty map if the filtered result is empty... that's what you meant, right?
– nullpointer
2 days ago
It should be completeEmpty map in this case. Like, return emptyMap(), not Map<String,emptyMap()>
– user1578872
2 days ago
It should be completeEmpty map in this case. Like, return emptyMap(), not Map<String,emptyMap()>
– user1578872
2 days ago
2
2
I think
put
would be more ideal rather than putIfAbsent
as the key
will always be unique.– Aomine
2 days ago
I think
put
would be more ideal rather than putIfAbsent
as the key
will always be unique.– Aomine
2 days ago
|
show 3 more comments
The function allMatch
only matches if every element in the stream matches the predicate; you can use anyMatch
to match if any element matches the predicate:
tempCollection.entrySet().stream()
.filter(i -> i.getValue().entrySet().stream().anyMatch(e-> "M".equals(e.getValue().getGender())))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
add a comment |
The function allMatch
only matches if every element in the stream matches the predicate; you can use anyMatch
to match if any element matches the predicate:
tempCollection.entrySet().stream()
.filter(i -> i.getValue().entrySet().stream().anyMatch(e-> "M".equals(e.getValue().getGender())))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
add a comment |
The function allMatch
only matches if every element in the stream matches the predicate; you can use anyMatch
to match if any element matches the predicate:
tempCollection.entrySet().stream()
.filter(i -> i.getValue().entrySet().stream().anyMatch(e-> "M".equals(e.getValue().getGender())))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
The function allMatch
only matches if every element in the stream matches the predicate; you can use anyMatch
to match if any element matches the predicate:
tempCollection.entrySet().stream()
.filter(i -> i.getValue().entrySet().stream().anyMatch(e-> "M".equals(e.getValue().getGender())))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
answered 2 days ago
Tordek
6,97622861
6,97622861
add a comment |
add a comment |
Seems like what you're after is given a Entry<String,Map<String,Employee>>
if there's any employee who has a gender of "M" then filter the inner Map<String,Employee>
to contain only entries with a gender "M".
in which case you can filter
along with anyMatch
for the first criterion. Further, at the collecting phase, you can then apply the filtering on the inner map:
tempCollection.entrySet().stream()
.filter(i -> i.getValue().values().stream().anyMatch(e -> "M".equals(e.getGender())))
.collect(toMap(Map.Entry::getKey,
v -> v.getValue().entrySet().stream()
.filter(i -> "M".equals(i.getValue().getGender()))
.collect(toMap(Map.Entry::getKey, Map.Entry::getValue))));
anyMatch returns all the values in the map even there is only one employee with Gender M.
– user1578872
2 days ago
@user1578872 right, thanks to the accepted answer, I can now understand your requirement. the description wasn't very clear IMO. anyway edited to accommodate.
– Aomine
2 days ago
add a comment |
Seems like what you're after is given a Entry<String,Map<String,Employee>>
if there's any employee who has a gender of "M" then filter the inner Map<String,Employee>
to contain only entries with a gender "M".
in which case you can filter
along with anyMatch
for the first criterion. Further, at the collecting phase, you can then apply the filtering on the inner map:
tempCollection.entrySet().stream()
.filter(i -> i.getValue().values().stream().anyMatch(e -> "M".equals(e.getGender())))
.collect(toMap(Map.Entry::getKey,
v -> v.getValue().entrySet().stream()
.filter(i -> "M".equals(i.getValue().getGender()))
.collect(toMap(Map.Entry::getKey, Map.Entry::getValue))));
anyMatch returns all the values in the map even there is only one employee with Gender M.
– user1578872
2 days ago
@user1578872 right, thanks to the accepted answer, I can now understand your requirement. the description wasn't very clear IMO. anyway edited to accommodate.
– Aomine
2 days ago
add a comment |
Seems like what you're after is given a Entry<String,Map<String,Employee>>
if there's any employee who has a gender of "M" then filter the inner Map<String,Employee>
to contain only entries with a gender "M".
in which case you can filter
along with anyMatch
for the first criterion. Further, at the collecting phase, you can then apply the filtering on the inner map:
tempCollection.entrySet().stream()
.filter(i -> i.getValue().values().stream().anyMatch(e -> "M".equals(e.getGender())))
.collect(toMap(Map.Entry::getKey,
v -> v.getValue().entrySet().stream()
.filter(i -> "M".equals(i.getValue().getGender()))
.collect(toMap(Map.Entry::getKey, Map.Entry::getValue))));
Seems like what you're after is given a Entry<String,Map<String,Employee>>
if there's any employee who has a gender of "M" then filter the inner Map<String,Employee>
to contain only entries with a gender "M".
in which case you can filter
along with anyMatch
for the first criterion. Further, at the collecting phase, you can then apply the filtering on the inner map:
tempCollection.entrySet().stream()
.filter(i -> i.getValue().values().stream().anyMatch(e -> "M".equals(e.getGender())))
.collect(toMap(Map.Entry::getKey,
v -> v.getValue().entrySet().stream()
.filter(i -> "M".equals(i.getValue().getGender()))
.collect(toMap(Map.Entry::getKey, Map.Entry::getValue))));
edited 2 days ago
answered 2 days ago


