Format Data In DataTable With or Without LINQ in VB.NET
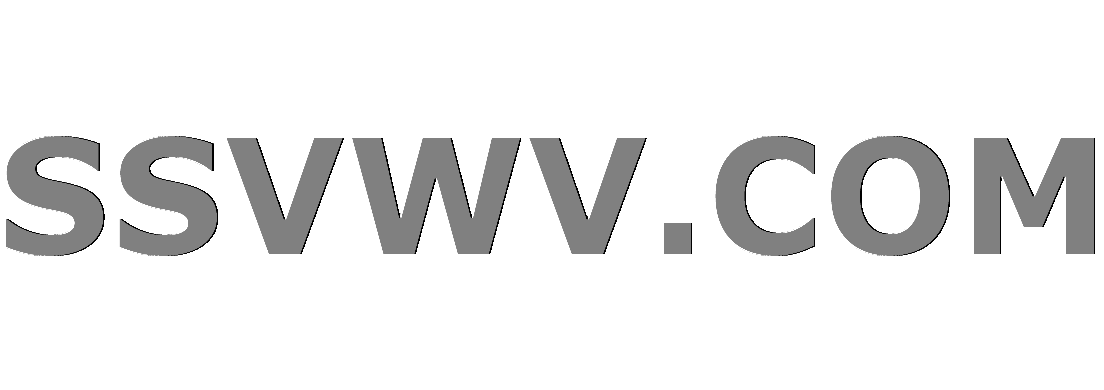
Multi tool use
I'm having a little trouble trying to get some data to format properly.
I'm not sure if LINQ is helpful in this case but below is:
Expected output
March 14
2:00 PM - 7:00 PM | Registration
5:30 PM - 7:00 PM | Meetup
7:30 PM - 9:00 PM | Dinner
March 15
2:00 PM - 7:00 PM | Registration
5:30 PM - 7:00 PM | Meetup
Current data output
March 14
2:00 PM - 7:00 PM | Registration
March 14
5:30 PM - 7:00 PM | Meetup
March 14
7:30 PM - 9:00 PM | Dinner
March 15
2:00 PM - 7:00 PM | Registration
March 15
6:00 PM - 7:00 PM | Meetup
Code Structure
Imports System
Imports System.Globalization
Imports System.Data
Imports System.Data.DataSetExtensions
Public Module Module1
Public Sub Main()
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
For Each session In sessions.AsEnumerable()
Dim sessionID = session.Item("SessionID")
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
Console.WriteLine("{0}", sessionDate)
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
'Add 5 Rows of Data to test
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
End With
Return table
End Function
End Module
I've referenced other SO questions such as THIS but I'm not sure if LINQ would make the expected output easier to obtain.
Any suggestions are welcomed. I'm trying to possibly add a nested for Each loop and then add a condition if the sessionDate is the same.
****** UPDATE *******
I really appreciate the feedback and helpful answers. I'm trying to do one more format change to the data. I have to add a specific SVG Icon to all the events items. The SVG icons are specific to the First, Last, Rest of events.
I'm trying to use the LINQ answer provided but I'm not well versed in using it yet but it does seem very easy to read. For the purposes of this example, A String variable can be used as place holders for the first / rest / last.
I've also added my attempt to try to add the icon but I was not using LINQ.
Below is the Updated Code and Expected Output
EXPECTED OUTPUT
March 14
(Start SVG) 2:00 PM - 7:00 PM | Registration
(Body SVG) 5:30 PM - 7:00 PM | Meetup
(End SVG) 7:30 PM - 9:00 PM | Dinner
March 15
(Start SVG) 2:00 PM - 7:00 PM | Registration
(End SVG) 5:30 PM - 7:00 PM | Meetup
Code Structure
Imports System
Imports System.Globalization
Imports System.Data
Imports System.Data.DataSetExtensions
Public Module Module1
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessionIcon = String.Empty
'Dim Sessions = SampleTable().AsEnumerable()
'For Each session In Sessions
' If Sessions.Rows.IndexOf(session) = 0 Then
' sessionIcon = "<svg>Start Icon</svg>"
' ElseIf Sessions.Rows.IndexOf(session) = (Sessions.Rows.Count - 1) Then
' sessionIcon = "<svg>End Icon</svg>"
' Else
' sessionIcon = "<svg>Rest Icon</svg>"
' End If
'Next
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key.SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key.SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key.SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key.SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Console.WriteLine("{0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName)
Next
Next
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
'Add 5 Rows of Data to test
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
End With
Return table
End Function
End Module
vb.net linq
add a comment |
I'm having a little trouble trying to get some data to format properly.
