Apply list of regex pattern on list python
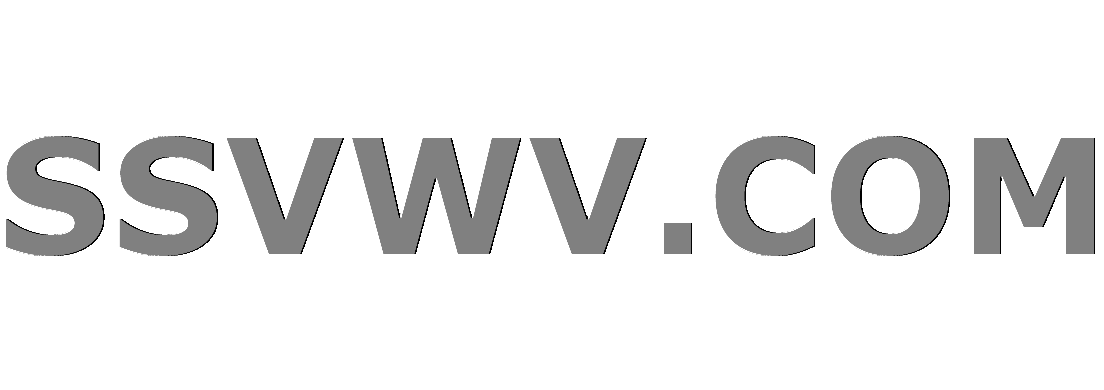
Multi tool use
I have data frame in which txt
column contains a list. I want to clean the txt
column using function clean_text().
data = {'value':['abc.txt', 'cda.txt'], 'txt':['[''2019/01/31-11:56:23.288258 1886 7F0ED4CDC704 asfasnfs: remove datepart'']',
'[''2019/02/01-11:56:23.288258 1886 7F0ED4CDC704 asfasnfs: remove datepart'']']}
df = pandas.DataFrame(data=data)
def clean_text(text):
"""
:param text: it is the plain text
:return: cleaned text
"""
patterns = [r"^{53}",
r"[A-Za-z]+[d]+[w]*|[d]+[A-Za-z]+[w]*",
r"[-=/':,?${}[]-_()>.~" ";+]"]
for p in patterns:
text = re.sub(p, '', text)
return text
My Solution:
df['txt'] = df['txt'].apply(lambda x: clean_text(x))
But I am getting below error:
Error
sre_constants.error: nothing to repeat at position 1
python regex list
add a comment |
I have data frame in which txt
column contains a list. I want to clean the txt
column using function clean_text().
data = {'value':['abc.txt', 'cda.txt'], 'txt':['[''2019/01/31-11:56:23.288258 1886 7F0ED4CDC704 asfasnfs: remove datepart'']',
'[''2019/02/01-11:56:23.288258 1886 7F0ED4CDC704 asfasnfs: remove datepart'']']}
df = pandas.DataFrame(data=data)
def clean_text(text):
"""
:param text: it is the plain text
:return: cleaned text
"""
patterns = [r"^{53}",
r"[A-Za-z]+[d]+[w]*|[d]+[A-Za-z]+[w]*",
r"[-=/':,?${}[]-_()>.~" ";+]"]
for p in patterns:
text = re.sub(p, '', text)
return text
My Solution:
df['txt'] = df['txt'].apply(lambda x: clean_text(x))
But I am getting below error:
Error
sre_constants.error: nothing to repeat at position 1
python regex list
Possible duplicate of Regex sre_constants.error: bad character range
– sophros
9 hours ago
@sophros, this question is different.
– user15051990
9 hours ago
in what way it is different? The error is the same.
– sophros
9 hours ago
add a comment |
I have data frame in which txt
column contains a list. I want to clean the txt
column using function clean_text().
data = {'value':['abc.txt', 'cda.txt'], 'txt':['[''2019/01/31-11:56:23.288258 1886 7F0ED4CDC704 asfasnfs: remove datepart'']',
'[''2019/02/01-11:56:23.288258 1886 7F0ED4CDC704 asfasnfs: remove datepart'']']}
df = pandas.DataFrame(data=data)
def clean_text(text):
"""
:param text: it is the plain text
:return: cleaned text
"""
patterns = [r"^{53}",
r"[A-Za-z]+[d]+[w]*|[d]+[A-Za-z]+[w]*",
r"[-=/':,?${}[]-_()>.~" ";+]"]
for p in patterns:
text = re.sub(p, '', text)
return text
My Solution:
df['txt'] = df['txt'].apply(lambda x: clean_text(x))
But I am getting below error:
Error
sre_constants.error: nothing to repeat at position 1
python regex list
I have data frame in which txt
column contains a list. I want to clean the txt
column using function clean_text().
