Find the needle in the haystack
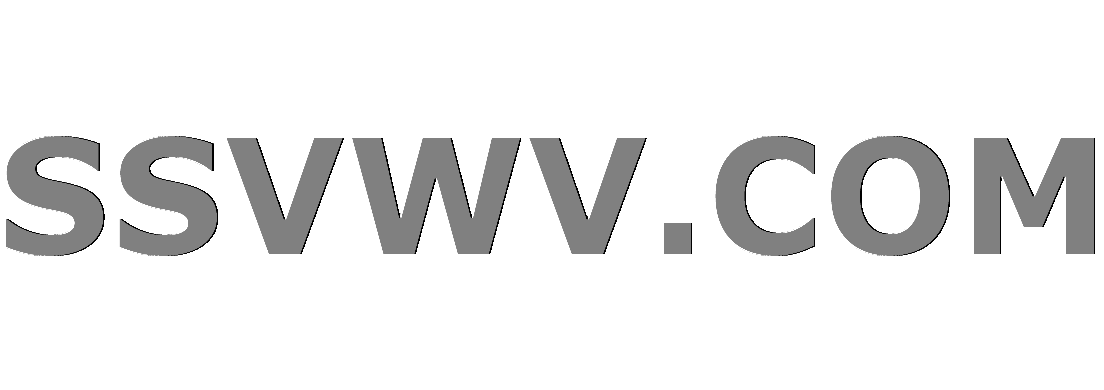
Multi tool use
$begingroup$
Given a rectangular haystack of size at least 2x2 composed of all the same printable ASCII characters, output the location (counting from the top-left) of the needle which is a different character.
For example, if the following haystack is input:
#####
###N#
#####
#####
The output should be 3,1
when zero-indexed (what I'll be using in this challenge) or 4,2
when one-indexed.
The haystack can be composed of any printable ASCII character:
^^^
^^^
^N^
^^^
^^^
^^^
output: 1,2
and the needle will be any other printable ASCII character:
jjjjjj
j@jjjj
jjjjjj
output 1,1
It's also possible to have a needle in the corner:
Z8
88
output 0,0
88
8Z
output 1,1
or to have the needle at the edge:
>>>>>>>>>>
>>>>>>>>>:
>>>>>>>>>>
output 9,1
Rules and Clarifications
- Input and output can be given by any convenient method. This means you can take input as a list of list of characters, as a single string, etc.
- You can print the result to STDOUT or return it as a function result. Please state in your submission what order the output is in (i.e., horizontal then vertical, as used in the challenge, or vice versa).
- Either a full program or a function are acceptable.
- You do not get to pick which characters to use. That's the challenge.
- The haystack is guaranteed to be at least 2x2 in size, so it's unambiguous which is the needle and which is the hay.
Standard loopholes are forbidden.- This is code-golf so all usual golfing rules apply, and the shortest code (in bytes) wins.
code-golf string
$endgroup$
|
show 2 more comments
$begingroup$
Given a rectangular haystack of size at least 2x2 composed of all the same printable ASCII characters, output the location (counting from the top-left) of the needle which is a different character.
For example, if the following haystack is input:
#####
###N#
#####
#####
The output should be 3,1
when zero-indexed (what I'll be using in this challenge) or 4,2
when one-indexed.
The haystack can be composed of any printable ASCII character:
^^^
^^^
^N^
^^^
^^^
^^^
output: 1,2
and the needle will be any other printable ASCII character:
jjjjjj
j@jjjj
jjjjjj
output 1,1
It's also possible to have a needle in the corner:
Z8
88
output 0,0
88
8Z
output 1,1
or to have the needle at the edge:
>>>>>>>>>>
>>>>>>>>>:
>>>>>>>>>>
output 9,1
Rules and Clarifications
- Input and output can be given by any convenient method. This means you can take input as a list of list of characters, as a single string, etc.
- You can print the result to STDOUT or return it as a function result. Please state in your submission what order the output is in (i.e., horizontal then vertical, as used in the challenge, or vice versa).
- Either a full program or a function are acceptable.
- You do not get to pick which characters to use. That's the challenge.
- The haystack is guaranteed to be at least 2x2 in size, so it's unambiguous which is the needle and which is the hay.
Standard loopholes are forbidden.- This is code-golf so all usual golfing rules apply, and the shortest code (in bytes) wins.
code-golf string
$endgroup$
$begingroup$
Suggested test case:88n8Z
(with any two characters of course).
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
Can we take input as a multi-dimensional array? i.e. [ ['#','#','#','#','#'], ['#','#','#','N','#'], ['#','#','#','#','#'], ['#','#','#','#','#'] ];
$endgroup$
– gwaugh
yesterday
2
$begingroup$
@gwaugh Like a list of list of characters? Yes, that's fine (and explicitly called out as OK).
$endgroup$
– AdmBorkBork
yesterday
2
$begingroup$
Can we take input as a pair of a string without newlines and the width (or height) of the haystack? i.e.("########N###########", 5)
$endgroup$
– someone
yesterday
2
$begingroup$
@someone Yes, though it doesn't have a real quorum, I feel that should be allowed.
$endgroup$
– AdmBorkBork
yesterday
|
show 2 more comments
$begingroup$
Given a rectangular haystack of size at least 2x2 composed of all the same printable ASCII characters, output the location (counting from the top-left) of the needle which is a different character.
For example, if the following haystack is input:
#####
###N#
#####
#####
The output should be 3,1
when zero-indexed (what I'll be using in this challenge) or 4,2
when one-indexed.
The haystack can be composed of any printable ASCII character:
^^^
^^^
^N^
^^^
^^^
^^^
output: 1,2
and the needle will be any other printable ASCII character:
jjjjjj
j@jjjj
jjjjjj
output 1,1
It's also possible to have a needle in the corner:
Z8
88
output 0,0
88
8Z
output 1,1
or to have the needle at the edge:
>>>>>>>>>>
>>>>>>>>>:
>>>>>>>>>>
output 9,1
Rules and Clarifications
- Input and output can be given by any convenient method. This means you can take input as a list of list of characters, as a single string, etc.
- You can print the result to STDOUT or return it as a function result. Please state in your submission what order the output is in (i.e., horizontal then vertical, as used in the challenge, or vice versa).
- Either a full program or a function are acceptable.
- You do not get to pick which characters to use. That's the challenge.
- The haystack is guaranteed to be at least 2x2 in size, so it's unambiguous which is the needle and which is the hay.
Standard loopholes are forbidden.- This is code-golf so all usual golfing rules apply, and the shortest code (in bytes) wins.
code-golf string
$endgroup$
Given a rectangular haystack of size at least 2x2 composed of all the same printable ASCII characters, output the location (counting from the top-left) of the needle which is a different character.
For example, if the following haystack is input:
#####
###N#
#####
#####
The output should be 3,1
when zero-indexed (what I'll be using in this challenge) or 4,2
when one-indexed.
The haystack can be composed of any printable ASCII character:
^^^
^^^
^N^
^^^
^^^
^^^
output: 1,2
and the needle will be any other printable ASCII character:
jjjjjj
j@jjjj
jjjjjj
output 1,1
It's also possible to have a needle in the corner:
Z8
88
output 0,0
88
8Z
output 1,1
or to have the needle at the edge:
>>>>>>>>>>
>>>>>>>>>:
>>>>>>>>>>
output 9,1
Rules and Clarifications
- Input and output can be given by any convenient method. This means you can take input as a list of list of characters, as a single string, etc.
- You can print the result to STDOUT or return it as a function result. Please state in your submission what order the output is in (i.e., horizontal then vertical, as used in the challenge, or vice versa).
- Either a full program or a function are acceptable.
- You do not get to pick which characters to use. That's the challenge.
- The haystack is guaranteed to be at least 2x2 in size, so it's unambiguous which is the needle and which is the hay.
Standard loopholes are forbidden.- This is code-golf so all usual golfing rules apply, and the shortest code (in bytes) wins.
code-golf string
code-golf string
edited yesterday
AdmBorkBork
asked yesterday