I'm not sure if LINQ is helpful in this case but below is:
Expected output
March 14
2:00 PM - 7:00 PM | Registration
5:30 PM - 7:00 PM | Meetup
7:30 PM - 9:00 PM | Dinner
March 15
2:00 PM - 7:00 PM | Registration
5:30 PM - 7:00 PM | Meetup
Current data output
March 14
2:00 PM - 7:00 PM | Registration
March 14
5:30 PM - 7:00 PM | Meetup
March 14
7:30 PM - 9:00 PM | Dinner
March 15
2:00 PM - 7:00 PM | Registration
March 15
6:00 PM - 7:00 PM | Meetup
Code Structure
Imports System
Imports System.Globalization
Imports System.Data
Imports System.Data.DataSetExtensions
Public Module Module1
Public Sub Main()
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
For Each session In sessions.AsEnumerable()
Dim sessionID = session.Item("SessionID")
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
Console.WriteLine("{0}", sessionDate)
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
'Add 5 Rows of Data to test
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
End With
Return table
End Function
End Module
I've referenced other SO questions such as THIS but I'm not sure if LINQ would make the expected output easier to obtain.
Any suggestions are welcomed. I'm trying to possibly add a nested for Each loop and then add a condition if the sessionDate is the same.
****** UPDATE *******
I really appreciate the feedback and helpful answers. I'm trying to do one more format change to the data. I have to add a specific SVG Icon to all the events items. The SVG icons are specific to the First, Last, Rest of events.
I'm trying to use the LINQ answer provided but I'm not well versed in using it yet but it does seem very easy to read. For the purposes of this example, A String variable can be used as place holders for the first / rest / last.
I've also added my attempt to try to add the icon but I was not using LINQ.
Below is the Updated Code and Expected Output
EXPECTED OUTPUT
March 14
(Start SVG) 2:00 PM - 7:00 PM | Registration
(Body SVG) 5:30 PM - 7:00 PM | Meetup
(End SVG) 7:30 PM - 9:00 PM | Dinner
March 15
(Start SVG) 2:00 PM - 7:00 PM | Registration
(End SVG) 5:30 PM - 7:00 PM | Meetup
Code Structure
Imports System
Imports System.Globalization
Imports System.Data
Imports System.Data.DataSetExtensions
Public Module Module1
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessionIcon = String.Empty
'Dim Sessions = SampleTable().AsEnumerable()
'For Each session In Sessions
' If Sessions.Rows.IndexOf(session) = 0 Then
' sessionIcon = "<svg>Start Icon</svg>"
' ElseIf Sessions.Rows.IndexOf(session) = (Sessions.Rows.Count - 1) Then
' sessionIcon = "<svg>End Icon</svg>"
' Else
' sessionIcon = "<svg>Rest Icon</svg>"
' End If
'Next
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key.SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key.SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key.SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key.SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Console.WriteLine("{0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName)
Next
Next
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
'Add 5 Rows of Data to test
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
End With
Return table
End Function
End Module
vb.net linq
If there are additional questions, it is better to do it before marking as resolved. If it has been settled, there is a high possibility that everyone will not notice the question.
– m mukai
Nov 28 '18 at 0:52
Sorry about that. I didn't need the extra formatting so the question remains resolved.
– cpt-crunchy
Nov 29 '18 at 17:23
add a comment |
I'm having a little trouble trying to get some data to format properly.
I'm not sure if LINQ is helpful in this case but below is:
Expected output
March 14
2:00 PM - 7:00 PM | Registration
5:30 PM - 7:00 PM | Meetup
7:30 PM - 9:00 PM | Dinner
March 15
2:00 PM - 7:00 PM | Registration
5:30 PM - 7:00 PM | Meetup
Current data output
March 14
2:00 PM - 7:00 PM | Registration
March 14
5:30 PM - 7:00 PM | Meetup
March 14
7:30 PM - 9:00 PM | Dinner
March 15
2:00 PM - 7:00 PM | Registration
March 15
6:00 PM - 7:00 PM | Meetup
Code Structure
Imports System
Imports System.Globalization
Imports System.Data
Imports System.Data.DataSetExtensions
Public Module Module1
Public Sub Main()
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
For Each session In sessions.AsEnumerable()
Dim sessionID = session.Item("SessionID")
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
Console.WriteLine("{0}", sessionDate)
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
'Add 5 Rows of Data to test
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
End With
Return table
End Function
End Module
I've referenced other SO questions such as THIS but I'm not sure if LINQ would make the expected output easier to obtain.
