How to change Style of Lines using PIXI.js Graphics?
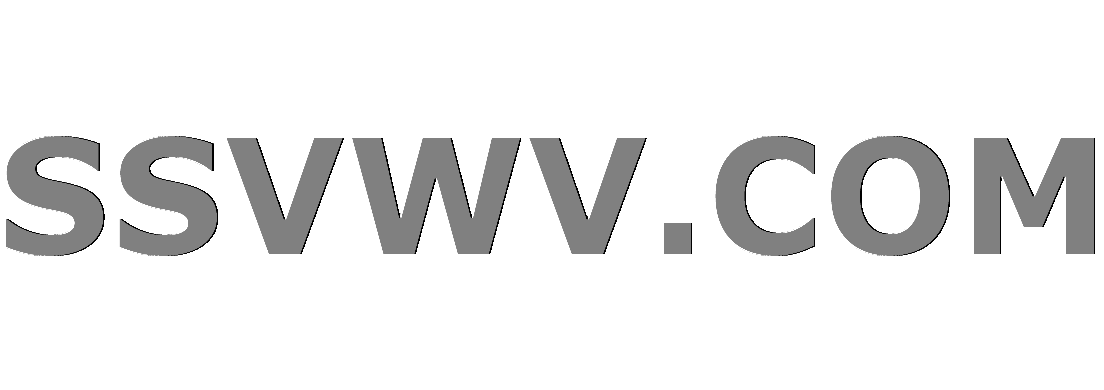
Multi tool use
I am trying to change alpha and color when mouseover or mousedown, but the display is not changing.
When I used the Text class, it worked. I changed the style from normal to bold as follows:
class Leaf extends PIXI.Text{
...
this.interactive = true;
this.on('mouseover', function () {
this.style.fontWeight = 'bold';
});
}
Then I tried to change the color of lines/links/edges of my view, but it is not working:
class TreeEdge extends Graphics{
constructor(d){
super();
this.data = d;
let p0 = project(d.source.x, d.source.y);
let p1 = project(d.target.x, d.target.y);
this.lineStyle(1, d.target.color, 1);
this.moveTo(p0[0], p0[1]);
this.lineTo(p1[0], p1[1]);
this.endFill();
this.hitArea = this.getBounds();
this.interactive = true;
this.on('mouseover', function () {
this.alpha = 1;
});
}
}
I tried this.lineAlpha
, this.alpha
, this.GraphicsData[0].lineAlpha
... this.clear()
and this.dirty++
. Nothing changes. I tried to change color as well, with this.color
and this.lineStyle(1, node.color, 1);
.
It seems I need to update the render or something.
What is the best approach to update graphics elements with user interaction?
I am using pixi.js - v4.7.3
Initiating my app like so:
var app = new PIXI.Application(width, height, {
backgroundColor: 0xffffff,
antialias: true,
transparent: false,
resolution: 1
});
javascript graphics pixi.js
add a comment |
I am trying to change alpha and color when mouseover or mousedown, but the display is not changing.
When I used the Text class, it worked. I changed the style from normal to bold as follows:
class Leaf extends PIXI.Text{
...
this.interactive = true;
this.on('mouseover', function () {
this.style.fontWeight = 'bold';
});
}
Then I tried to change the color of lines/links/edges of my view, but it is not working:
class TreeEdge extends Graphics{
constructor(d){
super();
this.data = d;
let p0 = project(d.source.x, d.source.y);
let p1 = project(d.target.x, d.target.y);
this.lineStyle(1, d.target.color, 1);
this.moveTo(p0[0], p0[1]);
this.lineTo(p1[0], p1[1]);
this.endFill();
this.hitArea = this.getBounds();
this.interactive = true;
this.on('mouseover', function () {
this.alpha = 1;
});
}
}
I tried this.lineAlpha
, this.alpha
, this.GraphicsData[0].lineAlpha
... this.clear()
and this.dirty++
. Nothing changes. I tried to change color as well, with this.color
and this.lineStyle(1, node.color, 1);
.
It seems I need to update the render or something.
What is the best approach to update graphics elements with user interaction?
I am using pixi.js - v4.7.3
Initiating my app like so:
var app = new PIXI.Application(width, height, {
backgroundColor: 0xffffff,
antialias: true,
transparent: false,
resolution: 1
});
javascript graphics pixi.js
add a comment |
I am trying to change alpha and color when mouseover or mousedown, but the display is not changing.