Aomine
39.3k73669
39.3k73669
anyMatch returns all the values in the map even there is only one employee with Gender M.
– user1578872
2 days ago
@user1578872 right, thanks to the accepted answer, I can now understand your requirement. the description wasn't very clear IMO. anyway edited to accommodate.
– Aomine
2 days ago
add a comment |
anyMatch returns all the values in the map even there is only one employee with Gender M.
– user1578872
2 days ago
@user1578872 right, thanks to the accepted answer, I can now understand your requirement. the description wasn't very clear IMO. anyway edited to accommodate.
– Aomine
2 days ago
anyMatch returns all the values in the map even there is only one employee with Gender M.
– user1578872
2 days ago
anyMatch returns all the values in the map even there is only one employee with Gender M.
– user1578872
2 days ago
@user1578872 right, thanks to the accepted answer, I can now understand your requirement. the description wasn't very clear IMO. anyway edited to accommodate.
– Aomine
2 days ago
@user1578872 right, thanks to the accepted answer, I can now understand your requirement. the description wasn't very clear IMO. anyway edited to accommodate.
– Aomine
2 days ago
add a comment |
You may do it like so,
Map<String, Map<String, Employee>> maleEmpMap = tempCollection.entrySet().stream()
.map(e -> new AbstractMap.SimpleEntry<>(e.getKey(),
e.getValue().entrySet().stream().filter(emp -> "M".equals(emp.getValue().getGender()))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue))))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
Take each map entry from the outer map and create a new map entry using the same key and the newly constructed nested map with the same key and which has only male employees as it's value. Finally collect all those matching entries into a new outer map. This will gracefully handle the empty scenario which you mentioned in the problem statement above.
add a comment |
You may do it like so,
Map<String, Map<String, Employee>> maleEmpMap = tempCollection.entrySet().stream()
.map(e -> new AbstractMap.SimpleEntry<>(e.getKey(),
e.getValue().entrySet().stream().filter(emp -> "M".equals(emp.getValue().getGender()))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue))))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
Take each map entry from the outer map and create a new map entry using the same key and the newly constructed nested map with the same key and which has only male employees as it's value. Finally collect all those matching entries into a new outer map. This will gracefully handle the empty scenario which you mentioned in the problem statement above.
add a comment |
You may do it like so,
Map<String, Map<String, Employee>> maleEmpMap = tempCollection.entrySet().stream()
.map(e -> new AbstractMap.SimpleEntry<>(e.getKey(),
e.getValue().entrySet().stream().filter(emp -> "M".equals(emp.getValue().getGender()))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue))))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
Take each map entry from the outer map and create a new map entry using the same key and the newly constructed nested map with the same key and which has only male employees as it's value. Finally collect all those matching entries into a new outer map. This will gracefully handle the empty scenario which you mentioned in the problem statement above.
You may do it like so,
Map<String, Map<String, Employee>> maleEmpMap = tempCollection.entrySet().stream()
.map(e -> new AbstractMap.SimpleEntry<>(e.getKey(),
e.getValue().entrySet().stream().filter(emp -> "M".equals(emp.getValue().getGender()))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue))))
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue));
Take each map entry from the outer map and create a new map entry using the same key and the newly constructed nested map with the same key and which has only male employees as it's value. Finally collect all those matching entries into a new outer map. This will gracefully handle the empty scenario which you mentioned in the problem statement above.
edited 2 days ago
answered 2 days ago


Ravindra Ranwala
8,31731634
8,31731634
add a comment |
add a comment |
Other way would be like this:
map.values()
.removeIf(entry->entry.values().stream().anyMatch(e -> !"M".equals(e.getGender())));
map.entrySet()
.removeIf(entry->entry.getValue().size() == 0);
add a comment |
Other way would be like this:
map.values()
.removeIf(entry->entry.values().stream().anyMatch(e -> !"M".equals(e.getGender())));
map.entrySet()
.removeIf(entry->entry.getValue().size() == 0);
add a comment |
Other way would be like this:
map.values()
.removeIf(entry->entry.values().stream().anyMatch(e -> !"M".equals(e.getGender())));
map.entrySet()
.removeIf(entry->entry.getValue().size() == 0);
Other way would be like this:
map.values()
.removeIf(entry->entry.values().stream().anyMatch(e -> !"M".equals(e.getGender())));
map.entrySet()
.removeIf(entry->entry.getValue().size() == 0);
answered 2 days ago


Hadi J
9,70731641
9,70731641
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53927656%2fjava-8-strem-filter-map-in-map-mapstring-mapstring-employee%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
D5o2DqN
Return all the employees whose gender is M.
– user1578872
2 days ago