Reverse dictionary where values are lists
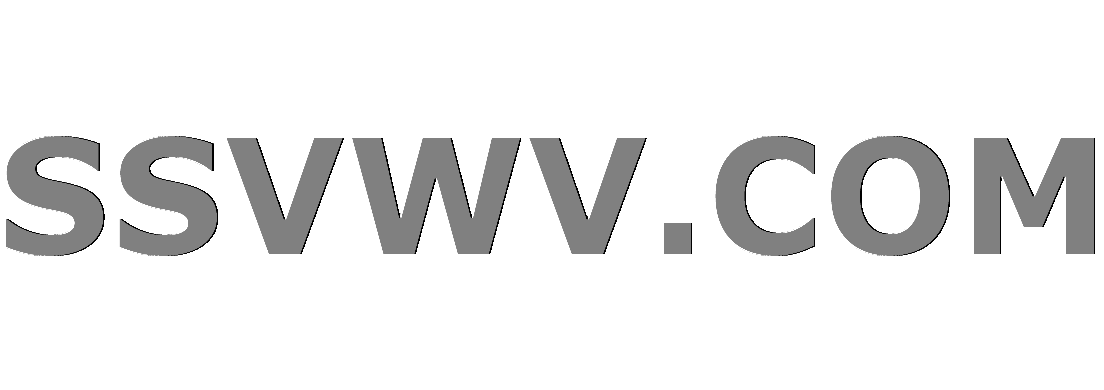
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ margin-bottom:0;
}
$begingroup$
I'm working on the following problem: I've got a dictionary like this one:
dic={0:[0,1,2],1:[3,4,5]}
And I want to reverse it so it looks like this:
dic2={0:0,1:0,2:0,3:1,4:1,5:1}
I managed to make it, but doing this:
dic2={}
for i in dic:
for j in dic[i]:
dic2[j]=i
I know about list and dict comprehensions and this code reeks of it, but I'm not good at them when there are nested for
and dicts
. How would you make it more efficiently?
python dictionary
$endgroup$
add a comment |
$begingroup$
I'm working on the following problem: I've got a dictionary like this one:
dic={0:[0,1,2],1:[3,4,5]}
And I want to reverse it so it looks like this:
dic2={0:0,1:0,2:0,3:1,4:1,5:1}
I managed to make it, but doing this:
dic2={}
for i in dic:
for j in dic[i]:
dic2[j]=i
I know about list and dict comprehensions and this code reeks of it, but I'm not good at them when there are nested for
and dicts
. How would you make it more efficiently?
python dictionary
$endgroup$
add a comment |
$begingroup$
I'm working on the following problem: I've got a dictionary like this one:
dic={0:[0,1,2],1:[3,4,5]}
And I want to reverse it so it looks like this:
dic2={0:0,1:0,2:0,3:1,4:1,5:1}
I managed to make it, but doing this:
dic2={}
for i in dic:
for j in dic[i]:
dic2[j]=i
I know about list and dict comprehensions and this code reeks of it, but I'm not good at them when there are nested for
and dicts
. How would you make it more efficiently?
python dictionary
$endgroup$
I'm working on the following problem: I've got a dictionary like this one:
dic={0:[0,1,2],1:[3,4,5]}
And I want to reverse it so it looks like this:
dic2={0:0,1:0,2:0,3:1,4:1,5:1}
I managed to make it, but doing this:
dic2={}
for i in dic:
for j in dic[i]:
dic2[j]=i
I know about list and dict comprehensions and this code reeks of it, but I'm not good at them when there are nested for
and dicts
. How would you make it more efficiently?
python dictionary
python dictionary
edited Apr 3 at 20:29


Sᴀᴍ Onᴇᴌᴀ
10.6k62168
10.6k62168
asked Apr 3 at 20:06


Juan CJuan C
1305
1305
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
$begingroup$
There's really not much to say about this code, it is straightforward.
Style
These are only nitpicks.
- Generic dictionary keys are typically named
k
instead ofi
orj
, but in a specific application a more descriptive name would be even better. - Collections should be named by their purpose in the application, not their type.
- By convention, assignments and other binary operators should be surrounded by spaces:
a = b
, nota=b
. See PEP 8 for reference, if you're not already aware of it. Following this style guide makes your code easier to read for others.
Code improvements
When iterating over the keys and values of a dictionary at the same time, you can use
for k, v in dic.items():
# ... use k, v ...
instead of
for k in dic:
v = dic[k]
# ...
The nested loop can be transformed to a dictionary comprehension like this:
dic2 = {v: k for k, values in dic.items() for v in values}
You can remember that the order of for
clauses in the comprehension is the same as the order of corresponding nested for
loops.
