Applying className/onClick/onChange not working on Custom React Component
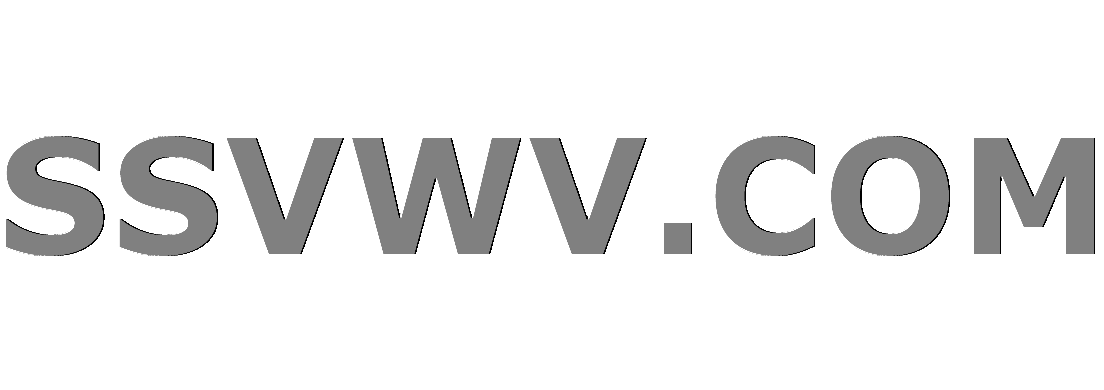
Multi tool use
I'm almost new to react
.
I'm trying to create a simple editing and creating mask.
Here is the code:
import React, { Component } from 'react';
import Company from './Company';
class CompanyList extends Component {
constructor(props) {
super(props);
this.state = {
search: '',
companies: props.companies
};
}
updateSearch(event) {
this.setState({ search: event.target.value.substr(0,20) })
}
addCompany(event) {
event.preventDefault();
let nummer = this.refs.nummer.value;
let bezeichnung = this.refs.bezeichnung.value;
let id = Math.floor((Math.random()*100) + 1);
$.ajax({
type: "POST",
context:this,
dataType: "json",
async: true,
url: "../data/post/json/companies",
data: ({
_token : window.Laravel.csrfToken,
nummer: nummer,
bezeichnung : bezeichnung,
}),
success: function (data) {
id = data.Nummer;
this.setState({
companies: this.state.companies.concat({id, nummer, bezeichnung})
})
this.refs.bezeichnung.value = '';
this.refs.nummer.value = '';
}
});
}
editCompany(event) {
alert('clicked');
event.preventDefault();
this.refs.bezeichnung.value = company.Bezeichnung;
this.refs.nummer.value = company.Nummer;
}
render() {
let filteredCompanies = this.state.companies.filter(
(company) => {
return company.bezeichnung.toLowerCase().indexOf(this.state.search.toLowerCase()) !== -1;
}
);
return (
<div>
<div className="row">
<div className="col-xs-12 col-sm-12 col-md-12 col-lg-12">Suche</div>
<div className="col-xs-12 col-sm-12 col-md-9 col-lg-9">
<div className="form-group">
<input className="form-control" type="text" value={this.state.search} placeholder="Search" onChange={this.updateSearch.bind(this)} />
</div>
</div>
</div>
<form onSubmit={this.addCompany.bind(this)}>
<div className="row">
<div className="col-xs-12 col-sm-12 col-md-12 col-lg-12">Neuen Eintrag erfassen</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<input className="form-control" type="text" ref="nummer" placeholder="Nummer" required />
</div>
</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<input className="form-control" type="text" ref="bezeichnung" placeholder="Firmenname" required />
</div>
</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<button type="submit" className="btn btn-default">Add new company</button>
</div>
</div>
</div>
</form>
<div className="row">
<div className="col-xs-10 col-sm-10 col-md-10 col-lg-10">
<ul>
{
filteredCompanies.map((company)=> {
return <Company company={company} key={company.id} onClick={this.editCompany.bind(this)} />
})
}
</ul>
</div>
</div>
</div>
);
}
}
export default CompanyList
The Company class looks like this:
import React, { Component } from 'react';
const Company = ({company}) =>
<li>
{company.Nummer} {company.Bezeichnung}
</li>
export default Company
My question now is, why is the onclick
event not being fired, when I click on a Company
.