AdmBorkBorkAdmBorkBork
26.9k365232
26.9k365232
$begingroup$
Suggested test case:88n8Z
(with any two characters of course).
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
Can we take input as a multi-dimensional array? i.e. [ ['#','#','#','#','#'], ['#','#','#','N','#'], ['#','#','#','#','#'], ['#','#','#','#','#'] ];
$endgroup$
– gwaugh
yesterday
2
$begingroup$
@gwaugh Like a list of list of characters? Yes, that's fine (and explicitly called out as OK).
$endgroup$
– AdmBorkBork
yesterday
2
$begingroup$
Can we take input as a pair of a string without newlines and the width (or height) of the haystack? i.e.("########N###########", 5)
$endgroup$
– someone
yesterday
2
$begingroup$
@someone Yes, though it doesn't have a real quorum, I feel that should be allowed.
$endgroup$
– AdmBorkBork
yesterday
|
show 2 more comments
$begingroup$
Suggested test case:88n8Z
(with any two characters of course).
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
Can we take input as a multi-dimensional array? i.e. [ ['#','#','#','#','#'], ['#','#','#','N','#'], ['#','#','#','#','#'], ['#','#','#','#','#'] ];
$endgroup$
– gwaugh
yesterday
2
$begingroup$
@gwaugh Like a list of list of characters? Yes, that's fine (and explicitly called out as OK).
$endgroup$
– AdmBorkBork
yesterday
2
$begingroup$
Can we take input as a pair of a string without newlines and the width (or height) of the haystack? i.e.("########N###########", 5)
$endgroup$
– someone
yesterday
2
$begingroup$
@someone Yes, though it doesn't have a real quorum, I feel that should be allowed.
$endgroup$
– AdmBorkBork
yesterday
$begingroup$
Suggested test case:
88n8Z
(with any two characters of course).$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
Suggested test case:
88n8Z
(with any two characters of course).$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
Can we take input as a multi-dimensional array? i.e. [ ['#','#','#','#','#'], ['#','#','#','N','#'], ['#','#','#','#','#'], ['#','#','#','#','#'] ];
$endgroup$
– gwaugh
yesterday
$begingroup$
Can we take input as a multi-dimensional array? i.e. [ ['#','#','#','#','#'], ['#','#','#','N','#'], ['#','#','#','#','#'], ['#','#','#','#','#'] ];
$endgroup$
– gwaugh
yesterday
2
2
$begingroup$
@gwaugh Like a list of list of characters? Yes, that's fine (and explicitly called out as OK).
$endgroup$
– AdmBorkBork
yesterday
$begingroup$
@gwaugh Like a list of list of characters? Yes, that's fine (and explicitly called out as OK).
$endgroup$
– AdmBorkBork
yesterday
2
2
$begingroup$
Can we take input as a pair of a string without newlines and the width (or height) of the haystack? i.e.
("########N###########", 5)
$endgroup$
– someone
yesterday
$begingroup$
Can we take input as a pair of a string without newlines and the width (or height) of the haystack? i.e.
("########N###########", 5)
$endgroup$
– someone
yesterday
2
2
$begingroup$
@someone Yes, though it doesn't have a real quorum, I feel that should be allowed.
$endgroup$
– AdmBorkBork
yesterday
$begingroup$
@someone Yes, though it doesn't have a real quorum, I feel that should be allowed.
$endgroup$
– AdmBorkBork
yesterday
|
show 2 more comments
29 Answers
29
active
oldest
votes
$begingroup$
R, 49 47 44 bytes
function(m,`?`=which)m==names(?table(m)<2)?T
Try it online!
Takes input as a matrix, returns 1-indexed coordinates
$endgroup$
3
$begingroup$
Thatwhich
assignment is disgracefully smooth.
$endgroup$
– CriminallyVulgar
yesterday
3
$begingroup$
I was so excited to try this challenge in R, then I saw this and decided to cry in awe instead
$endgroup$
– Sumner18
yesterday
add a comment |
$begingroup$
Perl 6, 41 38 37 bytes
3 bytes saved thanks to @nwellnhof.
1 byte saved thanks to Jo King.
{map {[+] ^∞Z*!<<.&[Z~~]},$_,.&[Z]}
Try it online!
Explanation
It takes the input as a list of lists of characters and returns list of length 2 containing zero-based X and Y coordinates of the needle.
It works by applying the block {[+] ^∞ Z* !<<.&[Z~~]}
on the input and on its transpose. .&[Z~~]
goes through all columns of the argument and returns True
if all the elements are the same, False
otherwise. We then negate all the values (so we have a list with one bool per column, where the bool answers the question "Is the needle in that column?"), multiply them element-wise with a sequence 0,1,2,... (True = 1
and False = 0
) and sum the list, so the result of the whole block is the 0-based number of the column where the needle was found.
Nwellnhof's better approach, Perl 6, 34 bytes
{map *.first(:k,*.Set>1),.&[Z],$_}
Try it online!
Explanation
Generally the same approach, just more effective. It still uses a block on the array and its transpose, but now the block converts all rows intoSets
and checks for the number of elements. The first
function then gives index (due to the :k
) of the first row that contained more than 1 element. Because of that, the order of $_
and .&[Z]
needed to be swapped.
$endgroup$
$begingroup$
Nice approach! 34 bytes withfirst(:k)
,Set
and.&[Z]
.
$endgroup$
– nwellnhof
yesterday
$begingroup$
@nwellnhof, very well done. You basically found what I wanted to find but failed to do that :—). (Also I had no idea that you could write.&[Z]
.)
$endgroup$
– Ramillies
yesterday
$begingroup$
In general,.&[op]
doesn't seem to be equivalent to[op] $_
but it works withZ
for some reason.
$endgroup$
– nwellnhof
yesterday
$begingroup$
@JoKing, thanks!
$endgroup$
– Ramillies
14 hours ago
add a comment |
$begingroup$
05AB1E, 9 6 bytes
Saved 3 bytes switching input format.
Input is taken as a string and a row-length.
Output is a zero-based list of the form [y, x]
D.mks‰
Try it online!
or as a Test Suite
Explanation
D # duplicate the input string
.m # get the least frequent character
k # get its index in the string
s # swap the row length to the top of the stack
‰ # divmod the index of the least frequent char with the row length
$endgroup$
$begingroup$
Dang, you beat me to it. Was working on an answer. Had just finished a 13-byter. But yours is way better, so +1 instead. :) Completely forgot about.m
..
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Yeah. I don't think I've ever used.m
before, but I was reasonably sure I'd seen it at some point :)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
Python 2, 57 bytes
lambda m:[map(len,map(set,a)).index(2)for a in zip(*m),m]
Try it online!
$endgroup$
add a comment |
$begingroup$
Brachylog, 20 bytes
c≡ᵍ∋Ȯ&;I∋₎;J∋₎gȮ∧I;J
Try it online!
Outputs [I,J]
, where I
is the row index and J
the column index, both 0-indexed.
Stupidely long, but getting indexes in Brachylog is usually very verbose.
Explanation
c Concatenate the Input into a single string
≡ᵍ Group identical characters together
∋Ȯ Ȯ is a list of One element, which is the needle character
&;I∋₎ Take the Ith row of the Input
;J∋₎ Take the Jth character of the Ith row
gȮ That character, when wrapped in a list, is Ȯ
∧I;J The output is the list [I,J]
$endgroup$
add a comment |
$begingroup$
Jelly, 5 bytes
Outputs [height, width] (1-indexed).
ŒĠLÐṂ
Try it online!
ŒĠLÐṂ – Monadic link / Full program. Takes a list of strings M as input.
ŒĠ – Group the multidimensional indices by their values (treating M as a matrix).
LÐṂ – And retrieve the shortest group of indices (those of the unique character).
Jelly, 5 bytes
ŒĠḊÐḟ
Try it online!
$endgroup$
add a comment |
$begingroup$
Jelly, 4 bytes
Maybe this could've just been a comment for Mr. Xcoder it is pretty similar...
ŒĠEƇ
A monadic link accepting the matrix of characters which yields a list of one item, the 1-indexed (row, column) co-ordinate from top-left.
(...As a full program given an argument formatted such that parsing results in a list of lists of characters -- that is a list of strings in Python format -- the single coordinate is printed.)
Try it online!
How?
ŒĠEƇ - Link: matrix, M
ŒĠ - multi-dimensional indices grouped by Value
- ...due to the 2*2 minimum size and one needle this will be a list of two lists one
- of which will have length one (the needle coordinates as a pair) and the other
- containing all other coordinates as pairs
Ƈ - filter keeping those for which this is truthy:
E - all equal?
- ... 1 for the list of length 1, 0 for the list of at least 3 non-equal coordinates
$endgroup$
1
$begingroup$
Well... this seems borderline, since theEƇ
is clever.
$endgroup$
– Erik the Outgolfer
yesterday
add a comment |
$begingroup$
Python 3, 75 bytes
import numpy as n
def f(x):x=x.view('uint32');return n.where(x-n.median(x))
Try it online!
This assumes that the input is a NumPy array. The output is zero-indexed, and first vertical, then horizontal.
It converts the input from char
to uint32
then calculates the median of the array, which will be the haystack character. We subtract that from the array, which makes the needle the only non-zero element. Finally, return the index of that element with numpy.where()
.
$endgroup$
1
$begingroup$
Since you know the input will be ASCII (i.e. fits in a byte) why not useuint8
for one byte less?
$endgroup$
– Draconis
yesterday
1
$begingroup$
Language has to be "Python 3 + numpy" here since numpy isn't included with the normal Python distribution
$endgroup$
– ASCII-only
18 hours ago
add a comment |
$begingroup$
MATL, 12 8 bytes
tX:XM-&f
Try it online!
Using the mode
function as the majority-detector. Returns 1-based indices.
t % duplicate the input
X: % turn the copy into a linear array
XM % find the arithmetic mode of that (the 'haystack' character)
- % Subtract that from the original input
&f % find the position of the non-zero value in that result
-4 characters thanks to @LuisMendo
$endgroup$
$begingroup$
I think you can shorten totX:XM-&f
(two-output version off
)
$endgroup$
– Luis Mendo
yesterday
1
$begingroup$
@LuisMendo Thanks. I don't think I knew about the 2 output version offind
, even in MATLAB. (Hi, btw!)
$endgroup$
– sundar
yesterday
add a comment |
$begingroup$
PHP, 99 85 bytes
Using string without newlines and the width (or height) ('########N###########', 5
) as input.
- -5 bytes by removing chr() call, props to @Titus
- -9 bytes by taking input as two function args, also props to @Titus
function($a,$l){return[($p=strpos($a,array_flip(count_chars($a,1))[1]))%$l,$p/$l|0];}
Try it online!
Ungolfed:
function need_hay( $a, $l ) {
// identify the "needle" by counting the chars and
// looking for the char with exactly 1 occurrence
// note: this is 1 byte shorter than using array_search()
$n = array_flip( count_chars( $a, 1 ) )[1];
// find the location in the input string
$p = strpos( $a, $n );
// row is location divided by row length, rounded down
$r = floor( $p / $l );
// column is remainder of location divided by row length
$c = $p % $l;
return array( $c, $r );
}
Output:
#####
###N#
#####
#####
[3,1]
^^^
^^^
^N^
^^^
^^^
^^^
[1,2]
jjjjjj
j@jjjj
jjjjjj
[1,1]
$endgroup$
1
$begingroup$
1) no need forchr
: If the second parameter for strpos is an integer, it will be interpreted as an ASCII code. -> -5 bytes. 2) Two function parameters$s,$w
can save another 9 bytes.
$endgroup$
– Titus
yesterday
$begingroup$
@Titus, removing the chr() that's brilliant. Thx! The func params did occur to me too, I just didn't want to run afowl of input req's. I'll clarify w/OP.
$endgroup$
– gwaugh
yesterday
add a comment |
$begingroup$
Wolfram Language 37 58 bytes
My earlier entry did not correctly handle the case where the "odd character out" was at the upper left corner of the matrix. This does.
#~Position~Keys[TakeSmallest[Counts@Flatten@#,1]][[1]]&
Counts@Flatten@#
lists how many of each character are in the array, #
.
TakeSmallest[...,1]
returns the least frequent count, in the form of an association rule such as <| "Z"->1|>
Keys...[[1]]
returns the "key" to the only item in the association, that of the least used character. ("Z" in the present case)
#~Position~...
returns then position of the key in the original matrix, #
.
$endgroup$
add a comment |
$begingroup$
Java 8, 132 111 bytes
m->{int c=m[0][0],i=0,j;for(c=m[1][0]!=c?m[1][1]:c;;i++)for(j=m[i].length;j-->0;)if(m[i][j]!=c)return i+","+j;}
-8 bytes (and -13 more implicitly) thanks to @dana.
Input as character-matrix.
Try it online.
Explanation:
m->{ // Method with char-matrix parameter and String return-type
int c=m[0][0], // Character to check, starting at the one at position 0,0
i=0,j; // Index integers
for(c=m[1][0]!=c? // If the second character does not equal the first:
m[1][1] // Use the character at position 1,1 instead
:c; // Else: keep the character the same
;i++) // Loop `i` from 0 indefinitely upwards:
for(j=m[i].length;j-->0;)
// Inner loop `j` in the range [0, amount_of_columns):
if(m[i][j]!=c) // If the `i,j`'th character doesn't equal our character to check:
return i+","+j;}// Return `i,j` as result
$endgroup$
1
$begingroup$
124 - the finalreturn
statement should never get hit. There might be a better way to keep the outer loop going?
$endgroup$
– dana
7 hours ago
$begingroup$
@dana Thanks! As for: "There might be a better way to keep the outer loop going?", there certainly is; just removing it so it becomes an infinite loop. And then thereturn"";
is unreachable and can be removed as well. :D So -21 bytes thanks to you.
$endgroup$
– Kevin Cruijssen
6 hours ago
$begingroup$
Interesting... I had tried removing the outer loop condition and was getting anunreachable code
error. Didn't know that removing the finalreturn
was the fix.
$endgroup$
– dana
5 hours ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 109 108 bytes
First() => Last() for -1 byte
(a,w)=>{var d=a.Where(b=>b!=a[0]).Select(b=>a.IndexOf(b));return d.Count()>1?(0,0):(d.Last()%w,d.Last()/w);}
Try it online!
$endgroup$
$begingroup$
(a,w)=>
toa=>w=>
saves 1 byte.
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
You can try it here
$endgroup$
– Embodiment of Ignorance
yesterday
add a comment |
$begingroup$
Python 3, 93 bytes
def f(s):x=s.find("n")+1;return[(i%x,i//x)for i,c in enumerate(s)if s.count(c)<2and" "<c][0]
Try it online!
Input is taken as a multiline string. Output is 0-indexed
$endgroup$
add a comment |
$begingroup$
JavaScript (ES6), 55 bytes
Takes input as $(s)(w)$, where $s$ is a string and $w$ is the width of the matrix. Returns $[x,y]$.
s=>w=>[(i=s.indexOf(/(.)1+(.)/.exec(s+s)[2]))%w,i/w|0]
Try it online!
JavaScript (ES6), 65 64 bytes
Saved 1 byte thanks to @Neil
Takes input as a matrix of characters. Returns $[x,y]$.
m=>m.some((r,y)=>r.some((c,x)=>!m[p=[x,y],~y&1].includes(c)))&&p
Try it online!
How?
We look for the first character $c$ located at $(x,y)$ which does not appear anywhere in another row $r[Y]$. We can perform this test on any row, as long as $Yne y$. Because the input matrix is guaranteed to be at least $2times 2$, we can simply use $Y=0$ if $y$ is odd or $Y=1$ if $y$ is even.
$endgroup$
1
$begingroup$
~y&1
saves a byte overy&1^1
.
$endgroup$
– Neil
yesterday
add a comment |
$begingroup$
Octave, 40 bytes
@(x){[r,c]=find(x-mode(+x(:))) [c,r]}{2}
Port of @sundar's MATL answer. Output is a two-element vector with 1-based column and row indices.
Try it online!
$endgroup$
add a comment |
$begingroup$
Retina 0.8.2, 41 bytes
s`(?=(.)+1)(.*?¶)*(.*)(?!1|¶).+
$.3,$#2
Try it online! 0-indexed. Explanation:
s`
Allow .
to match newlines. This costs 3 bytes (3rd byte is the ?
before the ¶
) but saves 6 bytes.
(?=(.)+1)
Look ahead for two identical characters. 1
then becomes the hay.
(.*?¶)*
Count the number of newlines before the needle.
(.*)
Capture the hay to the left of the needle.
(?!1|¶)
Ensure that the needle isn't hay or a newline.
.+
Match the rest of the hay so that the result replaces it.
$.3,$#2
Output the width of the left hay and the number of newlines.
$endgroup$
add a comment |
$begingroup$
Python 3, 93 bytes
def k(g):
t=''.join(g)
i,j=t.index({t.count(c):c for c in t}[1]),len(g[0])
return i//j,i%j
Try it online!
I know this could be a lot shorter, but I just wanted to try an approach around this dict
comprehension.
$endgroup$
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 82 bytes
x=>w=>{int y=x.IndexOf(x.GroupBy(c=>c).Last(g=>g.Count()<2).Key);return(y%w,y/w);}
Thanks to dana for shaving off 6 bytes!
Try it online!
Old solution, 106 bytes
n=>m=>{var z=n.Distinct();int d=n.IndexOf(n.Count(c=>c==z.First())>1?z.Last():z.First());return(d%m,d/m);}
Both take input as a string and an integer specifying the amount of columns.
Try it online!
$endgroup$
$begingroup$
@dana never knew thatEnumerable.Last()
accepted a delegate, thanks
$endgroup$
– Embodiment of Ignorance
9 hours ago
add a comment |
$begingroup$
Perl 5 -p00
, 52 bytes
/
/;$w="@+";/^(.)(1|
)+|^/;$_="@+"%$w.$".int"@+"/$w
-1 byte removing unnecessary /s
flag in second regex
TIO
How
-p00
: like-n
but also print, paragraph mode
/
: matches first new line character
/;- so
@+
array size is 1 and contains the position after the newline and$w="@+";
to store the width
/^(.)(1|
: matches either
)+|^/
- first character followed by one or more occurences of (same character or newline)
- or null string (position 0).
- so
@+
array size is one and contains the position of the "needle"
$_=
: assign the default input/argument variable
"@+"%$w
: the column index from 0
.$".
: concatenate with$"
space character and concatenate
int"@+"/$w
: the row index from 0
$endgroup$
add a comment |
$begingroup$
MATL, 11 bytes
tX:YmyYk-&f
Output is row, then column; 1-based.
Try it online!
Explanation
t % Implicit input. Duplicate
X: % Linearize into a column
Ym % Compute mean (characters are converted to ASCII codes)
y % Duplicate from below: pushes input again
Yk % Closest value: gives the input value that is closest to the mean
- % Subtract, element-wise. Gives non-zero for the value farthest from the mean
&f % Two-output find: gives row and column indices of nonzeros. Implicit display
$endgroup$
add a comment |
$begingroup$
Pyth, 15 14 12 bytes
.Dxz-zh.-z{z
Takes input as the length of the row and the input without lines and outputs as [row, column].
Try it here
Explanation
.Dxz-zh.-z{z
.-z{z Subtract one of each character from the input.
h Take the first.
-z Remove all instances from the input.
xz Find the remaining character in the input.
.D Q Take the result divmod the (implicit) length of the row.
Old approach
mxJmt{kdeSJ.TB
Try it here
Explanation
mxJmt{kdeSJ.TB
.TBQ Take the (implicit) input and its transpose...
m d ... and for each...
mt{k ... deduplicate each row...
xJ eSJ ... and find the index of the largest.
$endgroup$
add a comment |
$begingroup$
Charcoal, 40 bytes
≔§⎇⌕θ§θ¹ηθ⁰ζSθW⁼№θζLθ«⊞υωSθ»I⌕Eθ⁼ιζ⁰,ILυ
Try it online! Link is to verbose version of code. I must be doing something wrong because this is almost as long as the Retina answer. Explanation:
≔§⎇⌕θ§θ¹ηθ⁰ζ
Check whether the second character in the first string is also the first character, and take the first character of the first string if so otherwise the first character of the second string if not. This is then the hay.
SθW⁼№θζLθ«⊞υωSθ»
Keep reading strings until a string whose hay is less than its length is found.
I⌕Eθ⁼ιζ⁰,ILυ
Output the position of the mismatching element and then the number of strings previously read.
$endgroup$
add a comment |
$begingroup$
MATLAB, 68 22 bytes
[r,c]=find(v~=v(1));if size(r,1)>1 disp([1,1]);else disp([r,c]);end;
If I could exclude any one case, such as [1,1]
in this solution, I could have saved several bytes.
Updated solution:
@(v)find(v-mode(v(:)))
Thanks to @sundar for helping me with the special case problem and saving 42 bytes! Also, thanks to @Luis_Mendo for the suggestions and saving me another 2 bytes!
$endgroup$
$begingroup$
I think you can get rid of the check for[1,1]
case by usingmode(v(:))
instead ofv(1)
.
$endgroup$
– sundar
yesterday
$begingroup$
You need to wrap your code so that it is a full program or a function; you cannot assume that the input is in a variablev
. Also, you can probably replace~=
by-
, and remove the final;
$endgroup$
– Luis Mendo
yesterday
add a comment |
$begingroup$
Röda, 81 bytes
f a{i=indexOf;l=i("
",a)+1;chars a|sort|count|[[_2,_1]]|min|i _[1],a|[_%l,_1//l]}
Try it online!
Takes input as a string containing newline-terminated lines. Returns a stream containing 0-indexed horizontal and vertical indexes.
$endgroup$
add a comment |
$begingroup$
Perl 5 -n0
, 77 bytes
$t=(@b=sort/./g)[0]eq$b[1]?pop@b:$b[0];++$y&/Q$t/g&&say"$y,",pos for /.+$/mg
Try it online!
Output is row,column
starting from the top left, 1-indexed
$endgroup$
add a comment |
$begingroup$
C (clang), 83 bytes
a,i;h(char*s,z,x){for(i=0;++i<z;)*s-*++s?a=i-(*s==s[1]):0;printf("%d,%d",a%x,a/x);}
Try it online!
$endgroup$
add a comment |
$begingroup$
Haskell, 64 bytes
f s=[(r,c)|c<-[0..],r<-[0..length s-1],any(notElem$s!!r!!c)s]!!0
Try it online! f
takes a list of lines and returns zero-indexed (row,column)
.
Works by iterating through the columns and rows and looking for a character which does not appear on all rows.
$endgroup$
add a comment |
$begingroup$
C# .NET, 133 112 111 bytes
m=>{var c=m[0][0];c=m[1][0]!=c?m[1][1]:c;for(int i=0,j;;i++)for(j=m[i].Count;j-->0;)if(m[i][j]!=c)return(i,j);}
-1 byte thanks to @EmbodimentOfIgnorance.
Port of my Java answer, but with a List<List<char>>
input and int
-tuple output, instead of char
input and string
output.
Try it online.
$endgroup$
1
$begingroup$
Can't you save two bytes by turning youchar
into aList<List<char>>
? SinceList<T>.Count
is shorter thanArray.Length
$endgroup$
– Embodiment of Ignorance
8 hours ago
$begingroup$
@EmbodimentofIgnorance Thanks! :)
$endgroup$
– Kevin Cruijssen
6 hours ago
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "200"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f179351%2ffind-the-needle-in-the-haystack%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
29 Answers
29
active
oldest
votes
29 Answers
29
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
R, 49 47 44 bytes
function(m,`?`=which)m==names(?table(m)<2)?T
Try it online!
Takes input as a matrix, returns 1-indexed coordinates
$endgroup$
3
$begingroup$
Thatwhich
assignment is disgracefully smooth.
$endgroup$
– CriminallyVulgar
yesterday
3
$begingroup$
I was so excited to try this challenge in R, then I saw this and decided to cry in awe instead
$endgroup$
– Sumner18
yesterday
add a comment |
$begingroup$
R, 49 47 44 bytes
function(m,`?`=which)m==names(?table(m)<2)?T
Try it online!
Takes input as a matrix, returns 1-indexed coordinates
$endgroup$
3
$begingroup$
Thatwhich
assignment is disgracefully smooth.
$endgroup$
– CriminallyVulgar
yesterday
3
$begingroup$
I was so excited to try this challenge in R, then I saw this and decided to cry in awe instead
$endgroup$
– Sumner18
yesterday
add a comment |
$begingroup$
R, 49 47 44 bytes
function(m,`?`=which)m==names(?table(m)<2)?T
Try it online!
Takes input as a matrix, returns 1-indexed coordinates
$endgroup$
R, 49 47 44 bytes
function(m,`?`=which)m==names(?table(m)<2)?T
Try it online!
Takes input as a matrix, returns 1-indexed coordinates
edited yesterday
answered yesterday
Kirill L.Kirill L.
4,1851322
4,1851322
3
$begingroup$
Thatwhich
assignment is disgracefully smooth.
$endgroup$
– CriminallyVulgar
yesterday
3
$begingroup$
I was so excited to try this challenge in R, then I saw this and decided to cry in awe instead
$endgroup$
– Sumner18
yesterday
add a comment |
3
$begingroup$
Thatwhich
assignment is disgracefully smooth.
$endgroup$
– CriminallyVulgar
yesterday
3
$begingroup$
I was so excited to try this challenge in R, then I saw this and decided to cry in awe instead
$endgroup$
– Sumner18
yesterday
3
3
$begingroup$
That
which
assignment is disgracefully smooth.$endgroup$
– CriminallyVulgar
yesterday
$begingroup$
That
which
assignment is disgracefully smooth.$endgroup$
– CriminallyVulgar
yesterday
3
3
$begingroup$
I was so excited to try this challenge in R, then I saw this and decided to cry in awe instead
$endgroup$
– Sumner18
yesterday
$begingroup$
I was so excited to try this challenge in R, then I saw this and decided to cry in awe instead
$endgroup$
– Sumner18
yesterday
add a comment |
$begingroup$
Perl 6, 41 38 37 bytes
3 bytes saved thanks to @nwellnhof.
1 byte saved thanks to Jo King.
{map {[+] ^∞Z*!<<.&[Z~~]},$_,.&[Z]}
Try it online!
Explanation
It takes the input as a list of lists of characters and returns list of length 2 containing zero-based X and Y coordinates of the needle.
It works by applying the block {[+] ^∞ Z* !<<.&[Z~~]}
on the input and on its transpose. .&[Z~~]
goes through all columns of the argument and returns True
if all the elements are the same, False
otherwise. We then negate all the values (so we have a list with one bool per column, where the bool answers the question "Is the needle in that column?"), multiply them element-wise with a sequence 0,1,2,... (True = 1
and False = 0
) and sum the list, so the result of the whole block is the 0-based number of the column where the needle was found.
Nwellnhof's better approach, Perl 6, 34 bytes
{map *.first(:k,*.Set>1),.&[Z],$_}
Try it online!
Explanation
Generally the same approach, just more effective. It still uses a block on the array and its transpose, but now the block converts all rows intoSets
and checks for the number of elements. The first
function then gives index (due to the :k
) of the first row that contained more than 1 element. Because of that, the order of $_
and .&[Z]
needed to be swapped.
$endgroup$
$begingroup$
Nice approach! 34 bytes withfirst(:k)
,Set
and.&[Z]
.
$endgroup$
– nwellnhof
yesterday
$begingroup$
@nwellnhof, very well done. You basically found what I wanted to find but failed to do that :—). (Also I had no idea that you could write.&[Z]
.)
$endgroup$
– Ramillies
yesterday
$begingroup$
In general,.&[op]
doesn't seem to be equivalent to[op] $_
but it works withZ
for some reason.
$endgroup$
– nwellnhof
yesterday
$begingroup$
@JoKing, thanks!
$endgroup$
– Ramillies
14 hours ago
add a comment |
$begingroup$
Perl 6, 41 38 37 bytes
3 bytes saved thanks to @nwellnhof.
1 byte saved thanks to Jo King.
{map {[+] ^∞Z*!<<.&[Z~~]},$_,.&[Z]}
Try it online!
Explanation
It takes the input as a list of lists of characters and returns list of length 2 containing zero-based X and Y coordinates of the needle.
It works by applying the block {[+] ^∞ Z* !<<.&[Z~~]}
on the input and on its transpose. .&[Z~~]
goes through all columns of the argument and returns True
if all the elements are the same, False
otherwise. We then negate all the values (so we have a list with one bool per column, where the bool answers the question "Is the needle in that column?"), multiply them element-wise with a sequence 0,1,2,... (True = 1
and False = 0
) and sum the list, so the result of the whole block is the 0-based number of the column where the needle was found.
Nwellnhof's better approach, Perl 6, 34 bytes
{map *.first(:k,*.Set>1),.&[Z],$_}
Try it online!
Explanation
Generally the same approach, just more effective. It still uses a block on the array and its transpose, but now the block converts all rows intoSets
and checks for the number of elements. The first
function then gives index (due to the :k
) of the first row that contained more than 1 element. Because of that, the order of $_
and .&[Z]
needed to be swapped.
$endgroup$
$begingroup$
Nice approach! 34 bytes withfirst(:k)
,Set
and.&[Z]
.
$endgroup$
– nwellnhof
yesterday
$begingroup$
@nwellnhof, very well done. You basically found what I wanted to find but failed to do that :—). (Also I had no idea that you could write.&[Z]
.)
$endgroup$
– Ramillies
yesterday
$begingroup$
In general,.&[op]
doesn't seem to be equivalent to[op] $_
but it works withZ
for some reason.
$endgroup$
– nwellnhof
yesterday
$begingroup$
@JoKing, thanks!
$endgroup$
– Ramillies
14 hours ago
add a comment |
$begingroup$
Perl 6, 41 38 37 bytes
3 bytes saved thanks to @nwellnhof.
1 byte saved thanks to Jo King.
{map {[+] ^∞Z*!<<.&[Z~~]},$_,.&[Z]}
Try it online!
Explanation
It takes the input as a list of lists of characters and returns list of length 2 containing zero-based X and Y coordinates of the needle.
It works by applying the block {[+] ^∞ Z* !<<.&[Z~~]}
on the input and on its transpose. .&[Z~~]
goes through all columns of the argument and returns True
if all the elements are the same, False
otherwise. We then negate all the values (so we have a list with one bool per column, where the bool answers the question "Is the needle in that column?"), multiply them element-wise with a sequence 0,1,2,... (True = 1
and False = 0
) and sum the list, so the result of the whole block is the 0-based number of the column where the needle was found.
Nwellnhof's better approach, Perl 6, 34 bytes
{map *.first(:k,*.Set>1),.&[Z],$_}
Try it online!
Explanation
Generally the same approach, just more effective. It still uses a block on the array and its transpose, but now the block converts all rows intoSets
and checks for the number of elements. The first
function then gives index (due to the :k
) of the first row that contained more than 1 element. Because of that, the order of $_
and .&[Z]
needed to be swapped.
$endgroup$
Perl 6, 41 38 37 bytes
3 bytes saved thanks to @nwellnhof.
1 byte saved thanks to Jo King.
{map {[+] ^∞Z*!<<.&[Z~~]},$_,.&[Z]}
Try it online!
Explanation
It takes the input as a list of lists of characters and returns list of length 2 containing zero-based X and Y coordinates of the needle.
It works by applying the block {[+] ^∞ Z* !<<.&[Z~~]}
on the input and on its transpose. .&[Z~~]
goes through all columns of the argument and returns True
if all the elements are the same, False
otherwise. We then negate all the values (so we have a list with one bool per column, where the bool answers the question "Is the needle in that column?"), multiply them element-wise with a sequence 0,1,2,... (True = 1
and False = 0
) and sum the list, so the result of the whole block is the 0-based number of the column where the needle was found.
Nwellnhof's better approach, Perl 6, 34 bytes
{map *.first(:k,*.Set>1),.&[Z],$_}
Try it online!
Explanation
Generally the same approach, just more effective. It still uses a block on the array and its transpose, but now the block converts all rows intoSets
and checks for the number of elements. The first
function then gives index (due to the :k
) of the first row that contained more than 1 element. Because of that, the order of $_
and .&[Z]
needed to be swapped.
edited 14 hours ago
answered yesterday
RamilliesRamillies
1,571515
1,571515
$begingroup$
Nice approach! 34 bytes withfirst(:k)
,Set
and.&[Z]
.
$endgroup$
– nwellnhof
yesterday
$begingroup$
@nwellnhof, very well done. You basically found what I wanted to find but failed to do that :—). (Also I had no idea that you could write.&[Z]
.)
$endgroup$
– Ramillies
yesterday
$begingroup$
In general,.&[op]
doesn't seem to be equivalent to[op] $_
but it works withZ
for some reason.
$endgroup$
– nwellnhof
yesterday
$begingroup$
@JoKing, thanks!
$endgroup$
– Ramillies
14 hours ago
add a comment |
$begingroup$
Nice approach! 34 bytes withfirst(:k)
,Set
and.&[Z]
.
$endgroup$
– nwellnhof
yesterday
$begingroup$
@nwellnhof, very well done. You basically found what I wanted to find but failed to do that :—). (Also I had no idea that you could write.&[Z]
.)
$endgroup$
– Ramillies
yesterday
$begingroup$
In general,.&[op]
doesn't seem to be equivalent to[op] $_
but it works withZ
for some reason.
$endgroup$
– nwellnhof
yesterday
$begingroup$
@JoKing, thanks!
$endgroup$
– Ramillies
14 hours ago
$begingroup$
Nice approach! 34 bytes with
first(:k)
, Set
and .&[Z]
.$endgroup$
– nwellnhof
yesterday
$begingroup$
Nice approach! 34 bytes with
first(:k)
, Set
and .&[Z]
.$endgroup$
– nwellnhof
yesterday
$begingroup$
@nwellnhof, very well done. You basically found what I wanted to find but failed to do that :—). (Also I had no idea that you could write
.&[Z]
.)$endgroup$
– Ramillies
yesterday
$begingroup$
@nwellnhof, very well done. You basically found what I wanted to find but failed to do that :—). (Also I had no idea that you could write
.&[Z]
.)$endgroup$
– Ramillies
yesterday
$begingroup$
In general,
.&[op]
doesn't seem to be equivalent to [op] $_
but it works with Z
for some reason.$endgroup$
– nwellnhof
yesterday
$begingroup$
In general,
.&[op]
doesn't seem to be equivalent to [op] $_
but it works with Z
for some reason.$endgroup$
– nwellnhof
yesterday
$begingroup$
@JoKing, thanks!
$endgroup$
– Ramillies
14 hours ago
$begingroup$
@JoKing, thanks!
$endgroup$
– Ramillies
14 hours ago
add a comment |
$begingroup$
05AB1E, 9 6 bytes
Saved 3 bytes switching input format.
Input is taken as a string and a row-length.
Output is a zero-based list of the form [y, x]
D.mks‰
Try it online!
or as a Test Suite
Explanation
D # duplicate the input string
.m # get the least frequent character
k # get its index in the string
s # swap the row length to the top of the stack
‰ # divmod the index of the least frequent char with the row length
$endgroup$
$begingroup$
Dang, you beat me to it. Was working on an answer. Had just finished a 13-byter. But yours is way better, so +1 instead. :) Completely forgot about.m
..
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Yeah. I don't think I've ever used.m
before, but I was reasonably sure I'd seen it at some point :)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
05AB1E, 9 6 bytes
Saved 3 bytes switching input format.
Input is taken as a string and a row-length.
Output is a zero-based list of the form [y, x]
D.mks‰
Try it online!
or as a Test Suite
Explanation
D # duplicate the input string
.m # get the least frequent character
k # get its index in the string
s # swap the row length to the top of the stack
‰ # divmod the index of the least frequent char with the row length
$endgroup$
$begingroup$
Dang, you beat me to it. Was working on an answer. Had just finished a 13-byter. But yours is way better, so +1 instead. :) Completely forgot about.m
..
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Yeah. I don't think I've ever used.m
before, but I was reasonably sure I'd seen it at some point :)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
05AB1E, 9 6 bytes
Saved 3 bytes switching input format.
Input is taken as a string and a row-length.
Output is a zero-based list of the form [y, x]
D.mks‰
Try it online!
or as a Test Suite
Explanation
D # duplicate the input string
.m # get the least frequent character
k # get its index in the string
s # swap the row length to the top of the stack
‰ # divmod the index of the least frequent char with the row length
$endgroup$
05AB1E, 9 6 bytes
Saved 3 bytes switching input format.
Input is taken as a string and a row-length.
Output is a zero-based list of the form [y, x]
D.mks‰
Try it online!
or as a Test Suite
Explanation
D # duplicate the input string
.m # get the least frequent character
k # get its index in the string
s # swap the row length to the top of the stack
‰ # divmod the index of the least frequent char with the row length
edited yesterday
answered yesterday