Potential pitfalls
You should be aware that this transformation from a dictionary of lists to a reverse dictionary only works if all items in the original lists are unique. Counterexample:
>>> dic = {0: [1, 2], 1: [2, 3]}
>>> {v: k for k, values in dic.items() for v in values}
{1: 0, 2: 1, 3: 1} # missing 2: 0
To correct this, the output dictionary should have the same format as the input, namely mapping each of the items in the input lists to a list of corresponding keys.
If the input represents a directed graph (mapping nodes to lists of neighbours), this corresponds to computing the transposed or reversed graph. It can be done by using a collections.defaultdict
. I don't see an easy way to write it as a comprehension in this case.
from collections import defaultdict
graph = {0: [1, 2], 1: [2, 3]}
transposed_graph = defaultdict(list)
for node, neighbours in graph.items():
for neighbour in neighbours:
transposed_graph[neighbour].append(node)
# {1: [0], 2: [0, 1], 3: [1]}
$endgroup$
1
$begingroup$
Also, it's important to use an entry point in Python - the classicif __name__ == "__main__":
because tools like Sphinx which auto-document code will load the code to reflect what properties and methods each object has. Having the entry point separates the execution of the code, from the code itself (does that make sense?). The code in the current form will always execute, as opposed to having something likedo_transpose(graph)
after the entry point responsible for execution.
$endgroup$
– C. Harley
Apr 4 at 3:54
$begingroup$
Way better answer than what I was expecting and just what I need. I don't come from a programming career, so I'm learning the conventions as I go, so this was really helpful. Thanks a lot to both of you !
$endgroup$
– Juan C
Apr 4 at 15:16
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216814%2freverse-dictionary-where-values-are-lists%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
There's really not much to say about this code, it is straightforward.
Style
These are only nitpicks.
- Generic dictionary keys are typically named
k
instead ofi
orj
, but in a specific application a more descriptive name would be even better. - Collections should be named by their purpose in the application, not their type.
- By convention, assignments and other binary operators should be surrounded by spaces:
a = b
, nota=b
. See PEP 8 for reference, if you're not already aware of it. Following this style guide makes your code easier to read for others.
Code improvements
When iterating over the keys and values of a dictionary at the same time, you can use
for k, v in dic.items():
# ... use k, v ...
instead of
for k in dic:
v = dic[k]
# ...
The nested loop can be transformed to a dictionary comprehension like this:
dic2 = {v: k for k, values in dic.items() for v in values}
You can remember that the order of for
clauses in the comprehension is the same as the order of corresponding nested for
loops.
Potential pitfalls
You should be aware that this transformation from a dictionary of lists to a reverse dictionary only works if all items in the original lists are unique. Counterexample:
>>> dic = {0: [1, 2], 1: [2, 3]}
>>> {v: k for k, values in dic.items() for v in values}
{1: 0, 2: 1, 3: 1} # missing 2: 0
To correct this, the output dictionary should have the same format as the input, namely mapping each of the items in the input lists to a list of corresponding keys.
If the input represents a directed graph (mapping nodes to lists of neighbours), this corresponds to computing the transposed or reversed graph. It can be done by using a collections.defaultdict
. I don't see an easy way to write it as a comprehension in this case.
from collections import defaultdict
graph = {0: [1, 2], 1: [2, 3]}
transposed_graph = defaultdict(list)
for node, neighbours in graph.items():
for neighbour in neighbours:
transposed_graph[neighbour].append(node)
# {1: [0], 2: [0, 1], 3: [1]}
$endgroup$
1
$begingroup$
Also, it's important to use an entry point in Python - the classicif __name__ == "__main__":
because tools like Sphinx which auto-document code will load the code to reflect what properties and methods each object has. Having the entry point separates the execution of the code, from the code itself (does that make sense?). The code in the current form will always execute, as opposed to having something likedo_transpose(graph)
after the entry point responsible for execution.
$endgroup$
– C. Harley
Apr 4 at 3:54
$begingroup$
Way better answer than what I was expecting and just what I need. I don't come from a programming career, so I'm learning the conventions as I go, so this was really helpful. Thanks a lot to both of you !
$endgroup$
– Juan C
Apr 4 at 15:16
add a comment |
$begingroup$
There's really not much to say about this code, it is straightforward.
Style
These are only nitpicks.
