How to use 'unknown' without instanceof in a meaningful way?
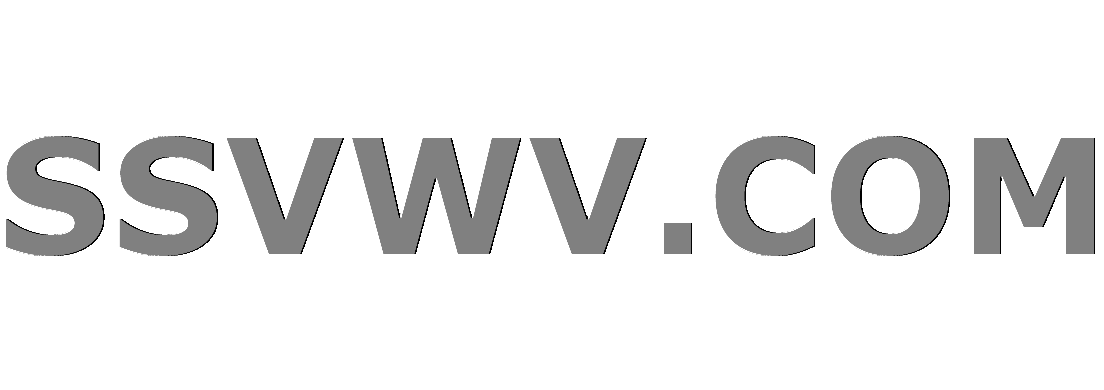
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
Since TypeScript 3.0 the new top type unknown is available as a type-safe counterpart of any.
I really love this type, as it makes the code even more robust.
My main problem with this is, that TypeScript (obviously) does not provide an instanceof
-feature at runtime for interfaces. If it would (be possible), this code would work quite well:
class Test {
doSomething(value: unknown) {
if(value instanceof MySuperInterface) {
return value.superFunction();
}
return false;
}
}
My question
How do I use this type in a meaningful way, without the need of checking each property of my interface?
typescript
add a comment |
Since TypeScript 3.0 the new top type unknown is available as a type-safe counterpart of any.
I really love this type, as it makes the code even more robust.
My main problem with this is, that TypeScript (obviously) does not provide an instanceof
-feature at runtime for interfaces. If it would (be possible), this code would work quite well:
class Test {
doSomething(value: unknown) {
if(value instanceof MySuperInterface) {
return value.superFunction();
}
return false;
}
}
My question
How do I use this type in a meaningful way, without the need of checking each property of my interface?
typescript
1
In conjunction with discriminated unions maybe?
– Fenton
Nov 23 '18 at 14:17
Pick a couple of properties that are significant and use custom type guard to narrowunknown
to your specific interface type ?
– Titian Cernicova-Dragomir
Nov 23 '18 at 14:19
add a comment |
Since TypeScript 3.0 the new top type unknown is available as a type-safe counterpart of any.
I really love this type, as it makes the code even more robust.
My main problem with this is, that TypeScript (obviously) does not provide an instanceof
-feature at runtime for interfaces. If it would (be possible), this code would work quite well:
class Test {
doSomething(value: unknown) {
if(value instanceof MySuperInterface) {
return value.superFunction();
}
return false;
}
}
My question
How do I use this type in a meaningful way, without the need of checking each property of my interface?
typescript
Since TypeScript 3.0 the new top type unknown is available as a type-safe counterpart of any.
I really love this type, as it makes the code even more robust.
My main problem with this is, that TypeScript (obviously) does not provide an instanceof
-feature at runtime for interfaces. If it would (be possible), this code would work quite well:
class Test {
doSomething(value: unknown) {
if(value instanceof MySuperInterface) {
return value.superFunction();
}
return false;
}
}
My question
How do I use this type in a meaningful way, without the need of checking each property of my interface?
typescript
typescript
asked Nov 23 '18 at 14:10


scipperscipper
1,4631424
1,4631424
1
In conjunction with discriminated unions maybe?
– Fenton
Nov 23 '18 at 14:17
Pick a couple of properties that are significant and use custom type guard to narrowunknown
to your specific interface type ?
– Titian Cernicova-Dragomir
Nov 23 '18 at 14:19
add a comment |
1
In conjunction with discriminated unions maybe?
– Fenton
Nov 23 '18 at 14:17
Pick a couple of properties that are significant and use custom type guard to narrowunknown
to your specific interface type ?
– Titian Cernicova-Dragomir
Nov 23 '18 at 14:19
1
1
In conjunction with discriminated unions maybe?
– Fenton
Nov 23 '18 at 14:17
In conjunction with discriminated unions maybe?