EmignaEmigna
46.1k432141
46.1k432141
$begingroup$
Dang, you beat me to it. Was working on an answer. Had just finished a 13-byter. But yours is way better, so +1 instead. :) Completely forgot about.m
..
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Yeah. I don't think I've ever used.m
before, but I was reasonably sure I'd seen it at some point :)
$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
Dang, you beat me to it. Was working on an answer. Had just finished a 13-byter. But yours is way better, so +1 instead. :) Completely forgot about.m
..
$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Yeah. I don't think I've ever used.m
before, but I was reasonably sure I'd seen it at some point :)
$endgroup$
– Emigna
yesterday
$begingroup$
Dang, you beat me to it. Was working on an answer. Had just finished a 13-byter. But yours is way better, so +1 instead. :) Completely forgot about
.m
..$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
Dang, you beat me to it. Was working on an answer. Had just finished a 13-byter. But yours is way better, so +1 instead. :) Completely forgot about
.m
..$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
@KevinCruijssen: Yeah. I don't think I've ever used
.m
before, but I was reasonably sure I'd seen it at some point :)$endgroup$
– Emigna
yesterday
$begingroup$
@KevinCruijssen: Yeah. I don't think I've ever used
.m
before, but I was reasonably sure I'd seen it at some point :)$endgroup$
– Emigna
yesterday
add a comment |
$begingroup$
Python 2, 57 bytes
lambda m:[map(len,map(set,a)).index(2)for a in zip(*m),m]
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 57 bytes
lambda m:[map(len,map(set,a)).index(2)for a in zip(*m),m]
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 57 bytes
lambda m:[map(len,map(set,a)).index(2)for a in zip(*m),m]
Try it online!
$endgroup$
Python 2, 57 bytes
lambda m:[map(len,map(set,a)).index(2)for a in zip(*m),m]
Try it online!
answered yesterday


