Finding occurences of a digit in a number by recursion in Matlab
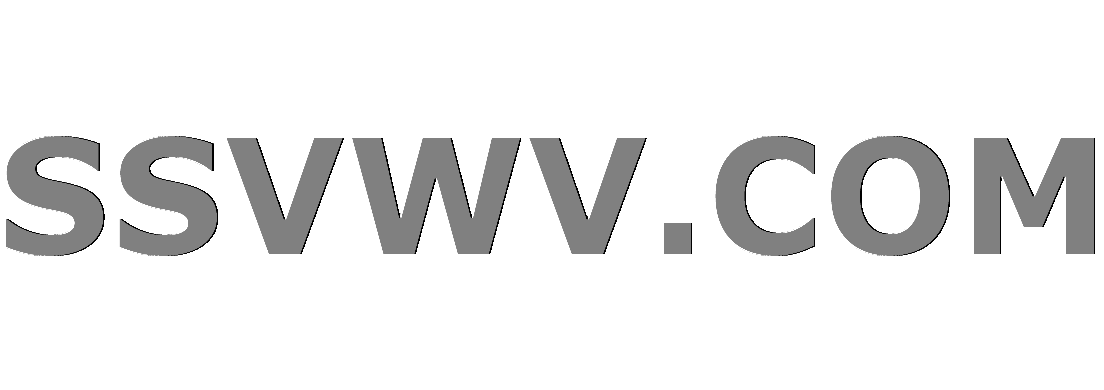
Multi tool use
up vote
0
down vote
favorite
This recursive function takes two input arguments, The first (A) is a number and the second (n) is a digit, checks the occurrence of n in A. (A is updated by removing its last digit in each recursion). it seems like the recursion is infinite and the base case (A == 0) is not valid but why.
function counts = countn(A,n)
if (A == 0)
counts= 0;
end
if (n == mod(A,10))
disp(A);
disp(floor(A/10));
disp(mod(A,10));
B = floor(A/10);
counts = countn(B,n) + 1;
else
B = floor(A/10);
countn(B,n);
end
end
matlab
add a comment |
up vote
0
down vote
favorite
This recursive function takes two input arguments, The first (A) is a number and the second (n) is a digit, checks the occurrence of n in A. (A is updated by removing its last digit in each recursion). it seems like the recursion is infinite and the base case (A == 0) is not valid but why.
function counts = countn(A,n)
if (A == 0)
counts= 0;
end
if (n == mod(A,10))
disp(A);
disp(floor(A/10));
disp(mod(A,10));
B = floor(A/10);
counts = countn(B,n) + 1;
else
B = floor(A/10);
countn(B,n);
end
end
matlab
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
This recursive function takes two input arguments, The first (A) is a number and the second (n) is a digit, checks the occurrence of n in A. (A is updated by removing its last digit in each recursion). it seems like the recursion is infinite and the base case (A == 0) is not valid but why.
function counts = countn(A,n)
if (A == 0)
counts= 0;
end
if (n == mod(A,10))
disp(A);
disp(floor(A/10));
disp(mod(A,10));
B = floor(A/10);
counts = countn(B,n) + 1;
else
B = floor(A/10);
countn(B,n);
end
end
matlab
This recursive function takes two input arguments, The first (A) is a number and the second (n) is a digit, checks the occurrence of n in A. (A is updated by removing its last digit in each recursion). it seems like the recursion is infinite and the base case (A == 0) is not valid but why.
function counts = countn(A,n)
if (A == 0)
counts= 0;
end
if (n == mod(A,10))
disp(A);
disp(floor(A/10));
disp(mod(A,10));
B = floor(A/10);
counts = countn(B,n) + 1;
else
B = floor(A/10);
countn(B,n);
end
end
matlab
matlab
asked Nov 17 at 10:47
Khalil Kaddoura
132
132
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
It does not stop because it first evaluates the first if statement if( A == 0)
and afterward the if (n == mod(A,10))
where it jumps in the else branch and recursively calls the function again. So it does not stop in the first if
statement as you likely expected it to do.
something like this should work:
function counts = countn(A,n)
if (A == 0)
counts = 0;
elseif (n == mod(A,10))
disp(A);
disp(floor(A/10));
disp(mod(A,10));
B = floor(A/10);
counts = countn(B,n) + 1;
else
B = floor(A/10);
counts = countn(B,n);
end
end
You also have to update count counts
variable in the else
branch to avoid the uninitialized use of variables.