– Fenton
Nov 23 '18 at 14:17
Pick a couple of properties that are significant and use custom type guard to narrow
unknown
to your specific interface type ?– Titian Cernicova-Dragomir
Nov 23 '18 at 14:19
Pick a couple of properties that are significant and use custom type guard to narrow
unknown
to your specific interface type ?– Titian Cernicova-Dragomir
Nov 23 '18 at 14:19
add a comment |
1 Answer
1
active
oldest
votes
Javascipt does not check for interface type at runtime.
Typescript: instanceof check on interface
But, you can use unknown type with classes as shown below:
interface MySuperInterface {
superfunction(): string;
}
class MySuperImplementation implements MySuperInterface {
superfunction(): string {
return "Hello from super function";
}
}
class Greeter {
greeting: string;
constructor(message: string) {
this.greeting = message;
}
greet() {
return "Hello, " + this.greeting;
}
testSuperFunction(value: unknown) {
if (value instanceof MySuperImplementation) {
return value.superfunction();
}
else {
return "Error";
}
}
}
let greeter = new Greeter("world");
const sfunc = new MySuperImplementation();
console.log(greeter.testSuperFunction(sfunc));
https://www.typescriptlang.org/play/index.html#src=interface%20MySuperInterface%20%7B%0D%0A%20%20%20%20superfunction()%3A%20string%3B%0D%0A%7D%0D%0A%0D%0Aclass%20MySuperImplementation%20implements%20MySuperInterface%20%7B%0D%0A%20%20%20%20superfunction()%3A%20string%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20%22Hello%20from%20super%20function%22%3B%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Aclass%20Greeter%20%7B%0D%0A%20%20%20%20greeting%3A%20string%3B%0D%0A%0D%0A%20%20%20%20constructor(message%3A%20string)%20%7B%0D%0A%20%20%20%20%20%20%20%20this.greeting%20%3D%20message%3B%0D%0A%20%20%20%20%7D%0D%0A%0D%0A%20%20%20%20greet()%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20%22Hello%2C%20%22%20%2B%20this.greeting%3B%0D%0A%20%20%20%20%7D%0D%0A%0D%0A%20%20%20%20testSuperFunction(value%3A%20unknown)%20%7B%0D%0A%20%20%20%20%20%20%20%20if%20(value%20instanceof%20MySuperImplementation)%20%7B%0D%0A%20%20%20%20%20%20%20%20%20%20%20%20return%20value.superfunction()%3B%0D%0A%20%20%20%20%20%20%20%20%7D%0D%0A%20%20%20%20%20%20%20%20else%20%7B%0D%0A%20%20%20%20%20%20%20%20%20%20%20%20return%20%22Error%22%3B%0D%0A%20%20%20%20%20%20%20%20%7D%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Alet%20greeter%20%3D%20new%20Greeter(%22world%22)%3B%0D%0A%0D%0Aconst%20sfunc%20%3D%20new%20MySuperImplementation()%3B%0D%0A%0D%0Aconsole.log(greeter.testSuperFunction(sfunc))%3B
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53448229%2fhow-to-use-unknown-without-instanceof-in-a-meaningful-way%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Javascipt does not check for interface type at runtime.
Typescript: instanceof check on interface
But, you can use unknown type with classes as shown below:
interface MySuperInterface {
superfunction(): string;
}
class MySuperImplementation implements MySuperInterface {
superfunction(): string {
return "Hello from super function";
}
}
class Greeter {
greeting: string;
constructor(message: string) {
this.greeting = message;
}
greet() {
return "Hello, " + this.greeting;
}
testSuperFunction(value: unknown) {
if (value instanceof MySuperImplementation) {
return value.superfunction();
}
else {
return "Error";
}
}
}
let greeter = new Greeter("world");
const sfunc = new MySuperImplementation();
console.log(greeter.testSuperFunction(sfunc));
https://www.typescriptlang.org/play/index.html#src=interface%20MySuperInterface%20%7B%0D%0A%20%20%20%20superfunction()%3A%20string%3B%0D%0A%7D%0D%0A%0D%0Aclass%20MySuperImplementation%20implements%20MySuperInterface%20%7B%0D%0A%20%20%20%20superfunction()%3A%20string%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20%22Hello%20from%20super%20function%22%3B%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Aclass%20Greeter%20%7B%0D%0A%20%20%20%20greeting%3A%20string%3B%0D%0A%0D%0A%20%20%20%20constructor(message%3A%20string)%20%7B%0D%0A%20%20%20%20%20%20%20%20this.greeting%20%3D%20message%3B%0D%0A%20%20%20%20%7D%0D%0A%0D%0A%20%20%20%20greet()%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20%22Hello%2C%20%22%20%2B%20this.greeting%3B%0D%0A%20%20%20%20%7D%0D%0A%0D%0A%20%20%20%20testSuperFunction(value%3A%20unknown)%20%7B%0D%0A%20%20%20%20%20%20%20%20if%20(value%20instanceof%20MySuperImplementation)%20%7B%0D%0A%20%20%20%20%20%20%20%20%20%20%20%20return%20value.superfunction()%3B%0D%0A%20%20%20%20%20%20%20%20%7D%0D%0A%20%20%20%20%20%20%20%20else%20%7B%0D%0A%20%20%20%20%20%20%20%20%20%20%20%20return%20%22Error%22%3B%0D%0A%20%20%20%20%20%20%20%20%7D%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Alet%20greeter%20%3D%20new%20Greeter(%22world%22)%3B%0D%0A%0D%0Aconst%20sfunc%20%3D%20new%20MySuperImplementation()%3B%0D%0A%0D%0Aconsole.log(greeter.testSuperFunction(sfunc))%3B
add a comment |
Javascipt does not check for interface type at runtime.
