@ColumnResult maps to Character instead of String in JPA 2.0
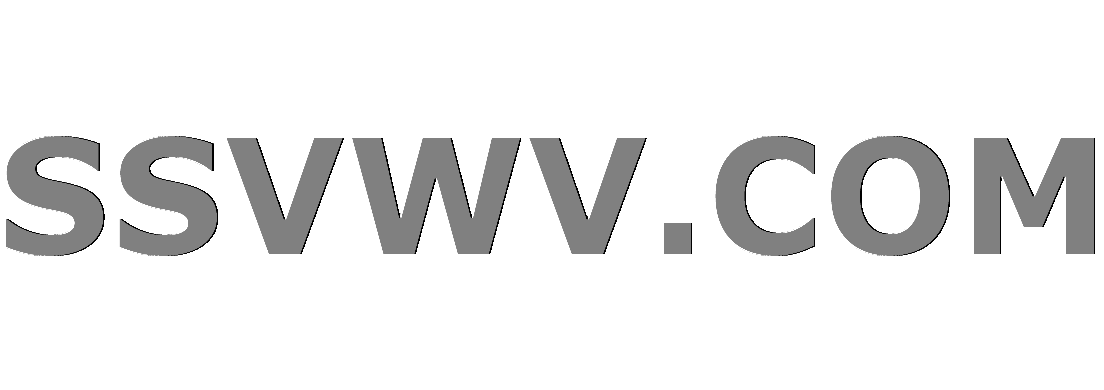
Multi tool use
In our software, we had a requirement that display name of one UI will be configurable according to user. So one user want to display another column from one table and another user want to display another column from same table. I think I can do this using SqlResultSetMappings and automatic name query. display_name column is not in database . I think that I can map column name(according to user selection) to displayName. The problem, Jpa 2.0 does not have type in Columnresult annotation. So display name returns Character. This is my entity definition. In this eaxample user want to display short_name in the UIs. But another user can want to show name in the UIs.
@SqlResultSetMappings({
@SqlResultSetMapping(name = "findAllByTypeList", entities = @EntityResult(entityClass = Element.class, fields = {
@FieldResult(name = "id", column = "ID"), @FieldResult(name = "variant", column = "VARIANT_ID"),
@FieldResult(name = "elementType", column = "ELEMENT_TYPE_ID"),
@FieldResult(name = "name", column = "NAME"),
@FieldResult(name = "shortName", column = "SHORT_NAME"),
@FieldResult(name = "displayName", column = "display_name")
}), columns = {
@ColumnResult(name = "display_name")
})
})
public class Element implements Serializable {
/**
*
*/
private static final long serialVersionUID = 2892631423222044026L;
public Element () {
super();
}
@Id
@Column(name = "ID")
private long id;
@ManyToOne
@JoinColumn(name = "VARIANT_ID")
private Variant variant;
@Column(name = "ELEMENT_TYPE_ID")
private int elementType;
@Column(name = "NAME")
private String name;
@Column(name = "SHORT_NAME")
private String shortName;
@Transient
private String displayName;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public Variant getVariant() {
return variant;
}
public void setVariant(Variant variant) {
this.variant = variant;
}
public ElementTypeConstants getElementType() {
return ElementTypeConstants .getInstance(elementType);
}
public void setElementType(NetworkElementTypeConstants elementType) {
this.elementType = elementType.getId();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getShortName() {
return shortName;
}
public void setShortName(String shortName) {
this.shortName = shortName;
}
public boolean equals(Object obj) {
if (obj == null || !(obj instanceof Element)) {
return false;
}
if (obj == this) {
return true;
}
return ((Element) obj).getName().equals(this.getName());
}
public String toString() {
StringBuffer buf = new StringBuffer("NE ID:");
buf.append(getId());
buf.append(", Name:");
buf.append(getName());
buf.append(", ShortName:");
buf.append(getShortName());
return buf.toString();
}
@Override
public int hashCode() {
return Long.valueOf(id).intValue();
}
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
This is my native query
/** adding table name */
sqlQuery = "select a.id, a.variant_id, a.ELEMENT_TYPE_ID, a.name, a.short_name, a.short_name as display_name from " + conf_element a;
Query query = em.createNativeQuery(sqlQuery
+ " where a.variant_id=:variantId and a.element_type_id in (:elementTypeIdList) order by a.name",
"findAllByTypeList");
query.setParameter("variantId", variantId);
query.setParameter("elementTypeIdList", elementTypeList);
return query.getResultList();
}
PS.: In the beginning, I think that I can implement without using ColumnResult.Following line can do the job.