In this part:
<ul>
{
filteredCompanies.map((company)=> {
return <Company company={company} key={company.id} onClick={this.editCompany.bind(this)} className="Company"/>
})
}
</ul>
javascript reactjs events jsx
add a comment |
I'm almost new to react
.
I'm trying to create a simple editing and creating mask.
Here is the code:
import React, { Component } from 'react';
import Company from './Company';
class CompanyList extends Component {
constructor(props) {
super(props);
this.state = {
search: '',
companies: props.companies
};
}
updateSearch(event) {
this.setState({ search: event.target.value.substr(0,20) })
}
addCompany(event) {
event.preventDefault();
let nummer = this.refs.nummer.value;
let bezeichnung = this.refs.bezeichnung.value;
let id = Math.floor((Math.random()*100) + 1);
$.ajax({
type: "POST",
context:this,
dataType: "json",
async: true,
url: "../data/post/json/companies",
data: ({
_token : window.Laravel.csrfToken,
nummer: nummer,
bezeichnung : bezeichnung,
}),
success: function (data) {
id = data.Nummer;
this.setState({
companies: this.state.companies.concat({id, nummer, bezeichnung})
})
this.refs.bezeichnung.value = '';
this.refs.nummer.value = '';
}
});
}
editCompany(event) {
alert('clicked');
event.preventDefault();
this.refs.bezeichnung.value = company.Bezeichnung;
this.refs.nummer.value = company.Nummer;
}
render() {
let filteredCompanies = this.state.companies.filter(
(company) => {
return company.bezeichnung.toLowerCase().indexOf(this.state.search.toLowerCase()) !== -1;
}
);
return (
<div>
<div className="row">
<div className="col-xs-12 col-sm-12 col-md-12 col-lg-12">Suche</div>
<div className="col-xs-12 col-sm-12 col-md-9 col-lg-9">
<div className="form-group">
<input className="form-control" type="text" value={this.state.search} placeholder="Search" onChange={this.updateSearch.bind(this)} />
</div>
</div>
</div>
<form onSubmit={this.addCompany.bind(this)}>
<div className="row">
<div className="col-xs-12 col-sm-12 col-md-12 col-lg-12">Neuen Eintrag erfassen</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<input className="form-control" type="text" ref="nummer" placeholder="Nummer" required />
</div>
</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<input className="form-control" type="text" ref="bezeichnung" placeholder="Firmenname" required />
</div>
</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<button type="submit" className="btn btn-default">Add new company</button>
</div>
</div>
</div>
</form>
<div className="row">
<div className="col-xs-10 col-sm-10 col-md-10 col-lg-10">
<ul>
{
filteredCompanies.map((company)=> {
return <Company company={company} key={company.id} onClick={this.editCompany.bind(this)} />
})
}
</ul>
</div>
</div>
</div>
);
}
}
export default CompanyList
The Company class looks like this:
import React, { Component } from 'react';
const Company = ({company}) =>
<li>
{company.Nummer} {company.Bezeichnung}
</li>
export default Company
My question now is, why is the onclick
event not being fired, when I click on a Company
.
In this part:
<ul>
{
filteredCompanies.map((company)=> {
return <Company company={company} key={company.id} onClick={this.editCompany.bind(this)} className="Company"/>
})
}
</ul>
javascript reactjs events jsx
add a comment |
I'm almost new to react
.
I'm trying to create a simple editing and creating mask.
