boost beast message with body_limit
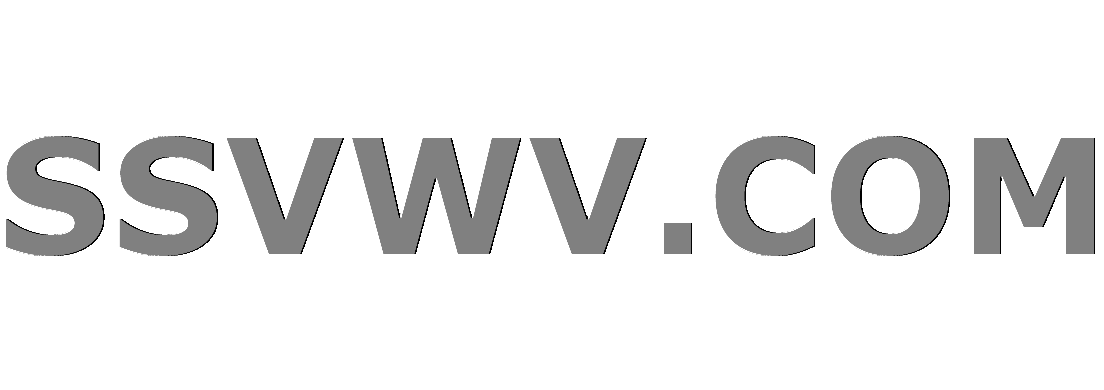
Multi tool use
My starting point is to create a simple downloader code from the boost beast http_client_async example at boost http_client_async. In this scenario i want to write the received body into a file.
So I exchanged the string body into a file_body, to write the received data:
http::response_parser<http::file_body> res_;
And simply rewrote the on_write method to
void on_write( boost::system::error_code ec,
std::size_t bytes_transferred )
{
boost::ignore_unused(bytes_transferred);
if(ec)
return fail(ec, "write");
boost::system::error_code ec_file;
res_.body().open("myTest.txt", boost::beast::file_mode::write, ec_file);
// test for ec_file missing
// Receive the HTTP response
http::async_read(socket_, buffer_, res_,
std::bind(
&session::on_read,
shared_from_this(),
std::placeholders::_1,
std::placeholders::_2));
}
So but now, some of the received data bodies are to big:
read: body limit exceeded
and I try to increase the body limit.
In case of using a parser instead of a message, the size limit of the requested body can be changed with the body_limit()
method.
Is there an easy way to increase the body size limit from a message as well?
c++ boost-beast
add a comment |
My starting point is to create a simple downloader code from the boost beast http_client_async example at boost http_client_async. In this scenario i want to write the received body into a file.
So I exchanged the string body into a file_body, to write the received data:
http::response_parser<http::file_body> res_;
And simply rewrote the on_write method to
void on_write( boost::system::error_code ec,
std::size_t bytes_transferred )
{
boost::ignore_unused(bytes_transferred);
if(ec)
return fail(ec, "write");
boost::system::error_code ec_file;
res_.body().open("myTest.txt", boost::beast::file_mode::write, ec_file);
// test for ec_file missing
// Receive the HTTP response
http::async_read(socket_, buffer_, res_,
std::bind(
&session::on_read,
shared_from_this(),
std::placeholders::_1,
std::placeholders::_2));
}
So but now, some of the received data bodies are to big:
read: body limit exceeded
and I try to increase the body limit.
In case of using a parser instead of a message, the size limit of the requested body can be changed with the body_limit()
method.
Is there an easy way to increase the body size limit from a message as well?
c++ boost-beast
1
to use theresponse_parser<http::file_body>
instead ofresponse<http::string_body>
is the best way to go, I think. It got confused here. A response parser is not a message. When trying to get the body for calling open, res_ needs a call tores_.get().body().open("myTest.txt", boost::beast::file_mode::write, ec_file);
. The response_parserres_
is able to handle the member variable body_limit().
– Tom
May 15 '18 at 12:18
add a comment |
My starting point is to create a simple downloader code from the boost beast http_client_async example at boost http_client_async. In this scenario i want to write the received body into a file.