Any suggestions are welcomed. I'm trying to possibly add a nested for Each loop and then add a condition if the sessionDate is the same.
****** UPDATE *******
I really appreciate the feedback and helpful answers. I'm trying to do one more format change to the data. I have to add a specific SVG Icon to all the events items. The SVG icons are specific to the First, Last, Rest of events.
I'm trying to use the LINQ answer provided but I'm not well versed in using it yet but it does seem very easy to read. For the purposes of this example, A String variable can be used as place holders for the first / rest / last.
I've also added my attempt to try to add the icon but I was not using LINQ.
Below is the Updated Code and Expected Output
EXPECTED OUTPUT
March 14
(Start SVG) 2:00 PM - 7:00 PM | Registration
(Body SVG) 5:30 PM - 7:00 PM | Meetup
(End SVG) 7:30 PM - 9:00 PM | Dinner
March 15
(Start SVG) 2:00 PM - 7:00 PM | Registration
(End SVG) 5:30 PM - 7:00 PM | Meetup
Code Structure
Imports System
Imports System.Globalization
Imports System.Data
Imports System.Data.DataSetExtensions
Public Module Module1
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessionIcon = String.Empty
'Dim Sessions = SampleTable().AsEnumerable()
'For Each session In Sessions
' If Sessions.Rows.IndexOf(session) = 0 Then
' sessionIcon = "<svg>Start Icon</svg>"
' ElseIf Sessions.Rows.IndexOf(session) = (Sessions.Rows.Count - 1) Then
' sessionIcon = "<svg>End Icon</svg>"
' Else
' sessionIcon = "<svg>Rest Icon</svg>"
' End If
'Next
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key.SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key.SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key.SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key.SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Console.WriteLine("{0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName)
Next
Next
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
'Add 5 Rows of Data to test
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
End With
Return table
End Function
End Module
vb.net linq
I'm having a little trouble trying to get some data to format properly.
I'm not sure if LINQ is helpful in this case but below is:
Expected output
March 14
2:00 PM - 7:00 PM | Registration
5:30 PM - 7:00 PM | Meetup
7:30 PM - 9:00 PM | Dinner
March 15
2:00 PM - 7:00 PM | Registration
5:30 PM - 7:00 PM | Meetup
Current data output
March 14
2:00 PM - 7:00 PM | Registration
March 14
5:30 PM - 7:00 PM | Meetup
March 14
7:30 PM - 9:00 PM | Dinner
March 15
2:00 PM - 7:00 PM | Registration
March 15
6:00 PM - 7:00 PM | Meetup
Code Structure
Imports System
Imports System.Globalization
Imports System.Data
Imports System.Data.DataSetExtensions
Public Module Module1
Public Sub Main()
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
For Each session In sessions.AsEnumerable()
Dim sessionID = session.Item("SessionID")
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
Console.WriteLine("{0}", sessionDate)
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
'Add 5 Rows of Data to test
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
End With
Return table
End Function
End Module
I've referenced other SO questions such as THIS but I'm not sure if LINQ would make the expected output easier to obtain.
Any suggestions are welcomed. I'm trying to possibly add a nested for Each loop and then add a condition if the sessionDate is the same.
****** UPDATE *******
I really appreciate the feedback and helpful answers. I'm trying to do one more format change to the data. I have to add a specific SVG Icon to all the events items. The SVG icons are specific to the First, Last, Rest of events.
I'm trying to use the LINQ answer provided but I'm not well versed in using it yet but it does seem very easy to read. For the purposes of this example, A String variable can be used as place holders for the first / rest / last.
I've also added my attempt to try to add the icon but I was not using LINQ.
Below is the Updated Code and Expected Output
EXPECTED OUTPUT
March 14
(Start SVG) 2:00 PM - 7:00 PM | Registration
(Body SVG) 5:30 PM - 7:00 PM | Meetup
(End SVG) 7:30 PM - 9:00 PM | Dinner
March 15
(Start SVG) 2:00 PM - 7:00 PM | Registration
(End SVG) 5:30 PM - 7:00 PM | Meetup
Code Structure
Imports System
Imports System.Globalization
Imports System.Data
Imports System.Data.DataSetExtensions
Public Module Module1
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessionIcon = String.Empty
'Dim Sessions = SampleTable().AsEnumerable()
'For Each session In Sessions
' If Sessions.Rows.IndexOf(session) = 0 Then
' sessionIcon = "<svg>Start Icon</svg>"
' ElseIf Sessions.Rows.IndexOf(session) = (Sessions.Rows.Count - 1) Then
' sessionIcon = "<svg>End Icon</svg>"
' Else
' sessionIcon = "<svg>Rest Icon</svg>"
' End If
'Next
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key.SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key.SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key.SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key.SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Console.WriteLine("{0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName)
Next
Next
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
'Add 5 Rows of Data to test
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
End With
Return table
End Function
End Module
vb.net linq
vb.net linq
edited Nov 26 '18 at 17:16
cpt-crunchy
asked Nov 21 '18 at 22:30
cpt-crunchycpt-crunchy
17313
17313
If there are additional questions, it is better to do it before marking as resolved. If it has been settled, there is a high possibility that everyone will not notice the question.