Here is the code:
import React, { Component } from 'react';
import Company from './Company';
class CompanyList extends Component {
constructor(props) {
super(props);
this.state = {
search: '',
companies: props.companies
};
}
updateSearch(event) {
this.setState({ search: event.target.value.substr(0,20) })
}
addCompany(event) {
event.preventDefault();
let nummer = this.refs.nummer.value;
let bezeichnung = this.refs.bezeichnung.value;
let id = Math.floor((Math.random()*100) + 1);
$.ajax({
type: "POST",
context:this,
dataType: "json",
async: true,
url: "../data/post/json/companies",
data: ({
_token : window.Laravel.csrfToken,
nummer: nummer,
bezeichnung : bezeichnung,
}),
success: function (data) {
id = data.Nummer;
this.setState({
companies: this.state.companies.concat({id, nummer, bezeichnung})
})
this.refs.bezeichnung.value = '';
this.refs.nummer.value = '';
}
});
}
editCompany(event) {
alert('clicked');
event.preventDefault();
this.refs.bezeichnung.value = company.Bezeichnung;
this.refs.nummer.value = company.Nummer;
}
render() {
let filteredCompanies = this.state.companies.filter(
(company) => {
return company.bezeichnung.toLowerCase().indexOf(this.state.search.toLowerCase()) !== -1;
}
);
return (
<div>
<div className="row">
<div className="col-xs-12 col-sm-12 col-md-12 col-lg-12">Suche</div>
<div className="col-xs-12 col-sm-12 col-md-9 col-lg-9">
<div className="form-group">
<input className="form-control" type="text" value={this.state.search} placeholder="Search" onChange={this.updateSearch.bind(this)} />
</div>
</div>
</div>
<form onSubmit={this.addCompany.bind(this)}>
<div className="row">
<div className="col-xs-12 col-sm-12 col-md-12 col-lg-12">Neuen Eintrag erfassen</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<input className="form-control" type="text" ref="nummer" placeholder="Nummer" required />
</div>
</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<input className="form-control" type="text" ref="bezeichnung" placeholder="Firmenname" required />
</div>
</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<button type="submit" className="btn btn-default">Add new company</button>
</div>
</div>
</div>
</form>
<div className="row">
<div className="col-xs-10 col-sm-10 col-md-10 col-lg-10">
<ul>
{
filteredCompanies.map((company)=> {
return <Company company={company} key={company.id} onClick={this.editCompany.bind(this)} />
})
}
</ul>
</div>
</div>
</div>
);
}
}
export default CompanyList
The Company class looks like this:
import React, { Component } from 'react';
const Company = ({company}) =>
<li>
{company.Nummer} {company.Bezeichnung}
</li>
export default Company
My question now is, why is the onclick
event not being fired, when I click on a Company
.
In this part:
<ul>
{
filteredCompanies.map((company)=> {
return <Company company={company} key={company.id} onClick={this.editCompany.bind(this)} className="Company"/>
})
}
</ul>
javascript reactjs events jsx
I'm almost new to react
.
I'm trying to create a simple editing and creating mask.