When I used the Text class, it worked. I changed the style from normal to bold as follows:
class Leaf extends PIXI.Text{
...
this.interactive = true;
this.on('mouseover', function () {
this.style.fontWeight = 'bold';
});
}
Then I tried to change the color of lines/links/edges of my view, but it is not working:
class TreeEdge extends Graphics{
constructor(d){
super();
this.data = d;
let p0 = project(d.source.x, d.source.y);
let p1 = project(d.target.x, d.target.y);
this.lineStyle(1, d.target.color, 1);
this.moveTo(p0[0], p0[1]);
this.lineTo(p1[0], p1[1]);
this.endFill();
this.hitArea = this.getBounds();
this.interactive = true;
this.on('mouseover', function () {
this.alpha = 1;
});
}
}
I tried this.lineAlpha
, this.alpha
, this.GraphicsData[0].lineAlpha
... this.clear()
and this.dirty++
. Nothing changes. I tried to change color as well, with this.color
and this.lineStyle(1, node.color, 1);
.
It seems I need to update the render or something.
What is the best approach to update graphics elements with user interaction?
I am using pixi.js - v4.7.3
Initiating my app like so:
var app = new PIXI.Application(width, height, {
backgroundColor: 0xffffff,
antialias: true,
transparent: false,
resolution: 1
});
javascript graphics pixi.js
I am trying to change alpha and color when mouseover or mousedown, but the display is not changing.
When I used the Text class, it worked. I changed the style from normal to bold as follows:
class Leaf extends PIXI.Text{
...
this.interactive = true;
this.on('mouseover', function () {
this.style.fontWeight = 'bold';
});
}
Then I tried to change the color of lines/links/edges of my view, but it is not working:
class TreeEdge extends Graphics{
constructor(d){
super();
this.data = d;
let p0 = project(d.source.x, d.source.y);
let p1 = project(d.target.x, d.target.y);
this.lineStyle(1, d.target.color, 1);
this.moveTo(p0[0], p0[1]);
this.lineTo(p1[0], p1[1]);
this.endFill();
this.hitArea = this.getBounds();
this.interactive = true;
this.on('mouseover', function () {
this.alpha = 1;
});
}
}
I tried this.lineAlpha
, this.alpha
, this.GraphicsData[0].lineAlpha
... this.clear()
and this.dirty++
. Nothing changes. I tried to change color as well, with this.color
and this.lineStyle(1, node.color, 1);
.
It seems I need to update the render or something.
What is the best approach to update graphics elements with user interaction?
I am using pixi.js - v4.7.3
Initiating my app like so:
var app = new PIXI.Application(width, height, {
backgroundColor: 0xffffff,
antialias: true,
transparent: false,
resolution: 1
});
javascript graphics pixi.js
javascript graphics pixi.js
asked May 7 '18 at 19:22


HenryHenry
124111
124111
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
In the latest PixiJS version (4.x.x), you need to use these flags:
this.dirty++;
this.clearDirty++;
You can implement the line update code as a function or add it to the Graphics object prototype as follows:
PIXI.Graphics.prototype.updateLineStyle = function(lineWidth, color, alpha){
var len = this.graphicsData.length;
for (var i = 0; i < len; i++) {
var data = this.graphicsData[i];
data.lineWidth = lineWidth;
data.lineColor = color;
data.alpha = alpha;
this.dirty++;
this.clearDirty++;
}
}
Then use it like this:
var line = new PIXI.Graphics();
line
.lineStyle(...)
.moveTo(...)
.lineTo(...);
line.hitArea = line.getBounds();
line.interactive = true;
line.mouseover = function(){
this.updateLineStyle(5, 0xff0000, 1);
}
Link to jsfiddle : http://jsfiddle.net/anuragsr/4170xkwu/1/
Source : http://www.html5gamedevs.com/topic/9374-change-the-style-of-line-that-is-already-drawn/?tab=comments#comment-55611
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f50221078%2fhow-to-change-style-of-lines-using-pixi-js-graphics%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In the latest PixiJS version (4.x.x), you need to use these flags:
this.dirty++;
this.clearDirty++;
You can implement the line update code as a function or add it to the Graphics object prototype as follows:
PIXI.Graphics.prototype.updateLineStyle = function(lineWidth, color, alpha){
var len = this.graphicsData.length;
for (var i = 0; i < len; i++) {
var data = this.graphicsData[i];
data.lineWidth = lineWidth;
data.lineColor = color;
data.alpha = alpha;
this.dirty++;
this.clearDirty++;
}
}
Then use it like this:
var line = new PIXI.Graphics();
line
.lineStyle(...)