Jonathan AllanJonathan Allan
51.9k535170
51.9k535170
add a comment |
add a comment |
$begingroup$
Brachylog, 20 bytes
c≡ᵍ∋Ȯ&;I∋₎;J∋₎gȮ∧I;J
Try it online!
Outputs [I,J]
, where I
is the row index and J
the column index, both 0-indexed.
Stupidely long, but getting indexes in Brachylog is usually very verbose.
Explanation
c Concatenate the Input into a single string
≡ᵍ Group identical characters together
∋Ȯ Ȯ is a list of One element, which is the needle character
&;I∋₎ Take the Ith row of the Input
;J∋₎ Take the Jth character of the Ith row
gȮ That character, when wrapped in a list, is Ȯ
∧I;J The output is the list [I,J]
$endgroup$
add a comment |
$begingroup$
Brachylog, 20 bytes
c≡ᵍ∋Ȯ&;I∋₎;J∋₎gȮ∧I;J
Try it online!
Outputs [I,J]
, where I
is the row index and J
the column index, both 0-indexed.
Stupidely long, but getting indexes in Brachylog is usually very verbose.
Explanation
c Concatenate the Input into a single string
≡ᵍ Group identical characters together
∋Ȯ Ȯ is a list of One element, which is the needle character
&;I∋₎ Take the Ith row of the Input
;J∋₎ Take the Jth character of the Ith row
gȮ That character, when wrapped in a list, is Ȯ
∧I;J The output is the list [I,J]
$endgroup$
add a comment |
$begingroup$
Brachylog, 20 bytes
c≡ᵍ∋Ȯ&;I∋₎;J∋₎gȮ∧I;J
Try it online!
Outputs [I,J]
, where I
is the row index and J
the column index, both 0-indexed.
Stupidely long, but getting indexes in Brachylog is usually very verbose.
Explanation
c Concatenate the Input into a single string
≡ᵍ Group identical characters together
∋Ȯ Ȯ is a list of One element, which is the needle character
&;I∋₎ Take the Ith row of the Input
;J∋₎ Take the Jth character of the Ith row
gȮ That character, when wrapped in a list, is Ȯ
∧I;J The output is the list [I,J]
$endgroup$
Brachylog, 20 bytes
c≡ᵍ∋Ȯ&;I∋₎;J∋₎gȮ∧I;J
Try it online!
Outputs [I,J]
, where I
is the row index and J
the column index, both 0-indexed.
Stupidely long, but getting indexes in Brachylog is usually very verbose.
Explanation
c Concatenate the Input into a single string
≡ᵍ Group identical characters together
∋Ȯ Ȯ is a list of One element, which is the needle character
&;I∋₎ Take the Ith row of the Input
;J∋₎ Take the Jth character of the Ith row
gȮ That character, when wrapped in a list, is Ȯ
∧I;J The output is the list [I,J]
answered yesterday


FatalizeFatalize
27.1k448135
27.1k448135
add a comment |
add a comment |
$begingroup$
Jelly, 5 bytes
Outputs [height, width] (1-indexed).
ŒĠLÐṂ
Try it online!
ŒĠLÐṂ – Monadic link / Full program. Takes a list of strings M as input.
ŒĠ – Group the multidimensional indices by their values (treating M as a matrix).
LÐṂ – And retrieve the shortest group of indices (those of the unique character).
Jelly, 5 bytes
ŒĠḊÐḟ
Try it online!
$endgroup$
add a comment |
$begingroup$
Jelly, 5 bytes
Outputs [height, width] (1-indexed).
ŒĠLÐṂ
Try it online!
ŒĠLÐṂ – Monadic link / Full program. Takes a list of strings M as input.
ŒĠ – Group the multidimensional indices by their values (treating M as a matrix).
LÐṂ – And retrieve the shortest group of indices (those of the unique character).
Jelly, 5 bytes
ŒĠḊÐḟ
Try it online!
$endgroup$
add a comment |
$begingroup$
Jelly, 5 bytes
Outputs [height, width] (1-indexed).
ŒĠLÐṂ
Try it online!
ŒĠLÐṂ – Monadic link / Full program. Takes a list of strings M as input.
ŒĠ – Group the multidimensional indices by their values (treating M as a matrix).
LÐṂ – And retrieve the shortest group of indices (those of the unique character).
Jelly, 5 bytes
ŒĠḊÐḟ
Try it online!
$endgroup$
Jelly, 5 bytes
Outputs [height, width] (1-indexed).
ŒĠLÐṂ
Try it online!
ŒĠLÐṂ – Monadic link / Full program. Takes a list of strings M as input.
ŒĠ – Group the multidimensional indices by their values (treating M as a matrix).
LÐṂ – And retrieve the shortest group of indices (those of the unique character).
Jelly, 5 bytes
ŒĠḊÐḟ
Try it online!
edited yesterday
answered yesterday


Mr. XcoderMr. Xcoder
31.9k759199
31.9k759199
add a comment |
add a comment |
$begingroup$
Jelly, 4 bytes
Maybe this could've just been a comment for Mr. Xcoder it is pretty similar...
ŒĠEƇ
A monadic link accepting the matrix of characters which yields a list of one item, the 1-indexed (row, column) co-ordinate from top-left.
(...As a full program given an argument formatted such that parsing results in a list of lists of characters -- that is a list of strings in Python format -- the single coordinate is printed.)
Try it online!
How?
ŒĠEƇ - Link: matrix, M
ŒĠ - multi-dimensional indices grouped by Value
- ...due to the 2*2 minimum size and one needle this will be a list of two lists one
- of which will have length one (the needle coordinates as a pair) and the other
- containing all other coordinates as pairs
Ƈ - filter keeping those for which this is truthy:
E - all equal?
- ... 1 for the list of length 1, 0 for the list of at least 3 non-equal coordinates
$endgroup$
1
$begingroup$
Well... this seems borderline, since theEƇ
is clever.
$endgroup$
– Erik the Outgolfer
yesterday
add a comment |
$begingroup$
Jelly, 4 bytes
Maybe this could've just been a comment for Mr. Xcoder it is pretty similar...
ŒĠEƇ
A monadic link accepting the matrix of characters which yields a list of one item, the 1-indexed (row, column) co-ordinate from top-left.
(...As a full program given an argument formatted such that parsing results in a list of lists of characters -- that is a list of strings in Python format -- the single coordinate is printed.)
Try it online!
How?
ŒĠEƇ - Link: matrix, M
ŒĠ - multi-dimensional indices grouped by Value
- ...due to the 2*2 minimum size and one needle this will be a list of two lists one
- of which will have length one (the needle coordinates as a pair) and the other
- containing all other coordinates as pairs
Ƈ - filter keeping those for which this is truthy:
E - all equal?
- ... 1 for the list of length 1, 0 for the list of at least 3 non-equal coordinates
$endgroup$
1
$begingroup$
Well... this seems borderline, since theEƇ
is clever.
$endgroup$
– Erik the Outgolfer
yesterday
add a comment |
$begingroup$
Jelly, 4 bytes
Maybe this could've just been a comment for Mr. Xcoder it is pretty similar...
ŒĠEƇ
A monadic link accepting the matrix of characters which yields a list of one item, the 1-indexed (row, column) co-ordinate from top-left.
(...As a full program given an argument formatted such that parsing results in a list of lists of characters -- that is a list of strings in Python format -- the single coordinate is printed.)
Try it online!
How?
ŒĠEƇ - Link: matrix, M
ŒĠ - multi-dimensional indices grouped by Value
- ...due to the 2*2 minimum size and one needle this will be a list of two lists one
- of which will have length one (the needle coordinates as a pair) and the other
- containing all other coordinates as pairs
Ƈ - filter keeping those for which this is truthy:
E - all equal?
- ... 1 for the list of length 1, 0 for the list of at least 3 non-equal coordinates
$endgroup$
Jelly, 4 bytes
Maybe this could've just been a comment for Mr. Xcoder it is pretty similar...
ŒĠEƇ
A monadic link accepting the matrix of characters which yields a list of one item, the 1-indexed (row, column) co-ordinate from top-left.
(...As a full program given an argument formatted such that parsing results in a list of lists of characters -- that is a list of strings in Python format -- the single coordinate is printed.)
Try it online!
How?
ŒĠEƇ - Link: matrix, M
ŒĠ - multi-dimensional indices grouped by Value
- ...due to the 2*2 minimum size and one needle this will be a list of two lists one
- of which will have length one (the needle coordinates as a pair) and the other
- containing all other coordinates as pairs
Ƈ - filter keeping those for which this is truthy:
E - all equal?
- ... 1 for the list of length 1, 0 for the list of at least 3 non-equal coordinates
edited yesterday
answered yesterday