– m mukai
Nov 28 '18 at 0:52
Sorry about that. I didn't need the extra formatting so the question remains resolved.
– cpt-crunchy
Nov 29 '18 at 17:23
add a comment |
If there are additional questions, it is better to do it before marking as resolved. If it has been settled, there is a high possibility that everyone will not notice the question.
– m mukai
Nov 28 '18 at 0:52
Sorry about that. I didn't need the extra formatting so the question remains resolved.
– cpt-crunchy
Nov 29 '18 at 17:23
If there are additional questions, it is better to do it before marking as resolved. If it has been settled, there is a high possibility that everyone will not notice the question.
– m mukai
Nov 28 '18 at 0:52
If there are additional questions, it is better to do it before marking as resolved. If it has been settled, there is a high possibility that everyone will not notice the question.
– m mukai
Nov 28 '18 at 0:52
Sorry about that. I didn't need the extra formatting so the question remains resolved.
– cpt-crunchy
Nov 29 '18 at 17:23
Sorry about that. I didn't need the extra formatting so the question remains resolved.
– cpt-crunchy
Nov 29 '18 at 17:23
add a comment |
4 Answers
4
active
oldest
votes
When using LINQ, like this
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key .SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key .SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key .SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key .SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Console.WriteLine("{0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName)
Next
Next
End Sub
Addition
Basically it is not wrong with your way of thinking.
The position to add that code is slightly different.
Because you want to process each row grouped by date
It must be done in that loop.
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key .SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key .SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key .SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key .SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Dim sessionIcon As String
If r.Equals(g.First) Then
sessionIcon = "<svg>Start Icon</svg>"
ElseIf r.Equals(g.Last) Then
sessionIcon = "<svg>End Icon</svg>"
Else
sessionIcon = "<svg>Body Icon</svg>"
End If
Console.WriteLine("{3} {0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName, sessionIcon)
Next
Next
End Sub
add a comment |
If you are not looking for something fancy, this will probably accomplish the end result:
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim dateList As New List(Of String)
For Each session In sessions.AsEnumerable()
Dim sessionID = session.Item("SessionID")
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
If Not dateList.Contains(sessionDate) Then
Console.WriteLine("{0}", sessionDate)
dateList.Add(sessionDate)
End If
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
I like this answer as well. However, the data would need to be provided in order prior to being looped through as it doesn't account for dates out of order. This is a minor issue as I'm using a Stored Procedure to populate the data and it is just as easy to do a SORT BY Date on the back end.
– cpt-crunchy
Nov 26 '18 at 16:18
add a comment |
I added a complication that allows the events to be entered in any order then sorted into a DataView.
Sub Main()
Dim sessions = SampleTable()
Dim SortedView As New DataView(sessions)
SortedView.Sort = "SessionStartTime"
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim row As DataRowView = SortedView(0)
Dim currentDate As String = #1/1/1950#.ToString("YYYY-MM-dd") 'CDate(row("SessionStartTime")).Date
For Each session As DataRowView In SortedView
If currentDate <> CDate(session.Item("SessionStartTime")).ToString("YYYY-MM-dd") Then
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Console.WriteLine("{0}", sessionDate)
currentDate = CDate(session.Item("SessionStartTime")).ToString("YYYY-MM-dd")
End If
Dim sessionID = session.Item("SessionID")
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
Console.ReadLine()
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
'Add 5 Rows of Data to test
'Notice that the Events do not have to added in order
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
End With
Return table
End Function
add a comment |
Readable LINQ with help of presenter class
Public Class Session
Private ReadOnly _ci As CultureInfo
Public ReadOnly Name As String
Public ReadOnly StartTime As Date
Public ReadOnly EndTime As Date
Public Sub New(row As DataRow, CultureInfo ci)
_ci= ci
Name = row.Field(Of String)("SessionName")
StartTime = row.Field(Of Date)("SessionStartTime")
EndTime = row.Field(Of Date)("SessionEndTime")
End Sub
Public Overrides Function ToString() As String
Return $"{StartTime.ToString("t", ci)} - {EndTime.ToString("t", ci)} | {Name}"
End Function
End Class
' Format rows with the key row
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
var output =
sessions.AsEnumerable().
