How to aggregate data with condition using two arrays?
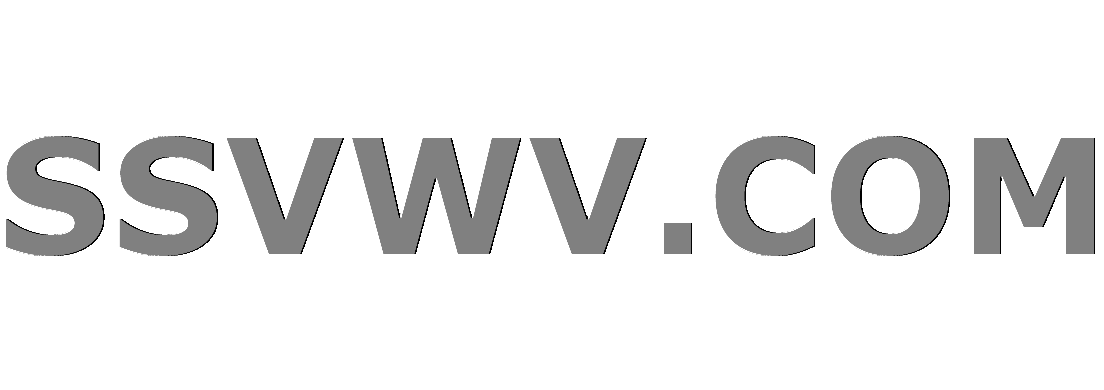
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I have M and W data sets and the structure is as follow:
M = [
'ModuleName': 'GDD',
{Fields: [
{'FieldID': 5, 'Date': '2018-12-01'},
{'FieldID': 6, 'Date': '2018-12-01'}
]
}
]
W = [
{'FieldID': 5, 'Value': 10, 'WDate': 2018-06-05'},
{'FieldID': 5, 'Value': 10, 'WDate': 2018-06-05'},
{'FieldID': 6, 'Value': 50, 'WDate': 2018-07-05'},
{'FieldID': 6, 'Value': 20, 'WDate': 2018-09-05'}
]
I want to calculate value of all fields from W data set and group by FieldID
with date condition from M data set.
M.forEach(m => {
if (m.ModuleName === 'GDD') {
m.Fields.forEach(f => {
const sum = W.map(w => w.Value).reduce((acc, value) => acc + value, 0);
});
}
});
Condition: If Date from M.Fields
is equal or more than date from W.wDate
.
Since the data sets are huge, I also need to consider efficiency.
The expected output is to add another property and store sum of Value in the m.Fields field object.
M = [
'ModuleName': 'GDD',
{Fields: [
{'FieldID': 5, 'Date': '2018-10-01', 'sum': 20},
{'FieldID': 6, 'Date': '2018-10-01', 'sum': 70}
]
}
]
javascript
add a comment |
I have M and W data sets and the structure is as follow:
M = [
'ModuleName': 'GDD',
{Fields: [
{'FieldID': 5, 'Date': '2018-12-01'},
{'FieldID': 6, 'Date': '2018-12-01'}
]
}
]
W = [
{'FieldID': 5, 'Value': 10, 'WDate': 2018-06-05'},
{'FieldID': 5, 'Value': 10, 'WDate': 2018-06-05'},
{'FieldID': 6, 'Value': 50, 'WDate': 2018-07-05'},
{'FieldID': 6, 'Value': 20, 'WDate': 2018-09-05'}
]
I want to calculate value of all fields from W data set and group by FieldID
with date condition from M data set.
M.forEach(m => {
if (m.ModuleName === 'GDD') {
m.Fields.forEach(f => {
const sum = W.map(w => w.Value).reduce((acc, value) => acc + value, 0);
});
}
});
Condition: If Date from M.Fields
is equal or more than date from W.wDate
.
Since the data sets are huge, I also need to consider efficiency.
The expected output is to add another property and store sum of Value in the m.Fields field object.