@FieldResult(name = "displayName", column = "display_name")
java jpa nativequery sqlresultsetmapping
add a comment |
In our software, we had a requirement that display name of one UI will be configurable according to user. So one user want to display another column from one table and another user want to display another column from same table. I think I can do this using SqlResultSetMappings and automatic name query. display_name column is not in database . I think that I can map column name(according to user selection) to displayName. The problem, Jpa 2.0 does not have type in Columnresult annotation. So display name returns Character. This is my entity definition. In this eaxample user want to display short_name in the UIs. But another user can want to show name in the UIs.
@SqlResultSetMappings({
@SqlResultSetMapping(name = "findAllByTypeList", entities = @EntityResult(entityClass = Element.class, fields = {
@FieldResult(name = "id", column = "ID"), @FieldResult(name = "variant", column = "VARIANT_ID"),
@FieldResult(name = "elementType", column = "ELEMENT_TYPE_ID"),
@FieldResult(name = "name", column = "NAME"),
@FieldResult(name = "shortName", column = "SHORT_NAME"),
@FieldResult(name = "displayName", column = "display_name")
}), columns = {
@ColumnResult(name = "display_name")
})
})
public class Element implements Serializable {
/**
*
*/
private static final long serialVersionUID = 2892631423222044026L;
public Element () {
super();
}
@Id
@Column(name = "ID")
private long id;
@ManyToOne
@JoinColumn(name = "VARIANT_ID")
private Variant variant;
@Column(name = "ELEMENT_TYPE_ID")
private int elementType;
@Column(name = "NAME")
private String name;
@Column(name = "SHORT_NAME")
private String shortName;
@Transient
private String displayName;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public Variant getVariant() {
return variant;
}
public void setVariant(Variant variant) {
this.variant = variant;
}
public ElementTypeConstants getElementType() {
return ElementTypeConstants .getInstance(elementType);
}
public void setElementType(NetworkElementTypeConstants elementType) {
this.elementType = elementType.getId();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getShortName() {
return shortName;
}
public void setShortName(String shortName) {
this.shortName = shortName;
}
public boolean equals(Object obj) {
if (obj == null || !(obj instanceof Element)) {
return false;
}
if (obj == this) {
return true;
}
return ((Element) obj).getName().equals(this.getName());
}
public String toString() {
StringBuffer buf = new StringBuffer("NE ID:");
buf.append(getId());
buf.append(", Name:");
buf.append(getName());
buf.append(", ShortName:");
buf.append(getShortName());
return buf.toString();
}
@Override
public int hashCode() {
return Long.valueOf(id).intValue();
}
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
This is my native query
/** adding table name */
sqlQuery = "select a.id, a.variant_id, a.ELEMENT_TYPE_ID, a.name, a.short_name, a.short_name as display_name from " + conf_element a;
Query query = em.createNativeQuery(sqlQuery
+ " where a.variant_id=:variantId and a.element_type_id in (:elementTypeIdList) order by a.name",
"findAllByTypeList");
query.setParameter("variantId", variantId);
query.setParameter("elementTypeIdList", elementTypeList);
return query.getResultList();
}
PS.: In the beginning, I think that I can implement without using ColumnResult.Following line can do the job.
@FieldResult(name = "displayName", column = "display_name")
java jpa nativequery sqlresultsetmapping
Why don't you implement the requirement in the code?