Select(Function(row) New Session(row, ci)).
GroupBy(Function(session) session.StartTime.Date).
Aggregate(new StringBuilder(),
Function(builder, day)
builder.AppendLine(day.Key.ToString("M", ci))
day.Aggregate(builder, Function(b, session) b.AppendLine(session))
Return builder
End Function).
ToString()
Console.WriteLine(output)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421344%2fformat-data-in-datatable-with-or-without-linq-in-vb-net%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
When using LINQ, like this
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key .SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key .SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key .SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key .SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Console.WriteLine("{0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName)
Next
Next
End Sub
Addition
Basically it is not wrong with your way of thinking.
The position to add that code is slightly different.
Because you want to process each row grouped by date
It must be done in that loop.
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key .SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key .SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key .SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key .SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Dim sessionIcon As String
If r.Equals(g.First) Then
sessionIcon = "<svg>Start Icon</svg>"
ElseIf r.Equals(g.Last) Then
sessionIcon = "<svg>End Icon</svg>"
Else
sessionIcon = "<svg>Body Icon</svg>"
End If
Console.WriteLine("{3} {0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName, sessionIcon)
Next
Next
End Sub
add a comment |
When using LINQ, like this
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key .SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key .SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key .SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key .SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Console.WriteLine("{0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName)
Next
Next
End Sub
Addition
Basically it is not wrong with your way of thinking.
The position to add that code is slightly different.
Because you want to process each row grouped by date
It must be done in that loop.
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key .SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key .SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key .SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key .SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Dim sessionIcon As String
If r.Equals(g.First) Then
sessionIcon = "<svg>Start Icon</svg>"
ElseIf r.Equals(g.Last) Then
sessionIcon = "<svg>End Icon</svg>"
Else
sessionIcon = "<svg>Body Icon</svg>"
End If
Console.WriteLine("{3} {0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName, sessionIcon)
Next
Next
End Sub
add a comment |
When using LINQ, like this
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key .SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key .SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key .SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key .SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Console.WriteLine("{0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName)
Next
Next
End Sub
Addition
Basically it is not wrong with your way of thinking.
The position to add that code is slightly different.
Because you want to process each row grouped by date
It must be done in that loop.
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key .SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key .SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key .SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key .SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Dim sessionIcon As String
If r.Equals(g.First) Then
sessionIcon = "<svg>Start Icon</svg>"
ElseIf r.Equals(g.Last) Then
sessionIcon = "<svg>End Icon</svg>"
Else
sessionIcon = "<svg>Body Icon</svg>"
End If
Console.WriteLine("{3} {0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName, sessionIcon)
Next
Next
End Sub
When using LINQ, like this
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key .SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key .SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key .SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key .SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Console.WriteLine("{0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName)
Next
Next
End Sub
Addition
Basically it is not wrong with your way of thinking.
The position to add that code is slightly different.
Because you want to process each row grouped by date
It must be done in that loop.
Public Sub Main()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim sessions = SampleTable().AsEnumerable().[Select](Function(x) New With {
Key .SessionDate = x.Field(Of Date)("SessionStartTime").ToString("M", ci),
Key .SessionStartTime = x.Field(Of Date)("SessionStartTime").ToString("t", ci),
Key .SessionEndTime = x.Field(Of Date)("SessionEndTime").ToString("t", ci),
Key .SessionName = x.Field(Of String)("SessionName")
})
For Each g In sessions.GroupBy(Function(s) s.SessionDate)
Console.WriteLine("{0}", g.Key)
For Each r In g
Dim sessionIcon As String
If r.Equals(g.First) Then
sessionIcon = "<svg>Start Icon</svg>"
ElseIf r.Equals(g.Last) Then
sessionIcon = "<svg>End Icon</svg>"
Else
sessionIcon = "<svg>Body Icon</svg>"
End If
Console.WriteLine("{3} {0} - {1} | {2}", r.SessionStartTime, r.SessionEndTime, r.SessionName, sessionIcon)
Next
Next
End Sub
edited Nov 27 '18 at 23:45
answered Nov 22 '18 at 6:56


m mukaim mukai
21616
21616
add a comment |
add a comment |
If you are not looking for something fancy, this will probably accomplish the end result:
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim dateList As New List(Of String)
For Each session In sessions.AsEnumerable()
Dim sessionID = session.Item("SessionID")
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
If Not dateList.Contains(sessionDate) Then
Console.WriteLine("{0}", sessionDate)
dateList.Add(sessionDate)
End If
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
I like this answer as well. However, the data would need to be provided in order prior to being looped through as it doesn't account for dates out of order. This is a minor issue as I'm using a Stored Procedure to populate the data and it is just as easy to do a SORT BY Date on the back end.