data = {'value':['abc.txt', 'cda.txt'], 'txt':['[''2019/01/31-11:56:23.288258 1886 7F0ED4CDC704 asfasnfs: remove datepart'']',
'[''2019/02/01-11:56:23.288258 1886 7F0ED4CDC704 asfasnfs: remove datepart'']']}
df = pandas.DataFrame(data=data)
def clean_text(text):
"""
:param text: it is the plain text
:return: cleaned text
"""
patterns = [r"^{53}",
r"[A-Za-z]+[d]+[w]*|[d]+[A-Za-z]+[w]*",
r"[-=/':,?${}[]-_()>.~" ";+]"]
for p in patterns:
text = re.sub(p, '', text)
return text
My Solution:
df['txt'] = df['txt'].apply(lambda x: clean_text(x))
But I am getting below error:
Error
sre_constants.error: nothing to repeat at position 1
python regex list
python regex list
edited 7 hours ago


coldspeed
130k23134220
130k23134220
asked 10 hours ago
user15051990user15051990
639614
639614
Possible duplicate of Regex sre_constants.error: bad character range
– sophros
9 hours ago
@sophros, this question is different.
– user15051990
9 hours ago
in what way it is different? The error is the same.
– sophros
9 hours ago
add a comment |
Possible duplicate of Regex sre_constants.error: bad character range
– sophros
9 hours ago
@sophros, this question is different.
– user15051990
9 hours ago
in what way it is different? The error is the same.
– sophros
9 hours ago
Possible duplicate of Regex sre_constants.error: bad character range
– sophros
9 hours ago
Possible duplicate of Regex sre_constants.error: bad character range
– sophros
9 hours ago
@sophros, this question is different.
– user15051990
9 hours ago
@sophros, this question is different.
– user15051990
9 hours ago
in what way it is different? The error is the same.
– sophros
9 hours ago
in what way it is different? The error is the same.
– sophros
9 hours ago
add a comment |
2 Answers
2
active
oldest
votes
^{53}
is not a valid regular expression, since the repeater {53}
must be preceded by a character or a pattern that can be repeated. If you mean to make it validate a string that is at least 53 characters long you can use the following pattern instead:
^.{53}
1
Thanks for answer. I have updated question, now I getAttribute error
.
– user15051990
9 hours ago
1
@user15051990 Does^.{53,}
work?
– MilkyWay90
6 hours ago
add a comment |
The culprit is the first pattern from the list - r"^{53}"
. It reads: ^
- match the beginning of the string and then {53}
repeat the previous character or group 53 times. Wait... but there is no other character than ^
which cannot be repeated! Indeed. Add a char that you want to match 53 repetitions of. Or, escape the sequence {53}
if you want to match it verbatim, e.g. using re.escape
.
Thanks for answer. I have updated question, now I getAttribute error
.
– user15051990
9 hours ago
1
This should really be another question. How a reader of the question can make any sense of the answers if you change the crucial elements of the question?
– sophros
9 hours ago
Alright I will post it as different question.
– user15051990
9 hours ago
And before you do that - please revert the change first so that the answers make sense with the question.
– sophros
9 hours ago
1
I have already done that although I believe you should reward the effort already made on answering this question.
– sophros
9 hours ago
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54620212%2fapply-list-of-regex-pattern-on-list-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
^{53}
is not a valid regular expression, since the repeater {53}
must be preceded by a character or a pattern that can be repeated. If you mean to make it validate a string that is at least 53 characters long you can use the following pattern instead:
^.{53}
1
Thanks for answer. I have updated question, now I getAttribute error
.
– user15051990
9 hours ago
1
@user15051990 Does^.{53,}
work?
– MilkyWay90
6 hours ago
add a comment |
^{53}
is not a valid regular expression, since the repeater {53}
must be preceded by a character or a pattern that can be repeated. If you mean to make it validate a string that is at least 53 characters long you can use the following pattern instead:
^.{53}
1
Thanks for answer. I have updated question, now I getAttribute error
.
– user15051990
9 hours ago
1
@user15051990 Does^.{53,}
work?
– MilkyWay90
6 hours ago
add a comment |
^{53}
is not a valid regular expression, since the repeater {53}
must be preceded by a character or a pattern that can be repeated. If you mean to make it validate a string that is at least 53 characters long you can use the following pattern instead:
^.{53}
^{53}
is not a valid regular expression, since the repeater {53}
must be preceded by a character or a pattern that can be repeated. If you mean to make it validate a string that is at least 53 characters long you can use the following pattern instead:
^.{53}
answered 9 hours ago
blhsingblhsing
31.9k41336
31.9k41336
1
Thanks for answer. I have updated question, now I getAttribute error
.
– user15051990
9 hours ago
1
@user15051990 Does^.{53,}
work?
– MilkyWay90
6 hours ago
add a comment |
1
Thanks for answer. I have updated question, now I getAttribute error
.
– user15051990
9 hours ago
1
@user15051990 Does^.{53,}
work?