Here is the code:
import React, { Component } from 'react';
import Company from './Company';
class CompanyList extends Component {
constructor(props) {
super(props);
this.state = {
search: '',
companies: props.companies
};
}
updateSearch(event) {
this.setState({ search: event.target.value.substr(0,20) })
}
addCompany(event) {
event.preventDefault();
let nummer = this.refs.nummer.value;
let bezeichnung = this.refs.bezeichnung.value;
let id = Math.floor((Math.random()*100) + 1);
$.ajax({
type: "POST",
context:this,
dataType: "json",
async: true,
url: "../data/post/json/companies",
data: ({
_token : window.Laravel.csrfToken,
nummer: nummer,
bezeichnung : bezeichnung,
}),
success: function (data) {
id = data.Nummer;
this.setState({
companies: this.state.companies.concat({id, nummer, bezeichnung})
})
this.refs.bezeichnung.value = '';
this.refs.nummer.value = '';
}
});
}
editCompany(event) {
alert('clicked');
event.preventDefault();
this.refs.bezeichnung.value = company.Bezeichnung;
this.refs.nummer.value = company.Nummer;
}
render() {
let filteredCompanies = this.state.companies.filter(
(company) => {
return company.bezeichnung.toLowerCase().indexOf(this.state.search.toLowerCase()) !== -1;
}
);
return (
<div>
<div className="row">
<div className="col-xs-12 col-sm-12 col-md-12 col-lg-12">Suche</div>
<div className="col-xs-12 col-sm-12 col-md-9 col-lg-9">
<div className="form-group">
<input className="form-control" type="text" value={this.state.search} placeholder="Search" onChange={this.updateSearch.bind(this)} />
</div>
</div>
</div>
<form onSubmit={this.addCompany.bind(this)}>
<div className="row">
<div className="col-xs-12 col-sm-12 col-md-12 col-lg-12">Neuen Eintrag erfassen</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<input className="form-control" type="text" ref="nummer" placeholder="Nummer" required />
</div>
</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<input className="form-control" type="text" ref="bezeichnung" placeholder="Firmenname" required />
</div>
</div>
<div className="col-xs-12 col-sm-12 col-md-3 col-lg-3">
<div className="form-group">
<button type="submit" className="btn btn-default">Add new company</button>
</div>
</div>
</div>
</form>
<div className="row">
<div className="col-xs-10 col-sm-10 col-md-10 col-lg-10">
<ul>
{
filteredCompanies.map((company)=> {
return <Company company={company} key={company.id} onClick={this.editCompany.bind(this)} />
})
}
</ul>
</div>
</div>
</div>
);
}
}
export default CompanyList
The Company class looks like this:
import React, { Component } from 'react';
const Company = ({company}) =>
<li>
{company.Nummer} {company.Bezeichnung}
</li>
export default Company
My question now is, why is the onclick
event not being fired, when I click on a Company
.
In this part:
<ul>
{
filteredCompanies.map((company)=> {
return <Company company={company} key={company.id} onClick={this.editCompany.bind(this)} className="Company"/>
})
}
</ul>
javascript reactjs events jsx
javascript reactjs events jsx
edited Nov 14 '17 at 8:27


Shubham Khatri
94.3k15119160
94.3k15119160
asked May 19 '17 at 12:29


dns_nxdns_nx
1,55811736
1,55811736
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The reason is pretty simple, when you onClick like
<Company company={company} key={company.id} onClick={this.editCompany.bind(this)} />
its not an event that is set on the component, rather a prop that is being passed to the Company
component and can be accessed like props.company
in the Company component,
what you need to do is to specify the onClick
event and className
in the Company component like
import React, { Component } from 'react';
const Company = ({company, onClick, className}) => (
<li onClick={onClick} className={className}>
{company.Nummer} {company.Bezeichnung}
</li>
)
export default Company
The function passed on as prop to the Company
component can be passed on by any name like
<Company company={company} key={company.id} editCompany={this.editCompany.bind(this)} className="Company"/>
and used like
import React, { Component } from 'react';
const Company = ({company, editCompany}) => (
<li onClick={editCompany}>
{company.Nummer} {company.Bezeichnung}
</li>
)
Thanks for your answer. But do I need to specify also the event itself then inCompany
or can I specify it also inCompanyList
to use the controls for input? I mean the methodeditCompany
.