– cpt-crunchy
Nov 26 '18 at 16:18
add a comment |
If you are not looking for something fancy, this will probably accomplish the end result:
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim dateList As New List(Of String)
For Each session In sessions.AsEnumerable()
Dim sessionID = session.Item("SessionID")
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
If Not dateList.Contains(sessionDate) Then
Console.WriteLine("{0}", sessionDate)
dateList.Add(sessionDate)
End If
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
I like this answer as well. However, the data would need to be provided in order prior to being looped through as it doesn't account for dates out of order. This is a minor issue as I'm using a Stored Procedure to populate the data and it is just as easy to do a SORT BY Date on the back end.
– cpt-crunchy
Nov 26 '18 at 16:18
add a comment |
If you are not looking for something fancy, this will probably accomplish the end result:
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim dateList As New List(Of String)
For Each session In sessions.AsEnumerable()
Dim sessionID = session.Item("SessionID")
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
If Not dateList.Contains(sessionDate) Then
Console.WriteLine("{0}", sessionDate)
dateList.Add(sessionDate)
End If
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
If you are not looking for something fancy, this will probably accomplish the end result:
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim dateList As New List(Of String)
For Each session In sessions.AsEnumerable()
Dim sessionID = session.Item("SessionID")
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
If Not dateList.Contains(sessionDate) Then
Console.WriteLine("{0}", sessionDate)
dateList.Add(sessionDate)
End If
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
answered Nov 21 '18 at 23:20


PopoPopo
2,05742347
2,05742347
I like this answer as well. However, the data would need to be provided in order prior to being looped through as it doesn't account for dates out of order. This is a minor issue as I'm using a Stored Procedure to populate the data and it is just as easy to do a SORT BY Date on the back end.
– cpt-crunchy
Nov 26 '18 at 16:18
add a comment |
I like this answer as well. However, the data would need to be provided in order prior to being looped through as it doesn't account for dates out of order. This is a minor issue as I'm using a Stored Procedure to populate the data and it is just as easy to do a SORT BY Date on the back end.
– cpt-crunchy
Nov 26 '18 at 16:18
I like this answer as well. However, the data would need to be provided in order prior to being looped through as it doesn't account for dates out of order. This is a minor issue as I'm using a Stored Procedure to populate the data and it is just as easy to do a SORT BY Date on the back end.
– cpt-crunchy
Nov 26 '18 at 16:18
I like this answer as well. However, the data would need to be provided in order prior to being looped through as it doesn't account for dates out of order. This is a minor issue as I'm using a Stored Procedure to populate the data and it is just as easy to do a SORT BY Date on the back end.
– cpt-crunchy
Nov 26 '18 at 16:18
add a comment |
I added a complication that allows the events to be entered in any order then sorted into a DataView.
Sub Main()
Dim sessions = SampleTable()
Dim SortedView As New DataView(sessions)
SortedView.Sort = "SessionStartTime"
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim row As DataRowView = SortedView(0)
Dim currentDate As String = #1/1/1950#.ToString("YYYY-MM-dd") 'CDate(row("SessionStartTime")).Date
For Each session As DataRowView In SortedView
If currentDate <> CDate(session.Item("SessionStartTime")).ToString("YYYY-MM-dd") Then
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Console.WriteLine("{0}", sessionDate)
currentDate = CDate(session.Item("SessionStartTime")).ToString("YYYY-MM-dd")
End If
Dim sessionID = session.Item("SessionID")
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
Console.ReadLine()
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
'Add 5 Rows of Data to test
'Notice that the Events do not have to added in order
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
End With
Return table
End Function
add a comment |
I added a complication that allows the events to be entered in any order then sorted into a DataView.