Jonathan AllanJonathan Allan
51.9k535170
51.9k535170
1
$begingroup$
Well... this seems borderline, since theEƇ
is clever.
$endgroup$
– Erik the Outgolfer
yesterday
add a comment |
1
$begingroup$
Well... this seems borderline, since theEƇ
is clever.
$endgroup$
– Erik the Outgolfer
yesterday
1
1
$begingroup$
Well... this seems borderline, since the
EƇ
is clever.$endgroup$
– Erik the Outgolfer
yesterday
$begingroup$
Well... this seems borderline, since the
EƇ
is clever.$endgroup$
– Erik the Outgolfer
yesterday
add a comment |
$begingroup$
Python 3, 75 bytes
import numpy as n
def f(x):x=x.view('uint32');return n.where(x-n.median(x))
Try it online!
This assumes that the input is a NumPy array. The output is zero-indexed, and first vertical, then horizontal.
It converts the input from char
to uint32
then calculates the median of the array, which will be the haystack character. We subtract that from the array, which makes the needle the only non-zero element. Finally, return the index of that element with numpy.where()
.
$endgroup$
1
$begingroup$
Since you know the input will be ASCII (i.e. fits in a byte) why not useuint8
for one byte less?
$endgroup$
– Draconis
yesterday
1
$begingroup$
Language has to be "Python 3 + numpy" here since numpy isn't included with the normal Python distribution
$endgroup$
– ASCII-only
18 hours ago
add a comment |
$begingroup$
Python 3, 75 bytes
import numpy as n
def f(x):x=x.view('uint32');return n.where(x-n.median(x))
Try it online!
This assumes that the input is a NumPy array. The output is zero-indexed, and first vertical, then horizontal.
It converts the input from char
to uint32
then calculates the median of the array, which will be the haystack character. We subtract that from the array, which makes the needle the only non-zero element. Finally, return the index of that element with numpy.where()
.
$endgroup$
1
$begingroup$
Since you know the input will be ASCII (i.e. fits in a byte) why not useuint8
for one byte less?
$endgroup$
– Draconis
yesterday
1
$begingroup$
Language has to be "Python 3 + numpy" here since numpy isn't included with the normal Python distribution
$endgroup$
– ASCII-only
18 hours ago
add a comment |
$begingroup$
Python 3, 75 bytes
import numpy as n
def f(x):x=x.view('uint32');return n.where(x-n.median(x))
Try it online!
This assumes that the input is a NumPy array. The output is zero-indexed, and first vertical, then horizontal.
It converts the input from char
to uint32
then calculates the median of the array, which will be the haystack character. We subtract that from the array, which makes the needle the only non-zero element. Finally, return the index of that element with numpy.where()
.
$endgroup$
Python 3, 75 bytes
import numpy as n
def f(x):x=x.view('uint32');return n.where(x-n.median(x))
Try it online!
This assumes that the input is a NumPy array. The output is zero-indexed, and first vertical, then horizontal.
It converts the input from char
to uint32
then calculates the median of the array, which will be the haystack character. We subtract that from the array, which makes the needle the only non-zero element. Finally, return the index of that element with numpy.where()
.
answered yesterday
hbadertshbaderts
20116
20116
1
$begingroup$
Since you know the input will be ASCII (i.e. fits in a byte) why not useuint8
for one byte less?
$endgroup$
– Draconis
yesterday
1
$begingroup$
Language has to be "Python 3 + numpy" here since numpy isn't included with the normal Python distribution
$endgroup$
– ASCII-only
18 hours ago
add a comment |
1
$begingroup$
Since you know the input will be ASCII (i.e. fits in a byte) why not useuint8
for one byte less?
$endgroup$
– Draconis
yesterday
1
$begingroup$
Language has to be "Python 3 + numpy" here since numpy isn't included with the normal Python distribution
$endgroup$
– ASCII-only
18 hours ago
1
1
$begingroup$
Since you know the input will be ASCII (i.e. fits in a byte) why not use
uint8
for one byte less?$endgroup$
– Draconis
yesterday
$begingroup$
Since you know the input will be ASCII (i.e. fits in a byte) why not use
uint8
for one byte less?$endgroup$
– Draconis
yesterday
1
1
$begingroup$
Language has to be "Python 3 + numpy" here since numpy isn't included with the normal Python distribution
$endgroup$
– ASCII-only
18 hours ago
$begingroup$
Language has to be "Python 3 + numpy" here since numpy isn't included with the normal Python distribution
$endgroup$
– ASCII-only
18 hours ago
add a comment |
$begingroup$
MATL, 12 8 bytes
tX:XM-&f
Try it online!
Using the mode
function as the majority-detector. Returns 1-based indices.
t % duplicate the input
X: % turn the copy into a linear array
XM % find the arithmetic mode of that (the 'haystack' character)
- % Subtract that from the original input
&f % find the position of the non-zero value in that result
-4 characters thanks to @LuisMendo
$endgroup$
$begingroup$
I think you can shorten totX:XM-&f
(two-output version off
)
$endgroup$
– Luis Mendo
yesterday
1
$begingroup$
@LuisMendo Thanks. I don't think I knew about the 2 output version offind
, even in MATLAB. (Hi, btw!)
$endgroup$
– sundar
yesterday
add a comment |
$begingroup$
MATL, 12 8 bytes
tX:XM-&f
Try it online!
Using the mode
function as the majority-detector. Returns 1-based indices.
t % duplicate the input
X: % turn the copy into a linear array
XM % find the arithmetic mode of that (the 'haystack' character)
- % Subtract that from the original input
&f % find the position of the non-zero value in that result
-4 characters thanks to @LuisMendo
$endgroup$
$begingroup$
I think you can shorten totX:XM-&f
(two-output version off
)
$endgroup$
– Luis Mendo
yesterday
1
$begingroup$
@LuisMendo Thanks. I don't think I knew about the 2 output version offind
, even in MATLAB. (Hi, btw!)
$endgroup$
– sundar
yesterday
add a comment |
$begingroup$
MATL, 12 8 bytes
tX:XM-&f
Try it online!
Using the mode
function as the majority-detector. Returns 1-based indices.
t % duplicate the input
X: % turn the copy into a linear array
XM % find the arithmetic mode of that (the 'haystack' character)
- % Subtract that from the original input
&f % find the position of the non-zero value in that result
-4 characters thanks to @LuisMendo
$endgroup$
MATL, 12 8 bytes
tX:XM-&f
Try it online!
Using the mode
function as the majority-detector. Returns 1-based indices.
t % duplicate the input
X: % turn the copy into a linear array
XM % find the arithmetic mode of that (the 'haystack' character)
- % Subtract that from the original input
&f % find the position of the non-zero value in that result
-4 characters thanks to @LuisMendo
edited yesterday
answered yesterday
sundarsundar
4,771829
4,771829
$begingroup$
I think you can shorten totX:XM-&f
(two-output version off
)
$endgroup$
– Luis Mendo
yesterday
1
$begingroup$
@LuisMendo Thanks. I don't think I knew about the 2 output version offind
, even in MATLAB. (Hi, btw!)
$endgroup$
– sundar
yesterday
add a comment |
$begingroup$
I think you can shorten totX:XM-&f
(two-output version off
)
$endgroup$
– Luis Mendo
yesterday
1
$begingroup$
@LuisMendo Thanks. I don't think I knew about the 2 output version offind
, even in MATLAB. (Hi, btw!)
$endgroup$
– sundar
yesterday
$begingroup$
I think you can shorten to
tX:XM-&f
(two-output version of f
)$endgroup$
– Luis Mendo
yesterday
$begingroup$
I think you can shorten to
tX:XM-&f
(two-output version of f
)$endgroup$
– Luis Mendo
yesterday
1
1
$begingroup$
@LuisMendo Thanks. I don't think I knew about the 2 output version of
find
, even in MATLAB. (Hi, btw!)$endgroup$
– sundar
yesterday
$begingroup$
@LuisMendo Thanks. I don't think I knew about the 2 output version of
find
, even in MATLAB. (Hi, btw!)$endgroup$
– sundar
yesterday
add a comment |
$begingroup$
PHP, 99 85 bytes
Using string without newlines and the width (or height) ('########N###########', 5
) as input.
- -5 bytes by removing chr() call, props to @Titus
- -9 bytes by taking input as two function args, also props to @Titus
function($a,$l){return[($p=strpos($a,array_flip(count_chars($a,1))[1]))%$l,$p/$l|0];}
Try it online!
Ungolfed:
function need_hay( $a, $l ) {
// identify the "needle" by counting the chars and
// looking for the char with exactly 1 occurrence
// note: this is 1 byte shorter than using array_search()
$n = array_flip( count_chars( $a, 1 ) )[1];
// find the location in the input string
$p = strpos( $a, $n );
// row is location divided by row length, rounded down
$r = floor( $p / $l );
// column is remainder of location divided by row length
$c = $p % $l;
return array( $c, $r );
}
Output:
#####
###N#
#####
#####
[3,1]
^^^
^^^
^N^
^^^
^^^
^^^
[1,2]
jjjjjj
j@jjjj
jjjjjj
[1,1]
$endgroup$
1
$begingroup$
1) no need forchr
: If the second parameter for strpos is an integer, it will be interpreted as an ASCII code. -> -5 bytes. 2) Two function parameters$s,$w
can save another 9 bytes.
$endgroup$
– Titus
yesterday
$begingroup$
@Titus, removing the chr() that's brilliant. Thx! The func params did occur to me too, I just didn't want to run afowl of input req's. I'll clarify w/OP.
$endgroup$
– gwaugh
yesterday
add a comment |
$begingroup$
PHP, 99 85 bytes
Using string without newlines and the width (or height) ('########N###########', 5
) as input.
- -5 bytes by removing chr() call, props to @Titus
- -9 bytes by taking input as two function args, also props to @Titus
function($a,$l){return[($p=strpos($a,array_flip(count_chars($a,1))[1]))%$l,$p/$l|0];}
Try it online!
Ungolfed:
function need_hay( $a, $l ) {
// identify the "needle" by counting the chars and
// looking for the char with exactly 1 occurrence
// note: this is 1 byte shorter than using array_search()
$n = array_flip( count_chars( $a, 1 ) )[1];
// find the location in the input string
$p = strpos( $a, $n );
// row is location divided by row length, rounded down
$r = floor( $p / $l );
// column is remainder of location divided by row length
$c = $p % $l;
return array( $c, $r );
}
Output:
#####
###N#
#####
#####
[3,1]
^^^
^^^
^N^
^^^
^^^
^^^
[1,2]
jjjjjj
j@jjjj
jjjjjj
[1,1]
$endgroup$
1
$begingroup$
1) no need forchr
: If the second parameter for strpos is an integer, it will be interpreted as an ASCII code. -> -5 bytes. 2) Two function parameters$s,$w
can save another 9 bytes.
$endgroup$
– Titus
yesterday
$begingroup$
@Titus, removing the chr() that's brilliant. Thx! The func params did occur to me too, I just didn't want to run afowl of input req's. I'll clarify w/OP.
$endgroup$
– gwaugh
yesterday
add a comment |
$begingroup$
PHP, 99 85 bytes
Using string without newlines and the width (or height) ('########N###########', 5
) as input.
- -5 bytes by removing chr() call, props to @Titus
- -9 bytes by taking input as two function args, also props to @Titus
function($a,$l){return[($p=strpos($a,array_flip(count_chars($a,1))[1]))%$l,$p/$l|0];}
Try it online!
Ungolfed:
function need_hay( $a, $l ) {
// identify the "needle" by counting the chars and
// looking for the char with exactly 1 occurrence
// note: this is 1 byte shorter than using array_search()
$n = array_flip( count_chars( $a, 1 ) )[1];
// find the location in the input string
$p = strpos( $a, $n );
// row is location divided by row length, rounded down
$r = floor( $p / $l );
// column is remainder of location divided by row length
$c = $p % $l;
return array( $c, $r );
}
Output:
#####
###N#
#####
#####
[3,1]
^^^
^^^
^N^
^^^
^^^
^^^
[1,2]
jjjjjj
j@jjjj
jjjjjj
[1,1]
$endgroup$
PHP, 99 85 bytes
Using string without newlines and the width (or height) ('########N###########', 5
) as input.
- -5 bytes by removing chr() call, props to @Titus
- -9 bytes by taking input as two function args, also props to @Titus
function($a,$l){return[($p=strpos($a,array_flip(count_chars($a,1))[1]))%$l,$p/$l|0];}
Try it online!
Ungolfed:
function need_hay( $a, $l ) {
// identify the "needle" by counting the chars and
// looking for the char with exactly 1 occurrence
// note: this is 1 byte shorter than using array_search()
$n = array_flip( count_chars( $a, 1 ) )[1];
// find the location in the input string
$p = strpos( $a, $n );
// row is location divided by row length, rounded down
$r = floor( $p / $l );
// column is remainder of location divided by row length
$c = $p % $l;
return array( $c, $r );
}
Output:
#####
###N#
#####
#####
[3,1]
^^^
^^^
^N^
^^^
^^^
^^^
[1,2]
jjjjjj
j@jjjj
jjjjjj
[1,1]
edited yesterday
answered yesterday


gwaughgwaugh
795312
795312
1
$begingroup$
1) no need forchr
: If the second parameter for strpos is an integer, it will be interpreted as an ASCII code. -> -5 bytes. 2) Two function parameters$s,$w
can save another 9 bytes.
$endgroup$
– Titus
yesterday
$begingroup$
@Titus, removing the chr() that's brilliant. Thx! The func params did occur to me too, I just didn't want to run afowl of input req's. I'll clarify w/OP.
$endgroup$
– gwaugh
yesterday
add a comment |
1
$begingroup$
1) no need forchr
: If the second parameter for strpos is an integer, it will be interpreted as an ASCII code. -> -5 bytes. 2) Two function parameters$s,$w
can save another 9 bytes.
$endgroup$
– Titus
yesterday
$begingroup$
@Titus, removing the chr() that's brilliant. Thx! The func params did occur to me too, I just didn't want to run afowl of input req's. I'll clarify w/OP.
$endgroup$
– gwaugh
yesterday
1
1
$begingroup$
1) no need for
chr
: If the second parameter for strpos is an integer, it will be interpreted as an ASCII code. -> -5 bytes. 2) Two function parameters $s,$w
can save another 9 bytes.$endgroup$
– Titus
yesterday
$begingroup$
1) no need for
chr
: If the second parameter for strpos is an integer, it will be interpreted as an ASCII code. -> -5 bytes. 2) Two function parameters $s,$w
can save another 9 bytes.$endgroup$
– Titus
yesterday
$begingroup$
@Titus, removing the chr() that's brilliant. Thx! The func params did occur to me too, I just didn't want to run afowl of input req's. I'll clarify w/OP.
$endgroup$
– gwaugh
yesterday
$begingroup$
@Titus, removing the chr() that's brilliant. Thx! The func params did occur to me too, I just didn't want to run afowl of input req's. I'll clarify w/OP.
$endgroup$
– gwaugh
yesterday
add a comment |
$begingroup$
Wolfram Language 37 58 bytes
My earlier entry did not correctly handle the case where the "odd character out" was at the upper left corner of the matrix. This does.
#~Position~Keys[TakeSmallest[Counts@Flatten@#,1]][[1]]&
Counts@Flatten@#
lists how many of each character are in the array, #
.
TakeSmallest[...,1]
returns the least frequent count, in the form of an association rule such as <| "Z"->1|>
Keys...[[1]]
returns the "key" to the only item in the association, that of the least used character. ("Z" in the present case)
#~Position~...
returns then position of the key in the original matrix, #
.
$endgroup$
add a comment |
$begingroup$
Wolfram Language 37 58 bytes
My earlier entry did not correctly handle the case where the "odd character out" was at the upper left corner of the matrix. This does.
#~Position~Keys[TakeSmallest[Counts@Flatten@#,1]][[1]]&
Counts@Flatten@#
lists how many of each character are in the array, #
.
TakeSmallest[...,1]
returns the least frequent count, in the form of an association rule such as <| "Z"->1|>
Keys...[[1]]
returns the "key" to the only item in the association, that of the least used character. ("Z" in the present case)
#~Position~...
returns then position of the key in the original matrix, #
.
$endgroup$
add a comment |
$begingroup$
Wolfram Language 37 58 bytes
My earlier entry did not correctly handle the case where the "odd character out" was at the upper left corner of the matrix. This does.
#~Position~Keys[TakeSmallest[Counts@Flatten@#,1]][[1]]&
Counts@Flatten@#
lists how many of each character are in the array, #
.
TakeSmallest[...,1]
returns the least frequent count, in the form of an association rule such as <| "Z"->1|>
Keys...[[1]]
returns the "key" to the only item in the association, that of the least used character. ("Z" in the present case)
#~Position~...
returns then position of the key in the original matrix, #
.
$endgroup$
Wolfram Language 37 58 bytes
My earlier entry did not correctly handle the case where the "odd character out" was at the upper left corner of the matrix. This does.
#~Position~Keys[TakeSmallest[Counts@Flatten@#,1]][[1]]&
Counts@Flatten@#
lists how many of each character are in the array, #
.
TakeSmallest[...,1]
returns the least frequent count, in the form of an association rule such as <| "Z"->1|>
Keys...[[1]]
returns the "key" to the only item in the association, that of the least used character. ("Z" in the present case)
#~Position~...
returns then position of the key in the original matrix, #
.
edited yesterday
answered yesterday


DavidCDavidC
24k243102
24k243102
add a comment |
add a comment |
$begingroup$
Java 8, 132 111 bytes
m->{int c=m[0][0],i=0,j;for(c=m[1][0]!=c?m[1][1]:c;;i++)for(j=m[i].length;j-->0;)if(m[i][j]!=c)return i+","+j;}
-8 bytes (and -13 more implicitly) thanks to @dana.
Input as character-matrix.
Try it online.
Explanation:
m->{ // Method with char-matrix parameter and String return-type
int c=m[0][0], // Character to check, starting at the one at position 0,0
i=0,j; // Index integers
for(c=m[1][0]!=c? // If the second character does not equal the first:
m[1][1] // Use the character at position 1,1 instead
:c; // Else: keep the character the same
;i++) // Loop `i` from 0 indefinitely upwards:
for(j=m[i].length;j-->0;)
// Inner loop `j` in the range [0, amount_of_columns):
if(m[i][j]!=c) // If the `i,j`'th character doesn't equal our character to check:
return i+","+j;}// Return `i,j` as result
$endgroup$
1
$begingroup$
124 - the finalreturn
statement should never get hit. There might be a better way to keep the outer loop going?
$endgroup$
– dana
7 hours ago
$begingroup$
@dana Thanks! As for: "There might be a better way to keep the outer loop going?", there certainly is; just removing it so it becomes an infinite loop. And then thereturn"";
is unreachable and can be removed as well. :D So -21 bytes thanks to you.
$endgroup$
– Kevin Cruijssen
6 hours ago
$begingroup$
Interesting... I had tried removing the outer loop condition and was getting anunreachable code
error. Didn't know that removing the finalreturn
was the fix.
$endgroup$
– dana
5 hours ago
add a comment |
$begingroup$
Java 8, 132 111 bytes
m->{int c=m[0][0],i=0,j;for(c=m[1][0]!=c?m[1][1]:c;;i++)for(j=m[i].length;j-->0;)if(m[i][j]!=c)return i+","+j;}
-8 bytes (and -13 more implicitly) thanks to @dana.
Input as character-matrix.
Try it online.
Explanation:
m->{ // Method with char-matrix parameter and String return-type
int c=m[0][0], // Character to check, starting at the one at position 0,0
i=0,j; // Index integers
for(c=m[1][0]!=c? // If the second character does not equal the first:
m[1][1] // Use the character at position 1,1 instead
:c; // Else: keep the character the same
;i++) // Loop `i` from 0 indefinitely upwards:
for(j=m[i].length;j-->0;)
// Inner loop `j` in the range [0, amount_of_columns):
if(m[i][j]!=c) // If the `i,j`'th character doesn't equal our character to check:
return i+","+j;}// Return `i,j` as result
$endgroup$
1
$begingroup$
124 - the finalreturn
statement should never get hit. There might be a better way to keep the outer loop going?
$endgroup$
– dana
7 hours ago
$begingroup$
@dana Thanks! As for: "There might be a better way to keep the outer loop going?", there certainly is; just removing it so it becomes an infinite loop. And then thereturn"";
is unreachable and can be removed as well. :D So -21 bytes thanks to you.
$endgroup$
– Kevin Cruijssen
6 hours ago
$begingroup$
Interesting... I had tried removing the outer loop condition and was getting anunreachable code
error. Didn't know that removing the finalreturn
was the fix.
$endgroup$
– dana
5 hours ago
add a comment |
$begingroup$
Java 8, 132 111 bytes
m->{int c=m[0][0],i=0,j;for(c=m[1][0]!=c?m[1][1]:c;;i++)for(j=m[i].length;j-->0;)if(m[i][j]!=c)return i+","+j;}
-8 bytes (and -13 more implicitly) thanks to @dana.
Input as character-matrix.
Try it online.
Explanation:
m->{ // Method with char-matrix parameter and String return-type
int c=m[0][0], // Character to check, starting at the one at position 0,0
i=0,j; // Index integers
for(c=m[1][0]!=c? // If the second character does not equal the first:
m[1][1] // Use the character at position 1,1 instead
:c; // Else: keep the character the same
;i++) // Loop `i` from 0 indefinitely upwards:
for(j=m[i].length;j-->0;)
// Inner loop `j` in the range [0, amount_of_columns):
if(m[i][j]!=c) // If the `i,j`'th character doesn't equal our character to check:
return i+","+j;}// Return `i,j` as result
$endgroup$
Java 8, 132 111 bytes
m->{int c=m[0][0],i=0,j;for(c=m[1][0]!=c?m[1][1]:c;;i++)for(j=m[i].length;j-->0;)if(m[i][j]!=c)return i+","+j;}
-8 bytes (and -13 more implicitly) thanks to @dana.
Input as character-matrix.
Try it online.
Explanation:
m->{ // Method with char-matrix parameter and String return-type
int c=m[0][0], // Character to check, starting at the one at position 0,0
i=0,j; // Index integers
for(c=m[1][0]!=c? // If the second character does not equal the first:
m[1][1] // Use the character at position 1,1 instead
:c; // Else: keep the character the same
;i++) // Loop `i` from 0 indefinitely upwards:
for(j=m[i].length;j-->0;)
// Inner loop `j` in the range [0, amount_of_columns):
if(m[i][j]!=c) // If the `i,j`'th character doesn't equal our character to check:
return i+","+j;}// Return `i,j` as result
edited 6 hours ago
answered yesterday