Typescript: instanceof check on interface
But, you can use unknown type with classes as shown below:
interface MySuperInterface {
superfunction(): string;
}
class MySuperImplementation implements MySuperInterface {
superfunction(): string {
return "Hello from super function";
}
}
class Greeter {
greeting: string;
constructor(message: string) {
this.greeting = message;
}
greet() {
return "Hello, " + this.greeting;
}
testSuperFunction(value: unknown) {
if (value instanceof MySuperImplementation) {
return value.superfunction();
}
else {
return "Error";
}
}
}
let greeter = new Greeter("world");
const sfunc = new MySuperImplementation();
console.log(greeter.testSuperFunction(sfunc));
https://www.typescriptlang.org/play/index.html#src=interface%20MySuperInterface%20%7B%0D%0A%20%20%20%20superfunction()%3A%20string%3B%0D%0A%7D%0D%0A%0D%0Aclass%20MySuperImplementation%20implements%20MySuperInterface%20%7B%0D%0A%20%20%20%20superfunction()%3A%20string%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20%22Hello%20from%20super%20function%22%3B%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Aclass%20Greeter%20%7B%0D%0A%20%20%20%20greeting%3A%20string%3B%0D%0A%0D%0A%20%20%20%20constructor(message%3A%20string)%20%7B%0D%0A%20%20%20%20%20%20%20%20this.greeting%20%3D%20message%3B%0D%0A%20%20%20%20%7D%0D%0A%0D%0A%20%20%20%20greet()%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20%22Hello%2C%20%22%20%2B%20this.greeting%3B%0D%0A%20%20%20%20%7D%0D%0A%0D%0A%20%20%20%20testSuperFunction(value%3A%20unknown)%20%7B%0D%0A%20%20%20%20%20%20%20%20if%20(value%20instanceof%20MySuperImplementation)%20%7B%0D%0A%20%20%20%20%20%20%20%20%20%20%20%20return%20value.superfunction()%3B%0D%0A%20%20%20%20%20%20%20%20%7D%0D%0A%20%20%20%20%20%20%20%20else%20%7B%0D%0A%20%20%20%20%20%20%20%20%20%20%20%20return%20%22Error%22%3B%0D%0A%20%20%20%20%20%20%20%20%7D%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Alet%20greeter%20%3D%20new%20Greeter(%22world%22)%3B%0D%0A%0D%0Aconst%20sfunc%20%3D%20new%20MySuperImplementation()%3B%0D%0A%0D%0Aconsole.log(greeter.testSuperFunction(sfunc))%3B
add a comment |
Javascipt does not check for interface type at runtime.