M = [
'ModuleName': 'GDD',
{Fields: [
{'FieldID': 5, 'Date': '2018-10-01', 'sum': 20},
{'FieldID': 6, 'Date': '2018-10-01', 'sum': 70}
]
}
]
javascript
1
Can you pls also tell the expected output
– Nitish Narang
Nov 23 '18 at 17:07
Can you also please include a little more of your data? You've just included 1 object from bothM
andW
.
– slider
Nov 23 '18 at 17:08
But why do you have 2 similar objects inFields
? Won't they have the same sum?
– slider
Nov 23 '18 at 17:16
add a comment |
I have M and W data sets and the structure is as follow:
M = [
'ModuleName': 'GDD',
{Fields: [
{'FieldID': 5, 'Date': '2018-12-01'},
{'FieldID': 6, 'Date': '2018-12-01'}
]
}
]
W = [
{'FieldID': 5, 'Value': 10, 'WDate': 2018-06-05'},
{'FieldID': 5, 'Value': 10, 'WDate': 2018-06-05'},
{'FieldID': 6, 'Value': 50, 'WDate': 2018-07-05'},
{'FieldID': 6, 'Value': 20, 'WDate': 2018-09-05'}
]
I want to calculate value of all fields from W data set and group by FieldID
with date condition from M data set.
M.forEach(m => {
if (m.ModuleName === 'GDD') {
m.Fields.forEach(f => {
const sum = W.map(w => w.Value).reduce((acc, value) => acc + value, 0);
});
}
});
Condition: If Date from M.Fields
is equal or more than date from W.wDate
.
Since the data sets are huge, I also need to consider efficiency.
The expected output is to add another property and store sum of Value in the m.Fields field object.
M = [
'ModuleName': 'GDD',
{Fields: [
{'FieldID': 5, 'Date': '2018-10-01', 'sum': 20},
{'FieldID': 6, 'Date': '2018-10-01', 'sum': 70}
]
}
]
javascript
I have M and W data sets and the structure is as follow:
M = [
'ModuleName': 'GDD',
{Fields: [
{'FieldID': 5, 'Date': '2018-12-01'},
{'FieldID': 6, 'Date': '2018-12-01'}
]
}
]
W = [
{'FieldID': 5, 'Value': 10, 'WDate': 2018-06-05'},
{'FieldID': 5, 'Value': 10, 'WDate': 2018-06-05'},
{'FieldID': 6, 'Value': 50, 'WDate': 2018-07-05'},
{'FieldID': 6, 'Value': 20, 'WDate': 2018-09-05'}
]
I want to calculate value of all fields from W data set and group by FieldID
with date condition from M data set.
M.forEach(m => {
if (m.ModuleName === 'GDD') {
m.Fields.forEach(f => {
const sum = W.map(w => w.Value).reduce((acc, value) => acc + value, 0);
});
}
});
Condition: If Date from M.Fields
is equal or more than date from W.wDate
.
Since the data sets are huge, I also need to consider efficiency.
The expected output is to add another property and store sum of Value in the m.Fields field object.
M = [
'ModuleName': 'GDD',
{Fields: [
{'FieldID': 5, 'Date': '2018-10-01', 'sum': 20},
{'FieldID': 6, 'Date': '2018-10-01', 'sum': 70}
]
}
]
javascript
javascript
edited Nov 23 '18 at 17:36
Maihan Nijat
asked Nov 23 '18 at 17:06


Maihan NijatMaihan Nijat
2,99822550
2,99822550
1
Can you pls also tell the expected output
– Nitish Narang
Nov 23 '18 at 17:07
Can you also please include a little more of your data? You've just included 1 object from bothM
andW
.
– slider
Nov 23 '18 at 17:08
But why do you have 2 similar objects inFields
? Won't they have the same sum?
– slider
Nov 23 '18 at 17:16
add a comment |
1
Can you pls also tell the expected output
– Nitish Narang
Nov 23 '18 at 17:07
Can you also please include a little more of your data? You've just included 1 object from bothM
andW
.