Kevin CruijssenKevin Cruijssen
37.4k556195
37.4k556195
1
$begingroup$
124 - the finalreturn
statement should never get hit. There might be a better way to keep the outer loop going?
$endgroup$
– dana
7 hours ago
$begingroup$
@dana Thanks! As for: "There might be a better way to keep the outer loop going?", there certainly is; just removing it so it becomes an infinite loop. And then thereturn"";
is unreachable and can be removed as well. :D So -21 bytes thanks to you.
$endgroup$
– Kevin Cruijssen
6 hours ago
$begingroup$
Interesting... I had tried removing the outer loop condition and was getting anunreachable code
error. Didn't know that removing the finalreturn
was the fix.
$endgroup$
– dana
5 hours ago
add a comment |
1
$begingroup$
124 - the finalreturn
statement should never get hit. There might be a better way to keep the outer loop going?
$endgroup$
– dana
7 hours ago
$begingroup$
@dana Thanks! As for: "There might be a better way to keep the outer loop going?", there certainly is; just removing it so it becomes an infinite loop. And then thereturn"";
is unreachable and can be removed as well. :D So -21 bytes thanks to you.
$endgroup$
– Kevin Cruijssen
6 hours ago
$begingroup$
Interesting... I had tried removing the outer loop condition and was getting anunreachable code
error. Didn't know that removing the finalreturn
was the fix.
$endgroup$
– dana
5 hours ago
1
1
$begingroup$
124 - the final
return
statement should never get hit. There might be a better way to keep the outer loop going?$endgroup$
– dana
7 hours ago
$begingroup$
124 - the final
return
statement should never get hit. There might be a better way to keep the outer loop going?$endgroup$
– dana
7 hours ago
$begingroup$
@dana Thanks! As for: "There might be a better way to keep the outer loop going?", there certainly is; just removing it so it becomes an infinite loop. And then the
return"";
is unreachable and can be removed as well. :D So -21 bytes thanks to you.$endgroup$
– Kevin Cruijssen
6 hours ago
$begingroup$
@dana Thanks! As for: "There might be a better way to keep the outer loop going?", there certainly is; just removing it so it becomes an infinite loop. And then the
return"";
is unreachable and can be removed as well. :D So -21 bytes thanks to you.$endgroup$
– Kevin Cruijssen
6 hours ago
$begingroup$
Interesting... I had tried removing the outer loop condition and was getting an
unreachable code
error. Didn't know that removing the final return
was the fix.$endgroup$
– dana
5 hours ago
$begingroup$
Interesting... I had tried removing the outer loop condition and was getting an
unreachable code
error. Didn't know that removing the final return
was the fix.$endgroup$
– dana
5 hours ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 109 108 bytes
First() => Last() for -1 byte
(a,w)=>{var d=a.Where(b=>b!=a[0]).Select(b=>a.IndexOf(b));return d.Count()>1?(0,0):(d.Last()%w,d.Last()/w);}
Try it online!
$endgroup$
$begingroup$
(a,w)=>
toa=>w=>
saves 1 byte.
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
You can try it here
$endgroup$
– Embodiment of Ignorance
yesterday
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 109 108 bytes
First() => Last() for -1 byte
(a,w)=>{var d=a.Where(b=>b!=a[0]).Select(b=>a.IndexOf(b));return d.Count()>1?(0,0):(d.Last()%w,d.Last()/w);}
Try it online!
$endgroup$
$begingroup$
(a,w)=>
toa=>w=>
saves 1 byte.
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
You can try it here
$endgroup$
– Embodiment of Ignorance
yesterday
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 109 108 bytes
First() => Last() for -1 byte
(a,w)=>{var d=a.Where(b=>b!=a[0]).Select(b=>a.IndexOf(b));return d.Count()>1?(0,0):(d.Last()%w,d.Last()/w);}
Try it online!
$endgroup$
C# (Visual C# Interactive Compiler), 109 108 bytes
First() => Last() for -1 byte
(a,w)=>{var d=a.Where(b=>b!=a[0]).Select(b=>a.IndexOf(b));return d.Count()>1?(0,0):(d.Last()%w,d.Last()/w);}
Try it online!
edited yesterday
answered yesterday


someonesomeone
492416
492416
$begingroup$
(a,w)=>
toa=>w=>
saves 1 byte.
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
You can try it here
$endgroup$
– Embodiment of Ignorance
yesterday
add a comment |
$begingroup$
(a,w)=>
toa=>w=>
saves 1 byte.
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
You can try it here
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
(a,w)=>
to a=>w=>
saves 1 byte.$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
(a,w)=>
to a=>w=>
saves 1 byte.$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
You can try it here
$endgroup$
– Embodiment of Ignorance
yesterday
$begingroup$
You can try it here
$endgroup$
– Embodiment of Ignorance
yesterday
add a comment |
$begingroup$
Python 3, 93 bytes
def f(s):x=s.find("n")+1;return[(i%x,i//x)for i,c in enumerate(s)if s.count(c)<2and" "<c][0]
Try it online!
Input is taken as a multiline string. Output is 0-indexed
$endgroup$
add a comment |
$begingroup$
Python 3, 93 bytes
def f(s):x=s.find("n")+1;return[(i%x,i//x)for i,c in enumerate(s)if s.count(c)<2and" "<c][0]
Try it online!
Input is taken as a multiline string. Output is 0-indexed
$endgroup$
add a comment |
$begingroup$
Python 3, 93 bytes
def f(s):x=s.find("n")+1;return[(i%x,i//x)for i,c in enumerate(s)if s.count(c)<2and" "<c][0]
Try it online!
Input is taken as a multiline string. Output is 0-indexed
$endgroup$
Python 3, 93 bytes
def f(s):x=s.find("n")+1;return[(i%x,i//x)for i,c in enumerate(s)if s.count(c)<2and" "<c][0]
Try it online!
Input is taken as a multiline string. Output is 0-indexed
answered yesterday
Black Owl KaiBlack Owl Kai
6108
6108
add a comment |
add a comment |
$begingroup$
JavaScript (ES6), 55 bytes
Takes input as $(s)(w)$, where $s$ is a string and $w$ is the width of the matrix. Returns $[x,y]$.
s=>w=>[(i=s.indexOf(/(.)1+(.)/.exec(s+s)[2]))%w,i/w|0]
Try it online!
JavaScript (ES6), 65 64 bytes
Saved 1 byte thanks to @Neil
Takes input as a matrix of characters. Returns $[x,y]$.
m=>m.some((r,y)=>r.some((c,x)=>!m[p=[x,y],~y&1].includes(c)))&&p
Try it online!
How?
We look for the first character $c$ located at $(x,y)$ which does not appear anywhere in another row $r[Y]$. We can perform this test on any row, as long as $Yne y$. Because the input matrix is guaranteed to be at least $2times 2$, we can simply use $Y=0$ if $y$ is odd or $Y=1$ if $y$ is even.
$endgroup$
1
$begingroup$
~y&1
saves a byte overy&1^1
.
$endgroup$
– Neil
yesterday
add a comment |
$begingroup$
JavaScript (ES6), 55 bytes
Takes input as $(s)(w)$, where $s$ is a string and $w$ is the width of the matrix. Returns $[x,y]$.
s=>w=>[(i=s.indexOf(/(.)1+(.)/.exec(s+s)[2]))%w,i/w|0]
Try it online!
JavaScript (ES6), 65 64 bytes
Saved 1 byte thanks to @Neil
Takes input as a matrix of characters. Returns $[x,y]$.
m=>m.some((r,y)=>r.some((c,x)=>!m[p=[x,y],~y&1].includes(c)))&&p
Try it online!
How?
We look for the first character $c$ located at $(x,y)$ which does not appear anywhere in another row $r[Y]$. We can perform this test on any row, as long as $Yne y$. Because the input matrix is guaranteed to be at least $2times 2$, we can simply use $Y=0$ if $y$ is odd or $Y=1$ if $y$ is even.
$endgroup$
1
$begingroup$
~y&1
saves a byte overy&1^1
.
$endgroup$
– Neil
yesterday
add a comment |
$begingroup$
JavaScript (ES6), 55 bytes
Takes input as $(s)(w)$, where $s$ is a string and $w$ is the width of the matrix. Returns $[x,y]$.
s=>w=>[(i=s.indexOf(/(.)1+(.)/.exec(s+s)[2]))%w,i/w|0]
Try it online!
JavaScript (ES6), 65 64 bytes
Saved 1 byte thanks to @Neil
Takes input as a matrix of characters. Returns $[x,y]$.
m=>m.some((r,y)=>r.some((c,x)=>!m[p=[x,y],~y&1].includes(c)))&&p
Try it online!
How?
We look for the first character $c$ located at $(x,y)$ which does not appear anywhere in another row $r[Y]$. We can perform this test on any row, as long as $Yne y$. Because the input matrix is guaranteed to be at least $2times 2$, we can simply use $Y=0$ if $y$ is odd or $Y=1$ if $y$ is even.
$endgroup$
JavaScript (ES6), 55 bytes
Takes input as $(s)(w)$, where $s$ is a string and $w$ is the width of the matrix. Returns $[x,y]$.
s=>w=>[(i=s.indexOf(/(.)1+(.)/.exec(s+s)[2]))%w,i/w|0]
Try it online!
JavaScript (ES6), 65 64 bytes
Saved 1 byte thanks to @Neil
Takes input as a matrix of characters. Returns $[x,y]$.
m=>m.some((r,y)=>r.some((c,x)=>!m[p=[x,y],~y&1].includes(c)))&&p
Try it online!
How?
We look for the first character $c$ located at $(x,y)$ which does not appear anywhere in another row $r[Y]$. We can perform this test on any row, as long as $Yne y$. Because the input matrix is guaranteed to be at least $2times 2$, we can simply use $Y=0$ if $y$ is odd or $Y=1$ if $y$ is even.
edited yesterday
answered yesterday


ArnauldArnauld
75k691314
75k691314
1
$begingroup$
~y&1
saves a byte overy&1^1
.
$endgroup$
– Neil
yesterday
add a comment |
1
$begingroup$
~y&1
saves a byte overy&1^1
.
$endgroup$
– Neil
yesterday
1
1
$begingroup$
~y&1
saves a byte over y&1^1
.$endgroup$
– Neil
yesterday
$begingroup$
~y&1
saves a byte over y&1^1
.$endgroup$
– Neil
yesterday
add a comment |
$begingroup$
Octave, 40 bytes
@(x){[r,c]=find(x-mode(+x(:))) [c,r]}{2}
Port of @sundar's MATL answer. Output is a two-element vector with 1-based column and row indices.
Try it online!
$endgroup$
add a comment |
$begingroup$
Octave, 40 bytes
@(x){[r,c]=find(x-mode(+x(:))) [c,r]}{2}
Port of @sundar's MATL answer. Output is a two-element vector with 1-based column and row indices.
Try it online!
$endgroup$
add a comment |
$begingroup$
Octave, 40 bytes
@(x){[r,c]=find(x-mode(+x(:))) [c,r]}{2}
Port of @sundar's MATL answer. Output is a two-element vector with 1-based column and row indices.
Try it online!
$endgroup$
Octave, 40 bytes
@(x){[r,c]=find(x-mode(+x(:))) [c,r]}{2}
Port of @sundar's MATL answer. Output is a two-element vector with 1-based column and row indices.
Try it online!
edited yesterday
answered yesterday


Luis MendoLuis Mendo
74.4k887291
74.4k887291
add a comment |
add a comment |
$begingroup$
Retina 0.8.2, 41 bytes
s`(?=(.)+1)(.*?¶)*(.*)(?!1|¶).+
$.3,$#2
Try it online! 0-indexed. Explanation:
s`
Allow .
to match newlines. This costs 3 bytes (3rd byte is the ?
before the ¶
) but saves 6 bytes.
(?=(.)+1)
Look ahead for two identical characters. 1
then becomes the hay.
(.*?¶)*
Count the number of newlines before the needle.
(.*)
Capture the hay to the left of the needle.
(?!1|¶)
Ensure that the needle isn't hay or a newline.
.+
Match the rest of the hay so that the result replaces it.
$.3,$#2
Output the width of the left hay and the number of newlines.
$endgroup$
add a comment |
$begingroup$
Retina 0.8.2, 41 bytes
s`(?=(.)+1)(.*?¶)*(.*)(?!1|¶).+
$.3,$#2
Try it online! 0-indexed. Explanation:
s`
Allow .
to match newlines. This costs 3 bytes (3rd byte is the ?
before the ¶
) but saves 6 bytes.
(?=(.)+1)
Look ahead for two identical characters. 1
then becomes the hay.
(.*?¶)*
Count the number of newlines before the needle.
(.*)
Capture the hay to the left of the needle.
(?!1|¶)
Ensure that the needle isn't hay or a newline.
.+
Match the rest of the hay so that the result replaces it.
$.3,$#2
Output the width of the left hay and the number of newlines.
$endgroup$
add a comment |
$begingroup$
Retina 0.8.2, 41 bytes
s`(?=(.)+1)(.*?¶)*(.*)(?!1|¶).+
$.3,$#2
Try it online! 0-indexed. Explanation:
s`
Allow .
to match newlines. This costs 3 bytes (3rd byte is the ?
before the ¶
) but saves 6 bytes.
(?=(.)+1)
Look ahead for two identical characters. 1
then becomes the hay.
(.*?¶)*
Count the number of newlines before the needle.
(.*)
Capture the hay to the left of the needle.
(?!1|¶)
Ensure that the needle isn't hay or a newline.
.+
Match the rest of the hay so that the result replaces it.
$.3,$#2
Output the width of the left hay and the number of newlines.
$endgroup$
Retina 0.8.2, 41 bytes
s`(?=(.)+1)(.*?¶)*(.*)(?!1|¶).+
$.3,$#2
Try it online! 0-indexed. Explanation:
s`
Allow .
to match newlines. This costs 3 bytes (3rd byte is the ?
before the ¶
) but saves 6 bytes.
(?=(.)+1)
Look ahead for two identical characters. 1
then becomes the hay.
(.*?¶)*
Count the number of newlines before the needle.
(.*)
Capture the hay to the left of the needle.
(?!1|¶)
Ensure that the needle isn't hay or a newline.
.+
Match the rest of the hay so that the result replaces it.
$.3,$#2
Output the width of the left hay and the number of newlines.
answered yesterday
NeilNeil
80.3k744178
80.3k744178
add a comment |
add a comment |
$begingroup$
Python 3, 93 bytes
def k(g):
t=''.join(g)
i,j=t.index({t.count(c):c for c in t}[1]),len(g[0])
return i//j,i%j
Try it online!
I know this could be a lot shorter, but I just wanted to try an approach around this dict
comprehension.
$endgroup$
add a comment |
$begingroup$
Python 3, 93 bytes
def k(g):
t=''.join(g)
i,j=t.index({t.count(c):c for c in t}[1]),len(g[0])
return i//j,i%j
Try it online!
I know this could be a lot shorter, but I just wanted to try an approach around this dict
comprehension.
$endgroup$
add a comment |
$begingroup$
Python 3, 93 bytes
def k(g):
t=''.join(g)
i,j=t.index({t.count(c):c for c in t}[1]),len(g[0])
return i//j,i%j
Try it online!
I know this could be a lot shorter, but I just wanted to try an approach around this dict
comprehension.
$endgroup$
Python 3, 93 bytes
def k(g):
t=''.join(g)
i,j=t.index({t.count(c):c for c in t}[1]),len(g[0])
return i//j,i%j
Try it online!
I know this could be a lot shorter, but I just wanted to try an approach around this dict
comprehension.
answered 18 hours ago