So I exchanged the string body into a file_body, to write the received data:
http::response_parser<http::file_body> res_;
And simply rewrote the on_write method to
void on_write( boost::system::error_code ec,
std::size_t bytes_transferred )
{
boost::ignore_unused(bytes_transferred);
if(ec)
return fail(ec, "write");
boost::system::error_code ec_file;
res_.body().open("myTest.txt", boost::beast::file_mode::write, ec_file);
// test for ec_file missing
// Receive the HTTP response
http::async_read(socket_, buffer_, res_,
std::bind(
&session::on_read,
shared_from_this(),
std::placeholders::_1,
std::placeholders::_2));
}
So but now, some of the received data bodies are to big:
read: body limit exceeded
and I try to increase the body limit.
In case of using a parser instead of a message, the size limit of the requested body can be changed with the body_limit()
method.
Is there an easy way to increase the body size limit from a message as well?
c++ boost-beast
My starting point is to create a simple downloader code from the boost beast http_client_async example at boost http_client_async. In this scenario i want to write the received body into a file.
So I exchanged the string body into a file_body, to write the received data:
http::response_parser<http::file_body> res_;
And simply rewrote the on_write method to
void on_write( boost::system::error_code ec,
std::size_t bytes_transferred )
{
boost::ignore_unused(bytes_transferred);
if(ec)
return fail(ec, "write");
boost::system::error_code ec_file;
res_.body().open("myTest.txt", boost::beast::file_mode::write, ec_file);
// test for ec_file missing
// Receive the HTTP response
http::async_read(socket_, buffer_, res_,
std::bind(
&session::on_read,
shared_from_this(),
std::placeholders::_1,
std::placeholders::_2));
}
So but now, some of the received data bodies are to big:
read: body limit exceeded
and I try to increase the body limit.
In case of using a parser instead of a message, the size limit of the requested body can be changed with the body_limit()
method.
Is there an easy way to increase the body size limit from a message as well?
c++ boost-beast
c++ boost-beast
asked May 15 '18 at 11:00
TomTom
112
112
1
to use theresponse_parser<http::file_body>
instead ofresponse<http::string_body>
is the best way to go, I think. It got confused here. A response parser is not a message. When trying to get the body for calling open, res_ needs a call tores_.get().body().open("myTest.txt", boost::beast::file_mode::write, ec_file);
. The response_parserres_
is able to handle the member variable body_limit().
– Tom
May 15 '18 at 12:18
add a comment |
1
to use theresponse_parser<http::file_body>
instead ofresponse<http::string_body>
is the best way to go, I think. It got confused here. A response parser is not a message. When trying to get the body for calling open, res_ needs a call tores_.get().body().open("myTest.txt", boost::beast::file_mode::write, ec_file);
. The response_parserres_
is able to handle the member variable body_limit().
– Tom
May 15 '18 at 12:18
1
1
to use the
response_parser<http::file_body>
instead of response<http::string_body>
is the best way to go, I think. It got confused here. A response parser is not a message. When trying to get the body for calling open, res_ needs a call to res_.get().body().open("myTest.txt", boost::beast::file_mode::write, ec_file);
. The response_parser res_
is able to handle the member variable body_limit().– Tom
May 15 '18 at 12:18
to use the
response_parser<http::file_body>
instead of response<http::string_body>
is the best way to go, I think. It got confused here. A response parser is not a message. When trying to get the body for calling open, res_ needs a call to res_.get().body().open("myTest.txt", boost::beast::file_mode::write, ec_file);
. The response_parser res_
is able to handle the member variable body_limit().– Tom
May 15 '18 at 12:18
add a comment |
1 Answer
1
active
oldest
votes
Beast's HTTP interfaces are grouped into layers. The first layer has the message-oriented stream algorithms, which operate on an HTTP message container. These are designed for simplicity, but allow for very little customization. The next layer is the serializer/parser oriented interface. This requires maintaining the lifetime of a serializer (for writing) or a parser (for reading) for the duration of the stream operation. It is a little more complex but correspondingly allows for more customization.