.moveTo(...)
.lineTo(...);
line.hitArea = line.getBounds();
line.interactive = true;
line.mouseover = function(){
this.updateLineStyle(5, 0xff0000, 1);
}
Link to jsfiddle : http://jsfiddle.net/anuragsr/4170xkwu/1/
Source : http://www.html5gamedevs.com/topic/9374-change-the-style-of-line-that-is-already-drawn/?tab=comments#comment-55611
add a comment |
In the latest PixiJS version (4.x.x), you need to use these flags:
this.dirty++;
this.clearDirty++;
You can implement the line update code as a function or add it to the Graphics object prototype as follows:
PIXI.Graphics.prototype.updateLineStyle = function(lineWidth, color, alpha){
var len = this.graphicsData.length;
for (var i = 0; i < len; i++) {
var data = this.graphicsData[i];
data.lineWidth = lineWidth;
data.lineColor = color;
data.alpha = alpha;
this.dirty++;
this.clearDirty++;
}
}
Then use it like this:
var line = new PIXI.Graphics();
line
.lineStyle(...)
.moveTo(...)
.lineTo(...);
line.hitArea = line.getBounds();
line.interactive = true;
line.mouseover = function(){
this.updateLineStyle(5, 0xff0000, 1);
}
Link to jsfiddle : http://jsfiddle.net/anuragsr/4170xkwu/1/
Source : http://www.html5gamedevs.com/topic/9374-change-the-style-of-line-that-is-already-drawn/?tab=comments#comment-55611
add a comment |
In the latest PixiJS version (4.x.x), you need to use these flags:
this.dirty++;
this.clearDirty++;
You can implement the line update code as a function or add it to the Graphics object prototype as follows:
PIXI.Graphics.prototype.updateLineStyle = function(lineWidth, color, alpha){
var len = this.graphicsData.length;
for (var i = 0; i < len; i++) {
var data = this.graphicsData[i];
data.lineWidth = lineWidth;
data.lineColor = color;
data.alpha = alpha;
this.dirty++;
this.clearDirty++;
}
}
Then use it like this:
var line = new PIXI.Graphics();
line
.lineStyle(...)
.moveTo(...)
.lineTo(...);
line.hitArea = line.getBounds();
line.interactive = true;
line.mouseover = function(){
this.updateLineStyle(5, 0xff0000, 1);
}
Link to jsfiddle : http://jsfiddle.net/anuragsr/4170xkwu/1/
Source : http://www.html5gamedevs.com/topic/9374-change-the-style-of-line-that-is-already-drawn/?tab=comments#comment-55611
In the latest PixiJS version (4.x.x), you need to use these flags:
this.dirty++;
this.clearDirty++;
You can implement the line update code as a function or add it to the Graphics object prototype as follows:
PIXI.Graphics.prototype.updateLineStyle = function(lineWidth, color, alpha){
var len = this.graphicsData.length;
for (var i = 0; i < len; i++) {
var data = this.graphicsData[i];
data.lineWidth = lineWidth;
data.lineColor = color;
data.alpha = alpha;
this.dirty++;
this.clearDirty++;
}
}
Then use it like this:
var line = new PIXI.Graphics();
line
.lineStyle(...)
.moveTo(...)
.lineTo(...);
line.hitArea = line.getBounds();
line.interactive = true;
line.mouseover = function(){
this.updateLineStyle(5, 0xff0000, 1);
}
Link to jsfiddle : http://jsfiddle.net/anuragsr/4170xkwu/1/
Source : http://www.html5gamedevs.com/topic/9374-change-the-style-of-line-that-is-already-drawn/?tab=comments#comment-55611
edited Nov 23 '18 at 8:36
answered Nov 22 '18 at 15:36
Anurag SrivastavaAnurag Srivastava
2,13311019
2,13311019
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f50221078%2fhow-to-change-style-of-lines-using-pixi-js-graphics%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Hl0g6of3v gYF3cYGQ1Jkncw2SaIXGKwWIhFx wqvH,3uk,VPvRU1RiRDTrt,BHgCJe MSF