- Generic dictionary keys are typically named
k
instead ofi
orj
, but in a specific application a more descriptive name would be even better. - Collections should be named by their purpose in the application, not their type.
- By convention, assignments and other binary operators should be surrounded by spaces:
a = b
, nota=b
. See PEP 8 for reference, if you're not already aware of it. Following this style guide makes your code easier to read for others.
Code improvements
When iterating over the keys and values of a dictionary at the same time, you can use
for k, v in dic.items():
# ... use k, v ...
instead of
for k in dic:
v = dic[k]
# ...
The nested loop can be transformed to a dictionary comprehension like this:
dic2 = {v: k for k, values in dic.items() for v in values}
You can remember that the order of for
clauses in the comprehension is the same as the order of corresponding nested for
loops.
Potential pitfalls
You should be aware that this transformation from a dictionary of lists to a reverse dictionary only works if all items in the original lists are unique. Counterexample:
>>> dic = {0: [1, 2], 1: [2, 3]}
>>> {v: k for k, values in dic.items() for v in values}
{1: 0, 2: 1, 3: 1} # missing 2: 0
To correct this, the output dictionary should have the same format as the input, namely mapping each of the items in the input lists to a list of corresponding keys.
If the input represents a directed graph (mapping nodes to lists of neighbours), this corresponds to computing the transposed or reversed graph. It can be done by using a collections.defaultdict
. I don't see an easy way to write it as a comprehension in this case.
from collections import defaultdict
graph = {0: [1, 2], 1: [2, 3]}
transposed_graph = defaultdict(list)
for node, neighbours in graph.items():
for neighbour in neighbours:
transposed_graph[neighbour].append(node)
# {1: [0], 2: [0, 1], 3: [1]}
$endgroup$
1
$begingroup$
Also, it's important to use an entry point in Python - the classicif __name__ == "__main__":
because tools like Sphinx which auto-document code will load the code to reflect what properties and methods each object has. Having the entry point separates the execution of the code, from the code itself (does that make sense?). The code in the current form will always execute, as opposed to having something likedo_transpose(graph)
after the entry point responsible for execution.
$endgroup$
– C. Harley
Apr 4 at 3:54
$begingroup$
Way better answer than what I was expecting and just what I need. I don't come from a programming career, so I'm learning the conventions as I go, so this was really helpful. Thanks a lot to both of you !
$endgroup$
– Juan C
Apr 4 at 15:16
add a comment |
$begingroup$
There's really not much to say about this code, it is straightforward.
Style
These are only nitpicks.
- Generic dictionary keys are typically named
k
instead ofi
orj
, but in a specific application a more descriptive name would be even better. - Collections should be named by their purpose in the application, not their type.
- By convention, assignments and other binary operators should be surrounded by spaces:
a = b
, nota=b
. See PEP 8 for reference, if you're not already aware of it. Following this style guide makes your code easier to read for others.
Code improvements
When iterating over the keys and values of a dictionary at the same time, you can use
for k, v in dic.items():
# ... use k, v ...
instead of
for k in dic:
v = dic[k]
# ...
The nested loop can be transformed to a dictionary comprehension like this:
dic2 = {v: k for k, values in dic.items() for v in values}
You can remember that the order of for
clauses in the comprehension is the same as the order of corresponding nested for
loops.
Potential pitfalls
You should be aware that this transformation from a dictionary of lists to a reverse dictionary only works if all items in the original lists are unique. Counterexample:
>>> dic = {0: [1, 2], 1: [2, 3]}
>>> {v: k for k, values in dic.items() for v in values}
{1: 0, 2: 1, 3: 1} # missing 2: 0
To correct this, the output dictionary should have the same format as the input, namely mapping each of the items in the input lists to a list of corresponding keys.
If the input represents a directed graph (mapping nodes to lists of neighbours), this corresponds to computing the transposed or reversed graph. It can be done by using a collections.defaultdict
. I don't see an easy way to write it as a comprehension in this case.
from collections import defaultdict
graph = {0: [1, 2], 1: [2, 3]}
transposed_graph = defaultdict(list)
for node, neighbours in graph.items():
for neighbour in neighbours:
transposed_graph[neighbour].append(node)
# {1: [0], 2: [0, 1], 3: [1]}
$endgroup$
There's really not much to say about this code, it is straightforward.
Style
These are only nitpicks.
- Generic dictionary keys are typically named
k
instead ofi
orj
, but in a specific application a more descriptive name would be even better. - Collections should be named by their purpose in the application, not their type.