steenberghsteenbergh
6,85411739
6,85411739
add a comment |
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 82 bytes
x=>w=>{int y=x.IndexOf(x.GroupBy(c=>c).Last(g=>g.Count()<2).Key);return(y%w,y/w);}
Thanks to dana for shaving off 6 bytes!
Try it online!
Old solution, 106 bytes
n=>m=>{var z=n.Distinct();int d=n.IndexOf(n.Count(c=>c==z.First())>1?z.Last():z.First());return(d%m,d/m);}
Both take input as a string and an integer specifying the amount of columns.
Try it online!
$endgroup$
$begingroup$
@dana never knew thatEnumerable.Last()
accepted a delegate, thanks
$endgroup$
– Embodiment of Ignorance
9 hours ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 82 bytes
x=>w=>{int y=x.IndexOf(x.GroupBy(c=>c).Last(g=>g.Count()<2).Key);return(y%w,y/w);}
Thanks to dana for shaving off 6 bytes!
Try it online!
Old solution, 106 bytes
n=>m=>{var z=n.Distinct();int d=n.IndexOf(n.Count(c=>c==z.First())>1?z.Last():z.First());return(d%m,d/m);}
Both take input as a string and an integer specifying the amount of columns.
Try it online!
$endgroup$
$begingroup$
@dana never knew thatEnumerable.Last()
accepted a delegate, thanks
$endgroup$
– Embodiment of Ignorance
9 hours ago
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 82 bytes
x=>w=>{int y=x.IndexOf(x.GroupBy(c=>c).Last(g=>g.Count()<2).Key);return(y%w,y/w);}
Thanks to dana for shaving off 6 bytes!
Try it online!
Old solution, 106 bytes
n=>m=>{var z=n.Distinct();int d=n.IndexOf(n.Count(c=>c==z.First())>1?z.Last():z.First());return(d%m,d/m);}
Both take input as a string and an integer specifying the amount of columns.
Try it online!
$endgroup$
C# (Visual C# Interactive Compiler), 82 bytes
x=>w=>{int y=x.IndexOf(x.GroupBy(c=>c).Last(g=>g.Count()<2).Key);return(y%w,y/w);}
Thanks to dana for shaving off 6 bytes!
Try it online!
Old solution, 106 bytes
n=>m=>{var z=n.Distinct();int d=n.IndexOf(n.Count(c=>c==z.First())>1?z.Last():z.First());return(d%m,d/m);}
Both take input as a string and an integer specifying the amount of columns.
Try it online!
edited 9 hours ago
answered yesterday
Embodiment of IgnoranceEmbodiment of Ignorance
813118
813118
$begingroup$
@dana never knew thatEnumerable.Last()
accepted a delegate, thanks
$endgroup$
– Embodiment of Ignorance
9 hours ago
add a comment |
$begingroup$
@dana never knew thatEnumerable.Last()
accepted a delegate, thanks
$endgroup$
– Embodiment of Ignorance
9 hours ago
$begingroup$
@dana never knew that
Enumerable.Last()
accepted a delegate, thanks$endgroup$
– Embodiment of Ignorance
9 hours ago
$begingroup$
@dana never knew that
Enumerable.Last()
accepted a delegate, thanks$endgroup$
– Embodiment of Ignorance
9 hours ago
add a comment |
$begingroup$
Perl 5 -p00
, 52 bytes
/
/;$w="@+";/^(.)(1|
)+|^/;$_="@+"%$w.$".int"@+"/$w
-1 byte removing unnecessary /s
flag in second regex
TIO
How
-p00
: like-n
but also print, paragraph mode
/
: matches first new line character
/;- so
@+
array size is 1 and contains the position after the newline and$w="@+";
to store the width
/^(.)(1|
: matches either
)+|^/
- first character followed by one or more occurences of (same character or newline)
- or null string (position 0).
- so
@+
array size is one and contains the position of the "needle"
$_=
: assign the default input/argument variable
"@+"%$w
: the column index from 0
.$".
: concatenate with$"
space character and concatenate
int"@+"/$w
: the row index from 0
$endgroup$
add a comment |
$begingroup$
Perl 5 -p00
, 52 bytes
/
/;$w="@+";/^(.)(1|
)+|^/;$_="@+"%$w.$".int"@+"/$w
-1 byte removing unnecessary /s
flag in second regex
TIO
How
-p00
: like-n
but also print, paragraph mode
/
: matches first new line character
/;- so
@+
array size is 1 and contains the position after the newline and$w="@+";
to store the width
/^(.)(1|
: matches either
)+|^/
- first character followed by one or more occurences of (same character or newline)
- or null string (position 0).
- so
@+
array size is one and contains the position of the "needle"
$_=
: assign the default input/argument variable
"@+"%$w
: the column index from 0
.$".
: concatenate with$"
space character and concatenate
int"@+"/$w
: the row index from 0
$endgroup$
add a comment |
$begingroup$
Perl 5 -p00
, 52 bytes
/
/;$w="@+";/^(.)(1|
)+|^/;$_="@+"%$w.$".int"@+"/$w
-1 byte removing unnecessary /s
flag in second regex
TIO
How
-p00
: like-n
but also print, paragraph mode
/
: matches first new line character
/;- so
@+
array size is 1 and contains the position after the newline and$w="@+";
to store the width
/^(.)(1|
: matches either
)+|^/
- first character followed by one or more occurences of (same character or newline)
- or null string (position 0).
- so
@+
array size is one and contains the position of the "needle"
$_=
: assign the default input/argument variable
"@+"%$w
: the column index from 0
.$".
: concatenate with$"
space character and concatenate
int"@+"/$w
: the row index from 0
$endgroup$
Perl 5 -p00
, 52 bytes
/
/;$w="@+";/^(.)(1|
)+|^/;$_="@+"%$w.$".int"@+"/$w
-1 byte removing unnecessary /s
flag in second regex
TIO
How
-p00
: like-n
but also print, paragraph mode
/
: matches first new line character
/;- so
@+
array size is 1 and contains the position after the newline and$w="@+";
to store the width
/^(.)(1|
: matches either
)+|^/
- first character followed by one or more occurences of (same character or newline)
- or null string (position 0).
- so
@+
array size is one and contains the position of the "needle"
$_=
: assign the default input/argument variable
"@+"%$w
: the column index from 0
.$".
: concatenate with$"
space character and concatenate
int"@+"/$w
: the row index from 0
edited 5 hours ago
answered 19 hours ago
Nahuel FouilleulNahuel Fouilleul
2,09029
2,09029
add a comment |
add a comment |
$begingroup$
MATL, 11 bytes
tX:YmyYk-&f
Output is row, then column; 1-based.
Try it online!
Explanation
t % Implicit input. Duplicate
X: % Linearize into a column
Ym % Compute mean (characters are converted to ASCII codes)
y % Duplicate from below: pushes input again
Yk % Closest value: gives the input value that is closest to the mean
- % Subtract, element-wise. Gives non-zero for the value farthest from the mean
&f % Two-output find: gives row and column indices of nonzeros. Implicit display
$endgroup$
add a comment |
$begingroup$
MATL, 11 bytes
tX:YmyYk-&f
Output is row, then column; 1-based.
Try it online!
Explanation
t % Implicit input. Duplicate
X: % Linearize into a column
Ym % Compute mean (characters are converted to ASCII codes)
y % Duplicate from below: pushes input again
Yk % Closest value: gives the input value that is closest to the mean
- % Subtract, element-wise. Gives non-zero for the value farthest from the mean
&f % Two-output find: gives row and column indices of nonzeros. Implicit display
$endgroup$
add a comment |
$begingroup$
MATL, 11 bytes
tX:YmyYk-&f
Output is row, then column; 1-based.
Try it online!
Explanation
t % Implicit input. Duplicate
X: % Linearize into a column
Ym % Compute mean (characters are converted to ASCII codes)
y % Duplicate from below: pushes input again
Yk % Closest value: gives the input value that is closest to the mean
- % Subtract, element-wise. Gives non-zero for the value farthest from the mean
&f % Two-output find: gives row and column indices of nonzeros. Implicit display
$endgroup$
MATL, 11 bytes
tX:YmyYk-&f
Output is row, then column; 1-based.
Try it online!
Explanation
t % Implicit input. Duplicate
X: % Linearize into a column
Ym % Compute mean (characters are converted to ASCII codes)
y % Duplicate from below: pushes input again
Yk % Closest value: gives the input value that is closest to the mean
- % Subtract, element-wise. Gives non-zero for the value farthest from the mean
&f % Two-output find: gives row and column indices of nonzeros. Implicit display
edited yesterday
answered yesterday


Luis MendoLuis Mendo
74.4k887291
74.4k887291
add a comment |
add a comment |
$begingroup$
Pyth, 15 14 12 bytes
.Dxz-zh.-z{z
Takes input as the length of the row and the input without lines and outputs as [row, column].
Try it here
Explanation
.Dxz-zh.-z{z
.-z{z Subtract one of each character from the input.
h Take the first.
-z Remove all instances from the input.
xz Find the remaining character in the input.
.D Q Take the result divmod the (implicit) length of the row.
Old approach
mxJmt{kdeSJ.TB
Try it here
Explanation
mxJmt{kdeSJ.TB
.TBQ Take the (implicit) input and its transpose...
m d ... and for each...
mt{k ... deduplicate each row...
xJ eSJ ... and find the index of the largest.
$endgroup$
add a comment |
$begingroup$
Pyth, 15 14 12 bytes
.Dxz-zh.-z{z
Takes input as the length of the row and the input without lines and outputs as [row, column].
Try it here
Explanation
.Dxz-zh.-z{z
.-z{z Subtract one of each character from the input.
h Take the first.
-z Remove all instances from the input.
xz Find the remaining character in the input.
.D Q Take the result divmod the (implicit) length of the row.
Old approach
mxJmt{kdeSJ.TB
Try it here
Explanation
mxJmt{kdeSJ.TB
.TBQ Take the (implicit) input and its transpose...
m d ... and for each...
mt{k ... deduplicate each row...
xJ eSJ ... and find the index of the largest.
$endgroup$
add a comment |
$begingroup$
Pyth, 15 14 12 bytes
.Dxz-zh.-z{z
Takes input as the length of the row and the input without lines and outputs as [row, column].
Try it here
Explanation
.Dxz-zh.-z{z
.-z{z Subtract one of each character from the input.
h Take the first.
-z Remove all instances from the input.
xz Find the remaining character in the input.
.D Q Take the result divmod the (implicit) length of the row.
Old approach
mxJmt{kdeSJ.TB
Try it here
Explanation
mxJmt{kdeSJ.TB
.TBQ Take the (implicit) input and its transpose...
m d ... and for each...
mt{k ... deduplicate each row...
xJ eSJ ... and find the index of the largest.
$endgroup$
Pyth, 15 14 12 bytes
.Dxz-zh.-z{z
Takes input as the length of the row and the input without lines and outputs as [row, column].
Try it here
Explanation
.Dxz-zh.-z{z
.-z{z Subtract one of each character from the input.
h Take the first.
-z Remove all instances from the input.
xz Find the remaining character in the input.
.D Q Take the result divmod the (implicit) length of the row.
Old approach
mxJmt{kdeSJ.TB
Try it here
Explanation
mxJmt{kdeSJ.TB
.TBQ Take the (implicit) input and its transpose...
m d ... and for each...
mt{k ... deduplicate each row...
xJ eSJ ... and find the index of the largest.
edited yesterday
answered yesterday
MnemonicMnemonic
4,6951730
4,6951730
add a comment |
add a comment |
$begingroup$
Charcoal, 40 bytes
≔§⎇⌕θ§θ¹ηθ⁰ζSθW⁼№θζLθ«⊞υωSθ»I⌕Eθ⁼ιζ⁰,ILυ
Try it online! Link is to verbose version of code. I must be doing something wrong because this is almost as long as the Retina answer. Explanation:
≔§⎇⌕θ§θ¹ηθ⁰ζ
Check whether the second character in the first string is also the first character, and take the first character of the first string if so otherwise the first character of the second string if not. This is then the hay.
SθW⁼№θζLθ«⊞υωSθ»
Keep reading strings until a string whose hay is less than its length is found.
I⌕Eθ⁼ιζ⁰,ILυ
Output the position of the mismatching element and then the number of strings previously read.
$endgroup$
add a comment |
$begingroup$
Charcoal, 40 bytes
≔§⎇⌕θ§θ¹ηθ⁰ζSθW⁼№θζLθ«⊞υωSθ»I⌕Eθ⁼ιζ⁰,ILυ
Try it online! Link is to verbose version of code. I must be doing something wrong because this is almost as long as the Retina answer. Explanation:
≔§⎇⌕θ§θ¹ηθ⁰ζ
Check whether the second character in the first string is also the first character, and take the first character of the first string if so otherwise the first character of the second string if not. This is then the hay.
SθW⁼№θζLθ«⊞υωSθ»
Keep reading strings until a string whose hay is less than its length is found.
I⌕Eθ⁼ιζ⁰,ILυ
Output the position of the mismatching element and then the number of strings previously read.
$endgroup$
add a comment |
$begingroup$
Charcoal, 40 bytes
≔§⎇⌕θ§θ¹ηθ⁰ζSθW⁼№θζLθ«⊞υωSθ»I⌕Eθ⁼ιζ⁰,ILυ
Try it online! Link is to verbose version of code. I must be doing something wrong because this is almost as long as the Retina answer. Explanation:
≔§⎇⌕θ§θ¹ηθ⁰ζ
Check whether the second character in the first string is also the first character, and take the first character of the first string if so otherwise the first character of the second string if not. This is then the hay.
SθW⁼№θζLθ«⊞υωSθ»
Keep reading strings until a string whose hay is less than its length is found.
I⌕Eθ⁼ιζ⁰,ILυ
Output the position of the mismatching element and then the number of strings previously read.
$endgroup$
Charcoal, 40 bytes
≔§⎇⌕θ§θ¹ηθ⁰ζSθW⁼№θζLθ«⊞υωSθ»I⌕Eθ⁼ιζ⁰,ILυ
Try it online! Link is to verbose version of code. I must be doing something wrong because this is almost as long as the Retina answer. Explanation:
≔§⎇⌕θ§θ¹ηθ⁰ζ
Check whether the second character in the first string is also the first character, and take the first character of the first string if so otherwise the first character of the second string if not. This is then the hay.
SθW⁼№θζLθ«⊞υωSθ»
Keep reading strings until a string whose hay is less than its length is found.
I⌕Eθ⁼ιζ⁰,ILυ
Output the position of the mismatching element and then the number of strings previously read.
answered yesterday
NeilNeil
80.3k744178
80.3k744178
add a comment |
add a comment |
$begingroup$
MATLAB, 68 22 bytes
[r,c]=find(v~=v(1));if size(r,1)>1 disp([1,1]);else disp([r,c]);end;
If I could exclude any one case, such as [1,1]
in this solution, I could have saved several bytes.
Updated solution:
@(v)find(v-mode(v(:)))
Thanks to @sundar for helping me with the special case problem and saving 42 bytes! Also, thanks to @Luis_Mendo for the suggestions and saving me another 2 bytes!
$endgroup$
$begingroup$
I think you can get rid of the check for[1,1]
case by usingmode(v(:))
instead ofv(1)
.
$endgroup$
– sundar
yesterday
$begingroup$
You need to wrap your code so that it is a full program or a function; you cannot assume that the input is in a variablev
. Also, you can probably replace~=
by-
, and remove the final;
$endgroup$
– Luis Mendo
yesterday
add a comment |
$begingroup$
MATLAB, 68 22 bytes
[r,c]=find(v~=v(1));if size(r,1)>1 disp([1,1]);else disp([r,c]);end;
If I could exclude any one case, such as [1,1]
in this solution, I could have saved several bytes.
Updated solution:
@(v)find(v-mode(v(:)))
Thanks to @sundar for helping me with the special case problem and saving 42 bytes! Also, thanks to @Luis_Mendo for the suggestions and saving me another 2 bytes!
$endgroup$
$begingroup$
I think you can get rid of the check for[1,1]
case by usingmode(v(:))
instead ofv(1)
.
$endgroup$
– sundar
yesterday
$begingroup$
You need to wrap your code so that it is a full program or a function; you cannot assume that the input is in a variablev
. Also, you can probably replace~=
by-
, and remove the final;
$endgroup$
– Luis Mendo
yesterday
add a comment |
$begingroup$
MATLAB, 68 22 bytes
[r,c]=find(v~=v(1));if size(r,1)>1 disp([1,1]);else disp([r,c]);end;
If I could exclude any one case, such as [1,1]
in this solution, I could have saved several bytes.
Updated solution:
@(v)find(v-mode(v(:)))
Thanks to @sundar for helping me with the special case problem and saving 42 bytes! Also, thanks to @Luis_Mendo for the suggestions and saving me another 2 bytes!
$endgroup$
MATLAB, 68 22 bytes
[r,c]=find(v~=v(1));if size(r,1)>1 disp([1,1]);else disp([r,c]);end;
If I could exclude any one case, such as [1,1]
in this solution, I could have saved several bytes.
Updated solution:
@(v)find(v-mode(v(:)))
Thanks to @sundar for helping me with the special case problem and saving 42 bytes! Also, thanks to @Luis_Mendo for the suggestions and saving me another 2 bytes!
edited yesterday
answered yesterday
DimPDimP
15117
15117
$begingroup$
I think you can get rid of the check for[1,1]
case by usingmode(v(:))
instead ofv(1)
.
$endgroup$
– sundar
yesterday
$begingroup$
You need to wrap your code so that it is a full program or a function; you cannot assume that the input is in a variablev
. Also, you can probably replace~=
by-
, and remove the final;
$endgroup$
– Luis Mendo
yesterday
add a comment |
$begingroup$
I think you can get rid of the check for[1,1]
case by usingmode(v(:))
instead ofv(1)
.
$endgroup$
– sundar
yesterday
$begingroup$
You need to wrap your code so that it is a full program or a function; you cannot assume that the input is in a variablev
. Also, you can probably replace~=
by-
, and remove the final;
$endgroup$
– Luis Mendo
yesterday
$begingroup$
I think you can get rid of the check for
[1,1]
case by using mode(v(:))
instead of v(1)
.$endgroup$
– sundar
yesterday
$begingroup$
I think you can get rid of the check for
[1,1]
case by using mode(v(:))
instead of v(1)
.$endgroup$
– sundar
yesterday
$begingroup$
You need to wrap your code so that it is a full program or a function; you cannot assume that the input is in a variable
v
. Also, you can probably replace ~=
by -
, and remove the final ;
$endgroup$
– Luis Mendo
yesterday
$begingroup$
You need to wrap your code so that it is a full program or a function; you cannot assume that the input is in a variable
v
. Also, you can probably replace ~=
by -
, and remove the final ;
$endgroup$
– Luis Mendo
yesterday
add a comment |
$begingroup$
Röda, 81 bytes
f a{i=indexOf;l=i("
",a)+1;chars a|sort|count|[[_2,_1]]|min|i _[1],a|[_%l,_1//l]}
Try it online!
Takes input as a string containing newline-terminated lines. Returns a stream containing 0-indexed horizontal and vertical indexes.
$endgroup$
add a comment |
$begingroup$
Röda, 81 bytes
f a{i=indexOf;l=i("
",a)+1;chars a|sort|count|[[_2,_1]]|min|i _[1],a|[_%l,_1//l]}
Try it online!
Takes input as a string containing newline-terminated lines. Returns a stream containing 0-indexed horizontal and vertical indexes.
$endgroup$
add a comment |
$begingroup$
Röda, 81 bytes
f a{i=indexOf;l=i("
",a)+1;chars a|sort|count|[[_2,_1]]|min|i _[1],a|[_%l,_1//l]}
Try it online!
Takes input as a string containing newline-terminated lines. Returns a stream containing 0-indexed horizontal and vertical indexes.
$endgroup$
Röda, 81 bytes
f a{i=indexOf;l=i("
",a)+1;chars a|sort|count|[[_2,_1]]|min|i _[1],a|[_%l,_1//l]}
Try it online!
Takes input as a string containing newline-terminated lines. Returns a stream containing 0-indexed horizontal and vertical indexes.
answered yesterday