– slider
Nov 23 '18 at 17:08
But why do you have 2 similar objects inFields
? Won't they have the same sum?
– slider
Nov 23 '18 at 17:16
1
1
Can you pls also tell the expected output
– Nitish Narang
Nov 23 '18 at 17:07
Can you pls also tell the expected output
– Nitish Narang
Nov 23 '18 at 17:07
Can you also please include a little more of your data? You've just included 1 object from both
M
and W
.– slider
Nov 23 '18 at 17:08
Can you also please include a little more of your data? You've just included 1 object from both
M
and W
.– slider
Nov 23 '18 at 17:08
But why do you have 2 similar objects in
Fields
? Won't they have the same sum?– slider
Nov 23 '18 at 17:16
But why do you have 2 similar objects in
Fields
? Won't they have the same sum?– slider
Nov 23 '18 at 17:16
add a comment |
1 Answer
1
active
oldest
votes
For efficiency:
- Convert to an object for quicker accessibility
- Use for loops, not forEach
'use strict';
const M = {
'ModuleName': 'GDD',
Fields: [
{'FieldID': 5, 'Date': '2018-05-01'},
{'FieldID': 6, 'Date': '2018-05-01'}
]
};
const W = [
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 6, 'Value': 50, 'WDate': '2018-07-05'},
{'FieldID': 6, 'Value': 20, 'WDate': '2018-09-05'}
];
let d, e, f = {};
// build object for quicker findability
for (e of M.Fields) {
f[e.FieldID] = e;
e.sum = 0;
}
for (e of W) {
if (d = f[e.FieldID]) {
if (e.WDate >= d.Date) {
d.sum += e.Value;
}
} else {
console.log(`could not find FieldID ${e.FieldID}`)
}
}
console.log(JSON.stringify(M, null, 4));
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450607%2fhow-to-aggregate-data-with-condition-using-two-arrays%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
For efficiency:
- Convert to an object for quicker accessibility
- Use for loops, not forEach
'use strict';
const M = {
'ModuleName': 'GDD',
Fields: [
{'FieldID': 5, 'Date': '2018-05-01'},
{'FieldID': 6, 'Date': '2018-05-01'}
]
};
const W = [
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 6, 'Value': 50, 'WDate': '2018-07-05'},
{'FieldID': 6, 'Value': 20, 'WDate': '2018-09-05'}
];
let d, e, f = {};
// build object for quicker findability
for (e of M.Fields) {
f[e.FieldID] = e;
e.sum = 0;
}
for (e of W) {
if (d = f[e.FieldID]) {
if (e.WDate >= d.Date) {
d.sum += e.Value;
}
} else {
console.log(`could not find FieldID ${e.FieldID}`)
}
}
console.log(JSON.stringify(M, null, 4));
add a comment |
For efficiency:
- Convert to an object for quicker accessibility
- Use for loops, not forEach
'use strict';
const M = {
'ModuleName': 'GDD',
Fields: [
{'FieldID': 5, 'Date': '2018-05-01'},
{'FieldID': 6, 'Date': '2018-05-01'}
]
};
const W = [
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 6, 'Value': 50, 'WDate': '2018-07-05'},
{'FieldID': 6, 'Value': 20, 'WDate': '2018-09-05'}
];
let d, e, f = {};
// build object for quicker findability
for (e of M.Fields) {
f[e.FieldID] = e;
e.sum = 0;
}
for (e of W) {
if (d = f[e.FieldID]) {
if (e.WDate >= d.Date) {
d.sum += e.Value;
}
} else {
console.log(`could not find FieldID ${e.FieldID}`)
}
}
console.log(JSON.stringify(M, null, 4));
add a comment |
For efficiency:
- Convert to an object for quicker accessibility
- Use for loops, not forEach