- By convention, assignments and other binary operators should be surrounded by spaces:
a = b
, nota=b
. See PEP 8 for reference, if you're not already aware of it. Following this style guide makes your code easier to read for others.
Code improvements
When iterating over the keys and values of a dictionary at the same time, you can use
for k, v in dic.items():
# ... use k, v ...
instead of
for k in dic:
v = dic[k]
# ...
The nested loop can be transformed to a dictionary comprehension like this:
dic2 = {v: k for k, values in dic.items() for v in values}
You can remember that the order of for
clauses in the comprehension is the same as the order of corresponding nested for
loops.
Potential pitfalls
You should be aware that this transformation from a dictionary of lists to a reverse dictionary only works if all items in the original lists are unique. Counterexample:
>>> dic = {0: [1, 2], 1: [2, 3]}
>>> {v: k for k, values in dic.items() for v in values}
{1: 0, 2: 1, 3: 1} # missing 2: 0
To correct this, the output dictionary should have the same format as the input, namely mapping each of the items in the input lists to a list of corresponding keys.
If the input represents a directed graph (mapping nodes to lists of neighbours), this corresponds to computing the transposed or reversed graph. It can be done by using a collections.defaultdict
. I don't see an easy way to write it as a comprehension in this case.
from collections import defaultdict
graph = {0: [1, 2], 1: [2, 3]}
transposed_graph = defaultdict(list)
for node, neighbours in graph.items():
for neighbour in neighbours:
transposed_graph[neighbour].append(node)
# {1: [0], 2: [0, 1], 3: [1]}
answered Apr 3 at 21:02


mkrieger1mkrieger1
1,4541824
1,4541824
1
$begingroup$
Also, it's important to use an entry point in Python - the classicif __name__ == "__main__":
because tools like Sphinx which auto-document code will load the code to reflect what properties and methods each object has. Having the entry point separates the execution of the code, from the code itself (does that make sense?). The code in the current form will always execute, as opposed to having something likedo_transpose(graph)
after the entry point responsible for execution.
$endgroup$
– C. Harley
Apr 4 at 3:54
$begingroup$
Way better answer than what I was expecting and just what I need. I don't come from a programming career, so I'm learning the conventions as I go, so this was really helpful. Thanks a lot to both of you !
$endgroup$
– Juan C
Apr 4 at 15:16
add a comment |
1
$begingroup$
Also, it's important to use an entry point in Python - the classicif __name__ == "__main__":
because tools like Sphinx which auto-document code will load the code to reflect what properties and methods each object has. Having the entry point separates the execution of the code, from the code itself (does that make sense?). The code in the current form will always execute, as opposed to having something likedo_transpose(graph)
after the entry point responsible for execution.
$endgroup$
– C. Harley
Apr 4 at 3:54
$begingroup$
Way better answer than what I was expecting and just what I need. I don't come from a programming career, so I'm learning the conventions as I go, so this was really helpful. Thanks a lot to both of you !
$endgroup$
– Juan C
Apr 4 at 15:16
1
1
$begingroup$
Also, it's important to use an entry point in Python - the classic
if __name__ == "__main__":
because tools like Sphinx which auto-document code will load the code to reflect what properties and methods each object has. Having the entry point separates the execution of the code, from the code itself (does that make sense?). The code in the current form will always execute, as opposed to having something like do_transpose(graph)
after the entry point responsible for execution.$endgroup$
– C. Harley
Apr 4 at 3:54
$begingroup$
Also, it's important to use an entry point in Python - the classic
if __name__ == "__main__":
because tools like Sphinx which auto-document code will load the code to reflect what properties and methods each object has. Having the entry point separates the execution of the code, from the code itself (does that make sense?). The code in the current form will always execute, as opposed to having something like do_transpose(graph)
after the entry point responsible for execution.$endgroup$
– C. Harley
Apr 4 at 3:54
$begingroup$
Way better answer than what I was expecting and just what I need. I don't come from a programming career, so I'm learning the conventions as I go, so this was really helpful. Thanks a lot to both of you !
$endgroup$
– Juan C
Apr 4 at 15:16
$begingroup$
Way better answer than what I was expecting and just what I need. I don't come from a programming career, so I'm learning the conventions as I go, so this was really helpful. Thanks a lot to both of you !
$endgroup$
– Juan C
Apr 4 at 15:16
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216814%2freverse-dictionary-where-values-are-lists%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
30,OEdx flhM0,MHvc,Oo6GKZ8vfBL5,x8v