– Simon Martinelli
Nov 20 at 9:53
it is not a basic if else statament. Sometimes it can join from other tables. So I create automatic sql statrement
– user725455
Nov 20 at 10:02
add a comment |
In our software, we had a requirement that display name of one UI will be configurable according to user. So one user want to display another column from one table and another user want to display another column from same table. I think I can do this using SqlResultSetMappings and automatic name query. display_name column is not in database . I think that I can map column name(according to user selection) to displayName. The problem, Jpa 2.0 does not have type in Columnresult annotation. So display name returns Character. This is my entity definition. In this eaxample user want to display short_name in the UIs. But another user can want to show name in the UIs.
@SqlResultSetMappings({
@SqlResultSetMapping(name = "findAllByTypeList", entities = @EntityResult(entityClass = Element.class, fields = {
@FieldResult(name = "id", column = "ID"), @FieldResult(name = "variant", column = "VARIANT_ID"),
@FieldResult(name = "elementType", column = "ELEMENT_TYPE_ID"),
@FieldResult(name = "name", column = "NAME"),
@FieldResult(name = "shortName", column = "SHORT_NAME"),
@FieldResult(name = "displayName", column = "display_name")
}), columns = {
@ColumnResult(name = "display_name")
})
})
public class Element implements Serializable {
/**
*
*/
private static final long serialVersionUID = 2892631423222044026L;
public Element () {
super();
}
@Id
@Column(name = "ID")
private long id;
@ManyToOne
@JoinColumn(name = "VARIANT_ID")
private Variant variant;
@Column(name = "ELEMENT_TYPE_ID")
private int elementType;
@Column(name = "NAME")
private String name;
@Column(name = "SHORT_NAME")
private String shortName;
@Transient
private String displayName;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public Variant getVariant() {
return variant;
}
public void setVariant(Variant variant) {
this.variant = variant;
}
public ElementTypeConstants getElementType() {
return ElementTypeConstants .getInstance(elementType);
}
public void setElementType(NetworkElementTypeConstants elementType) {
this.elementType = elementType.getId();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getShortName() {
return shortName;
}
public void setShortName(String shortName) {
this.shortName = shortName;
}
public boolean equals(Object obj) {
if (obj == null || !(obj instanceof Element)) {
return false;
}
if (obj == this) {
return true;
}
return ((Element) obj).getName().equals(this.getName());
}
public String toString() {
StringBuffer buf = new StringBuffer("NE ID:");
buf.append(getId());
buf.append(", Name:");
buf.append(getName());
buf.append(", ShortName:");
buf.append(getShortName());
return buf.toString();
}
@Override
public int hashCode() {
return Long.valueOf(id).intValue();
}
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
This is my native query
/** adding table name */
sqlQuery = "select a.id, a.variant_id, a.ELEMENT_TYPE_ID, a.name, a.short_name, a.short_name as display_name from " + conf_element a;
Query query = em.createNativeQuery(sqlQuery
+ " where a.variant_id=:variantId and a.element_type_id in (:elementTypeIdList) order by a.name",
"findAllByTypeList");
query.setParameter("variantId", variantId);
query.setParameter("elementTypeIdList", elementTypeList);
return query.getResultList();
}
PS.: In the beginning, I think that I can implement without using ColumnResult.Following line can do the job.
@FieldResult(name = "displayName", column = "display_name")
java jpa nativequery sqlresultsetmapping
In our software, we had a requirement that display name of one UI will be configurable according to user. So one user want to display another column from one table and another user want to display another column from same table. I think I can do this using SqlResultSetMappings and automatic name query. display_name column is not in database . I think that I can map column name(according to user selection) to displayName. The problem, Jpa 2.0 does not have type in Columnresult annotation. So display name returns Character. This is my entity definition. In this eaxample user want to display short_name in the UIs. But another user can want to show name in the UIs.