– dns_nx
May 19 '17 at 12:44
event will be specified at the component level, so in the Company component itself, the function that you nee to call on that event can be passed on by any name
– Shubham Khatri
May 19 '17 at 12:46
add a comment |
you have to bind the event on you child component :
import React, { Component } from 'react';
const Company = ({ company, onClick }) =>
<li onClick={onClick}>
{company.Nummer} {company.Bezeichnung}
</li>
export default Company
or
const Company = ({ company, ...props }) =>
<li {...props}>
{company.Nummer} {company.Bezeichnung}
</li>
if you want that all props passed to your Compagny component (exept compagny) goes to your li tag. see ES6 spread operator and rest.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f44070162%2fapplying-classname-onclick-onchange-not-working-on-custom-react-component%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The reason is pretty simple, when you onClick like
<Company company={company} key={company.id} onClick={this.editCompany.bind(this)} />
its not an event that is set on the component, rather a prop that is being passed to the Company
component and can be accessed like props.company
in the Company component,
what you need to do is to specify the onClick
event and className
in the Company component like
import React, { Component } from 'react';
const Company = ({company, onClick, className}) => (
<li onClick={onClick} className={className}>
{company.Nummer} {company.Bezeichnung}
</li>
)
export default Company
The function passed on as prop to the Company
component can be passed on by any name like
<Company company={company} key={company.id} editCompany={this.editCompany.bind(this)} className="Company"/>
and used like
import React, { Component } from 'react';
const Company = ({company, editCompany}) => (
<li onClick={editCompany}>
{company.Nummer} {company.Bezeichnung}
</li>
)
Thanks for your answer. But do I need to specify also the event itself then inCompany
or can I specify it also inCompanyList
to use the controls for input? I mean the methodeditCompany
.
– dns_nx
May 19 '17 at 12:44
event will be specified at the component level, so in the Company component itself, the function that you nee to call on that event can be passed on by any name
– Shubham Khatri
May 19 '17 at 12:46
add a comment |
The reason is pretty simple, when you onClick like
<Company company={company} key={company.id} onClick={this.editCompany.bind(this)} />
its not an event that is set on the component, rather a prop that is being passed to the Company
component and can be accessed like props.company
in the Company component,
what you need to do is to specify the onClick
event and className
in the Company component like
import React, { Component } from 'react';
const Company = ({company, onClick, className}) => (
<li onClick={onClick} className={className}>
{company.Nummer} {company.Bezeichnung}
</li>
)
export default Company
The function passed on as prop to the Company
component can be passed on by any name like
<Company company={company} key={company.id} editCompany={this.editCompany.bind(this)} className="Company"/>
and used like
import React, { Component } from 'react';
const Company = ({company, editCompany}) => (
<li onClick={editCompany}>
{company.Nummer} {company.Bezeichnung}
</li>
)
Thanks for your answer. But do I need to specify also the event itself then inCompany
or can I specify it also inCompanyList
to use the controls for input? I mean the methodeditCompany
.
– dns_nx
May 19 '17 at 12:44
event will be specified at the component level, so in the Company component itself, the function that you nee to call on that event can be passed on by any name
– Shubham Khatri
May 19 '17 at 12:46
add a comment |
The reason is pretty simple, when you onClick like
<Company company={company} key={company.id} onClick={this.editCompany.bind(this)} />
its not an event that is set on the component, rather a prop that is being passed to the Company
component and can be accessed like props.company
in the Company component,
what you need to do is to specify the onClick
event and className
in the Company component like
import React, { Component } from 'react';
const Company = ({company, onClick, className}) => (
<li onClick={onClick} className={className}>
{company.Nummer} {company.Bezeichnung}
</li>
)
export default Company
The function passed on as prop to the Company
component can be passed on by any name like
<Company company={company} key={company.id} editCompany={this.editCompany.bind(this)} className="Company"/>
and used like
import React, { Component } from 'react';
const Company = ({company, editCompany}) => (
<li onClick={editCompany}>
{company.Nummer} {company.Bezeichnung}
</li>
)
The reason is pretty simple, when you onClick like
<Company company={company} key={company.id} onClick={this.editCompany.bind(this)} />
its not an event that is set on the component, rather a prop that is being passed to the Company
component and can be accessed like props.company
in the Company component,
what you need to do is to specify the onClick
event and className
in the Company component like
import React, { Component } from 'react';
const Company = ({company, onClick, className}) => (
<li onClick={onClick} className={className}>
{company.Nummer} {company.Bezeichnung}
</li>
)
export default Company
The function passed on as prop to the Company
component can be passed on by any name like
<Company company={company} key={company.id} editCompany={this.editCompany.bind(this)} className="Company"/>
and used like
import React, { Component } from 'react';
const Company = ({company, editCompany}) => (
<li onClick={editCompany}>
{company.Nummer} {company.Bezeichnung}
</li>
)
edited Sep 4 '17 at 12:33
answered May 19 '17 at 12:39


Shubham KhatriShubham Khatri
94.3k15119160
94.3k15119160
Thanks for your answer. But do I need to specify also the event itself then inCompany
or can I specify it also inCompanyList
to use the controls for input? I mean the methodeditCompany
.