Sub Main()
Dim sessions = SampleTable()
Dim SortedView As New DataView(sessions)
SortedView.Sort = "SessionStartTime"
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim row As DataRowView = SortedView(0)
Dim currentDate As String = #1/1/1950#.ToString("YYYY-MM-dd") 'CDate(row("SessionStartTime")).Date
For Each session As DataRowView In SortedView
If currentDate <> CDate(session.Item("SessionStartTime")).ToString("YYYY-MM-dd") Then
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Console.WriteLine("{0}", sessionDate)
currentDate = CDate(session.Item("SessionStartTime")).ToString("YYYY-MM-dd")
End If
Dim sessionID = session.Item("SessionID")
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
Console.ReadLine()
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
'Add 5 Rows of Data to test
'Notice that the Events do not have to added in order
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
End With
Return table
End Function
add a comment |
I added a complication that allows the events to be entered in any order then sorted into a DataView.
Sub Main()
Dim sessions = SampleTable()
Dim SortedView As New DataView(sessions)
SortedView.Sort = "SessionStartTime"
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim row As DataRowView = SortedView(0)
Dim currentDate As String = #1/1/1950#.ToString("YYYY-MM-dd") 'CDate(row("SessionStartTime")).Date
For Each session As DataRowView In SortedView
If currentDate <> CDate(session.Item("SessionStartTime")).ToString("YYYY-MM-dd") Then
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Console.WriteLine("{0}", sessionDate)
currentDate = CDate(session.Item("SessionStartTime")).ToString("YYYY-MM-dd")
End If
Dim sessionID = session.Item("SessionID")
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
Console.ReadLine()
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
'Add 5 Rows of Data to test
'Notice that the Events do not have to added in order
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
End With
Return table
End Function
I added a complication that allows the events to be entered in any order then sorted into a DataView.
Sub Main()
Dim sessions = SampleTable()
Dim SortedView As New DataView(sessions)
SortedView.Sort = "SessionStartTime"
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
Dim row As DataRowView = SortedView(0)
Dim currentDate As String = #1/1/1950#.ToString("YYYY-MM-dd") 'CDate(row("SessionStartTime")).Date
For Each session As DataRowView In SortedView
If currentDate <> CDate(session.Item("SessionStartTime")).ToString("YYYY-MM-dd") Then
Dim sessionDate = CDate(session.Item("SessionStartTime")).ToString("M", ci)
Console.WriteLine("{0}", sessionDate)
currentDate = CDate(session.Item("SessionStartTime")).ToString("YYYY-MM-dd")
End If
Dim sessionID = session.Item("SessionID")
Dim sessionStart = CDate(session.Item("SessionStartTime")).ToString("t", ci)
Dim sessionEnd = CDate(session.Item("SessionEndTime")).ToString("t", ci)
Dim sessionTitle = CStr(session.Item("SessionName"))
Console.WriteLine("{0} - {1} | {2}", sessionStart, sessionEnd, sessionTitle)
Next
Console.ReadLine()
End Sub
Public Function SampleTable() As DataTable
Dim table As New DataTable
With table
'Add Named Columns
.Columns.Add("SessionID", GetType(Integer))
.Columns.Add("SessionStartTime", GetType(Date))
.Columns.Add("SessionEndTime", GetType(Date))
.Columns.Add("SessionName", GetType(String))
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
'Add 5 Rows of Data to test
'Notice that the Events do not have to added in order
.Rows.Add(2, #03/15/2019 18:00:00#, #03/15/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 14:00:00#, #03/14/2019 19:00:00#, "Registration")
.Rows.Add(1, #03/14/2019 17:30:00#, #03/14/2019 19:00:00#, "Meetup")
.Rows.Add(1, #03/14/2019 19:30:00#, #03/14/2019 21:00:00#, "Dinner")
.Rows.Add(2, #03/15/2019 14:00:00#, #03/15/2019 19:00:00#, "Registration")
End With
Return table
End Function
answered Nov 22 '18 at 3:56


MaryMary
3,4862819
3,4862819
add a comment |
add a comment |
Readable LINQ with help of presenter class
Public Class Session
Private ReadOnly _ci As CultureInfo
Public ReadOnly Name As String
Public ReadOnly StartTime As Date
Public ReadOnly EndTime As Date
Public Sub New(row As DataRow, CultureInfo ci)
_ci= ci
Name = row.Field(Of String)("SessionName")
StartTime = row.Field(Of Date)("SessionStartTime")
EndTime = row.Field(Of Date)("SessionEndTime")
End Sub
Public Overrides Function ToString() As String
Return $"{StartTime.ToString("t", ci)} - {EndTime.ToString("t", ci)} | {Name}"
End Function
End Class
' Format rows with the key row
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
var output =
sessions.AsEnumerable().