Adjusting the maximum size of the message body requires using the parser-oriented interface, as you have noted in your comment:
namespace http = boost::beast::http;
http::response_parser<http::file_body> parser;
// Allow for an unlimited body size
parser.body_limit((std::numeric_limits<std::uint64_t>::max)());
...
http::read(socket, buffer, parser);
1
Thanks for the clarification! I worked with the code for a while now. Reading the code + ducumentation API helps more than the documentation examples at the moment as the possibilities to use beast are really huge!
– Tom
Jun 4 '18 at 13:08
I think std::numeric_limits<std::uint64_t>::max should be std::numeric_limits<std::uint64_t>::max().
– sank
Nov 22 '18 at 7:10
Yes, you are right! Also, the function name should be surrounded with parenthesis in case someone definesmax
as a macro (tsk tsk Windows.h)
– Vinnie Falco
Nov 22 '18 at 11:07
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f50348516%2fboost-beast-message-with-body-limit%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Beast's HTTP interfaces are grouped into layers. The first layer has the message-oriented stream algorithms, which operate on an HTTP message container. These are designed for simplicity, but allow for very little customization. The next layer is the serializer/parser oriented interface. This requires maintaining the lifetime of a serializer (for writing) or a parser (for reading) for the duration of the stream operation. It is a little more complex but correspondingly allows for more customization.
Adjusting the maximum size of the message body requires using the parser-oriented interface, as you have noted in your comment:
namespace http = boost::beast::http;
http::response_parser<http::file_body> parser;
// Allow for an unlimited body size
parser.body_limit((std::numeric_limits<std::uint64_t>::max)());
...
http::read(socket, buffer, parser);
1
Thanks for the clarification! I worked with the code for a while now. Reading the code + ducumentation API helps more than the documentation examples at the moment as the possibilities to use beast are really huge!
– Tom
Jun 4 '18 at 13:08
I think std::numeric_limits<std::uint64_t>::max should be std::numeric_limits<std::uint64_t>::max().
– sank
Nov 22 '18 at 7:10
Yes, you are right! Also, the function name should be surrounded with parenthesis in case someone definesmax
as a macro (tsk tsk Windows.h)
– Vinnie Falco
Nov 22 '18 at 11:07
add a comment |
Beast's HTTP interfaces are grouped into layers. The first layer has the message-oriented stream algorithms, which operate on an HTTP message container. These are designed for simplicity, but allow for very little customization. The next layer is the serializer/parser oriented interface. This requires maintaining the lifetime of a serializer (for writing) or a parser (for reading) for the duration of the stream operation. It is a little more complex but correspondingly allows for more customization.
Adjusting the maximum size of the message body requires using the parser-oriented interface, as you have noted in your comment:
namespace http = boost::beast::http;
http::response_parser<http::file_body> parser;
// Allow for an unlimited body size
parser.body_limit((std::numeric_limits<std::uint64_t>::max)());
...
http::read(socket, buffer, parser);
1
Thanks for the clarification! I worked with the code for a while now. Reading the code + ducumentation API helps more than the documentation examples at the moment as the possibilities to use beast are really huge!
– Tom
Jun 4 '18 at 13:08
I think std::numeric_limits<std::uint64_t>::max should be std::numeric_limits<std::uint64_t>::max().
– sank
Nov 22 '18 at 7:10
Yes, you are right! Also, the function name should be surrounded with parenthesis in case someone definesmax
as a macro (tsk tsk Windows.h)
– Vinnie Falco
Nov 22 '18 at 11:07
add a comment |
Beast's HTTP interfaces are grouped into layers. The first layer has the message-oriented stream algorithms, which operate on an HTTP message container. These are designed for simplicity, but allow for very little customization. The next layer is the serializer/parser oriented interface. This requires maintaining the lifetime of a serializer (for writing) or a parser (for reading) for the duration of the stream operation. It is a little more complex but correspondingly allows for more customization.