– dns_nx
May 19 '17 at 12:44
event will be specified at the component level, so in the Company component itself, the function that you nee to call on that event can be passed on by any name
– Shubham Khatri
May 19 '17 at 12:46
add a comment |
Thanks for your answer. But do I need to specify also the event itself then inCompany
or can I specify it also inCompanyList
to use the controls for input? I mean the methodeditCompany
.
– dns_nx
May 19 '17 at 12:44
event will be specified at the component level, so in the Company component itself, the function that you nee to call on that event can be passed on by any name
– Shubham Khatri
May 19 '17 at 12:46
Thanks for your answer. But do I need to specify also the event itself then in
Company
or can I specify it also in CompanyList
to use the controls for input? I mean the method editCompany
.– dns_nx
May 19 '17 at 12:44
Thanks for your answer. But do I need to specify also the event itself then in
Company
or can I specify it also in CompanyList
to use the controls for input? I mean the method editCompany
.– dns_nx
May 19 '17 at 12:44
event will be specified at the component level, so in the Company component itself, the function that you nee to call on that event can be passed on by any name
– Shubham Khatri
May 19 '17 at 12:46
event will be specified at the component level, so in the Company component itself, the function that you nee to call on that event can be passed on by any name
– Shubham Khatri
May 19 '17 at 12:46
add a comment |
you have to bind the event on you child component :
import React, { Component } from 'react';
const Company = ({ company, onClick }) =>
<li onClick={onClick}>
{company.Nummer} {company.Bezeichnung}
</li>
export default Company
or
const Company = ({ company, ...props }) =>
<li {...props}>
{company.Nummer} {company.Bezeichnung}
</li>
if you want that all props passed to your Compagny component (exept compagny) goes to your li tag. see ES6 spread operator and rest.
add a comment |
you have to bind the event on you child component :
import React, { Component } from 'react';
const Company = ({ company, onClick }) =>
<li onClick={onClick}>
{company.Nummer} {company.Bezeichnung}
</li>
export default Company
or
const Company = ({ company, ...props }) =>
<li {...props}>
{company.Nummer} {company.Bezeichnung}
</li>
if you want that all props passed to your Compagny component (exept compagny) goes to your li tag. see ES6 spread operator and rest.
add a comment |
you have to bind the event on you child component :
import React, { Component } from 'react';
const Company = ({ company, onClick }) =>
<li onClick={onClick}>
{company.Nummer} {company.Bezeichnung}
</li>
export default Company
or
const Company = ({ company, ...props }) =>
<li {...props}>
{company.Nummer} {company.Bezeichnung}
</li>
if you want that all props passed to your Compagny component (exept compagny) goes to your li tag. see ES6 spread operator and rest.
you have to bind the event on you child component :
import React, { Component } from 'react';
const Company = ({ company, onClick }) =>
<li onClick={onClick}>
{company.Nummer} {company.Bezeichnung}
</li>
export default Company
or
const Company = ({ company, ...props }) =>
<li {...props}>
{company.Nummer} {company.Bezeichnung}
</li>
if you want that all props passed to your Compagny component (exept compagny) goes to your li tag. see ES6 spread operator and rest.
answered May 19 '17 at 12:41
gouroujogouroujo
40136
40136
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f44070162%2fapplying-classname-onclick-onchange-not-working-on-custom-react-component%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Kw,I6LX9W7hNf3Y2fK,vQrU8qux7TcDIX hXb1K70 ZK5IwAgf1bm My0 ROyqlHcSrT7WZqvrR Tm3kpNbGfUk,w cx