Typescript: instanceof check on interface
But, you can use unknown type with classes as shown below:
interface MySuperInterface {
superfunction(): string;
}
class MySuperImplementation implements MySuperInterface {
superfunction(): string {
return "Hello from super function";
}
}
class Greeter {
greeting: string;
constructor(message: string) {
this.greeting = message;
}
greet() {
return "Hello, " + this.greeting;
}
testSuperFunction(value: unknown) {
if (value instanceof MySuperImplementation) {
return value.superfunction();
}
else {
return "Error";
}
}
}
let greeter = new Greeter("world");
const sfunc = new MySuperImplementation();
console.log(greeter.testSuperFunction(sfunc));
https://www.typescriptlang.org/play/index.html#src=interface%20MySuperInterface%20%7B%0D%0A%20%20%20%20superfunction()%3A%20string%3B%0D%0A%7D%0D%0A%0D%0Aclass%20MySuperImplementation%20implements%20MySuperInterface%20%7B%0D%0A%20%20%20%20superfunction()%3A%20string%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20%22Hello%20from%20super%20function%22%3B%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Aclass%20Greeter%20%7B%0D%0A%20%20%20%20greeting%3A%20string%3B%0D%0A%0D%0A%20%20%20%20constructor(message%3A%20string)%20%7B%0D%0A%20%20%20%20%20%20%20%20this.greeting%20%3D%20message%3B%0D%0A%20%20%20%20%7D%0D%0A%0D%0A%20%20%20%20greet()%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20%22Hello%2C%20%22%20%2B%20this.greeting%3B%0D%0A%20%20%20%20%7D%0D%0A%0D%0A%20%20%20%20testSuperFunction(value%3A%20unknown)%20%7B%0D%0A%20%20%20%20%20%20%20%20if%20(value%20instanceof%20MySuperImplementation)%20%7B%0D%0A%20%20%20%20%20%20%20%20%20%20%20%20return%20value.superfunction()%3B%0D%0A%20%20%20%20%20%20%20%20%7D%0D%0A%20%20%20%20%20%20%20%20else%20%7B%0D%0A%20%20%20%20%20%20%20%20%20%20%20%20return%20%22Error%22%3B%0D%0A%20%20%20%20%20%20%20%20%7D%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Alet%20greeter%20%3D%20new%20Greeter(%22world%22)%3B%0D%0A%0D%0Aconst%20sfunc%20%3D%20new%20MySuperImplementation()%3B%0D%0A%0D%0Aconsole.log(greeter.testSuperFunction(sfunc))%3B
Javascipt does not check for interface type at runtime.
Typescript: instanceof check on interface
But, you can use unknown type with classes as shown below:
interface MySuperInterface {
superfunction(): string;
}
class MySuperImplementation implements MySuperInterface {
superfunction(): string {
return "Hello from super function";
}
}
class Greeter {
greeting: string;
constructor(message: string) {
this.greeting = message;
}
greet() {
return "Hello, " + this.greeting;
}
testSuperFunction(value: unknown) {
if (value instanceof MySuperImplementation) {
return value.superfunction();
}
else {
return "Error";
}
}
}
let greeter = new Greeter("world");
const sfunc = new MySuperImplementation();
console.log(greeter.testSuperFunction(sfunc));
https://www.typescriptlang.org/play/index.html#src=interface%20MySuperInterface%20%7B%0D%0A%20%20%20%20superfunction()%3A%20string%3B%0D%0A%7D%0D%0A%0D%0Aclass%20MySuperImplementation%20implements%20MySuperInterface%20%7B%0D%0A%20%20%20%20superfunction()%3A%20string%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20%22Hello%20from%20super%20function%22%3B%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Aclass%20Greeter%20%7B%0D%0A%20%20%20%20greeting%3A%20string%3B%0D%0A%0D%0A%20%20%20%20constructor(message%3A%20string)%20%7B%0D%0A%20%20%20%20%20%20%20%20this.greeting%20%3D%20message%3B%0D%0A%20%20%20%20%7D%0D%0A%0D%0A%20%20%20%20greet()%20%7B%0D%0A%20%20%20%20%20%20%20%20return%20%22Hello%2C%20%22%20%2B%20this.greeting%3B%0D%0A%20%20%20%20%7D%0D%0A%0D%0A%20%20%20%20testSuperFunction(value%3A%20unknown)%20%7B%0D%0A%20%20%20%20%20%20%20%20if%20(value%20instanceof%20MySuperImplementation)%20%7B%0D%0A%20%20%20%20%20%20%20%20%20%20%20%20return%20value.superfunction()%3B%0D%0A%20%20%20%20%20%20%20%20%7D%0D%0A%20%20%20%20%20%20%20%20else%20%7B%0D%0A%20%20%20%20%20%20%20%20%20%20%20%20return%20%22Error%22%3B%0D%0A%20%20%20%20%20%20%20%20%7D%0D%0A%20%20%20%20%7D%0D%0A%7D%0D%0A%0D%0Alet%20greeter%20%3D%20new%20Greeter(%22world%22)%3B%0D%0A%0D%0Aconst%20sfunc%20%3D%20new%20MySuperImplementation()%3B%0D%0A%0D%0Aconsole.log(greeter.testSuperFunction(sfunc))%3B
answered Nov 23 '18 at 17:31
SSKSSK
62212
62212
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53448229%2fhow-to-use-unknown-without-instanceof-in-a-meaningful-way%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XhLuxG,LXizuq
1
In conjunction with discriminated unions maybe?
– Fenton
Nov 23 '18 at 14:17
Pick a couple of properties that are significant and use custom type guard to narrow
unknown
to your specific interface type ?– Titian Cernicova-Dragomir
Nov 23 '18 at 14:19