Adjusting the maximum size of the message body requires using the parser-oriented interface, as you have noted in your comment:
namespace http = boost::beast::http;
http::response_parser<http::file_body> parser;
// Allow for an unlimited body size
parser.body_limit((std::numeric_limits<std::uint64_t>::max)());
...
http::read(socket, buffer, parser);
Beast's HTTP interfaces are grouped into layers. The first layer has the message-oriented stream algorithms, which operate on an HTTP message container. These are designed for simplicity, but allow for very little customization. The next layer is the serializer/parser oriented interface. This requires maintaining the lifetime of a serializer (for writing) or a parser (for reading) for the duration of the stream operation. It is a little more complex but correspondingly allows for more customization.
Adjusting the maximum size of the message body requires using the parser-oriented interface, as you have noted in your comment:
namespace http = boost::beast::http;
http::response_parser<http::file_body> parser;
// Allow for an unlimited body size
parser.body_limit((std::numeric_limits<std::uint64_t>::max)());
...
http::read(socket, buffer, parser);
edited Nov 22 '18 at 11:06
answered May 15 '18 at 22:27
Vinnie FalcoVinnie Falco
2,7631432
2,7631432
1
Thanks for the clarification! I worked with the code for a while now. Reading the code + ducumentation API helps more than the documentation examples at the moment as the possibilities to use beast are really huge!
– Tom
Jun 4 '18 at 13:08
I think std::numeric_limits<std::uint64_t>::max should be std::numeric_limits<std::uint64_t>::max().
– sank
Nov 22 '18 at 7:10
Yes, you are right! Also, the function name should be surrounded with parenthesis in case someone definesmax
as a macro (tsk tsk Windows.h)
– Vinnie Falco
Nov 22 '18 at 11:07
add a comment |
1
Thanks for the clarification! I worked with the code for a while now. Reading the code + ducumentation API helps more than the documentation examples at the moment as the possibilities to use beast are really huge!
– Tom
Jun 4 '18 at 13:08
I think std::numeric_limits<std::uint64_t>::max should be std::numeric_limits<std::uint64_t>::max().
– sank
Nov 22 '18 at 7:10
Yes, you are right! Also, the function name should be surrounded with parenthesis in case someone definesmax
as a macro (tsk tsk Windows.h)
– Vinnie Falco
Nov 22 '18 at 11:07
1
1
Thanks for the clarification! I worked with the code for a while now. Reading the code + ducumentation API helps more than the documentation examples at the moment as the possibilities to use beast are really huge!
– Tom
Jun 4 '18 at 13:08
Thanks for the clarification! I worked with the code for a while now. Reading the code + ducumentation API helps more than the documentation examples at the moment as the possibilities to use beast are really huge!
– Tom
Jun 4 '18 at 13:08
I think std::numeric_limits<std::uint64_t>::max should be std::numeric_limits<std::uint64_t>::max().
– sank
Nov 22 '18 at 7:10
I think std::numeric_limits<std::uint64_t>::max should be std::numeric_limits<std::uint64_t>::max().
– sank
Nov 22 '18 at 7:10
Yes, you are right! Also, the function name should be surrounded with parenthesis in case someone defines
max
as a macro (tsk tsk Windows.h)– Vinnie Falco
Nov 22 '18 at 11:07
Yes, you are right! Also, the function name should be surrounded with parenthesis in case someone defines
max
as a macro (tsk tsk Windows.h)– Vinnie Falco
Nov 22 '18 at 11:07
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f50348516%2fboost-beast-message-with-body-limit%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XyHYtpXVQaB32ixSie8ht fgrnyIDCTDmcSX FP,g0x,0gCv,IuaBr E6d,6qI1Z,AP
1
to use the
response_parser<http::file_body>
instead ofresponse<http::string_body>
is the best way to go, I think. It got confused here. A response parser is not a message. When trying to get the body for calling open, res_ needs a call tores_.get().body().open("myTest.txt", boost::beast::file_mode::write, ec_file);
. The response_parserres_
is able to handle the member variable body_limit().– Tom
May 15 '18 at 12:18