– MilkyWay90
6 hours ago
1
1
Thanks for answer. I have updated question, now I get
Attribute error
.– user15051990
9 hours ago
Thanks for answer. I have updated question, now I get
Attribute error
.– user15051990
9 hours ago
1
1
@user15051990 Does
^.{53,}
work?– MilkyWay90
6 hours ago
@user15051990 Does
^.{53,}
work?– MilkyWay90
6 hours ago
add a comment |
The culprit is the first pattern from the list - r"^{53}"
. It reads: ^
- match the beginning of the string and then {53}
repeat the previous character or group 53 times. Wait... but there is no other character than ^
which cannot be repeated! Indeed. Add a char that you want to match 53 repetitions of. Or, escape the sequence {53}
if you want to match it verbatim, e.g. using re.escape
.
Thanks for answer. I have updated question, now I getAttribute error
.
– user15051990
9 hours ago
1
This should really be another question. How a reader of the question can make any sense of the answers if you change the crucial elements of the question?
– sophros
9 hours ago
Alright I will post it as different question.
– user15051990
9 hours ago
And before you do that - please revert the change first so that the answers make sense with the question.
– sophros
9 hours ago
1
I have already done that although I believe you should reward the effort already made on answering this question.
– sophros
9 hours ago
|
show 1 more comment
The culprit is the first pattern from the list - r"^{53}"
. It reads: ^
- match the beginning of the string and then {53}
repeat the previous character or group 53 times. Wait... but there is no other character than ^
which cannot be repeated! Indeed. Add a char that you want to match 53 repetitions of. Or, escape the sequence {53}
if you want to match it verbatim, e.g. using re.escape
.
Thanks for answer. I have updated question, now I getAttribute error
.
– user15051990
9 hours ago
1
This should really be another question. How a reader of the question can make any sense of the answers if you change the crucial elements of the question?
– sophros
9 hours ago
Alright I will post it as different question.
– user15051990
9 hours ago
And before you do that - please revert the change first so that the answers make sense with the question.
– sophros
9 hours ago
1
I have already done that although I believe you should reward the effort already made on answering this question.
– sophros
9 hours ago
|
show 1 more comment
The culprit is the first pattern from the list - r"^{53}"
. It reads: ^
- match the beginning of the string and then {53}
repeat the previous character or group 53 times. Wait... but there is no other character than ^
which cannot be repeated! Indeed. Add a char that you want to match 53 repetitions of. Or, escape the sequence {53}
if you want to match it verbatim, e.g. using re.escape
.
The culprit is the first pattern from the list - r"^{53}"
. It reads: ^
- match the beginning of the string and then {53}
repeat the previous character or group 53 times. Wait... but there is no other character than ^
which cannot be repeated! Indeed. Add a char that you want to match 53 repetitions of. Or, escape the sequence {53}
if you want to match it verbatim, e.g. using re.escape
.
answered 9 hours ago


sophrossophros
2,5061728
2,5061728
Thanks for answer. I have updated question, now I getAttribute error
.
– user15051990
9 hours ago
1
This should really be another question. How a reader of the question can make any sense of the answers if you change the crucial elements of the question?
– sophros
9 hours ago
Alright I will post it as different question.
– user15051990
9 hours ago
And before you do that - please revert the change first so that the answers make sense with the question.
– sophros
9 hours ago
1
I have already done that although I believe you should reward the effort already made on answering this question.
– sophros
9 hours ago
|
show 1 more comment
Thanks for answer. I have updated question, now I getAttribute error
.
– user15051990
9 hours ago
1
This should really be another question. How a reader of the question can make any sense of the answers if you change the crucial elements of the question?
– sophros
9 hours ago
Alright I will post it as different question.
– user15051990
9 hours ago
And before you do that - please revert the change first so that the answers make sense with the question.
– sophros
9 hours ago
1
I have already done that although I believe you should reward the effort already made on answering this question.
– sophros
9 hours ago
Thanks for answer. I have updated question, now I get
Attribute error
.– user15051990
9 hours ago
Thanks for answer. I have updated question, now I get
Attribute error
.– user15051990
9 hours ago
1
1
This should really be another question. How a reader of the question can make any sense of the answers if you change the crucial elements of the question?
– sophros
9 hours ago
This should really be another question. How a reader of the question can make any sense of the answers if you change the crucial elements of the question?
– sophros
9 hours ago
Alright I will post it as different question.
– user15051990
9 hours ago
Alright I will post it as different question.
– user15051990
9 hours ago
And before you do that - please revert the change first so that the answers make sense with the question.
– sophros
9 hours ago
And before you do that - please revert the change first so that the answers make sense with the question.
– sophros
9 hours ago
1
1
I have already done that although I believe you should reward the effort already made on answering this question.
– sophros
9 hours ago
I have already done that although I believe you should reward the effort already made on answering this question.
– sophros
9 hours ago
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54620212%2fapply-list-of-regex-pattern-on-list-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
flUT,jXkWs QJ,QaCiJIbBOQNkTG,9nKyZvUWwViy2Ut fgPpQZ aFDxAM2 DQDzLopI e6,5wcEJEaAGg
Possible duplicate of Regex sre_constants.error: bad character range
– sophros
9 hours ago
@sophros, this question is different.
– user15051990
9 hours ago
in what way it is different? The error is the same.
– sophros
9 hours ago