Select(Function(row) New Session(row, ci)).
GroupBy(Function(session) session.StartTime.Date).
Aggregate(new StringBuilder(),
Function(builder, day)
builder.AppendLine(day.Key.ToString("M", ci))
day.Aggregate(builder, Function(b, session) b.AppendLine(session))
Return builder
End Function).
ToString()
Console.WriteLine(output)
add a comment |
Readable LINQ with help of presenter class
Public Class Session
Private ReadOnly _ci As CultureInfo
Public ReadOnly Name As String
Public ReadOnly StartTime As Date
Public ReadOnly EndTime As Date
Public Sub New(row As DataRow, CultureInfo ci)
_ci= ci
Name = row.Field(Of String)("SessionName")
StartTime = row.Field(Of Date)("SessionStartTime")
EndTime = row.Field(Of Date)("SessionEndTime")
End Sub
Public Overrides Function ToString() As String
Return $"{StartTime.ToString("t", ci)} - {EndTime.ToString("t", ci)} | {Name}"
End Function
End Class
' Format rows with the key row
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
var output =
sessions.AsEnumerable().
Select(Function(row) New Session(row, ci)).
GroupBy(Function(session) session.StartTime.Date).
Aggregate(new StringBuilder(),
Function(builder, day)
builder.AppendLine(day.Key.ToString("M", ci))
day.Aggregate(builder, Function(b, session) b.AppendLine(session))
Return builder
End Function).
ToString()
Console.WriteLine(output)
add a comment |
Readable LINQ with help of presenter class
Public Class Session
Private ReadOnly _ci As CultureInfo
Public ReadOnly Name As String
Public ReadOnly StartTime As Date
Public ReadOnly EndTime As Date
Public Sub New(row As DataRow, CultureInfo ci)
_ci= ci
Name = row.Field(Of String)("SessionName")
StartTime = row.Field(Of Date)("SessionStartTime")
EndTime = row.Field(Of Date)("SessionEndTime")
End Sub
Public Overrides Function ToString() As String
Return $"{StartTime.ToString("t", ci)} - {EndTime.ToString("t", ci)} | {Name}"
End Function
End Class
' Format rows with the key row
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
var output =
sessions.AsEnumerable().
Select(Function(row) New Session(row, ci)).
GroupBy(Function(session) session.StartTime.Date).
Aggregate(new StringBuilder(),
Function(builder, day)
builder.AppendLine(day.Key.ToString("M", ci))
day.Aggregate(builder, Function(b, session) b.AppendLine(session))
Return builder
End Function).
ToString()
Console.WriteLine(output)
Readable LINQ with help of presenter class
Public Class Session
Private ReadOnly _ci As CultureInfo
Public ReadOnly Name As String
Public ReadOnly StartTime As Date
Public ReadOnly EndTime As Date
Public Sub New(row As DataRow, CultureInfo ci)
_ci= ci
Name = row.Field(Of String)("SessionName")
StartTime = row.Field(Of Date)("SessionStartTime")
EndTime = row.Field(Of Date)("SessionEndTime")
End Sub
Public Overrides Function ToString() As String
Return $"{StartTime.ToString("t", ci)} - {EndTime.ToString("t", ci)} | {Name}"
End Function
End Class
' Format rows with the key row
Dim sessions = SampleTable()
Dim ci = CultureInfo.CreateSpecificCulture("en-us")
var output =
sessions.AsEnumerable().
Select(Function(row) New Session(row, ci)).
GroupBy(Function(session) session.StartTime.Date).
Aggregate(new StringBuilder(),
Function(builder, day)
builder.AppendLine(day.Key.ToString("M", ci))
day.Aggregate(builder, Function(b, session) b.AppendLine(session))
Return builder
End Function).
ToString()
Console.WriteLine(output)
edited Nov 22 '18 at 9:21
answered Nov 22 '18 at 8:51
FabioFabio
19.7k22047
19.7k22047
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421344%2fformat-data-in-datatable-with-or-without-linq-in-vb-net%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Jr0a0JbolLXHQ,5Z4eK07 l,N,DxgRPjKur9MkbFNJ2Zj
If there are additional questions, it is better to do it before marking as resolved. If it has been settled, there is a high possibility that everyone will not notice the question.
– m mukai
Nov 28 '18 at 0:52
Sorry about that. I didn't need the extra formatting so the question remains resolved.
– cpt-crunchy
Nov 29 '18 at 17:23