'use strict';
const M = {
'ModuleName': 'GDD',
Fields: [
{'FieldID': 5, 'Date': '2018-05-01'},
{'FieldID': 6, 'Date': '2018-05-01'}
]
};
const W = [
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 6, 'Value': 50, 'WDate': '2018-07-05'},
{'FieldID': 6, 'Value': 20, 'WDate': '2018-09-05'}
];
let d, e, f = {};
// build object for quicker findability
for (e of M.Fields) {
f[e.FieldID] = e;
e.sum = 0;
}
for (e of W) {
if (d = f[e.FieldID]) {
if (e.WDate >= d.Date) {
d.sum += e.Value;
}
} else {
console.log(`could not find FieldID ${e.FieldID}`)
}
}
console.log(JSON.stringify(M, null, 4));
For efficiency:
- Convert to an object for quicker accessibility
- Use for loops, not forEach
'use strict';
const M = {
'ModuleName': 'GDD',
Fields: [
{'FieldID': 5, 'Date': '2018-05-01'},
{'FieldID': 6, 'Date': '2018-05-01'}
]
};
const W = [
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 6, 'Value': 50, 'WDate': '2018-07-05'},
{'FieldID': 6, 'Value': 20, 'WDate': '2018-09-05'}
];
let d, e, f = {};
// build object for quicker findability
for (e of M.Fields) {
f[e.FieldID] = e;
e.sum = 0;
}
for (e of W) {
if (d = f[e.FieldID]) {
if (e.WDate >= d.Date) {
d.sum += e.Value;
}
} else {
console.log(`could not find FieldID ${e.FieldID}`)
}
}
console.log(JSON.stringify(M, null, 4));
'use strict';
const M = {
'ModuleName': 'GDD',
Fields: [
{'FieldID': 5, 'Date': '2018-05-01'},
{'FieldID': 6, 'Date': '2018-05-01'}
]
};
const W = [
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 6, 'Value': 50, 'WDate': '2018-07-05'},
{'FieldID': 6, 'Value': 20, 'WDate': '2018-09-05'}
];
let d, e, f = {};
// build object for quicker findability
for (e of M.Fields) {
f[e.FieldID] = e;
e.sum = 0;
}
for (e of W) {
if (d = f[e.FieldID]) {
if (e.WDate >= d.Date) {
d.sum += e.Value;
}
} else {
console.log(`could not find FieldID ${e.FieldID}`)
}
}
console.log(JSON.stringify(M, null, 4));
'use strict';
const M = {
'ModuleName': 'GDD',
Fields: [
{'FieldID': 5, 'Date': '2018-05-01'},
{'FieldID': 6, 'Date': '2018-05-01'}
]
};
const W = [
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 5, 'Value': 10, 'WDate': '2018-06-05'},
{'FieldID': 6, 'Value': 50, 'WDate': '2018-07-05'},
{'FieldID': 6, 'Value': 20, 'WDate': '2018-09-05'}
];
let d, e, f = {};
// build object for quicker findability
for (e of M.Fields) {
f[e.FieldID] = e;
e.sum = 0;
}
for (e of W) {
if (d = f[e.FieldID]) {
if (e.WDate >= d.Date) {
d.sum += e.Value;
}
} else {
console.log(`could not find FieldID ${e.FieldID}`)
}
}
console.log(JSON.stringify(M, null, 4));
answered Nov 23 '18 at 17:47
niryniry
1,4791222
1,4791222
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450607%2fhow-to-aggregate-data-with-condition-using-two-arrays%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
quUohcaVKx6vekW0GZkK 9X2Z10LrFRmjX KoKI,M DFuaiHRn3rQ2WencEXis2484KhuiiMKZQYlQOzrUfyAi3trCEN
1
Can you pls also tell the expected output
– Nitish Narang
Nov 23 '18 at 17:07
Can you also please include a little more of your data? You've just included 1 object from both
M
andW
.– slider
Nov 23 '18 at 17:08
But why do you have 2 similar objects in
Fields
? Won't they have the same sum?– slider
Nov 23 '18 at 17:16