@SqlResultSetMappings({
@SqlResultSetMapping(name = "findAllByTypeList", entities = @EntityResult(entityClass = Element.class, fields = {
@FieldResult(name = "id", column = "ID"), @FieldResult(name = "variant", column = "VARIANT_ID"),
@FieldResult(name = "elementType", column = "ELEMENT_TYPE_ID"),
@FieldResult(name = "name", column = "NAME"),
@FieldResult(name = "shortName", column = "SHORT_NAME"),
@FieldResult(name = "displayName", column = "display_name")
}), columns = {
@ColumnResult(name = "display_name")
})
})
public class Element implements Serializable {
/**
*
*/
private static final long serialVersionUID = 2892631423222044026L;
public Element () {
super();
}
@Id
@Column(name = "ID")
private long id;
@ManyToOne
@JoinColumn(name = "VARIANT_ID")
private Variant variant;
@Column(name = "ELEMENT_TYPE_ID")
private int elementType;
@Column(name = "NAME")
private String name;
@Column(name = "SHORT_NAME")
private String shortName;
@Transient
private String displayName;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public Variant getVariant() {
return variant;
}
public void setVariant(Variant variant) {
this.variant = variant;
}
public ElementTypeConstants getElementType() {
return ElementTypeConstants .getInstance(elementType);
}
public void setElementType(NetworkElementTypeConstants elementType) {
this.elementType = elementType.getId();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getShortName() {
return shortName;
}
public void setShortName(String shortName) {
this.shortName = shortName;
}
public boolean equals(Object obj) {
if (obj == null || !(obj instanceof Element)) {
return false;
}
if (obj == this) {
return true;
}
return ((Element) obj).getName().equals(this.getName());
}
public String toString() {
StringBuffer buf = new StringBuffer("NE ID:");
buf.append(getId());
buf.append(", Name:");
buf.append(getName());
buf.append(", ShortName:");
buf.append(getShortName());
return buf.toString();
}
@Override
public int hashCode() {
return Long.valueOf(id).intValue();
}
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
This is my native query
/** adding table name */
sqlQuery = "select a.id, a.variant_id, a.ELEMENT_TYPE_ID, a.name, a.short_name, a.short_name as display_name from " + conf_element a;
Query query = em.createNativeQuery(sqlQuery
+ " where a.variant_id=:variantId and a.element_type_id in (:elementTypeIdList) order by a.name",
"findAllByTypeList");
query.setParameter("variantId", variantId);
query.setParameter("elementTypeIdList", elementTypeList);
return query.getResultList();
}
PS.: In the beginning, I think that I can implement without using ColumnResult.Following line can do the job.
@FieldResult(name = "displayName", column = "display_name")
java jpa nativequery sqlresultsetmapping
java jpa nativequery sqlresultsetmapping
asked Nov 20 at 8:07
user725455
140424
140424
Why don't you implement the requirement in the code?
– Simon Martinelli
Nov 20 at 9:53
it is not a basic if else statament. Sometimes it can join from other tables. So I create automatic sql statrement
– user725455
Nov 20 at 10:02
add a comment |
Why don't you implement the requirement in the code?
– Simon Martinelli
Nov 20 at 9:53
it is not a basic if else statament. Sometimes it can join from other tables. So I create automatic sql statrement
– user725455
Nov 20 at 10:02
Why don't you implement the requirement in the code?
– Simon Martinelli
Nov 20 at 9:53
Why don't you implement the requirement in the code?
– Simon Martinelli
Nov 20 at 9:53
it is not a basic if else statament. Sometimes it can join from other tables. So I create automatic sql statrement
– user725455
Nov 20 at 10:02
it is not a basic if else statament. Sometimes it can join from other tables. So I create automatic sql statrement
– user725455
Nov 20 at 10:02
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53388650%2fcolumnresult-maps-to-character-instead-of-string-in-jpa-2-0%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53388650%2fcolumnresult-maps-to-character-instead-of-string-in-jpa-2-0%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6EZq4GkBhJxY54 W,vEf,FX6u4t2OQsaqnA,ZU VloAS3osAQ555fpR,Bit0odmgsUHCRvO,eBQjH4FTAgQLvC8nbns
Why don't you implement the requirement in the code?
– Simon Martinelli
Nov 20 at 9:53
it is not a basic if else statament. Sometimes it can join from other tables. So I create automatic sql statrement
– user725455
Nov 20 at 10:02