Have a look at how to use a debugger manual. Simply click on the line number inside your function and run your code. Use the F10 and F11 keys to evaluate your code line by line. This helps you understand what your program does.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
It does not stop because it first evaluates the first if statement if( A == 0)
and afterward the if (n == mod(A,10))
where it jumps in the else branch and recursively calls the function again. So it does not stop in the first if
statement as you likely expected it to do.
something like this should work:
function counts = countn(A,n)
if (A == 0)
counts = 0;
elseif (n == mod(A,10))
disp(A);
disp(floor(A/10));
disp(mod(A,10));
B = floor(A/10);
counts = countn(B,n) + 1;
else
B = floor(A/10);
counts = countn(B,n);
end
end
You also have to update count counts
variable in the else
branch to avoid the uninitialized use of variables.
Have a look at how to use a debugger manual. Simply click on the line number inside your function and run your code. Use the F10 and F11 keys to evaluate your code line by line. This helps you understand what your program does.
add a comment |
up vote
1
down vote
accepted
It does not stop because it first evaluates the first if statement if( A == 0)
and afterward the if (n == mod(A,10))
where it jumps in the else branch and recursively calls the function again. So it does not stop in the first if
statement as you likely expected it to do.
something like this should work:
function counts = countn(A,n)
if (A == 0)
counts = 0;
elseif (n == mod(A,10))
disp(A);
disp(floor(A/10));
disp(mod(A,10));
B = floor(A/10);
counts = countn(B,n) + 1;
else
B = floor(A/10);
counts = countn(B,n);
end
end
You also have to update count counts
variable in the else
branch to avoid the uninitialized use of variables.
Have a look at how to use a debugger manual. Simply click on the line number inside your function and run your code. Use the F10 and F11 keys to evaluate your code line by line. This helps you understand what your program does.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
It does not stop because it first evaluates the first if statement if( A == 0)
and afterward the if (n == mod(A,10))
where it jumps in the else branch and recursively calls the function again. So it does not stop in the first if
statement as you likely expected it to do.
something like this should work:
function counts = countn(A,n)
if (A == 0)
counts = 0;
elseif (n == mod(A,10))
disp(A);
disp(floor(A/10));
disp(mod(A,10));
B = floor(A/10);
counts = countn(B,n) + 1;
else
B = floor(A/10);
counts = countn(B,n);
end
end
You also have to update count counts
variable in the else
branch to avoid the uninitialized use of variables.
Have a look at how to use a debugger manual. Simply click on the line number inside your function and run your code. Use the F10 and F11 keys to evaluate your code line by line. This helps you understand what your program does.
It does not stop because it first evaluates the first if statement if( A == 0)
and afterward the if (n == mod(A,10))
where it jumps in the else branch and recursively calls the function again. So it does not stop in the first if
statement as you likely expected it to do.
something like this should work:
function counts = countn(A,n)
if (A == 0)
counts = 0;
elseif (n == mod(A,10))
disp(A);
disp(floor(A/10));
disp(mod(A,10));
B = floor(A/10);
counts = countn(B,n) + 1;
else
B = floor(A/10);
counts = countn(B,n);
end
end
You also have to update count counts
variable in the else
branch to avoid the uninitialized use of variables.
Have a look at how to use a debugger manual. Simply click on the line number inside your function and run your code. Use the F10 and F11 keys to evaluate your code line by line. This helps you understand what your program does.
answered Nov 17 at 11:30
user7431005
837214
837214
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53350469%2ffinding-occurences-of-a-digit-in-a-number-by-recursion-in-matlab%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
p VPzq77ZRk8i EX7QVZcw8nCl0c1 3RD,eJY98WOqAPvZZH2YVwuK,Ss