fergusqfergusq
4,65211036
4,65211036
add a comment |
add a comment |
$begingroup$
Perl 5 -n0
, 77 bytes
$t=(@b=sort/./g)[0]eq$b[1]?pop@b:$b[0];++$y&/Q$t/g&&say"$y,",pos for /.+$/mg
Try it online!
Output is row,column
starting from the top left, 1-indexed
$endgroup$
add a comment |
$begingroup$
Perl 5 -n0
, 77 bytes
$t=(@b=sort/./g)[0]eq$b[1]?pop@b:$b[0];++$y&/Q$t/g&&say"$y,",pos for /.+$/mg
Try it online!
Output is row,column
starting from the top left, 1-indexed
$endgroup$
add a comment |
$begingroup$
Perl 5 -n0
, 77 bytes
$t=(@b=sort/./g)[0]eq$b[1]?pop@b:$b[0];++$y&/Q$t/g&&say"$y,",pos for /.+$/mg
Try it online!
Output is row,column
starting from the top left, 1-indexed
$endgroup$
Perl 5 -n0
, 77 bytes
$t=(@b=sort/./g)[0]eq$b[1]?pop@b:$b[0];++$y&/Q$t/g&&say"$y,",pos for /.+$/mg
Try it online!
Output is row,column
starting from the top left, 1-indexed
edited 23 hours ago
answered yesterday
XcaliXcali
5,265520
5,265520
add a comment |
add a comment |
$begingroup$
C (clang), 83 bytes
a,i;h(char*s,z,x){for(i=0;++i<z;)*s-*++s?a=i-(*s==s[1]):0;printf("%d,%d",a%x,a/x);}
Try it online!
$endgroup$
add a comment |
$begingroup$
C (clang), 83 bytes
a,i;h(char*s,z,x){for(i=0;++i<z;)*s-*++s?a=i-(*s==s[1]):0;printf("%d,%d",a%x,a/x);}
Try it online!
$endgroup$
add a comment |
$begingroup$
C (clang), 83 bytes
a,i;h(char*s,z,x){for(i=0;++i<z;)*s-*++s?a=i-(*s==s[1]):0;printf("%d,%d",a%x,a/x);}
Try it online!
$endgroup$
C (clang), 83 bytes
a,i;h(char*s,z,x){for(i=0;++i<z;)*s-*++s?a=i-(*s==s[1]):0;printf("%d,%d",a%x,a/x);}
Try it online!
edited 21 hours ago
answered yesterday
AZTECCOAZTECCO
614
614
add a comment |
add a comment |
$begingroup$
Haskell, 64 bytes
f s=[(r,c)|c<-[0..],r<-[0..length s-1],any(notElem$s!!r!!c)s]!!0
Try it online! f
takes a list of lines and returns zero-indexed (row,column)
.
Works by iterating through the columns and rows and looking for a character which does not appear on all rows.
$endgroup$
add a comment |
$begingroup$
Haskell, 64 bytes
f s=[(r,c)|c<-[0..],r<-[0..length s-1],any(notElem$s!!r!!c)s]!!0
Try it online! f
takes a list of lines and returns zero-indexed (row,column)
.
Works by iterating through the columns and rows and looking for a character which does not appear on all rows.
$endgroup$
add a comment |
$begingroup$
Haskell, 64 bytes
f s=[(r,c)|c<-[0..],r<-[0..length s-1],any(notElem$s!!r!!c)s]!!0
Try it online! f
takes a list of lines and returns zero-indexed (row,column)
.
Works by iterating through the columns and rows and looking for a character which does not appear on all rows.
$endgroup$
Haskell, 64 bytes
f s=[(r,c)|c<-[0..],r<-[0..length s-1],any(notElem$s!!r!!c)s]!!0
Try it online! f
takes a list of lines and returns zero-indexed (row,column)
.
Works by iterating through the columns and rows and looking for a character which does not appear on all rows.
answered 18 hours ago


LaikoniLaikoni
19.9k43699
19.9k43699
add a comment |
add a comment |
$begingroup$
C# .NET, 133 112 111 bytes
m=>{var c=m[0][0];c=m[1][0]!=c?m[1][1]:c;for(int i=0,j;;i++)for(j=m[i].Count;j-->0;)if(m[i][j]!=c)return(i,j);}
-1 byte thanks to @EmbodimentOfIgnorance.
Port of my Java answer, but with a List<List<char>>
input and int
-tuple output, instead of char
input and string
output.
Try it online.
$endgroup$
1
$begingroup$
Can't you save two bytes by turning youchar
into aList<List<char>>
? SinceList<T>.Count
is shorter thanArray.Length
$endgroup$
– Embodiment of Ignorance
8 hours ago
$begingroup$
@EmbodimentofIgnorance Thanks! :)
$endgroup$
– Kevin Cruijssen
6 hours ago
add a comment |
$begingroup$
C# .NET, 133 112 111 bytes
m=>{var c=m[0][0];c=m[1][0]!=c?m[1][1]:c;for(int i=0,j;;i++)for(j=m[i].Count;j-->0;)if(m[i][j]!=c)return(i,j);}
-1 byte thanks to @EmbodimentOfIgnorance.
Port of my Java answer, but with a List<List<char>>
input and int
-tuple output, instead of char
input and string
output.
Try it online.
$endgroup$
1
$begingroup$
Can't you save two bytes by turning youchar
into aList<List<char>>
? SinceList<T>.Count
is shorter thanArray.Length
$endgroup$
– Embodiment of Ignorance
8 hours ago
$begingroup$
@EmbodimentofIgnorance Thanks! :)
$endgroup$
– Kevin Cruijssen
6 hours ago
add a comment |
$begingroup$
C# .NET, 133 112 111 bytes
m=>{var c=m[0][0];c=m[1][0]!=c?m[1][1]:c;for(int i=0,j;;i++)for(j=m[i].Count;j-->0;)if(m[i][j]!=c)return(i,j);}
-1 byte thanks to @EmbodimentOfIgnorance.
Port of my Java answer, but with a List<List<char>>
input and int
-tuple output, instead of char
input and string
output.
Try it online.
$endgroup$
C# .NET, 133 112 111 bytes
m=>{var c=m[0][0];c=m[1][0]!=c?m[1][1]:c;for(int i=0,j;;i++)for(j=m[i].Count;j-->0;)if(m[i][j]!=c)return(i,j);}
-1 byte thanks to @EmbodimentOfIgnorance.
Port of my Java answer, but with a List<List<char>>
input and int
-tuple output, instead of char
input and string
output.
Try it online.
edited 6 hours ago
answered yesterday


Kevin CruijssenKevin Cruijssen
37.4k556195
37.4k556195
1
$begingroup$
Can't you save two bytes by turning youchar
into aList<List<char>>
? SinceList<T>.Count
is shorter thanArray.Length
$endgroup$
– Embodiment of Ignorance
8 hours ago
$begingroup$
@EmbodimentofIgnorance Thanks! :)
$endgroup$
– Kevin Cruijssen
6 hours ago
add a comment |
1
$begingroup$
Can't you save two bytes by turning youchar
into aList<List<char>>
? SinceList<T>.Count
is shorter thanArray.Length
$endgroup$
– Embodiment of Ignorance
8 hours ago
$begingroup$
@EmbodimentofIgnorance Thanks! :)
$endgroup$
– Kevin Cruijssen
6 hours ago
1
1
$begingroup$
Can't you save two bytes by turning you
char
into a List<List<char>>
? Since List<T>.Count
is shorter than Array.Length
$endgroup$
– Embodiment of Ignorance
8 hours ago
$begingroup$
Can't you save two bytes by turning you
char
into a List<List<char>>
? Since List<T>.Count
is shorter than Array.Length
$endgroup$
– Embodiment of Ignorance
8 hours ago
$begingroup$
@EmbodimentofIgnorance Thanks! :)
$endgroup$
– Kevin Cruijssen
6 hours ago
$begingroup$
@EmbodimentofIgnorance Thanks! :)
$endgroup$
– Kevin Cruijssen
6 hours ago
add a comment |
If this is an answer to a challenge…
…Be sure to follow the challenge specification. However, please refrain from exploiting obvious loopholes. Answers abusing any of the standard loopholes are considered invalid. If you think a specification is unclear or underspecified, comment on the question instead.
…Try to optimize your score. For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one.
Explanations of your answer make it more interesting to read and are very much encouraged.…Include a short header which indicates the language(s) of your code and its score, as defined by the challenge.
More generally…
…Please make sure to answer the question and provide sufficient detail.
…Avoid asking for help, clarification or responding to other answers (use comments instead).
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f179351%2ffind-the-needle-in-the-haystack%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QB,dj,vpxEuL,BJmRe Ye,pjwsjgvqi0GnXo,h5,pQ2Mz7zzx,n,RQ3Fau,ZJsqCXBhS 6m5veC0mhJJU,Tefhn,I5,yeai
$begingroup$
Suggested test case:
88n8Z
(with any two characters of course).$endgroup$
– Kevin Cruijssen
yesterday
$begingroup$
Can we take input as a multi-dimensional array? i.e. [ ['#','#','#','#','#'], ['#','#','#','N','#'], ['#','#','#','#','#'], ['#','#','#','#','#'] ];
$endgroup$
– gwaugh
yesterday
2
$begingroup$
@gwaugh Like a list of list of characters? Yes, that's fine (and explicitly called out as OK).
$endgroup$
– AdmBorkBork
yesterday
2
$begingroup$
Can we take input as a pair of a string without newlines and the width (or height) of the haystack? i.e.
("########N###########", 5)
$endgroup$
– someone
yesterday
2
$begingroup$
@someone Yes, though it doesn't have a real quorum, I feel that should be allowed.
$endgroup$
– AdmBorkBork
yesterday